# Matlab Job Manager
Manages computational jobs. Here, a job is a function (typically expensive to run) that is called with some input and returns some output. This Job Manager is useful if you have many such jobs to run (perhaps in parallel), or you want to cache the results of the function for the benefit of front end code such-as data visualisation.
This library provides:
* Memoisation cache. Previously computed results are loaded from the cache instead of being recomputed. The cache is automatically invalidated when the relevant code is modified.
* Parallel execution of jobs with:
* Matlab's Parallel Computing Toolbox, or
* A compute cluster running a Portable Batch System (PBS) scheduler, or
* The included job server that distributes tasks to remote workers over a network connection.
This framework applies to functions with the signature:
```matlab
result = solver(config, display_config);
```
where
* `result` is the output of the computation (typically a struct)
* `solver` is a function that implements the computation
* `config` is a struct that includes all the settings necessary to describe the task to be performed. Any setting that could influence the return value must be included in this structure so that the memoisation cache can identify when to return a previously saved result.
* `display_config` is a struct that includes settings that **cannot** influence the return value `result`. For example, this structure could specify how verbose the solver should be in printing messages to the command window.
To use this library, you must organise your solver according to that function template.
There are two ways to use this package:
1. The low-level interface to the memoisation cache. Use this if you implement your own execution framework but want to add memoisation.
2. The high-level interface for running jobs. This automatically takes advantage of the memoisation cache.
## Example usage
Basic example:
```matlab
% Prepare the configs to process
c1 = struct();
c1.solver = @solver_fn; % you must set the "solver" field to a function handle
...
c2 = ...;
c3 = ...;
configs = {c1, c2, c3}; % Prepare a cell array of configs to process
r = jobmgr.run(configs); % Jobs will run in parallel with the Matlab parfor loop
% The return value is a cell array of results.
% Results are memoised so that subsequent calls return almost immediately
```
A more advanced example using a Portable Batch System (PBS) cluster, which is an asynchronous execution method:
```matlab
configs = {c1, c2, c3}; % Prepare a cell array of configs to process
run_opts.execution_method = 'qsub'; % Use the qsub command to schedule the jobs on the cluster
run_opts.configs_per_job = 2; % Run two configs (in series) per qsub job
run_opts.allow_partial_result = false; % Throw an exception if the jobs are not yet finished running
r = jobmgr.run(configs, run_opts); % Submit the jobs
%
% The qsub method queues the jobs and returns immediately, throwing 'jobmgr:incomplete'.
%
% Run this code again later when the jobs are finished and then the return value will
% be a cell array of results.
```
## Installation
This code assumes that it will be placed in a Matlab package called `+jobmgr`. You must ensure that the repository is cloned into a directory with this name.
The recommended way to install is to add this as a git subtree to your existing project.
$ git remote add -f matlab-job-manager https://github.com/bronsonp/matlab-job-manager.git
$ git subtree add --prefix +jobmgr matlab-job-manager master
At a later time, if there are updates released that you wish to add to your project:
$ git fetch matlab-job-manager
$ git subtree pull --prefix +jobmgr matlab-job-manager master
If you do not intend to use git subtree, you can simply clone the repository:
$ git clone https://github.com/bronsonp/matlab-job-manager.git +jobmgr
### Job Server (Linux)
The optional job server (for remote execution) requires some C++ code to be compiled.
$ sudo apt-get install libzmq3-dev
$ cd +jobmgr/+netsrv/private
$ make
### Job Server (Windows)
The optional job server (for remote execution) requires some C++ code to be compiled. Run the `compile_for_windows.m` script in the `+jobmgr/+netsrv/private` directory.
## Using the high-level interface
**Summary:** Look in the `+example` folder and copy this code to get started.
Prerequisites:
1. The solver must implement the function signature above.
2. The solver must explicitly tag its dependencies so that the memoisation cache
can be cleared when these dependencies change. See the "Dependency
tagging" section for instructions.
3. The solver must accept a string input `display_config.run_name` which gives a descriptive label to each job. Typically, this would be printed at the beginning of any status messages displayed during calculations. Run names are passed to the job manager with a cell array in `run_opts.run_names`.
4. The solver must accept a logical input `display_config.animate` which is intended to specify whether to draw an animation of progress during the calculation. This defaults of `false` when running in the job manager. You can ignore this field if it is not relevant.
