# Matlab Job Manager
Manages computational jobs. Here, a job is a function (typically expensive to run) that is called with some input and returns some output. This Job Manager is useful if you have many such jobs to run (perhaps in parallel), or you want to cache the results of the function for the benefit of front end code such-as data visualisation.
This library provides:
* Memoisation cache. Previously computed results are loaded from the cache instead of being recomputed. The cache is automatically invalidated when the relevant code is modified.
* Parallel execution of jobs with:
* Matlab's Parallel Computing Toolbox, or
* A compute cluster running a Portable Batch System (PBS) scheduler, or
* The included job server that distributes tasks to remote workers over a network connection.
This framework applies to functions with the signature:
```matlab
result = solver(config, display_config);
```
where
* `result` is the output of the computation (typically a struct)
* `solver` is a function that implements the computation
* `config` is a struct that includes all the settings necessary to describe the task to be performed. Any setting that could influence the return value must be included in this structure so that the memoisation cache can identify when to return a previously saved result.
* `display_config` is a struct that includes settings that **cannot** influence the return value `result`. For example, this structure could specify how verbose the solver should be in printing messages to the command window.
To use this library, you must organise your solver according to that function template.
There are two ways to use this package:
1. The low-level interface to the memoisation cache. Use this if you implement your own execution framework but want to add memoisation.
2. The high-level interface for running jobs. This automatically takes advantage of the memoisation cache.
## Example usage
Basic example:
```matlab
% Prepare the configs to process
c1 = struct();
c1.solver = @solver_fn; % you must set the "solver" field to a function handle
...
c2 = ...;
c3 = ...;
configs = {c1, c2, c3}; % Prepare a cell array of configs to process
r = jobmgr.run(configs); % Jobs will run in parallel with the Matlab parfor loop
% The return value is a cell array of results.
% Results are memoised so that subsequent calls return almost immediately
```
A more advanced example using a Portable Batch System (PBS) cluster, which is an asynchronous execution method:
```matlab
configs = {c1, c2, c3}; % Prepare a cell array of configs to process
run_opts.execution_method = 'qsub'; % Use the qsub command to schedule the jobs on the cluster
run_opts.configs_per_job = 2; % Run two configs (in series) per qsub job
run_opts.allow_partial_result = false; % Throw an exception if the jobs are not yet finished running
r = jobmgr.run(configs, run_opts); % Submit the jobs
%
% The qsub method queues the jobs and returns immediately, throwing 'jobmgr:incomplete'.
%
% Run this code again later when the jobs are finished and then the return value will
% be a cell array of results.
```
## Installation
This code assumes that it will be placed in a Matlab package called `+jobmgr`. You must ensure that the repository is cloned into a directory with this name.
The recommended way to install is to add this as a git subtree to your existing project.
$ git remote add -f matlab-job-manager https://github.com/bronsonp/matlab-job-manager.git
$ git subtree add --prefix +jobmgr matlab-job-manager master
At a later time, if there are updates released that you wish to add to your project:
$ git fetch matlab-job-manager
$ git subtree pull --prefix +jobmgr matlab-job-manager master
If you do not intend to use git subtree, you can simply clone the repository:
$ git clone https://github.com/bronsonp/matlab-job-manager.git +jobmgr
### Job Server (Linux)
The optional job server (for remote execution) requires some C++ code to be compiled.
$ sudo apt-get install libzmq3-dev
$ cd +jobmgr/+netsrv/private
$ make
### Job Server (Windows)
The optional job server (for remote execution) requires some C++ code to be compiled. Run the `compile_for_windows.m` script in the `+jobmgr/+netsrv/private` directory.
## Using the high-level interface
**Summary:** Look in the `+example` folder and copy this code to get started.
Prerequisites:
1. The solver must implement the function signature above.
2. The solver must explicitly tag its dependencies so that the memoisation cache
can be cleared when these dependencies change. See the "Dependency
tagging" section for instructions.
3. The solver must accept a string input `display_config.run_name` which gives a descriptive label to each job. Typically, this would be printed at the beginning of any status messages displayed during calculations. Run names are passed to the job manager with a cell array in `run_opts.run_names`.
