from tensorflow.keras.layers import Conv2D, Input, MaxPool2D, Flatten, Dense, Permute
from tensorflow.keras.layers import PReLU
from tensorflow.keras.models import Model
import numpy as np
import cv2
# -----------------------------#
# 计算原始输入图像
# 每一次缩放的比例
# -----------------------------#
def calculateScales(img):
copy_img = img.copy()
pr_scale = 1.0
# 获取原始图像宽高
h, w, _ = copy_img.shape
# 计算调整后的图像宽高
if min(w, h) > 500:
pr_scale = 500.0 / min(h, w)
w = int(w * pr_scale)
h = int(h * pr_scale)
elif max(w, h) < 500:
pr_scale = 500.0 / max(h, w)
w = int(w * pr_scale)
h = int(h * pr_scale)
scales = []
factor = 0.709
factor_count = 0
minl = min(h, w)
# 计算缩放比例
while minl >= 12:
scales.append(pr_scale * pow(factor, factor_count))
minl *= factor
factor_count += 1
return scales
# -------------------------------------#
# 对pnet处理后的结果进行处理
# -------------------------------------#
def detect_face_12net(cls_prob, roi, out_side, scale, width, height, threshold):
cls_prob = np.swapaxes(cls_prob, 0, 1) # 获取预测人脸置信度得分
roi = np.swapaxes(roi, 0, 2)
stride = 0
# stride略等于2
if out_side != 1:
stride = float(2 * out_side - 1) / (out_side - 1)
# 获取置信度得分大于阈值的人脸框位置
(x, y) = np.where(cls_prob >= threshold)
boundingbox = np.array([x, y]).T
# 找到对应原图的位置
bb1 = np.fix((stride * (boundingbox) + 0) * scale)
bb2 = np.fix((stride * (boundingbox) + 11) * scale)
boundingbox = np.concatenate((bb1, bb2), axis=1)
# 获取人脸框位置调整量
dx1 = roi[0][x, y]
dx2 = roi[1][x, y]
dx3 = roi[2][x, y]
dx4 = roi[3][x, y]