5. The solver should check for the presence of a global variable `statusline_hook_fn`. If this variable exists, the solver should periodically call this function with a short string indicating current progress towards solving the task. The job server displays a table of currently executing jobs, and this status appears next to the job. Additionally, the server can detect crashed clients if a specified time has passed since the last status update. Lost jobs can be resubmitted to a new client.
An example solver that implements this API is included in the `+example` folder.
## Using the low-level interface
Prerequisites:
1. The solver must implement the function signature above.
2. The solver must explicitly tag its dependencies so that the cache can be emptied when these dependencies change. See the "Dependency tagging" section for instructions.
3. Call the `check_cache` function first before any other functions are called. This will create a new empty cache directory, or delete old cache entries if the solver code has been modified.
Use the following functions:
* `check_cache` to delete old cache entries if the code has been changed.
* `struct_hash` to convert a config structure into a SHA1 hash for use with the `store`, `is_memoised`, and `recall` functions.
* `store` to save a value to be recalled later
* `is_memoised` to check whether a saved value exists in the cache
* `recall` to recover a previously stored item.
## Dependency tagging
If you modify your code, then the memoisation cache needs to be cleared so that new results are calculated using the new version of your code. If your solver is fully self-contained, then you don't need to do anything. On the other hand, if your solver is split up into multiple M files, then you need to tag file dependencies.
The example code in the `+example` folder demonstrates how to do this.
File dependencies are tagged by inserting comments into your code:
% +FILE_DEPENDENCY relative/path/to/file.m
% +FILE_DEPENDENCY relative/path/*.m
% +MEX_DEPENDENCY path/to/mex/binary
You can use wildcards as indicated above. Tags with `FILE_DEPENDENCY` refer to text files (i.e. Matlab code). Tags with `MEX_DEPENDENCY` are a special case for MEX code. You must specify the path to the MEX binary *without* any file extension. The file extension as appropriate for your system is automatically appended to the file. For example, the above example would match `binary.mexa64` on Linux, and `binary.mexw64` on Windows.
## Execution methods
The method used to run the jobs is specified in the `run_opts.execution_method` field (in the second argument to `jobmgr.run`). The following execution methods are defined:
### Matlab's Parallel Computing Toolbox (p
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
