<?xml version="1.0"?>
<doc>
<assembly>
<name>SixLabors.ImageSharp</name>
</assembly>
<members>
<member name="T:SixLabors.ImageSharp.Advanced.AdvancedImageExtensions">
<summary>
Extension methods over Image{TPixel}
</summary>
</member>
<member name="M:SixLabors.ImageSharp.Advanced.AdvancedImageExtensions.DetectEncoder(SixLabors.ImageSharp.Image,System.String)">
<summary>
For a given file path find the best encoder to use via its extension.
</summary>
<param name="source">The source image.</param>
<param name="filePath">The target file path to save the image to.</param>
<exception cref="T:System.ArgumentNullException">The file path is null.</exception>
<exception cref="T:System.NotSupportedException">No encoder available for provided path.</exception>
<returns>The matching <see cref="T:SixLabors.ImageSharp.Formats.IImageEncoder"/>.</returns>
</member>
<member name="M:SixLabors.ImageSharp.Advanced.AdvancedImageExtensions.AcceptVisitor(SixLabors.ImageSharp.Image,SixLabors.ImageSharp.Advanced.IImageVisitor)">
<summary>
Accepts a <see cref="T:SixLabors.ImageSharp.Advanced.IImageVisitor"/> to implement a double-dispatch pattern in order to
apply pixel-specific operations on non-generic <see cref="T:SixLabors.ImageSharp.Image"/> instances
</summary>
<param name="source">The source image.</param>
<param name="visitor">The image visitor.</param>
</member>
<member name="M:SixLabors.ImageSharp.Advanced.AdvancedImageExtensions.AcceptVisitorAsync(SixLabors.ImageSharp.Image,SixLabors.ImageSharp.Advanced.IImageVisitorAsync,System.Threading.CancellationToken)">
<summary>
Accepts a <see cref="T:SixLabors.ImageSharp.Advanced.IImageVisitor"/> to implement a double-dispatch pattern in order to
apply pixel-specific operations on non-generic <see cref="T:SixLabors.ImageSharp.Image"/> instances
</summary>
<param name="source">The source image.</param>
<param name="visitor">The image visitor.</param>
<param name="cancellationToken">The token to monitor for cancellation requests.</param>
<returns>A <see cref="T:System.Threading.Tasks.Task"/> representing the asynchronous operation.</returns>
</member>
<member name="M:SixLabors.ImageSharp.Advanced.AdvancedImageExtensions.GetConfiguration(SixLabors.ImageSharp.Image)">
<summary>
Gets the configuration for the image.
</summary>
<param name="source">The source image.</param>
<returns>Returns the configuration.</returns>
</member>
<member name="M:SixLabors.ImageSharp.Advanced.AdvancedImageExtensions.GetConfiguration(SixLabors.ImageSharp.ImageFrame)">
<summary>
Gets the configuration for the image frame.
</summary>
<param name="source">The source image.</param>
<returns>Returns the configuration.</returns>
</member>
<member name="M:SixLabors.ImageSharp.Advanced.AdvancedImageExtensions.GetConfiguration(SixLabors.ImageSharp.Advanced.IConfigurationProvider)">
<summary>
Gets the configuration.
</summary>
<param name="source">The source image</param>
<returns>Returns the bounds of the image</returns>
</member>
<member name="M:SixLabors.ImageSharp.Advanced.AdvancedImageExtensions.GetPixelMemoryGroup``1(SixLabors.ImageSharp.ImageFrame{``0})">
<summary>
Gets the representation of the pixels as a <see cref="T:SixLabors.ImageSharp.Memory.IMemoryGroup`1"/> containing the backing pixel data of the image
stored in row major order, as a list of contiguous <see cref="T:System.Memory`1"/> blocks in the source image's pixel format.
</summary>
<param name="source">The source image.</param>
<typeparam name="TPixel">The type of the pixel.</typeparam>
<returns>The <see cref="T:SixLabors.ImageSharp.Memory.IMemoryGroup`1"/>.</returns>
<remarks>
Certain Image Processors may invalidate the returned <see cref="T:SixLabors.ImageSharp.Memory.IMemoryGroup`1"/> and all it's buffers,
therefore it's not recommended to mutate the image while holding a reference to it's <see cref="T:SixLabors.ImageSharp.Memory.IMemoryGroup`1"/>.