# 将置信度得分和人脸框位置调整量转成ndarray
score = np.array([cls_prob[x, y]]).T
offset = np.array([dx1, dx2, dx3, dx4]).T
# 获取人脸框在原始图像中位置
boundingbox = boundingbox + offset * 12.0 * scale
rectangles = np.concatenate((boundingbox, score), axis=1)
# 转化成正方形
rectangles = rect2square(rectangles)
pick = []
# 遍历人脸框,防止人脸框越界
for i in range(len(rectangles)):
x1 = int(max(0, rectangles[i][0]))
y1 = int(max(0, rectangles[i][1]))
x2 = int(min(width, rectangles[i][2]))
y2 = int(min(height, rectangles[i][3]))
sc = rectangles[i][4]
if x2 > x1 and y2 > y1:
pick.append([x1, y1, x2, y2, sc])
# 对人脸框做非极大值抑制处理
return NMS(pick, 0.3)
# -----------------------------#
# 将长方形调整为正方形
# -----------------------------#
def rect2square(rectangles):
# 计算人脸框宽和高
# print(rectangles.shape)
w = rectangles[:, 2] - rectangles[:, 0]
h = rectangles[:, 3] - rectangles[:, 1]
# 计算宽高中的最大值
l = np.maximum(w, h).T
# 调整人脸框为正方形
rectangles[:, 0] = rectangles[:, 0] + w * 0.5 - l * 0.5
rectangles[:, 1] = rectangles[:, 1] + h * 0.5 - l * 0.5
rectangles[:, 2:4] = rectangles[:, 0:2] + np.repeat([l], 2, axis=0).T
return rectangles
# -------------------------------------#
# 非极大抑制
# -------------------------------------#
def NMS(rectangles, threshold):
# 如果无框则返回
if len(rectangles) == 0:
return rectangles
# 将人脸框转ndarray
boxes = np.array(rectangles)
# 获取人脸框坐标
x1 = boxes[:, 0]
y1 = boxes[:, 1]
x2 = boxes[:, 2]
y2 = boxes[:, 3]
# 获取人脸框置信度
s = boxes[:, 4]
# 计算人脸框面积
area = np.multiply(x2 - x1 + 1, y2 - y1 + 1)
# 对人脸框置信度得分排序
I = np.array(s.argsort())
