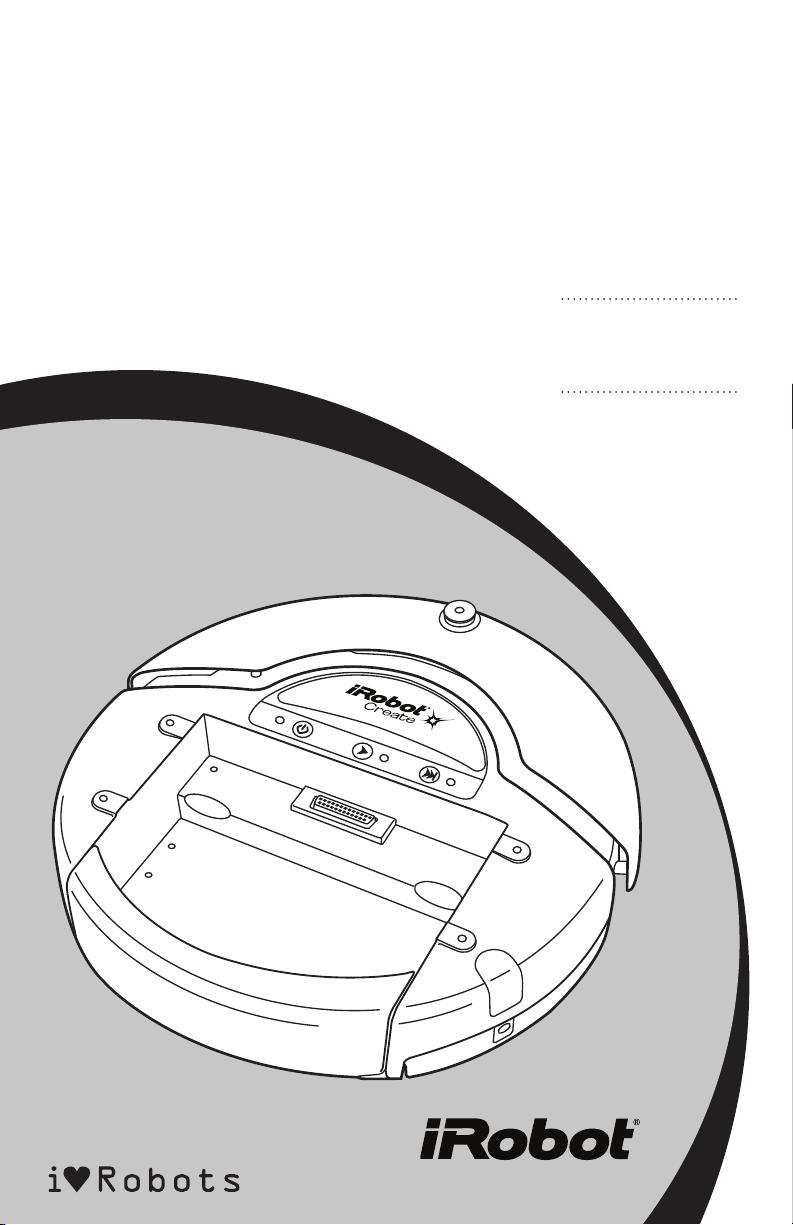
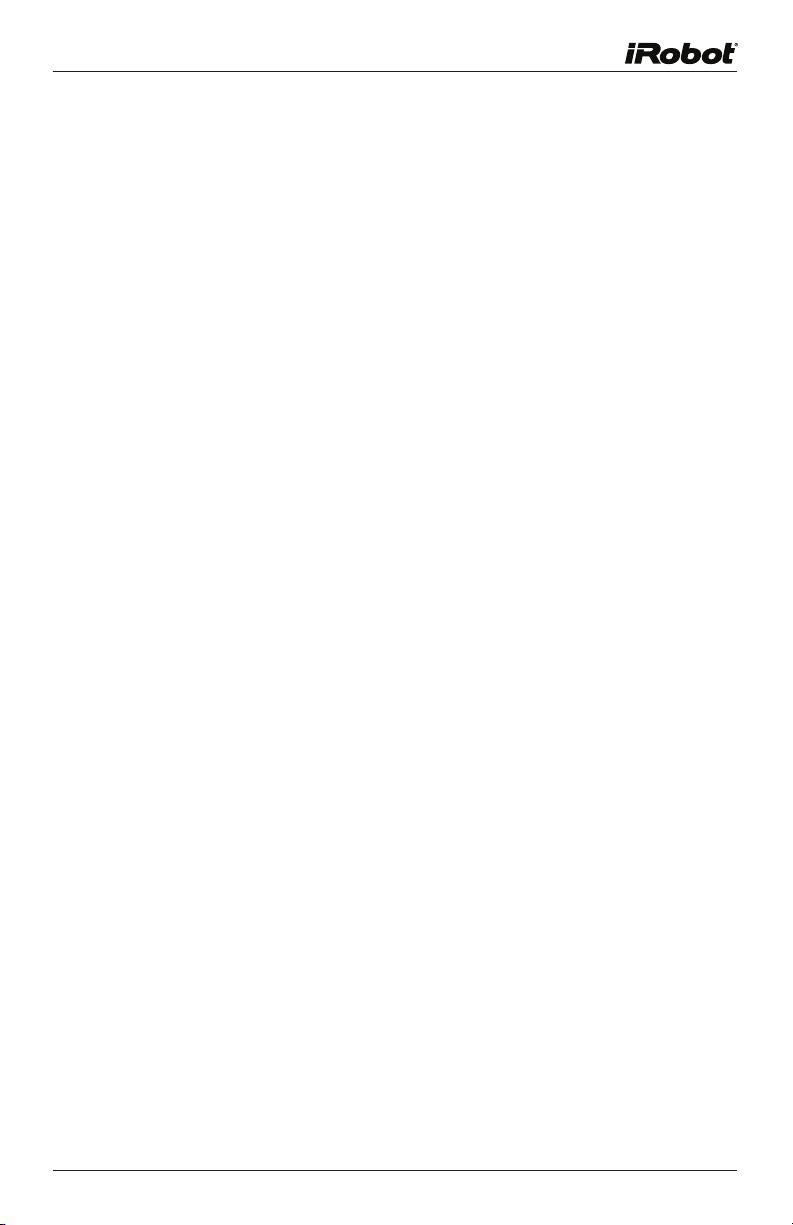
3
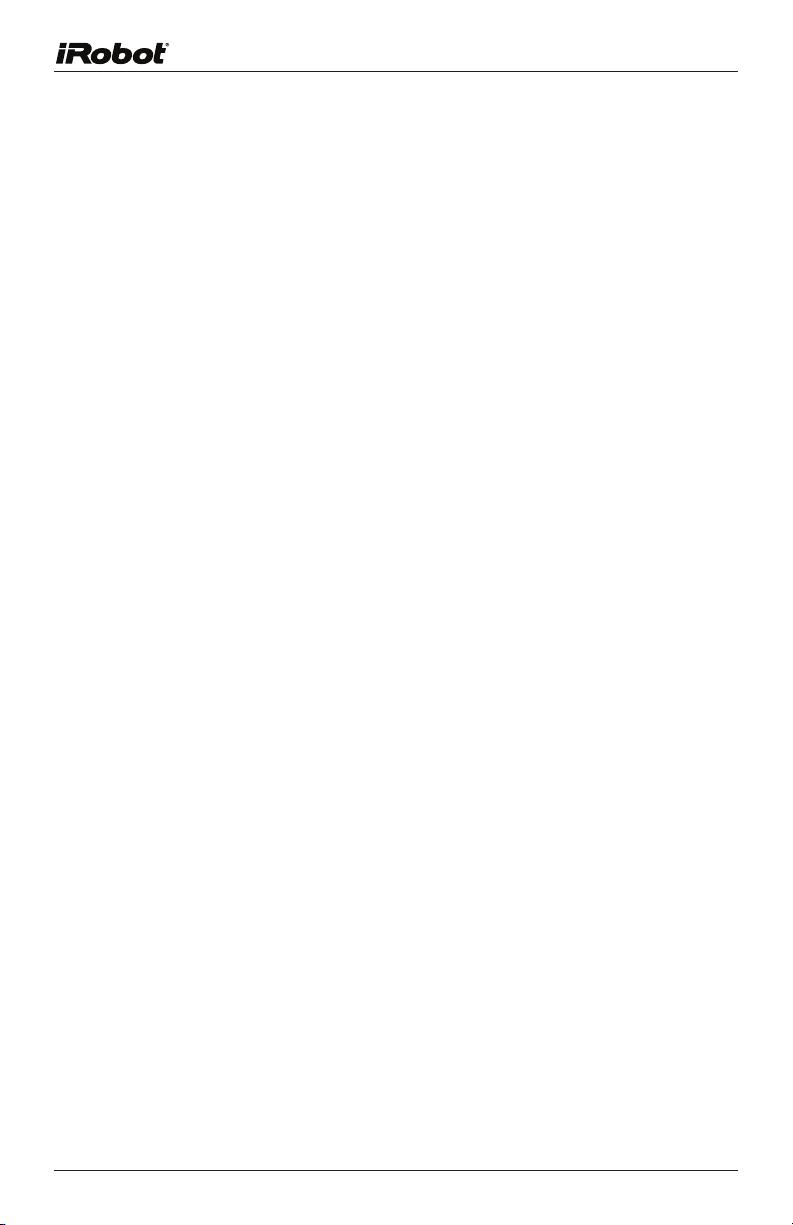
Preface
Peter C0rke
The practice of robotics and computer vision
each involve the application of computational algo-
rithms to data. The research community has devel-
oped a very large body of algorithms but for a
newcomer to the field this can be quite daunting.
