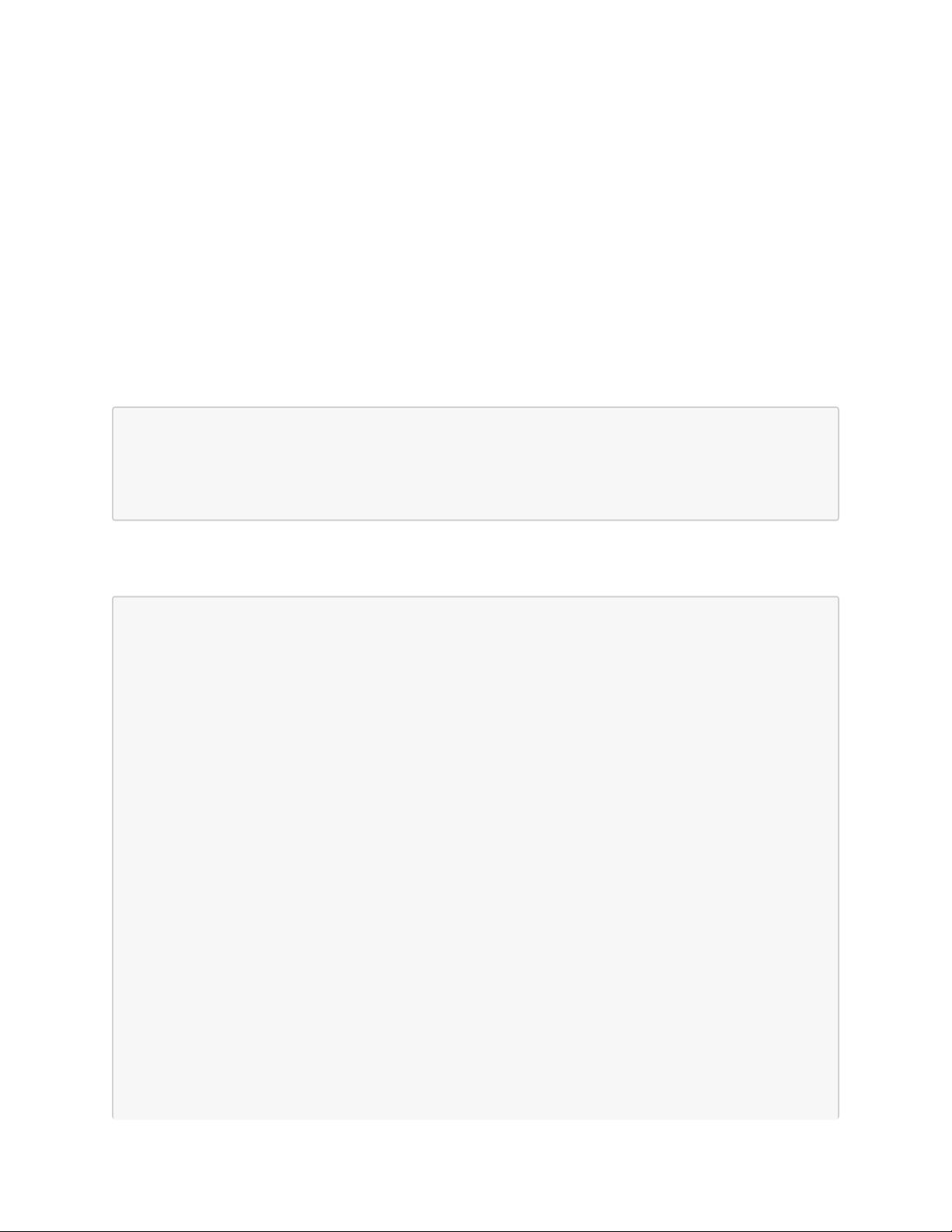
LSTM
2023 年 4 月 26 日
1 多步时间序列预测-LSTM-手术风险
[1]: import pandas as pd
import numpy as np
from numpy import array
%matplotlib inline
1.1 - 1:导入数据集,划分数据集
[2]: n_steps_in, n_steps_out = 9,1
data = pd.read_excel("SGH_data(1).xls",usecols="C:K,N")
print(data.head())
data = data.values
# 划分训练集与测试集
traindata = data[0:6500,:]
testdata = data[6500:len(data),:]
x_train = traindata[:,0:n_steps_in]
y_train = traindata[:,n_steps_in:n_steps_in+n_steps_out]
y_train = y_train.ravel()
y_train[y_train>1] = 1
print("shape of train data: ",x_train.shape,y_train.shape)
x_test = testdata[:,0:n_steps_in]
y_test = testdata[:,n_steps_in:n_steps_in+n_steps_out]
y_test = y_test.ravel()
