#include <windows.h>
#include <math.h>
#include <gl\gl.h>
#include "Class.h"
#include "fmod.h"
#include "texture.h"
//各变量定义:
extern TextureTga playertex[3]; // 纹理数组,保存纹理名字
extern TextureTga computertex[3];
extern TextureTga ammunitiontex[4];
extern TextureTga awardtex[3];
extern TextureTga othertex[4];
extern PlayerPlane myPlane; // 我方飞机
extern ComputerPlane computers[MAX_COMPUTER]; // 电脑飞机数组
extern Ammunition ammunitions[MAX_AMMUNITION]; // 子弹数组
extern Award awards[MAX_AWARD]; // 物品数组
int ammunitionIndex=0;
int awardIndex=0;
extern FSOUND_SAMPLE *sound_1; // 发射子弹的声音指针
extern FSOUND_SAMPLE *sound_2; // 射击中的声音指针
extern FSOUND_SAMPLE *sound_3; // 遇到物品的声音指针
extern FSOUND_SAMPLE *sound_4; // 游戏的背影音乐指针
extern int myPlaneNum; // 我方目前余下的飞机数目
extern bool end; // 控制游戏结束的布尔值
extern DWORD endtime; // 结束动画的起始时间
extern int timer; // 这一帧的运行时间
// 计算点(x1,y1)与点(x2,y2)的距离
float distance(float x1,float y1,float x2,float y2)
{
return (float)sqrt((x1-x2)*(x1-x2)+(y1-y2)*(y1-y2));
}
// 玩家飞机类定义
// 玩家飞机初始化
void PlayerPlane::initPlane(float a,float b,int l,float s)
{
x=a;
y=b;
life=l;
speed=s;
blastNum=0;
blastTime=0;
bombNum=START_BOMB_NUMBER;
planeTexture=0;
fireTime=0;
ammunitionKind=0;
score=0;
}
PlayerPlane::PlayerPlane(float a,float b,int l,float s)
{
initPlane(a,b,l,s);
}
// 更新飞机
void PlayerPlane::update()
{
if (life<=0&&blastNum==8){ // 玩家飞机爆炸完毕
myPlaneNum--;
if (myPlaneNum!=0){ // 玩家还有剩余飞机
int temp=score;
initPlane(0,-230,100,2);
score=temp;
}else{
// 游戏结束(失败)
endtime=GetTickCount();
end=true;
}
}else if(score>=WIN_SCORE){ // 游戏结束(胜利)
endtime=GetTickCount();
end=true;
}
}
// 发射子弹
void PlayerPlane::fire()
{
if(life>0){
fireTime+=timer;
if(fireTime>100){
Ammunition t1(x-12,y+1,90,4,100.0f,ammunitionKind);
Ammunition t2(x+12,y+1,90,4,100.0f,ammunitionKind);
t1.setOwner(1);
t2.setOwner(1);
ammunitions[ammunitionIndex++]=t1;
