Part 3:GridWorld Classes and Interfaces - 2015年软件工程实训 - SoYa Wik
在GridWorld中,我们主要关注的是几个核心的类和接口,它们构成了这个模拟环境的基础。GridWorld是一个用于教学目的的编程环境,它允许用户创建和交互复杂的网格状系统,其中包含各种活动对象,如Actor。在Part 3:GridWorld Classes and Interfaces中,我们将深入探讨这些关键组件。 `Actor`类是所有GridWorld中演员对象的基类。用户通常会创建继承自`Actor`的自定义类来表示他们想要的行为和外观。每个`Actor`都有一个位置和一个方向,这两个属性是通过`Location`类和`Grid`接口来管理的。 `Location`类封装了网格中演员位置的坐标。它不仅存储了行(row)和列(column)值,还提供了判断不同位置间关系的方法,例如判断两个位置是否相邻或者在特定的 compass 方向上。`Location`类还定义了8个常量来表示指南针上的方向,例如`NORTH`、`EAST`、`SOUTH`和`WEST`,以及45度角的偏转值,如`NORTHEAST`、`SOUTHEAST`等。此外,还有表示常见转角的常量,如`HALF_RIGHT`,这使得可以方便地计算演员转向的角度。 `Grid`接口是表示网格结构的核心,有两个实现类:`BoundedGrid`和`UnboundedGrid`。`BoundedGrid`用于表示有边界的网格,即演员不能超出网格边界;而`UnboundedGrid`则允许演员在无限大的网格中移动。