/**
******************************************************************************
* @file stm32f7xx_hal_cryp_ex.c
* @author MCD Application Team
* @brief Extended CRYP HAL module driver
* This file provides firmware functions to manage the following
* functionalities of CRYP extension peripheral:
* + Extended AES processing functions
*
@verbatim
==============================================================================
##### How to use this driver #####
==============================================================================
[..]
The CRYP Extension HAL driver can be used as follows:
(#)Initialize the CRYP low level resources by implementing the HAL_CRYP_MspInit():
(##) Enable the CRYP interface clock using __HAL_RCC_CRYP_CLK_ENABLE()
(##) In case of using interrupts (e.g. HAL_CRYPEx_AESGCM_Encrypt_IT())
(+++) Configure the CRYP interrupt priority using HAL_NVIC_SetPriority()
(+++) Enable the CRYP IRQ handler using HAL_NVIC_EnableIRQ()
(+++) In CRYP IRQ handler, call HAL_CRYP_IRQHandler()
(##) In case of using DMA to control data transfer (e.g. HAL_AES_ECB_Encrypt_DMA())
(+++) Enable the DMAx interface clock using __DMAx_CLK_ENABLE()
(+++) Configure and enable two DMA streams one for managing data transfer from
memory to peripheral (input stream) and another stream for managing data
transfer from peripheral to memory (output stream)
(+++) Associate the initialized DMA handle to the CRYP DMA handle
using __HAL_LINKDMA()
(+++) Configure the priority and enable the NVIC for the transfer complete
interrupt on the two DMA Streams. The output stream should have higher
priority than the input stream HAL_NVIC_SetPriority() and HAL_NVIC_EnableIRQ()
(#)Initialize the CRYP HAL using HAL_CRYP_Init(). This function configures mainly:
(##) The data type: 1-bit, 8-bit, 16-bit and 32-bit
(##) The key size: 128, 192 and 256. This parameter is relevant only for AES
(##) The encryption/decryption key. Its size depends on the algorithm
used for encryption/decryption
(##) The initialization vector (counter). It is not used ECB mode.
(#)Three processing (encryption/decryption) functions are available:
(##) Polling mode: encryption and decryption APIs are blocking functions
i.e. they process the data and wait till the processing is finished
e.g. HAL_CRYPEx_AESGCM_Encrypt()
(##) Interrupt mode: encryption and decryption APIs are not blocking functions
i.e. they process the data under interrupt
e.g. HAL_CRYPEx_AESGCM_Encrypt_IT()
(##) DMA mode: encryption and decryption APIs are not blocking functions
i.e. the data transfer is ensured by DMA
e.g. HAL_CRYPEx_AESGCM_Encrypt_DMA()
(#)When the processing function is called at first time after HAL_CRYP_Init()
the CRYP peripheral is initialized and processes the buffer in input.
At second call, the processing function performs an append of the already
processed buffer.
When a new data block is to be processed, call HAL_CRYP_Init() then the
processing function.
(#)In AES-GCM and AES-CCM modes are an authenticated encryption algorithms
which provide authentication messages.
HAL_AES_GCM_Finish() and HAL_AES_CCM_Finish() are used to provide those
authentication messages.
Call those functions after the processing ones (polling, interrupt or DMA).
e.g. in AES-CCM mode call HAL_CRYPEx_AESCCM_Encrypt() to encrypt the plain data
then call HAL_CRYPEx_AESCCM_Finish() to get the authentication message
-@- For CCM Encrypt/Decrypt API's, only DataType = 8-bit is supported by this version.
-@- The HAL_CRYPEx_AESGCM_xxxx() implementation is limited to 32bits inputs data length
(Plain/Cyphertext, Header) compared with GCM standards specifications (800-38D).
(#)Call HAL_CRYP_DeInit() to deinitialize the CRYP peripheral.
@endverbatim
******************************************************************************
* @attention
*
* <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2>
*
* Redistribution and use in source and binary forms, with or without modification,
* are permitted provided that the following conditions are met:
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
* 3. Neither the name of STMicroelectronics nor the names of its contributors
* may be used to endorse or promote products derived from this software
* without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
* FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
* DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
* OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
******************************************************************************
*/
/* Includes ------------------------------------------------------------------*/
#include "stm32f7xx_hal.h"
/** @addtogroup STM32F7xx_HAL_Driver
* @{
*/
/** @defgroup CRYPEx CRYPEx
* @brief CRYP Extension HAL module driver.
