# HPSocket.Net
## Overview
the C# SDK for [HP-Socket](https://github.com/ldcsaa/HP-Socket)
#### .Net Framework Supported
* `.Net Framework 2.0+`
* `.Net Core 2.0+`
* `.Net 5.0`
* `.Net 6.0`
#### Platform supported
* `Windows 7+ x86/x64`
* `Linux kernel 2.6.32+ x86/x64`
* `mac OS 10.12+ x64`
## Installation Guide
`HPSocket.Net` deploy via NuGet package manager
Use the following command in the Package Manager console to manually install `HPSocket.Net`
```
Install-Package HPSocket.Net
```
Or right-click on the project name in the solution of Visual Studio-> Manage NuGet Packages-> Browse the page-> search box and enter HPSocket.Net and then install
### About macOS
`HPSocket.Net` now supports development using` .net core2.0 + `in` osx 10.12 + `
`Libhpsocket4c.dylib` in Nuget package is compiled from [HP-Socket-for-macOS](https://gitee.com/xin_chong/HP-Socket-for-macOS)
## Components List
### Basic Components
Basic component is the original component provided by HP-Socket. For related usage, please refer to [HP-Socket Doc](https://github.com/ldcsaa/HP-Socket/tree/master/Doc)
##### TCP
+ `ITcpServer`
+ `ITcpAgent`
+ `ITcpClient`
+ `ITcpPullServer`
+ `ITcpPullAgent`
+ `ITcpPullClient`
+ `ITcpPackServer`
+ `ITcpPackAgent`
+ `ITcpPackClient`
##### UDP
+ `IUdpServer`
+ `IUdpClient`
+ `IUdpCast`
+ `IUdpArqServer`
+ `IUdpArqClient`
+ `IUdpNode`
##### SSL
+ `ISslServer`
+ `ISslAgent`
+ `ISslClient`
+ `ISslPullServer`
+ `ISslPullAgent`
+ `ISslPullClient`
+ `ISslPackServer`
+ `ISslPackAgent`
+ `ISslPackClient`
##### HTTP
+ `IHttpServer`
+ `IHttpsServer`
+ `IHttpAgent`
+ `IHttpsAgent`
+ `IHttpClient`
+ `IHttpsClient`
+ `IHttpSyncClient`
+ `IHttpsSyncClient`
#### ThreadPool
+ `ThreadPool`
### Extended components
+ `ITcpPortForwarding`
+ `IHttpEasyServer`
+ `IHttpsEasyServer`
+ `IHttpEasyAgent`
+ `IHttpsEasyAgent`
+ `IHttpEasyClient`
+ `IHttpsEasyClient`
+ `IWebSocketServer`
+ `IWebSocketAgent`
+ `ITcpServer<TRequestBodyType>`
+ `ITcpClient<TRequestBodyType>`
+ `ITcpAgent<TRequestBodyType>`
+ `ISslServer<TRequestBodyType>`
+ `ISslClient<TRequestBodyType>`
+ `ISslAgent<TRequestBodyType>`
+ `AsyncQueue`
`HPSocket.Net` provides a TCP port forwarding component` ITcpPortForwarding`, 10 lines of code can complete TCP port forwarding.
`HPSocket.Net` currently provides 6 Easy components and 2 WebSocket components for easier processing of http / https / ws data packets. The basic http components provided by `HP-Socket` need to implement the data packets themselves. Complete acquisition, Easy component has done these processing, http / https Easy component is bound to the following events, when the event arrives, you can get the complete data packet.
+ `OnEasyChunkData` Complete packet event for http CHUNK message
+ `OnEasyMessageData` Complete packet event for http GET or POST message
+ `OnEasyWebSocketMessageData` Complete packet event for WebSocket message
`WebSocket` can also use the following two components directly
+ `IWebSocketServer` WebSocket Server
+ `IWebSocketAgent` WebSocket Client (Unlike other Agent components, the WebSocket Agent component does not support connecting to different WebSocket Servers, which means that all connections of the `IWebSocketAgent` component can only connect to the same server)
`AsyncQueue` from [qq:842352715](https://gitee.com/zhige777/HPSocket.Net)
## Instructions
1. For the use of most components, please refer to the project in the `demo` directory.
2. In addition to the `Pack` series model, the `Agent` series components provided by `HPSocket.Net` (including the `ITcpPortForwarding` component) support to setting `HTTP` or `Socks5` proxy, which can be set in the manner of`List<IProxy>`. Multiple proxies can be set at the same time, which will be used randomly, and can be mixed with `HTTP` and `Socks5` proxy at the same time. For the usage method, refer to the `demo` of each` Agent` component.