</remarks>
</member>
<member name="M:SixLabors.ImageSharp.Advanced.AdvancedImageExtensions.GetPixelMemoryGroup``1(SixLabors.ImageSharp.Image{``0})">
<summary>
Gets the representation of the pixels as a <see cref="T:SixLabors.ImageSharp.Memory.IMemoryGroup`1"/> containing the backing pixel data of the image
stored in row major order, as a list of contiguous <see cref="T:System.Memory`1"/> blocks in the source image's pixel format.
</summary>
<param name="source">The source image.</param>
<typeparam name="TPixel">The type of the pixel.</typeparam>
<returns>The <see cref="T:SixLabors.ImageSharp.Memory.IMemoryGroup`1"/>.</returns>
<remarks>
Certain Image Processors may invalidate the returned <see cref="T:SixLabors.ImageSharp.Memory.IMemoryGroup`1"/> and all it's buffers,
therefore it's not recommended to mutate the image while holding a reference to it's <see cref="T:SixLabors.ImageSharp.Memory.IMemoryGroup`1"/>.
</remarks>
</member>
<member name="M:SixLabors.ImageSharp.Advanced.AdvancedImageExtensions.DangerousGetPixelRowMemory``1(SixLabors.ImageSharp.ImageFrame{``0},System.Int32)">
<summary>
Gets the representation of the pixels as a <see cref="T:System.Span`1"/> of contiguous memory
at row <paramref name="rowIndex"/> beginning from the the first pixel on that row.
</summary>
<typeparam name="TPixel">The type of the pixel.</typeparam>
<param name="source">The source.</param>
<param name="rowIndex">The row.</param>
<returns>The <see cref="T:System.Span`1"/></returns>
</member>
<member name="M:SixLabors.ImageSharp.Advanced.AdvancedImageExtensions.DangerousGetPixelRowMemory``1(SixLabors.ImageSharp.Image{``0},System.Int32)">
<summary>
Gets the representation of the pixels as <see cref="T:System.Span`1"/> of of contiguous memory
at row <paramref name="rowIndex"/> beginning from the the first pixel on that row.
</summary>
<typeparam name="TPixel">The type of the pixel.</typeparam>
<param name="source">The source.</param>
<param name="rowIndex">The row.</param>
<returns>The <see cref="T:System.Span`1"/></returns>
</member>
<member name="M:SixLabors.ImageSharp.Advanced.AdvancedImageExtensions.GetMemoryAllocator(SixLabors.ImageSharp.Advanced.IConfigurationProvider)">
<summary>
Gets the <see cref="T:SixLabors.ImageSharp.Memory.MemoryAllocator"/> assigned to 'source'.
</summary>
<param name="source">The source image.</param>
<returns>Returns the configuration.</returns>
</member>
<member name="T:SixLabors.ImageSharp.Advanced.AotCompilerTools">
<summary>
Unlike traditional Mono/.NET, code on the iPhone is statically compiled ahead of time instead of being
compiled on demand by a JIT compiler. This means there are a few limitations with respect to generics,
these are caused because not every possible generic instantiation can be determined up front at compile time.
