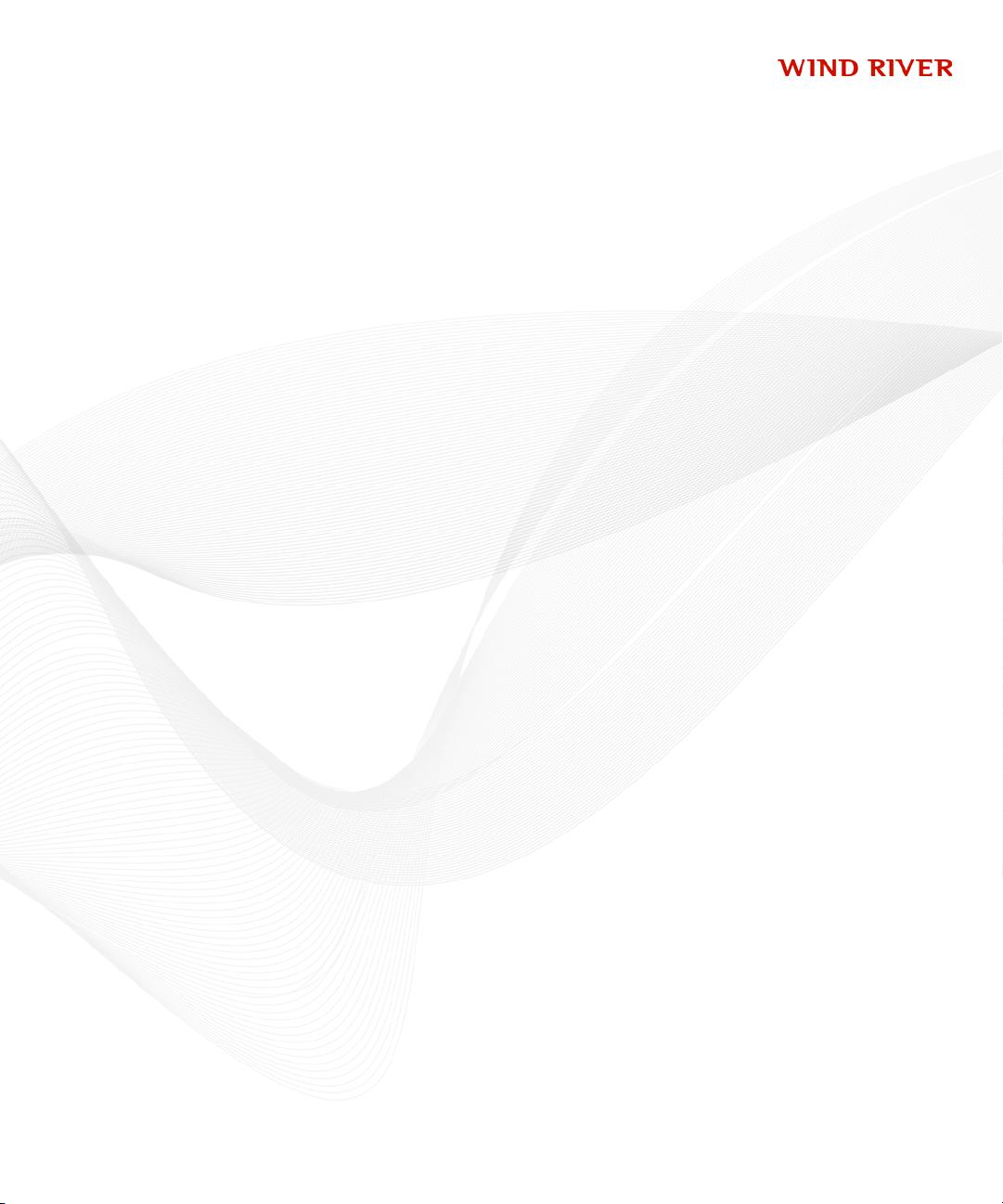
VxWorks
APPLICATION PROGRAMMER'S GUIDE
®
6.6
VxWorks Application Programmer's Guide, 6.6
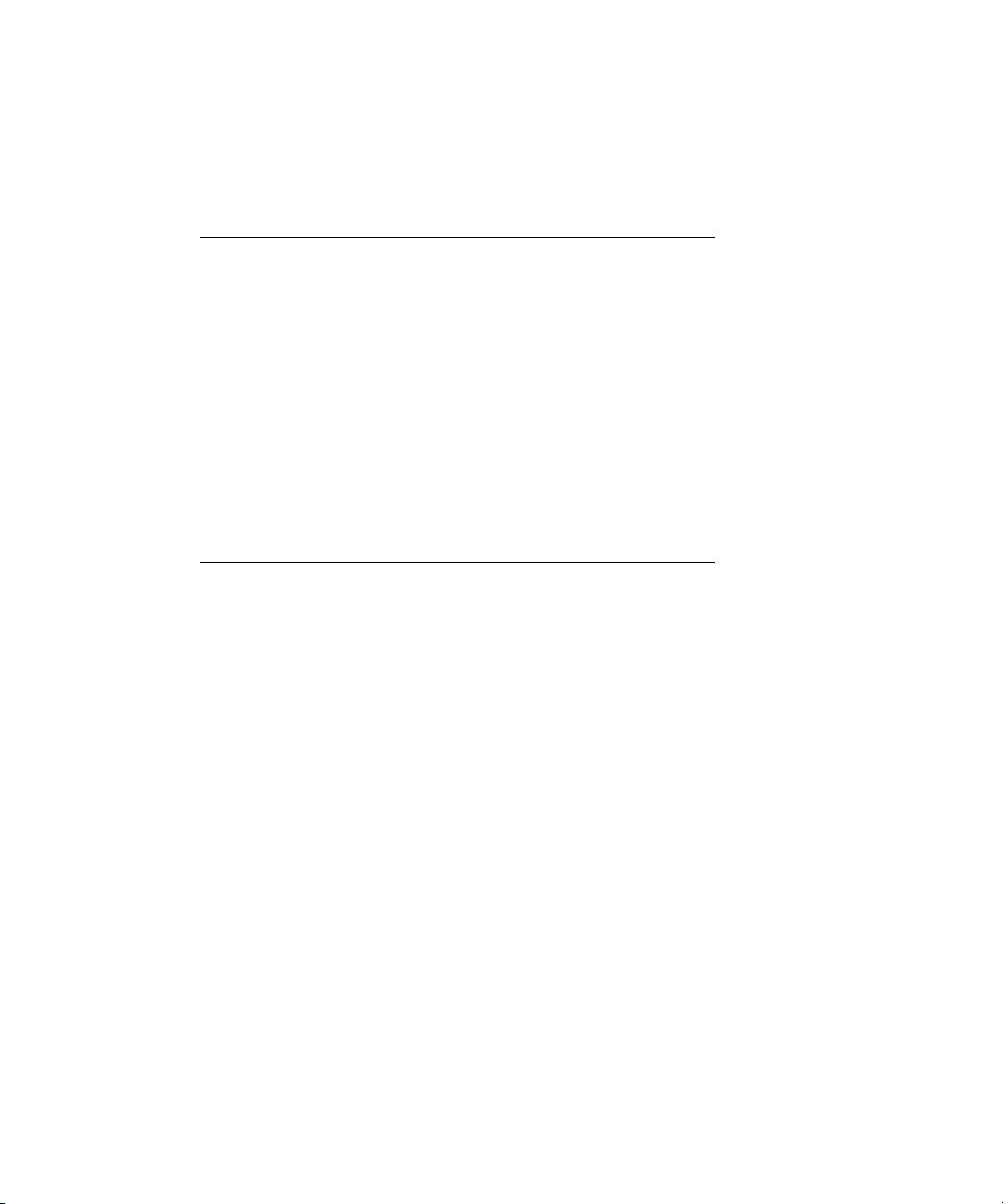
Copyright © 2007 Wind River Systems, Inc.
All rights reserved. No part of this publication may be reproduced or transmitted in any
form or by any means without the prior written permission of Wind River Systems, Inc.
Wind River, Tornado, and VxWorks are registered trademarks of Wind River Systems, Inc.
The Wind River logo is a trademark of Wind River Systems, Inc. Any third-party
trademarks referenced are the property of their respective owners. For further information
regarding Wind River trademarks, please see:
http://www.windriver.com/company/terms/trademark.html
This product may include software licensed to Wind River by third parties. Relevant
notices (if any) are provided in your product installation at the following location:
installDir/product_name/3rd_party_licensor_notice.pdf.
