# rosserial for VEX Cortex
This package contains everything needed to run rosserial on the [VEX Cortex](https://www.vexrobotics.com/276-2194.html), on the [PROS Kernel](https://pros.cs.purdue.edu/cortex/index.html).
# Requirements
- Software:
1. Linux (Only tested on Ubuntu 18.04LTS) (possible on windows with a Linux virtual machine, provided there is USB support)
2. ROS installed on Linux (Only tested on ROS Melodic) - [installation guide](http://wiki.ros.org/melodic/Installation/Source).
3. PROS installed on Linux - [installation guide](https://pros.cs.purdue.edu/cortex/getting-started/index.html)
- Hardware:
1. VEX essentials:
- VEX Cortex
- VEX Joystick
- VEXnet keys
- VEX Competition battery
- VEX Programming Cable
2. (optional, for debugging) a [USB-serial adapter](https://www.adafruit.com/product/954)
3. (optional, for debugging) Three Male-Male jumper wires for USB-serial adapter
# Table Of Contents
- [Setup](#setup)
- [Examples](#examples)
- [Hello World Example](#hello-world-example)
- [Keyboard or Android Phone Driving Example](#keyboard-or-android-driving-example)
- [Alternative Joystick Example](#alternative-joystick-example)
- [Physical Serial Connections](#physical-serial-connections)
- [Generating Custom Messages](#generating-custom-messages)
- [Limitations](#limitations)
- [Speed](#speed)
- [Troubleshooting](#troubleshooting)
# Setup
This setup requires knowledge of entering commands into a Linux terminal.
Note: it is possible to follow along with this guide without understanding basic ROS constructs
(such as workspaces, projects, rosrun, roslaunch, and catkin_make). However, in order to work
with ROS beyond the examples in this project, you will need to learn about the ROS framework itself. See the
[ROS documentation, getting-started, and tutorials pages](http://wiki.ros.org/) for more information.
### ROS Workspace
This workspace is used to generate a PROS project, and then help the Cortex interact with ROS using that generated project.
Notice the first `source` command below. This includes ROS commands into the terminal (such as catkin_make), so it will come first in
most of the terminal commands in this setup process. Make sure you replace `melodic` with your corresponding ROS version name, if it
is not `melodic` (e.g. `kinetic`).
Open up a terminal, and enter:
```bash
source /opt/ros/melodic/setup.bash
mkdir -p ~/ros-vex-workspace/src; cd ~/ros-vex-workspace/src
git clone https://github.com/ros-drivers/rosserial.git
cd ..; catkin_make; catkin_make install
```
Next, generate a PROS project, which has the code that runs on the Cortex.
The project can exist anywhere (it does NOT need to be inside the ROS workspace).
```bash
source ~/ros-vex-workspace/install/setup.bash
rosrun rosserial_vex_cortex genscript.sh ~/path/to/prosproject
```
# Examples
These examples are made to run out-of-the-box, and they made to be proof-of-concepts of what ROS can provide.
ROS allows standardized messages to be sent to and from the Cortex and an outside computer, which allows for all kinds
of ideas and projects to be organized with messages!
To understand what is going on with the example code, look at the tutorials for the sister project, [Rosserial Arduino](http://wiki.ros.org/rosserial_arduino/Tutorials).
There are some differences between the two projects (namely, in the PROS c++ code, [global scope is not allowed](#limitations)).
Set up the physical download connection by plugging in
the VEX Programming cable to the computer and the joystick, and then pluging the VEXnet keys into the Cortex and the joystick.
This connection serves as BOTH the downloading channel AND the serial connection, so leave the
programming cable plugged in! (to alter the connection to use something else, see [physical serial connections](#physical-serial-connections)).
Between downloads, power-cycle the Joystick and Cortex for optimal usage.
## Hello World Example
```bash
source ~/your-workspace-name/install/setup.bash
cd ~/path/to/prosproject
pros make upload; roslaunch rosserial_vex_cortex hello_world.launch
```
If everything is working properly, you should see "hello world" messages in the terminal!
