// cvtest.cpp : 定义应用程序的入口点。
//
#include "stdafx.h"
#include "cvtest.h"
#include <cv.h>
#include <highgui.h>
#include <opencv2/opencv.hpp>
#include "iostream"
using namespace cv;
using namespace std;
#pragma comment (linker, "/subsystem:\"windows\" /entry:\"mainCRTStartup\"")
#define MAX_LOADSTRING 100
cv::Mat image,gray,gray1,gray2,result,result1,result2,highM,lowM;
VideoCapture m_capture;//视频来源,文件或摄像头
//VideoCapture m_video;
DWORD lineBytes;
int x=0,y=0;
float Icount;
typedef struct frame
{ int **fr;
int num;
}f;
// 全局变量:
HINSTANCE hInst; // 当前实例
TCHAR szTitle[MAX_LOADSTRING]; // 标题栏文本
TCHAR szWindowClass[MAX_LOADSTRING]; // 主窗口类名
bool a;
// 此代码模块中包含的函数的前向声明:
ATOM MyRegisterClass(HINSTANCE hInstance);
BOOL InitInstance(HINSTANCE, int);
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);
INT_PTR CALLBACK About(HWND, UINT, WPARAM, LPARAM);
//void showavi(VideoCapture &m_capture,HDC hdc)
//{
// Mat m_curFrame;//当前帧,用于绘制或处理
// if(m_capture.isOpened())
// {
// while(true)
// {
// if(m_curFrame.cols!=m_capture.get(CV_CAP_PROP_FRAME_WIDTH)||m_curFrame.rows!=m_capture.get(CV_CAP_PROP_FRAME_HEIGHT))
// {//CV_CAP_PROP_FRAME_WIDTH - 视频帧宽度 CV_CAP_PROP_FRAME_HEIGHT - 视频帧高度 cols代表列。rows代表行
// //m_curFrame.release();
// m_curFrame.create(m_capture.get(CV_CAP_PROP_FRAME_HEIGHT),m_capture.get(CV_CAP_PROP_FRAME_WIDTH),CV_8UC3);
// }
// m_capture>>m_curFrame; //在retrieve之前加grab也可以
//
// ::BITMAPINFO info;
// memset(&info,0,sizeof(info));
// info.bmiHeader.biClrImportant=0;
// info.bmiHeader.biClrUsed=0;
// info.bmiHeader.biCompression=0L;
// info.bmiHeader.biPlanes=1;
// info.bmiHeader.biSize=sizeof(BITMAPINFOHEADER);
// info.bmiHeader.biXPelsPerMeter=0;
// info.bmiHeader.biYPelsPerMeter=0;
// info.bmiHeader.biBitCount=m_curFrame.channels()*8;
// info.bmiHeader.biHeight=m_curFrame.rows;
// info.bmiHeader.biWidth=m_curFrame.cols;
// lineBytes=(m_curFrame.cols*8+31)/32*4;
// info.bmiHeader.biSizeImage=info.bmiHeader.biHeight*lineBytes;
// if(m_curFrame.empty()) break;
// ::SetStretchBltMode(hdc,COLORONCOLOR);
// ::StretchDIBits(hdc,0,0,100,100,0,m_curFrame.rows-1,m_curFrame.cols,-m_curFrame.rows,m_curFrame.data,&info,DIB_RGB_COLORS,SRCCOPY);
// Sleep(66.9);
// }
// }
//}
//bool showImage(Mat &Img,HDC hdc,int x,int y)
void showImage(Mat &Img,HDC hdc,int x,int y)
{
typedef struct
{
BITMAPINFO info;
RGBQUAD rgb[255];
} mybitmapinfo;
mybitmapinfo myinfo;
memset(&myinfo,0,sizeof(myinfo));
myinfo.info.bmiHeader.biBitCount=Img.channels()*8;
myinfo.info.bmiHeader.biClrImportant=0;