* @{
*/
#ifdef HAL_CRYP_MODULE_ENABLED
#if defined (CRYP)
/* Private typedef -----------------------------------------------------------*/
/* Private define ------------------------------------------------------------*/
/** @addtogroup CRYPEx_Private_define
* @{
*/
#define CRYPEx_TIMEOUT_VALUE 1
/**
* @}
*/
/* Private macro -------------------------------------------------------------*/
/* Private variables ---------------------------------------------------------*/
/* Private function prototypes -----------------------------------------------*/
/** @defgroup CRYPEx_Private_Functions_prototypes CRYP Private Functions Prototypes
* @{
*/
static void CRYPEx_GCMCCM_SetInitVector(CRYP_HandleTypeDef *hcryp, uint8_t *InitVector);
static void CRYPEx_GCMCCM_SetKey(CRYP_HandleTypeDef *hcryp, uint8_t *Key, uint32_t KeySize);
static HAL_StatusTypeDef CRYPEx_GCMCCM_ProcessData(CRYP_HandleTypeDef *hcryp, uint8_t *Input, uint16_t Ilength, uint8_t *Output, uint32_t Timeout);
static HAL_StatusTypeDef CRYPEx_GCMCCM_SetHeaderPhase(CRYP_HandleTypeDef *hcryp, uint8_t* Input, uint16_t Ilength, uint32_t Timeout);
static void CRYPEx_GCMCCM_DMAInCplt(DMA_HandleTypeDef *hdma);
static void CRYPEx_GCMCCM_DMAOutCplt(DMA_HandleTypeDef *hdma);