Wind River may refer to third-party documentation by listing publications or providing
links to third-party Web sites for informational purposes. Wind River accepts no
responsibility for the information provided in such third-party documentation.
Corporate Headquarters
Wind River Systems, Inc.
500 Wind River Way
Alameda, CA 94501-1153
U.S.A.
toll free (
U.S.): (800) 545-WIND
telephone: (510) 748-4100
facsimile: (510) 749-2010
For additional contact information, please visit the Wind River URL:
http://www.windriver.com
For information on how to contact Customer Support, please visit the following URL:
http://www.windriver.com/support
VxWorks Application Programmer's Guide, 6.6
14 Nov 07
Part #: DOC-16076-ND-00
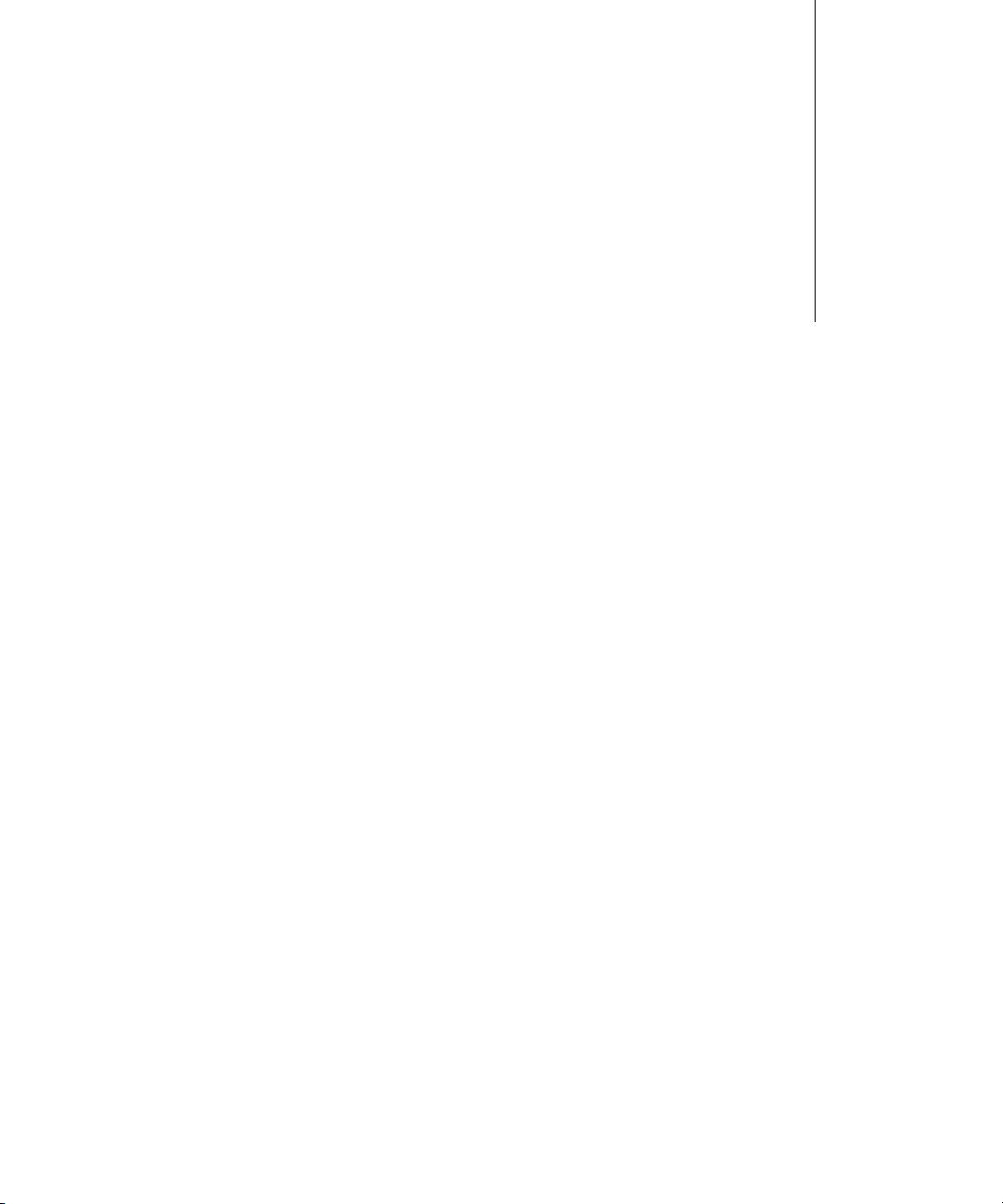
iii
Contents
1 Overview ............................................................................................... 1
1.1 Introduction ............................................................................................................. 1
1.2 Related Documentation Resources ..................................................................... 2
1.3 VxWorks Configuration and Build ..................................................................... 3
2 Applications and Processes ............................................................... 5
2.1 Introduction ............................................................................................................. 6
RTP Applications and VxWorks SMP ................................................... 8
Migrating Kernel Applications to RTP Applications .......................... 8
2.2 Configuring VxWorks For Real-time Processes .............................................. 9
2.3 Real-time Processes ................................................................................................ 11
2.3.1 Real-time Process Life-Cycle .................................................................. 13
2.3.2 Processes and Memory ............................................................................ 14
2.3.3 Processes and Tasks ................................................................................. 16