DeepBTSeg是一款基于MATLAB的深度学习工具箱,专门用于医学图像中的肿瘤分割。它利用深度学习技术,特别是卷积神经网络(CNN),来实现对MRI或CT扫描图像中肿瘤区域的高精度分割。以下是DeepBTSeg的主要特点: 深度学习驱动:采用先进的深度学习算法,提供准确的肿瘤分割结果。 用户友好的界面:提供简洁直观的用户界面,简化操作流程,便于非专业用户使用。 预训练模型:包含预训练的深度学习模型,用户可以无需额外训练即可进行分割。 可定制性:支持用户根据自己的数据集对模型进行再训练和优化。 多模态支持:适用于多种医学图像模态,包括但不限于MRI和CT图像。 自动化处理:实现自动化的图像分割流程,减少人工干预,提高效率。 高精度分割:通过深度学习技术,实现对肿瘤边缘的精确识别和分割。 科研和临床应用:适用于医学研究和临床诊断,辅助医生进行更准确的肿瘤分析。 文档和支持:提供详细的使用说明和技术支持,帮助用户解决使用中的问题。 DeepBTSeg是医学图像处理领域中肿瘤分割的有力工具。立即下载DeepBTSeg,提升您的医学图像分析能力。
资源推荐
资源详情
资源评论
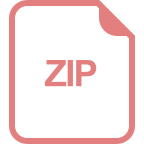
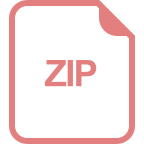
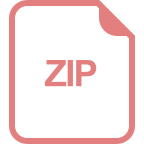
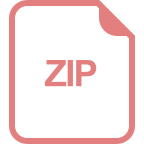
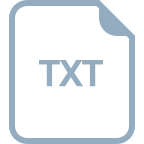
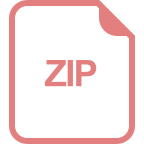
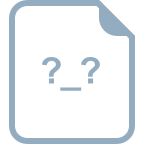
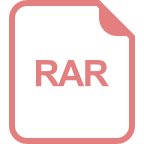
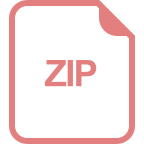
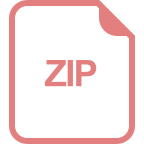
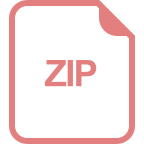
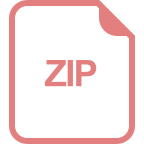
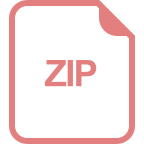
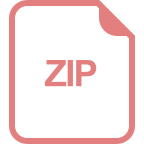
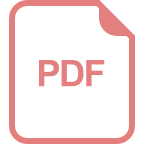
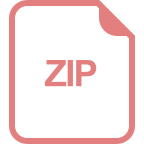
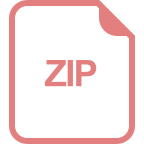
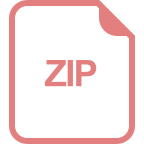
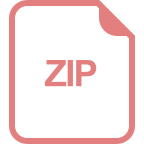
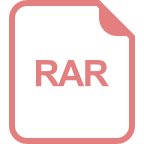
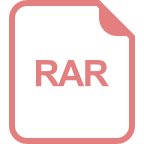
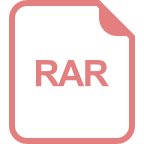
收起资源包目录

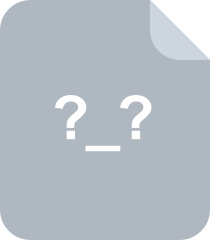
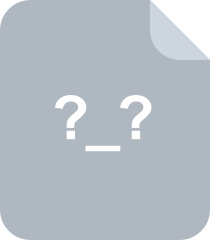
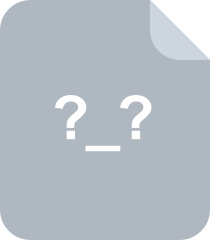
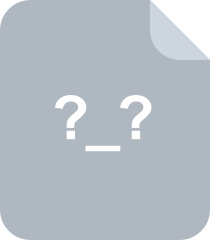
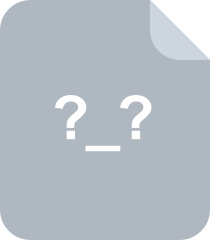
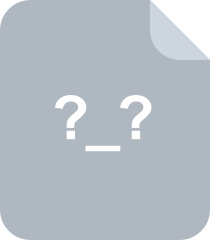
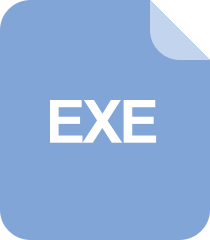
