#ifndef __PYWINTYPES_H__
#define __PYWINTYPES_H__
// If building under a GCC, tweak what we need.
#if defined(__GNUC__) && defined(_POSIX_C_SOURCE)
// python.h complains if _POSIX_C_SOURCE is already defined
#undef _POSIX_C_SOURCE
#endif
// early msvc versions complain about pragma #s it doesn't understand
// C:\mssdk\VC\INCLUDE\string.h(142) : warning C4616: #pragma warning : warning number '6059' out of range, must be
// between '4001' and '4999' and: C:\mssdk\include\wingdi.h(4340) : warning C4068: unknown pragma I doubt we ever care
// about that warning, so unconditionally nuke em!
#pragma warning(disable : 4616 4068)
// Python.h and Windows.h both protect themselves from multiple
// includes - so it is safe to do here (and provides a handy
// choke point for #include vagaries
// Some other warnings generated by MSVC6 which we don't care about
#if (PY_VERSION_HEX < 0x02060000)
#pragma warning(disable : 4035)
#endif
// windows rpc.h defines "small" as "char" which breaks Python's accu.h,
// so we undefine it before including python.
#ifdef small
#undef small
#endif
#include "Python.h"
// many many files need python's structmember.h, and its possible people
// #included windows.h before including us...
#ifdef WRITE_RESTRICTED
#undef WRITE_RESTRICTED
#endif
#include "structmember.h"
// and python's structmember.h #defines this, conflicting with windows.h
#ifdef WRITE_RESTRICTED
#undef WRITE_RESTRICTED
#endif
#include "windows.h"
#undef WRITE_RESTRICTED // stop anyone using the wrong one accidently...
// Helpers for our modules.
// Some macros to help the pywin32 modules co-exist in py2x and py3k.
// Creates and initializes local variables called 'module' and 'dict'.
#if (PY_VERSION_HEX < 0x03000000)
// Use to define the function itself (ie, its name, linkage, params)
#define PYWIN_MODULE_INIT_FUNC(module_name) extern "C" __declspec(dllexport) void init##module_name(void)
// If the module needs to early-exit on an error condition.
#define PYWIN_MODULE_INIT_RETURN_ERROR return;
// When the module has successfully initialized.
#define PYWIN_MODULE_INIT_RETURN_SUCCESS return;
// To setup the module object itself and the module's dictionary.
#define PYWIN_MODULE_INIT_PREPARE(module_name, functions, docstring) \
PyObject *dict, *module; \
if (PyWinGlobals_Ensure() == -1) \
return; \
if (!(module = Py_InitModule(#module_name, functions))) \
return; \
if (!(dict = PyModule_GetDict(module))) \
return;
#else
// py3k module helpers.
// Use to define the function itself (ie, its name, linkage, params)
#define PYWIN_MODULE_INIT_FUNC(module_name) extern "C" __declspec(dllexport) PyObject *PyInit_##module_name(void)
// If the module needs to early-exit on an error condition.
#define PYWIN_MODULE_INIT_RETURN_ERROR return NULL;
// When the module has successfully initialized.
#define PYWIN_MODULE_INIT_RETURN_SUCCESS return module;
// To setup the module object itself and the module's dictionary.
#define PYWIN_MODULE_INIT_PREPARE(module_name, functions, docstring) \
PyObject *dict, *module; \
static PyModuleDef module_name##_def = {PyModuleDef_HEAD_INIT, #module_name, docstring, -1, functions}; \
if (PyWinGlobals_Ensure() == -1) \
return NULL; \
if (!(module = PyModule_Create(&module_name##_def))) \
return NULL; \
if (!(dict = PyModule_GetDict(module))) \
return NULL;
#endif // PY_VERSION_HEX
// Helpers for our types.
#if (PY_VERSION_HEX < 0x03000000)
#define PYWIN_OBJECT_HEAD PyObject_HEAD_INIT(&PyType_Type) 0,
#define PYWIN_ATTR_CONVERT PyString_AsString
#else // Py3k definitions
// Macro to handle PyObject layout changes in Py3k
#define PYWIN_OBJECT_HEAD PyVarObject_HEAD_INIT(NULL, 0)
/* Attribute names are passed as Unicode in Py3k, so use a macro to
switch between string and unicode conversion. This function is not
documented, but is used extensively in the Python codebase itself,
so it's reasonable to assume it won't disappear anytime soon.
