/*
* Licensed under the GNU General Public License version 2 with exceptions. See
* LICENSE file in the project root for full license information
*/
/**
* \file
* \brief
* Main EtherCAT functions.
*
* Initialisation, state set and read, mailbox primitives, EEPROM primitives,
* SII reading and processdata exchange.
*
* Defines ec_slave[]. All slave information is put in this structure.
* Needed for most user interaction with slaves.
*/
#include <stdio.h>
#include <string.h>
#include "osal.h"
#include "oshw.h"
#include "ethercat.h"
/** delay in us for eeprom ready loop */
#define EC_LOCALDELAY 200
/** record for ethercat eeprom communications */
PACKED_BEGIN
typedef struct PACKED
{
uint16 comm;
uint16 addr;
uint16 d2;
} ec_eepromt;
PACKED_END
/** mailbox error structure */
PACKED_BEGIN
typedef struct PACKED
{
ec_mbxheadert MbxHeader;
uint16 Type;
uint16 Detail;
} ec_mbxerrort;
PACKED_END
/** emergency request structure */
PACKED_BEGIN
typedef struct PACKED
{
ec_mbxheadert MbxHeader;
uint16 CANOpen;
uint16 ErrorCode;
uint8 ErrorReg;
uint8 bData;
uint16 w1,w2;
} ec_emcyt;
PACKED_END
#ifdef EC_VER1
/** Main slave data array.
* Each slave found on the network gets its own record.
* ec_slave[0] is reserved for the master. Structure gets filled
* in by the configuration function ec_config().
*/
ec_slavet ec_slave[EC_MAXSLAVE];
/** number of slaves found on the network */
int ec_slavecount;
/** slave group structure */
ec_groupt ec_group[EC_MAXGROUP];
/** cache for EEPROM read functions */
static uint8 ec_esibuf[EC_MAXEEPBUF];
/** bitmap for filled cache buffer bytes */
static uint32 ec_esimap[EC_MAXEEPBITMAP];
/** current slave for EEPROM cache buffer */
static ec_eringt ec_elist;
static ec_idxstackT ec_idxstack;
/** SyncManager Communication Type struct to store data of one slave */
static ec_SMcommtypet ec_SMcommtype[EC_MAX_MAPT];
/** PDO assign struct to store data of one slave */
static ec_PDOassignt ec_PDOassign[EC_MAX_MAPT];
/** PDO description struct to store data of one slave */
static ec_PDOdesct ec_PDOdesc[EC_MAX_MAPT];
/** buffer for EEPROM SM data */
static ec_eepromSMt ec_SM;
/** buffer for EEPROM FMMU data */
static ec_eepromFMMUt ec_FMMU;
/** Global variable TRUE if error available in error stack */
boolean EcatError = FALSE;
int64 ec_DCtime;
ecx_portt ecx_port;
ecx_redportt ecx_redport;
ecx_contextt ecx_context = {
&ecx_port, // .port =
&ec_slave[0], // .slavelist =
&ec_slavecount, // .slavecount =
EC_MAXSLAVE, // .maxslave =
&ec_group[0], // .grouplist =
EC_MAXGROUP, // .maxgroup =
&ec_esibuf[0], // .esibuf =
&ec_esimap[0], // .esimap =
0, // .esislave =
&ec_elist, // .elist =
&ec_idxstack, // .idxstack =
&EcatError, // .ecaterror =
&ec_DCtime, // .DCtime =
&ec_SMcommtype[0], // .SMcommtype =
&ec_PDOassign[0], // .PDOassign =
&ec_PDOdesc[0], // .PDOdesc =
&ec_SM, // .eepSM =
&ec_FMMU, // .eepFMMU =
NULL, // .FOEhook()
NULL, // .EOEhook()
0, // .manualstatechange
NULL, // .userdata
};
#endif
/** Create list over available network adapters.
*
* @return First element in list over available network adapters.
*/
ec_adaptert * ec_find_adapters (void)
{
ec_adaptert * ret_adapter;
ret_adapter = oshw_find_adapters ();
return ret_adapter;
}
/** Free dynamically allocated list over available network adapters.
*
* @param[in] adapter = Struct holding adapter name, description and pointer to next.