pick = []
while len(I) > 0:
# 计算置信度得分最高的人脸框x1坐标与其他框的x1坐标最大值
xx1 = np.maximum(x1[I[-1]], x1[I[0:-1]])
# 计算置信度得分最高的人脸框y1坐标与其他框的y1坐标最大值
yy1 = np.maximum(y1[I[-1]], y1[I[0:-1]])
# 计算置信度得分最高的人脸框x2坐标与其他框的x2坐标最小值
xx2 = np.minimum(x2[I[-1]], x2[I[0:-1]])
# 计算置信度得分最高的人脸框y2坐标与其他框的y2坐标最小值
yy2 = np.minimum(y2[I[-1]], y2[I[0:-1]])
# 计算重合区域宽高
w = np.maximum(0.0, xx2 - xx1 + 1)
h = np.maximum(0.0, yy2 - yy1 + 1)
# 计算重合区域面积
inter = w * h
# 计算交并比IOU
o = inter / (area[I[-1]] + area[I[0:-1]] - inter)
pick.append(I[-1])
I = I[np.where(o <= threshold)[0]]
result_rectangle = boxes[pick].tolist()
return result_rectangle
# -------------------------------------#
# 对pnet处理后的结果进行处理
# -------------------------------------#
def filter_face_24net(cls_prob, roi, rectangles, width, height, threshold):
prob = cls_prob[:, 1] # 获取预测人脸置信度得分
pick = np.where(prob >= threshold) # 获取置信度得分大于阈值的索引
# 人脸框转ndarray
rectangles = np.array(rectangles)
# 获取置信度大于阈值的人脸框
x1 = rectangles[pick, 0]
y1 = rectangles[pick, 1]
x2 = rectangles[pick, 2]
y2 = rectangles[pick, 3]
# 获取置信度得分大于阈值的置信度得分
sc = np.array([prob[pick]]).T
# 获取人脸框偏移量
dx1 = roi[pick, 0]
dx2 = roi[pick, 1]
dx3 = roi[pick, 2]
dx4 = roi[pick, 3]
# 计算人脸框宽高
w = x2 - x1
h = y2 - y1
# 计算人脸框在原图上的位置
x1 = np.array([(x1 + dx1 * w)[0]]).T
y1 = np.array([(y1 + dx2 * h)[0]]).T
x2 = np.array([(x2 + dx3 * w)[0]]).T
y2 = np.array([(y2 + dx4 * h)[0]]).T
rectangles = np.concatenate((x1, y1, x2, y2, sc), axis=1)
# 人脸框转成正方形
rectangles = rect2square(rectangles)