*/
#define PYWIN_ATTR_CONVERT (char *)_PyUnicode_AsString
/* Some API functions changed/removed in python 3.0
Definitions for the string functions are in stringobject.h,
but comments indicate that this header is likely to go away in 3.1.
*/
#define PyString_Check PyBytes_Check
#define PyString_Size PyBytes_Size
#define PyString_AsString PyBytes_AsString
#define PyString_AsStringAndSize PyBytes_AsStringAndSize
#define PyString_FromString PyBytes_FromString
#define PyString_FromStringAndSize PyBytes_FromStringAndSize
#define _PyString_Resize _PyBytes_Resize
#define PyString_AS_STRING PyBytes_AS_STRING
#define PyString_GET_SIZE PyBytes_GET_SIZE
#define PyString_Concat PyBytes_Concat
#define PyInt_Check PyLong_Check
#define PyInt_FromLong PyLong_FromLong
#define PyInt_AsLong PyLong_AsLong
#define PyInt_AS_LONG PyLong_AS_LONG
#define PyInt_FromSsize_t PyLong_FromSsize_t
#define PyInt_AsSsize_t PyLong_AsSsize_t
#define PyInt_AsUnsignedLongMask PyLong_AsUnsignedLongMask
#define PyNumber_Int PyNumber_Long
#endif // (PY_VERSION_HEX < 0x03000000)
// See PEP-353 - this is the "official" test...
#if PY_VERSION_HEX < 0x02050000 && !defined(PY_SSIZE_T_MIN)
// 2.3 and before have no Py_ssize_t
typedef int Py_ssize_t;
#define PyInt_FromSsize_t PyInt_FromLong
#define PyInt_AsSsize_t PyInt_AsLong
#define PY_SSIZE_T_MAX INT_MAX
#define PY_SSIZE_T_MIN INT_MIN
#endif
// Py_hash_t was introduced as the size of a pointer in python 3.2 - it
// was a simple long before that.
#if PY_VERSION_HEX < 0x03020000
typedef long Py_hash_t;
#else
typedef Py_ssize_t Py_hash_t;
#endif
// This only enables runtime checks in debug builds - so we use
// our own so we can enable it always should we desire...
#define PyWin_SAFE_DOWNCAST Py_SAFE_DOWNCAST
// Lars: for WAVEFORMATEX
#include "mmsystem.h"
// *** NOTE *** FREEZE_PYWINTYPES is deprecated. It used to be used
// by the 'freeze' tool, but now py2exe etc do a far better job, and
// don't require a custom built pywintypes DLL.
#ifdef FREEZE_PYWINTYPES
/* The pywintypes module is being included in a frozen .EXE/.DLL */
#define PYWINTYPES_EXPORT
#else
#ifdef BUILD_PYWINTYPES
/* We are building pywintypesxx.dll */
#define PYWINTYPES_EXPORT __declspec(dllexport)
#else
/* This module uses pywintypesxx.dll */
#define PYWINTYPES_EXPORT __declspec(dllimport)
#if defined(_MSC_VER)
#if defined(DEBUG) || defined(_DEBUG)
#pragma comment(lib, "pywintypes_d.lib")
#else
#pragma comment(lib, "pywintypes.lib")
#endif // DEBUG/_DEBUG
#endif // _MSC_VER
#endif // BUILD_PYWINTYPES
#endif // FREEZE_PYWINTYPES
#if (PY_VERSION_HEX >= 0x03000000)
// Py3k uses memoryview object in place of buffer
extern PYWINTYPES_EXPORT PyObject *PyBuffer_New(Py_ssize_t size);
extern PYWINTYPES_EXPORT PyObject *PyBuffer_FromMemory(void *buf, Py_ssize_t size);
#endif
// Formats a python traceback into a character string - result must be free()ed
PYWINTYPES_EXPORT char *GetPythonTraceback(PyObject *exc_type, PyObject *exc_value, PyObject *exc_tb);
#include <tchar.h>
/*
** Error/Exception handling
*/
extern PYWINTYPES_EXPORT PyObject *PyWinExc_ApiError;
// Register a Windows DLL that contains the messages in the specified range.