*/
void ec_free_adapters (ec_adaptert * adapter)
{
oshw_free_adapters (adapter);
}
/** Pushes an error on the error list.
*
* @param[in] context = context struct
* @param[in] Ec pointer describing the error.
*/
void ecx_pusherror(ecx_contextt *context, const ec_errort *Ec)
{
context->elist->Error[context->elist->head] = *Ec;
context->elist->Error[context->elist->head].Signal = TRUE;
context->elist->head++;
if (context->elist->head > EC_MAXELIST)
{
context->elist->head = 0;
}
if (context->elist->head == context->elist->tail)
{
context->elist->tail++;
}
if (context->elist->tail > EC_MAXELIST)
{
context->elist->tail = 0;
}
*(context->ecaterror) = TRUE;
}
/** Pops an error from the list.
*
* @param[in] context = context struct
* @param[out] Ec = Struct describing the error.
* @return TRUE if an error was popped.
*/
boolean ecx_poperror(ecx_contextt *context, ec_errort *Ec)
{
boolean notEmpty = (context->elist->head != context->elist->tail);
*Ec = context->elist->Error[context->elist->tail];
context->elist->Error[context->elist->tail].Signal = FALSE;
if (notEmpty)
{
context->elist->tail++;
if (context->elist->tail > EC_MAXELIST)
{
context->elist->tail = 0;
}
}
else
{
*(context->ecaterror) = FALSE;
}
return notEmpty;
}
/** Check if error list has entries.
*
* @param[in] context = context struct
* @return TRUE if error list contains entries.
*/
boolean ecx_iserror(ecx_contextt *context)
{
return (context->elist->head != context->elist->tail);
}