if(ammunitionIndex==MAX_AMMUNITION)
ammunitionIndex=0;
ammunitions[ammunitionIndex++]=t2;
if(ammunitionIndex==MAX_AMMUNITION)
ammunitionIndex=0;
fireTime-=100;
}
}
}
// 发射炸弹
void PlayerPlane::fireBomb()
{
if(life>0 && bombNum>0){
Ammunition t1(x,y+30,90,4,100.0f,3);
t1.setOwner(1);
ammunitions[ammunitionIndex++]=t1;
if(ammunitionIndex==MAX_AMMUNITION)
ammunitionIndex=0;
bombNum--;
}
}
// 检测并绘制飞机爆炸
void PlayerPlane::blast()
{
if(life<=0){
blastTime+=timer;
if(blastNum<8){
// 绘制爆炸图像
glPushMatrix();
glTranslatef(x, y, 0.0f);
glEnable(GL_TEXTURE_2D);
glBindTexture(GL_TEXTURE_2D, othertex[2].texID);
glBegin(GL_QUADS);
glTexCoord2d(blastNum*0.125f,0); glVertex2f(-50.0f,-50.0f);
glTexCoord2d((blastNum+1)*0.125f,0);glVertex2f( 50.0f,-50.0f);
glTexCoord2d((blastNum+1)*0.125f,1);glVertex2f( 50.0f, 50.0f);
glTexCoord2d(blastNum*0.125f,1); glVertex2f(-50.0f, 50.0f);
glEnd();
glDisable(GL_TEXTURE_2D);
glPopMatrix();
// 更新爆炸相关参数
if(blastTime>30){
if(blastNum==0)
FSOUND_PlaySound(FSOUND_FREE,sound_2);
blastNum++;
blastTime=0;
}
}
}
}
// 检测飞机是否相撞
void PlayerPlane::hitcomPlane()
{
if(life>0){
for(int i=0; i<MAX_COMPUTER; i++){
if(distance(x,y,computers[i].getX(),computers[i].getY())<30 && computers[i].getLife()>0){
life-=100; // 飞机相撞,生命值减少100
computers[i].setLife(0); // 对方飞机爆炸
computers[i].setExplosible(true);
return ;
}
}
}
}
// 飞机向上移动
void PlayerPlane::moveUp()
{
if(y<280 && life>0){
y+=speed*timer/20;
planeTexture=0;
}
}
// 飞机向下移动
void PlayerPlane::moveDown()
{
if(y>-230 && life>0){
y-=speed*timer/20;
planeTexture=0;
}
}
// 飞机向右移动
void PlayerPlane::moveRight()
{
if(life>0){
planeTexture=2;
if(x<230.0){
x+=speed*timer/20;
}
}
}
// 飞机向左移动
void PlayerPlane::moveLeft()
{
if(life>0){
planeTexture=1;
if(x>-230.0){
x-=speed*timer/20;
}
}
}
// 飞机原地不动
void PlayerPlane::stay()
{
if(life>0)
planeTexture=0;