### Easy component event binding example
#### IHttpEasyServer
```cs
// Create HttpEasyServer instance
using(IHttpEasyServer httpServer = new HttpEasyServer())
{
// ... other settings
// Binding OnEasyMessageData event
httpServer.OnEasyMessageData += (sender, id, data) =>
{
// The data parameter is a complete packet each time
// ... Process data
return HttpParseResult.Ok;
};
}
```
#### IHttpEasyAgent
```cs
// Create HttpEasyAgent instance
using(IHttpEasyAgent httpAgent = new HttpEasyAgent())
{
// ... other settings
// Binding OnEasyMessageData event
httpAgent.OnEasyMessageData += (sender, id, data) =>
{
// The data parameter is a complete packet each time
// ... Process data
return HttpParseResult.Ok;
};
}
```
#### IHttpEasyClient
```cs
// Create HttpEasyClient instance
using(IHttpEasyClient httpClient = new HttpEasyClient())
{
// ... other settings
// Binding OnEasyMessageData event
httpClient.OnEasyMessageData += (sender, data) =>
{
// The data parameter is a complete packet each time
// ... Process data
return HttpParseResult.Ok;
};
}
```
### Data receiving adapter component
Full Example: `demo/TcpServer-TestEcho-Adapter`
This series of components are `Data Receive Adapter` extension components of `HPSocket.Net`. Users can process `sticky packets`,`half packets`, etc. that may occur in TCP communication through `Custom Data Receive Adapter`. The `Data Receive Adapter` component looks similar to the `Pack` component of HP-Socket, but it is more flexible and the adaptation is very simple and convenient.
+ `ITcpServer<TRequestBodyType>`/`ITcpClient<TRequestBodyType>`/`ITcpAgent<TRequestBodyType>`
+ `ISslServer<TRequestBodyType>`/`ISslClient<TRequestBodyType>`/`ISslAgent<TRequestBodyType>`
`<TRequestBodyType>` generic type object will be called back in the `OnParseRequestBody` event of the components listed above.
```cs
using (ITcpServer<byte[]> server = new TcpServer<byte[]>
{
// Specify the data receiving adapter
DataReceiveAdapter = new BinaryDataReceiveAdapter(),
})
{
// No need to bind OnReceive event
// The data of the request body event parsed here is the data returned by BinaryDataReceiveAdapter.ParseRequestBody ()
// The type of data is the byte[] specified when ITcpServer <byte[]> is instantiated
server.OnParseRequestBody += (sender, id, data) =>
{
Console.WriteLine($"OnParseRequestBody({id}) -> data length: {data.Length}");
return HandleResult.Ok;
};
}
```
`4` adapters currently supported
#### 1. `FixedHeaderDataReceiveAdapter` Fixed header data receiving adapter
Usage scenario: The header length of the data packet is fixed and the header contains the body length.
Example: The first `4 bytes` identifies the body length (little-endian). `0x00000003` indicates that the length of the body is` 3 bytes`, and `{0x61, 0x62 0x63}` is the body.
