# Cube SLAM #
This code contains two mode:
1) object SLAM integrated with ORB SLAM. See ```orb_object_slam``` Online SLAM with ros bag input. It reads the offline detected 3D object.
2) Basic implementation for Cube only SLAM. See ```object_slam``` Given RGB and 2D object detection, the algorithm detects 3D cuboids from each frame then formulate an object SLAM to optimize both camera pose and cuboid poses. is main package. ```detect_3d_cuboid``` is the C++ version of single image cuboid detection, corresponding to a [matlab version](https://github.com/shichaoy/matlab_cuboid_detect).
**Authors:** [Shichao Yang](https://shichaoy.github.io./)
**Related Paper:**
* **CubeSLAM: Monocular 3D Object SLAM**, IEEE Transactions on Robotics 2019, S. Yang, S. Scherer [**PDF**](https://arxiv.org/abs/1806.00557)
If you use the code in your research work, please cite the above paper. Feel free to contact the authors if you have any further questions.
## Installation
### Prerequisites
This code contains several ros packages. We test it in **ROS indigo/kinetic, Ubuntu 14.04/16.04, Opencv 2/3**. Create or use existing a ros workspace.
```bash
mkdir -p ~/cubeslam_ws/src
cd ~/cubeslam_ws/src
catkin_init_workspace
git clone git@github.com:shichaoy/cube_slam.git
cd cube_slam
```
### Compile dependency g2o
```bash
sh install_dependenices.sh
```
### Compile
```bash
cd ~/cubeslam_ws
catkin_make -j4
```
## Running #
```bash
source devel/setup.bash
roslaunch object_slam object_slam_example.launch
```
You will see results in Rviz. Default rviz file is for ros indigo. A kinetic version is also provided.
To run orb-object SLAM in folder ```orb_object_slam```, download [data](https://drive.google.com/open?id=1FrBdmYxrrM6XeBe_vIXCuBTfZeCMgApL). See correct path in ```mono.launch```, then run following in two terminal:
``` bash
roslaunch orb_object_slam mono.launch
rosbag play mono.bag --clock -r 0.5
```
To run dynamic orb-object SLAM mentioned in the paper, download [data](https://drive.google.com/drive/folders/1T2PmK3Xt5Bq9Z7UhV8FythvramqhOo0a?usp=sharing). Similar to above, set correct path in ```mono_dynamic.launch```, then run the launch file with bag file.
If compiling problems met, please refer to ORB_SLAM.
### Notes
1. For the online orb object SLAM, we simply read the offline detected 3D object txt in each image. Many other deep learning based 3D detection can also be used similarly especially in KITTI data.
2. In the launch file (```object_slam_example.launch```), if ```online_detect_mode=false```, it requires the matlab saved cuboid images, cuboid pose txts and camera pose txts. if ```true```, it reads the 2D object bounding box txt then online detects 3D cuboids poses using C++.
3. ```object_slam/data/``` contains all the preprocessing data. ```depth_imgs/``` is just for visualization. ```pred_3d_obj_overview/``` is the offline matlab cuboid detection images. ```detect_cuboids_saved.txt``` is the offline cuboid poses in local ground frame, in the format "3D position, 1D yaw, 3D scale, score". ```pop_cam_poses_saved.txt``` is the camera poses to generate offline cuboids (camera x/y/yaw = 0, truth camera roll/pitch/height) ```truth_cam_poses.txt``` is mainly used for visulization and comparison.
```filter_2d_obj_txts/``` is the 2D object bounding box txt. We use Yolo to detect 2D objects. Other similar methods can also be used. ```preprocessing/2D_object_detect``` is our prediction code to save images and txts. Sometimes there might be overlapping box of the same object instance. We need to filter and clean some detections. See the [```filter_match_2d_boxes.m```](https://github.com/shichaoy/matlab_cuboid_detect/blob/master/filter_match_2d_boxes.m) in our matlab detection package.