static void CRYPEx_GCMCCM_DMAError(DMA_HandleTypeDef *hdma);
static void CRYPEx_GCMCCM_SetDMAConfig(CRYP_HandleTypeDef *hcryp, uint32_t inputaddr, uint16_t Size, uint32_t outputaddr);
/**
* @}
*/
/* Private functions ---------------------------------------------
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
EtherCAT主站-SOEM专栏的源代码。 EtherCAT主站SOEM -- 28 -- STM32F767-SOEM通过 CSV模式(周期同步速度模式)控制一个电机转圈圈。 博客链接:( https://blog.csdn.net/qq_50808730/category_12482257.html ) 视频链接: ( https://space.bilibili.com/436089624/channel/collectiondetail?sid=1893755&ctype=0 ) 硬件环境:基于正点原子开发板阿波罗 STM32F767IGT6 上进行搭建SOEM主站。 源代码 主要功能: 初始化MAC,配置EtherCAT网络,等待从站进入OP状态,检查EtherCAT主站和从站状态等等。 并且对1个EtherCAT从站 电机进行操作,通过 CSV模式(周期同步速度模式)控制一个电机转圈圈,正转,反转及停止,及电机运行过程中停止。 key0是电机使能、key1是电机反转、key2是电机正转 、 key_UP是电机停止。
资源推荐
资源详情
资源评论
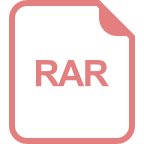
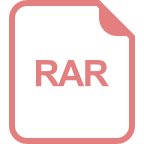
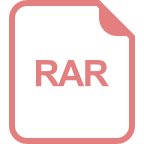
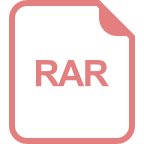
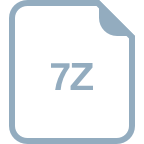
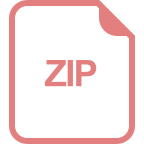
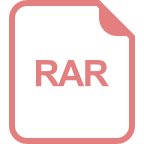
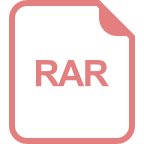
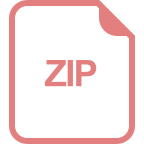
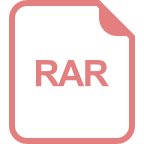
