<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>python智能运维</title>
<style>
/*这里是css样式*/
body {
font-family: Arial, sans-serif;
text-align: center;
background-color: #f5f5f5;
}
.header{
width: 100%;
height: 100px;
display: flex;
justify-content: center;
.header-text{
font-size: 30px;
color: #000;
font-weight: bold;
}
}
.container {
display: flex;
justify-content: center;
margin-top: 50px;
width: 100%;
}
.section {
border: 1px solid #ccc;
padding: 1%;
margin: 0 3.5%;
background-color: white;
width: 20%;
}
.section h3 {
margin-bottom: 20px;
}
.section button {
display: block;
width: 100%;
padding: 2.5%;
margin: 2% 0;
background-color: #5b9bd5;
color: white;
border: none;
cursor: pointer;
border-radius: 5px;
}
.section button:hover {
background-color: #45a049;
}
.result{
width: 100%;
display: flex;
justify-content: center;
.display{
width: 80%;
text-align: left;
.result-text{
height: 300px;
font-size: 16px;
color: #000;
border:1px solid #ccc;
background: #fff;
}
}
}
/* 弹出层样式 */
.modal {
display: none;
position: fixed;
z-index: 1;
left: 0;
top: 0;
width: 100%;
height: 100%;
overflow: auto;
background-color: rgba(0, 0, 0, 0.5);
}
.modal-content {
background-color: #fff;
margin: 15% auto;
padding: 20px;
border: 1px solid #888;
width: 450px;
}
.close {
color: #aaa;
float: right;
font-size: 28px;
font-weight: bold;
}
.close:hover,
.close:focus {
color: black;
text-decoration: none;
cursor: pointer;
}
.modal-content input {
width: 100%;
padding: 8px;
margin: 8px 0;
}
.modal-content button {
background-color: #5b9bd5;
color: white;
padding: 10px;
border: none;
width: 100%;
cursor: pointer;
border-radius: 5px;
}
.modal-content button:hover {
background-color: #45a049;
}
#deviceForm{
.form-item{
display: flex;
align-items: center;
label{
width: 35%;
text-align: left;
}
}
}
</style>
</head>
<body>
<div class="header">
<P class="header-text">python智能运维</P>
</div>
<div class="container">
<!-- 监控 Section -->
<div class="section">
<h3>监控</h3>
<button onclick="openModal('告警采集')">告警采集</button>
<button onclick="openModal('端口流量信息采集')">端口流量信息采集</button>
<button onclick="openModal('端口流量生成')">端口流量生成</button>
<button onclick="openModal('全量告警信息采集')">全量告警信息采集</button>
</div>
<!-- 故障处理 Section -->
<div class="section">
<h3>故障处理</h3>
<button onclick="openModal('Ping功能')">Ping功能</button>
<button onclick="openModal('添加配置')">添加配置</button>
<button onclick="openModal('全量配置采集')">全量配置采集</button>
<button onclick="openModal('OSPF信息采集')">OSPF信息采集</button>
</div>
<!-- 手动维护 Section -->
<div class="section">
<h3>手动维护</h3>
<button onclick="openModal('端口恢复')">端口恢复</button>
<button onclick="openModal('端口关闭')">端口关闭</button>
<button onclick="openModal('端口信息')">端口信息</button>
<button onclick="openModal('告警是否上报')">告警是否上报</button>
</div>
</div>
<!-- 接口返回的数据放在这里-->
<div class="result">
<div class="display">
<h3 class="modal-result">结果呈现</h3>
<div class="result-text"></div>
</div>
</div>
<!-- 弹出的窗口 -->
<div id="myModal" class="modal">
<div class="modal-content">
<span class="close" onclick="closeModal()">×</span>
<h3 id="modal-title">提交设备信息</h3> <!-- 标题动态更新 -->
<form id="deviceForm" onsubmit="submitForm(event)">
<div class="form-item">
<label for="ip">设备IP地址:</label>
<input type="text" id="ip" name="ip" required placeholder="输入IP地址">
</div>
<div class="form-item">
<label for="username">登录账号:</label>
<input type="text" id="username" name="username" required placeholder="输入登录账号">
</div>
<div class="form-item">
<label for="password">登录密码:</label>
<input type="password" id="password" name="password" required placeholder="输入登录密码">
</div>
<!-- 动态显示的端口信息部分 -->
<div id="port-section" class="port-section">
<div class="form-item">
<label for="port">端口:</label>
<input type="text" id="port" name="port" placeholder="输入端口">
</div>
</div>
<div id="peer-port-section" class="peer-port-section">
<div class="form-item">
<label for="peer_port">端口:</label>
<input type="text" id="peer_port" name="peer_port" placeholder="输入端口">
</div>
</div>
<div id="config-info-section" class="config-info-section">
<div class="form-item">
<label for="peer_port">配置指令</label>
<input type="text" id="config_info" name="config_info" placeholder="输入配置指令">
</div>
</div>
<button type="submit">提交</button>
</form>
</div>
</div>
</body>
<script>
//这里是js脚本
//一些动态变量可以调整
let baseUrl = 'http://127.0.0.1:57000'
let path = '/wqh'
let currentButtonText = ''; // 全局变量存储当前点击的按钮文字
//需要 port的字段
const portButtonText = ['端口信息','端口关闭','端口恢复']
//需要 peer_port的字段
const peerPortButtonText = ['Ping功能']
//需要 add_config的字段
const addConfigButtonText = ['添加配置']
//不需要参数的接口
const noParamButtonText = ['端口流量生成']
// 打开模态框
function openModal(buttonText) {
document.getElementById("modal-title").innerText = buttonText; // 动态设置标题
document.getElementById("myModal").style.display = "block";
// 检查是否是需要port的字段,如果是则显示端口输入框,否则隐藏
if (portButtonText.includes(buttonText)) {
document.getElementById("port-section").style.display = "block";
} else {
document.getElementById("port-section").style.display = "none";
}
// 检查是否是需要peer_port的字段,如果是则显示端口输入框,否则隐藏
if (peerPortButtonText.includes(buttonText)) {
document.getElementById("peer-port-section").style.display = "block";
} else {
document.getElementById("peer-port-section").style.display = "none";
}
// 检查是否是需要add_config的字段,如果是则显示配置输入框,否则隐藏
if (addConfigButtonText.includes(buttonText)) {
document.getElementById("config-info-section").style.display = "block";
} else {
document.getElementById("config-info-section").style.display = "none";
}
currentButtonText = buttonText;
}
// 关闭模态框
function closeModal() {
document.getElementById("myModal").style.display = "none";
document.getElementById("deviceForm").reset(); // 清空表单数据
}
// 表单提交事件处理
function submitForm(event) {
event.preventDefault(); // 防止表单自动提交
const ip = document.getElementById("ip").value;
const username = document.getElementById("username").value;
const password = d
没有合适的资源?快使用搜索试试~ 我知道了~
可视化python采集设备信息功能详细介绍
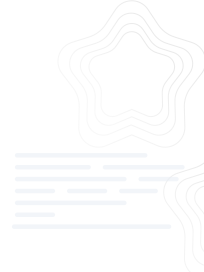
共4个文件
zip:1个
html:1个
pptx:1个

