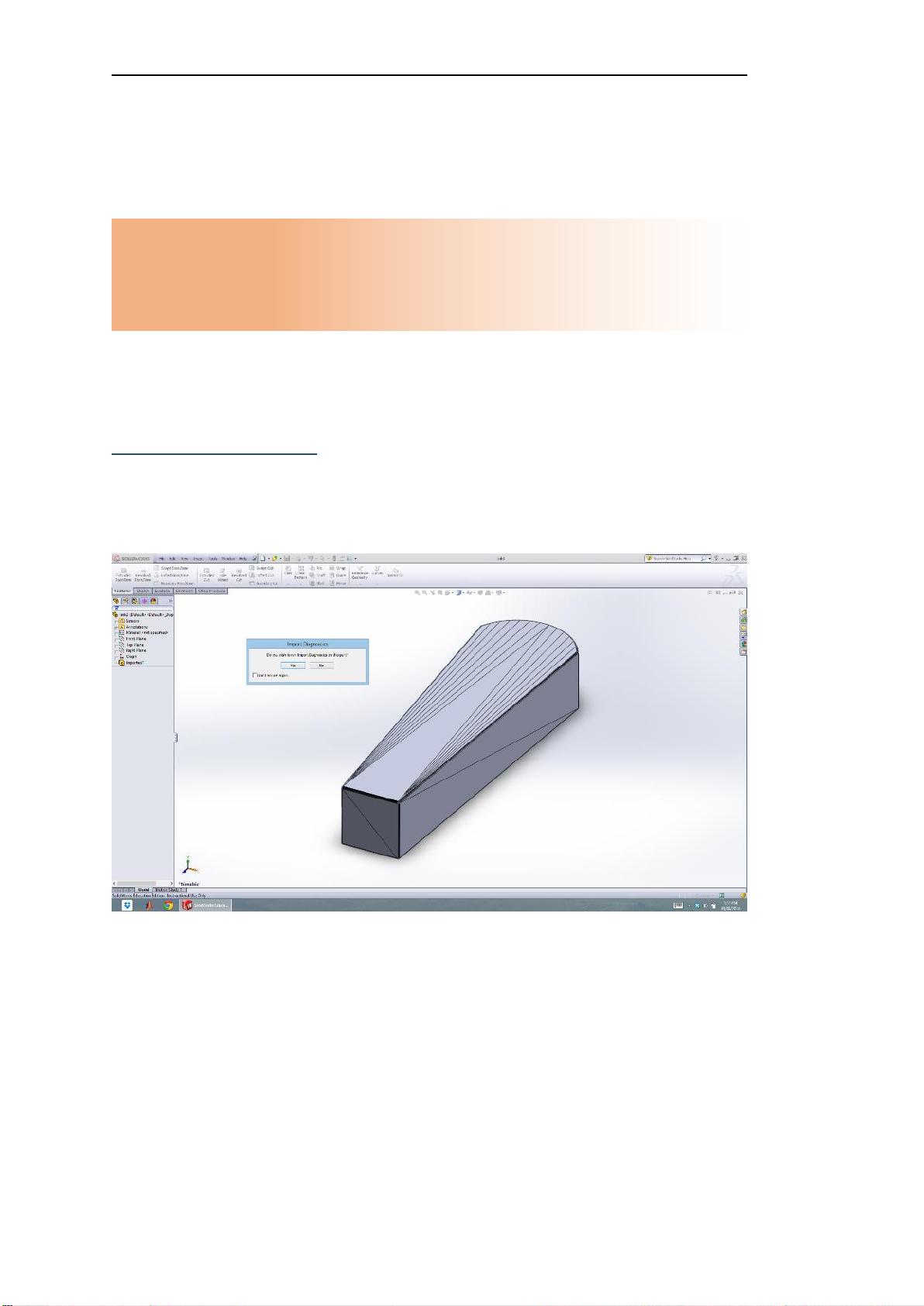
Robotics Toolbox
for MATLAB
R
Release 10
Peter Corke
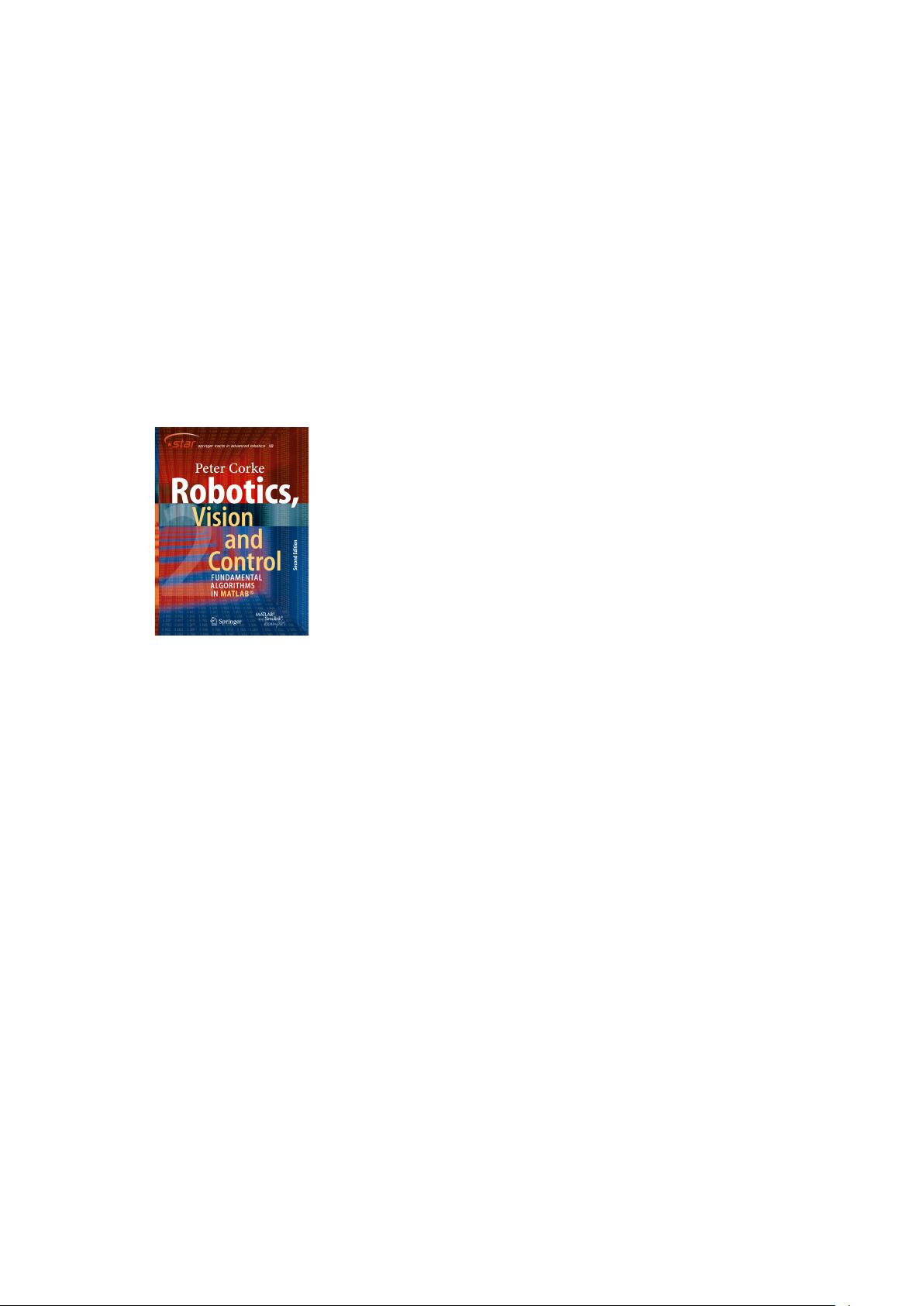
Preface
This, the tenth major release of the Toolbox, represent-
ing over twenty five years of continuous development
and a substantial level of maturity. This version cor-
responds to the second edition of the book “Robotics,
Vision & Control” published in June 2017 – RVC2.
This MATLAB
R
Toolbox has a rich collection of func-
tions that are useful for the study and simulation of
robots: arm-type robot manipulators and mobile robots.