1
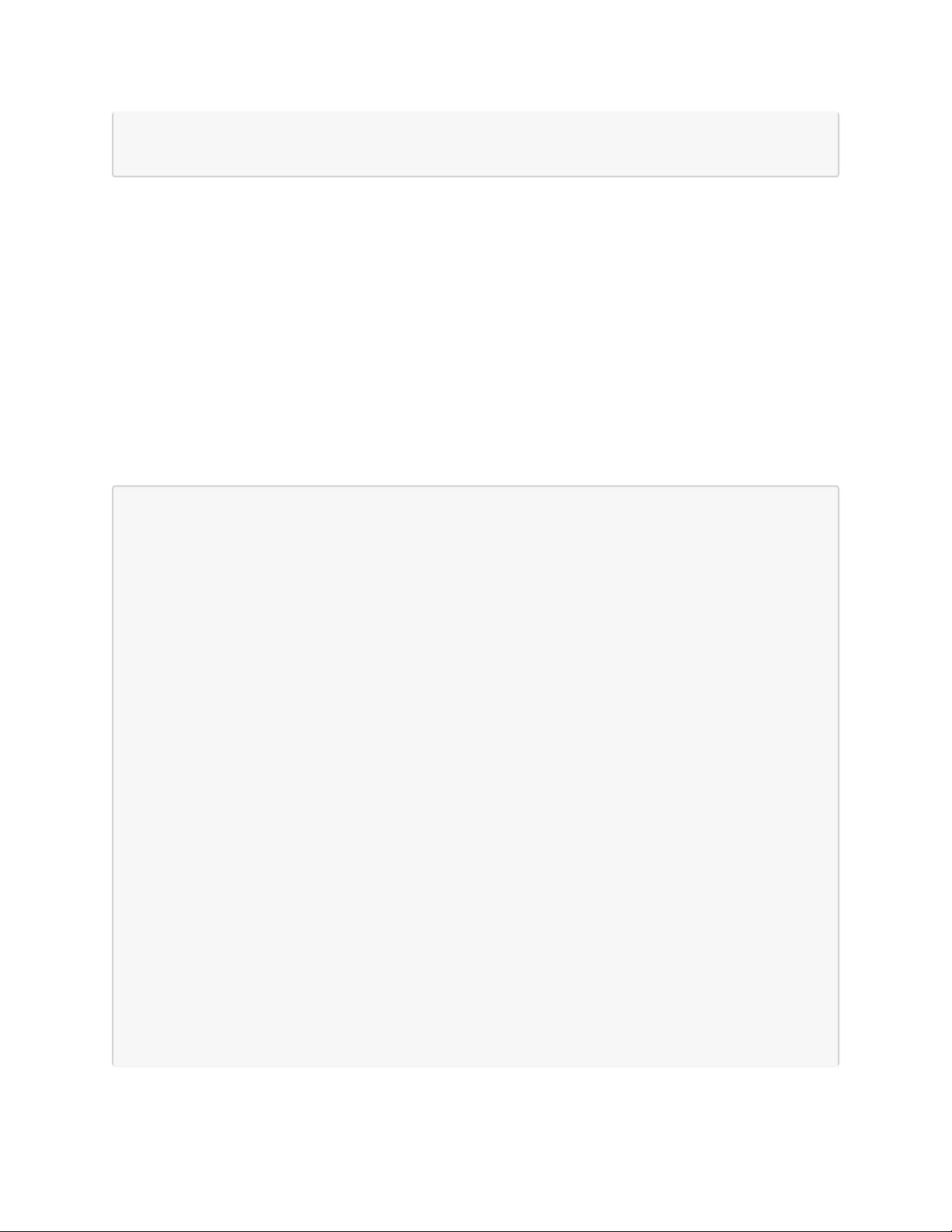
y_test[y_test>1] = 1
print("shape of test data: ",x_test.shape,y_test.shape)
G age surgeon proced type reop diabetes bleeding resuture in/out
0 1 46 1 4 2 0 0 0 0 0
1 1 60 1 1 1 0 0 0 0 0
2 1 44 1 1 1 0 0 0 0 0
3 1 61 1 1 2 0 0 0 0 0
4 1 74 1 1 1 0 1 0 0 0
shape of train data: (6500, 9) (6500,)
shape of test data: (494, 9) (494,)
1.2 - 2:建模进行二分类的预测
[3]: import time,math
from keras.models import Sequential
from keras.layers import Dense
from keras.layers import LSTM
import keras.metrics as km
# LSTM
def␣
↪MODEL_01classification(x_train,x_test,y_train,y_test,n_steps_in,n_steps_out,Epochs,Hidden):
↪
n_features = 1