The Aot Compiler is designed to overc
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
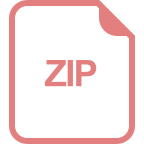
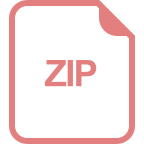
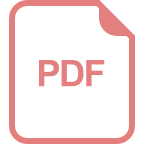
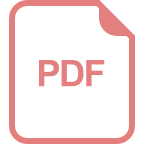
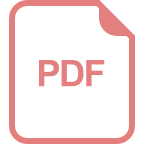
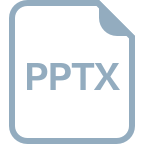
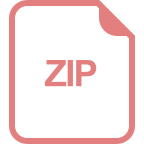
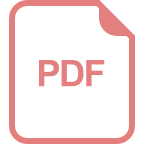
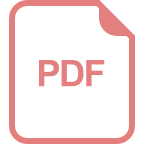
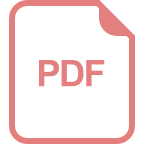
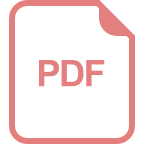
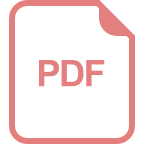
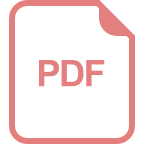
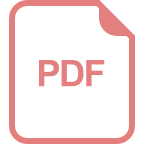
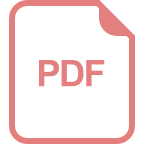
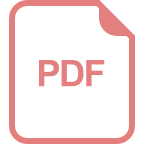
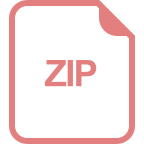
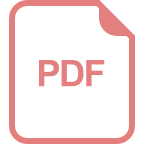
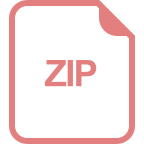
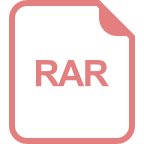
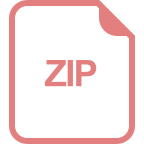
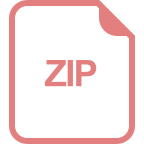
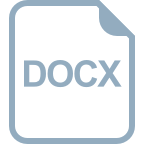
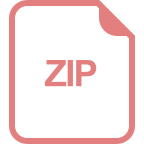
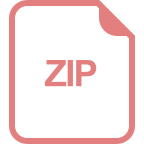