4. The solver must accept a logical input `display_config.animate` which is intended to specify whether to draw an animation of progress during the calculation. This defaults of `false` when running in the job manager. You can ignore this field if it is not relevant.
5. The solver should check for the presence of a global variable `statusline_hook_fn`. If this variable exists, the solver should periodically call this function with a short string indicating current progress towards solving the task. The job server displays a table of currently executing jobs, and this status appears next to the job. Additionally, the server can detect crashed clients if a specified time has passed since the last status update. Lost jobs can be resubmitted to a new client.
An example solver that implements this API is included in the `+example` folder.
## Using the low-level interface
Prerequisites:
1. The solver must implement the function signature above.
2. The solver must explicitly tag its dependencies so that the cache can be emptied when these dependencies change. See the "Dependency tagging" section for instructions.
3. Call the `check_cache` function first before any other functions are called. This will create a new empty cache directory, or delete old cache entries if the solver code has been modified.
Use the following functions:
* `check_cache` to delete old cache entries if the code has been changed.
* `struct_hash` to convert a config structure into a SHA1 hash for use with the `store`, `is_memoised`, and `recall` functions.
* `store` to save a value to be recalled later
* `is_memoised` to check whether a saved value exists in the cache
* `recall` to recover a previously stored item.
## Dependency tagging
If you modify your code, then the memoisation cache needs to be cleared so that new results are calculated using the new version of your code. If your solver is fully self-contained, then you don't need to do anything. On the other hand, if your solver is split up into multiple M files, then you need to tag file dependencies.
The example code in the `+example` folder demonstrates how to do this.
File dependencies are tagged by inserting comments into your code:
% +FILE_DEPENDENCY relative/path/to/file.m
% +FILE_DEPENDENCY relative/path/*.m
% +MEX_DEPENDENCY path/to/mex/binary
You can use wildcards as indicated above. Tags with `FILE_DEPENDENCY` refer to text files (i.e. Matlab code). Tags with `MEX_DEPENDENCY` are a special case for MEX code. You must specify the path to the MEX binary *without* any file extension. The file extension as appropriate for your system is automatically appended to the file. For example, the above example would match `binary.mexa64` on Linux, and `binary.mexw64` on Windows.
## Execution methods
The method used to run the jobs is specified in the `run_opts.execution_method` field (in the second argument to `jobmgr.run`). The following execution methods are defined:
### Matlab's Parallel Computing Toolbox (p
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
DeepBTSeg是一个专为医学图像处理领域设计的深度学习模型,它利用先进的深度学习技术来实现脑肿瘤图像的自动分割。这一工具的开发,极大地简化了医学图像分析的过程,使得非专业人士也能够轻松进行精确的脑肿瘤分割。 以下是针对“matlab图像分割肿瘤代码-DeepBTSeg”资源的介绍: **"DeepBTSeg:Matlab深度学习脑肿瘤图像分割工具"**:这个资源提供了一个基于Matlab的用户友好图形用户界面(GUI),使得用户可以无需复杂的编程知识,轻松进行深度学习脑肿瘤图像分割。DeepBTSeg利用了深度学习的强大能力,通过一个简单易用的界面,为用户提供了一个高效、准确的图像分割解决方案。 该资源特别适合医疗研究人员、放射科医生以及对医学图像分析感兴趣的学生和专业人士。它不仅减少了对高配置硬件的需求,还简化了软件的安装和配置过程,使得用户可以快速上手并专注于图像分割任务本身。 使用DeepBTSeg,用户可以期待获得一个强大的工具,它不仅能够提高脑肿瘤分割的精度和效率,还能够为医学图像分析提供有力的支持。请注意,在下载和使用这个资源时,应确保遵守相关的版权和使用条款。
资源推荐
资源详情
资源评论
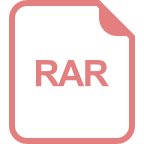
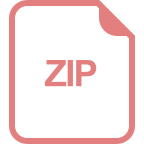
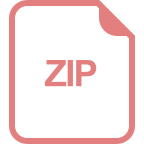
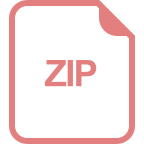
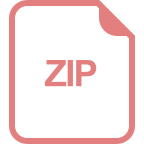
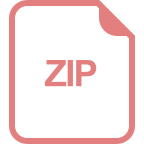
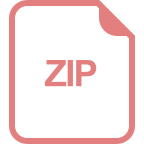
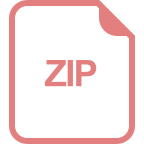
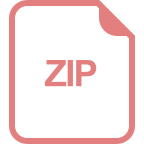
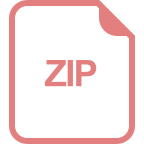
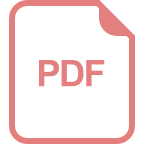
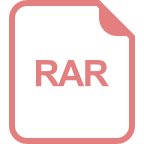
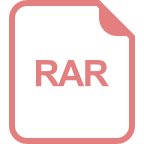
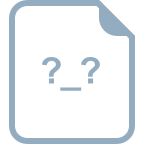
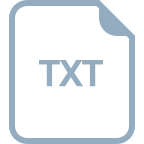
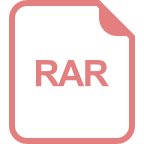
收起资源包目录

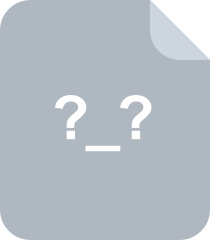
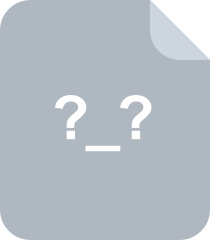