## Keyboard or Android Driving Example
This example showcases an integrated demo with a VEX EDR Robot, such as the [clawbot](https://www.vexrobotics.com/276-2600.html), and an alternative method of control: a keyboard!
Open up "src/twistdrive.cpp" and modify the motor control code, to specify how you want to control your robot's drive .
Modify `src/opcontrol.cpp` in your generated PROS project to include the `twistdrive.cpp` file instead of the `helloworld.cpp` file. Then, open a terminal and run the following:
```bash
source ~/your-workspace-name/install/setup.bash
cd /path/to/prosproject
pros make clean; pros make upload; roslaunch rosserial_vex_cortex minimal_robot.launch
```
Android phones can now control this robot.
Download this [ROS Map Navigation](https://play.google.com/store/apps/details?id=com.github.rosjava.android_apps.map_nav.kinetic) app.
Configure the app to connect to your computer, and you can now drive your robot with a phone!
For keyboard input instead, install the keyboard twist publisher: http://wiki.ros.org/teleop_twist_keyboard
Run the following in another terminal:
```bash
source /opt/ros/melodic/setup.bash
rosrun teleop_twist_keyboard teleop_twist_keyboard.py
```
Now, you can use the keys listed in the command to drive the robot!
## Alternative Joystick Example
This is for using an alternative joystick, such as a Logitech Wireless Gamepad F710 or a PS3 controller.
This controlling example is extensibile: anything publishing a [sensor_msgs/Joy message](http://wiki.ros.org/sensor_msgs) can control your VEX robot,
so feel free to program your own controller!
Open up `src/joydrive.cpp` and make modifications to make this demo work on your robot.
Modify `src/opcontrol.cpp` in your generated PROS project to include the `joydrive.cpp` file instead of the `helloworld.cpp` file. Then, open a terminal and run the following:
```bash
cd /path/to/prosproject
pros make upload
roslaunch rosserial_vex_cortex joystick.launch
```
Now, you can use the alternative joystick to control the robot!
# Physical Serial Connections
The optimal setup for this project is with two physical serial connections, one for rosserial to function, and one for debugging. The default connection for rosserial is the VEX Programming Cable, and the default debugging serial connection is UART2.
Since the The VEX programming cable provides the default rosserial connection, the baud rate of the ROS serial connecting node must be 115200 Hz, which is why the command reads:
This overrides the default (57600) Hz. To switch the rosserial/debug serial connections, see `include/ros_lib/logger.h` in your generated PROS project.
If you do end up using UART1 or UART2 for rosserial instead of debugging,
open the `launch/` directory (which is inside the ROS workspace in `src/rosserial/rosserial_vex_cortex`).
Inside `launch/minimal_robot.launch`, update the `mainport` USB device argument to be `/dev/ttyUSB0`,
and update the `mainbaud` argument to be `57600`.
There are also `debugport` and `debugbaud` arguments in the launchfile, which are unused for now but can be used for project generation later.
Also, the USB device path for the VEX Programming cable on Linux may either be `/dev/ttyACM0` or `/dev/ttyACM1`. To figure out which to use as an argument, use `pros lsusb`, or unplug/replug the cable from/into the computer and run `dmesg` and look at the last lines. Usually, unplugging the programming cable for 10 seconds and then re-plugging it should revert
the device back to `/dev/ttyUSB0`
Viewing the UART debug stream requires a [USB-serial adapter](https://www.adafruit.com/product/954) for your computer, and it needs to be plugged in correctly.
To set up the wires with the cable linked above, use this layout:
[layout](./uartdiagram.