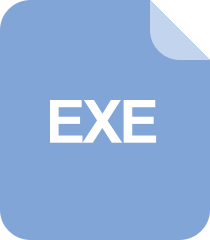
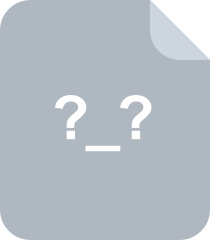
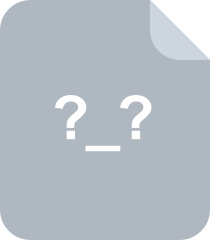
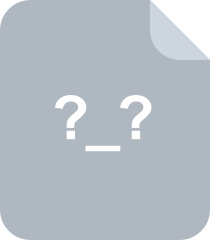
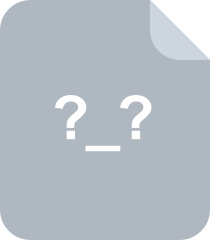
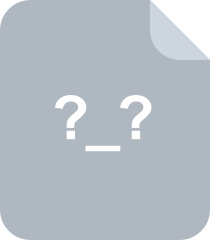
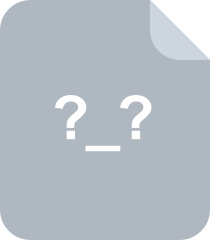
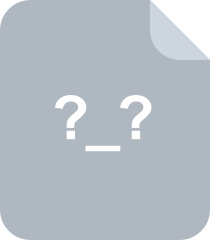
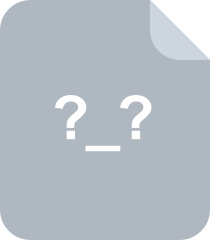
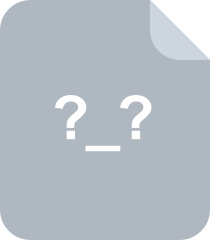
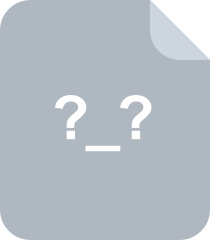
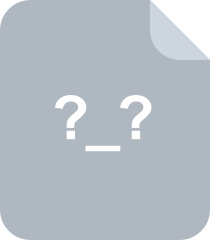
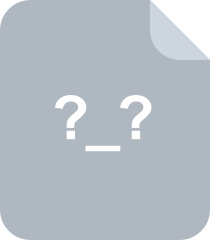
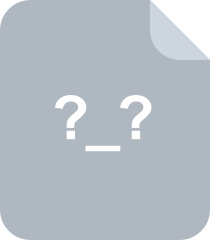
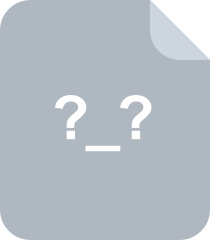
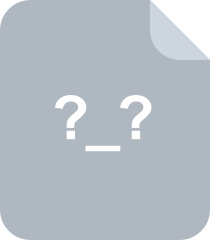
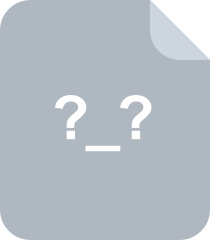
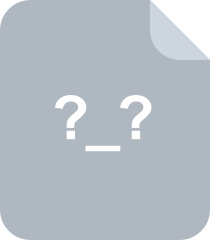
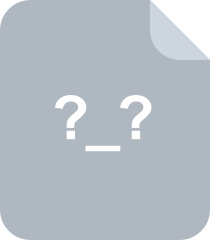
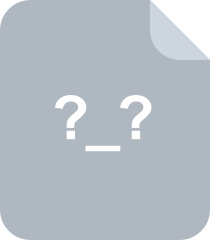
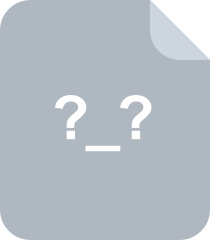
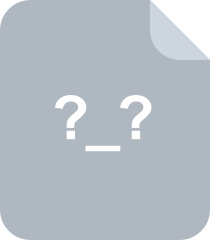
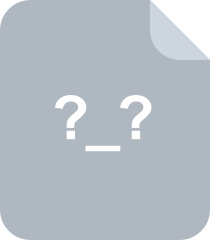
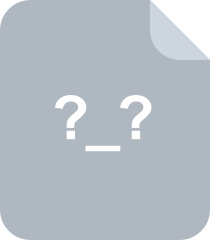
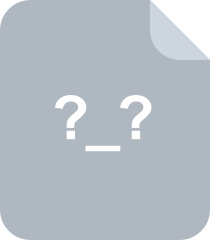
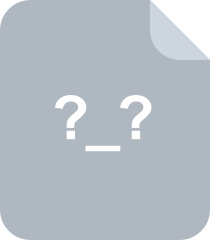
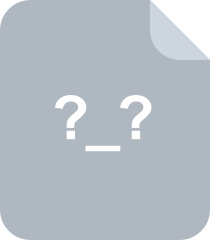
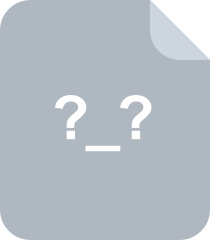
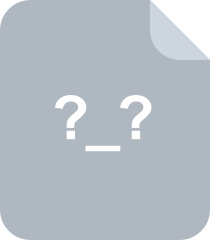
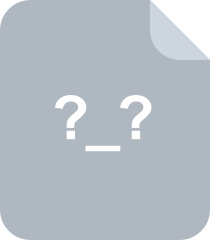
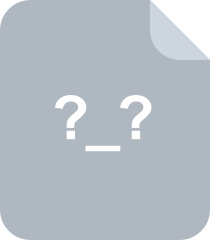
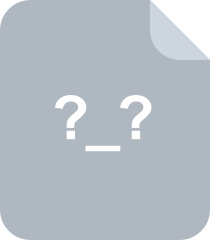
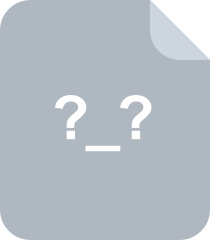
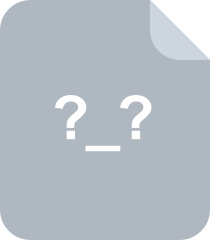
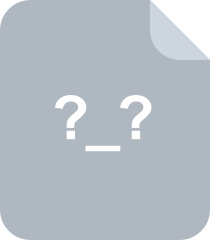
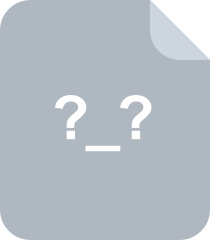
