function [thresh MSE PSNR EI] = bft(OI,A,B,C,level,n,type,thresh)
% This function bft implements Brute Force Thresholding Algorithm as
% specified in the paper entitled as Despeckling of SAR Image Using
% Adaptive and Mean Filters, by Syed Musharaf Ali, Muhammad Younus Javed,
% and Naveed Sarfraz Khattak
%
% INPUT:
% OI = Original Image
% A = Nondecimated 2-D wavelet transform of OI
% B = Nondecimated 2-D wavelet transform of Filtered OI
% C = Nondecimated 2-D wavelet transform of Filtered OI
% level = Decomposition level
% n = Number of spacing between minimum and maximum threshold
% (Larger n results time consuming)
% OUTPUT:
% thresh = matrix of dimenshion (3*level,n)containing threshold
% value
% MSE = Mean Square Error
% PSNR = Peak Singal to Noise Ratio
%
%
% Implemented by ASHISH MESHRAM
% meetashish85@gmail.com http://www.facebook.com/ashishmeet
% Checking Input Arguments
if nargout==4 && nargin<8
error('Not enough input arguments');
end
if nargin<7||isempty(type)
type = 'try';
end
if nargin<6,error('Not enough input arguments');end
if nargin<5,error('Not enough input arguments');end
if nargin<4,error('Not enough input arguments');end
if nargin<3,error('Not enough input arguments');end
if nargin<2,error('Not enough input arguments');end
if nargin<1,error('Not enough input arguments');end
% Implementation Starts Here
switch lower(type)
case 'try' % Case for finding Best Threshold
if level == 1 % First level decomposition
ALH1 = A.dec{2,1};BLH1 = B.dec{2,1};CLH1 = C.dec{2,1}; % Vertical Detail
AHL1 = A.dec{3,1};BHL1 = B.dec{3,1};CHL1 = C.dec{3,1}; % Horizonatal Detail
AHH1 = A.dec{4,1};BHH1 = B.dec{4,1};CHH1 = C.dec{4,1}; % Diagonal Detail
% Find minimum and maximum value of coefficients of each detail sub band
Aminmax = [min(min(ALH1)) max(max(ALH1));...
min(min(AHL1)) max(max(AHL1));...
min(min(AHH1)) max(max(AHH1))];
% Preallocating
thresh = zeros(3,n);
MSE = zeros(3,n);
PSNR = zeros(3,n);
% Vertical Detail
coeff = linspace(Aminmax(1,1),Aminmax(1,2),n);
for k = 1:length(coeff)
% Replacing Low Level pixel of Image A with Image B
disp('Replacing Low Level pixel of Image A with Image B');
ALH1(ALH1<coeff(k)) = CLH1(ALH1<coeff(k));
% Applying Direction Dependent Mask
disp('Applying Direction Dependent Mask');
ALH1 = ddm(ALH1,BLH1,coeff(k),'LH');
% Applying Direction Smoothing Filter
disp('Applying Direction Smoothing Filter');
ALH1 = dsf(ALH1,3);
% Replacing decomposition coefficients with new coefficients
disp('Replacing decomposition coefficients with new coefficients');
A.dec{2,1} = ALH1;
A.dec{3,1} = AHL1;
A.dec{4,1} = AHH1;
% Inverse nondecimated 2-D wavelet transform
disp('Inverse nondecimated 2-D wavelet transform');
EI = indwt2(A,'aa',1);
% Computing Results
disp('Computing Results');
[MSE(1,k) PSNR(1,k)] = MetricsMeasurement(OI,EI);
thresh(1,k) = coeff(k);
end
% Horizontal Detail
coeff = linspace(Aminmax(2,1),Aminmax(2,2),n);
for k = 1:length(coeff)
% Replacing Low Level pixel of Image A with Image B
disp('Replacing Low Level pixel of Image A with Image B');
AHL1(AHL1<coeff(k)) = CHL1(AHL1<coeff(k));
% Applying Direction Dependent Mask
disp('Applying Direction Dependent Mask');
AHL1 = ddm(AHL1,BHL1,coeff(k),'HL');
% Applying Direction Smoothing Filter