pick = []
# 遍历人脸框,防止人脸框越界
for i in range(len(rectangles)):
x1 = int(max(0, rectangles[i][0]))
y1 = int(max(0, rectangles[i][1]))
x2 = int(min(width, rectangles[i][2]))
y2 = int(min(height, rectangles[i][3]))
sc = rectangles[i][4]
if x2 > x1 and y2 > y1:
pick.append([x1, y1, x2, y2, sc])
# 对人脸框做非极大值抑制处理
return NMS(pick, 0.3)
# -------------------------------------#
# 对onet处理后的结果进行处理
# -------------------------------------#
def filter_face_48net(cls_prob, roi, pts, rectangles, width, height, threshold):
prob = cls_prob[:, 1] # 获取预测人脸置信度得分
pick = np.where(prob >= threshold) # 获取置信度得分大于阈值的索引
rectangles = np.array(rectangles) # 人脸框转ndarray
# 获取置信度大于阈值的人脸框
x1 = rectangles[pick, 0]
y1 = rectangles[pick, 1]
x2 = rectangles[pick, 2]
y2 = rectangles[pick, 3]
# 获取置信度得分大于阈值的置信度得分
sc = np.array([prob[pick]]).T
# 获取人脸框偏移量
dx1 = roi[pick, 0]
dx2 = roi[pick, 1]
dx3 = roi[pick, 2]
dx4 = roi[pick, 3]
# 计算人脸框宽高
w = x2 - x1
h = y2 - y1
# 计算人脸关键点在原图中的位置
pts0 = np.array([(w * pts[pick, 0] + x1)[0]]).T
pts1 = np.array([(h * pts[pick, 5] + y1)[0]]).T
pts2 = np.array([(w * pts[pick, 1] + x1)[0]]).T
pts3 = np.array([(h * pts[pick, 6] + y1)[0]]).T
pts4 = np.array([(w * pts[pick, 2] + x1)[0]]).T
pt
没有合适的资源?快使用搜索试试~ 我知道了~
基于FaceNet和MTCNN可在win10上可视化界面的人脸识别2.0版app
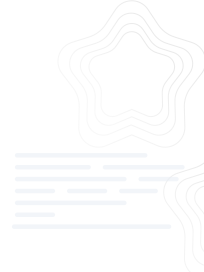
共76个文件
jpg:52个
py:11个
pyc:6个

1 下载量 97 浏览量
2023-07-10
13:15:21
上传
评论
收藏 574.34MB ZIP 举报
温馨提示
软件用到了pyqt5,tensorflow,opencv-python,pandas和numpy,使用之前要安装好这些python相应的库。人脸特征提取模型是基于LWF数据集使用FaceNet构造的模型,判定测试图像是否包含人脸是使用MTCNN算法生成的模型