extern PYWINTYPES_EXPORT BOOL PyWin_RegisterErrorMessageModule(DWORD first, DWORD last, HINSTANCE hmod);
// Ge
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【资源说明】 基于Python知识图谱的心理咨询智能问答系统源码+数据集+详细文档(高分毕业设计).zip基于Python知识图谱的心理咨询智能问答系统源码+数据集+详细文档(高分毕业设计).zip基于Python知识图谱的心理咨询智能问答系统源码+数据集+详细文档(高分毕业设计).zip 【备注】 1、该资源内项目代码都经过测试运行成功,功能ok的情况下才上传的,请放心下载使用! 2、本项目适合计算机相关专业(如软件工程、计科、人工智能、通信工程、自动化、电子信息等)的在校学生、老师或者企业员工下载使用,也可作为毕设项目、课程设计、作业、项目初期立项演示等,当然也适合小白学习进阶。 3、如果基础还行,可以在此代码基础上进行修改,以实现其他功能,也可直接用于毕设、课设、作业等。 欢迎下载,沟通交流,互相学习,共同进步!
资源推荐
资源详情
资源评论
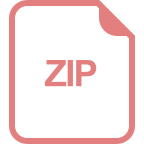
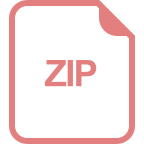
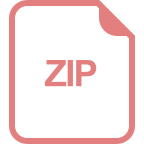
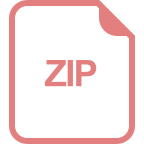
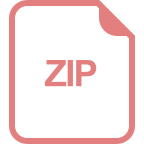
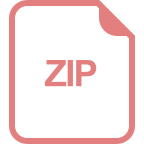
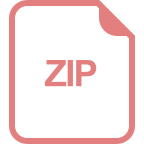
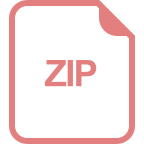
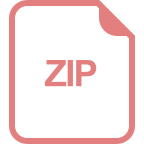
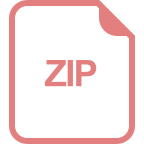
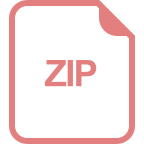
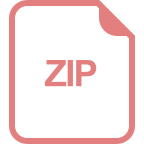
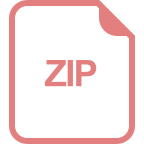
