<p align="center">
<img src="https://github.com/jina-ai/jina/blob/master/.github/logo-only.gif?raw=true" alt="Jina banner" width="200px">
</p>
<p align="center">
<b>Cloud-Native <ins>Neural Search</ins><sup><a href=".github/2.0/neural-search.md">[?]</a></sup> Framework for <i>Any</i> Kind of Data</b>
</p>
<p align=center>
<a href="https://pypi.org/project/jina/"><img src="https://github.com/jina-ai/jina/blob/master/.github/badges/python-badge.svg?raw=true" alt="Python 3.7 3.8 3.9" title="Jina supports Python 3.7 and above"></a>
<a href="https://hub.docker.com/r/jinaai/jina/tags"><img src="https://img.shields.io/docker/v/jinaai/jina?color=%23099cec&label=Docker&logo=docker&logoColor=white&sort=semver" alt="Docker Image Version (latest semver)"></a>
<a href="https://pepy.tech/project/jina"><img src="https://pepy.tech/badge/jina/month"></a>
<a href="https://codecov.io/gh/jina-ai/jina"><img src="https://codecov.io/gh/jina-ai/jina/branch/master/graph/badge.svg" alt="codecov"></a>
<a href="https://slack.jina.ai"><img src="https://img.shields.io/badge/Slack-600%2B-blueviolet?logo=slack&logoColor=white"></a>
</p>
<!-- start elevator-pitch -->
Jina<sup><a href=".github/pronounce-jina.mp3">`ð`</a></sup> allows you to build deep learning-powered search-as-a-service in just minutes.
<!-- end elevator-pitch -->
ð **All data type** - Large-scale indexing and querying of any kind of unstructured data: video, image, long/short text, music, source code, PDF, etc.
ð©ï¸ **Fast & cloud-native** - Distributed architecture from day one. Scalable & cloud-native by design: enjoy
containerizing, distributing, streaming, paralleling, sharding, async scheduling with REST/gRPC/WebSocket.
â±ï¸ **Save time** - *The* design pattern of neural search systems, from zero to a production-ready system in minutes.
ð± **Own your stack** - Keep an end-to-end stack ownership of your solution, avoid integration pitfalls with
fragmented, multi-vendor, generic legacy tools.
## Run Quick Demo
- [ð Fashion image search](./.github/pages/hello-world.md#-fashion-image-search): `pip install --pre && jina hello fashion`
- [ð¤ QA chatbot](./.github/pages/hello-world.md#-covid-19-chatbot): `pip install --pre "jina[chatbot]" && jina hello chatbot`
- [ð° Multimodal search](./.github/pages/hello-world.md#-multimodal-document-search): `pip install --pre "jina[multimodal]" && jina hello multimodal`
- ð´ Fork the source of a demo to your folder: `jina hello fork fashion ../my-proj/`
## Install
2.0 is in pre-release, **add `--pre`** to install it.
```console
$ pip install --pre jina
$ jina -v
2.0.0rcN
```
#### via Docker
```console
$ docker run jinaai/jina:master -v
2.0.0rcN
```
<details>
<summary>ð¦ More installation options</summary>
| <br><sub><sup>x86/64,arm64,v6,v7,Apple M1</sup></sub> | On Linux/macOS & Python 3.7/3.8/3.9 | Docker Users|
| --- | --- | --- |
| Standard | `pip install --pre jina` | `docker run jinaai/jina:master` |
| <sub><a href="https://api.jina.ai/daemon/">Daemon</a></sub> | <sub>`pip install --pre "jina[daemon]"`</sub> | <sub>`docker run --network=host jinaai/jina:master-daemon`</sub> |
| <sub>With Extras</sub> | <sub>`pip install --pre "jina[devel]"`</sub> | <sub>`docker run jinaai/jina:master-devel`</sub> |
Version identifiers [are explained here](https://github.com/jina-ai/jina/blob/master/RELEASE.md). Jina can run
on [Windows Subsystem for Linux](https://docs.microsoft.com/en-us/windows/wsl/install-win10). We welcome the community
to help us with [native Windows support](https://github.com/jina-ai/jina/issues/1252).