没有合适的资源?快使用搜索试试~ 我知道了~
基于ROS和arduino实现的履带车控制程序完整代码(上位机+下位机)+使用说明,含激光雷达、GPS等传感器信号的数据读取
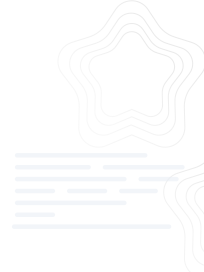
共421个文件
h:79个
cpp:72个
py:57个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 52 浏览量
2024-07-23
16:06:27
上传
评论
收藏 886KB ZIP 举报
温馨提示
这是一个关于履带车的控制程序,包括上位机(ROS)和下位机arduino代码,以及对激光雷达、IMU、kinect v2.0、GPS等传感器信号的数据读取。 本程序包含: 1、履带车底盘驱动程序 2、激光雷达驱动程序 3、惯性元件IMU驱动程序 4、GPS元件驱动程序 你需要配置ROS运行环境。同时设置好USB端口号,建议用UDEv端口配置规则 运行: 1、履带车底盘驱动程序---rosrun rosserial_python serial_node 2、激光雷达驱动程序-----roslaunch rplidar_ros rplidar_s1.launch 3、惯性元件GPS驱动程序---roslaunch nmea_navsat_driver nmea_serial_driver.launch 4、IMU元件驱动程序----roslaunch razor_imu_9dof razor-pub.launch
资源推荐
资源详情
资源评论
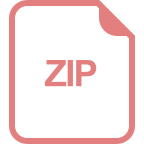
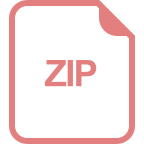
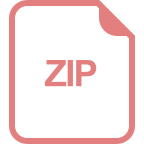
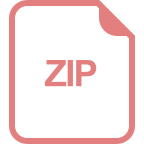
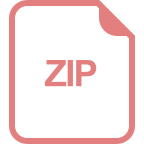
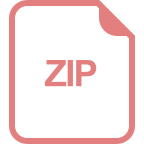
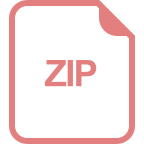
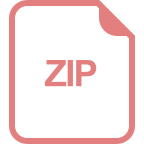
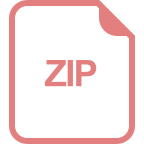
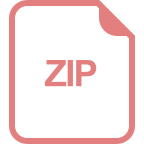
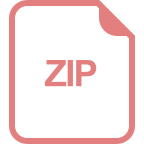