}
// 绘制飞机
void PlayerPlane::draw()
{
if(life>0){
glPushMatrix();
glTranslatef(x, y, 0.0f);
glEnable(GL_TEXTURE_2D);
glBindTexture(GL_TEXTURE_2D, playertex[planeTexture].texID);
// 设置飞机颜色
if(life>50)
glColor3f(1.0f, 1.0f, 1.0f);
else
glColor3f(0.7f, 0.7f, 0.7f);
glBegin(GL_QUADS);
glTexCoord2i(0.0f, 0.0f);glVertex2i(-20,-20);
glTexCoord2i(1.0f, 0.0f);glVertex2i( 20,-20);
glTexCoord2i(1.0f, 1.0f);glVertex2i( 20, 20);
glTexCoord2i(0.0f, 1.0f);glVertex2i(-20, 20);
glEnd();
glColor3f(1.0f, 1.0f, 1.0f);
glDisable(GL_TEXTURE_2D);
glPopMatrix();
}
}
// 电脑飞机类成员定义
ComputerPlane::ComputerPlane(float a,float b,int l,float d,float s,int k):BaseObject(a,b,l,s,d,k)
{
fireTime=0;
}
// 电脑飞机的种类分为0,1,2三种,对于不同的种类,将会有不同的生命值和子弹属性等。
void ComputerPlane::setKind(int k)
{
if(k==0){
kind=0;
speed=2;
life=150;
rewardScore=100;
}else if(k==1){
kind=1;
speed=3;
life=100;
rewardScore=200;
}else if(k==2){
kind=2;
speed=1;
life=200;
rewardScore=500;
}
}
// 电脑飞机初始化
void ComputerPlane::compinit()
{
x=(float)(rand()%500-250);
y=(float)(rand()%100+300);
direction=(float)(rand()%90-135);
blastNum=0;
explosible=false;
// 初始化电脑飞机种类kind
int temp=rand()%8;
if(temp<=4)
setKind(0);
else if(temp==5 || temp==6)
setKind(1);
else if(temp==7)
setKind(2);
}
// 更新电脑飞机
void ComputerPlane::update()
{
if(life<=0 && blastNum==8 && explosible || life<=0 && !explosible){
compinit();
blastNum=0;
}
}
// 绘制电脑飞机
void ComputerPlane::draw()
{
if(life>0){
glPushMatrix();
glTranslatef(x, y, 0.0f);
glEnable(GL_TEXTURE_2D);
glBindTexture(GL_TEXTURE_2D, computertex[kind].texID);
glBegin(GL_QUADS);
glTexCoord2i(0, 0);glVertex2i(-25,-25);
glTexCoord2i(1, 0);glVertex2i( 25,-25);
glTexCoord2i(1, 1);glVertex2i( 25, 25);
glTexCoord2i(0, 1);glVertex2i(-25, 25);
glEnd();
glDisable(GL_TEXTURE_2D);
glPopMatrix();
}
}
// 电脑飞机移动
void ComputerPlane::move()
{