For robot manipulators, functions include kinematics,
trajectory generation, dynamics and control. For mobile
robots, functions include path planning, kinodynamic
planning, localization, map building and simultaneous
localization and mapping (SLAM).
The Toolbox makes strong use of classes to represent robots and such things as sen-
sors and maps. It includes Simulink
R
models to describe the evolution of arm or
mobile robot state over time for a number of classical control strategies. The Tool-
box also provides functions for manipulating and converting between datatypes such
as vectors, rotation matrices, unit-quaternions, quaternions, homogeneous transforma-
tions and twists which are necessary to represent position and orientation in 2- and
3-dimensions.
The code is written in a straightforward manner which allows for easy understanding,
perhaps at the expense of computational efficiency. If you feel strongly about computa-
tional efficiency then you can always rewrite the function to be more efficient, compile
the M-file using the MATLAB compiler, or create a MEX version.
The bulk of this manual is auto-generated from the comments in the MATLAB code
itself. For elaboration on the underlying principles, extensive illustrations and worked
examples please consult “Robotics, Vision & Control, second edition” which provides
a detailed discussion (720 pages, nearly 500 figures and over 1000 code examples) of
how to use the Toolbox functions to solve many types of problems in robotics.
Robotics Toolbox 10.3.1 for MATLAB
R
3 Copyright
c
Peter Corke 2018
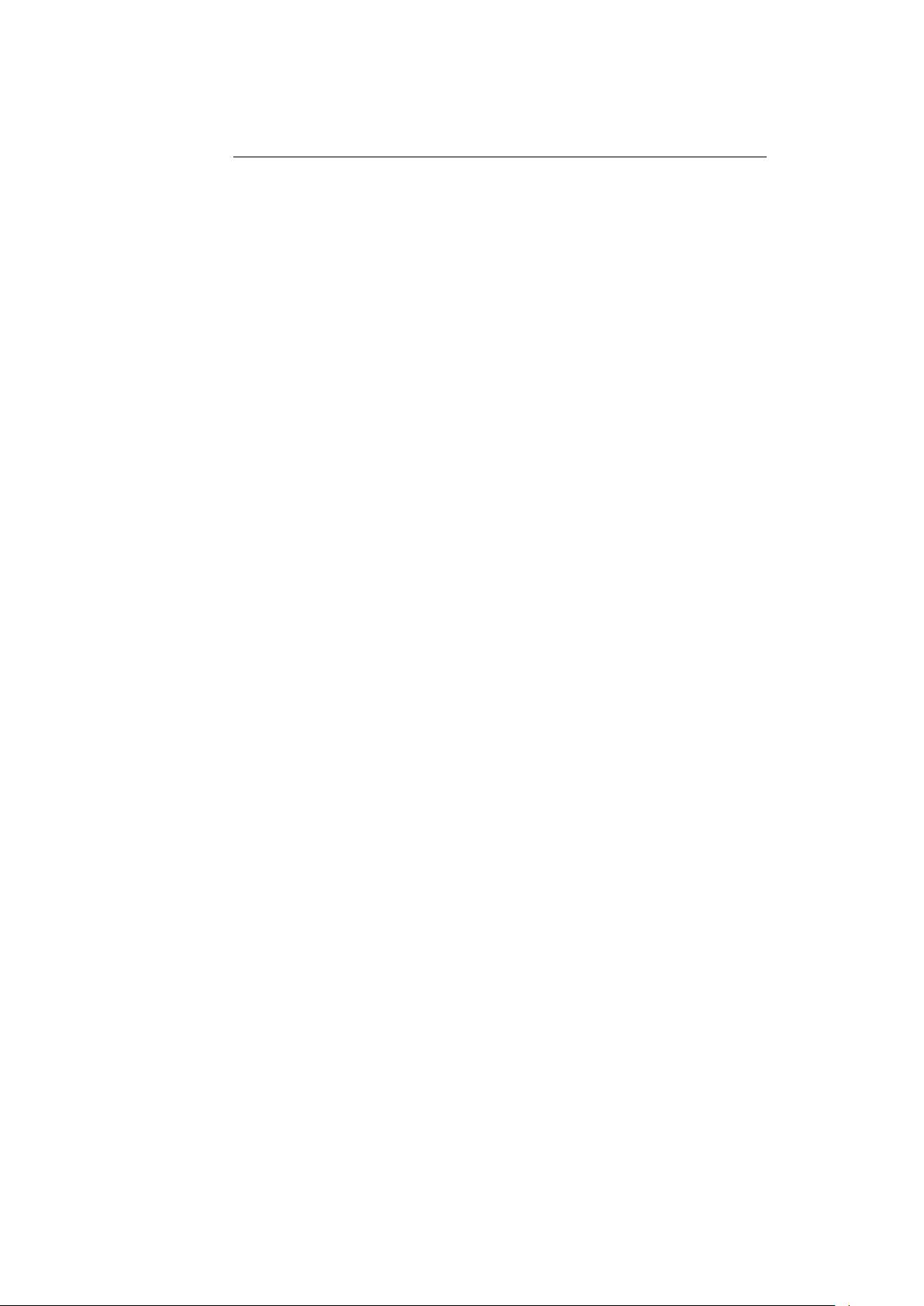
Robotics Toolbox 10.3.1 for MATLAB
R
4 Copyright
c
Peter Corke 2018
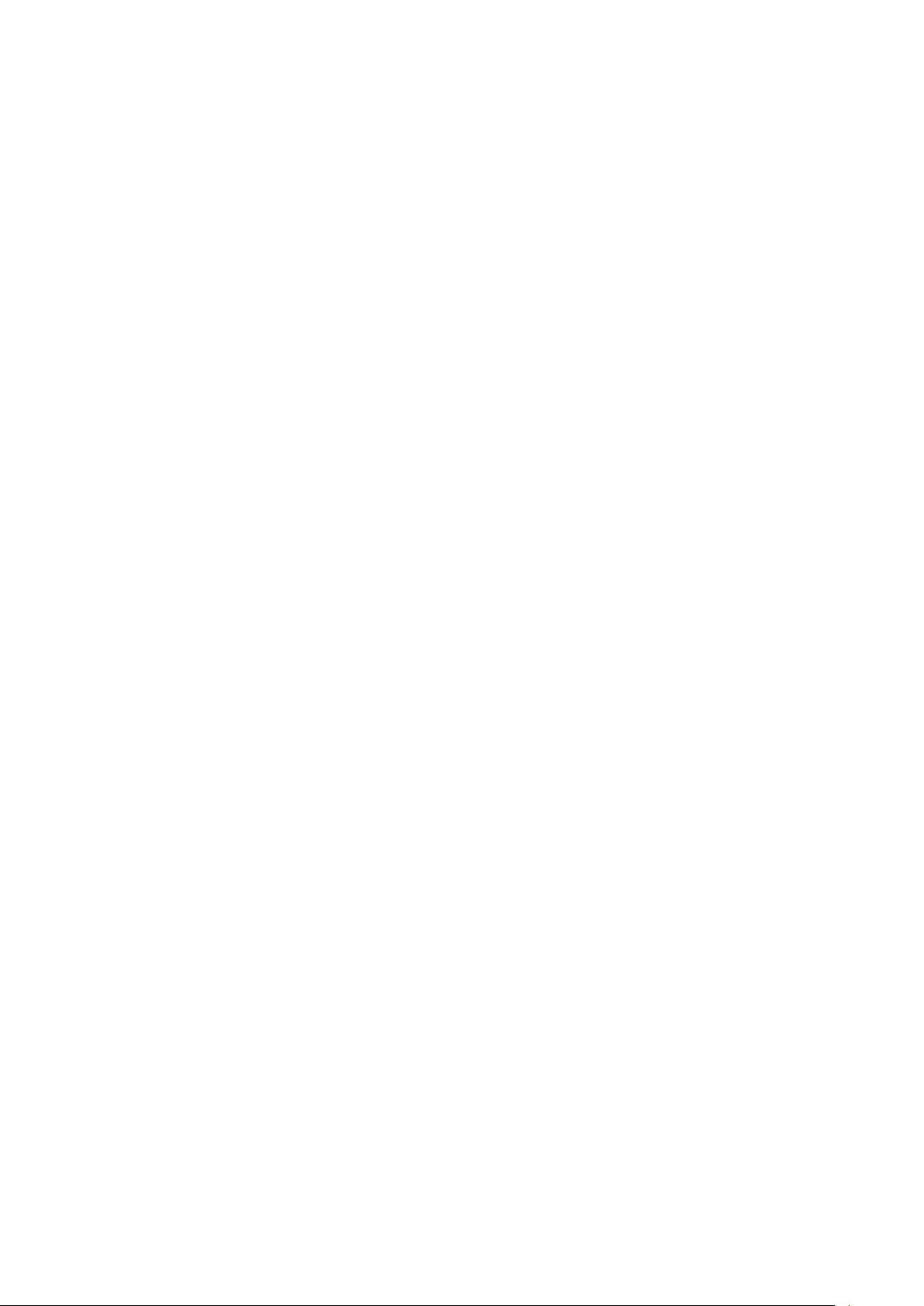
Functions by category
Homogeneous transforma-
tions 3D
angvec2r . . . . . . . . . . . . . . . . . . . . . . . . . . . 25
