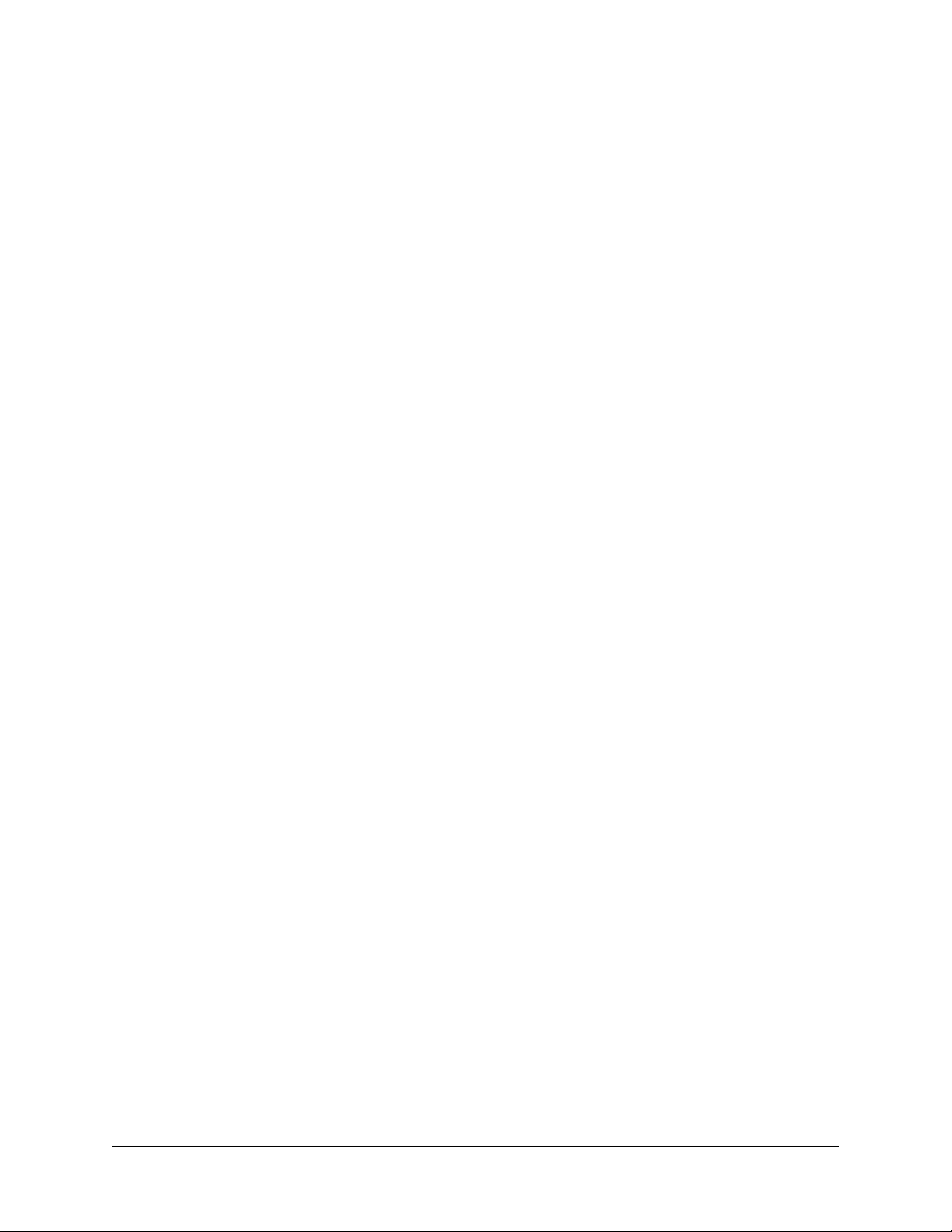
4.6 Assertion introspection details . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 29
5 pytest fixtures: explicit, modular, scalable 31
5.1 What fixtures are . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 32
5.2 “Requesting” fixtures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 33
5.3 Autouse fixtures (fixtures you don’t have to request) . . . . . . . . . . . . . . . . . . . . . . . . . . 38
5.4 Scope: sharing fixtures across classes, modules, packages or session . . . . . . . . . . . . . . . . . . 39
5.5 Fixture errors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 42
5.6 Teardown/Cleanup (AKA Fixture finalization) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 42
5.7 Safe teardowns . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 46
5.8 Fixture availability . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 48
5.9 Sharing test data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 51
5.10 Fixture instantiation order . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 51
5.11 Running multiple assert statements safely . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 57
5.12 Fixtures can introspect the requesting test context . . . . . . . . . . . . . . . . . . . . . . . . . . . . 59
5.13 Using markers to pass data to fixtures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 60
5.14 Factories as fixtures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 60
5.15 Parametrizing fixtures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 61
5.16 Using marks with parametrized fixtures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 64
5.17 Modularity: using fixtures from a fixture function . . . . . . . . . . . . . . . . . . . . . . . . . . . . 64
5.18 Automatic grouping of tests by fixture instances . . . . . . . . . . . . . . . . . . . . . . . . . . . . 65
5.19 Use fixtures in classes and modules with usefixtures . . . . . . . . . . . . . . . . . . . . . . . 67
5.20 Overriding fixtures on various levels . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 68
5.21 Using fixtures from other projects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 72
6 Marking test functions with attributes 73
6.1 Registering marks . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 73
6.2 Raising errors on unknown marks . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 74
7 Monkeypatching/mocking modules and environments 75
7.1 Simple example: monkeypatching functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 76
7.2 Monkeypatching returned objects: building mock classes . . . . . . . . . . . . . . . . . . . . . . . . 76
7.3 Global patch example: preventing “requests” from remote operations . . . . . . . . . . . . . . . . . 78
7.4 Monkeypatching environment variables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 79
7.5 Monkeypatching dictionaries . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 80
7.6 API Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 82
8 Temporary directories and files 83
8.1 The tmp_path fixture . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 83
8.2 The tmp_path_factory fixture . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 84
8.3 The ‘tmpdir’ fixture . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 84
8.4 The ‘tmpdir_factory’ fixture . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 85
8.5 The default base temporary directory . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 85
9 Capturing of the stdout/stderr output 87
9.1 Default stdout/stderr/stdin capturing behaviour . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 87
9.2 Setting capturing methods or disabling capturing . . . . . . . . . . . . . . . . . . . . . . . . . . . . 87
9.3 Using print statements for debugging . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 88
9.4 Accessing captured output from a test function . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 88
10 Warnings Capture 91
10.1 @pytest.mark.filterwarnings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 92
10.2 Disabling warnings summary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 93
10.3 Disabling warning capture entirely . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 93
10.4 DeprecationWarning and PendingDeprecationWarning . . . . . . . . . . . . . . . . . . . . . . . . . 93
ii