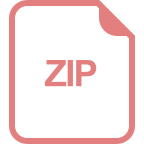
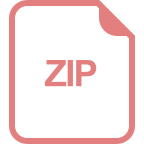
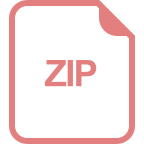
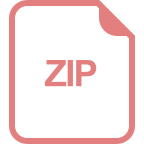
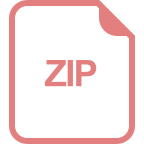
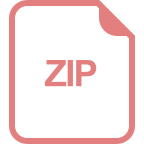
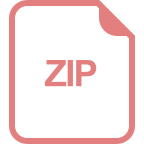
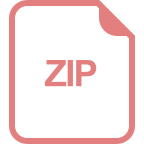
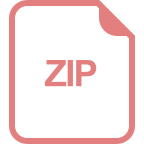
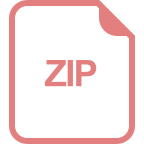
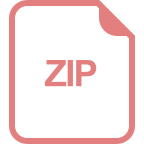
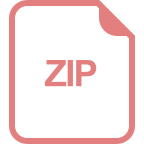
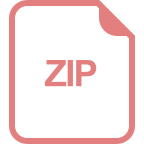
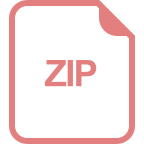
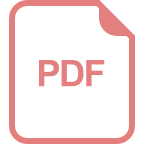
收起资源包目录

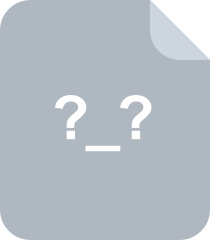
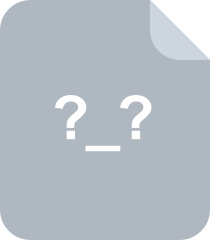
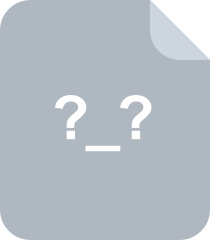
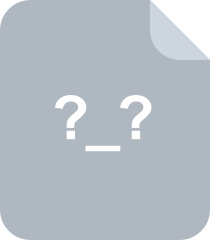
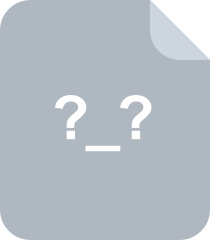
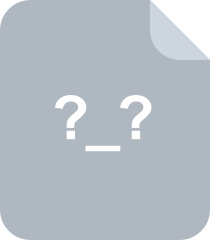
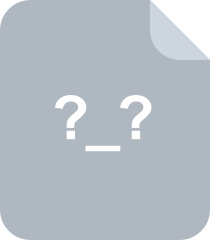
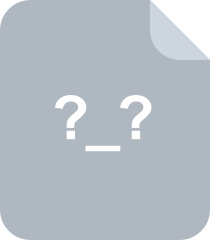
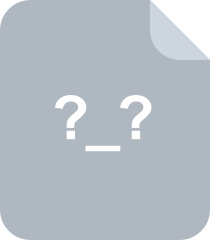
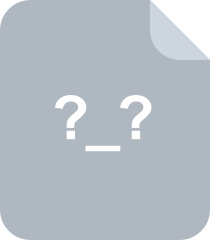
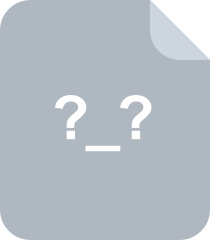
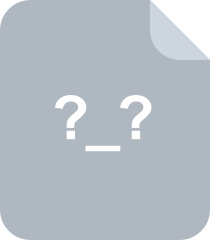