这两个类都提供了添加、移除和获取网格中演员的方法,以及检查位置是否有效、移动演员等操作。 在GridWorld中,演员不仅知道自己的位置,也知道自己所在的网格。这意味着每个`Actor`对象都有指向其所在`Grid`的引用,以及表示其方向的整数值。演员的方向可以通过加减上述的转角常量来改变,例如,要使演员向右转90度,只需将当前方向加上`EAST`的值即可。 在实际编程中,用户可能会创建自己的`Actor`子类,并重写`act()`方法来定义演员的行为。例如,可以编写一个`Bee`类,使其在每次`act()`时随机移动或按照某种模式移动。`Actor`还可以有自己的图形表示,通过`DisplayInfo`类来定义显示的图像和颜色。 GridWorld提供了一个结构化的框架,用于学习面向对象编程、多线程以及复杂系统中的互动。通过理解`Actor`、`Location`、`Grid`以及它们之间的关系,开发者能够构建出各种有趣且动态的网格世界。在实训中,学生可以结合这些基础知识,编写自己的GridWorld程序,提高编程技能并体验到游戏化学习的乐趣。
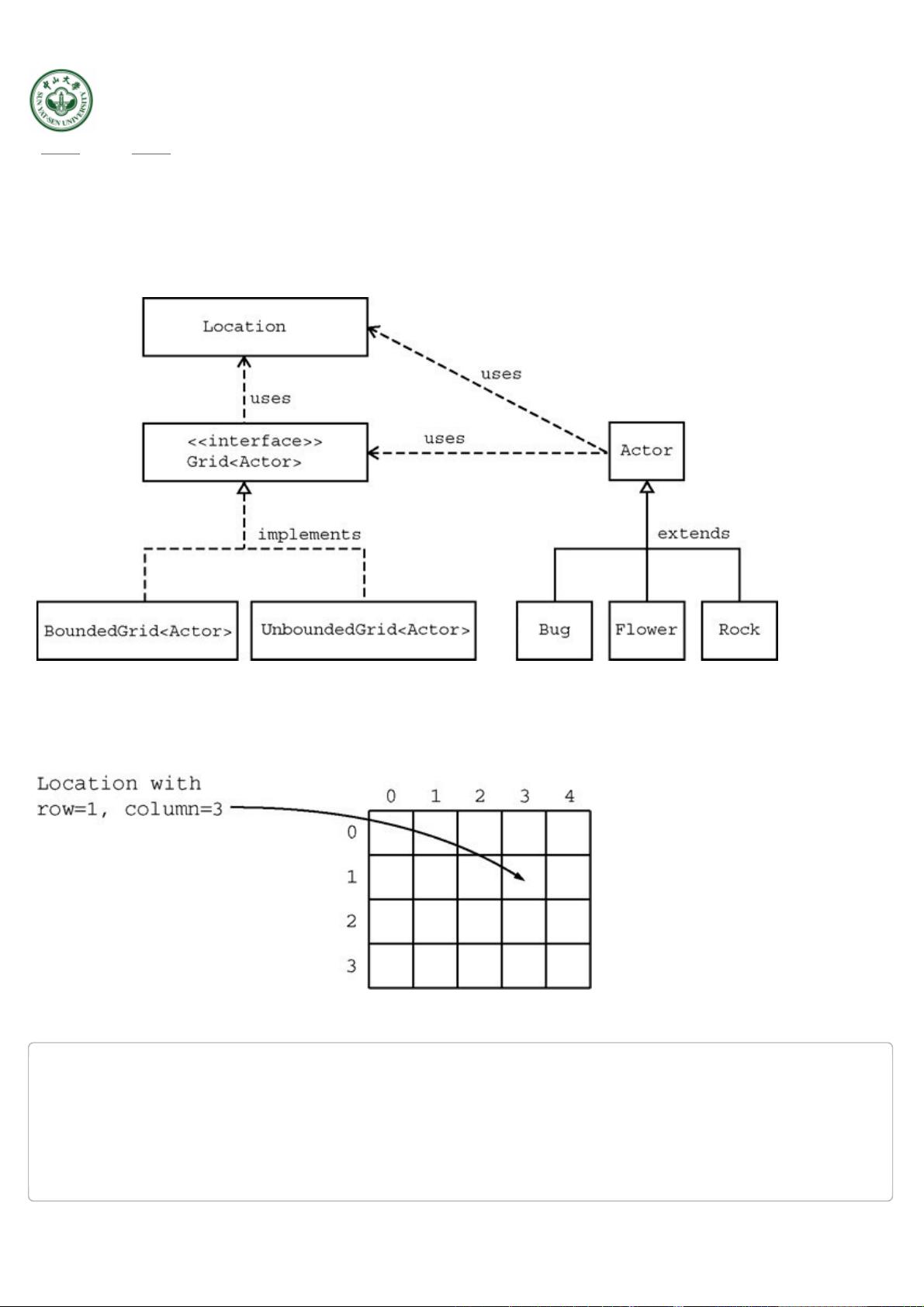
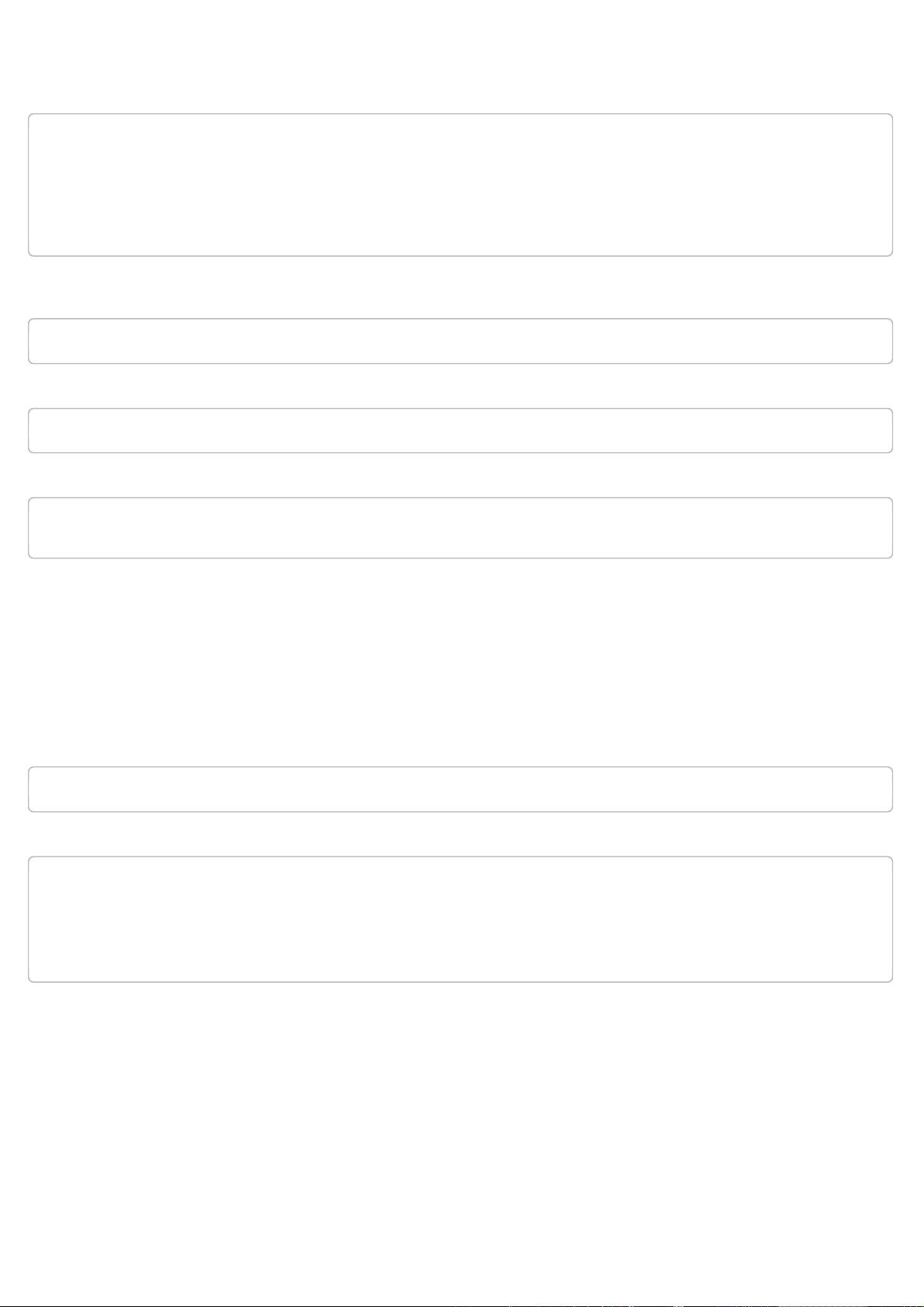
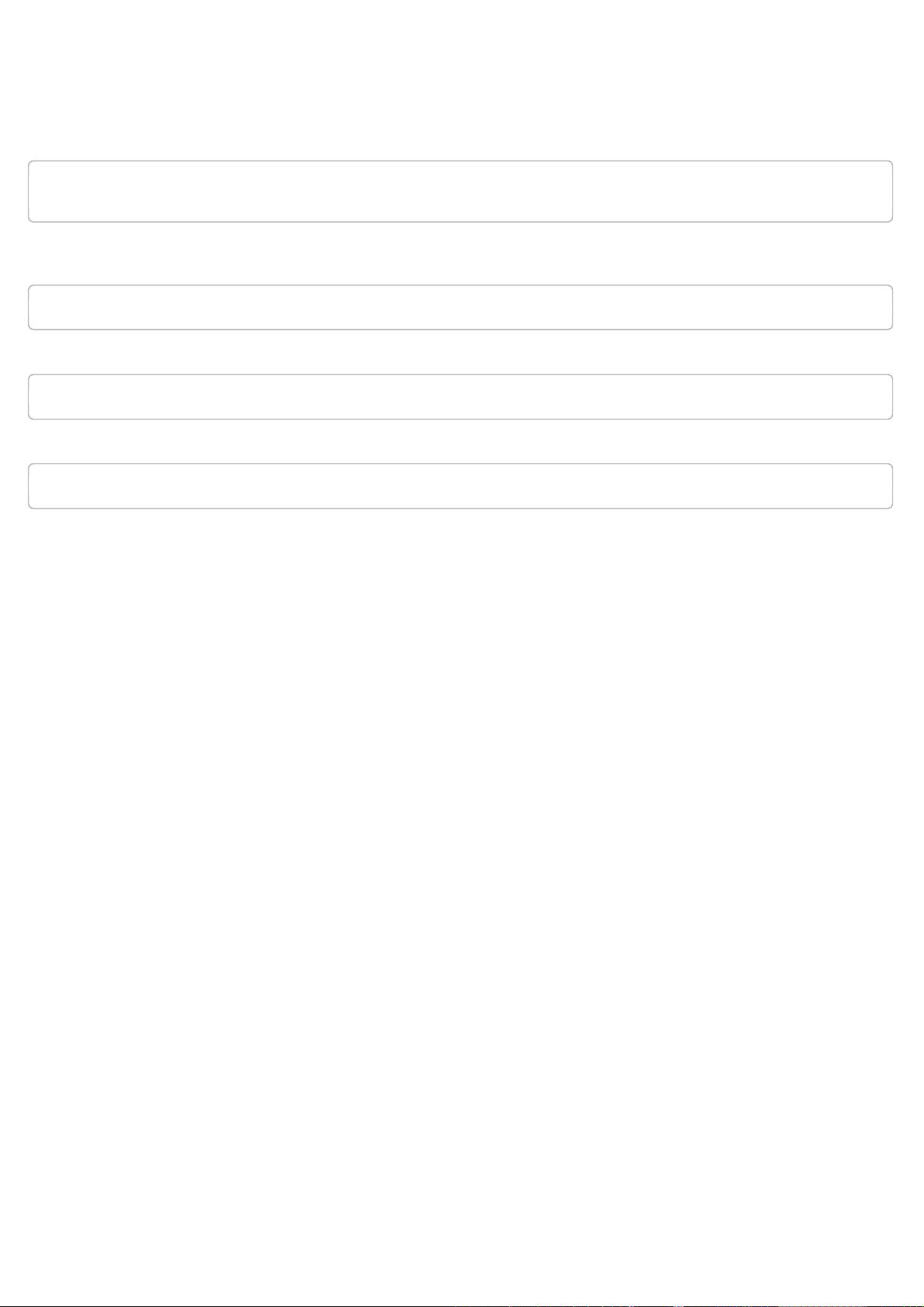
剩余14页未读,继续阅读
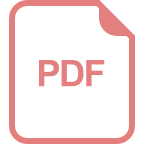
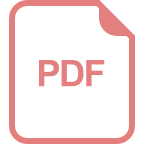