x_train = x_train.reshape((x_train.shape[0], x_train.shape[1], n_features))
print("after reshape, the train data shape:",x_train.shape)
x_test = x_test.reshape((x_test.shape[0], x_test.shape[1], n_features))
print("after reshape, the test data shape: ",x_test.shape)
model = Sequential()
model.add(LSTM(Hidden, activation='relu',␣
↪input_shape=(n_steps_in,n_features)))
model.add(Dense(n_steps_out, activation='sigmoid'))
#model.add(Dense(n_steps_out, activation='relu'))
2
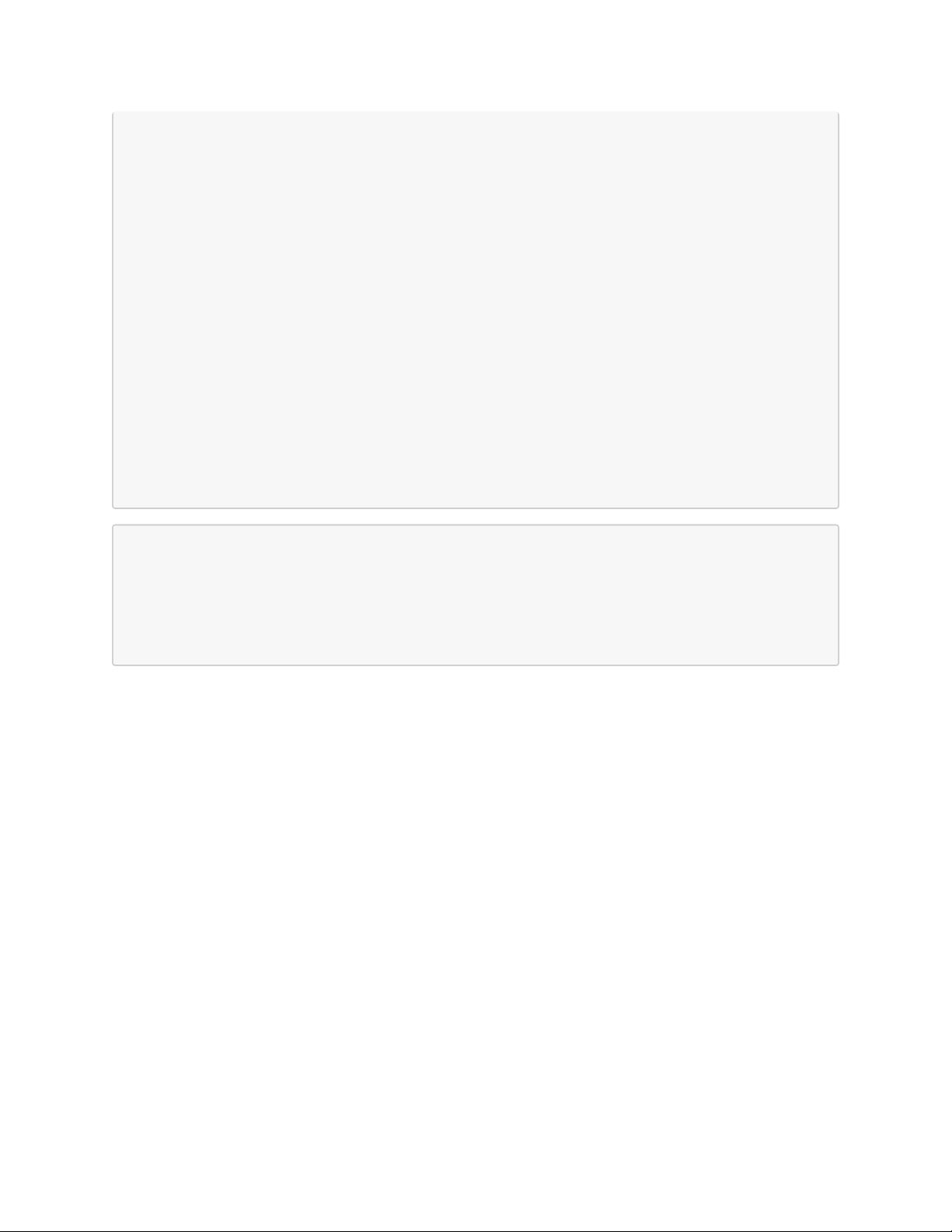
model.compile(optimizer='adam', loss='binary_crossentropy',metrics=[km.
↪BinaryAccuracy()])
model.summary()
start_time=time.time()
model.fit(x_train, y_train, epochs=Epochs,batch_size=64, verbose=2,␣
↪shuffle=False)
y_predicttrain = model.predict(x_train)
y_predicttest = model.predict(x_test)
print("Total time for calculating time sequence is",time.time()-start_time)
allpre = np.concatenate((y_predicttrain,y_predicttest))
alldata = np.concatenate((y_train,y_test))
return allpre, alldata
[4]: #Num_Exp = 1
Epochs = 30
Hidden = 10
allpre,alldata =␣
↪MODEL_01classification(x_train,x_test,y_train,y_test,n_steps_in,n_steps_out,Epochs,Hidden)
after reshape, the train data shape: (6500, 9, 1)
after reshape, the test data shape: (494, 9, 1)
WARNING:tensorflow:Layer lstm will not use cuDNN kernels since it doesn't meet
the criteria. It will use a generic GPU kernel as fallback when running on GPU.
