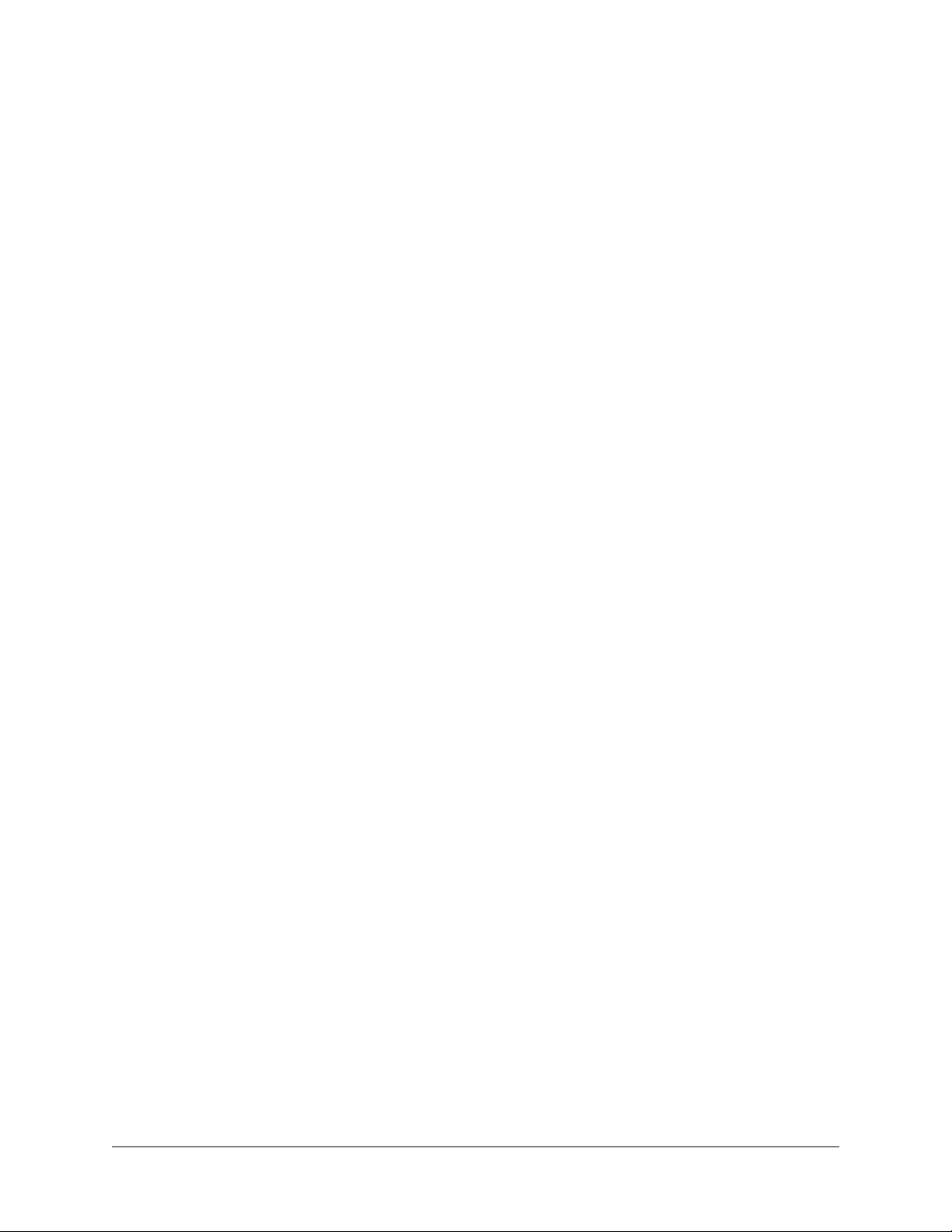
CONTENTS
1 Start here 3
1.1 Get Started . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
2 How-to guides 9
2.1 How to invoke pytest . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 9
2.2 How to write and report assertions in tests . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 12
2.3 How to use fixtures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 17
2.4 How to mark test functions with attributes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 46
2.5 How to parametrize fixtures and test functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 48
2.6 How to use temporary directories and files in tests . . . . . . . . . . . . . . . . . . . . . . . . . . . 52
2.7 How to monkeypatch/mock modules and environments . . . . . . . . . . . . . . . . . . . . . . . . . 54
2.8 How to run doctests . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 60
2.9 How to re-run failed tests and maintain state between test runs . . . . . . . . . . . . . . . . . . . . . 65
2.10 How to manage logging . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 71
2.11 How to capture stdout/stderr output . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 75
2.12 How to capture warnings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 77
2.13 How to use skip and xfail to deal with tests that cannot succeed . . . . . . . . . . . . . . . . . . . . 83
2.14 How to install and use plugins . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 90
2.15 Writing plugins . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 91
2.16 Writing hook functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 98
2.17 How to use pytest with an existing test suite . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 103
2.18 How to use unittest-based tests with pytest . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 103
2.19 How to run tests written for nose . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 107
2.20 How to implement xunit-style set-up . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 108
2.21 How to set up bash completion . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 110
3 Reference guides 111
3.1 Fixtures reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 111
3.2 Plugin List . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 121
3.3 Configuration . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 140
3.4 API Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 143
4 Explanation 239
4.1 Anatomy of a test . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 239
4.2 About fixtures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 240
4.3 Good Integration Practices . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 242
4.4 Flaky tests . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 246
4.5 pytest import mechanisms and sys.path/PYTHONPATH . . . . . . . . . . . . . . . . . . . . . . . 248
5 Further topics 251
i