```
{ 0x03, 0x00, 0x00, 0x00, 0x61, 0x62, 0x63 }
```
`FixedHeaderDataReceiveAdapter` Designed for this structure
Subclasses inherit `FixedHeaderDataReceiveAdapter`
Call the parent class constructor in its own `constructor`, passing in` header length` and `maximum allowed packet length`
Override the `GetBodySize ()` method, the length of its parameter `header` is the length of the header specified in the constructor, the user needs to parse this parameter and return the actual` body` length
Override the `ParseRequestBody ()` method to deserialize the current `bytes` into a generic type (` <TRequestBodyType> `) object
```cs
using System;
using System.Text;
using HPSocket.Adapter;
using Models;
using Newtonsoft.Json;
namespace TcpServerTestEchoAdapter.DataReceiveAdapter
{
/// <summary>
/// Fixed header data receiving adapter
/// </summary>
public class PacketDataReceiveAdapter : FixedHeaderDataReceiveAdapter<Packet>
{
/// <summary>
/// Call the parent class constructor, specifying the length of the fixed header and the maximum p
没有合适的资源?快使用搜索试试~ 我知道了~
c# HPSocket 例程 包含tcp udp 等 希望帮到有用的人
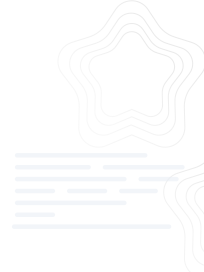
共498个文件
cs:292个
resx:40个
config:40个

需积分: 12 13 下载量 43 浏览量
2022-11-03
18:58:04