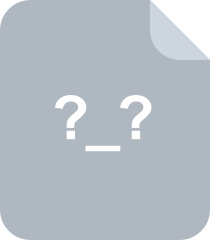
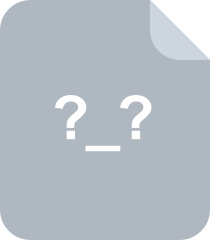
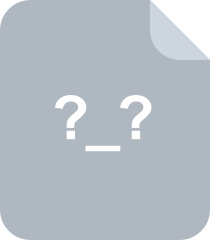
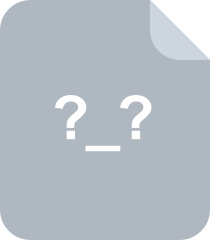
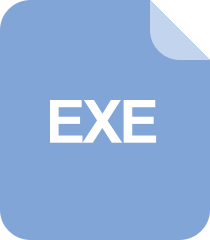
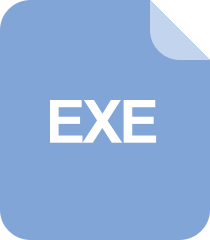
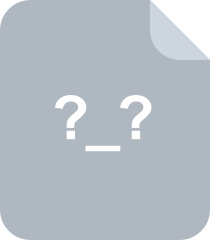
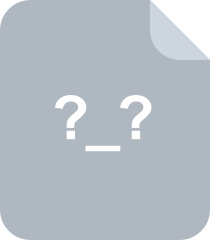
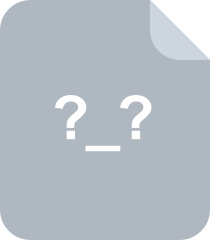
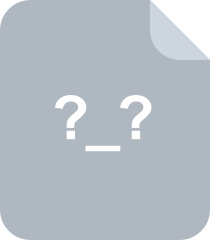
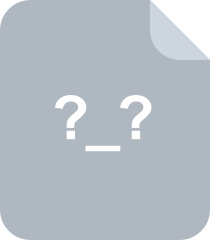
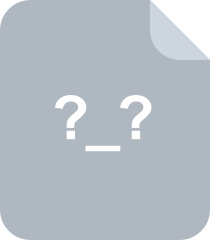
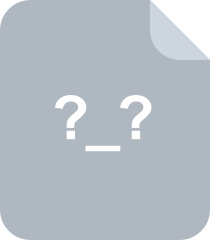
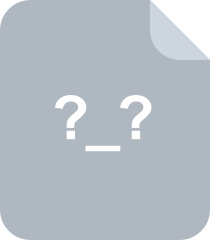
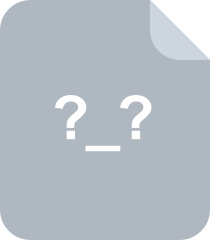
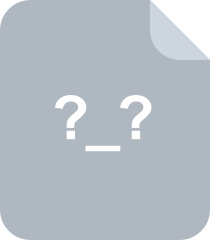
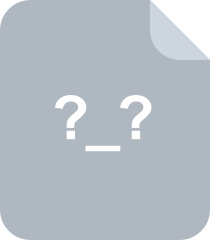
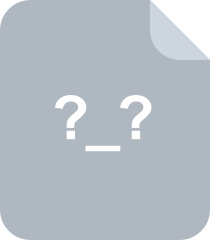
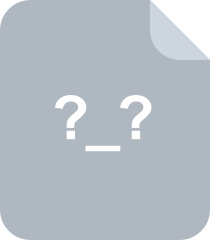
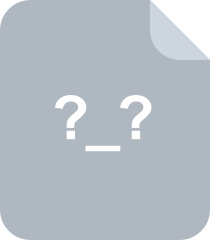
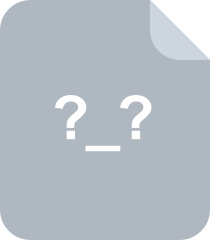
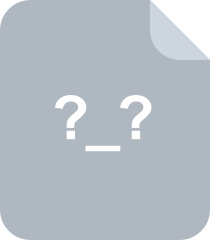
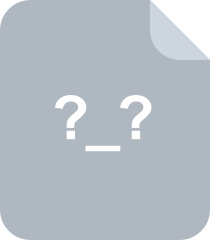
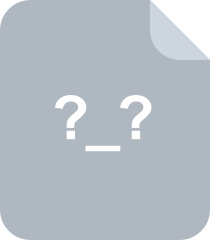
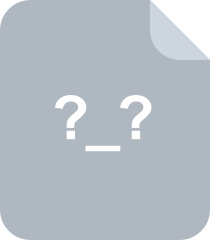
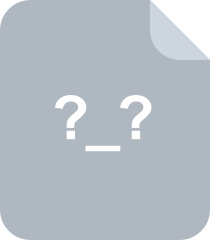
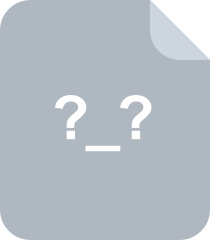
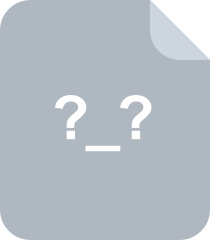
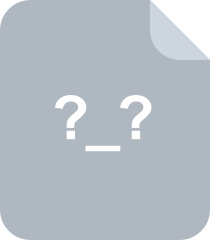
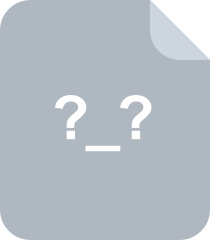
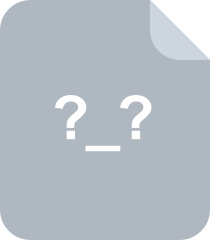
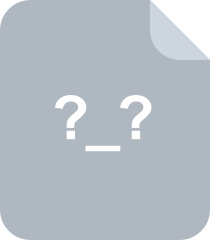
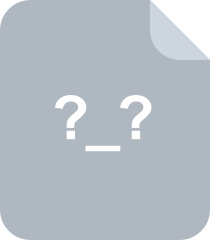
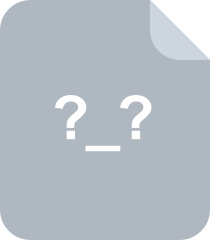
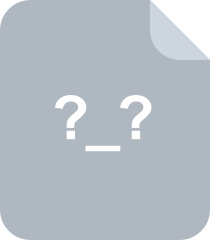