资源推荐
资源详情
资源评论
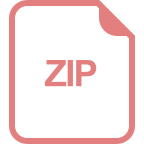
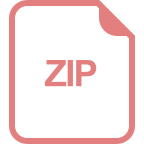
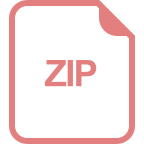
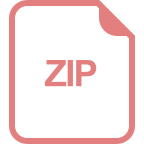
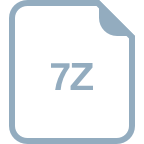
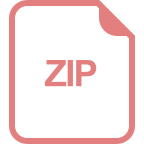
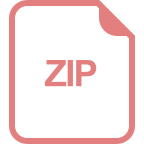
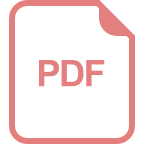
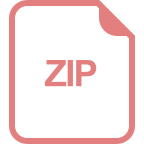
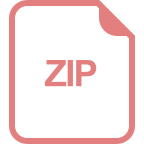
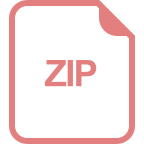
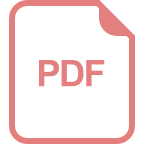
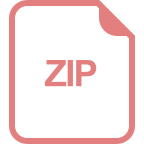
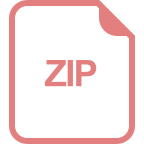
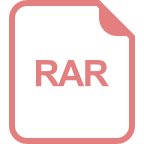
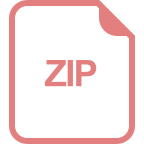
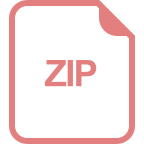
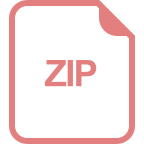
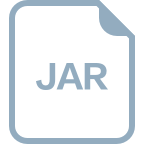
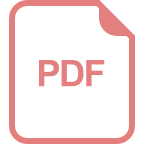
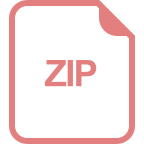
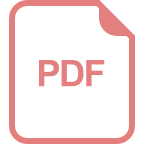
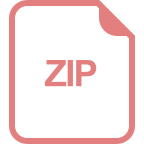
收起资源包目录


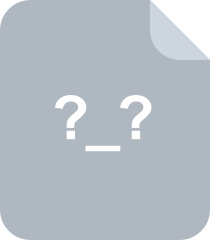
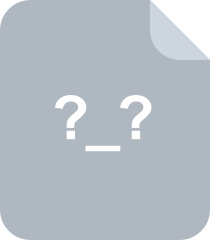

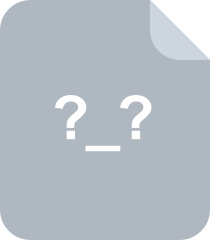

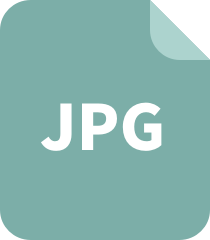
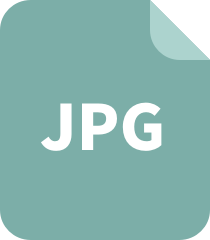
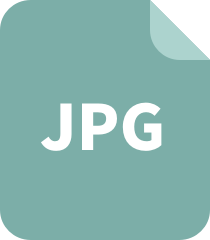
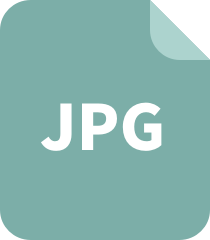
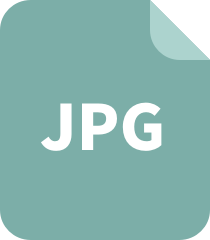
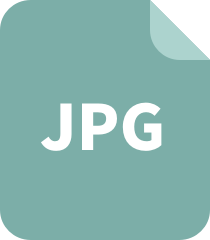
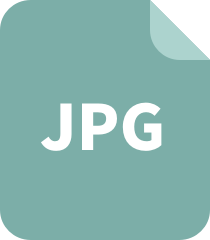
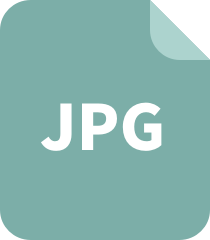