</details>
## Get Started
Document, Executor, and Flow are the three fundamental concepts in Jina.
- [ð **Document**](.github/2.0/cookbooks/Document.md) is the basic data type in Jina;
- [âï¸ **Executor**](.github/2.0/cookbooks/Executor.md) is how Jina processes Documents;
- [ð **Flow**](.github/2.0/cookbooks/Flow.md) is how Jina streamlines and distributes Executors.
<a href="https://colab.research.google.com/github/jina-ai/jupyter-notebooks/blob/main/2.0-readme-38l.ipynb"><img align="right" src="https://colab.research.google.com/assets/colab-badge.svg?raw=true"/></a>
Copy-paste the minimum example below and run it:
<sup>ð¡ Preliminaries: <a href="https://en.wikipedia.org/wiki/Word_embedding">character embedding</a>, <a href="https://computersciencewiki.org/index.php/Max-pooling_/_Pooling">pooling</a>, <a href="https://en.wikipedia.org/wiki/Euclidean_distance">Euclidean distance</a></sup>
<img src="https://github.com/jina-ai/jina/blob/master/.github/2.0/simple-arch.svg" alt="Get started system diagram">
```python
import numpy as np
from jina import Document, DocumentArray, Executor, Flow, requests
class CharEmbed(Executor): # a simple character embedding with mean-pooling
offset = 32 # letter `a`
dim = 127 - offset + 1 # last pos reserved for `UNK`
char_embd = np.eye(dim) * 1 # one-hot embedding for all chars
@requests
def foo(self, docs: DocumentArray, **kwargs):
for d in docs:
r_emb = [ord(c) - self.offset if self.offset <= ord(c) <= 127 else (self.dim - 1) for c in d.text]
d.embedding = self.char_embd[r_emb, :].mean(axis=0) # average pooling
class Indexer(Executor):
_docs = DocumentArray() # for storing all documents in memory
@requests(on='/index')
def foo(self, docs: DocumentArray, **kwargs):
self._docs.extend(docs) # extend stored `docs`
@requests(on='/search')
def bar(self, docs: DocumentArray, **kwargs):
q = np.stack(docs.get_attributes('embedding')) # get all embeddings from query docs
d = np.stack(self._docs.get_attributes('embedding')) # get all embeddings from stored docs
euclidean_dist = np.linalg.norm(q[:, None, :] - d[None, :, :], axis=-1) # pairwise euclidean distance
for dist, query in zip(euclidean_dist, docs): # add & sort match
query.matches = [Document(self._docs[int(idx)], copy=True, scores={'euclid': d}) for idx, d in enumerate(dist)]
query.matches.sort(key=lambda m: m.scores['euclid'].value) # sort matches by their values
f = Flow(port_expose=12345).add(uses=CharEmbed, parallel=2).add(uses=Indexer) # build a Flow, with 2 parallel CharEmbed, tho unnecessary
with f:
f.post('/index', (Document(text=t.strip()) for t in open(__file__) if t.strip())) # index all lines of this file
f.block() # block for listening request
```
Keep the above running and start a simple client:
```python
from jina import Client, Document
from jina.types.request import Response
def print_matches(resp: Response): # the callback function invoked when task is done
for idx, d in enumerate(resp.docs[0].matches[:3]): # print top-3 matches
print(f'[{idx}]{d.scores["euclid"].value:2f}: "{d.text}"')
c = Client(host='localhost', port_expose=12345) # connect to localhost:12345
c.post('/search', Document(text='request(on=something)'), on_done=print_matches)
```
It finds the lines most similar to "`request(on=something)`" from the server code snippet and prints the following:
```text
Client@1608[S]:connected to the gateway at localhost:12345!