/** Report packet error
*
* @param[in] context = context struct
* @param[in] Slave = Slave number
* @param[in] Index = Index that generated error
* @param[in] SubIdx = Subindex that generated error
* @param[in] ErrorCode = Error code
*/
void ecx_packeterror(ecx_contextt *context, uint16 Slave, uint16 Index, uint8 SubIdx, uint16 ErrorCode)
{
ec_errort Ec;
memset(&Ec, 0, sizeof(Ec));
Ec.Time = osal_current_time();
Ec.Slave = Slave;
Ec.Index = Index;
Ec.SubIdx = SubIdx;
*(context->ecaterror) = TRUE;
Ec.Etype = EC_ERR_TYPE_PACKET_ERROR;
Ec.ErrorCode = ErrorCode;
ecx_pusherror(context, &Ec);
}
/** Report Mailbox Error
*
* @param[in] context = context struct
* @param[in] Slave = Slave number
* @param[in] Detail = Following EtherCAT specification
*/
static void ecx_mbxerror(ecx_contextt *context, uint16 Slave,uint16 Detail)
{
ec_errort Ec;
memset(&Ec, 0, sizeof(Ec));
Ec.Time = osal_current_time();
Ec.Slave = Slave;
Ec.Index = 0;
Ec.SubIdx = 0;
Ec.Etype = EC_ERR_TYPE_MBX_ERROR;
Ec.ErrorCode = Detail;
ecx_pusherror(context, &Ec);
}
/** Report Mailbox Emergency Error
*
* @param[in] context = context struct
* @param[in] Slave = Slave number
* @param[in] ErrorCode = Following EtherCAT specification
* @param[in] ErrorReg
* @param[in] b1
* @param[in] w1
* @param[in] w2
*/
static void ecx_mbxemergencyerror(ecx_contextt *context, uint16 Slave,uint16 ErrorCode,uint16 ErrorReg,
uint8 b1, uint16 w1, uint16 w2)
{
ec_errort Ec;
memset(&Ec, 0, sizeof(Ec));