myinfo.info.bmiHeader.biClrUsed=0;
myinfo.info.bmiHeader.biCompression=BI_RGB;
myinfo.info.bmiHeader.biHeight=Img.rows;
myinfo.info.bmiHeader.biPlanes=1;
myinfo.info.bmiHeader.biSize=sizeof(BITMAPINFOHEADER);
myinfo.info.bmiHeader.biSizeImage=myinfo.info.bmiHeader.biHeight * ( (Img.cols*myinfo.info.bmiHeader.biBitCount+31)/32*4 );
myinfo.info.bmiHeader.biWidth=Img.cols;
myinfo.info.bmiHeader.biXPelsPerMeter=0;
myinfo.info.bmiHeader.biYPelsPerMeter=0;
myinfo.info.bmiColors[0].rgbBlue=myinfo.info.bmiColors[0].rgbGreen=myinfo.info.bmiColors[0].rgbRed=0;
for(int i=0;i<255;i++)
{
myinfo.rgb[i].rgbBlue=i+1;
myinfo.rgb[i].rgbGreen=i+1;
myinfo.rgb[i].rgbRed=i+1;
}
SetStretchBltMode(hdc,COLORONCOLOR);
StretchDIBits(hdc,x,y,100,100,0,Img.rows-1,Img.cols,-Img.rows,Img.data,&myinfo.info,DIB_RGB_COLORS,SRCCOPY);
}
//累积背景图像和前后帧图像差值的绝对值
//当累积够一定数量后就将其转换成一个背景统计模型
void accumulateBackground(Mat &image)
{
static int first=1; //局部静态变量,只初始化一次,意思就是第一次被赋值为1
cvCvtScale(image,gray,1,0);
if (!first)
{
cvAcc(gray,result);
cvAbsDiff(gray,gray1,result1);
cvAcc(result1,gray2);
Icount+=1.0;
}
first=0;
cvCopy(gray,gray1);
}
void setHighThreshold(float scale)
{
cvConvertScale(gray2,gray,scale);
cvAdd(gray,result,highM);
}
void setLowThreshold(float scale)
{
cvConvertScale(gray2,gray,scale);
cvSub(result,gray,lowM);
}
//当累积足够多的帧图后,就将其转化成一个背景统计模型
//该函数用于计算每个像素的均值和平均绝对差分
void Createmode()
{
cvConvertScale(result,result,(double)(1.0/Icount));
cvConvertScale(gray2,gray2,(double)(1.0/Icount));
setHighThreshold(7.0);
setLowThreshold(6.0);
}
//图像分割
void backgroundDiff(Mat &image)
{
cvCvtScale(image,gray,1,0);
cvInRange(gray,highM,lowM,result2);
cvSubRS(result2,CvScalar(255),result2);
}
void Targetdetect(VideoCapture &m_capture,HDC hdc)
{
Mat m_curFrame;//当前帧,用于绘制或处理
Mat m_Frame,m_Frame1;
Mat m0; //背景
// Mat target;
int T=15;
unsigned char *np=NULL,*nm0=NULL; // 图像指针*data=new target.data
int t=0;//第t帧
if(m_capture.isOpened())
{
while(true)
{
if(m_curFrame.cols!=m_capture.get(CV_CAP_PROP_FRAME_WIDTH)||m_curFrame.rows!=m_capture.get(CV_CAP_PROP_FRAME_HEIGHT))
{//CV_CAP_PROP_FRAME_WIDTH - 视频帧宽度 CV_CAP_PROP_FRAME_HEIGHT - 视频帧高度 cols代表列。rows代表行
//m_curFrame.release();
m_curFrame.create(m_capture.get(CV_CAP_PROP_FRAME_HEIGHT),m_capture.get(CV_CAP_PROP_FRAME_WIDTH),CV_8UC3);
}
// m_capture>>m_curFrame; //在retrieve之前加grab也可以
//捕获当前帧
m_capture.grab();
m_capture.retrieve(m_curFrame);
if(m_curFrame.empty())
break;
//Rgb24ToGray8(m_curFrame,m_Frame);
cvtColor(m_curFrame,m_Frame1,CV_BGR2GRAY);