[0]0.168526: "@requests(on='/index')"
[1]0.181676: "@requests(on='/search')"
[2]0.192049: "query.matches = [Document(self._docs[int(idx)], copy=True, score=d) for idx, d in enumerate(dist)]"
```
<sup>ð Doesn't work? Our bad! <a href="https://github.com/jina-ai/jina/issues/new?assignees=&labels=kind%2Fbug&template=---found-a-bug-and-i-solved-it.md&title=">Please report it here.</a></sup>
## Read Tutorials
- ð§ [What is "Neural Search"?](.github/2.0/neural-search.md)
- ð `Document` & `DocumentArray`: the basic data type in Jina.
- [Minimum Working Example](.github/2.0/cookbooks/Document.md#minimum-working-example)
- [`Document` API](.github/2.0/cookbooks/Document.md#document-api)
- [`DocumentArray` API](.github/2.0/cookbooks/Document.md#documentar
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
资源分类:Python库 所属语言:Python 资源全名:jina-2.0.0rc11.dev4.tar.gz 资源来源:官方 安装方法:https://lanzao.blog.csdn.net/article/details/101784059
资源推荐
资源详情
资源评论
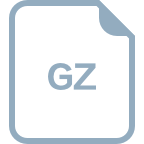
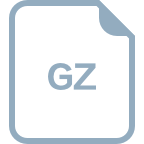
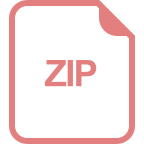
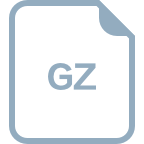