上传
评论
收藏 701KB ZIP 举报
温馨提示
HP-Socket 是一套通用的高性能 TCP/UDP/HTTP 通信框架,包含服务端组件、客户端组件和Agent组件,广泛适用于各种不同应用场景的 TCP/UDP/HTTP 通信系统, 此源码为基于HP_Socket框架的socket通讯源码。c# 环境
资源推荐
资源详情
资源评论
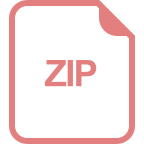
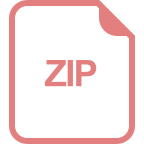
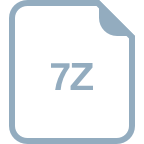
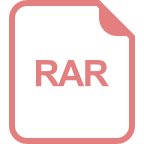
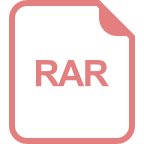
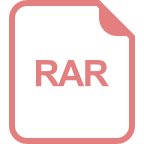
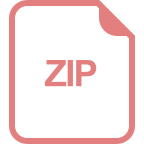
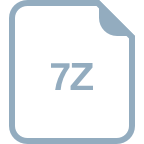
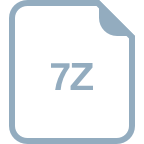
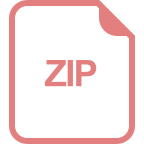
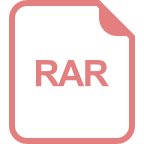
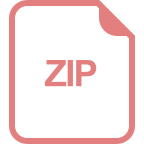
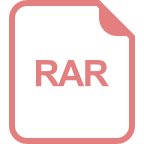
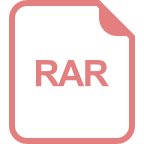
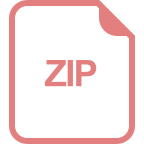
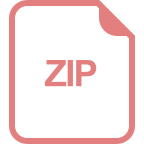
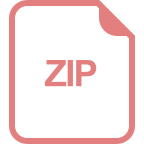
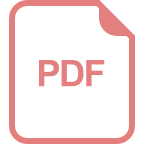
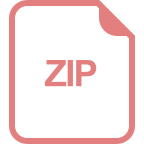
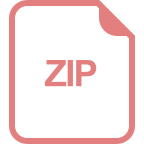
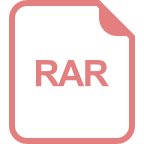
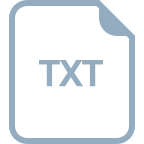
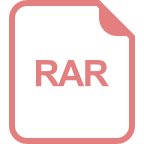
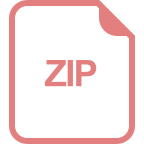
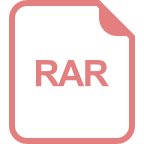
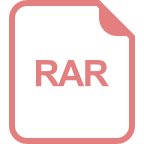
