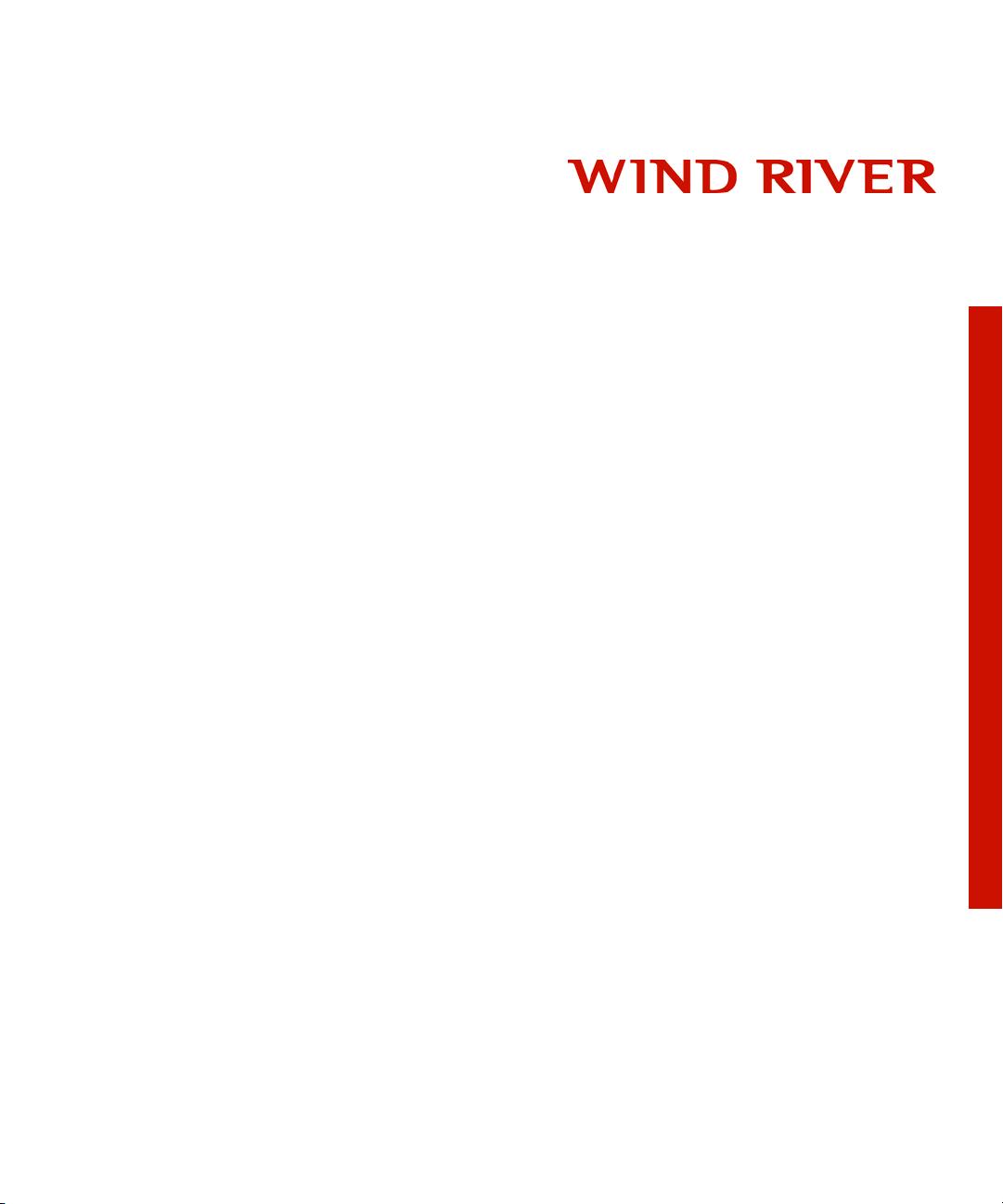
VxWorks
DEVICE DRIVER DEVELOPER’S GUIDE
®
6.4
VxWorks Device Driver Developer's Guide
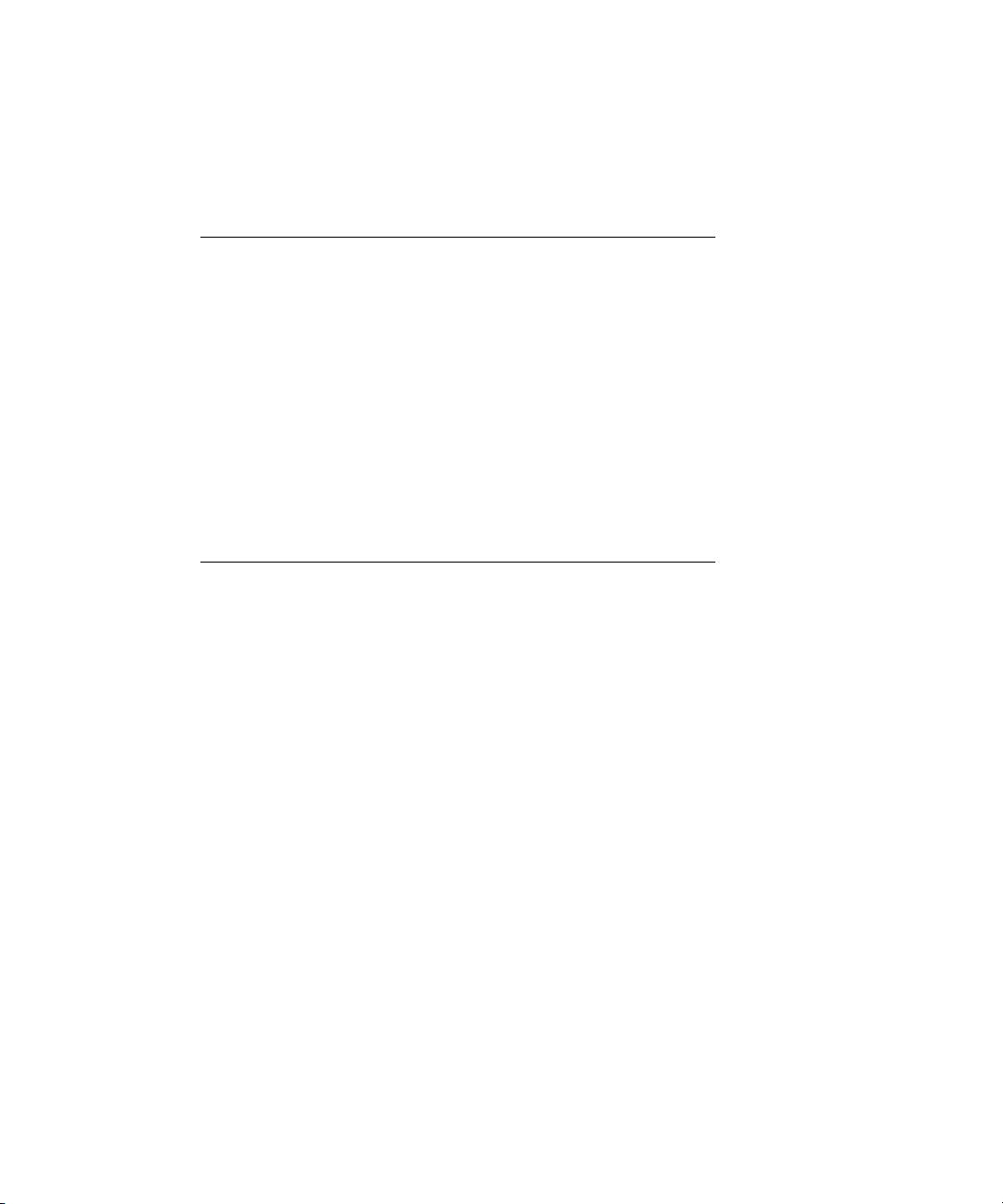
Copyright © 2006 Wind River Systems, Inc.
All rights reserved. No part of this publication may be reproduced or transmitted in any
form or by any means without the prior written permission of Wind River Systems, Inc.
Wind River, the Wind River logo, Tornado, and VxWorks are registered trademarks of
Wind River Systems, Inc. Any third-party trademarks referenced are the property of their
respective owners. For further information regarding Wind River trademarks, please see:
http://www.windriver.com/company/terms/trademark.html
This product may include software licensed to Wind River by third parties. Relevant
notices (if any) are provided in your product installation at the following location:
installDir/product_name/3rd_party_licensor_notice.pdf.