没有合适的资源?快使用搜索试试~ 我知道了~
SLAM-单目3D物体检测+SLAM算法实现源码.zip
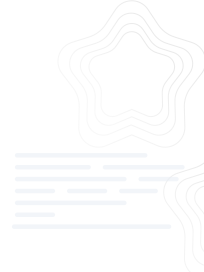
共623个文件
h:150个
jpg:118个
txt:90个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 57 浏览量
2024-05-15
09:46:42
上传
评论
收藏 8.34MB ZIP 举报
温馨提示
SLAM-单目3D物体检测+SLAM算法实现源码.zipSLAM-单目3D物体检测+SLAM算法实现源码.zipSLAM-单目3D物体检测+SLAM算法实现源码.zipSLAM-单目3D物体检测+SLAM算法实现源码.zipSLAM-单目3D物体检测+SLAM算法实现源码.zipSLAM-单目3D物体检测+SLAM算法实现源码.zipSLAM-单目3D物体检测+SLAM算法实现源码.zipSLAM-单目3D物体检测+SLAM算法实现源码.zipSLAM-单目3D物体检测+SLAM算法实现源码.zipSLAM-单目3D物体检测+SLAM算法实现源码.zip
资源推荐
资源详情
资源评论
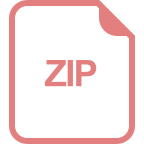
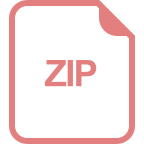
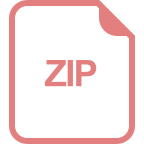
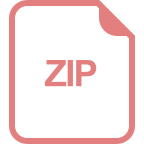
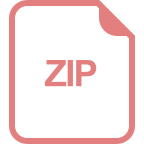
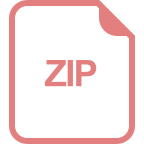
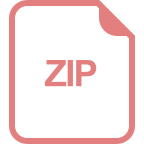
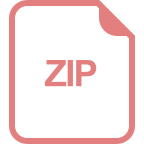
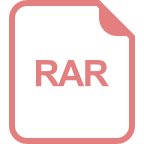
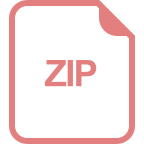
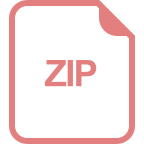
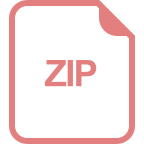
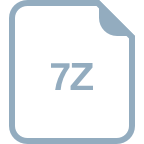
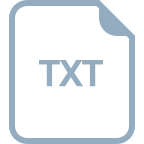
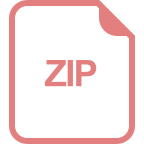
收起资源包目录

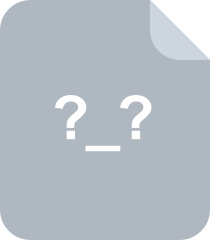