需积分: 0 0 下载量 95 浏览量
2024-10-10
15:28:21
上传
评论
收藏 1.29MB ZIP 举报
温馨提示
可视化python采集设备信息功能详细介绍
资源推荐
资源详情
资源评论
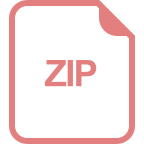
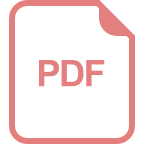
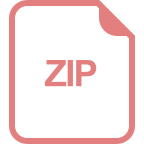
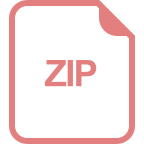
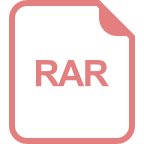
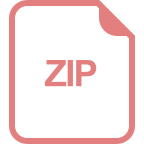
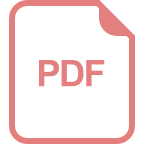
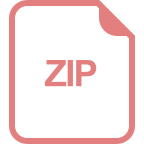
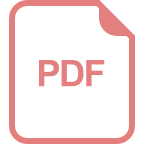
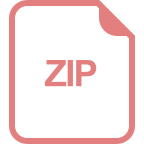
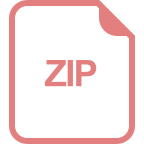
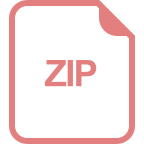
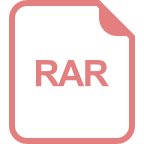
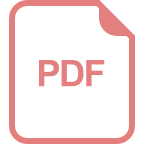
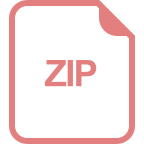
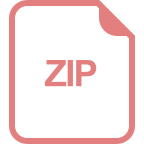
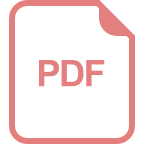
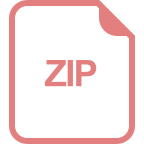
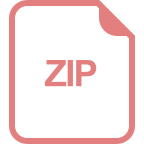
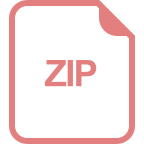
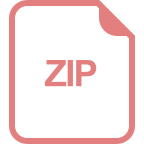
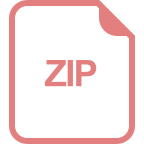
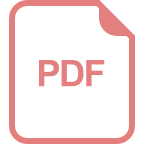
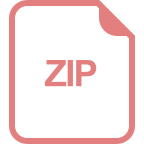
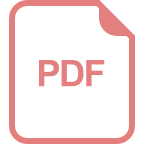
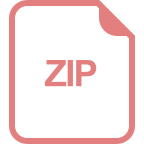
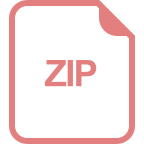
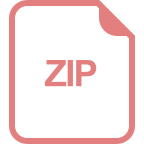
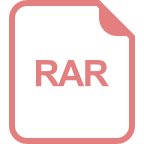
收起资源包目录