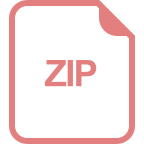
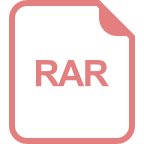
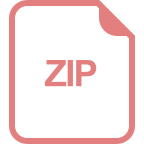
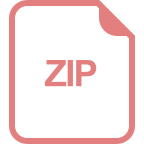
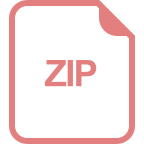
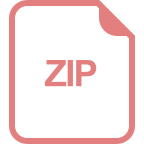
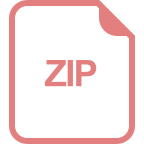
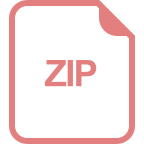
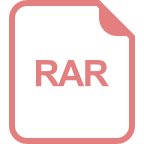
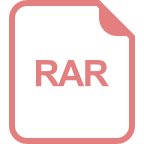
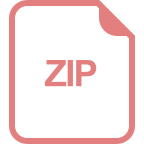
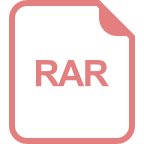
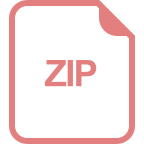
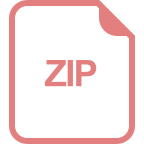
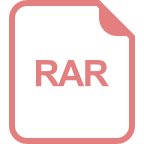
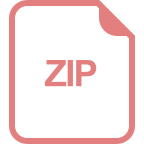
收起资源包目录

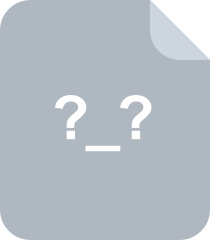
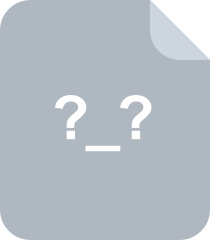
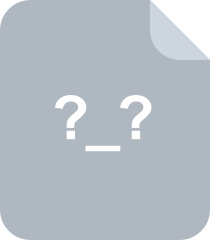
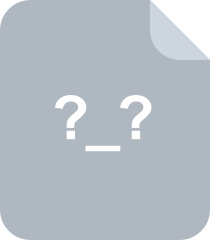
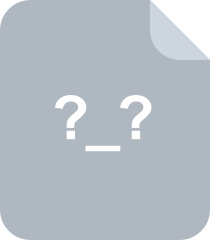
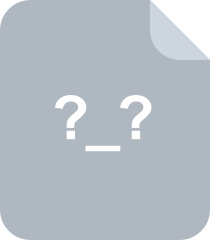
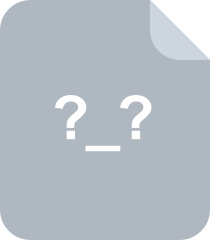
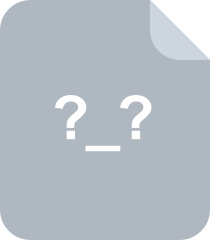
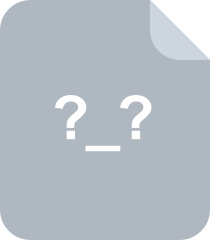
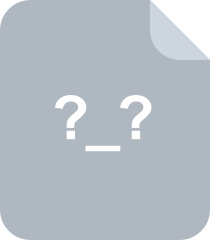
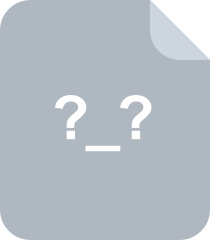