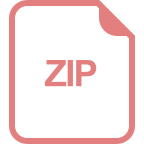
收起资源包目录

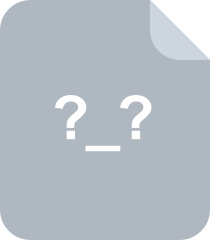
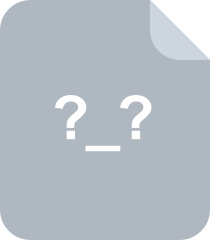
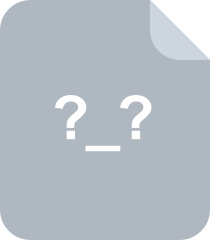
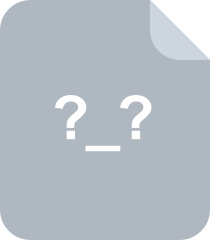
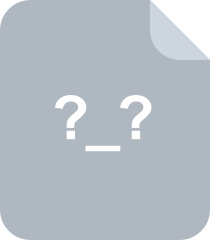
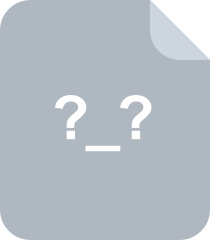
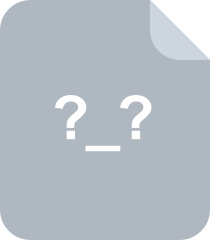
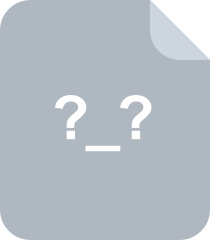
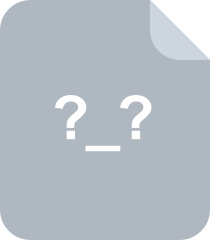
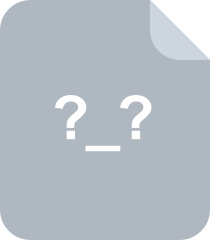
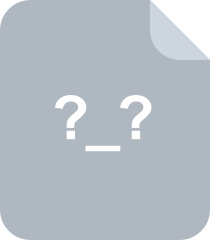
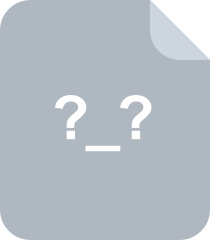
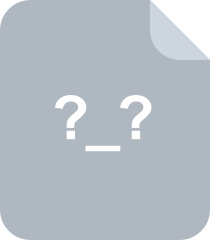
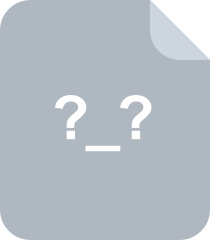
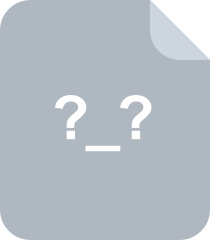
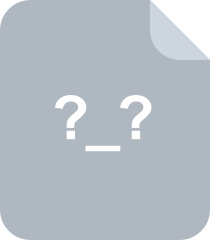
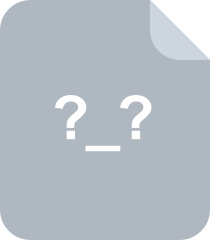
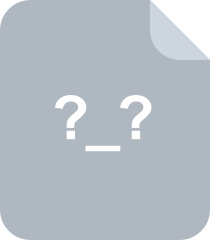
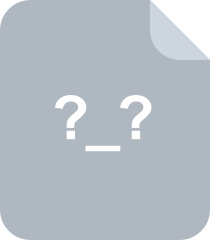
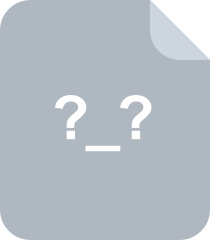
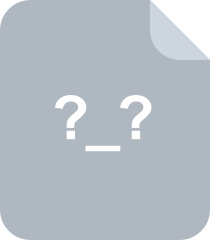
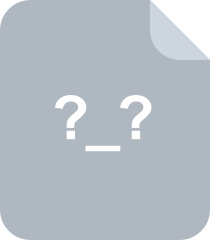
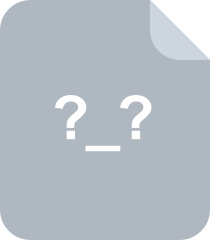
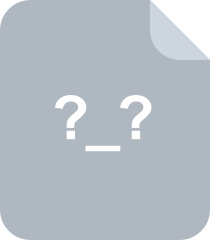
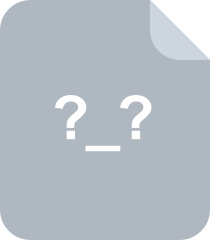