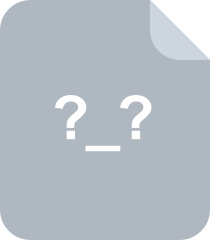
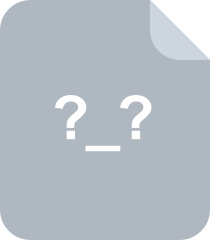
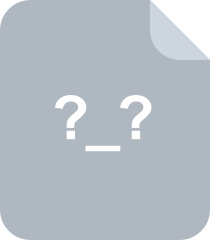
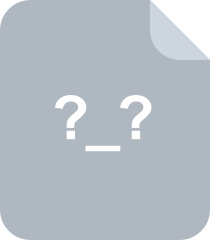
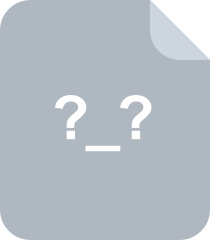
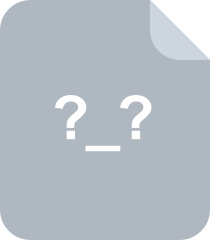
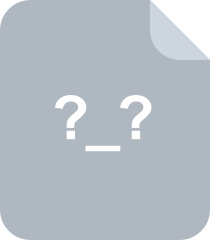
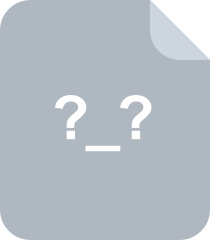
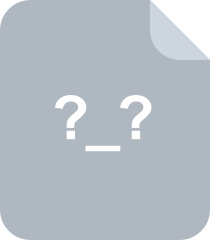
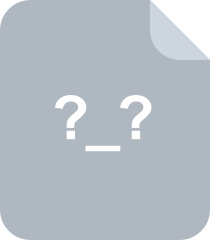
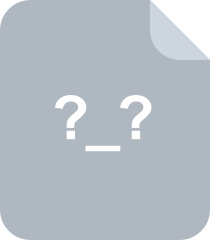
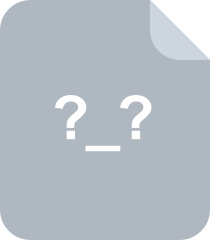
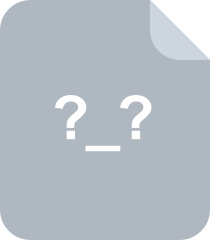
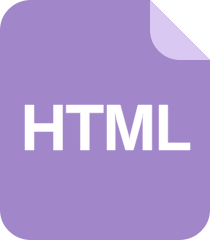
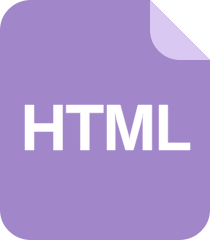
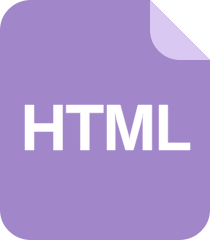
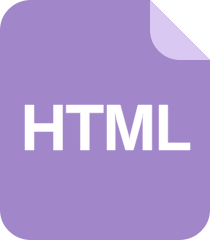
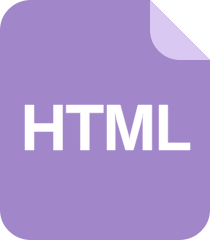
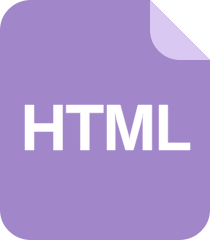
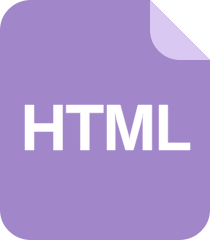
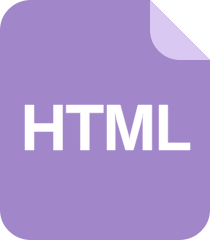
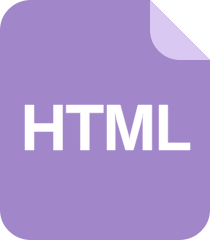
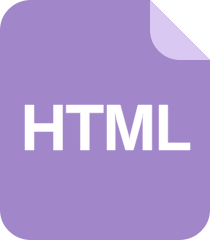
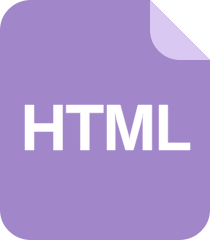
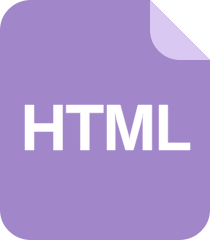
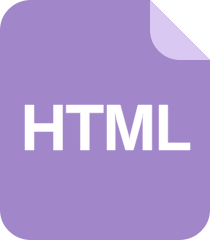
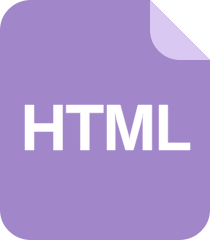