Ec.Time = osal_current_time();
Ec.Slave = Slave;
Ec.Index = 0;
Ec.SubIdx = 0;
Ec.Etype = EC_ERR_TYPE_EMERGENCY;
Ec.ErrorCode = ErrorCode;
Ec.ErrorReg = (uint8)ErrorReg;
Ec.b1 = b1;
Ec.w1 = w1;
Ec.w2 = w2;
ecx_pusherror(context, &Ec);
}
/** Initialise lib in single NIC mode
* @param[in] context = context struct
* @param[in] ifname = Dev name, f.e. "eth0"
* @return >0 if OK
*/
int ecx_init(ecx_contextt *context, const char * ifname)
{
return ecx_setupnic(context->port, ifname, FALSE);
}
/** Initialise lib in redundant NIC mode
* @param[in] context = context struct
* @param[in] redport = pointer to redport, redundant port data
* @param[in
没有合适的资源?快使用搜索试试~ 我知道了~
win-soem-win10及11系统QT-SOEM-1个电机转圈圈-周期同步力矩模式(CST模式)-添加代码注释-CSDN
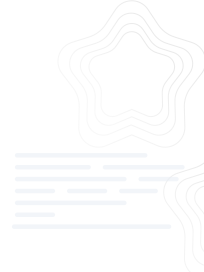
共134个文件
h:77个
c:35个
lib:9个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 90 浏览量
2024-04-27
13:20:03
上传
评论
收藏 478KB RAR 举报
温馨提示
硬件环境: windows10及windows11系统利用QT平台搭建EtherCAT主站(SOEM)。 EtherCAT主站-SOEM专栏的源代码。 EtherCAT主站SOEM -- 39 -- win-soem-win10及win11系统QT-SOEM-EtherCAT主站1个电机转圈圈-周期同步力矩模式(CST模式) 博客链接:( https://blog.csdn.net/qq_50808730/category_12482257.html ) 视频链接: ( https://space.bilibili.com/436089624/channel/collectiondetail?sid=1893755&ctype=0 ) 源代码 主要功能: 获取网卡信息,绑定网卡,配置EtherCAT网络,等待从站进入OP状态,检查EtherCAT主站和从站状等等。 Soem主站识别到 几 个从站。 并且对1个EtherCAT从站 电机进行操作,通过周期同步力矩模式(CST模式)控制一个电机转圈圈,正转,反转及停止,及电机运行过程中停止。
资源推荐
资源详情
资源评论
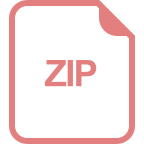
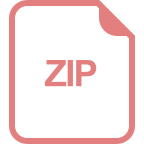