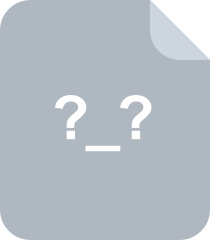
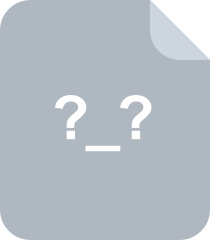
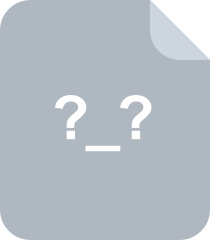
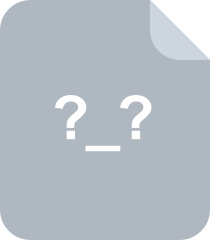
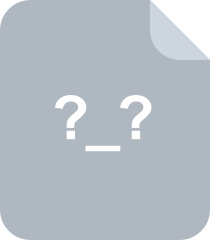
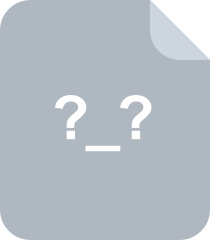
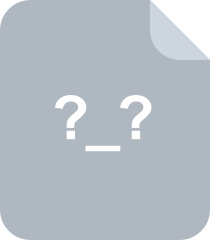
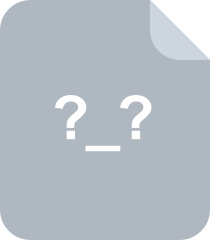
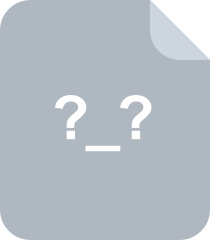
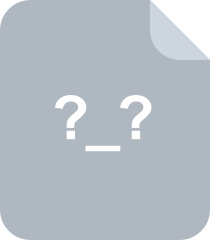
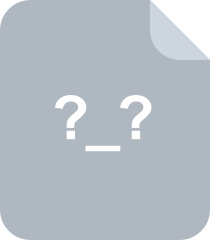
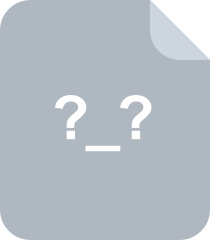
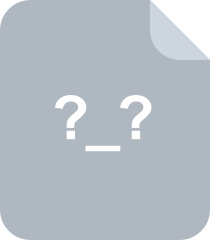
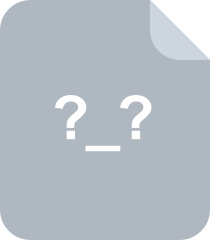
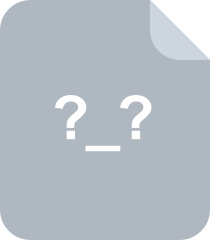
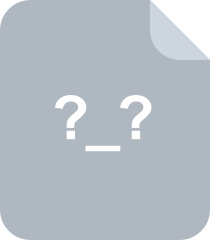
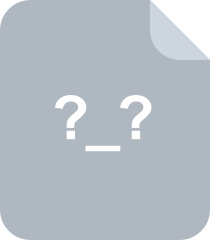
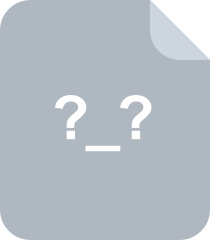
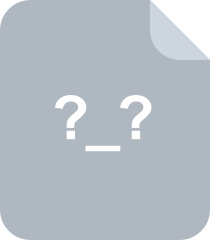
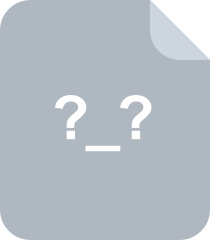
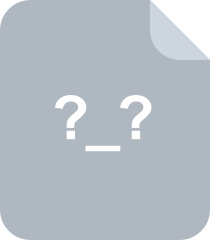
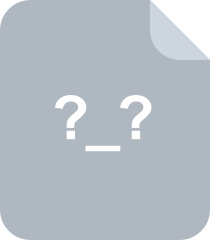
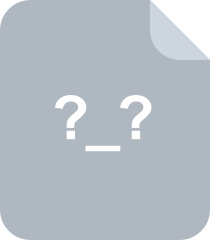
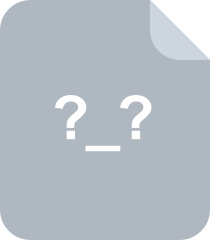
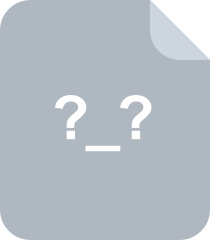
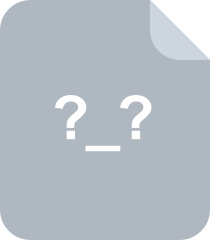
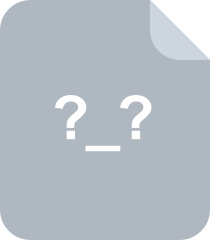
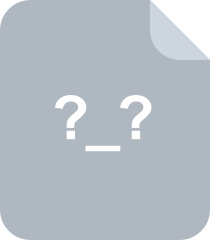
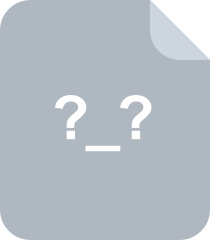