disp('Applying Direction Smoothing Filter');
AHL1 = dsf(AHL1,3);
% Replacing decomposition coefficients with new coefficients
disp('Replacing decomposition coefficients with new coefficients');
A.dec{2,1} = ALH1;
A.dec{3,1} = AHL1;
A.dec{4,1} = AHH1;
% Inverse nondecimated 2-D wavelet transform
disp('Inverse nondecimated 2-D wavelet transform');
EI = indwt2(A,'aa',1);
% Computing Results
disp('Computing Results');
[MSE(2,k) PSNR(2,k)] = MetricsMeasurement(OI,EI);
thresh(2,k) = coeff(k);
end
% Diagonal Detail
coeff = linspace(Aminmax(3,1),Aminmax(3,2),n);
for k = 1:length(coeff)
% Replacing Low Level pixel of Image A with Image B
disp('Replacing Low Level pixel of Image A with Image B');
AHH1(AHH1<coeff(k)) = CHH1(AHH1<coeff(k));
% Applying Direction Dependent Mask
disp('Applying Direction Dependent Mask');
AHH1 = ddm(AHH1,BHH1,coeff(k),'HH');
% Applying Direction Smoothing Filter
disp('Applying Direction Smoothing Filter');
AHH1 = dsf(AHH1,3);
% Replacing decomposition coefficients with new coefficients
disp('Replacing decomposition coefficients with new coefficients');
A.dec{2,1} = ALH1;
A.dec{3,1} = AHL1;
A.dec{4,1} = AHH1;
% Inverse nondecimated 2-D wavelet transform
disp('Inverse nondecimated 2-D wavelet transform');
EI = indwt2(A,'aa',1);
% Computing Results
disp('Computing Results');
[MSE(3,k) PSNR(3,k)] = MetricsMeasurement(OI,EI);
thresh(3,k) = coeff(k);
end
elseif level == 2 % Second level decomposition
ALH2 = A.dec{2,1};BLH2 = B.dec{2,1};CLH2 = C.dec{2,1}; % Vertical Detail
AHL2 = A.dec{3,1};BHL2 = B.dec{3,1};CHL2 = C.dec{3,1}; % Horizonatal Detail
AHH2 = A.dec{4,1};BHH2 = B.dec{4,1};CHH2 = C.dec{4,1}; % Diagonal Detail
ALH1 = A.dec{5,1};BLH1 = B.dec{5,1};CLH1 = C.dec{5,1}; % Vertical Detail
AHL1 = A.dec{6,1};BHL1 = B.dec{6,1};CHL1 = C.dec{6,1}; % Horizonatal Detail
AHH1 = A.dec{7,1};BHH1 = B.dec{7,1};CHH1 = C.dec{7,1}; % Diagonal Detail
% Find minimum and maximum value of coefficients of each detail sub band
Aminmax = [min(min(ALH2)) max(max(ALH2));...
min(min(AHL2)) max(max(AHL2));...
min(min(AHH2)) max(max(AHH2));...
min(min(ALH1)) max(max(ALH1));...
min(min(AHL1)) max(max(AHL1));...
min(min(AHH1)) max(max(AHH1))];
% Preallocating
thresh = zeros(6,n);
MSE = zeros(6,n);
PSNR = zeros(6,n);
% Vertical Detail
coeff = linspace(Aminmax(1,1),Aminmax(1,2),n);
for k = 1:length(coeff)
% Replacing Low Level pixel of Image A with Image B
disp('Replacing Low Level pixel of Image A with Image B');
ALH2(ALH2<coeff(k)) = CLH2(ALH2<coeff(k));
% Applying Direction Dependent Mask
disp('Applying Direction Dependent Mask');
ALH2 = ddm(ALH2,BLH2,coeff(k),'LH');
% Applying Direction Smoothing Filter
disp('Applying Direction Smoothing Filter');


天天Matlab科研工作室
- 粉丝: 4w+
- 资源: 1万+
最新资源
- 基于RBAC模型的权限控制的一整套基础开发平台,前后端分离,后端采用 django+django-rest-framework,前端采用 vue+ElementUI
- 扰动观测器(时域)-Matlab/Simulink开发
- 【java毕业设计】小学生身体素质测评管理系统设计与实现源码(springboot+vue+mysql+说明文档+LW).zip
- 计算机组成原理中操作系统(慕课版)部分课后习题
- 嵌入式:小熊派实验(包含7个实验)+源码+文档说明(高分作品)
- 【java毕业设计】失物招领平台的设计与实现源码(springboot+vue+mysql+说明文档+LW).zip
- 昆泰3D霍尔KTH57xx手表旋钮应用手册.pdf
- 【java毕业设计】电商应用系统的设计与实现源码(springboot+vue+mysql+说明文档+LW).zip
- 利用Matlab/Simulink实现一个扰动观测器(频域)
- 应用信息AppInfo1.9.0
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


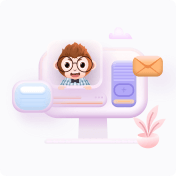