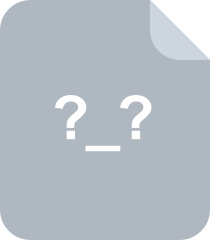
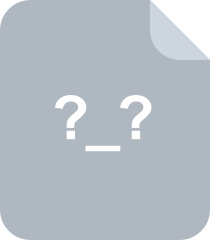
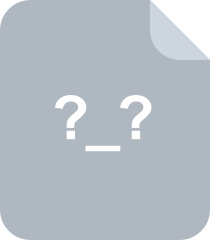
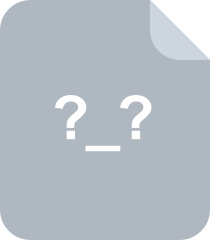
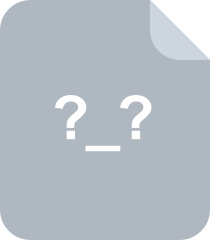
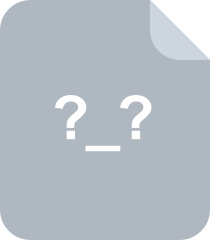
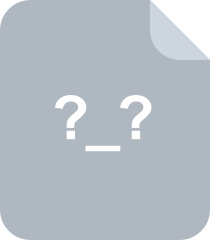
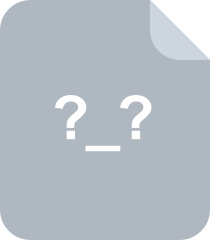
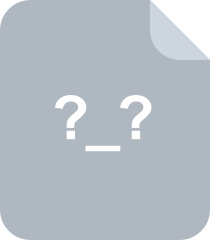
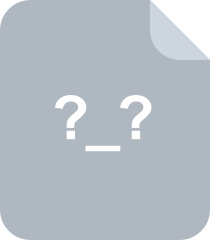
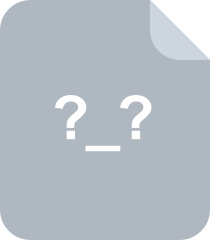
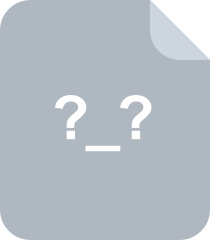
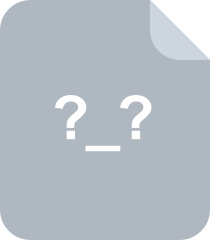
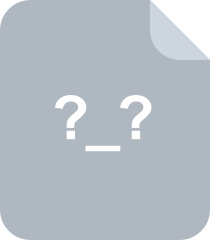
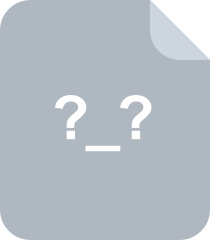
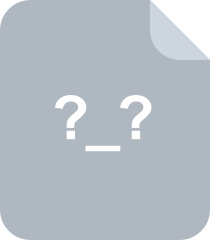
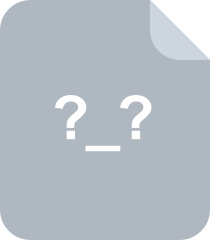
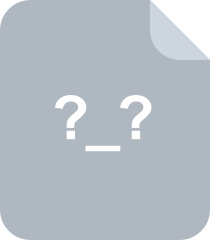
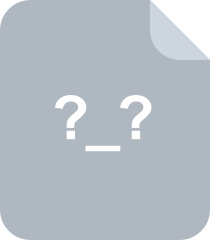
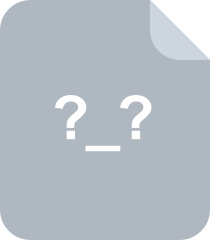
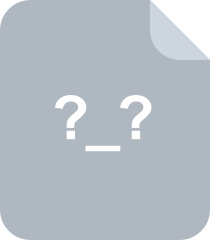
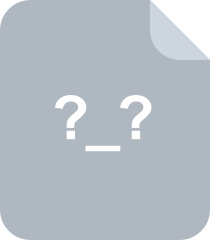
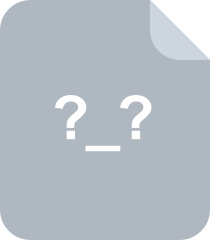
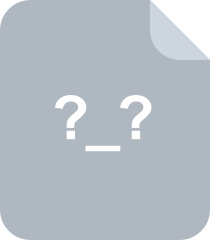
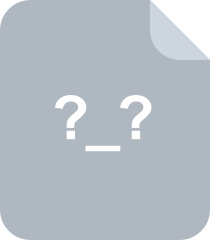
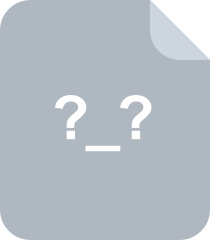
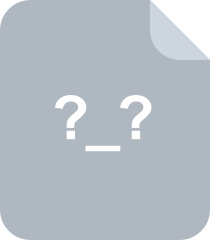