cvConvert(m_Frame,m_Frame1);
for (t=0;;t++)
{
if (t<30)
{
accumulateBackground(m_Frame);
//前30帧用于累积计算背景图像
if (t=30)
Createmode();
//将前30帧转换成背景模型
}
else
backgroundDiff(m_Frame);
//进行分割
}
// if(t==0)
// m_Frame.copyTo(m0);
// /*m0=m_Frame;*///前一帧为背景
// if(t>0)
// {
// for(int i=0;i<m_curFrame.rows;i++)
// { np=m_Frame.ptr<uchar>(i);
// nm0=m0.ptr<uchar>(i);
// for(int j=0;j<m_curFrame.cols;j++)
// {
// int n=abs(np[j]-nm0[j]);
// if (n>=T)
// {np[j]=255; }
// else np[j]=0;
// }
// }
// //target.data=data;
// }
//t++;
//x+=100;
//if(x>1000){x=0;y+=100;}
showImage(result2,hdc,x,y);
}
}
}
int main(int argc,char **argv)
{
HINSTANCE hInstance=NULL;
HINSTANCE hPrevInstance=NULL;
LPTSTR lpCmdLine;
int nCmdShow=SW_SHOW;
UNREFERENCED_PARAMETER(hPrevInstance);
UNREFERENCED_PARAMETER(lpCmdLine);
//image=imread("top.bmp");
// TODO: 在此放置代码。F:\vs2010\新建文件夹\cv003\
MSG msg;
HACCEL hAccelTable;
// 初始化全局字符串
LoadString(hInstance, IDS_APP_TITLE, szTitle, MAX_LOADSTRING);
LoadString(hInstance, IDC_CVTEST, szWindowClass, MAX_LOADSTRING);
MyRegisterClass(hInstance);
// 执行应用程序初始化:
if (!InitInstance (hInstance, nCmdShow))
{
return FALSE;
}
hAccelTable = LoadAccelerators(hInstance, MAKEINTRESOURCE(IDC_CVTEST));
// 主消息循环:
while (GetMessage(&msg, NULL, 0, 0))
{
if (!TranslateAccelerator(msg.hwnd, hAccelTable, &msg))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
}
return (int) msg.wParam;
}
//
// 函数: MyRegisterClass()
//
// 目的: 注册窗口类。
//
// 注释:
//
// 仅当希望
// 此代码与添加到 Windows 95 中的“RegisterClassEx”
// 函数之前的 Win32 系统兼容时,才需要此函数及其用法。调用此函数十分重要,
// 这样应用程序就可以获得关联的
// “格式正确的”小图标。
//
ATOM MyRegisterClass(HINSTANCE hInstance)
{
WNDCLASSEX wcex;
wcex.cbSize = sizeof(WNDCLASSEX);
wcex.style = CS_HREDRAW | CS_VREDRAW;
wcex.lpfnWndProc = WndProc;
wcex.cbClsExtra = 0;
wcex.cbWndExtra

yxkfw
- 粉丝: 82
- 资源: 2万+
最新资源
- 基于大数据的图书馆个性化服务研究及信息管理系统设计(基于pyhton和MySQL的信息管理系统,源代码开发环境为MacOS,使用前请将编码格式转换为utf-8)
- c# RSA加解密工具,.netRSA加解密工具
- 基于yolov8的基建裂缝目标检测系统Python源码+文档说明+数据集(高分项目)
- 无线路由器行业市场调研报告:未来几年年复合增长率CAGR为8.1%
- 第2周实验答案.ipynb
- 180度液压翻转机(sw16可编辑+工程图)全套技术资料100%好用.zip
- 基于python+yolov8开发的基建裂缝目标检测系统源码+文档说明+数据集(高分项目)
- 5斤装颗粒物粉剂食品包装机sw16可编辑全套技术资料100%好用.zip
- Blade Pin Module 弹片探针模组step全套技术资料100%好用.zip
- 葡萄酒基因型分类数据,葡萄酒分类数据集
- 500T钻铣中心钣金防护罩sw18全套技术资料100%好用.zip
- 18650电池装盆机(sw16可编辑+工程图)全套技术资料100%好用.zip
- CC2530原理图例程
- Braun线束对裁设备(sw15可编辑+工程图)全套技术资料100%好用.zip
- C语言-数据结构手写实现全部经典数据结构操作合集
- RCO活性炭吸附脱附和催化燃烧设备sw16可编辑全套技术资料100%好用.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


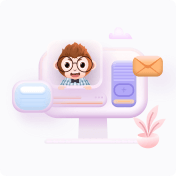