### VHDL实现的可配置乘法器设计 #### 概述 本文将详细介绍一个使用VHDL语言编写的乘法器的设计与实现过程。该乘法器的特点在于可以通过设置参数来控制输入数据的位宽,这使得它具有高度的灵活性和可配置性,能够满足不同应用场景的需求。 #### VHDL代码解析 ### 1. 库导入 ```vhdl library ieee; use ieee.std_logic_1164.all; ``` 这部分代码指定了要使用的库以及需要调用的包。`ieee`是标准的VHDL库之一,而`std_logic_1164`则定义了标准逻辑数据类型和信号属性,是实现数字电路的基础。 ### 2. 实体声明 ```vhdl entity multi8 is generic ( datawidth : integer := 8; -- 输入数据位宽 outwidth : integer := 16 -- 输出数据位宽 ); port ( a, b : in std_logic_vector(datawidth - 1 downto 0); -- 输入端口 y : out std_logic_vector(outwidth - 1 downto 0) -- 输出端口 ); end entity multi8; ``` 实体声明部分定义了一个名为`multi8`的实体,该实体具有两个可配置的参数:`datawidth`和`outwidth`,分别表示输入数据的位宽和输出数据的位宽,默认值分别为8和16。实体的端口包括两个输入端口`a`和`b`,以及一个输出端口`y`。 ### 3. 结构体行为声明 ```vhdl architecture behavioral of multi8 is begin -- 乘法器的核心逻辑在此处实现 end architecture behavioral; ``` 结构体声明部分定义了实体的行为逻辑,这里使用的是`behavioral`风格的架构。具体的行为逻辑在接下来的部分进行描述。 ### 4. 乘法器核心逻辑 ```vhdl behavior: process (a, b) is variable a_in : std_logic_vector(datawidth - 1 downto 0); variable b_in : std_logic_vector(datawidth - 1 downto 0); variable y_out : std_logic_vector(outwidth - 1 downto 0); variable carry_in, carry : std_logic; begin a_in := a; b_in := b; y_out := (others => '0'); for count in 0 to datawidth - 1 loop carry := '0'; if (b_in(count) = '1') then for index in 0 to datawidth - 1 loop carry_in := carry; carry := (y_out(index + count) and a_in(index)) or (carry_in and (y_out(index + count) xor a_in(index))); y_out(index + count) := y_out(index + count) xor a_in(index) xor carry_in; end loop; y_out(count + datawidth) := carry; end if; end loop; y <= y_out; end process behavior; ``` 这段代码实现了乘法器的核心算法。通过两个内部变量`a_in`和`b_in`分别存储输入端口的数据,然后初始化输出结果`y_out`为全0向量。接下来通过两个嵌套循环实现了二进制乘法运算:外层循环遍历乘数`b`中的每一位,如果该位为1,则执行内层循环,即逐位对被乘数`a`与当前输出结果`y_out`进行加法运算,并计算进位。将计算结果赋值给输出端口`y`。 ### 总结 本篇介绍了一种基于VHDL的乘法器设计方法,该设计具有良好的灵活性和扩展性,用户可以通过调整实体中的通用参数来指定输入数据的位宽和输出数据的位宽。此外,该设计采用的乘法算法简单高效,适用于多种应用场景。对于想要深入了解VHDL语言或数字电路设计的人来说,这是一个很好的学习案例。
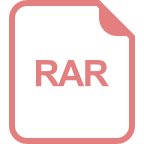
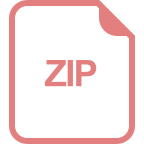
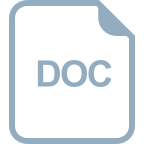
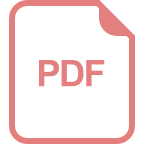
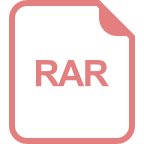
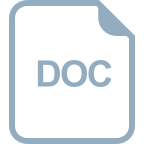
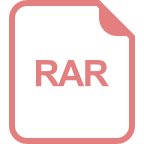
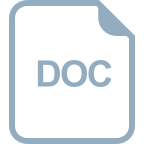
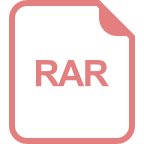
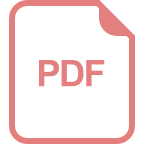
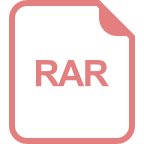
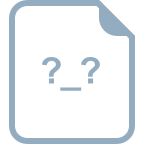
use ieee.std_logic_1164.all;
entity multi8 is
generic(datawidth : integer :=8;
outwidth : integer :=16);
port(a,b:in std_logic_vector(datawidth-1 downto 0);
y:out std_logic_vector(outwidth-1 downto 0));
end entity multi8;
architecture behavioral of multi8 is
begin
behavior:process(a,b) is
variable a_in:std_logic_vector(datawidth-1 downto 0);
variable b_in:std_logic_vector(datawidth-1 downto 0);
variable y_out:std_logic_vector(outwidth-1 downto 0);
variable carry_in,carry:std_logic;
begin
a_in:=a;
b_in:=b;
y_out:=(others=>'0');
for count in 0 to datawidth-1 loop
carry:='0';
if(b_in(count)='1') then
for index in 0 to datawidth-1 loop
carry_in:=carry;
carry:=(y_out(index+count) and a_in(index))
or (carry_in and (y_out(index+count) xor a_in(index)));
y_out(index+count):=y_out(index+count) xor a_in(index) xor carry_in;
end loop;
y_out(count+datawidth):=carry;
end if;
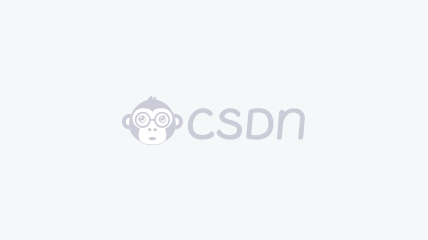

- 粉丝: 0
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

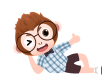
最新资源

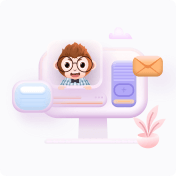