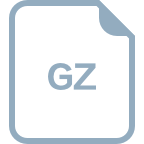
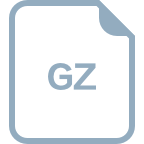
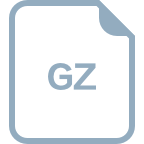
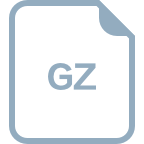
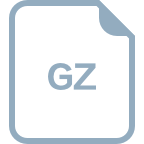
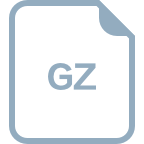
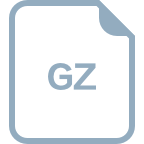
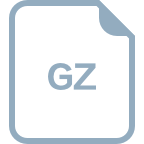
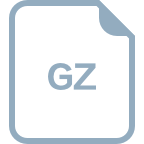
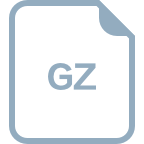
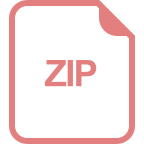
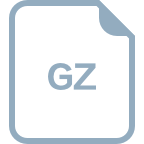
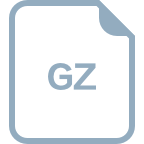
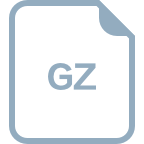
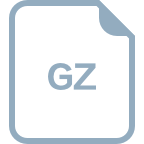
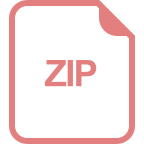
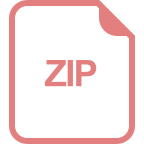
收起资源包目录

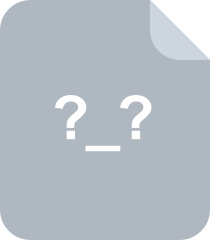
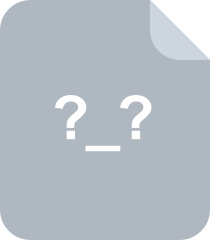
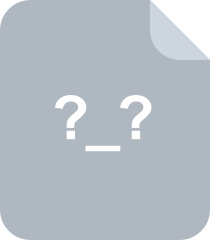
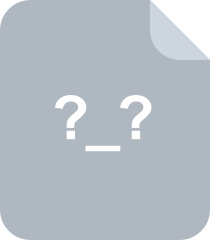
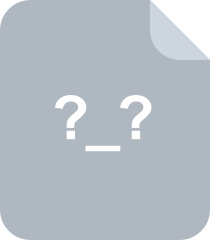
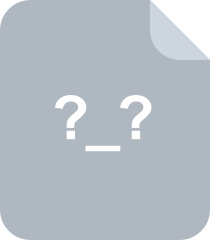
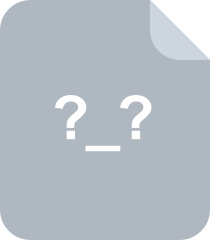
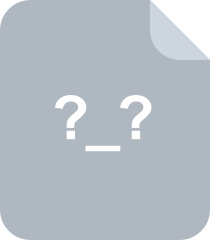
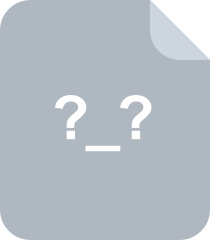