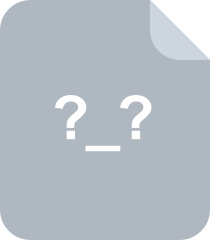
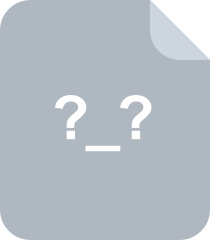
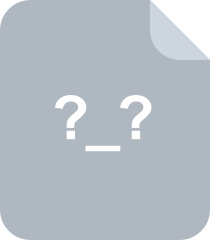
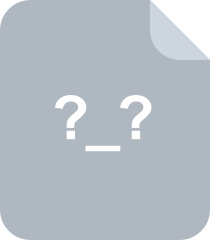
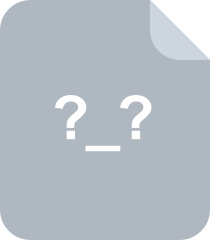
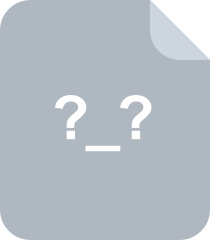
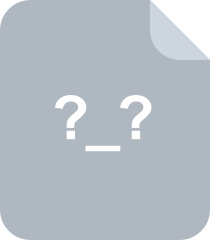
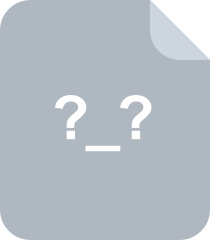
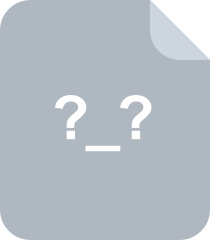
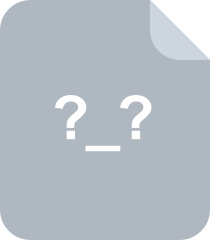
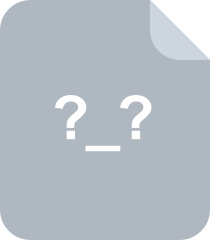
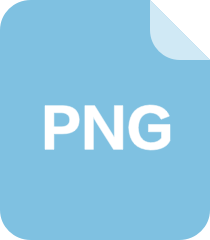
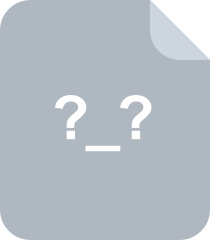
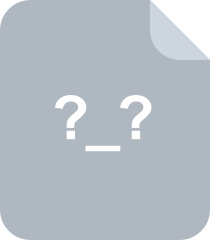
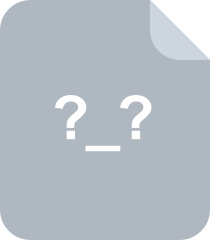
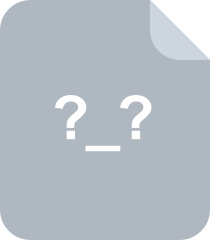
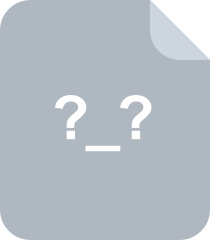
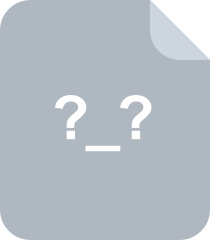
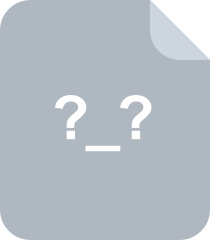
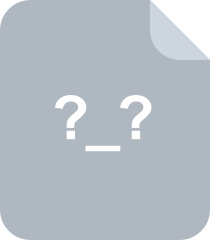
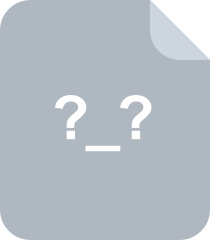
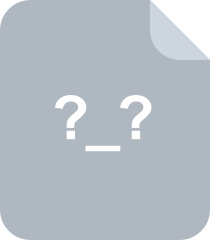
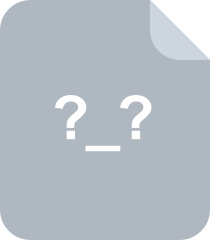
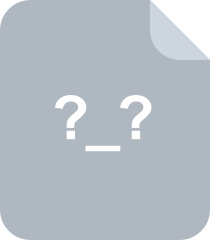
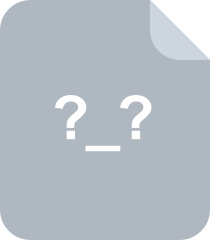
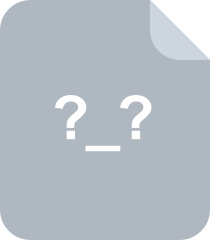