Model: "sequential"
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
lstm (LSTM) (None, 10) 480
dense (Dense) (None, 1) 11
=================================================================
Total params: 491
Trainable params: 491
Non-trainable params: 0
3
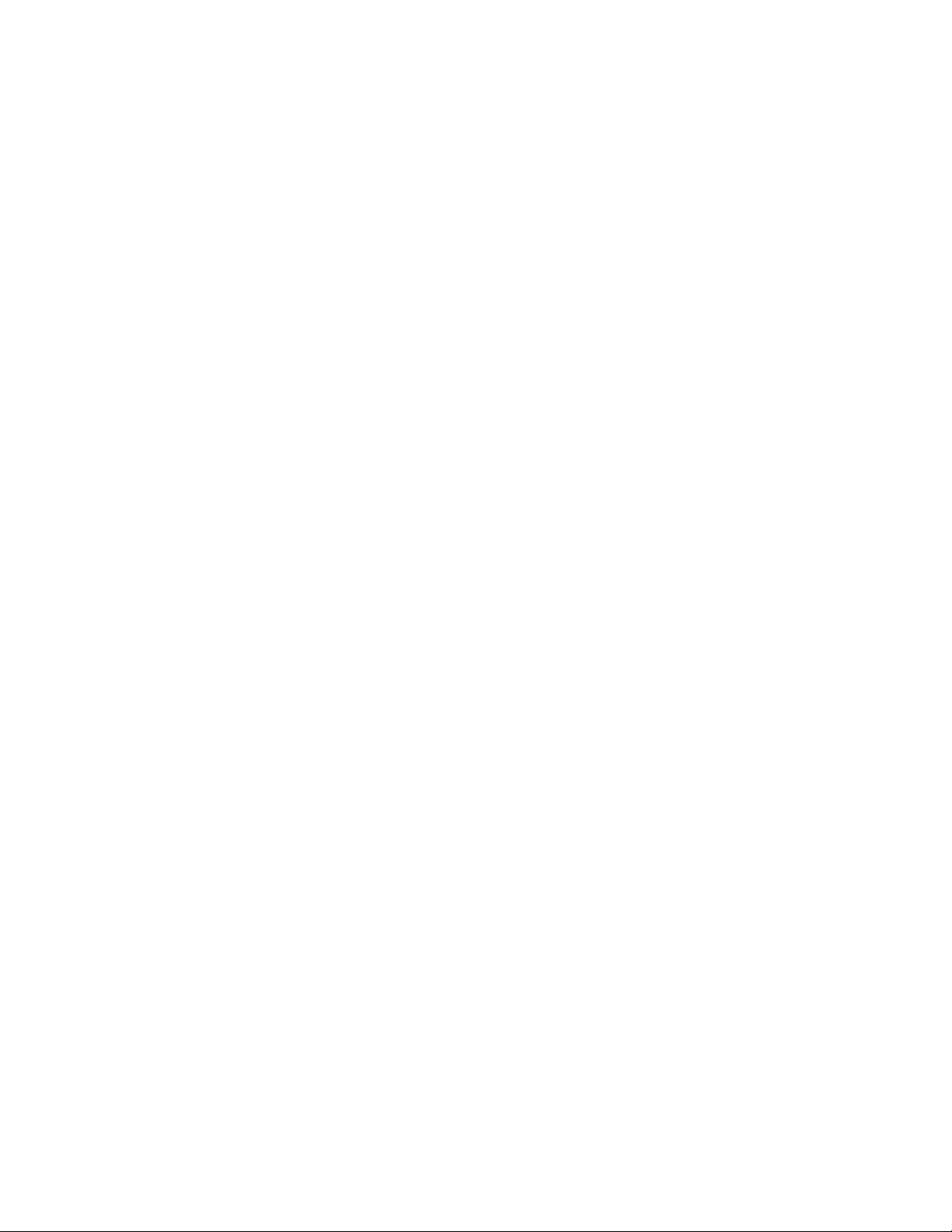
_________________________________________________________________
Epoch 1/30
102/102 - 7s - loss: 0.4891 - binary_accuracy: 0.7486 - 7s/epoch - 72ms/step
Epoch 2/30
102/102 - 6s - loss: 0.3688 - binary_accuracy: 0.8855 - 6s/epoch - 55ms/step
Epoch 3/30
102/102 - 6s - loss: 0.3522 - binary_accuracy: 0.8855 - 6s/epoch - 54ms/step
Epoch 4/30
102/102 - 6s - loss: 0.3384 - binary_accuracy: 0.8855 - 6s/epoch - 54ms/step
Epoch 5/30
102/102 - 5s - loss: 0.3239 - binary_accuracy: 0.8855 - 5s/epoch - 54ms/step
Epoch 6/30
102/102 - 6s - loss: 0.3159 - binary_accuracy: 0.8855 - 6s/epoch - 54ms/step
Epoch 7/30
102/102 - 5s - loss: 0.3127 - binary_accuracy: 0.8855 - 5s/epoch - 54ms/step
Epoch 8/30
102/102 - 6s - loss: 0.3109 - binary_accuracy: 0.8855 - 6s/epoch - 54ms/step
Epoch 9/30
102/102 - 5s - loss: 0.3098 - binary_accuracy: 0.8855 - 5s/epoch - 54ms/step
Epoch 10/30