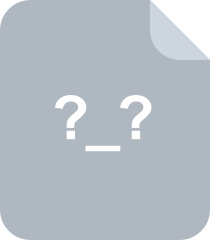
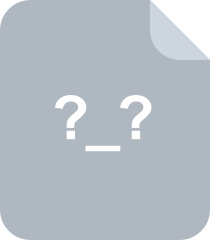
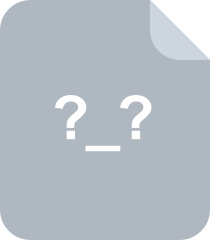
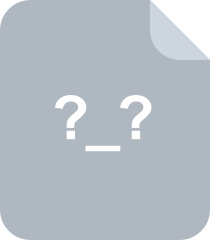
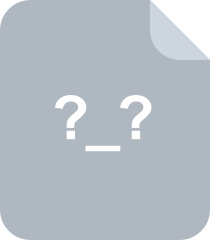
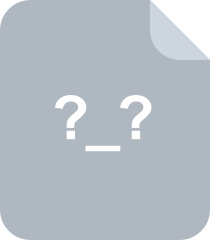
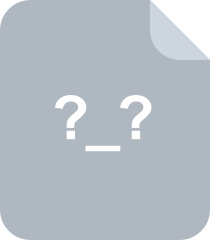
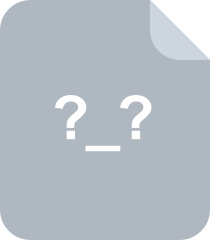
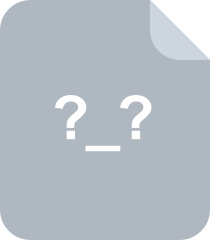
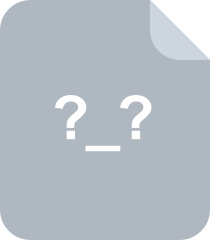
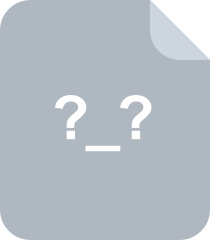
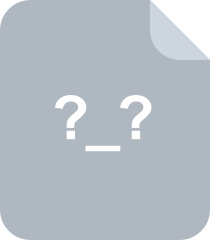
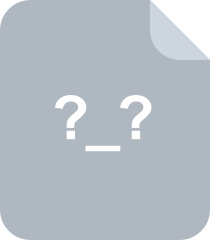
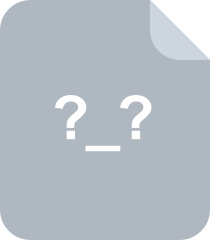
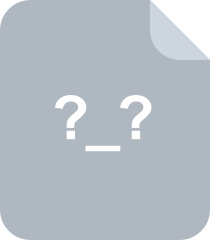
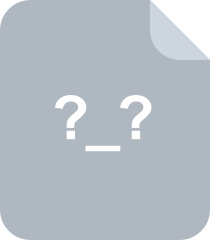
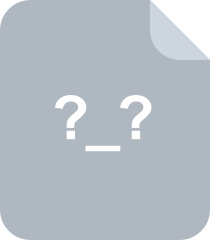
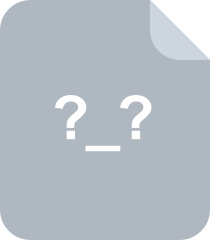
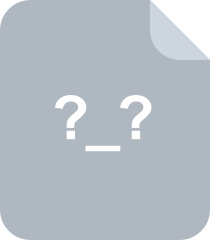
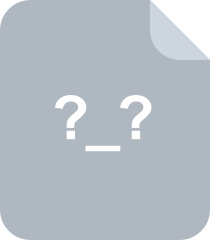
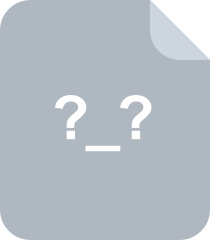
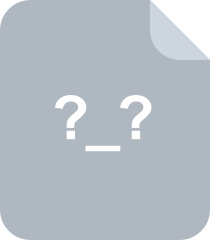
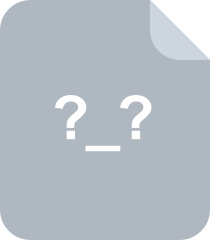
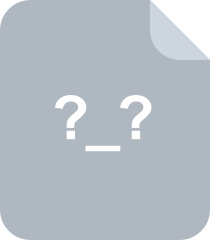
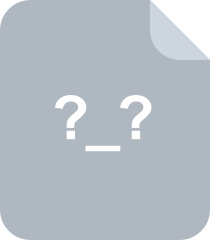
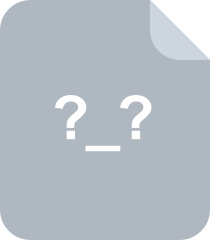
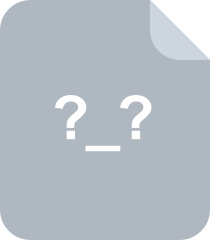
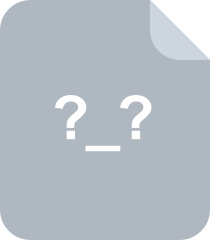