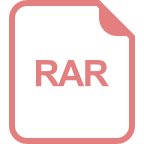
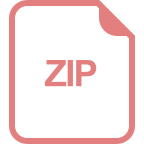
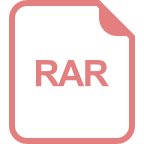
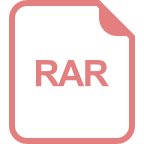
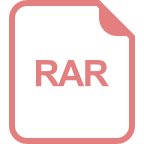
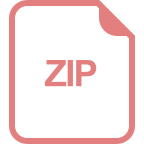
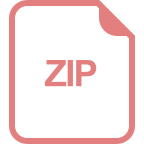
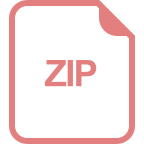
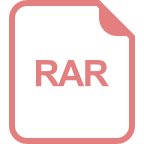
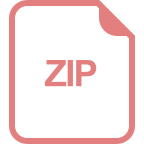
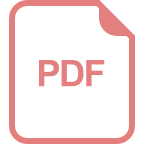
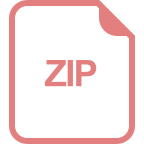
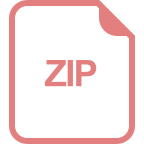
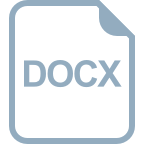
收起资源包目录

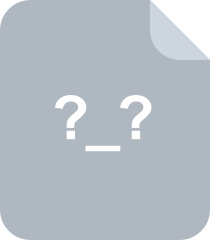
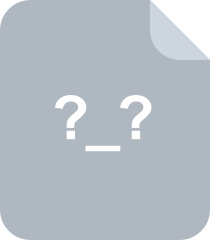
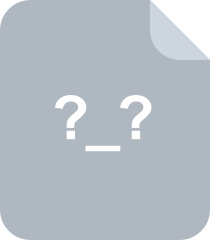
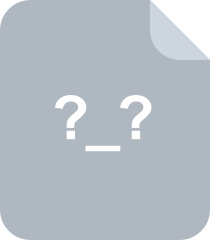
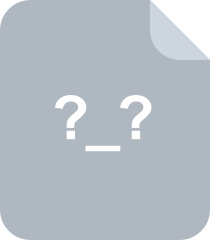
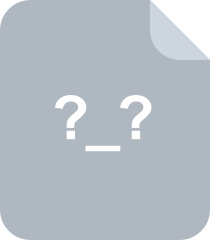
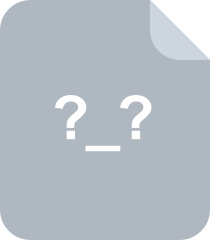
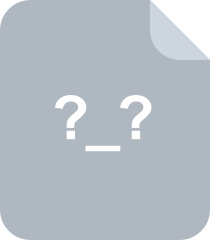
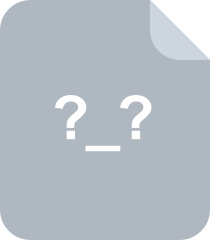
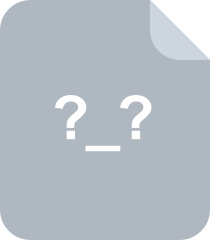
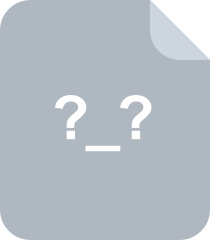
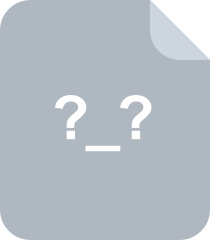
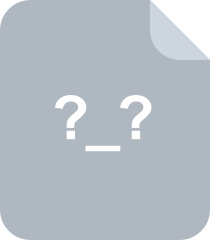
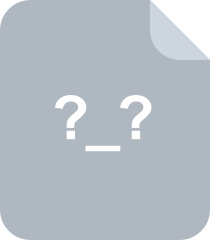
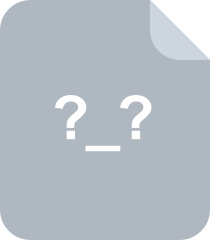
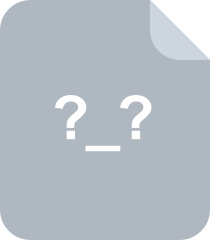