angvec2tr . . . . . . . . . . . . . . . . . . . . . . . . . . 26
eul2r . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 117
eul2tr . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 118
ishomog . . . . . . . . . . . . . . . . . . . . . . . . . . 120
isrot . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 121
isunit . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 122
oa2r . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 184
oa2tr . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 184
rotx . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 268
roty . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 269
rotz . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 269
rpy2r . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 270
rpy2tr. . . . . . . . . . . . . . . . . . . . . . . . . . . . . 271
tr2angvec . . . . . . . . . . . . . . . . . . . . . . . . . 391
tr2eul . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 393
tr2rpy. . . . . . . . . . . . . . . . . . . . . . . . . . . . . 394
transl . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 398
trchain . . . . . . . . . . . . . . . . . . . . . . . . . . . . 399
trexp. . . . . . . . . . . . . . . . . . . . . . . . . . . . . .401
trinterp . . . . . . . . . . . . . . . . . . . . . . . . . . . 403
trlog . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 404
trnorm . . . . . . . . . . . . . . . . . . . . . . . . . . . . 404
trotx . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 406
troty . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 406
trotz . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 407
trprint . . . . . . . . . . . . . . . . . . . . . . . . . . . . 410
trscale . . . . . . . . . . . . . . . . . . . . . . . . . . . . 411
Homogeneous transforma-
tions 2D
ishomog2 . . . . . . . . . . . . . . . . . . . . . . . . . 121