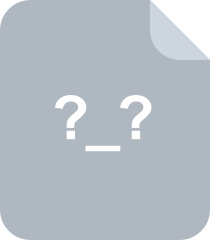
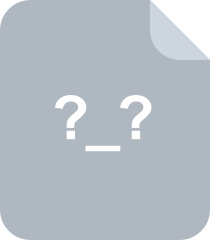
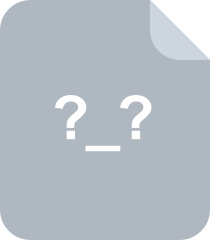
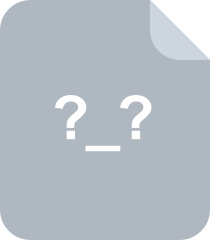
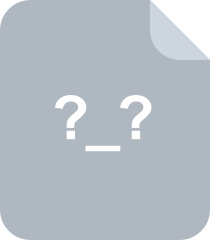
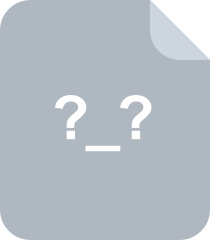
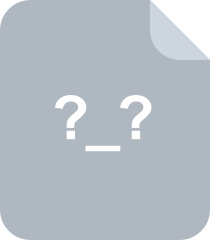
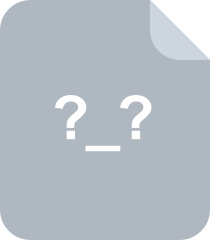
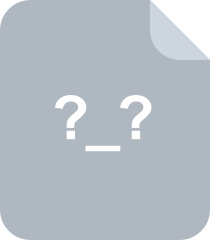
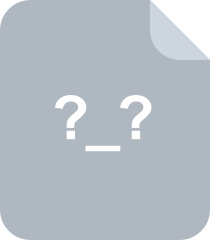
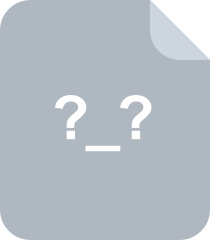
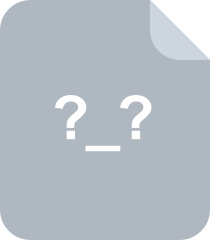
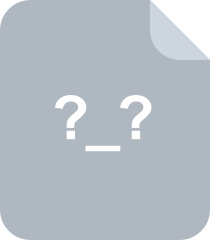
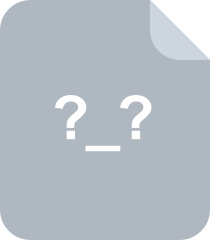
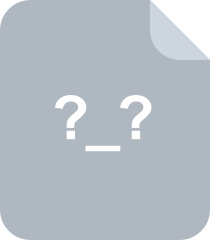
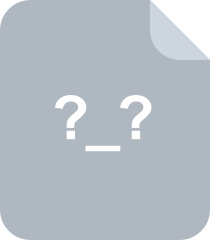
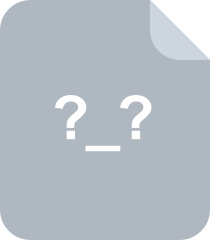
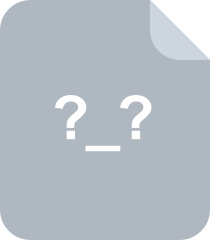
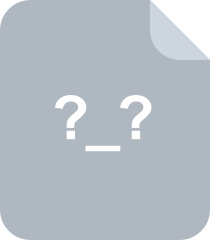
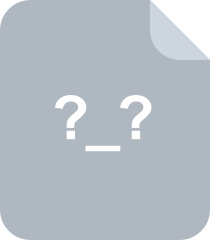
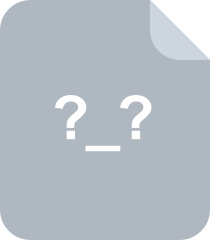
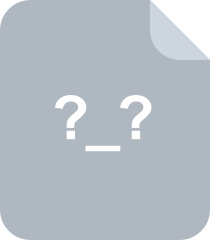
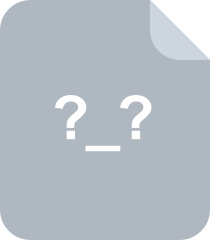
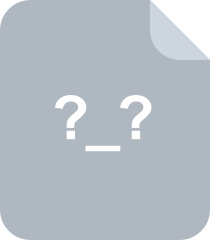
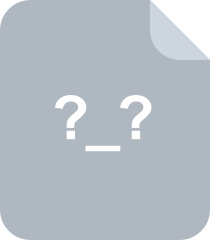
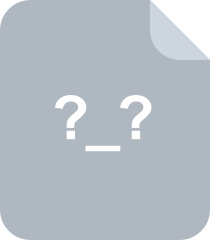
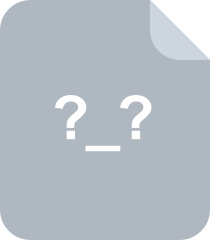