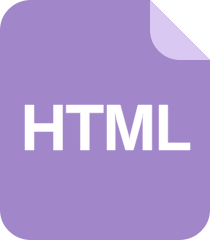
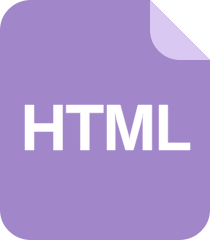
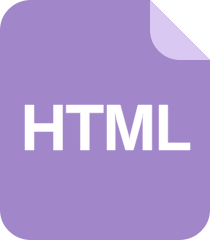
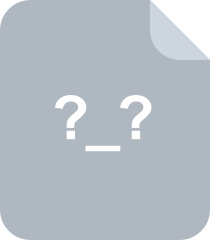
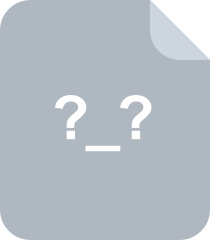
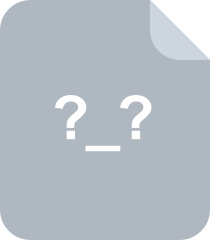
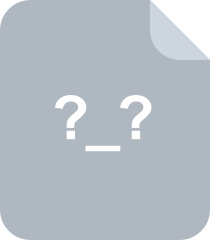
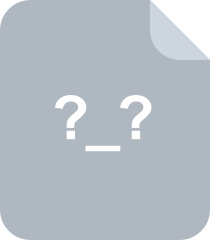
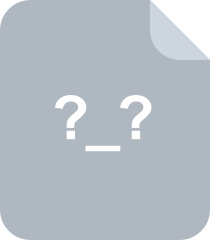
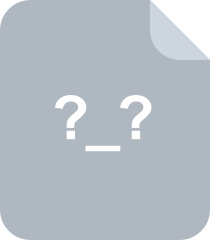
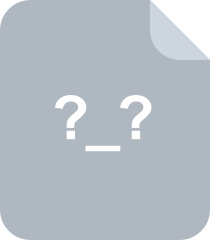
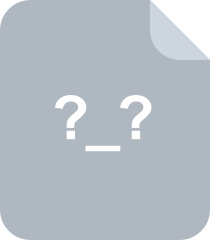
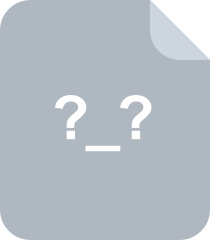
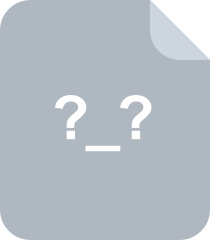
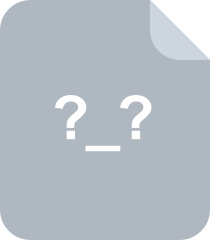
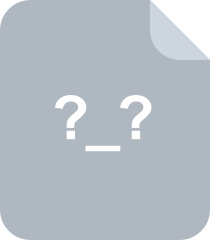
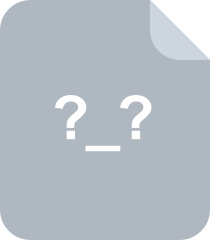
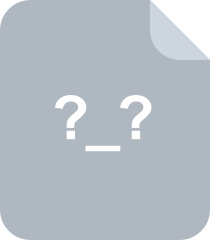
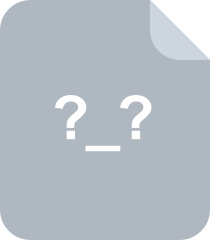
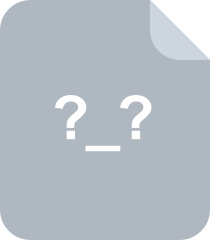
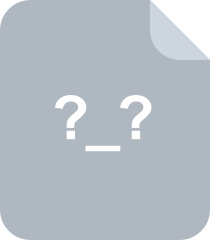
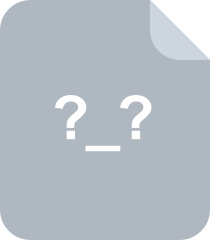
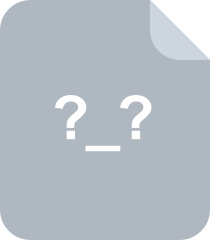
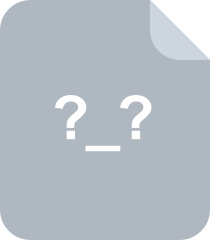
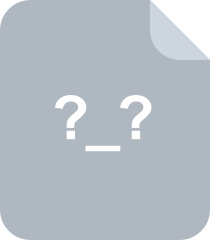
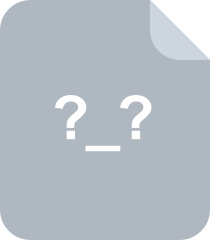
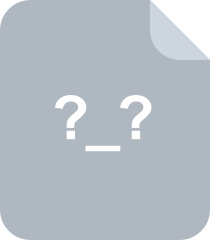