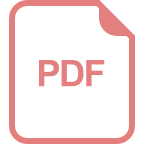
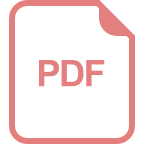
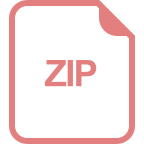
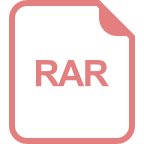
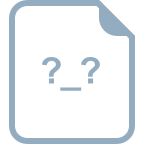
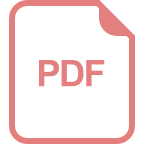
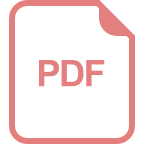
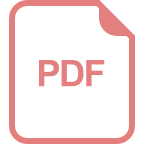
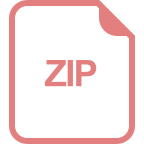
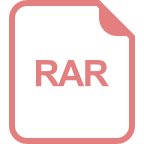
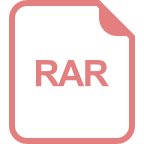
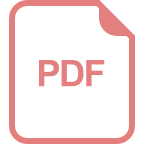
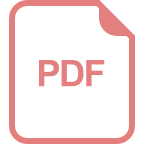
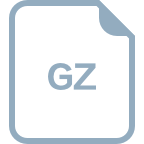
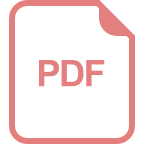
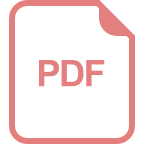
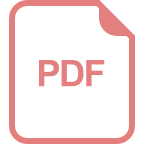
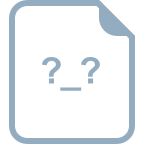