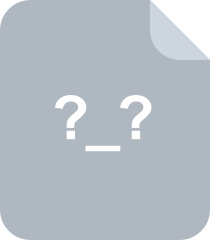
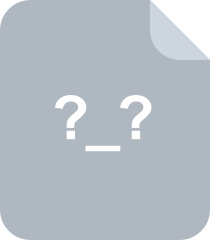
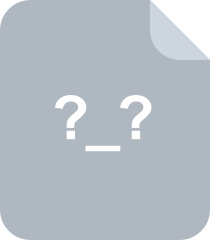
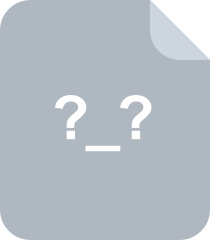
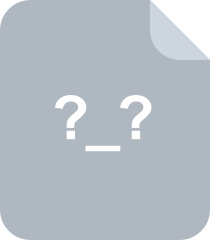
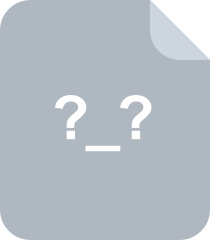
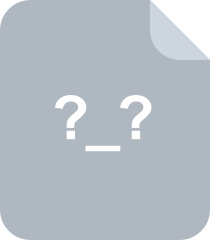
共 421 条
- 1
- 2
- 3
- 4
- 5
资源评论
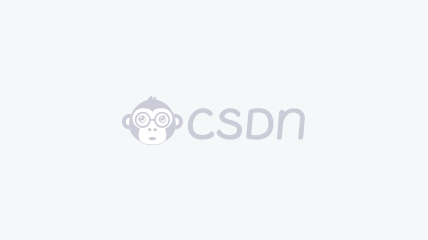

yanglamei1962
- 粉丝: 2468
- 资源: 794

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

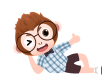
最新资源
- js-leetcode题解之158-read-n-characters-given-read4-ii-call
- js-leetcode题解之157-read-n-characters-given-read4.js
- js-leetcode题解之156-binary-tree-upside-down.js
- js-leetcode题解之155-min-stack.js
- js-leetcode题解之154-find-minimum-in-rotated-sorted-array-ii.js
- js-leetcode题解之153-find-minimum-in-rotated-sorted-array.js
- js-leetcode题解之152-maximum-product-subarray.js
- js-leetcode题解之151-reverse-words-in-a-string.js
- js-leetcode题解之150-evaluate-reverse-polish-notation.js
- js-leetcode题解之149-max-points-on-a-line.js
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


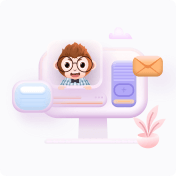
安全验证
文档复制为VIP权益,开通VIP直接复制