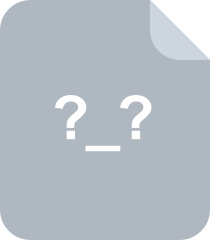
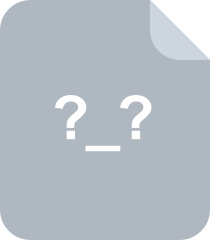
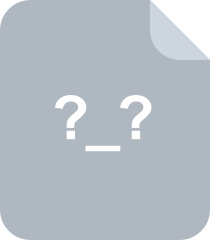
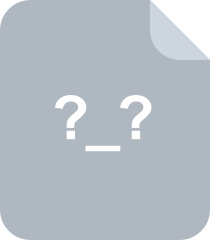
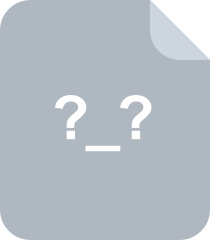
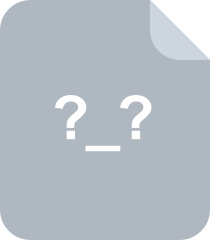
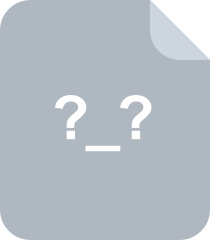
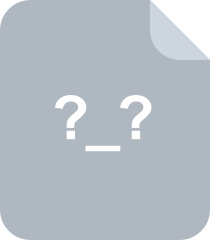
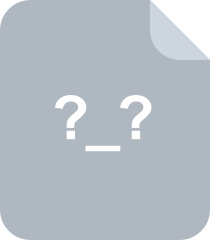
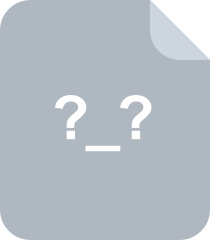
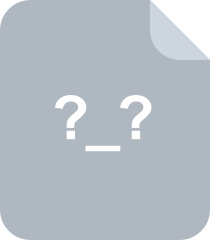
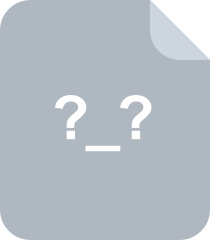
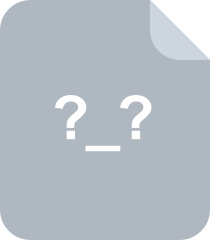
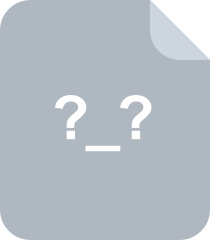
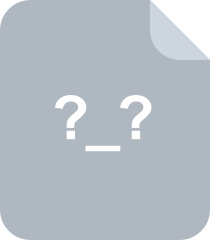
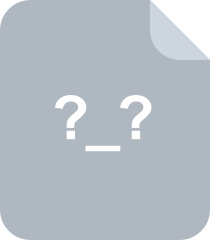
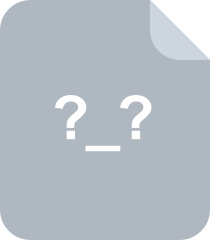
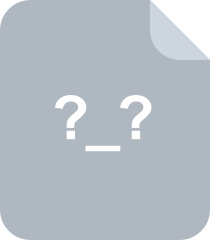
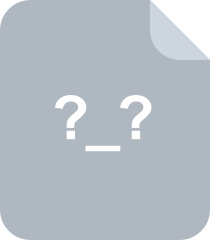
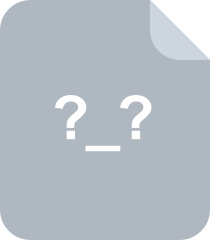
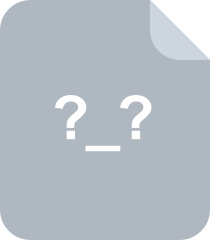
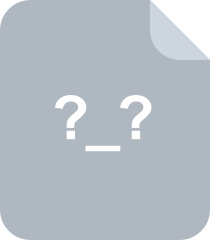
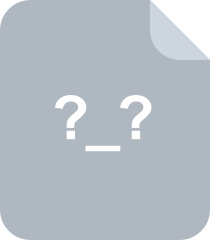
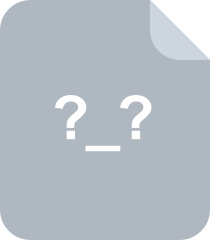
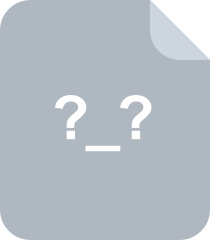
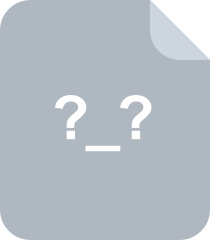
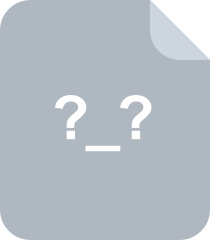
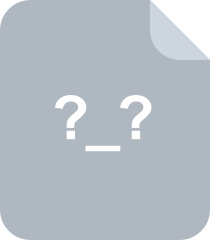
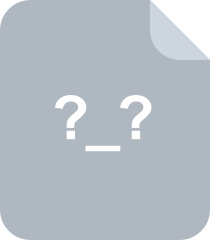
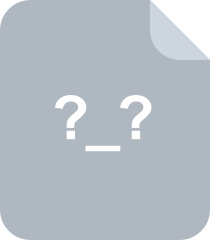
