function varargout = thickness(y,u,r)
% THICKNESS(Y,U,R): Calculates the different boundary layer thicknesses
% for a rake of coordiantes Y, velocities U, and densities R (optional)
% in COLUMN form. The integral thicknesses from Schlichting are derived
% from trapezoidal integrations. The 99% thicknesses are robust metrics
% for the standard 'delta99'. Three BL profiles are provided as sample.
%
% INTEGRAL, INCOMPRESSIBLE:
% (1) Displacement (incompressible)
% (2) Momentum (incompressible)
% (3) Energy (incompressible)
%
% INTEGRAL, COMPRESSIBLE
% (4) Displacement (compressible: ONLY when R is passed)
% (5) Momentum (compressible: ONLY when R is passed)
% (6) Energy (compressible: ONLY when R is passed)
%
% GEOMETRIC, ROBUST DELTA 99
% (7) 99% variance thickness (4 for incompressible)
% (8) 99% asymptotic thickness (5 for incompressible)
%
% If zero outputs are passed, a plot is generated with all thicknesses.
% If one output is passed, all thicknesses are returned in vector form.
% If multiple outputs are passed, the indexed thicknesses are returned.
%
%
% EXAMPLES:
%
% thickness;
% t = thickness(Y,U);
% t = thickness(Y,U,R);
% t = thickness([Y,U,R]);
% [t1,...,tn] = thickness(...);
%
%
% Miguel Moreno, August 2024
%% PARSE INPUTS
if nargin==0
% Load sample BL data
[y,u,r] = sample(1); % CHANGE: 1, 2 or 3
compressible = true;
n = length(y);
% Compressible mode
elseif nargin==3
compressible = true;
n = length(y);
% Incompressible mode
elseif nargin==2
compressible = false;
n = length(y);
% Parse matrix
else
[y,u,r,n,compressible] = checkinputs(y);
end
%% CALCULATE THICKNESSES
% INTEGRAL THICKNESSES
u = u/u(n);
if compressible
% Calculate [displacement, momentum, energy] for INCOMP. and COMP.
m = 8;
r = r/r(n);
t(m) = 0;
t(1:6) = trapz(y-y(1), [1-u, u.*(1-u), u.*(1-u.*u), 1-r.*u, ...
r.*u.*(1-u), r.*u.*(1-u.*u)]);
else
% Calculate incompressible [displacement, momentum, energy]
m = 5;
t(m) = 0;
t(1:3) = trapz(y-y(1),[1-u, u.*(1-u), u.*(1-u.*u)]);
end
% VARIANCE DELTA 99
for i = n:-1:1
% Find index with maximum cumulative self-variance from freestream
if max(abs(u(i:n)-sum(u(i:n))/(n-i+1)))>1e-2
% When maximum variance exceeds 1%, stop search
t(m-1) = y(i+1);
break
end
end
% ASYMPTOTIC DELTA 99
% Use last 10% of the data points to calculate asymptote
i = ceil(0.9*n);
% Asymptotic delta99: 1% discrepancy with freestream asymptote
t(m) = y(min(n,find(abs(u-lval(lfit(y(i:n),u(i:n)),y))>1e-2,1,'last')+1));
%% PARSE OUTPUTS
% No-input case
n = nargout;
if nargin==0
% Display sample results
createfigure(y,u,t,m)
% Empty workspace
switch n
case 0
clear
otherwise
for i = 1:n
varargout{i} = []; %#ok
end
end
return
end
% Return thicknesses
switch n
case 0
% Prompt to workspace and PLOT results
varargout{1} = t;
createfigure(y,u,t,m)
case 1
% Vector mode
varargout{1} = t;
otherwise
% Individual entries
for i = 1:n
if i>m
varargout{i} = []; %#ok
else
varargout{i} = t(i); %#ok
end
end
end
end
%% SUBFUNCTIONS
% Load sample BL
function [y,u,r] = sample(n)
% Three examples of turbulent BLs
switch n
% Accelerated BL with SBLIs
case 1
y = [0;1.99999999495049e-06;4.15308113588253e-06;6.47095794192865e-06;8.96624169399729e-06;1.16525061457651e-05;1.45443646033527e-05;1.76575504156062e-05;2.10089947358938e-05;2.46169256570283e-05;2.85009682556847e-05;3.26822446368169e-05;3.71834867110010e-05;4.20291762566194e-05;4.72456558782142e-05;5.28612836205866e-05;5.89065821259283e-05;6.54144096188247e-05;7.24201381672174e-05;7.99618283053860e-05;8.80804509506561e-05;9.68201056821272e-05;0.000106228268123232;0.000116356015496422;0.000127258346765302;0.000138994422741234;0.000151627929881215;0.000165227422257885;0.000179866663529538;0.000195625019841827;0.000212587925489061;0.000230847290367819;0.000250502052949742;0.000271658616838