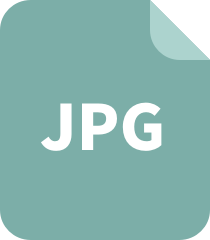
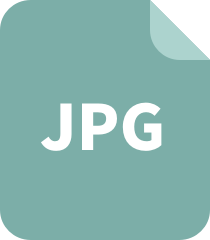
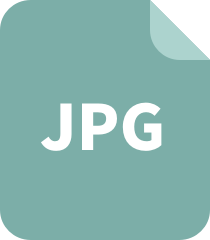
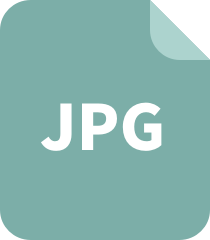
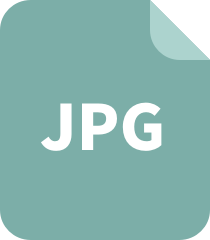
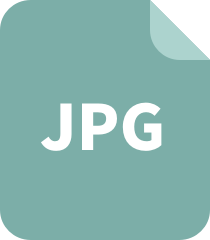
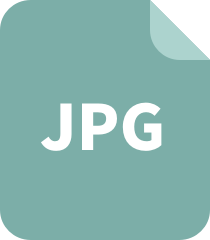
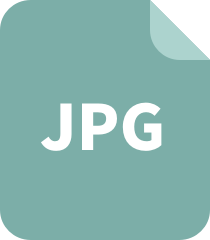
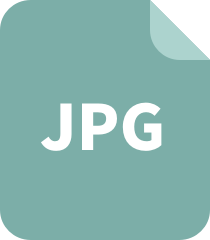
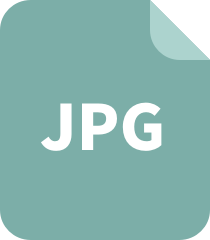
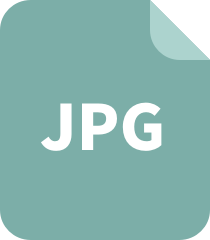
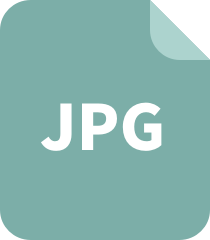
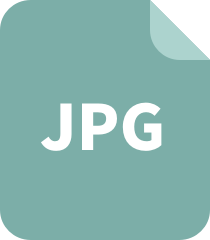
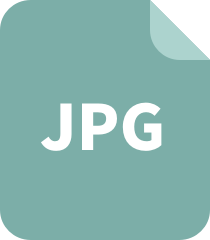
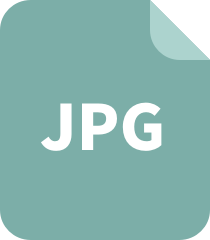
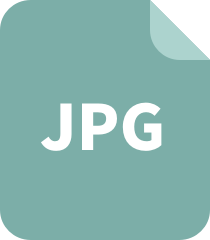
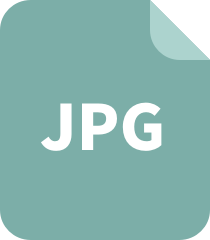
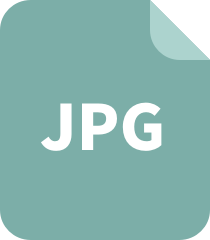
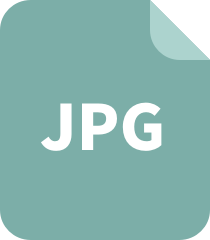
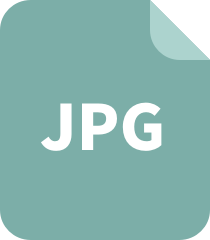
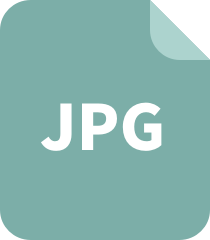
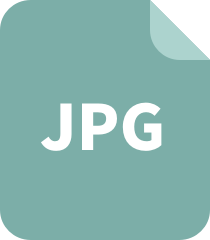
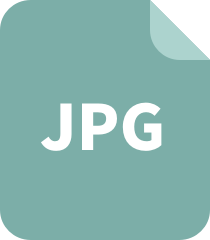
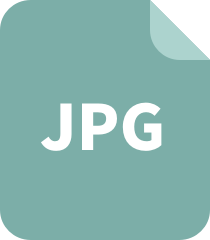
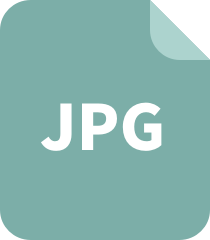
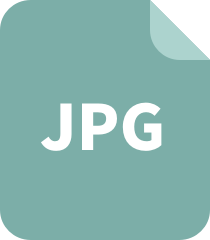
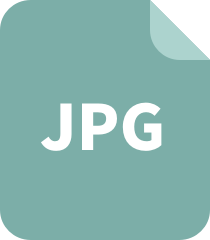
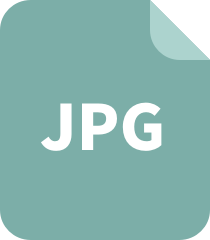
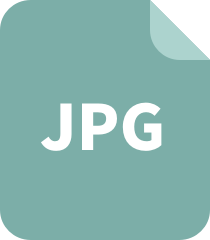
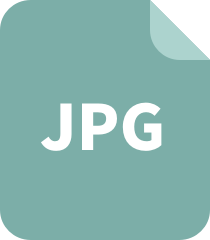
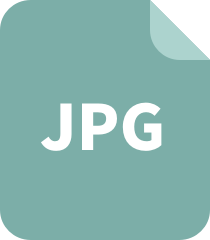
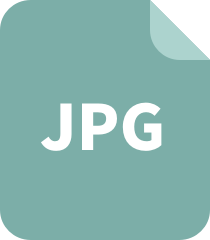
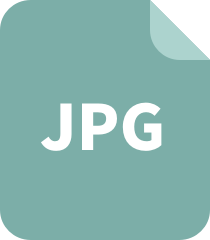
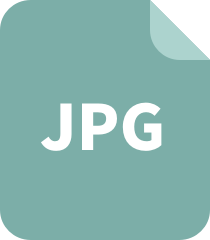
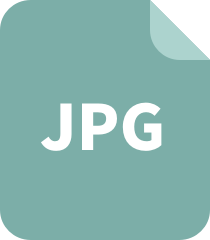
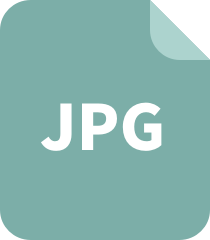