if(x>-275 && x<275 && y>-325){
x=x+speed*cos(direction/180*PI)*timer/20;
y=y+speed*sin(direction/180*PI)*timer/20;
}else{
life=0;
explosible=false;
}
}
// 电脑飞机移动以及子弹发射
void ComputerPlane::fire()
{
if(life>0){
fireTime+=timer;
// 射击速度随机
if((fireTime>1000+rand()%5000) && y>-100){
if(kind==0){
Ammunition t1(x,y-25,-90,3,100.0f,0);
t1.setOwner(3);
ammunitions[ammunitionIndex++]=t1;
if(ammunitionIndex==MAX_AMMUNITION)
ammunitionIndex=0;
fireTime=0;
}else if(kind==1){
int temp=atan((myPlane.getY()-(y-25))/(myPlane.getX()-x))/PI*180;
if(myPlane.getX()<x) // 若飞机在子弹的左边,则加180度
temp+=180;
Ammunition t1(x,y-25,temp,3,100.0f,0);
t1.setOwner(3);
ammunitions[ammunitionIndex++]=t1;
if(ammunitionIndex==MAX_AMMUNITION)
ammunitionIndex=0;
fireTime=0;
}else if(kind==2){
Ammunition t1(x,y-25,-90,3,100.0f,2);
t1.setOwner(3);
ammunitions[ammunitionIndex++]=t1;
if(ammunitionIndex==MAX_AMMUNITION)
ammunitionIndex=0;
fireTime=0;
}
}
}
}
// 电脑飞机爆炸
void ComputerPlane::blast()
{
if(life<=0 && explosible && blastNum<8){
glPushMatrix();
glTranslatef(x, y, 0.0f);
glEnable(GL_TEXTURE_2D);
glBindTexture(GL_TEXTURE_2D,othertex[2].texID);
glBegin(GL_QUADS);
glTexCoord2f(blastNum*0.125f, 0.0f); glVertex2f(-50.0f,-50.0f);
gl
没有合适的资源?快使用搜索试试~ 我知道了~
基于openGL开发的射击小游戏
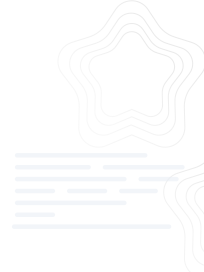
共60个文件
tga:32个
wav:6个
cpp:4个


温馨提示
这是一个基于opengl开发的射击游戏,从中你可以得到利用opengl开发图形处理程序的一个基本概念和思想
资源推荐
资源详情
资源评论
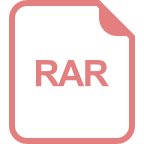
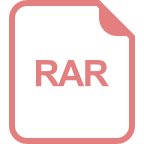
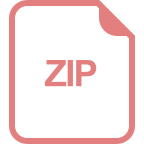
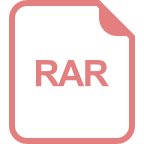
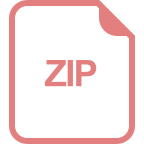
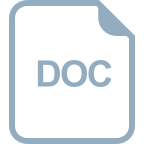
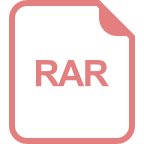
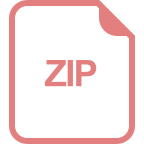
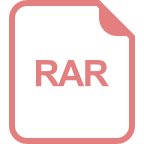
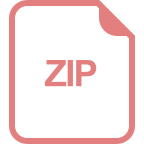
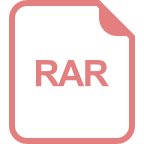