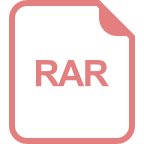
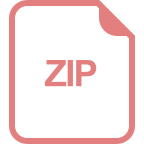
收起资源包目录

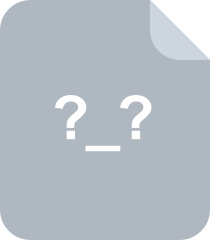
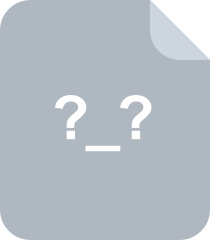
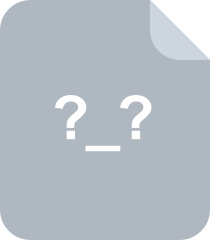
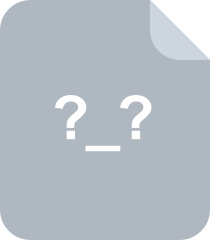
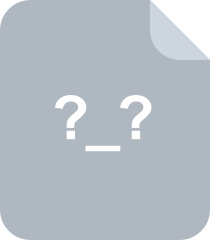
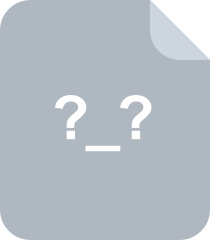
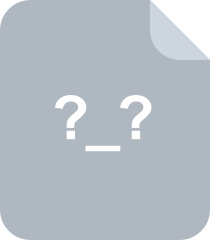
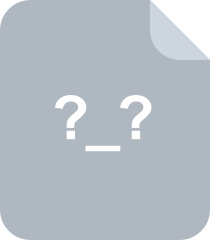
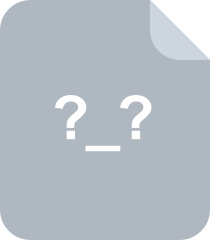
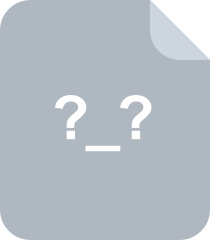
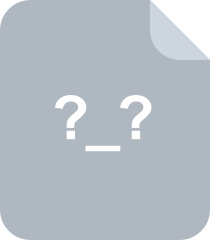
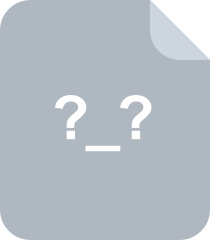
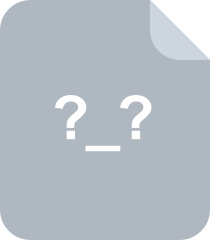
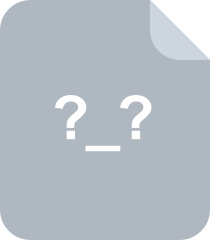
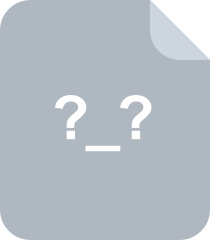
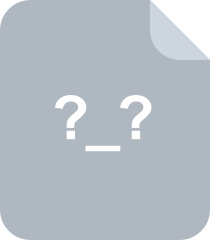
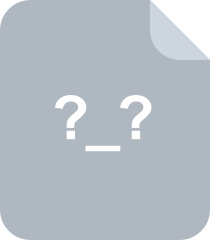
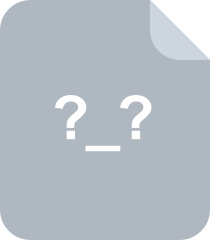
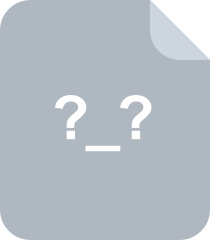
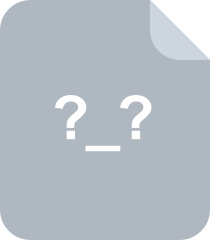
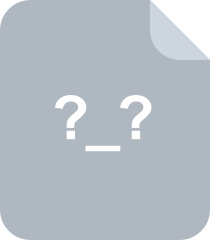
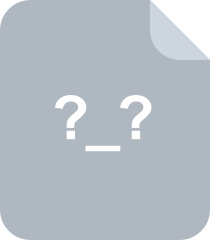
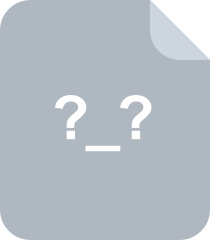
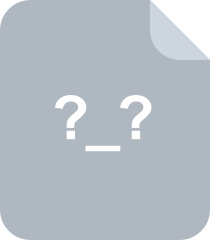
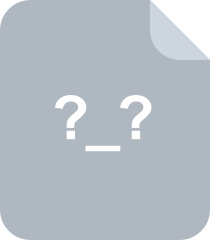
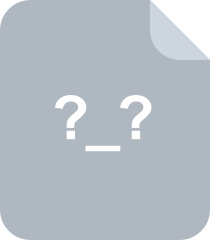
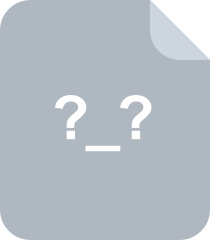