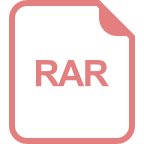
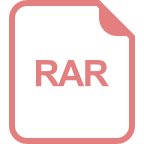
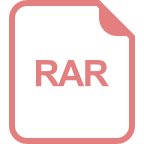
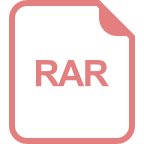
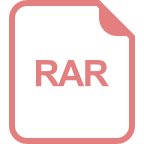
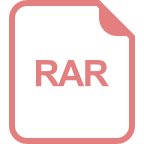
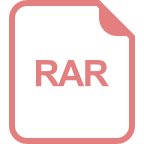
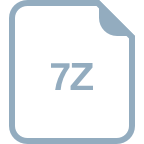
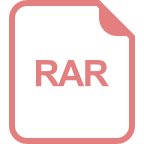
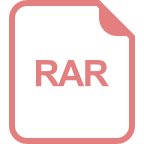
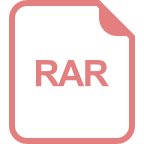
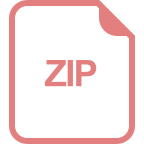
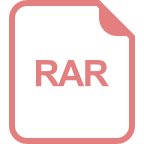
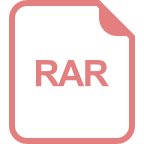
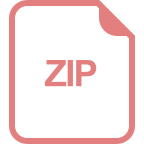
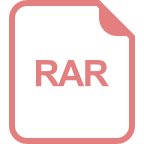
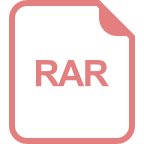
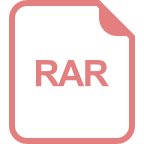
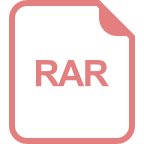
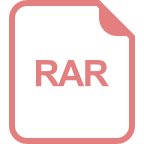
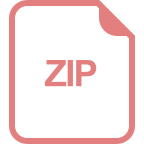
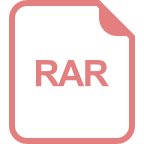
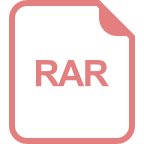
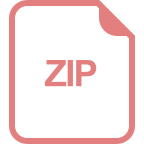
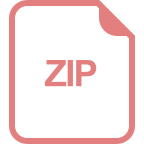
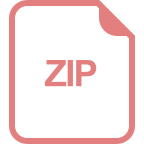
收起资源包目录

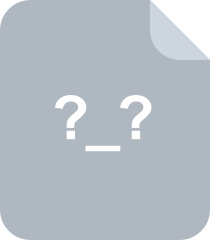
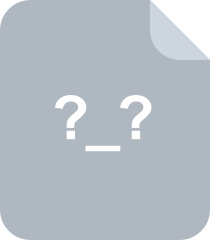
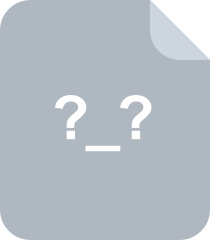
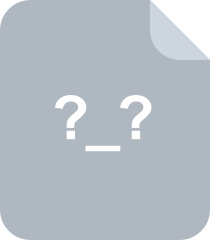
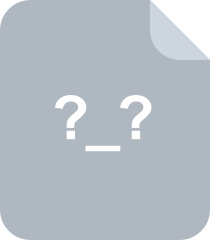
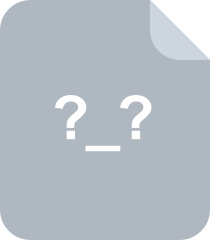
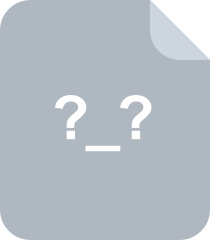
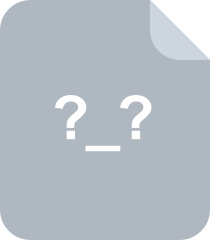
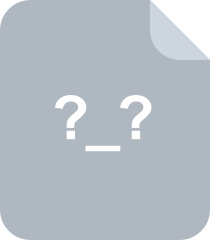