Wind River may refer to third-party documentation by listing publications or providing
links to third-party Web sites for informational purposes. Wind River accepts no
responsibility for the information provided in such third-party documentation.
Corporate Headquarters
Wind River Systems, Inc.
500 Wind River Way
Alameda, CA 94501-1153
U.S.A.
toll free (
U.S.): (800) 545-WIND
telephone: (510) 748-4100
facsimile: (510) 749-2010
For additional contact information, please visit the Wind River URL:
http://www.windriver.com
For information on how to contact Customer Support, please visit the following URL:
http://www.windriver.com/support
VxWorks Device Driver Developer’s Guide, 6.4
26 Sep 06
Part #: DOC-15882-ND-00
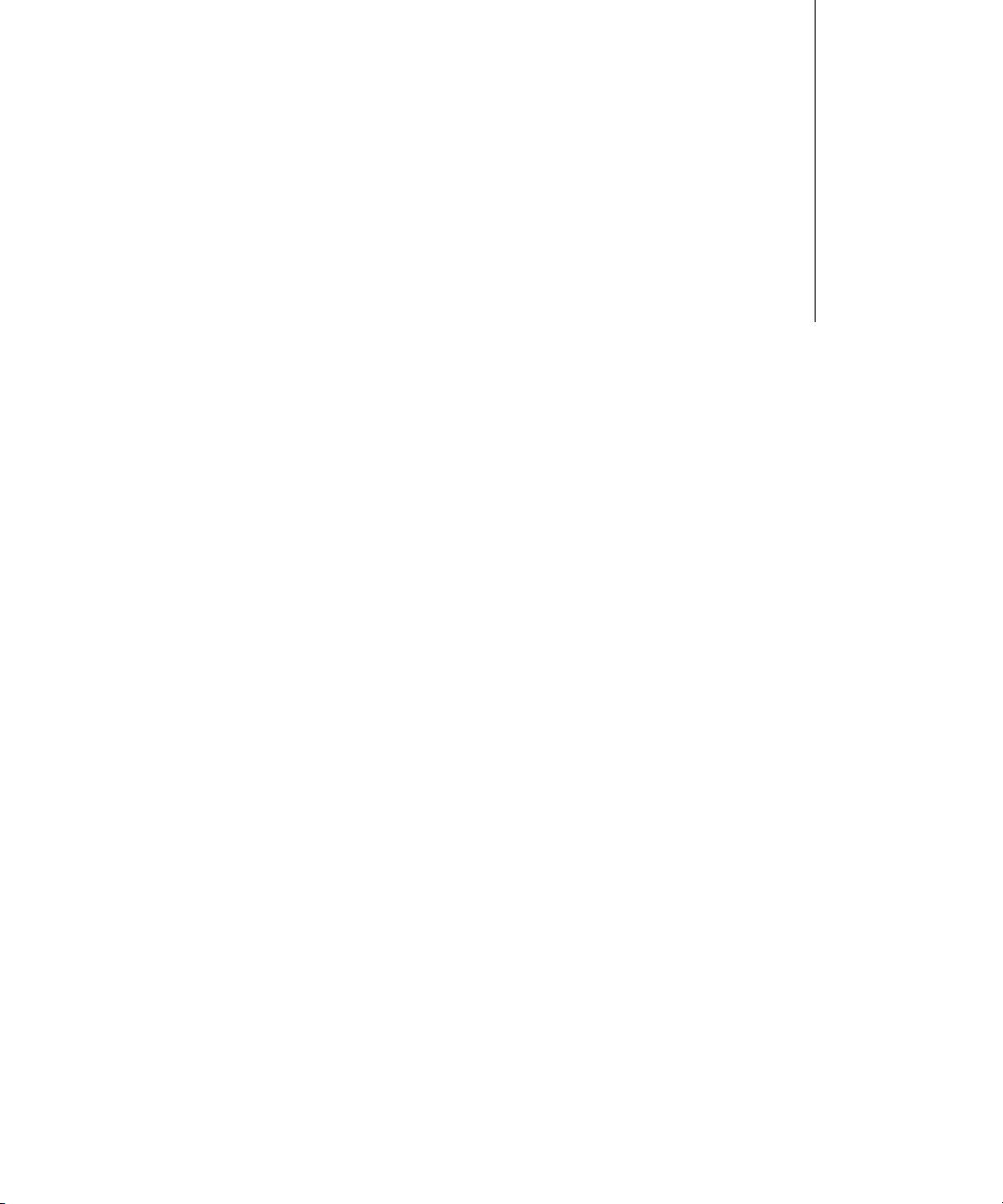
iii
Contents
1 Introduction .......................................................................................... 1
1.1 About This Document ........................................................................................... 1
1.2 Additional Documentation .................................................................................. 2
1.3 Terminology ............................................................................................................. 3
2 Design Overview .................................................................................. 5
2.1 Introduction ............................................................................................................. 5
2.2 Design Goals ........................................................................................................... 5
2.2.1 Performance .............................................................................................. 6
2.2.2 Code Flexibility and Portability ............................................................. 6
2.2.3 Maintenance and Readability ................................................................. 7
2.2.4 Ease of Configuration .............................................................................. 7
2.2.5 Performance Testing ................................................................................ 7