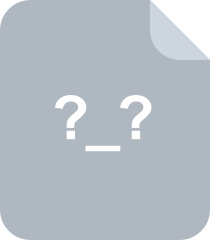
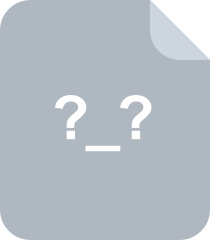
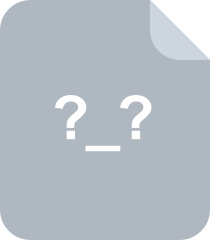
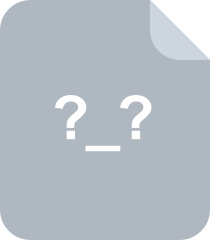
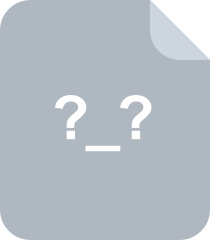
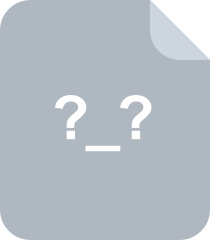
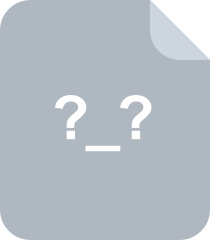
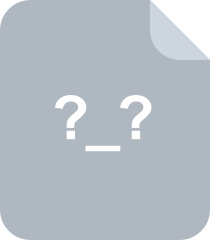
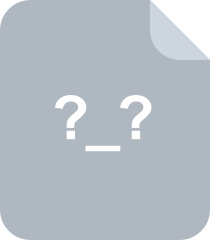
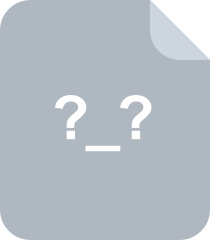
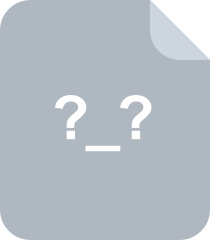
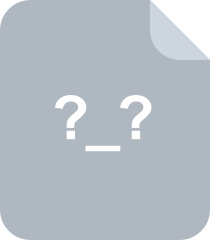
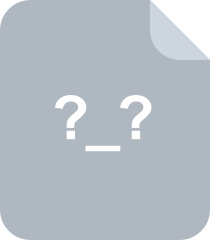
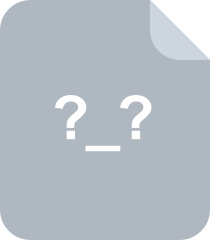
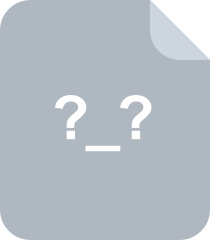
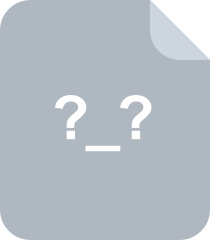
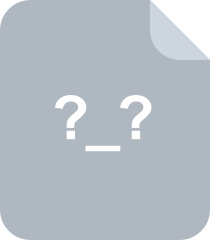
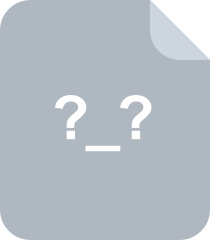
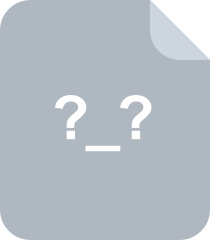
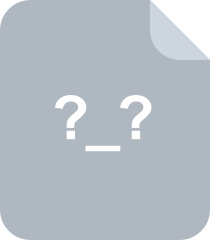
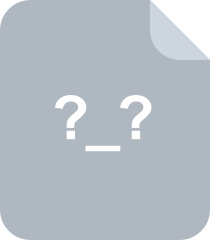
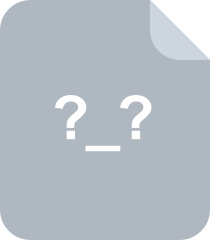
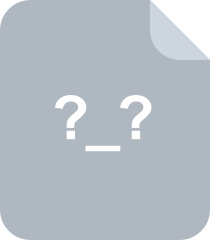
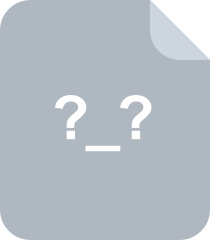
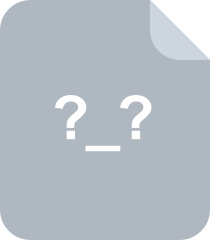
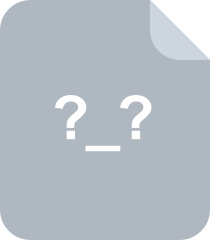
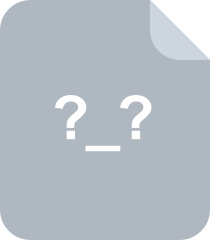