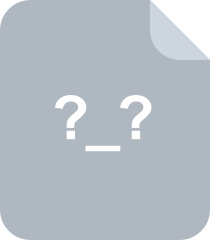
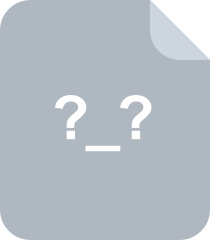
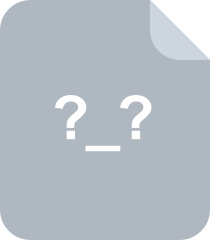
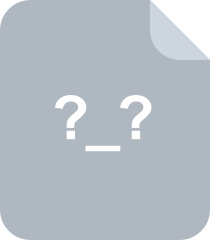
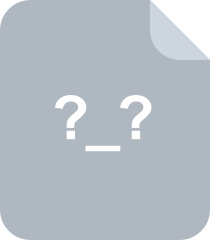
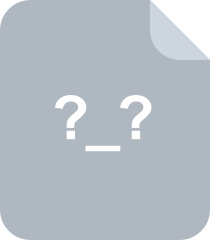
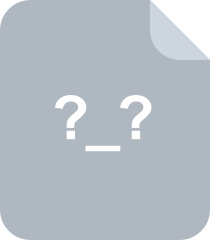
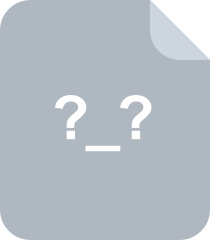
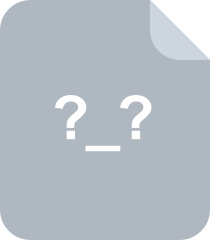
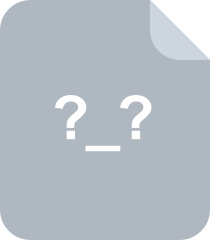
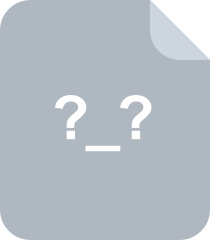
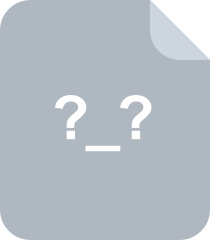
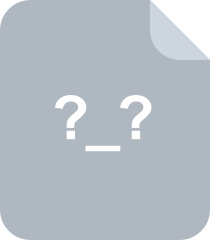
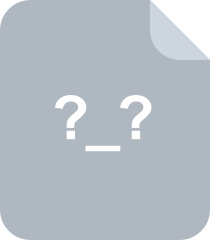
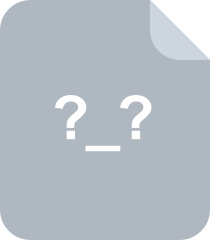
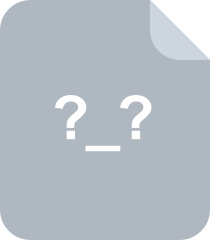
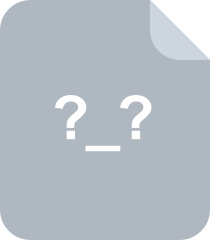
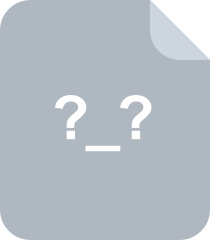
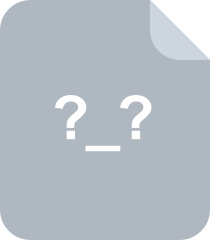
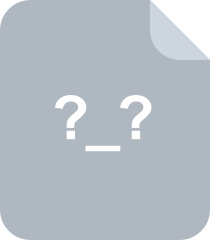
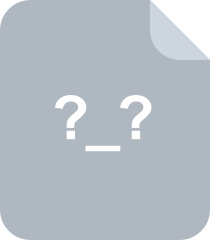
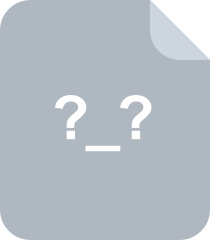
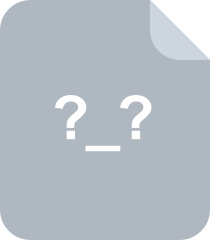
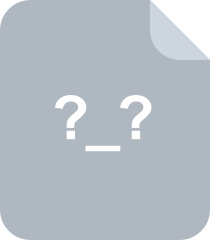
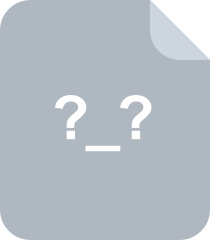
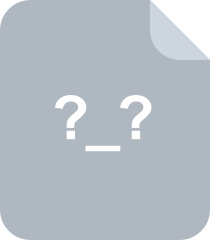
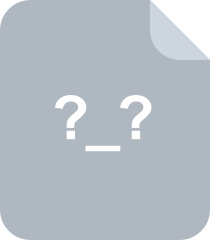
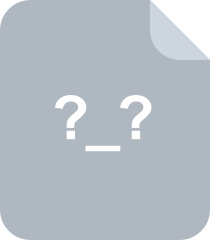
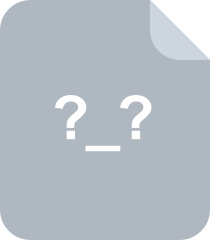