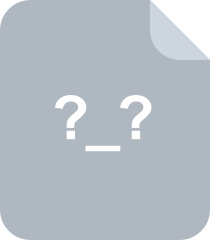
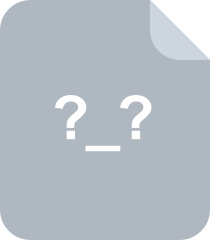
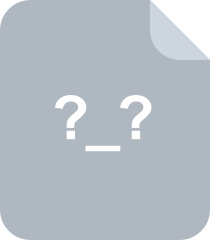
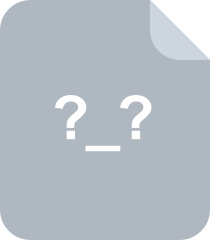
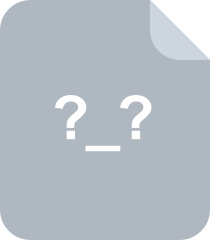
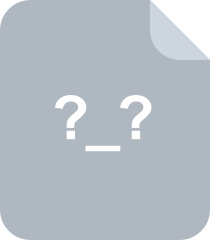
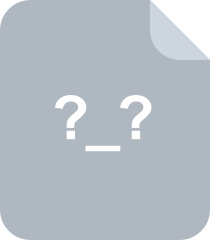
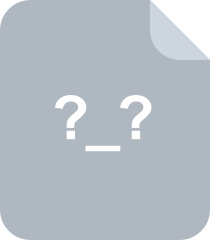
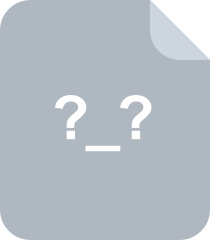
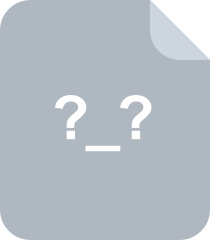
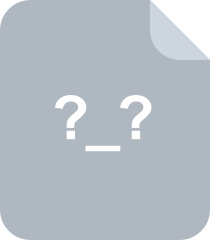
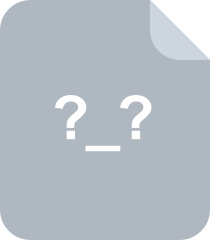
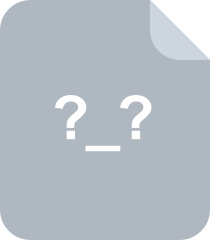
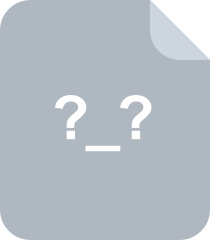
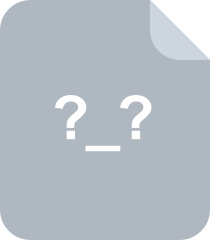
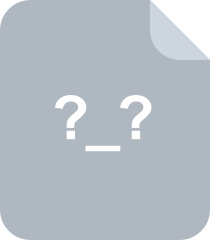
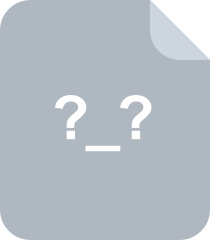
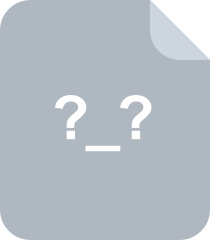
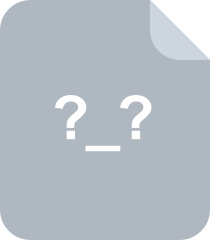
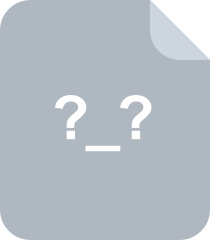
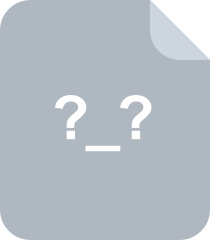
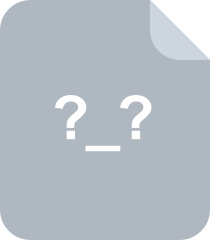
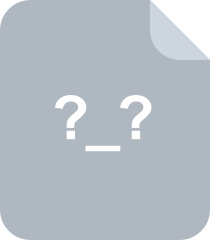
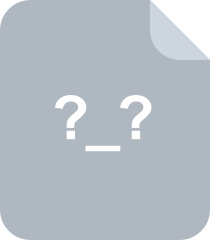
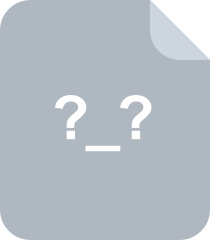
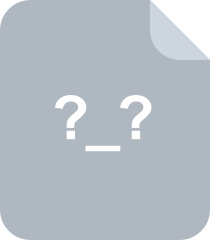