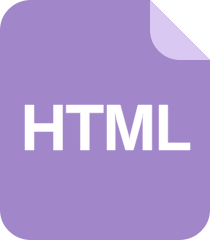
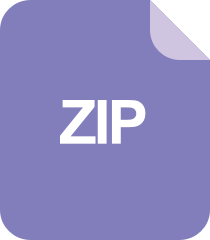
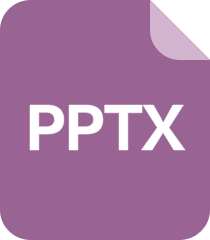
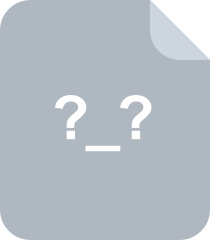
共 4 条
- 1
资源评论
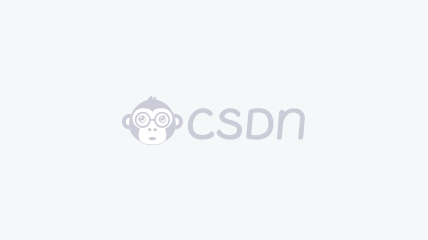

XmrZeiJbCool
- 粉丝: 24
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

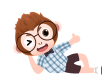
最新资源
- 基于CSS与JavaScript的积分系统设计源码
- 生物化学作业_1_生物化学作业资料.pdf
- 基于libgdx引擎的Java开发连连看游戏设计源码
- 基于MobileNetV3的SSD目标检测算法PyTorch实现设计源码
- 基于Java JDK的全面框架设计源码学习项目
- 基于Python黑魔法原理的Python编程技巧设计源码
- 基于Python的EducationCRM管理系统前端设计源码
- 基于Django4.0+Python3.10的在线学习系统Scss设计源码
- 基于activiti6和jeesite4的dreamFlow工作流管理设计源码
- 基于Python实现的简单植物大战僵尸脚本设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


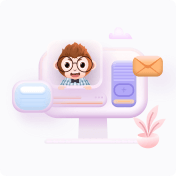
安全验证
文档复制为VIP权益,开通VIP直接复制