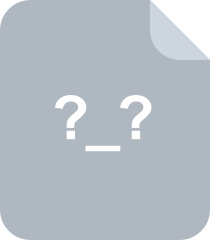
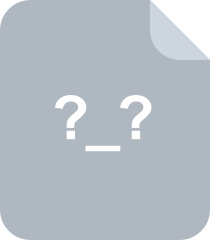
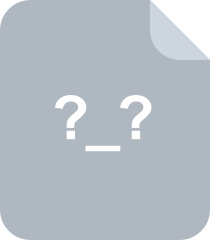
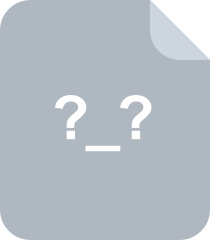
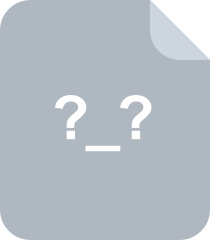
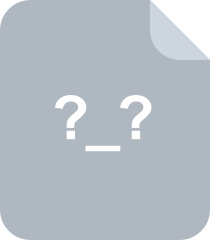
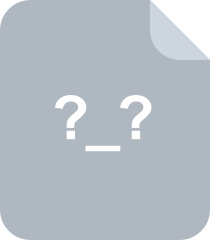
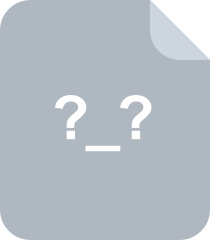
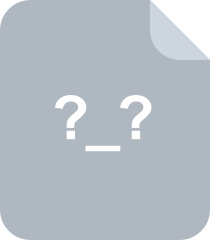
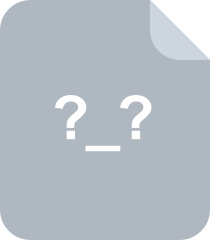
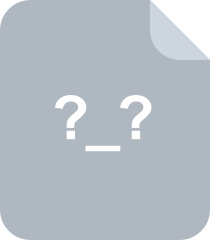
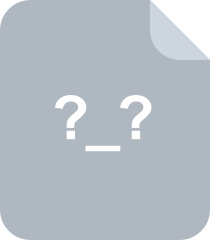
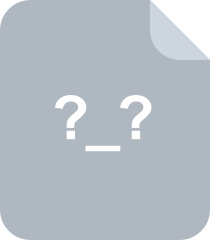
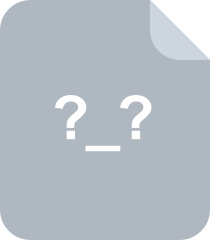
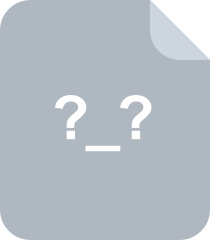
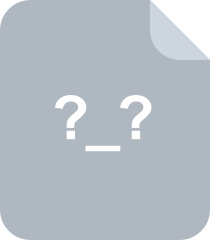
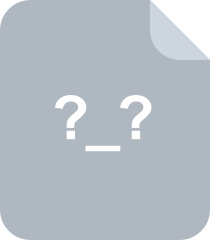
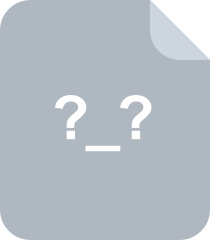
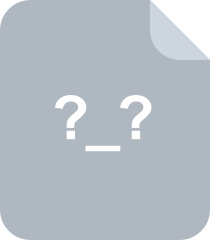
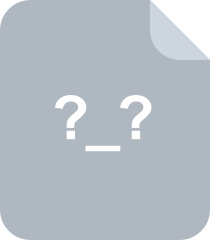
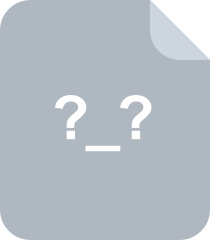
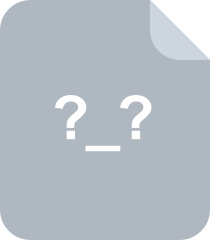
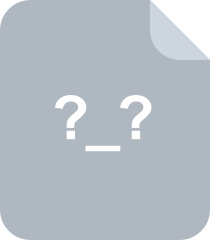
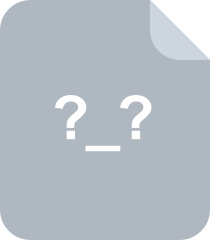
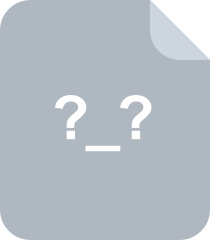
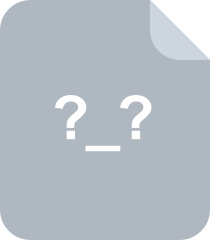
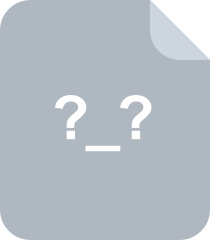