2.3.4 Processes, Inheritance, and Resource Reclamation ............................. 16
2.3.5 Processes and Environment Variables .................................................. 18
2.3.6 Processes and POSIX ............................................................................... 18
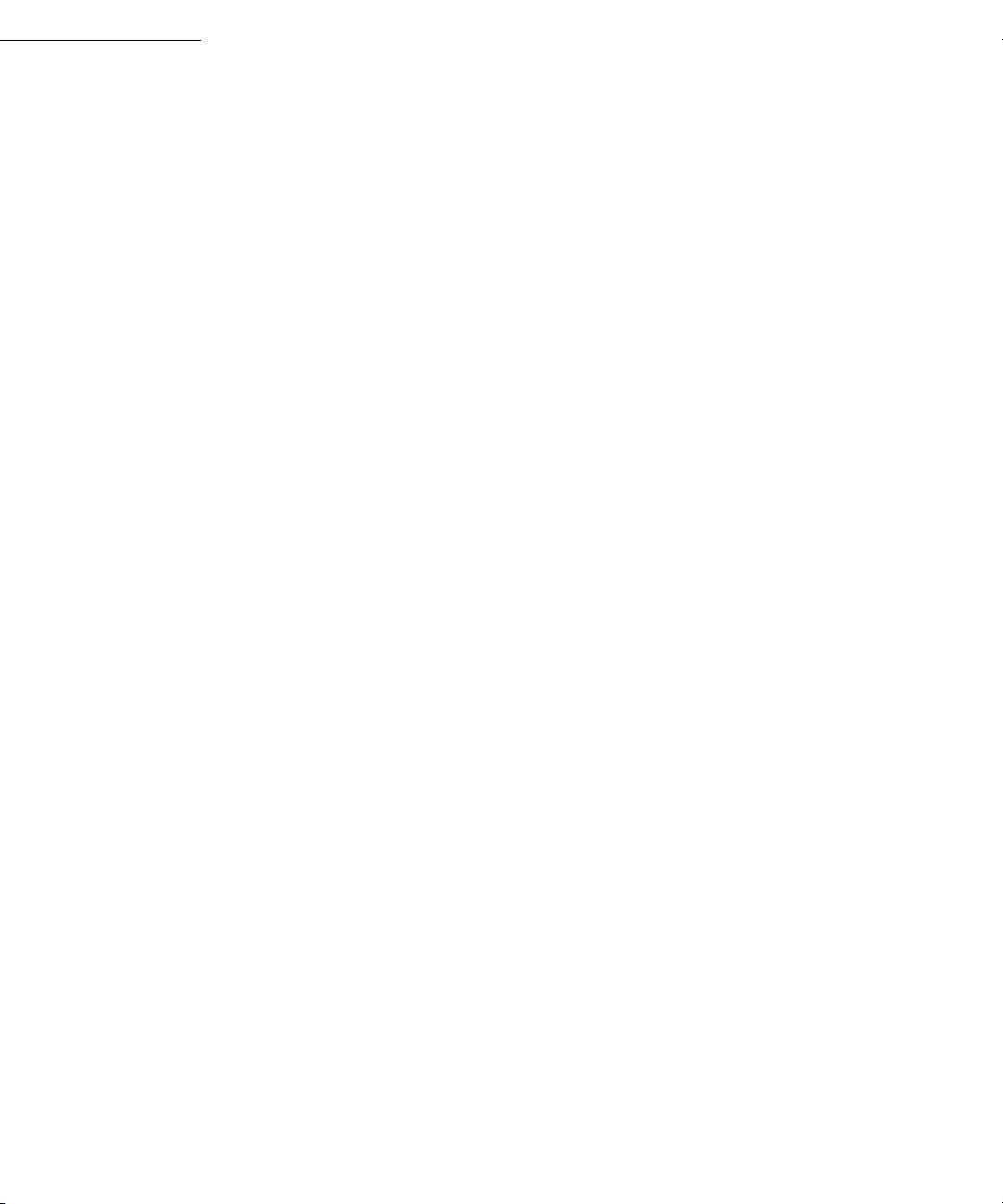
VxWorks
Application Programmer's Guide, 6.6
iv
2.4 POSIX PSE52 Support ........................................................................................... 19
2.5 Developing VxWorks Applications .................................................................... 19
2.5.1 C and C++ Libraries ................................................................................ 19
2.5.2 Application Structure .............................................................................. 20
2.5.3 VxWorks Header Files ............................................................................. 20
2.5.4 Applications, Processes, and Tasks ....................................................... 23
2.5.5 Applications and VxWorks Kernel Component Requirements ........ 24
2.5.6 Building Applications .............................................................................. 25
2.5.7 C++ Applications ..................................................................................... 25
2.5.8 Processes and Hook Routines ................................................................. 26
2.5.9 Application APIs, System Calls, and Library Routines ...................... 26
System Calls .............................................................................................. 26
VxWorks Libraries ................................................................................... 27
API Documentation ................................................................................. 27
2.5.10 POSIX ......................................................................................................... 27
2.6 Developing Application Libraries ...................................................................... 28