For more th an 10 years th e author has ma intained two ope n-
source
matlab
®
Toolboxes, one for robotics and one for vision.
They provide implementations of many important algorithms and
allow users to work with real problems, not just trivial examples.
This new book makes the fundamental algorithms of robotics,
vision and control accessible to all. It weaves together theory, algo-
rithms and examples in a narrative that covers robotics and com-
puter vision separately and together. Using the latest versions
of the Toolboxes the author shows how complex probl ems can be
decomposed and solved using just a few simple lines of code.
The topics covered are guided by real problems observed by the
author over many years as a practitioner of both robotics and
computer vision. It is w ritten in a light but informative style, it is
easy to read and absorb, and includes over 1000
matlab
®
and
Simulink
®
examples and figures. The book is a real walk through
the fundamentals of mobile robots, navigation, localization, arm-
robot kinematics, dynamics and joint level control, then camera
models, image processing, feature extraction and multi-view
geometry, and finally bringing it all together with an extensive
discussion of visual servo systems.
Peter Corke
Robotics,
Vision
and
Control
Robotics, Vision and Control
isbn 978-3-642-20143-1
1
›
springer.com
123
Corke
FUNDAMENTAL
ALGORITHMS
IN MATLAB®
783642 2014319
Robotics,
Vision
and
Control
This, the ninth major release of the Toolbox, repre-
sents twenty years of development and a substantial
level of maturity. This version captures a large number
of changes and extensions generated over the last two
years which support my new book “Robotics, Vision &
Control” shown to the left.
The Toolbox has always provided many functions that
are useful for the study and simulation of classical arm-
type robotics, for example such things as kinematics,
dynamics, and trajectory generation. The Toolbox is
based on a very general method of representing the
kinematics and dynamics of serial-link manipulators.
These parameters are encapsulated in MATLAB
R
ob-
jects — robot objects can be created by the user for any serial-link manipulator and a
number of examples are provided for well know robots such as the Puma 560 and the
Stanford arm amongst others. The Toolbox also provides functions for manipulating
and converting between datatypes such as vectors, homogeneous transformations and
unit-quaternions which are necessary to represent 3-dimensional position and orienta-
tion.
This ninth release of the Toolbox has been significantly extended to support mobile
robots. For ground robots the Toolbox includes standard path planning algorithms
(bug, distance transform, D*, PRM), kinodynamic planning (RRT), localization (EKF,
particle filter), map building (EKF) and simultaneous localization and mapping (EKF),
and a Simulink model a of non-holonomic vehicle. The Toolbox also includes a de-
tailed Simulink model for a quadrotor flying robot.
The routines are generally written in a straightforward manner which allows for easy
understanding, perhaps at the expense of computational efficiency. If you feel strongly
about computational efficiency then you can always rewrite the function to be more
efficient, compile the M-file using the MATLAB
R
compiler, or create a MEX version.
This manual is now essentially auto-generated from the comments in the MATLAB
R
code itself which reduces the effort in maintaining code and a separate manual as I used
to — the downside is that there are no worked examples and figures in the manual.
However the book “Robotics, Vision & Control” provides a detailed discussion (600
pages, nearly 400 figures and 1000 code examples) of how to use the Toolbox functions
to solve many types of problems in robotics.