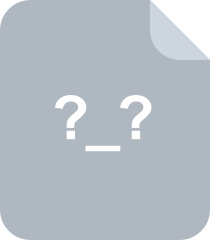
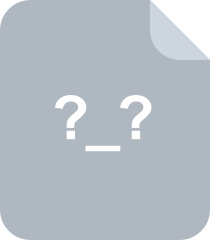
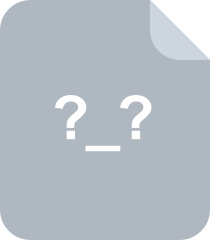
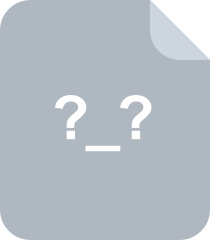
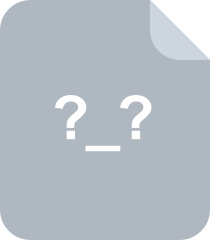
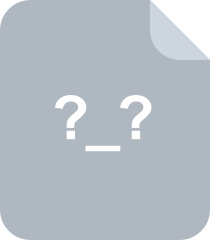
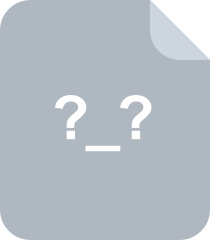
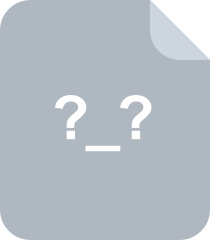
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
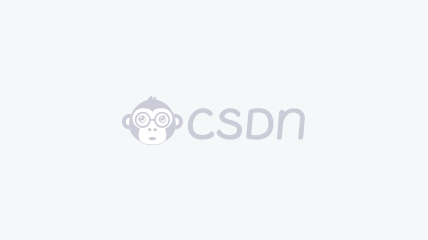

不走小道
- 粉丝: 3367
- 资源: 5054
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

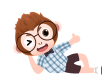
最新资源
- 国开-网络安全技术-实验一 搭建网络安全演练环境.doc
- 国开-网络安全技术-实验八 SQL注入工具使用.doc
- 用python写的一些小工具
- 国开-微积分基础-微积分基础下载作业word版.doc
- 国开-微积分基础-大作业word版.docx
- 排序算法 Sorting 查找算法
- HTML5实现好看的艺术设计师作品展示模板.zip
- HTML5实现好看的音乐乐队演出票务网站模板.zip
- HTML5实现好看的营销推广公司网站模板.zip
- HTML5实现好看的音频播客个人主页模板.zip
- HTML5实现好看的婴儿护理中心网站模板.zip
- HTML5实现好看的应用程序设计网站模板.zip
- HTML5实现好看的游戏碟片厂商官网模板.zip
- HTML5实现好看的游轮帆船租赁网站模板.zip
- HTML5实现好看的瑜伽培训运动网站模板.zip
- HTML5实现好看的游艇租赁服务公司网站模板.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


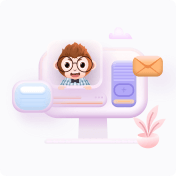
安全验证
文档复制为VIP权益,开通VIP直接复制