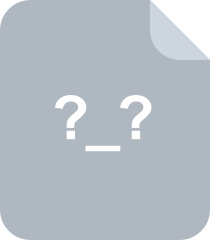
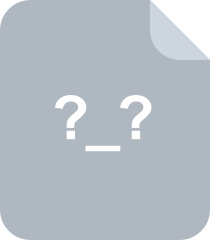
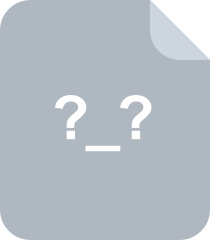
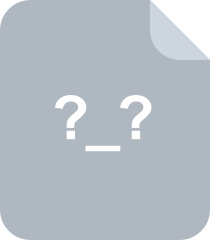
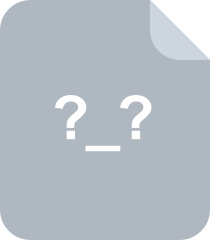
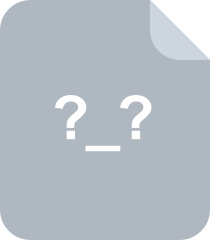
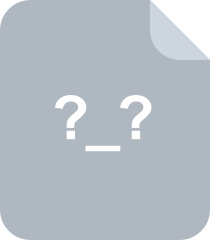
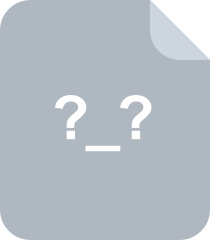
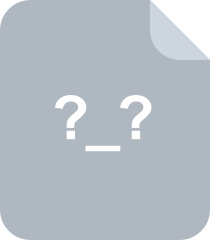
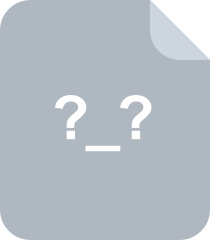
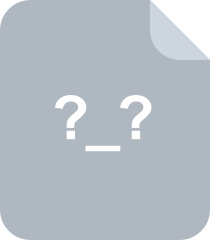
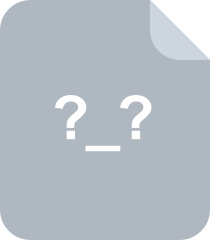
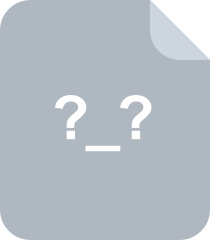
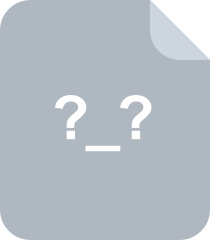
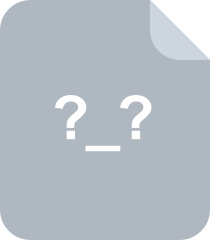
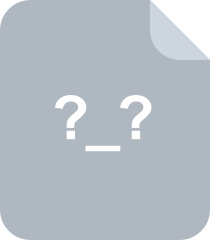
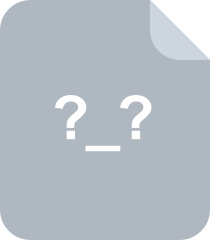
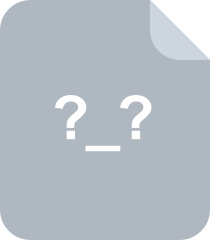
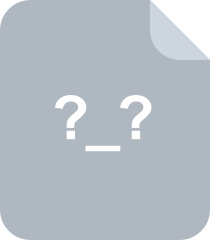
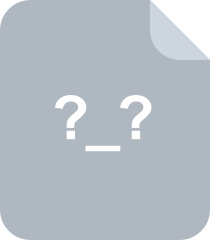
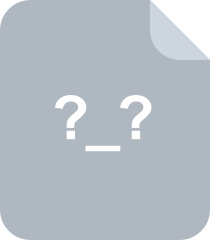
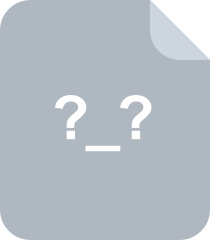
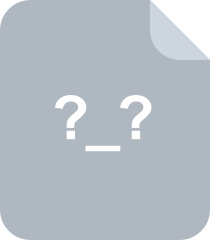
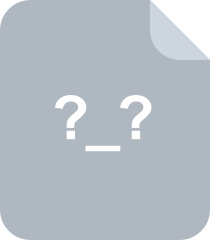
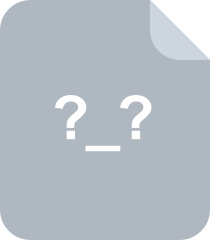
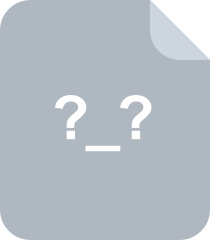
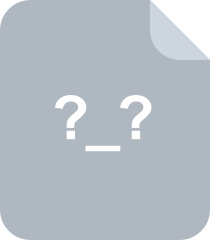
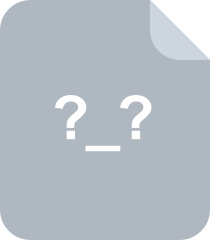
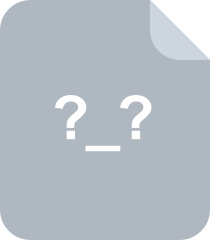
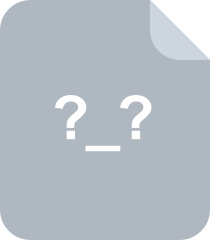
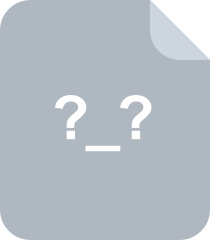
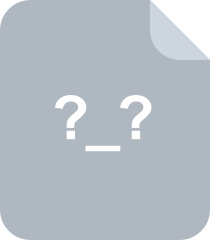
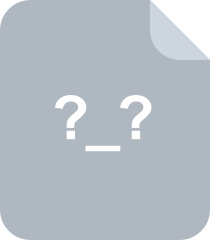
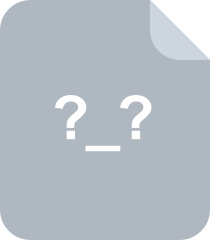
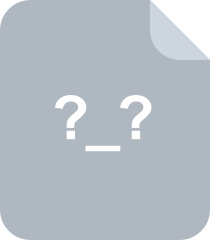
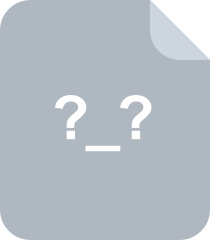
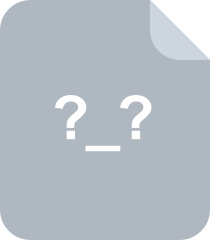