2.2.6 Code Size ................................................................................................... 8
2.2.7 Reentrancy and Number of Devices ...................................................... 8
2.3 Common Design Concerns ................................................................................... 8
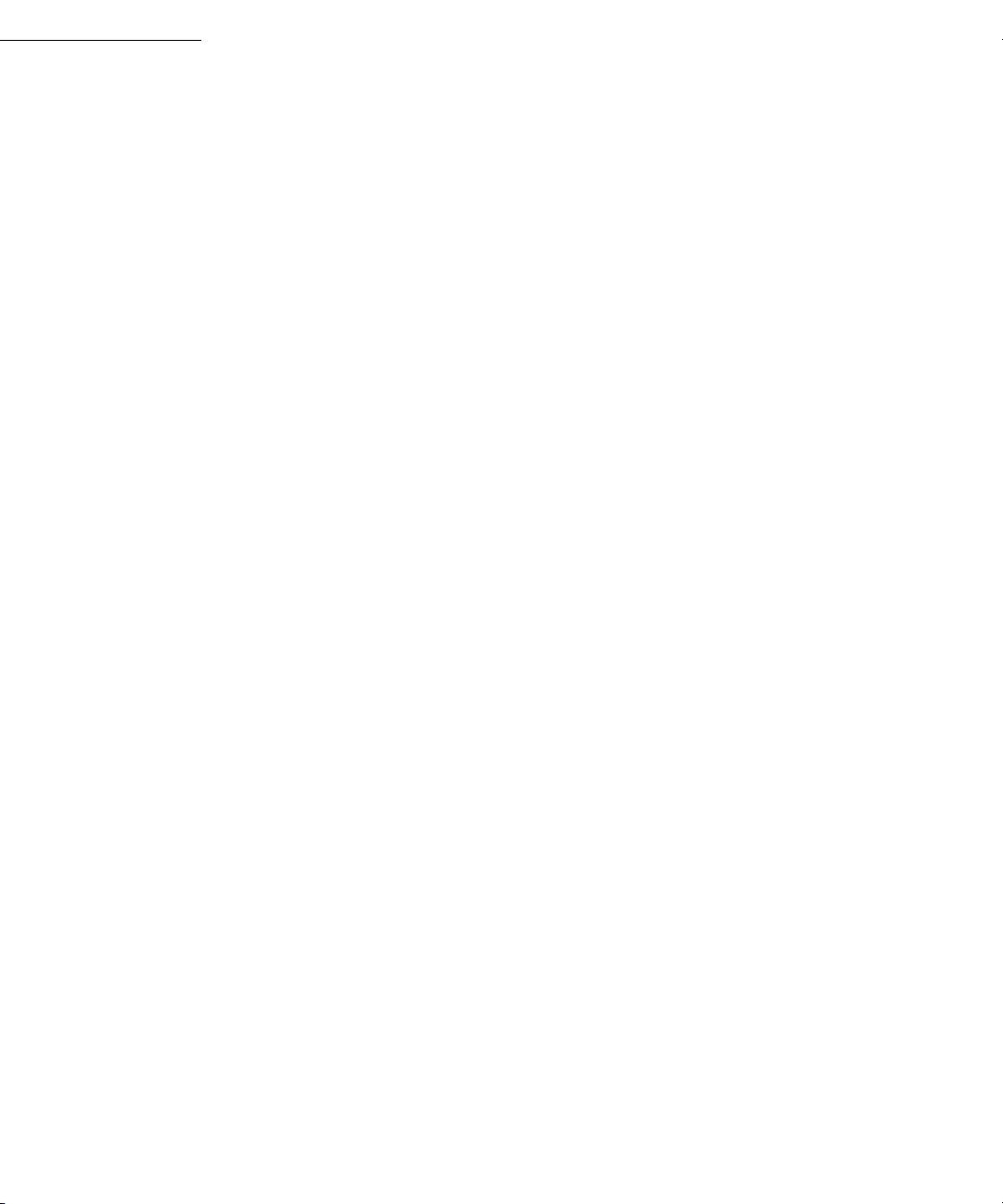
VxWorks
Device Driver Developer’s Guide, 6.4
iv
2.3.1 Varied Hardware Designs ....................................................................... 9
2.3.2 Memory-Mapped Chips .......................................................................... 9
2.3.3 I/O-Mapped Chips .................................................................................. 9
2.3.4 Multi-Function Chips .............................................................................. 10
2.3.5 Bus Support ............................................................................................... 10
VxBus-Compliant Drivers ....................................................................... 11
Legacy Drivers .......................................................................................... 11
VxBus Bus Controller Device Drivers ................................................... 12
2.3.6 Interrupt Controllers ................................................................................ 12
2.4 Design Guidelines ................................................................................................. 12
2.4.1 Names and Locations .............................................................................. 13
2.4.2 Documentation and Standards ............................................................... 13
2.4.3 Per-Device Data Structure ....................................................................... 15
2.4.4 Per-Driver Data Structure ....................................................................... 15
2.4.5 Driver Interrupt Service Routines .......................................................... 15