2.6.1 Library Initialization ................................................................................ 28
C++ Library Initialization ....................................................................... 29
Handling Initialization Failures ............................................................. 30
2.6.2 Library Termination ................................................................................. 30
Using atexit() for Termination Routines ............................................... 31
2.6.3 Developing Static Libraries ..................................................................... 31
2.6.4 Developing Shared Libraries .................................................................. 32
Configuring VxWorks for Shared Libraries ......................................... 36
Building Shared Libraries and Dynamic Applications ....................... 37
VxWorks Run-time C Library libc.so .................................................... 51
Using Plug-Ins .......................................................................................... 52
Using readelf to Examine Dynamic ELF Files ...................................... 55
Getting Runtime Information About Shared Libraries ....................... 55
Debugging Shared Libraries ................................................................... 59
Working With Shared Libraries From a Windows Host .................... 59
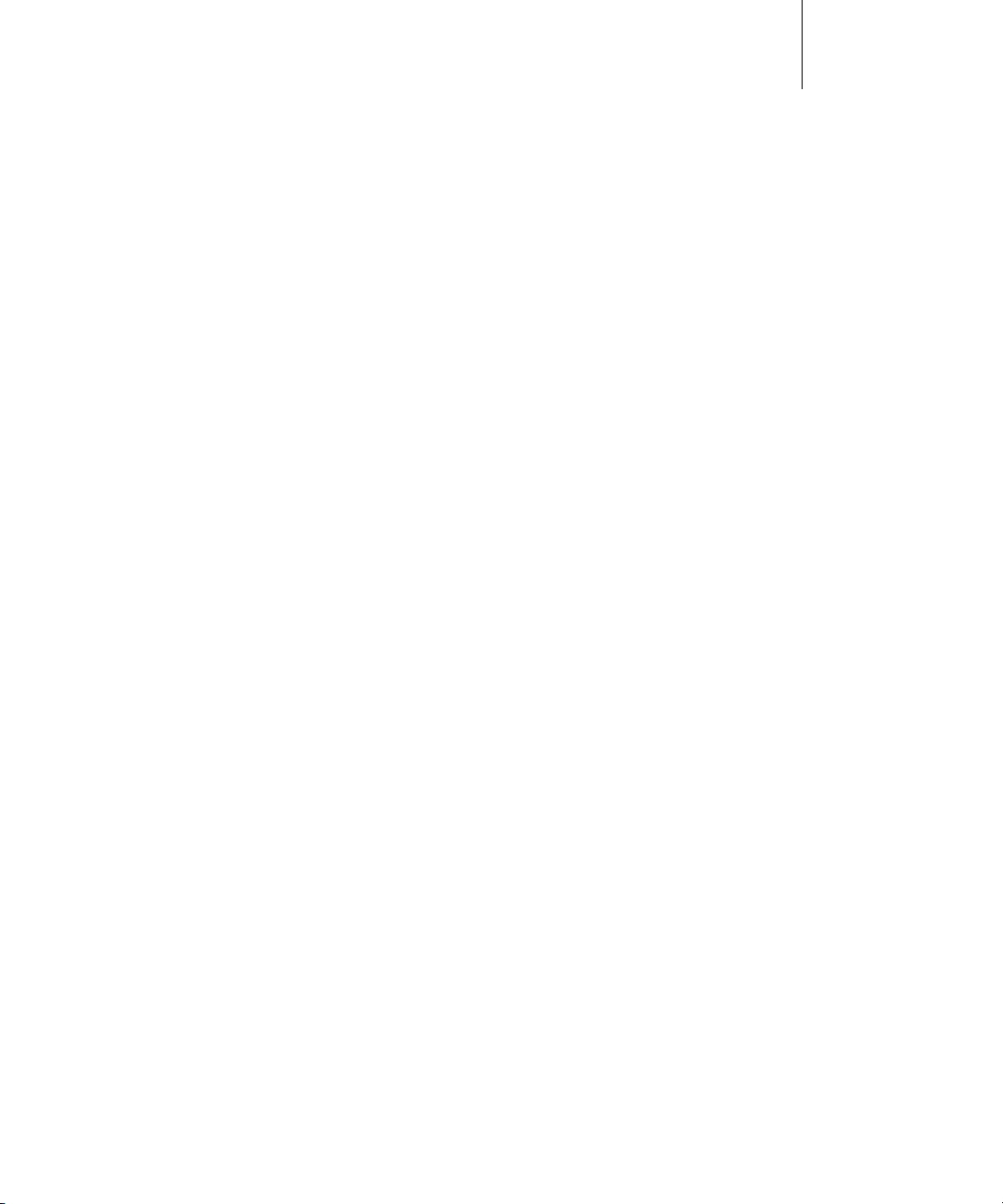
Contents
v
2.7 Creating and Managing Shared Data Regions ................................................. 61
2.7.1 Configuring VxWorks for Shared Data Regions ................................. 62
2.7.2 Creating Shared Data Regions ............................................................... 62
2.7.3 Accessing Shared Data Regions ............................................................. 63
2.7.4 Deleting Shared Data Regions ................................................................ 64
2.8 Executing Applications ......................................................................................... 64