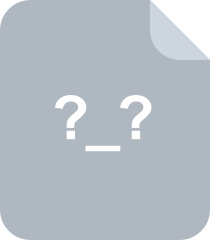
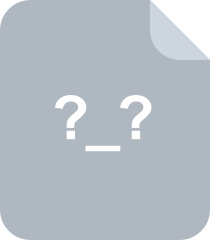
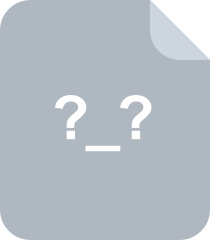
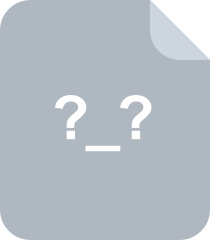
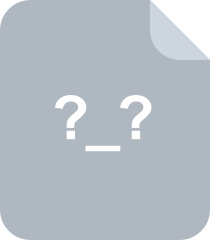
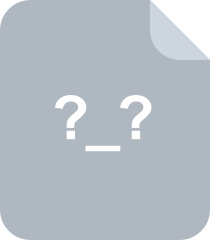
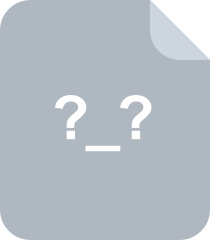
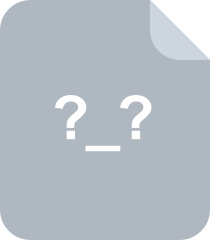
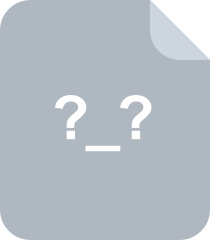
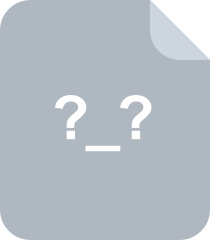
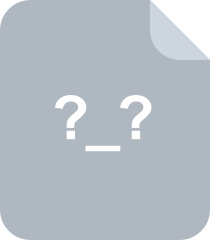
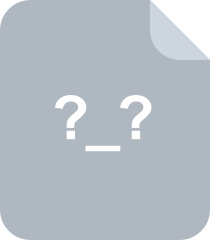
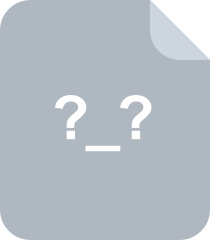
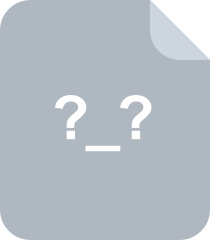
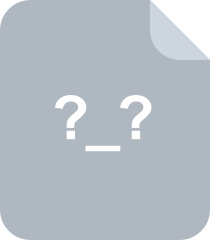
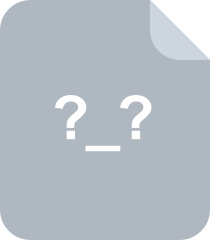
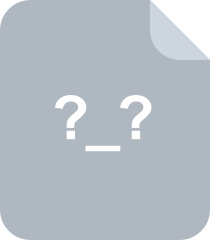
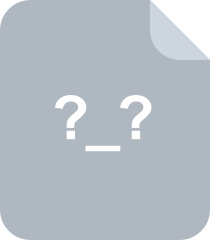
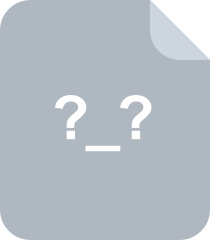
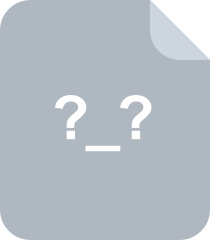
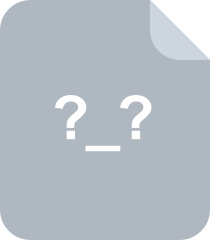
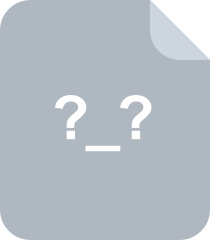
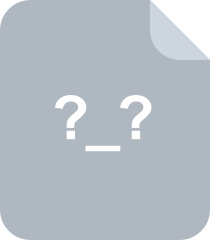
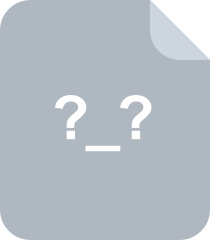
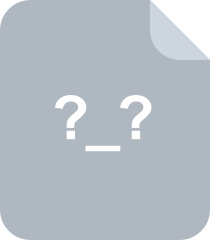
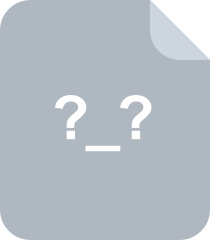
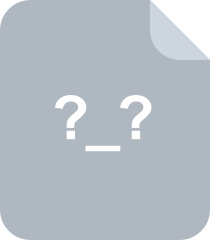
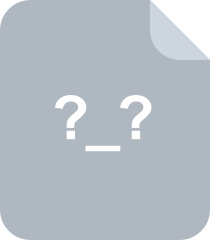
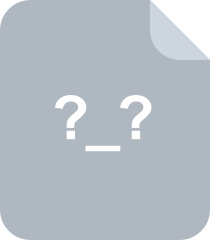
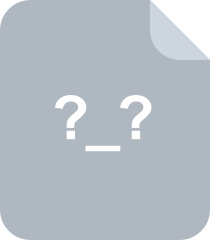