102/102 - 6s - loss: 0.3089 - binary_accuracy: 0.8855 - 6s/epoch - 54ms/step
Epoch 11/30
102/102 - 5s - loss: 0.3084 - binary_accuracy: 0.8855 - 5s/epoch - 54ms/step
Epoch 12/30
102/102 - 5s - loss: 0.3078 - binary_accuracy: 0.8855 - 5s/epoch - 54ms/step
Epoch 13/30
102/102 - 5s - loss: 0.3075 - binary_accuracy: 0.8855 - 5s/epoch - 54ms/step
Epoch 14/30
102/102 - 6s - loss: 0.3071 - binary_accuracy: 0.8855 - 6s/epoch - 54ms/step
Epoch 15/30
102/102 - 6s - loss: 0.3068 - binary_accuracy: 0.8857 - 6s/epoch - 54ms/step
Epoch 16/30
102/102 - 5s - loss: 0.3064 - binary_accuracy: 0.8857 - 5s/epoch - 54ms/step
Epoch 17/30
102/102 - 5s - loss: 0.3062 - binary_accuracy: 0.8858 - 5s/epoch - 54ms/step
Epoch 18/30
102/102 - 6s - loss: 0.3060 - binary_accuracy: 0.8858 - 6s/epoch - 54ms/step
4
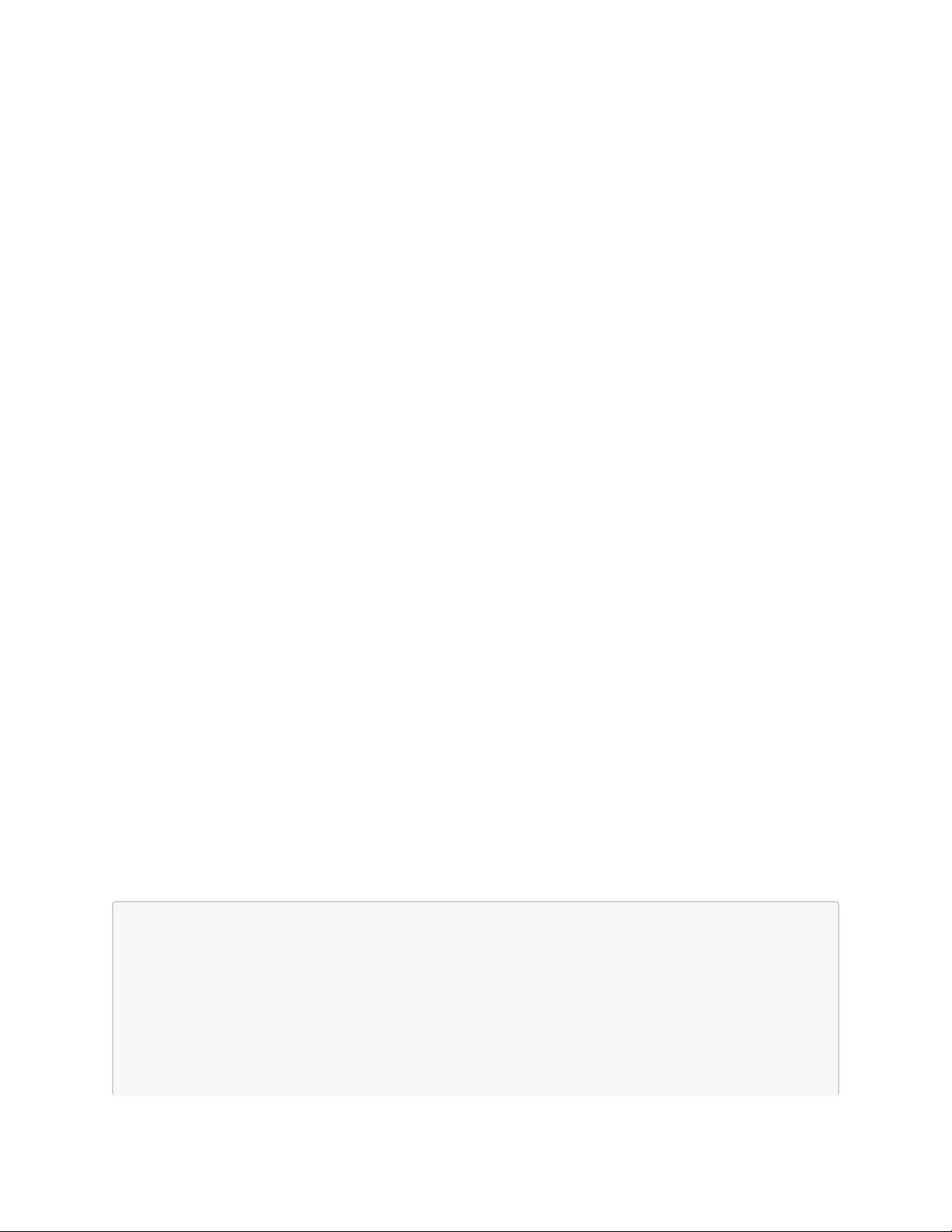
Epoch 19/30
102/102 - 6s - loss: 0.3058 - binary_accuracy: 0.8858 - 6s/epoch - 54ms/step
Epoch 20/30
102/102 - 6s - loss: 0.3056 - binary_accuracy: 0.8858 - 6s/epoch - 54ms/step
Epoch 21/30
102/102 - 6s - loss: 0.3055 - binary_accuracy: 0.8858 - 6s/epoch - 55ms/step
Epoch 22/30
102/102 - 5s - loss: 0.3054 - binary_accuracy: 0.8858 - 5s/epoch - 54ms/step