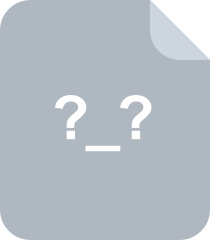
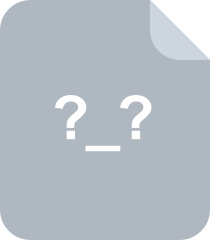
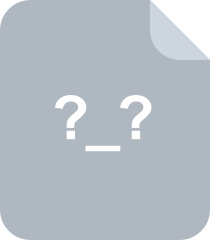
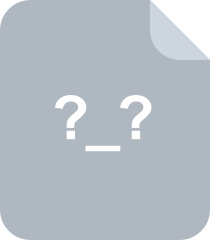
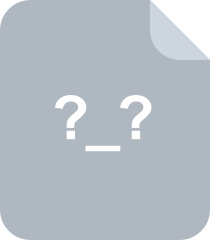
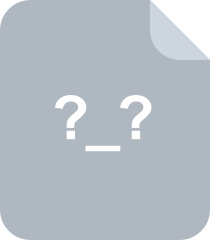
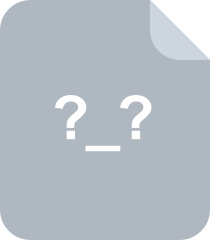
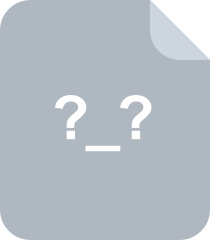
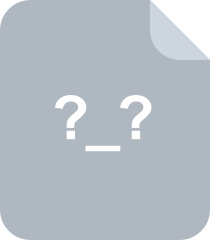
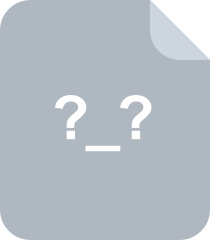
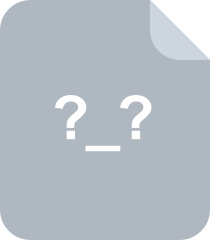
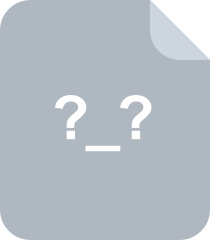
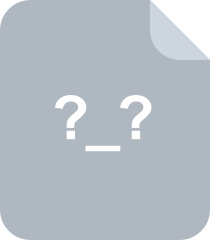
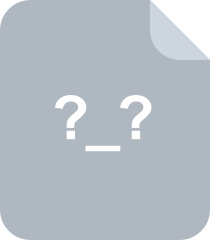
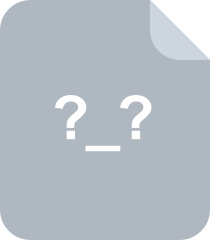
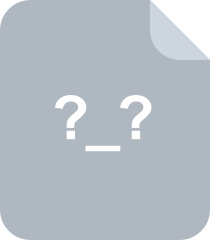
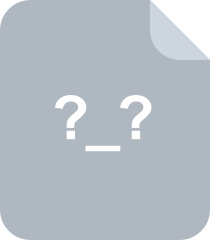
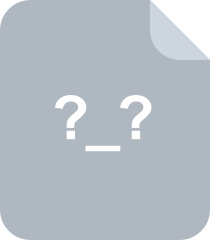
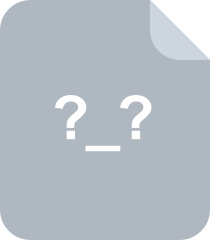
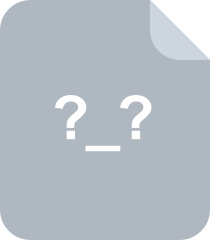
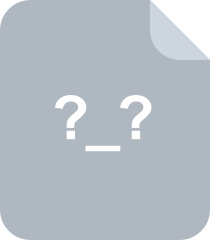
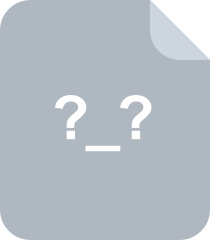
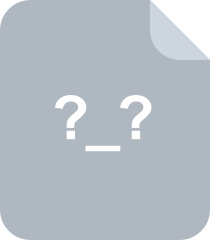
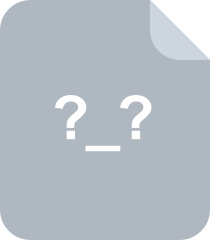
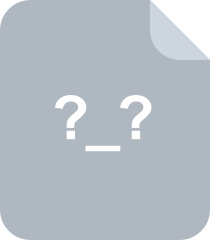
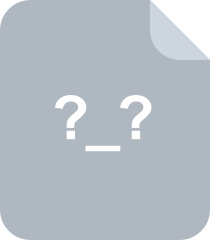
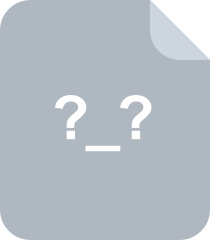
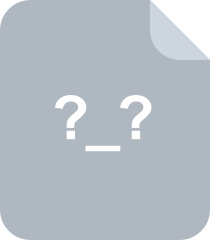
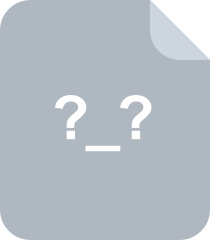
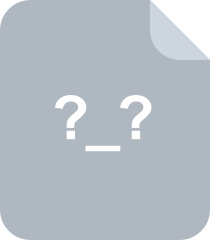