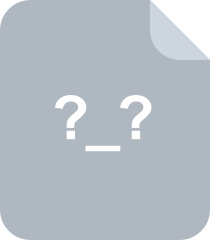
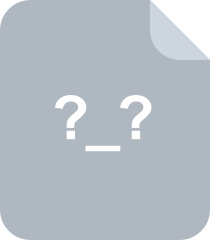
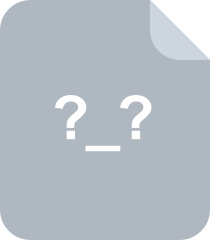
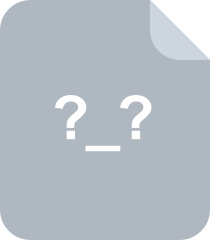
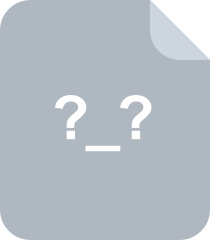
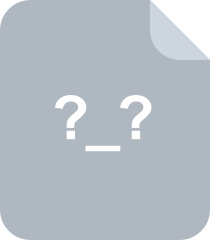
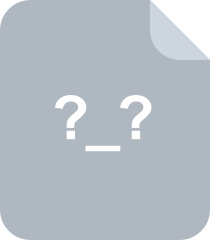
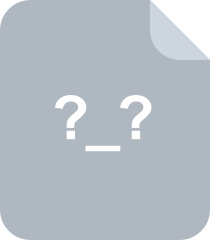
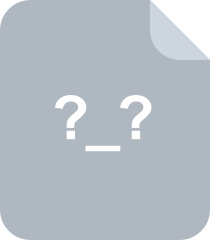
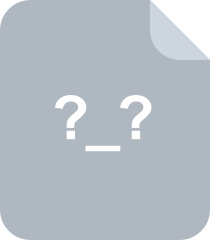
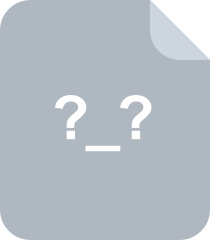
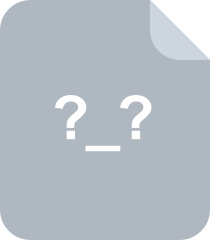
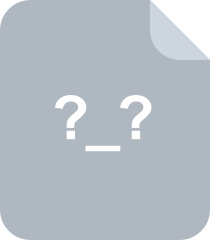
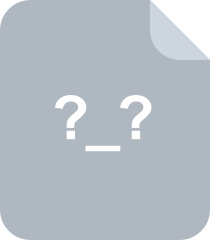
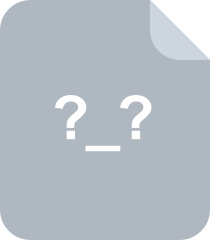
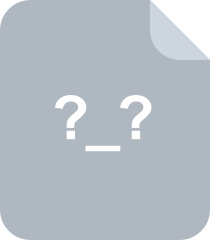
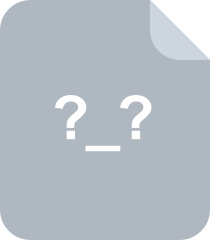
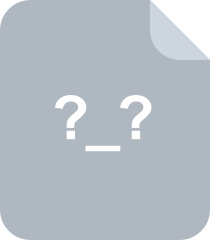
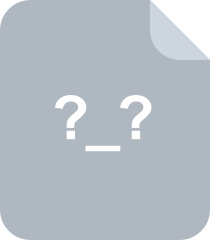
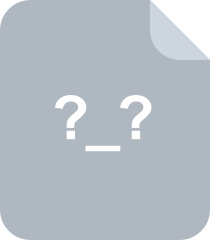
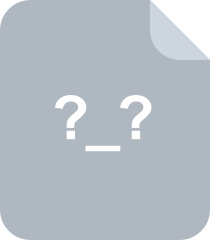
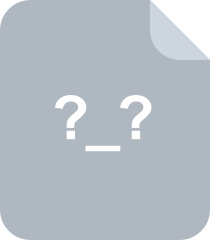
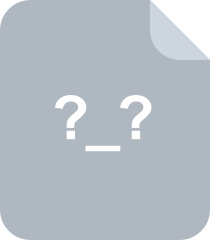
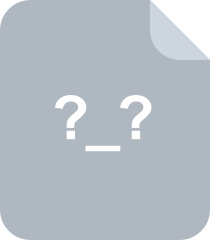
共 470 条
- 1
- 2
- 3
- 4
- 5
资源评论
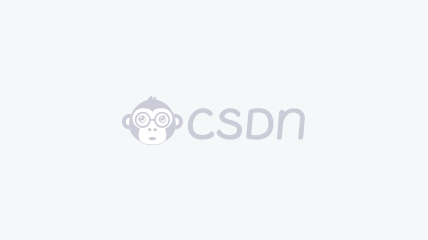

常驻客栈
- 粉丝: 1w+
- 资源: 1366
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

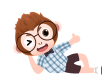
安全验证
文档复制为VIP权益,开通VIP直接复制