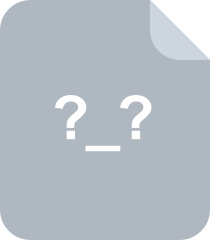
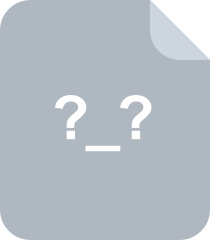
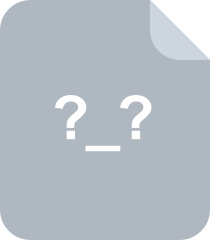
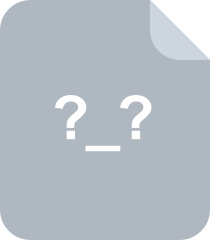
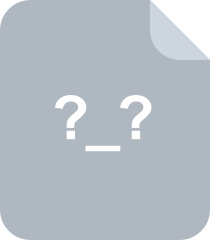
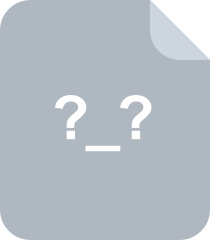
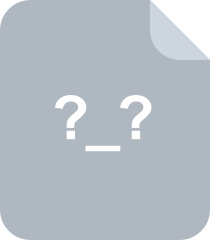
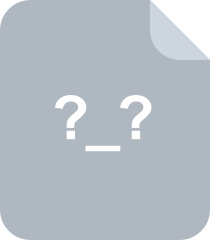
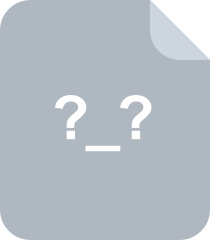
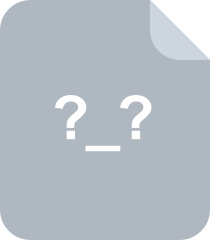
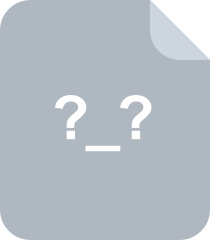
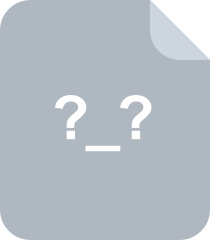
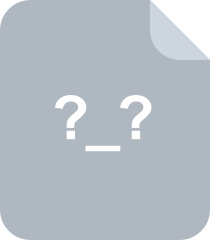
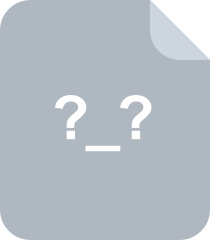
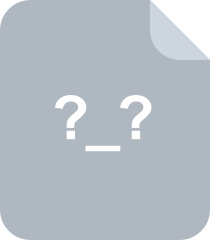
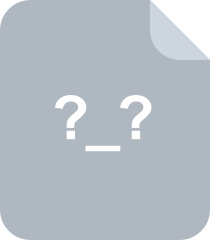
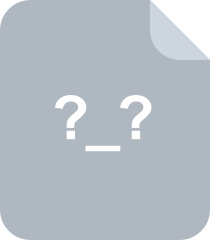
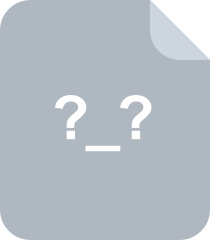
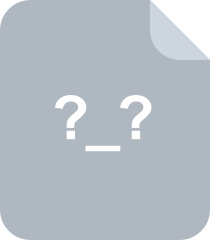
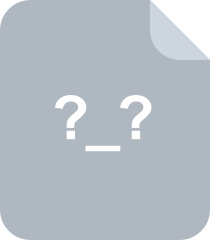
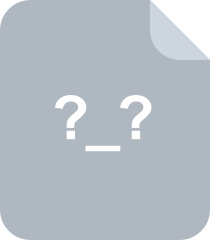
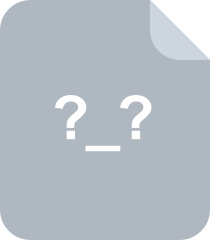
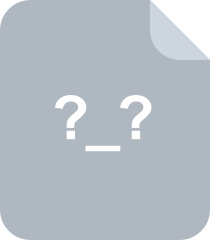
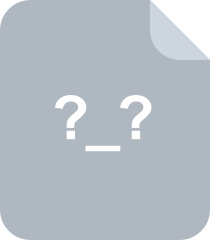
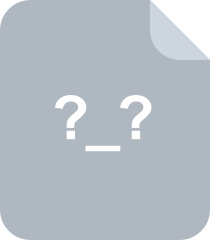
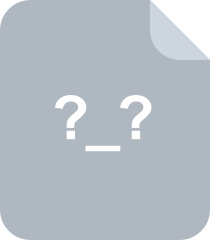
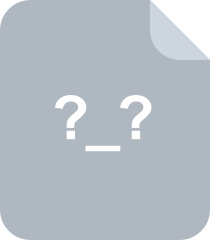
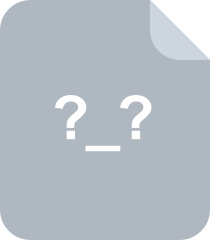
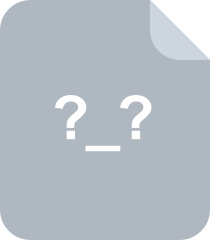