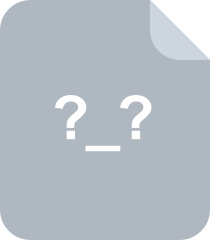
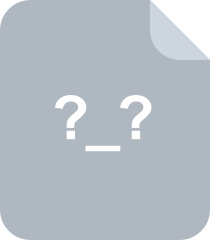
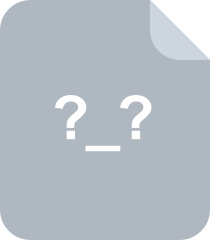
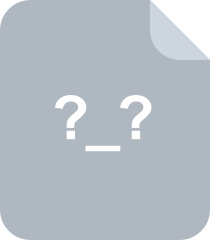
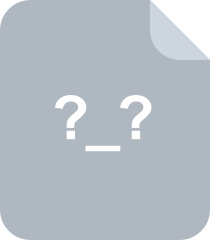
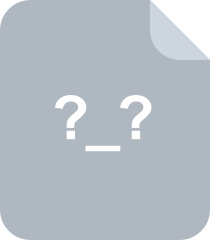
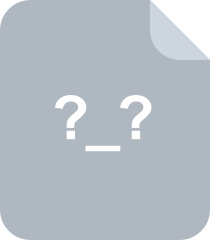
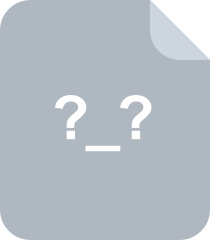
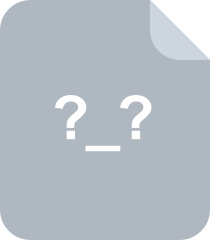
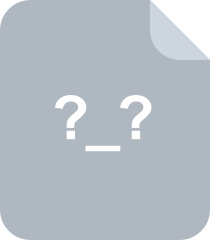
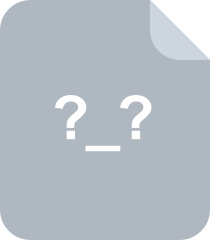
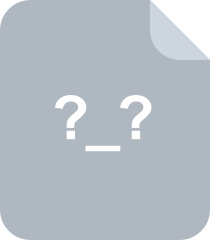
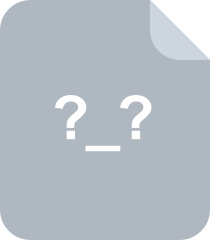
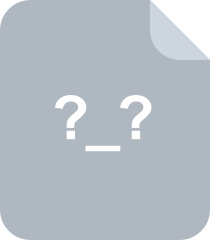
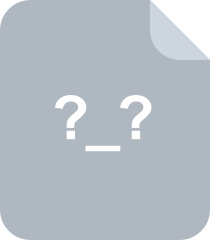
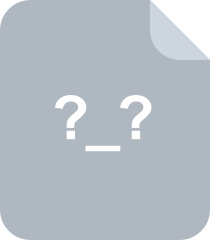
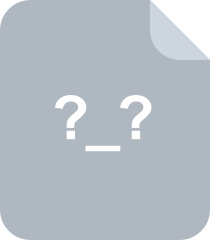
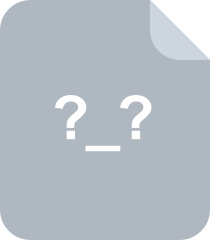
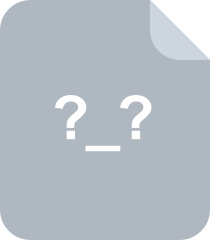
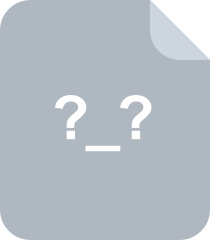
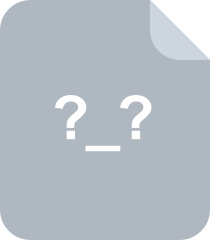
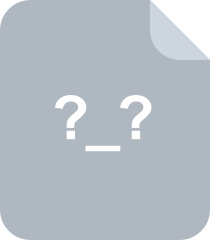
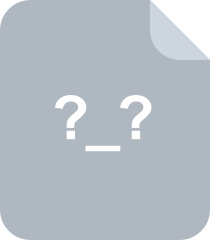
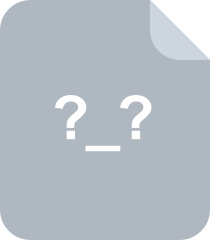
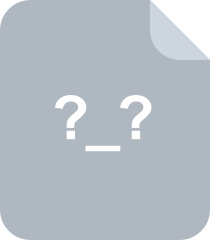
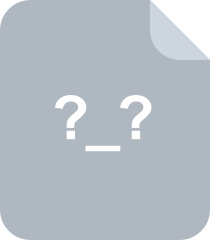
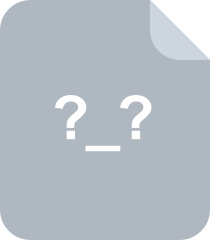
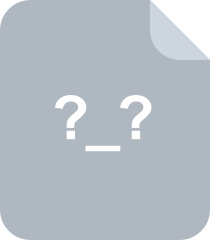
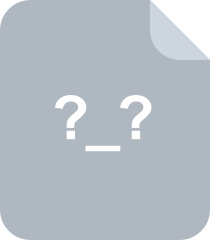
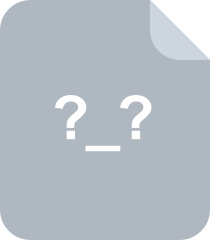
