最大信息系数(MIC)是一种衡量两个变量之间关系强度的统计工具,由Michael L. Resnik提出,其目的在于提供一种无参数、非线性的方法来评估变量间的依赖性。不同于传统的依赖性测量方法,MIC的优势在于它不受数据分布的影响,能够识别出变量间的线性和非线性关系。在Python环境下,我们可以借助一定的算法步骤实现MIC的计算。接下来,我们将详细探讨这一过程,包括数据预处理、互信息的计算、排序和重采样,以及如何从排序版本中得出最终的最大信息系数。 数据预处理是实现MIC的前提步骤。在进行相关性分析之前,必须确保涉及的所有变量均为数值型数据,因为MIC算法不适用于分类数据。对于分类数据,一般需要通过编码技术(如one-hot编码)将其转换成数值型数据。这一处理对于算法的准确性和有效性至关重要。 计算两个变量的联合分布和边缘分布是实现MIC的关键。联合分布表征了两个变量共同出现的频率,而边缘分布则描述了单个变量出现的频率。在Python中,我们可以使用直方图方法或者核密度估计(Kernel Density Estimation, KDE)技术来估计这些分布。直方图方法是通过将数据分割成一系列连续的区间(桶),统计每个区间内数据点的数量,从而得到分布的估计。核密度估计则是一种更为平滑的非参数方法,它通过核函数对数据点进行加权,形成连续的密度曲线。 接下来的步骤是排序和重采样。这一步骤的目的是为了探索变量之间可能存在的不同排列方式,并寻找在何种排列下,两个变量的互信息达到最大。具体来说,对于每一个变量,我们将数据按照其值进行排序,然后按照排序后的顺序重新排列数据,形成变量的多种排序版本。这一步骤可能会涉及到多次重采样,目的是减少由于数据集大小限制带来的随机性。 互信息的计算是基于已经计算出的联合分布和边缘分布。互信息是描述两个随机变量间共享信息量的度量,它等于两个变量的联合分布熵与各自边缘分布熵的差。在数学表达上,如果X和Y是两个随机变量,它们的联合分布是P(X,Y),边缘分布分别是P(X)和P(Y),那么互信息I(X;Y)可以表达为: I(X;Y) = ∑∑ P(x,y) * log(P(x,y) / (P(x) * P(y))) 其中,求和是对所有可能的x和y值进行的。计算出每个排序版本下的互信息之后,我们需要找出使互信息最大的那个排序。在这个排序下,两个变量之间的依赖性最为强烈,而这个最大互信息值即为所求的最大信息系数MIC。 解释结果是整个流程的终点。在得到MIC值之后,我们可以根据其数值判断变量间的关系强度。通常,MIC值越接近1,表示变量间的关系越强,而接近0则意味着变量间关系较弱。然而,需要注意的是,MIC值的解释还需要结合实际问题的上下文,并不是绝对的标准,特别是在低样本量情况下,MIC值可能不够稳定。 Python中的实现代码将围绕上述步骤构建。我们需要导入必要的库,如NumPy、SciPy和Matplotlib等。然后,可以编写函数来处理数据预处理,计算联合分布和边缘分布,执行排序和重采样,并最终计算互信息以及寻找最大信息系数MIC。整个过程可以通过精心设计的函数和类来实现,以提高代码的可读性和复用性。此外,使用Python的简洁语法和强大的库支持,可以有效地处理复杂的数据分析任务,包括MIC算法的实现。
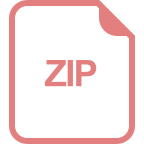
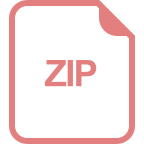
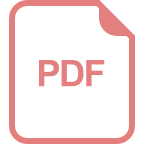
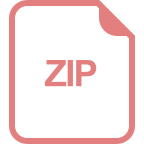
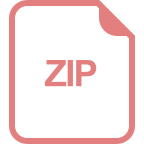
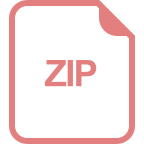
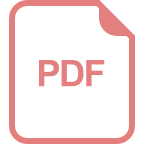
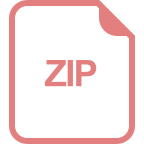
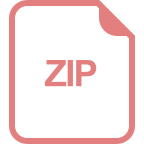
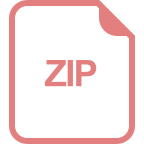
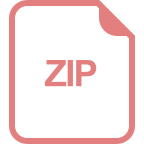
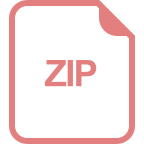
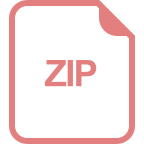
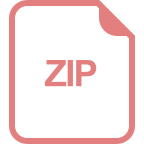
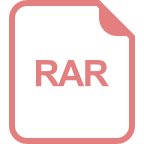
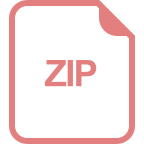
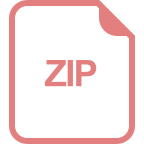
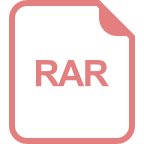
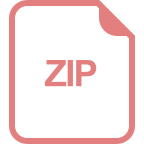
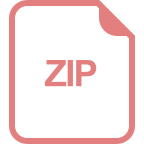
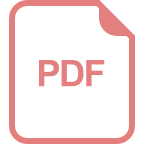