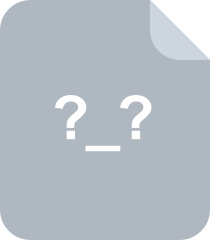
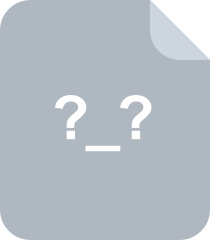
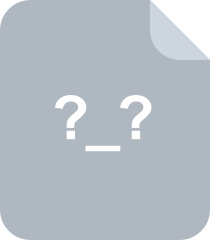
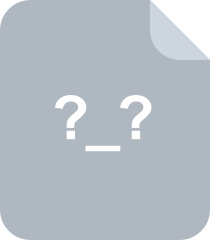
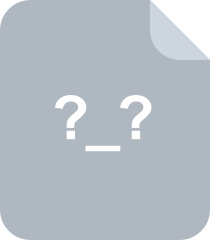
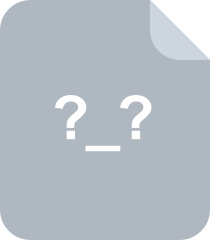
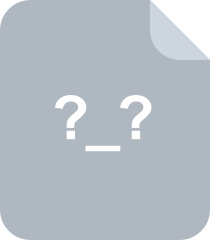
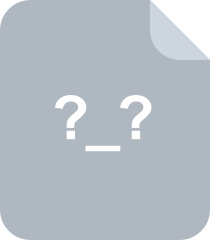
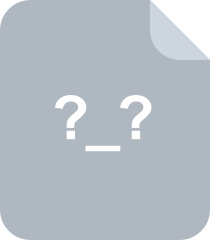
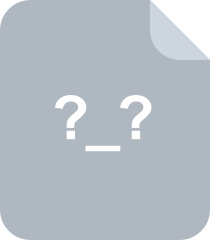
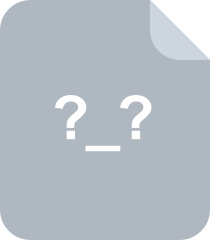
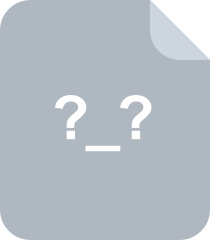
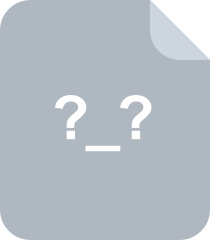
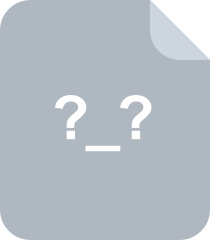
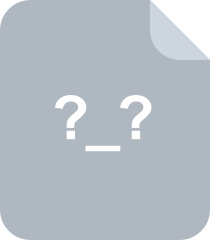
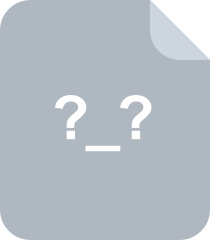
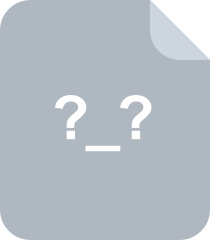
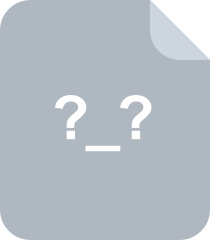
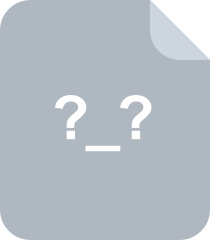
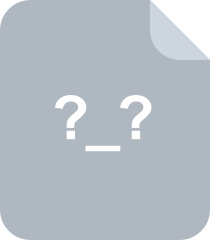
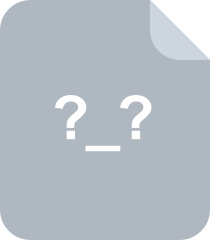
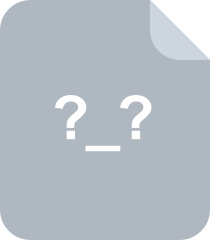
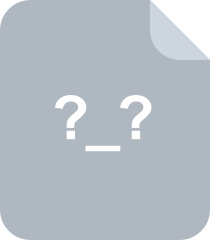
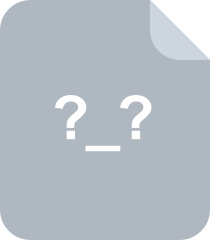
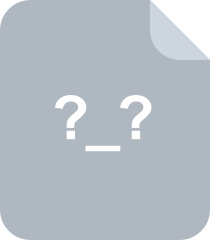
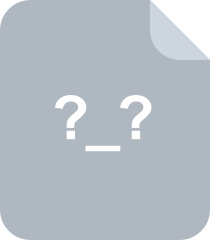
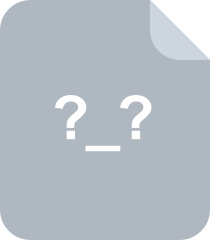
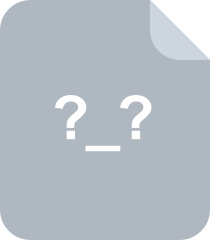
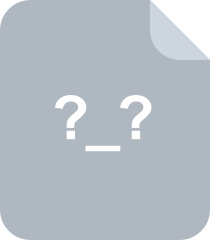
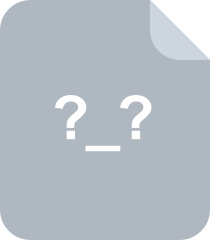
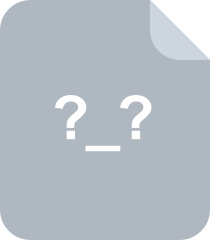
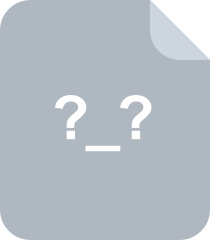
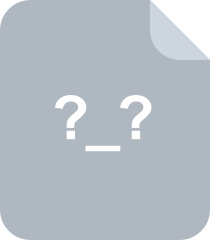
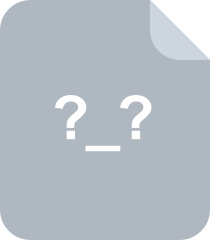
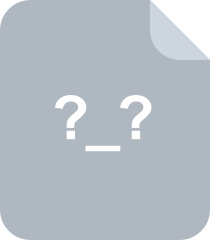
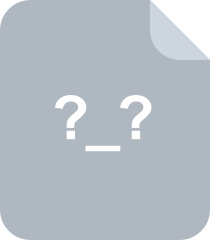