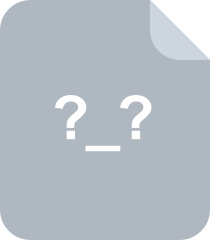
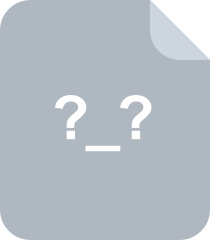
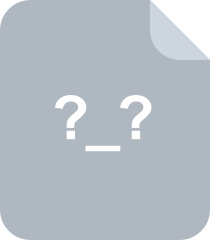
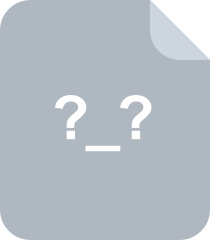
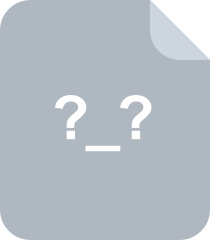
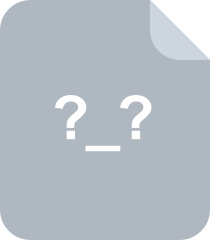
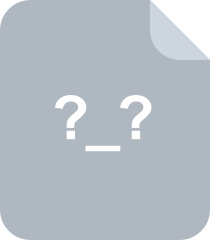
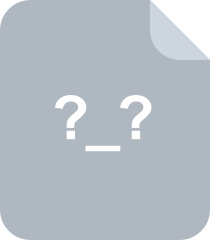
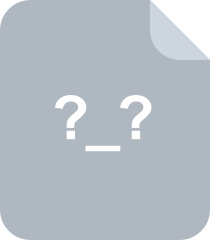
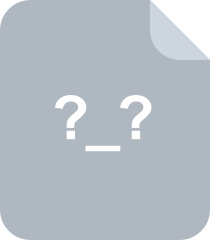
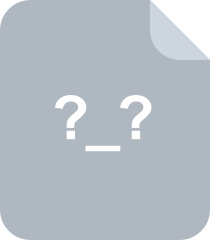
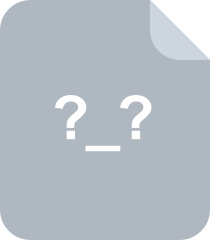
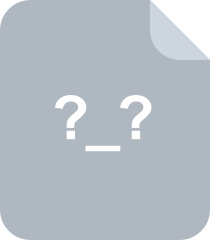
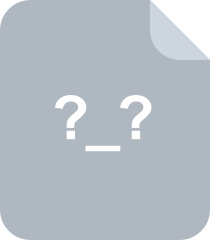
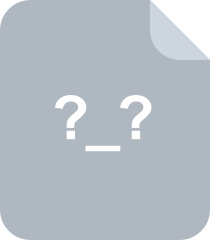
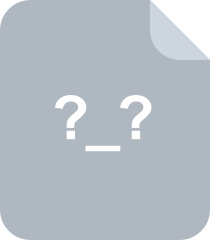
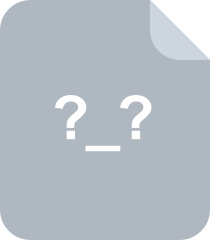
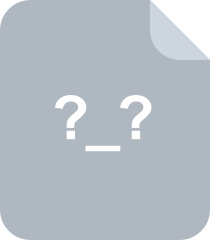
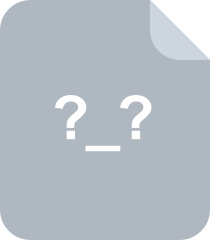
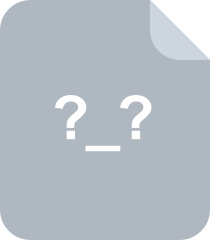
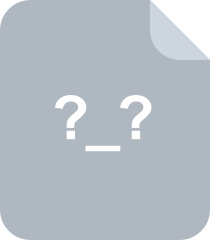
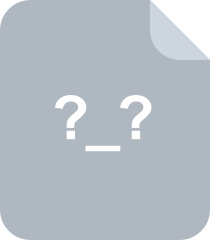
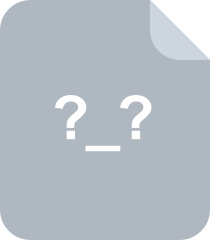
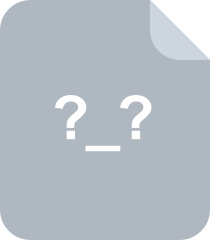
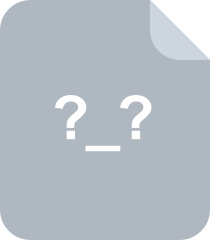
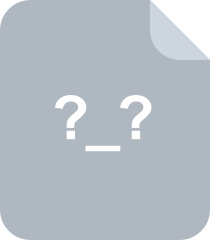
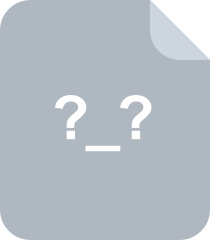
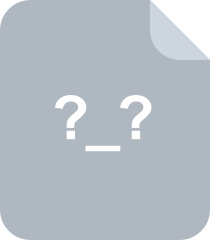
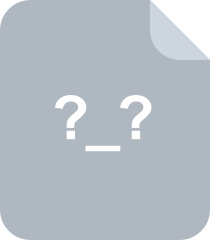
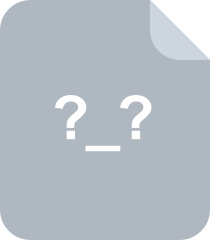
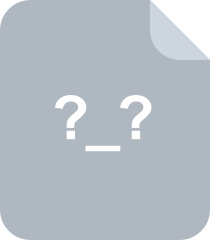
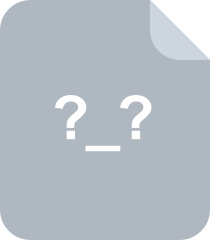
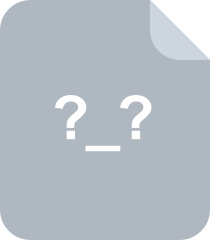
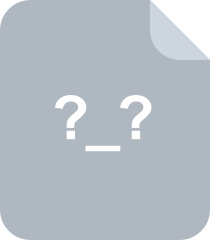
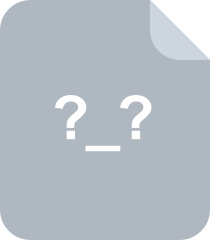
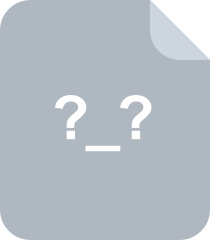