2.8.1 Running Applications Interactively ...................................................... 65
Starting Applications ............................................................................... 65
Stopping Applications ............................................................................. 66
2.8.2 Running Applications Automatically ................................................... 67
Startup Facility Options .......................................................................... 68
Application Startup String Syntax ......................................................... 68
Specifying Applications with a Startup Configuration Parameter ... 70
Specifying Applications with a Boot Loader Parameter .................... 70
Specifying Applications with a VxWorks Shell Script ........................ 71
Specifying Applications with usrRtpAppInit( ) ................................. 72
2.8.3 Applications and Symbol Registration ................................................. 73
2.9 Bundling Applications with a VxWorks System using ROMFS .................. 74
2.9.1 Configuring VxWorks with ROMFS ..................................................... 75
2.9.2 Building a System With ROMFS and Applications ............................ 75
2.9.3 Accessing Files in ROMFS ...................................................................... 76
2.9.4 Using ROMFS to Start Applications Automatically ........................... 76
3 Multitasking .......................................................................................... 77
3.1 Introduction ............................................................................................................. 79
3.2 Tasks and Multitasking ....................................................................................... 80
3.2.1 Task States and Transitions .................................................................... 81
3.3 Task Scheduling ..................................................................................................... 85
3.3.1 Task Priorities ........................................................................................... 85