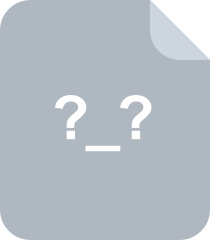
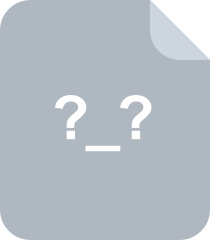
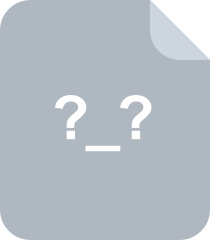
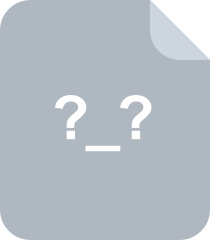
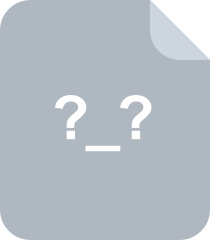
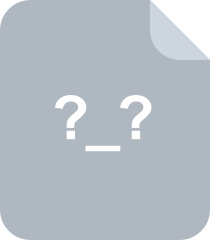
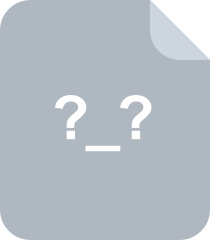
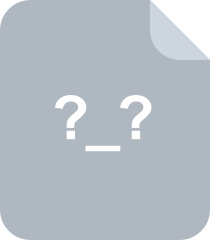
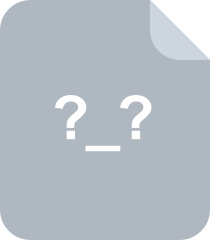
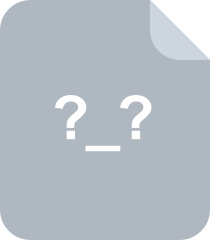
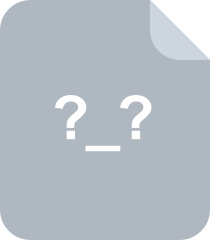
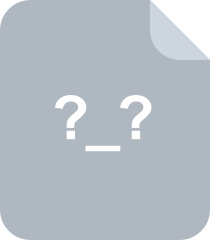
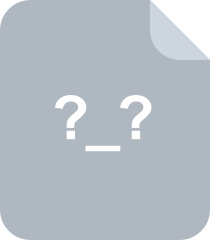
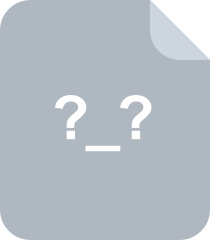
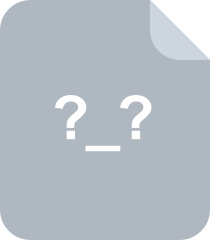
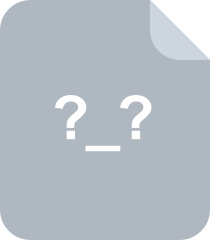
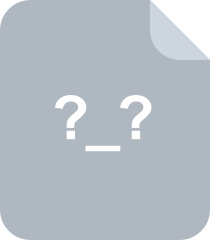
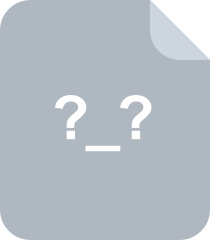
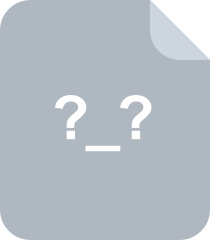
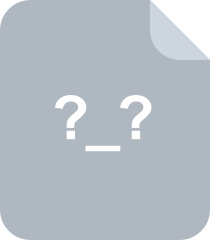
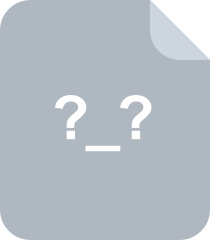
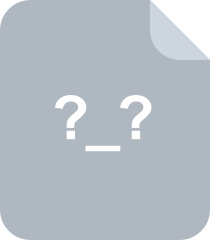
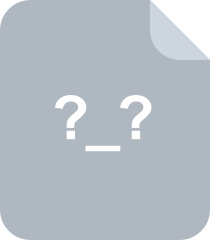
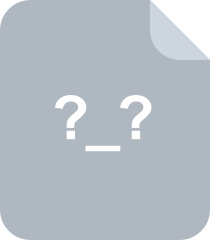
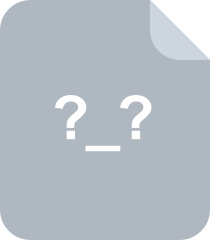
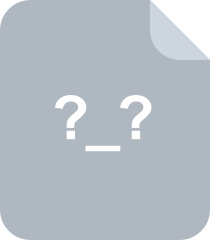
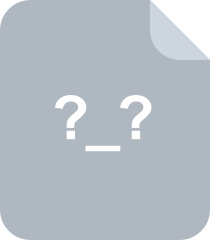
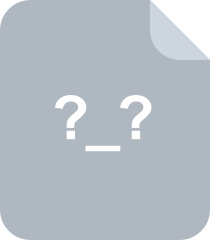
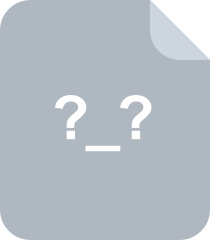
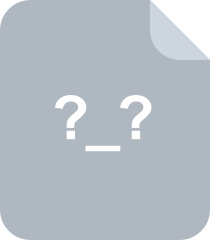
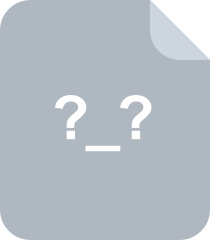
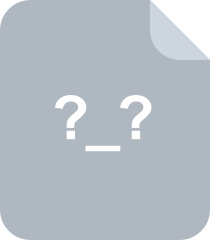
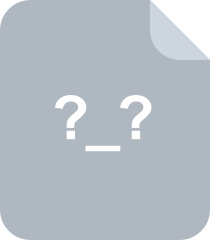
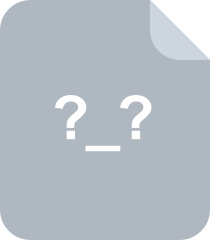
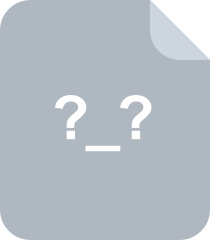
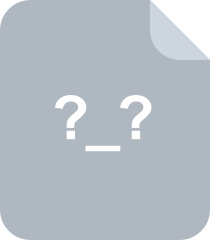