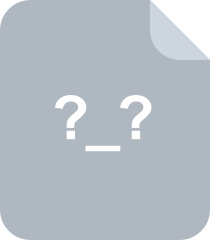
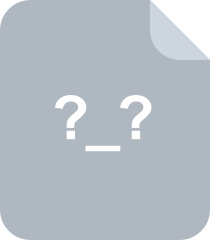
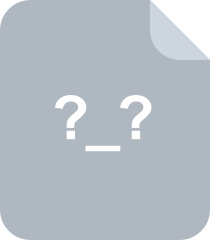
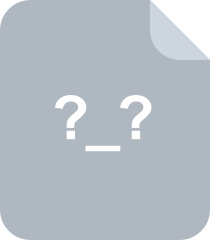
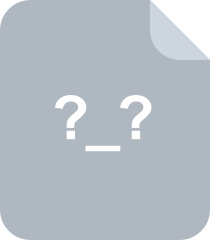
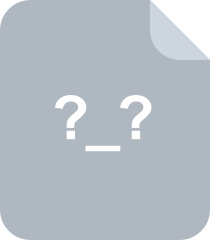
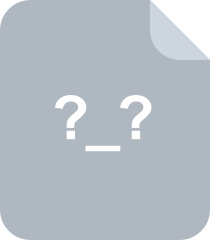
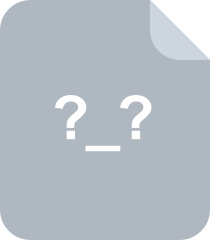
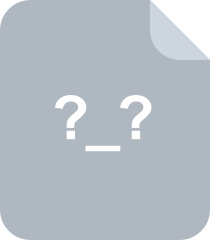
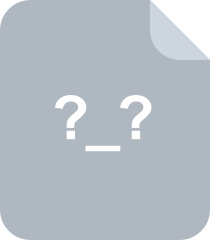
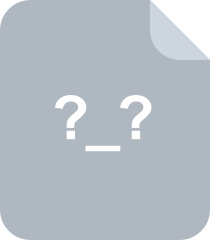
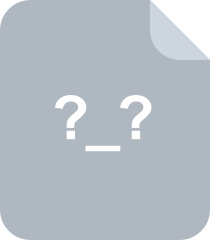
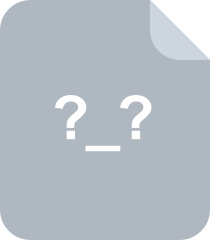
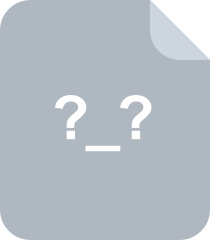
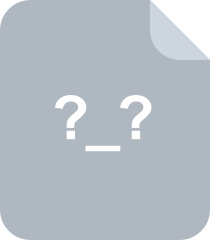
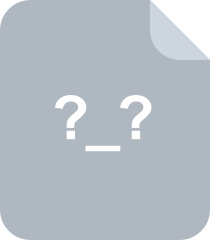
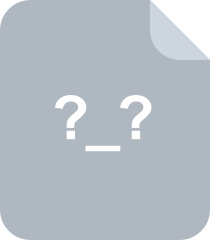
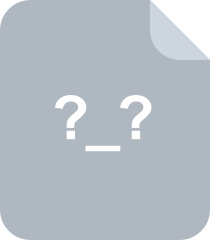
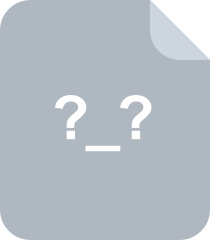
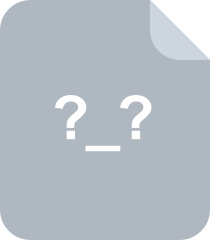
共 147 条
- 1
- 2
资源评论
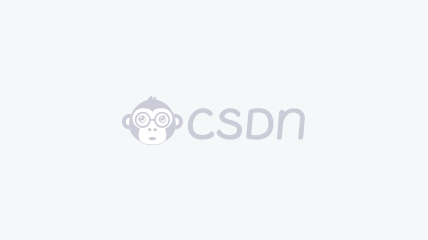

Xs_layla
- 粉丝: 1371
- 资源: 195
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

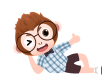
最新资源
- 系统学习linux命令
- java毕业设计-基于SSM的党务政务服务热线平台【代码+论文+PPT】.zip
- YOLOv3 在 GPU 上使用自己的数据进行训练 YOLOv3 的 Keras 实现.zip
- YOLOv3 和 YOLOv3-tiny 的 Tensorflow js 实现.zip
- 石头剪刀布-YOLOV7标记的数据集
- YOLOV3 pytorch 实现为 python 包.zip
- 石头剪刀布-YOLOV8标记的数据集
- YOLOv2 在 TF,Keras 中的实现 允许在不同的特征检测器(MobileNet、Darknet-19)上进行实验 论文.zip
- 石头剪刀布-YOLOV11标记的数据集
- YoloV1的tensorflow实现.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


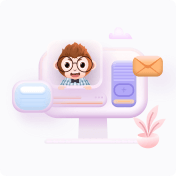
安全验证
文档复制为VIP权益,开通VIP直接复制