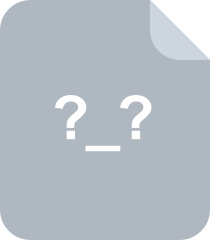
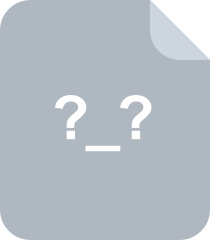
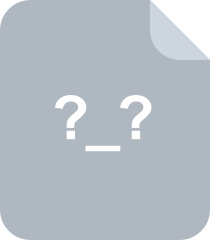
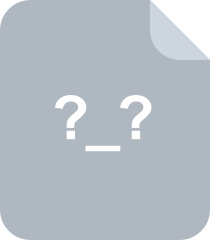
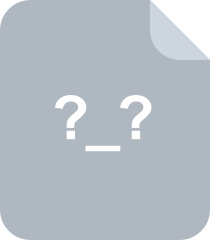
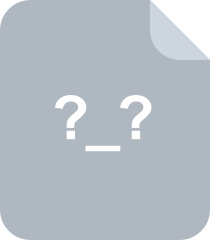
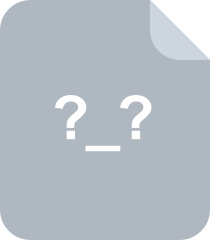
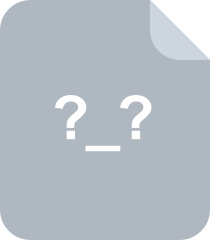
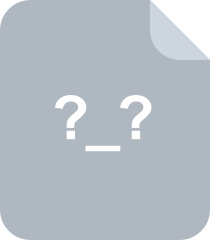
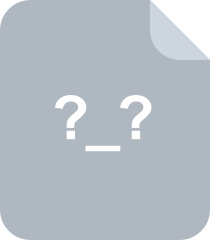
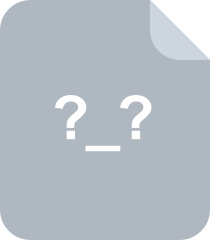
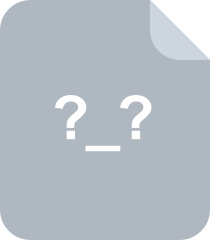
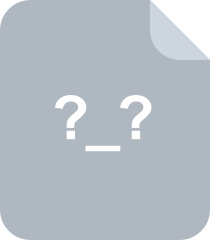
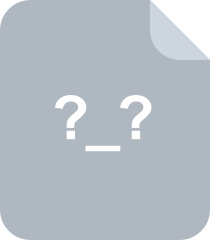
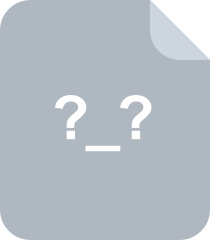
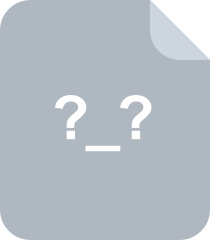
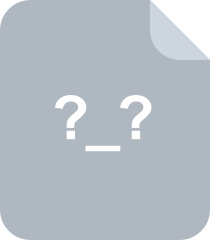
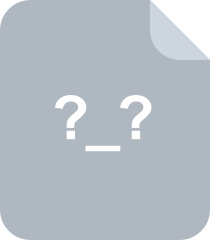
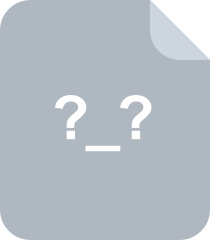
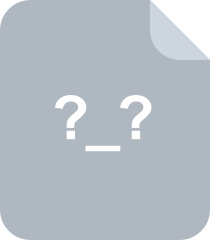
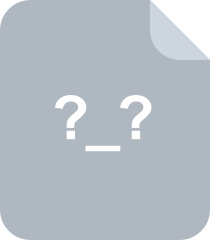
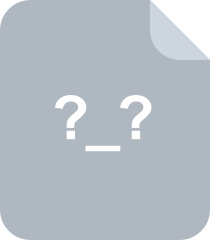
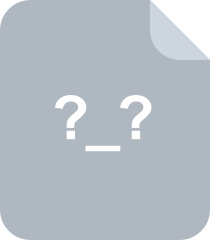
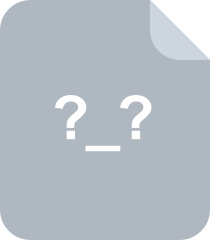
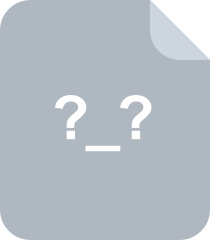
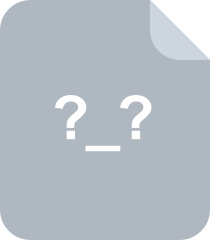
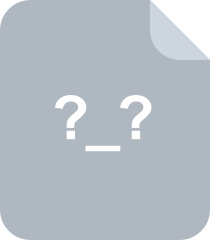