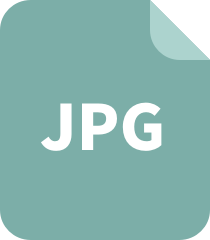
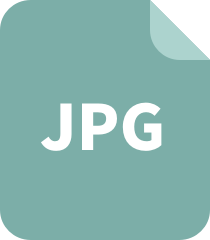
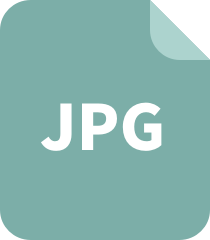
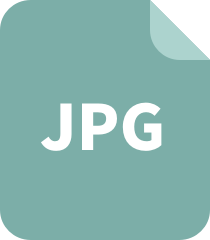
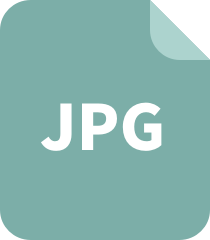
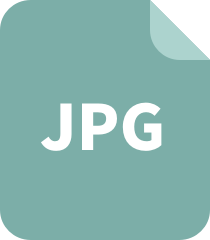
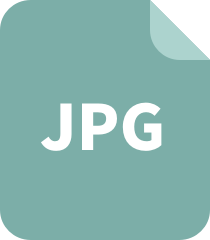
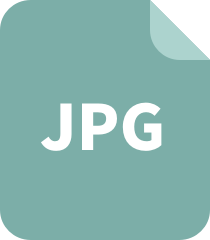
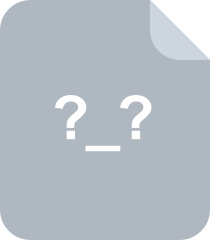
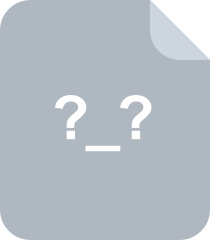
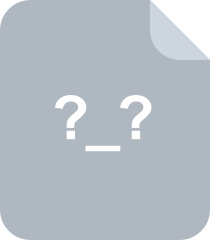
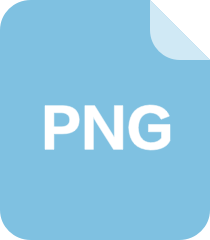

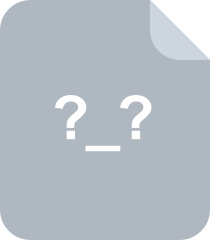
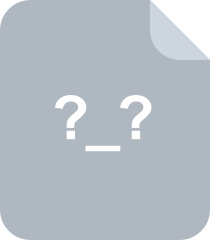
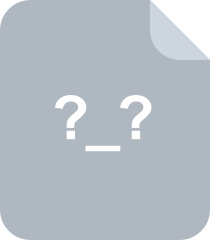
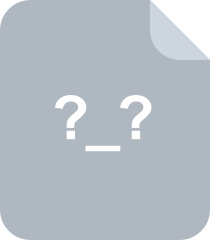
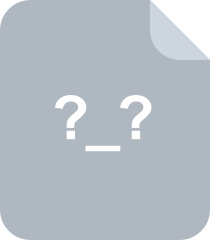
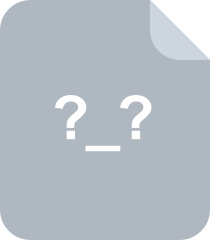
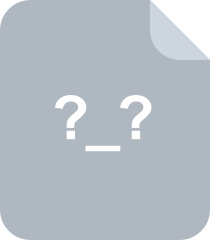

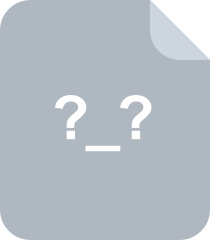
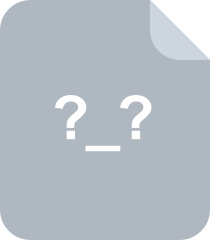
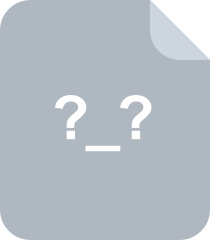
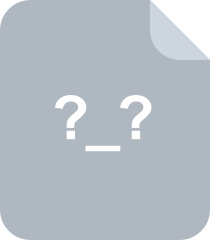
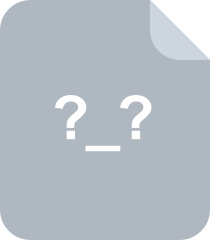
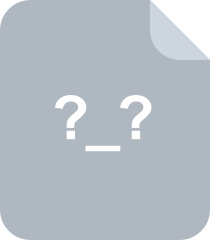
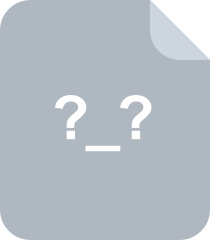

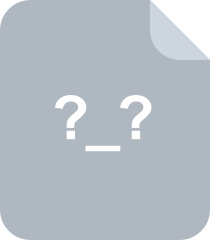
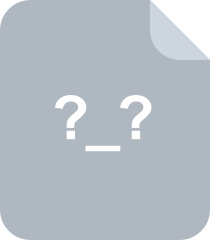
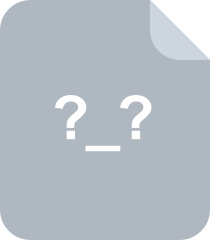
共 76 条
- 1
资源评论
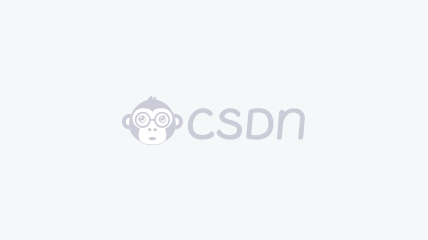

刘同敏
- 粉丝: 15
- 资源: 30
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

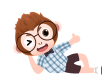
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


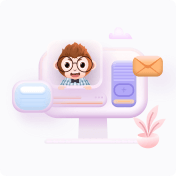
安全验证
文档复制为VIP权益,开通VIP直接复制