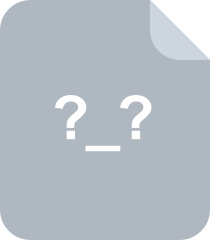
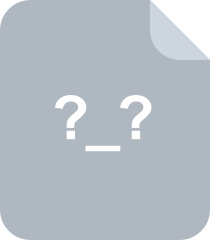
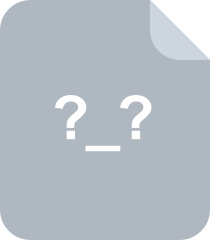
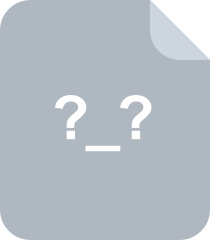
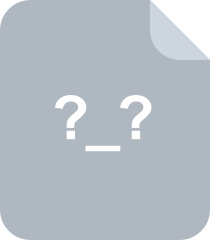
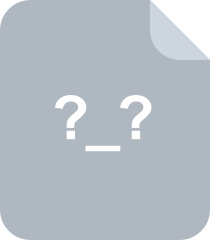
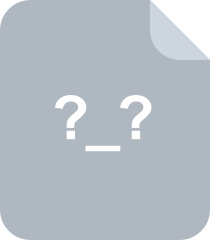
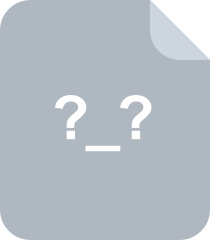
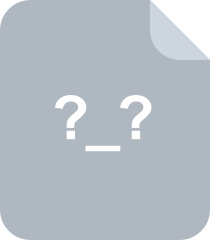
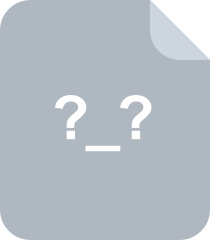
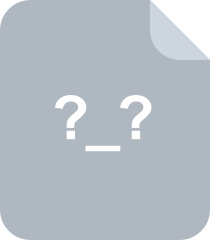
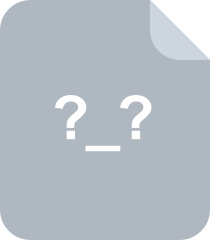
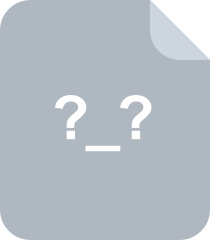
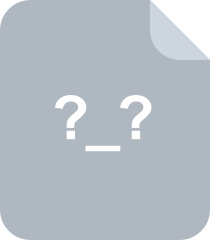
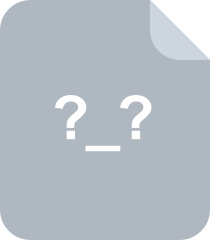
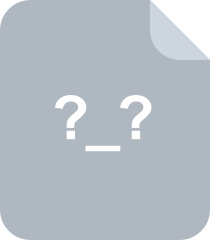
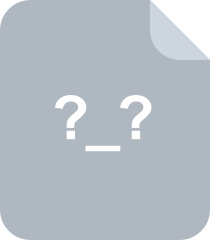
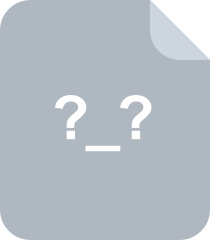
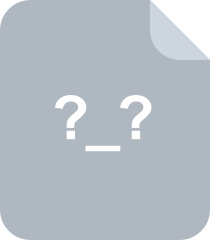
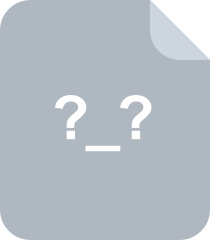
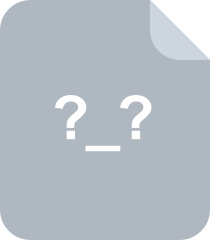
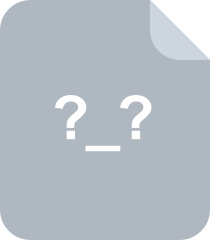
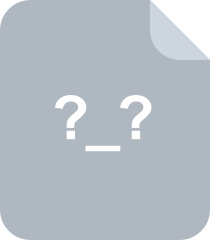
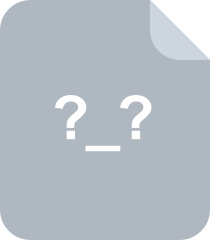
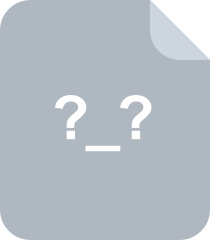
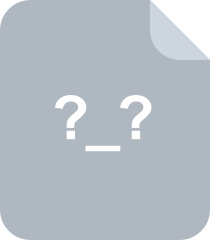
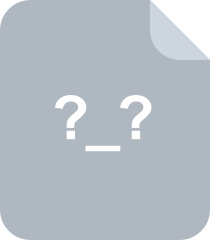