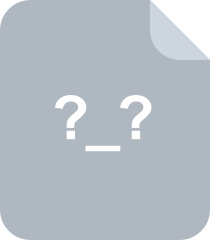
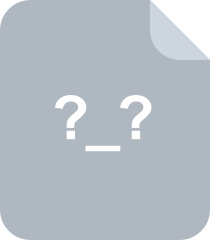
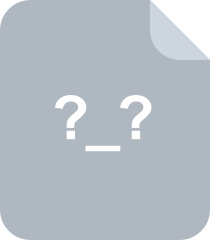
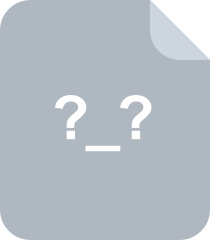
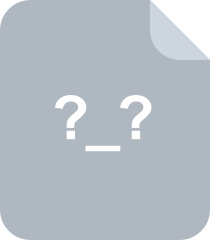
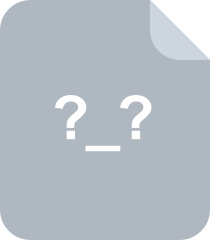
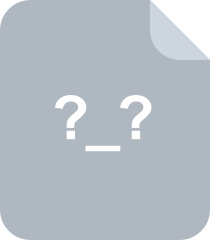
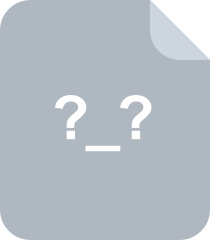
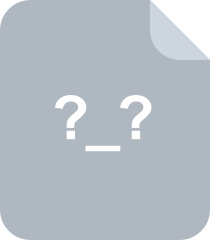
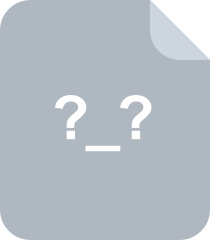
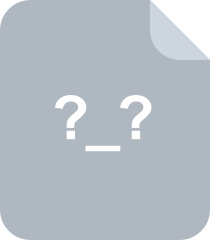
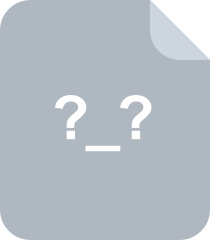
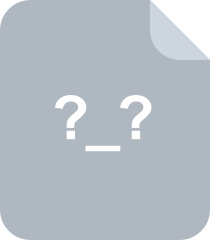
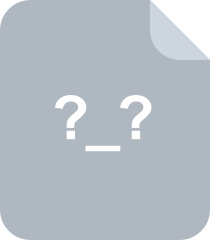
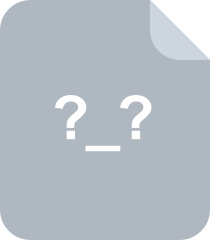
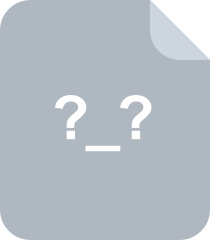
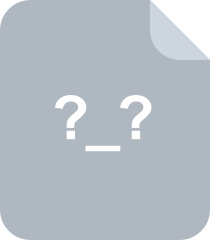
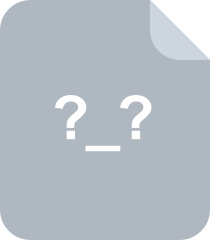
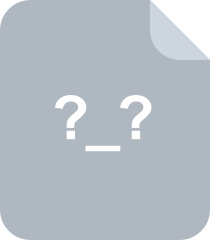
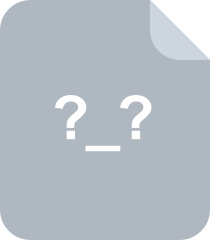
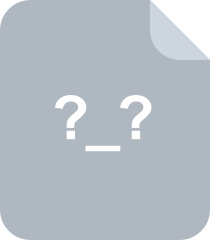
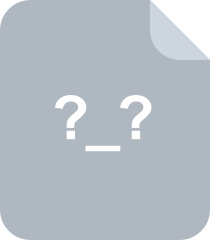
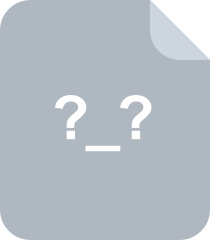
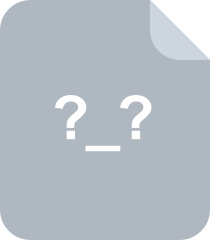
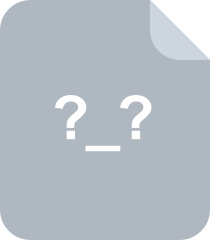
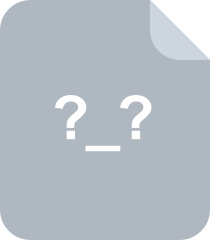
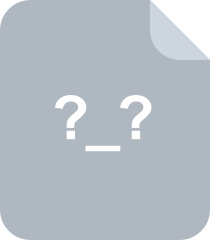
共 147 条
- 1
- 2
资源评论
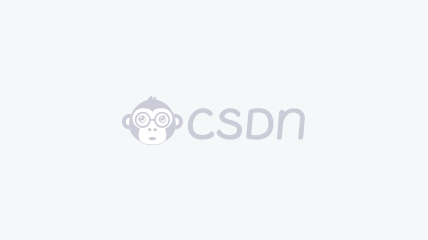

赵无极写JAVA
- 粉丝: 2574
- 资源: 172
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

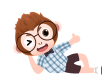
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


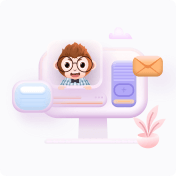
安全验证
文档复制为VIP权益,开通VIP直接复制