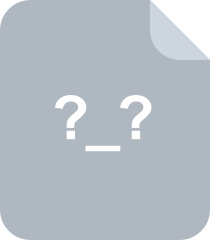
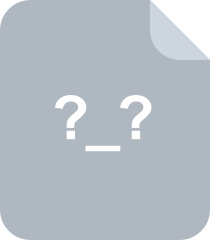
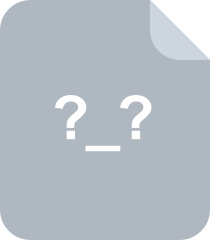
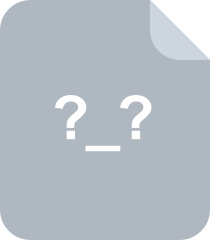
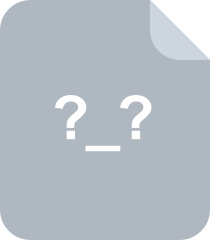
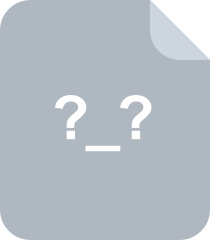
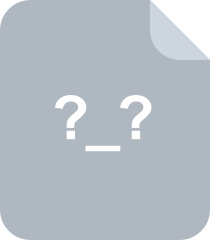
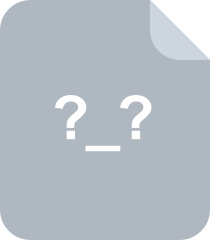
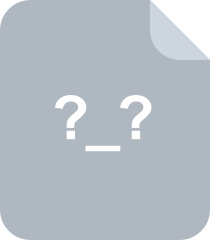
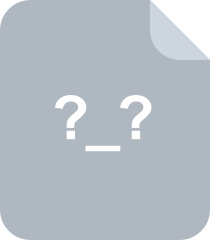
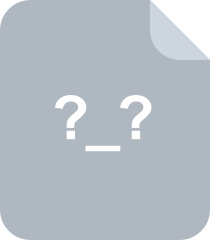
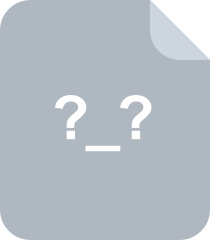
共 623 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源评论
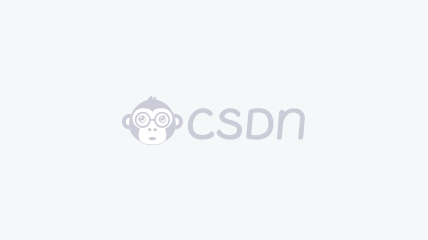

FL1768317420
- 粉丝: 4927
- 资源: 5741

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

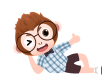
最新资源
- 华为打印机,华为打印机资料
- mac os button功能demo
- 如何在Ubuntu上安装软件?
- 华为HCIA-WLAN 3.0 课程视频(20 熟悉命令行.mp4)
- 三峡职业技术学院的GeoJSON 坐标点数据集
- 华为HCIA-WLAN 3.0 课程视频(19 华为VRP系统概述(下).mp4)
- 三峡职业技术学院的GeoJSON地图区域数据
- 华为HCIA-WLAN 3.0 课程视频(18 华为VRP系统概述(上).mp4)
- 编程实战项目:基于asp.net技术的学生成绩管理系统(最全的源代码+最全的文档)
- 华为HCIA-WLAN 3.0 课程视频(17 Wi-Fi6产品介绍(2).mp4)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


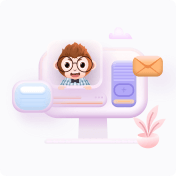
安全验证
文档复制为VIP权益,开通VIP直接复制