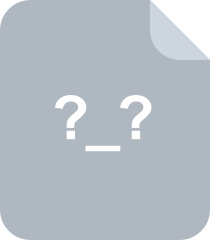
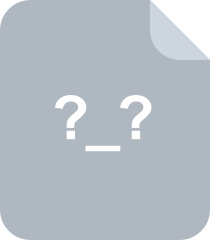
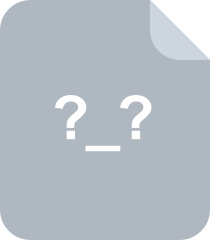
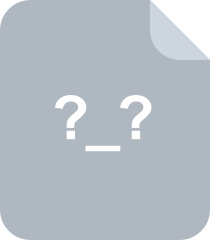
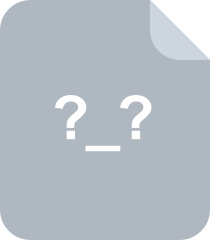
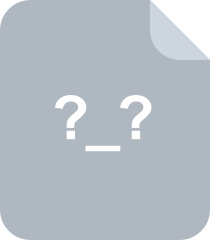
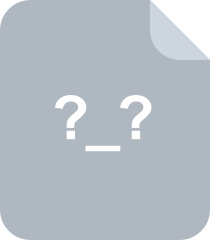
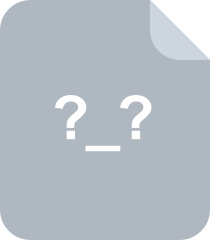
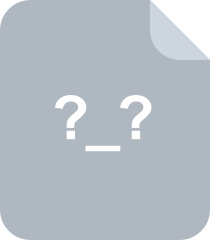
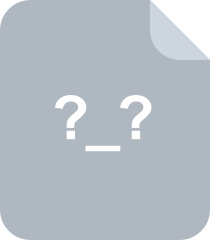
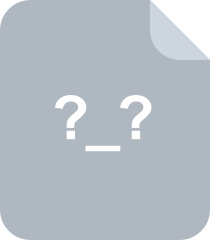
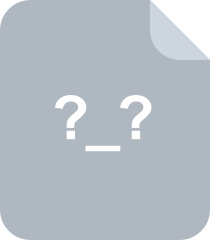
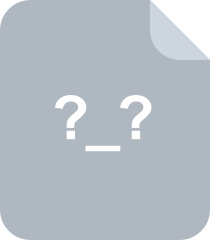
共 498 条
- 1
- 2
- 3
- 4
- 5
资源评论
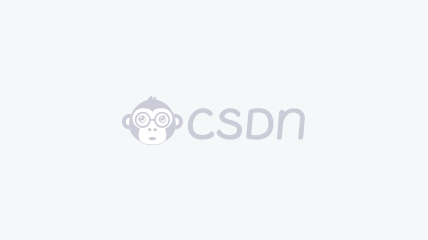

业精于勤818
- 粉丝: 0
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

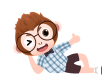
最新资源
- C#微信营销平台源码 微信营销后台管理系统源码数据库 文本存储源码类型 WebForm
- 技术资料分享65C02汇编指令集很好的技术资料.zip
- 课程作业《用51单片机实现的红外人体检测装置》+C语言项目源码+文档说明
- app自动化小白之appium环境安装
- 课程设计-哲学家就餐问题(并发算法问题)-解决策略:资源分级、最多允许四个哲学家同时拿筷子、服务员模式、尝试等待策略
- C#大型公司财务系统源码 企业财务管理系统源码数据库 SQL2008源码类型 WebForm
- MDK文件编译配套工程
- java项目,课程设计-ssm企业人事管理系统ssm.zip
- ton区块链func语言web3智能合约入门课程
- java项目,课程设计-ssm-框架的网上招聘系统的设计与实现
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


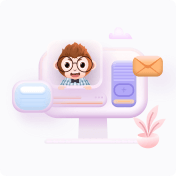
安全验证
文档复制为VIP权益,开通VIP直接复制