isrot2 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 122
rot2 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 268
transl2 . . . . . . . . . . . . . . . . . . . . . . . . . . . . 398
trchain2 . . . . . . . . . . . . . . . . . . . . . . . . . . . 400
trexp2 . . . . . . . . . . . . . . . . . . . . . . . . . . . . 402
trinterp2 . . . . . . . . . . . . . . . . . . . . . . . . . . 403
trot2 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 405
trprint2 . . . . . . . . . . . . . . . . . . . . . . . . . . . 411
Homogeneous transforma-
tion utilities
r2t . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 254
rt2tr . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 276
t2r . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 388
tr2rt . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 395
Homogeneous points and
lines
e2h . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 90
h2e . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 119
homline . . . . . . . . . . . . . . . . . . . . . . . . . . . 119
homtrans . . . . . . . . . . . . . . . . . . . . . . . . . . 120
Differential motion
delta2tr . . . . . . . . . . . . . . . . . . . . . . . . . . . . 79
eul2jac . . . . . . . . . . . . . . . . . . . . . . . . . . . .117
rpy2jac . . . . . . . . . . . . . . . . . . . . . . . . . . . 270
skewa. . . . . . . . . . . . . . . . . . . . . . . . . . . . . 364
skew . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 363
tr2delta . . . . . . . . . . . . . . . . . . . . . . . . . . . 392
tr2jac . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 394
vexa . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 450
vex . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 449
wtrans . . . . . . . . . . . . . . . . . . . . . . . . . . . . 482
Robotics Toolbox 10.3.1 for MATLAB
R
5 Copyright
c
Peter Corke 2018