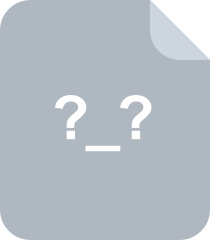
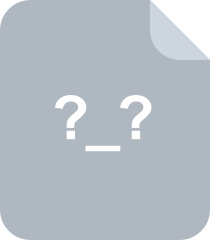
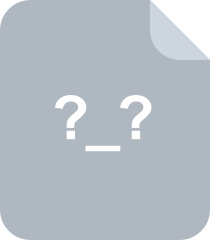
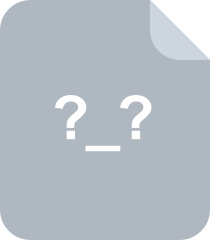
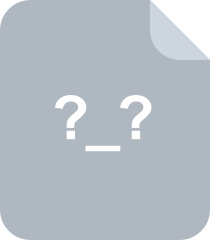
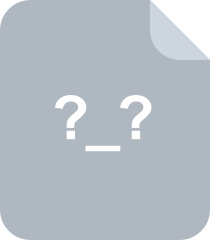
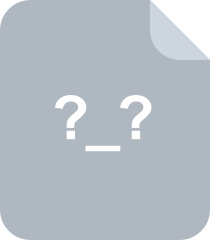
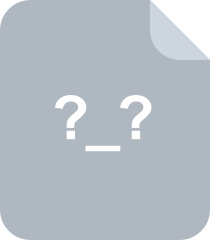
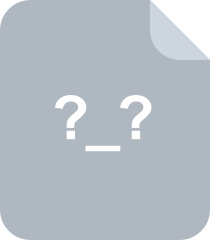
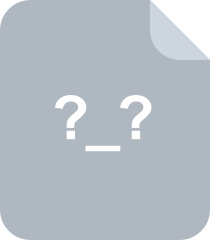
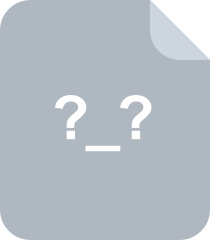
共 232 条
- 1
- 2
- 3
资源评论
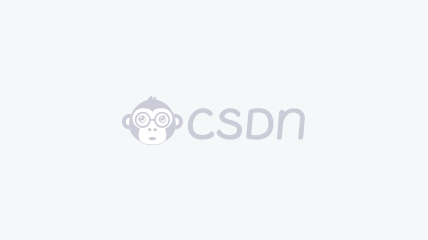

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

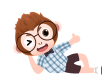
最新资源
- fish-kong,Yolov5-Instance-Seg-Tensorrt-CPP.zip
- 排球场地的排球识别 yolov7标记
- 微信小程序毕业设计-基于SSM的英语学习激励系统【代码+论文+PPT】.zip
- DOTA 中的 YOLOX 损失了 KLD (定向物体检测)(Rotated BBox)基于YOLOX的旋转目标检测.zip
- caffe-yolo-9000.zip
- 11sadsadfasfsafasf
- Android 凭证交换和更新协议 - “你只需登录一次”.zip
- 2024 年 ICONIP 展会.zip
- 微信小程序毕业设计-基于SSM的电影交流小程序【代码+论文+PPT】.zip
- 微信小程序毕业设计-基于SSM的食堂线上预约点餐小程序【代码+论文+PPT】.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


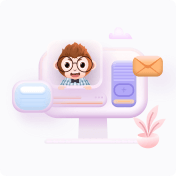
安全验证
文档复制为VIP权益,开通VIP直接复制