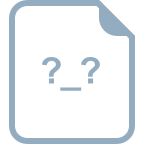
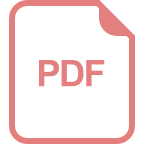
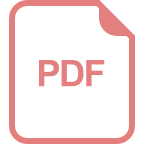
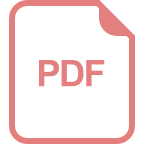
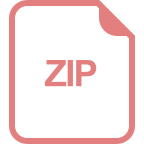

- 粉丝: 25
- 资源: 297
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

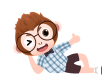
最新资源
- 从XML生成可与Ajax共同使用的JSON中文WORD版最新版本
- silverlight通过WebService连接数据库中文WORD版最新版本
- 使用NetBeans连接SQLserver2008数据库教程中文WORD版最新版本
- XPath实例中文WORD版最新版本
- XPath语法规则中文WORD版最新版本
- XPath入门教程中文WORD版最新版本
- ORACLE数据库管理系统体系结构中文WORD版最新版本
- Sybase数据库安装以及新建数据库中文WORD版最新版本
- tomcat6.0配置oracle数据库连接池中文WORD版最新版本
- hibernate连接oracle数据库中文WORD版最新版本

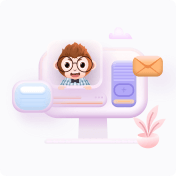

评论0