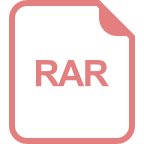
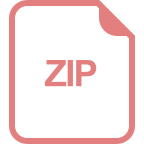
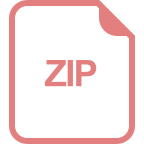
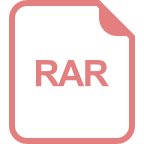
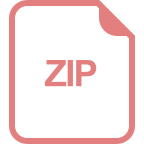
收起资源包目录


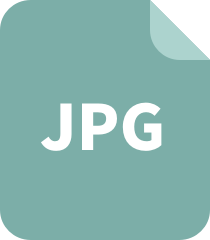
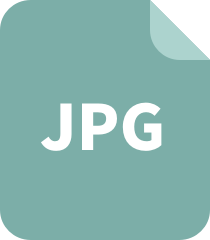
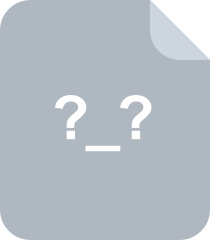

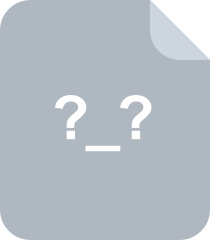
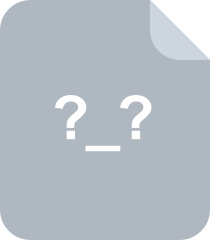
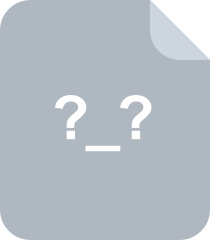
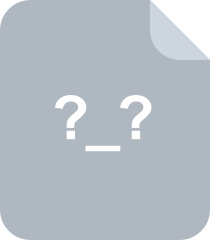
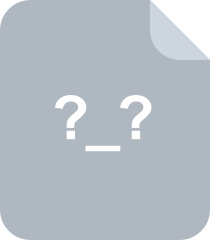
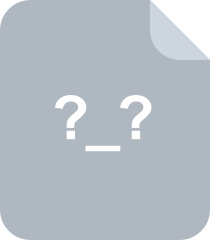
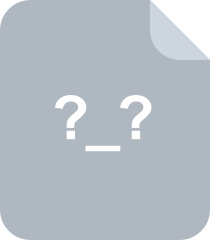
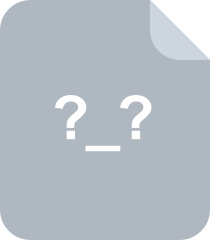
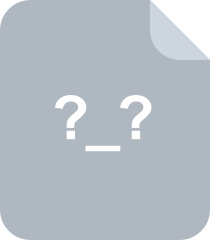
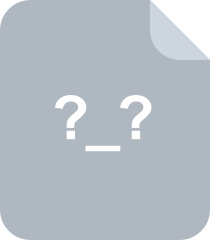
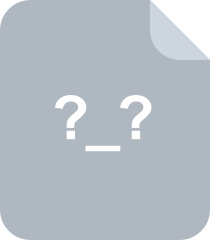
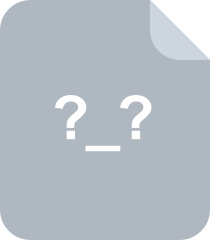
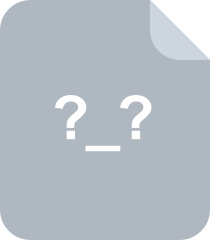
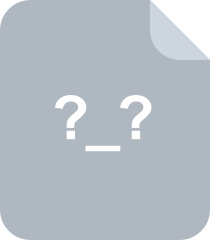
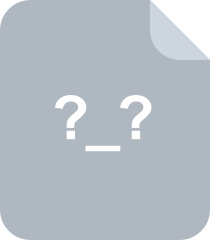
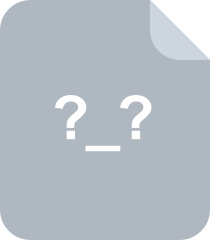
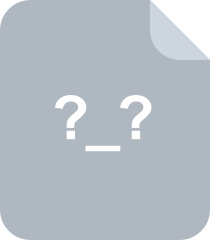
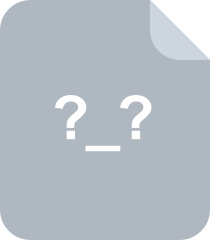
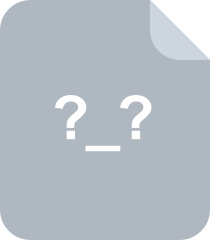
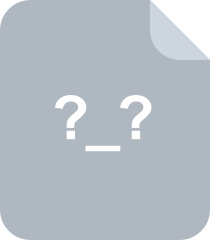
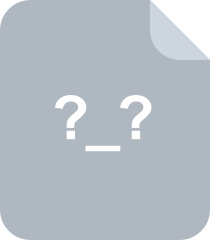


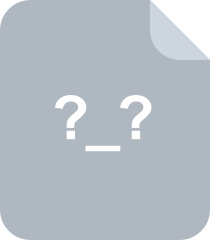
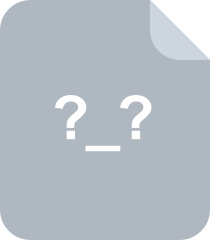
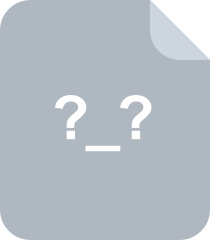
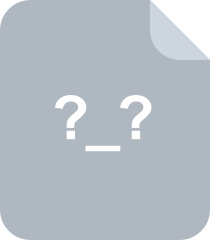
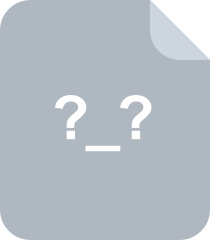
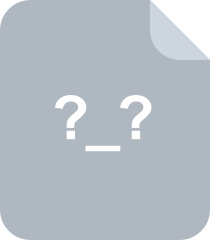
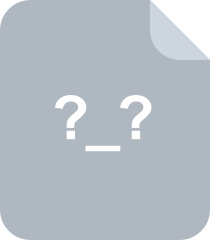
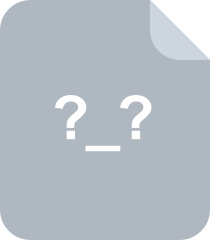
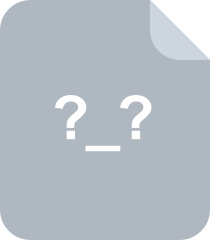
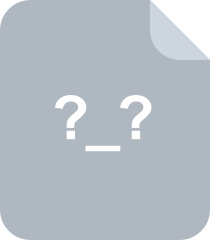
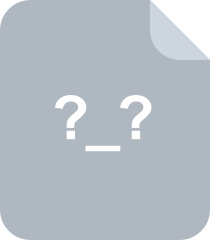
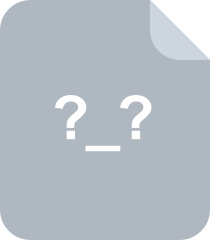
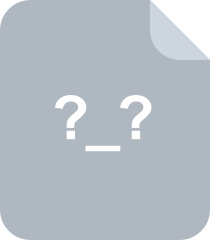
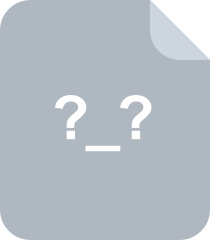
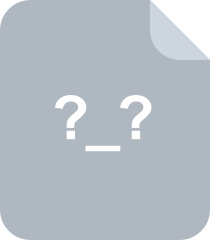