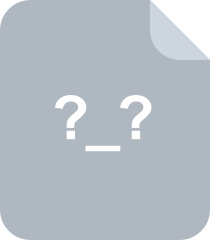
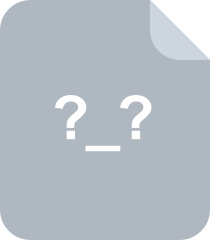
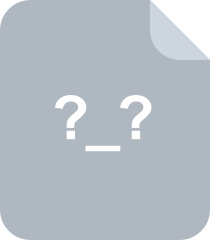
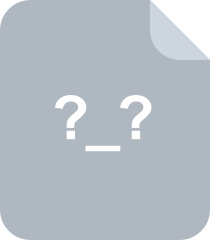
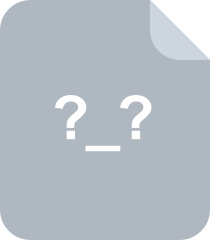
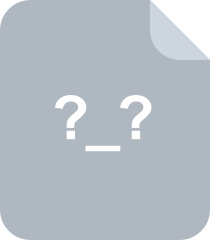
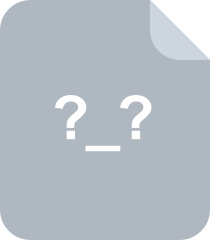
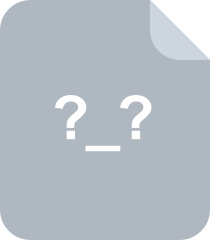
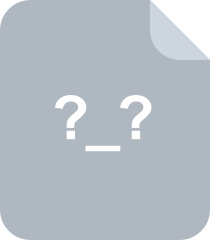
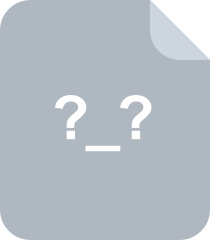
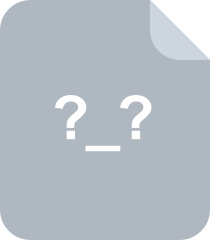
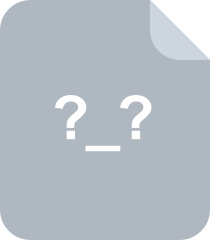
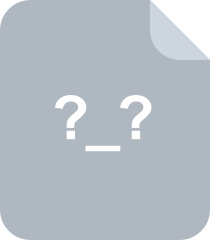
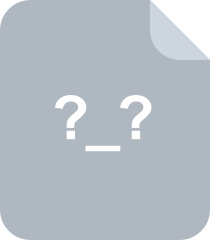
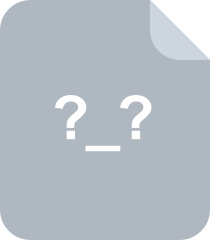
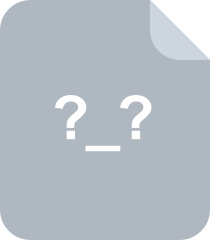
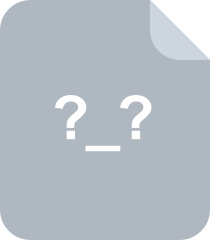
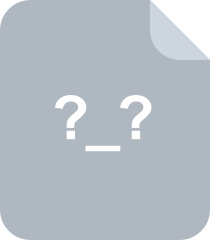
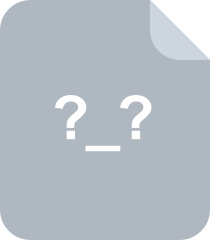
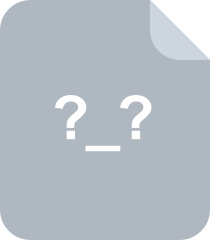
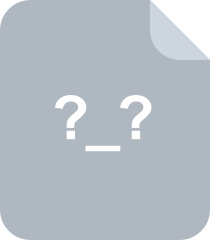
共 1248 条
- 1
- 2
- 3
- 4
- 5
- 6
- 13
资源评论
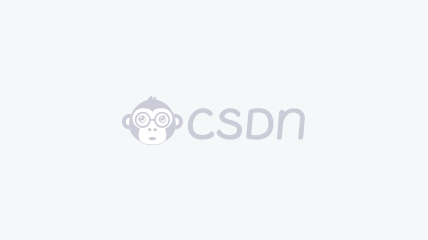

咕噜奥
- 粉丝: 5
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

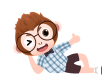
安全验证
文档复制为VIP权益,开通VIP直接复制