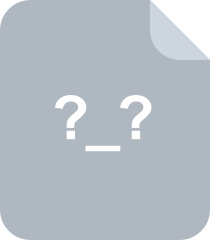
- 1
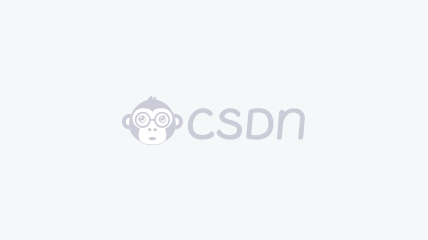

- 粉丝: 327
- 资源: 42
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

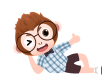
最新资源
- 毕设和企业适用springboot社交应用平台类及虚拟人类交互系统源码+论文+视频.zip
- 毕设和企业适用springboot人力资源管理类及智能会议管理平台源码+论文+视频.zip
- 毕设和企业适用springboot商城类及城市智能运营平台源码+论文+视频.zip
- 毕设和企业适用springboot商城类及车联网管理平台源码+论文+视频.zip
- 毕设和企业适用springboot社交互动平台类及食品配送平台源码+论文+视频.zip
- 毕设和企业适用springboot社交互动平台类及视频监控系统源码+论文+视频.zip
- 毕设和企业适用springboot社交互动平台类及视频内容分发平台源码+论文+视频.zip
- 毕设和企业适用springboot社交应用平台类及云计算资源管理平台源码+论文+视频.zip
- 毕设和企业适用springboot社交应用平台类及用户反馈平台源码+论文+视频.zip
- 毕设和企业适用springboot社交应用平台类及用户数据分析平台源码+论文+视频.zip
- 毕设和企业适用springboot商城类及个性化推荐系统源码+论文+视频.zip
- 毕设和企业适用springboot商城类及电子产品维修平台源码+论文+视频.zip
- 毕设和企业适用springboot商城类及风险控制平台源码+论文+视频.zip
- 毕设和企业适用springboot社交互动平台类及数据存储平台源码+论文+视频.zip
- 毕设和企业适用springboot社交互动平台类及数据智能化平台源码+论文+视频.zip
- 毕设和企业适用springboot社交互动平台类及投票平台源码+论文+视频.zip

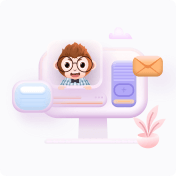