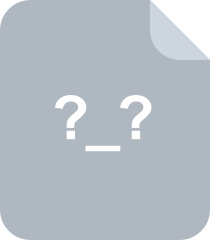
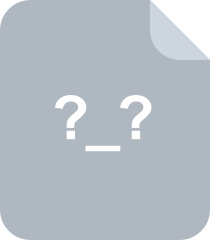
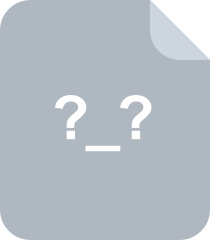
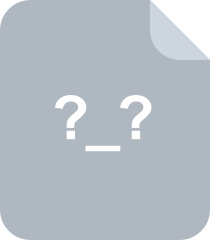
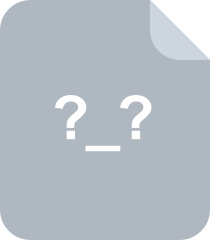
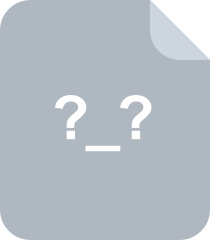
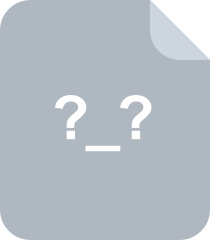
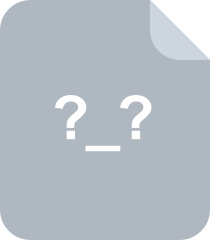
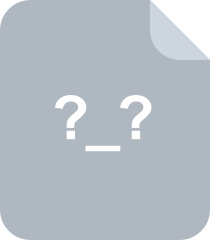
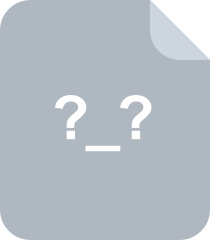
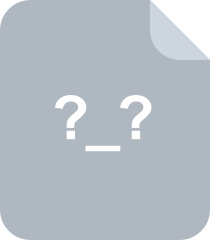
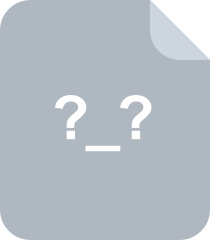
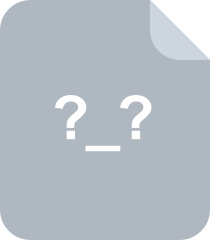
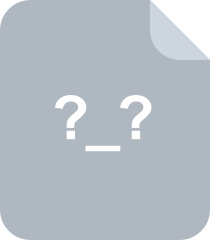
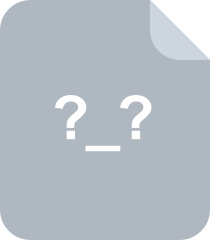
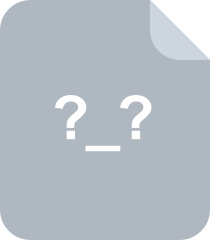
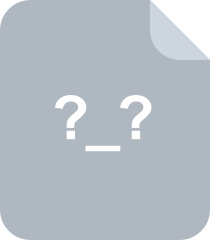
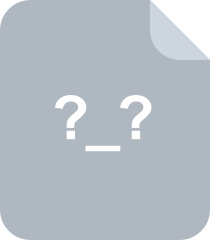
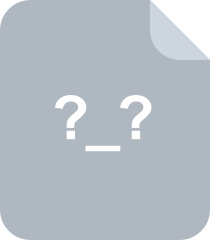
共 134 条
- 1
- 2
资源评论
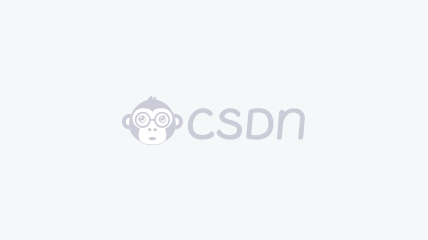

常驻客栈
- 粉丝: 1w+
- 资源: 1378
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

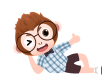
最新资源
- 面向初学者的 Java 教程(包含 500 个代码示例).zip
- 阿里云OSS Java版SDK.zip
- 阿里云api网关请求签名示例(java实现).zip
- 通过示例学习 Android 的 RxJava.zip
- 通过多线程编程在 Java 中发现并发模式和特性 线程、锁、原子等等 .zip
- 通过在终端中进行探索来学习 JavaScript .zip
- 通过不仅针对初学者而且针对 JavaScript 爱好者(无论他们的专业水平如何)设计的编码挑战,自然而自信地拥抱 JavaScript .zip
- 适用于 Kotlin 和 Java 的现代 JSON 库 .zip
- yolo5实战-yolo资源
- english-chinese-dictionary-数据结构课程设计
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


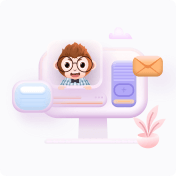
安全验证
文档复制为VIP权益,开通VIP直接复制