Robotics Toolbox 9.10 for MATLAB
R
4 Copyright
c
Peter Corke 2015
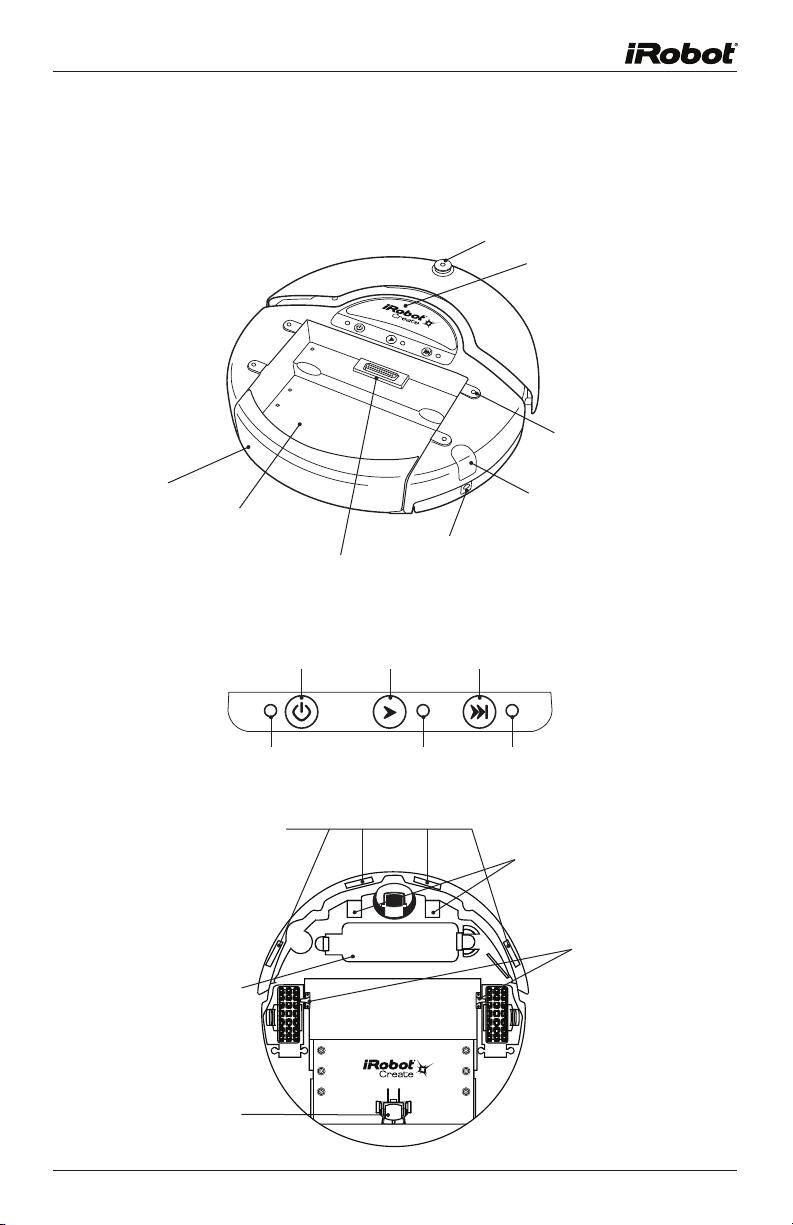
Contents
Preface . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
Functions by category . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10
1 Introduction 13
1.1 What’s changed . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13
1.1.1 New features and changes to RTB 9.10 . . . . . . . . . . . . 13
1.1.2 Earlier changes to RTB 9 . . . . . . . . . . . . . . . . . . . . 14
1.2 Migrating from RTB 8 and earlier . . . . . . . . . . . . . . . . . . . 16
1.2.1 New functions . . . . . . . . . . . . . . . . . . . . . . . . . 17
1.2.2 General improvements . . . . . . . . . . . . . . . . . . . . . 18
1.3 How to obtain the Toolbox . . . . . . . . . . . . . . . . . . . . . . . 18
1.3.1 Documentation . . . . . . . . . . . . . . . . . . . . . . . . . 19
1.4 MATLAB version issues . . . . . . . . . . . . . . . . . . . . . . . . 19
1.5 Use in teaching . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19
1.6 Use in research . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19
1.7 Support . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 20
1.8 Related software . . . . . . . . . . . . . . . . . . . . . . . . . . . . 20
1.8.1 Octave . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 20
1.8.2 Python version . . . . . . . . . . . . . . . . . . . . . . . . . 21
1.8.3 Machine Vision toolbox . . . . . . . . . . . . . . . . . . . . 21
1.9 Contributing to the Toolboxes . . . . . . . . . . . . . . . . . . . . . 21
1.10 Acknowledgements . . . . . . . . . . . . . . . . . . . . . . . . . . . 21
2 Functions and classes 22
about . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 22
angdiff . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 22
angvec2r . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 23
angvec2tr . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 23
Animate . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 24
Arbotix . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 25
bresenham . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 33
Bug2 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 34
ccodefunctionstring . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 35
circle . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 36
CodeGenerator . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 37
colnorm . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 68
colorname . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 68
ctraj . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 69
Robotics Toolbox 9.10 for MATLAB
R
5 Copyright
c
Peter Corke 2015