2.4.6 Access Macros ........................................................................................... 16
2.4.7 Multi-Function Devices and VxBus ....................................................... 18
3 Adding Drivers to VxWorks ................................................................. 19
3.1 Adding an Existing Driver to Your BSP ............................................................. 19
3.1.1 BSP Support for Legacy (Non-VxBus) Device Drivers ....................... 20
3.1.2 Project Facility ........................................................................................... 20
3.1.3 Component Descriptor Files ................................................................... 21
3.2 Porting Drivers ........................................................................................................ 21
3.2.1 Porting a Driver From An Earlier Version of VxWorks ...................... 21
3.2.2 Porting a Legacy Driver to the VxBus Model ...................................... 21
3.2.3 Porting From Another OS ....................................................................... 22
3.3 Creating a New Driver Using the Legacy Model ............................................. 23
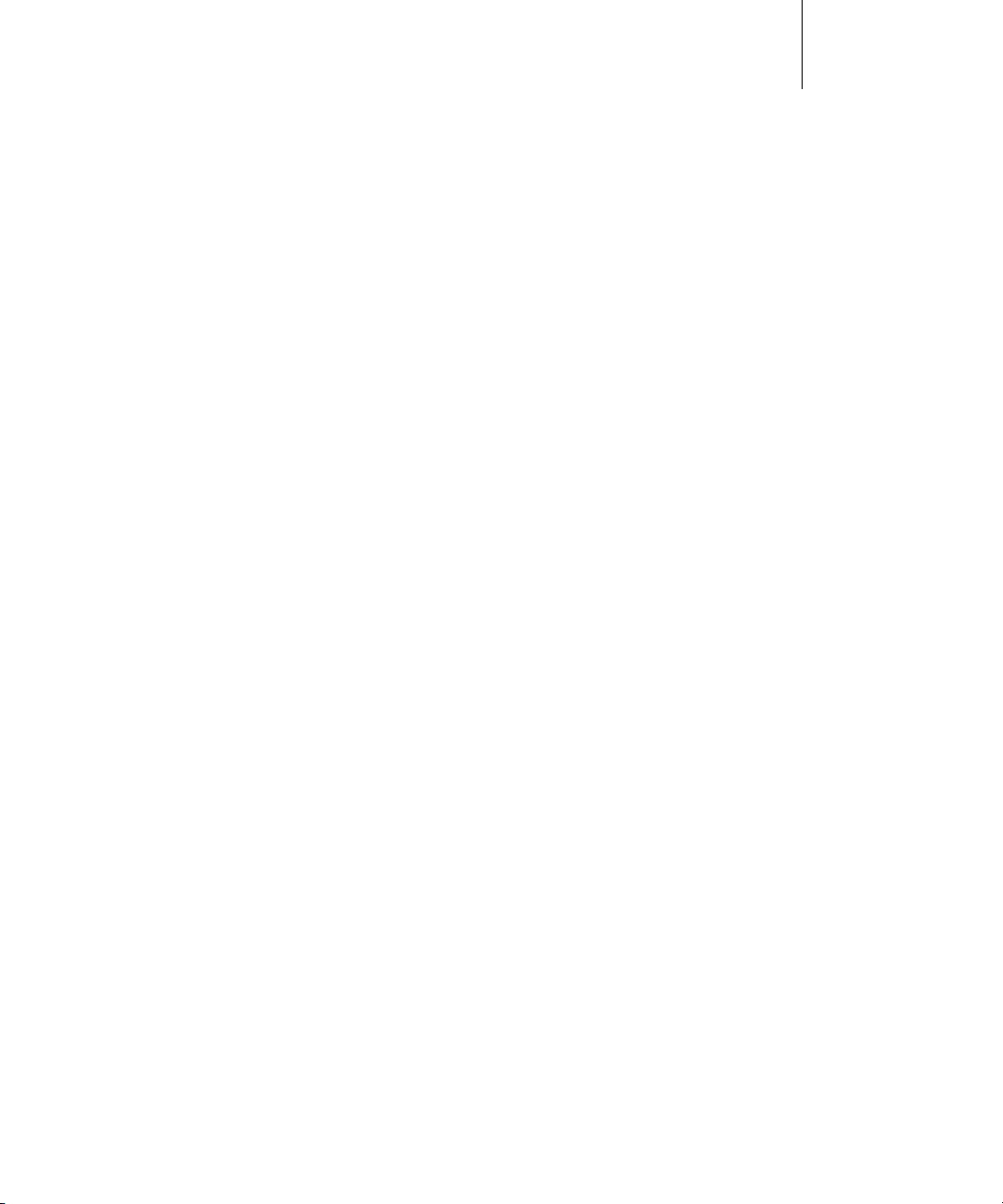
Contents
v
3.3.1 Step-By-Step Instructions ........................................................................ 23
Top-Down Design .................................................................................... 23
Bottom-Up Implementation ................................................................... 25
Helpful Hints ............................................................................................ 26
3.3.2 Cache Considerations .............................................................................. 26
Using the Cache Library .......................................................................... 27
Developing a cacheLib Strategy ............................................................. 31
3.3.3 Hotswap Support ..................................................................................... 35
3.3.4 Downloadable Driver Support ............................................................... 35
4 END Ethernet Drivers ........................................................................... 37
4.1 Introduction ............................................................................................................. 37
4.2 END Driver Overview ........................................................................................... 38
4.2.1 Driver Environment ................................................................................. 38
The MUX ................................................................................................... 38
Network Interface Drivers and Protocols ............................................. 39
The MUX, Protocol, and Driver API ..................................................... 40
Driver Components ................................................................................. 42
Protocols That Use the MUX API .......................................................... 43
Interactions With the MUX API ............................................................. 47
Network Layer to Data Link Layer Address Resolution .................... 53
4.2.2 VxWorks OS Interface .............................................................................. 54
Understanding How VxWorks Launches and Uses Your Driver ...... 54
Executing Calls Waiting In the Network Job Queue ........................... 58
Adding Your Network Interface Driver to VxWorks .......................... 59
Allocating, Initializing, and Utilizing Memory Resources ................. 61
Handling Packet Reception .................................................................... 69
Handling Packet Transmission ............................................................... 82
Implementing Checksum Offloading .................................................... 88
Implementing Required Entry Points and Structures ......................... 95
4.3 The END Driver Development Process ............................................................. 118