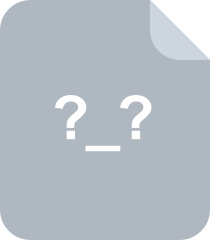
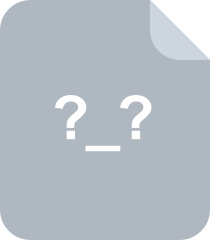
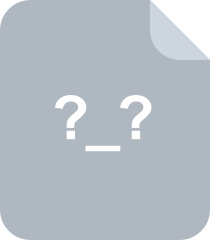
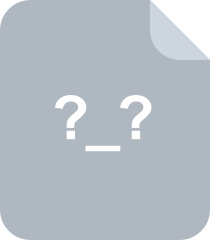
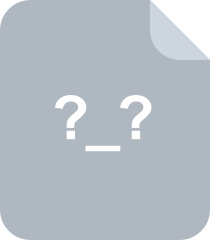
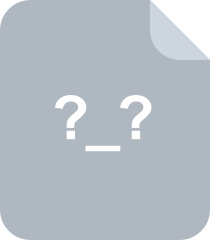
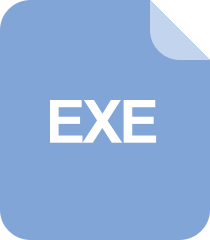
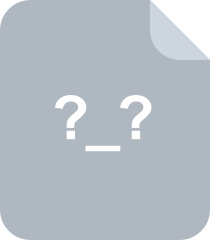
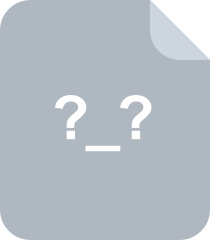
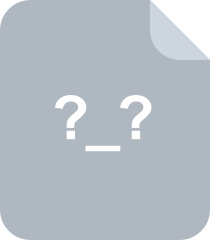
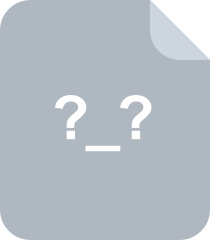
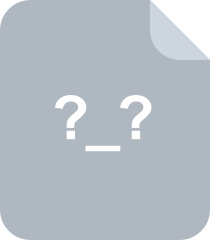
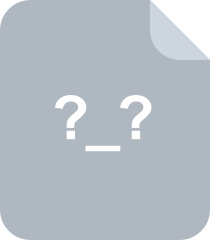
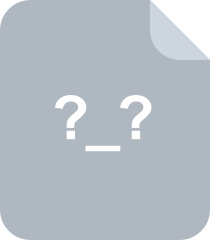
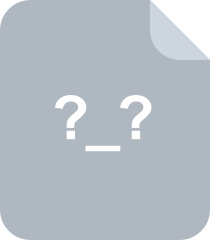
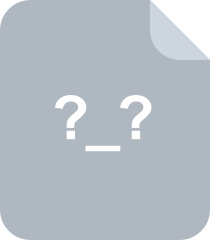
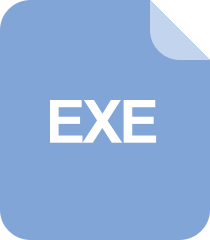
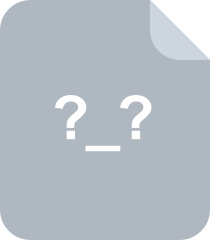
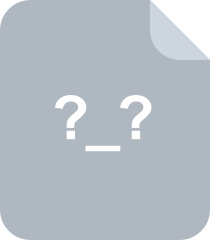
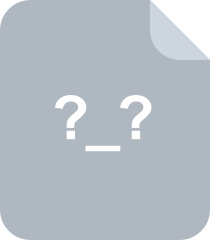
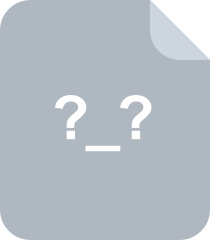
共 60 条
- 1
资源评论
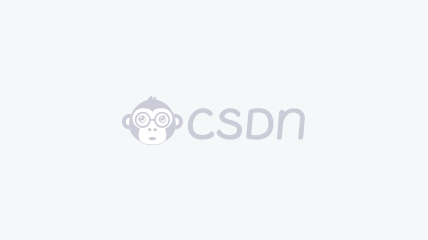
- wyx1191202012-05-14第一人称射击游戏,很好的例子,想写cs这类游戏的可以参考下。
- ShangPoLu2013-11-19很不错 适合参考
- orisoncj2012-12-073d不错了,就是少了些鼠标交互
- jiaming1220gd2012-12-05第一人称射击游戏,很好的例子,想写cs这类游戏的可以参考下
- 逆影之锋2023-11-30可以很强, 我vs2019运行有LNK2026 模块对于 SAFESEH 映像是不安全的报错,记得添加/SAFESEH:NO

iloveytu
- 粉丝: 1
- 资源: 9
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

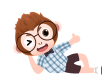
最新资源
- 基于Java和Kotlin的炉石传说自动化脚本项目源码+说明文档.zip
- 实习日报12.2.docx
- GO语言基础、学习笔记、项目规范.zip
- 免费功能一定要安装(安装上不用管)_sign.apk
- Goutte,一个简单的 PHP Web 爬虫.zip
- JAVA的Springboot个人博客系统源码带本地搭建教程数据库 MySQL源码类型 WebForm
- 2024-12-2 二阶问题(复杂区域)
- 架构师学习笔记,涵盖JAVA基础、MySQL、Spring、SpringBoot、SpringCloud、SpringCloudAlibaba、Redis、Kafka、设计模式、JVM等
- 开卡工具SM2258XT(AD)-B16A-PKGT1216A-FWT1125A0
- 电力场景变电站红外检测数据集VOC+YOLO格式6042张21类别.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


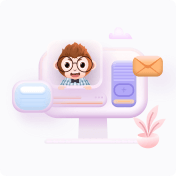
安全验证
文档复制为VIP权益,开通VIP直接复制