977;0.000294431491056457;0.000318943930324167;0.000345328473486006;0.000373727700207382;0.000404295074986294;0.000437195616541430;0.000472606858238578;0.000510719662997872;0.000551739416550845;0.000595887016970664;0.000643399835098535;0.000694533227942884;0.000749561819247901;0.000808780547231436;0.000872506760060787;0.000941081438213587;0.00101487082429230;0.00109426875133067;0.00117969815619290;0.00127161329146475;0.00137050217017531;0.00147688854485750;0.00159133540000767;0.00171444611623883;0.00184686866123229;0.00198929803445935;0.00214248010888696;0.00230721384286881;0.00248435628600419;0.00267482572235167;0.00287960562855005;0.00309974886476994;0.00333638256415725;0.00359071278944612;0.00386402895674109;0.00415770849213004;0.00447322381660342;0.00481214607134461;0.00517615070566535;0.00556702492758632;0.00598667142912746;0.00643711583688855;0.00692051183432341;0.00743914581835270;0.00799544714391232;0.00859198812395334;0.00923149287700653;0.00991684012115002;0.0106510706245899;0.0114373881369829;0.0122791621834040;0.0131799355149269;0.0141434157267213;0.0151734827086329;0.0162741821259260;0.0174497254192829;0.0187044795602560;0.0200429558753967;0.0214698072522879;0.0229898076504469;0.0246078334748745;0.0263288393616676;0.0281578414142132;0.0300998743623495;0.0321599654853344;0.0343430899083614;0.0366541221737862;0.0390977896749973;0.0416786149144173;0.0444008558988571;0.0472684428095818;0.0502848960459232;0.0534532591700554;0.0567760281264782;0.0602550692856312;0.0638915300369263;0.0676857829093933;0.0716373175382614;0.0757447183132172;0.0800055488944054;0.0844163522124291;0.0889725461602211;0.0936684533953667;0.0984972491860390;0.103450976312160;0.108520574867725;0.113695889711380;0.118965782225132;0.124318152666092;0.129740089178085;0.135217934846878;0.140737473964691;0.146284013986588;0.151842594146729;0.157398104667664;0.162935495376587;0.168439894914627;0.173896789550781;0.179292127490044;0.184612601995468;0.189845591783524;0.194979354739189;0.200003176927567;0.204907327890396;0.209683179855347;0.214323252439499;0.218821167945862];
u = [-0.551870584487914;-0.851362402229443;-0.616410248775832;0.211779454477307;1.62932274355219;3.51114881673560;5.58005730356145;7.53683961063929;9.23286236295779;10.6722495724363;11.9145172276030;13.0177871338018;14.0253934586106;14.9673981026777;15.8642899976517;16.7302962650622;17.5747383372861;18.4036303169777;19.2257806368195;20.0472645223029;20.8705675749267;21.6984100425310;22.5334569043569;23.3779135117346;24.2339127894409;25.1033702800103;25.9879955932996;26.8894163925308;27.8091097351745;28.7484497651058;29.7087134433067;30.6910023530747;31.6964239135751;32.7262554298042;33.7818926085127;34.8644356628873;35.9747064908296;37.1134546921931;38.2817161884409;39.4809124375051;40.7135905669582;41.9841177543230;43.3001577142940;44.6734643671199;46.1192868932895;47.6562440573638;49.3071599672873;51.0913807544153;53.0218369689450;55.1124258842637;57.3814645256371;59.8506562

天天Matlab代码科研顾问
- 粉丝: 3w+
- 资源: 2504
最新资源
- 2022年江苏省高职组信息安全管理与评估竞赛2卷
- 基于UC3842芯片的AC-DC反激式开关电源 multisim仿真图源文件
- 小型博客系统的设计与实现
- 在线博客系统,个人学习整理,仅供参考
- 七自由度车辆动力学模型验证(Dugoff轮胎模型,B08-01基础上建模) 1.软件: MATLAB 2018以上;CarSim 2020.0 2.介绍: 基于Dugoff轮胎模型和车身动力学公式,搭
- 基于SSM的个人博客系统的设计与实现.zip
- Java源码springboot+vue二手图书交易平台-毕业设计论文-大作业.zip
- 【二维码识别】基于matlab GUI机器学习二维码生成与识别【含Matlab源码 635期】.mp4
- 数字信号处理期末复习基础知识
- 2022年江苏省高职组信息安全管理与评估竞赛3卷
- 批量一键取关公众号我们可以选择用最简单的方式 1.手机上按键精灵模拟实现 2.PC电脑上模拟
- PFC-LLC谐振开关电源设计方案整套学习资料 程序+仿真+硬件软件说明报告+原理图+计算书等等 注:该方案性价比很高,一套资料下来可以自己做个实物验证,要想看细节可以咨询我,我给你看资料的详细展示视
- 数字信号处理复习总结-最终版 --【60页】.doc
- ZenIdentityServer4 资源拥有者模式
- 利用pytorch搭建卷积神经网络(CNN)训练简单手写数字数据集(MNIST)
- jz2440衔接12期,uboot,文件系统,内核移植
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


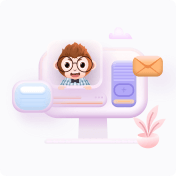