Epoch 23/30
102/102 - 5s - loss: 0.3052 - binary_accuracy: 0.8858 - 5s/epoch - 54ms/step
Epoch 24/30
102/102 - 6s - loss: 0.3051 - binary_accuracy: 0.8858 - 6s/epoch - 54ms/step
Epoch 25/30
102/102 - 6s - loss: 0.3050 - binary_accuracy: 0.8858 - 6s/epoch - 54ms/step
Epoch 26/30
102/102 - 5s - loss: 0.3049 - binary_accuracy: 0.8858 - 5s/epoch - 54ms/step
Epoch 27/30
102/102 - 6s - loss: 0.3048 - binary_accuracy: 0.8858 - 6s/epoch - 55ms/step
Epoch 28/30
102/102 - 5s - loss: 0.3047 - binary_accuracy: 0.8860 - 5s/epoch - 54ms/step
Epoch 29/30
102/102 - 6s - loss: 0.3046 - binary_accuracy: 0.8860 - 6s/epoch - 54ms/step
Epoch 30/30
102/102 - 6s - loss: 0.3045 - binary_accuracy: 0.8860 - 6s/epoch - 54ms/step
204/204 [==============================] - 2s 7ms/step
16/16 [==============================] - 0s 7ms/step
Total time for calculating time sequence is 169.34249925613403
预测结果如图
[5]: import matplotlib.pyplot as plt
colors = np.random.rand(allpre.shape[0])
x_data = np.linspace(0,allpre.shape[0],num=allpre.shape[0])
line = np.linspace(0.5,0.5,allpre.shape[0])
plt.plot(x_data,line,c="red")
plt.scatter(x_data, allpre,s=10,c=colors)
5