/*
* Copyright 2015-2021 the original author or authors.
*
* All rights reserved. This program and the accompanying materials are
* made available under the terms of the Eclipse Public License v2.0 which
* accompanies this distribution and is available at
*
* https://www.eclipse.org/legal/epl-v20.html
*/
package org.junit.jupiter.api;
import static org.apiguardian.api.API.Status.EXPERIMENTAL;
import static org.apiguardian.api.API.Status.STABLE;
import java.time.Duration;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.function.BooleanSupplier;
import java.util.function.Supplier;
import java.util.stream.Stream;
import org.apiguardian.api.API;
import org.junit.jupiter.api.function.Executable;
import org.junit.jupiter.api.function.ThrowingSupplier;
import org.opentest4j.MultipleFailuresError;
/**
* {@code Assertions} is a collection of utility methods that support asserting
* conditions in tests.
*
* <p>Unless otherwise noted, a <em>failed</em> assertion will throw an
* {@link org.opentest4j.AssertionFailedError} or a subclass thereof.
*
* <h3>Object Equality</h3>
*
* <p>Assertion methods comparing two objects for <em>equality</em>, such as the
* {@code assertEquals(expected, actual)} and {@code assertNotEquals(unexpected, actual)}
* variants, are <em>only</em> intended to test equality for an (un-)expected value
* and an actual value. They are not designed for testing whether a class correctly
* implements {@link Object#equals(Object)}. For example, {@code assertEquals()}
* might immediately return {@code true} when provided the same object for the
* expected and actual values, without calling {@code equals(Object)} at all.
* Tests that aim to verify the {@code equals(Object)} implementation should instead
* be written to explicitly verify the {@link Object#equals(Object)} contract by
* using {@link #assertTrue(boolean) assertTrue()} or {@link #assertFalse(boolean)
* assertFalse()} — for example, {@code assertTrue(expected.equals(actual))},
* {@code assertTrue(actual.equals(expected))}, {@code assertFalse(expected.equals(null))},
* etc.
*
* <h3>Kotlin Support</h3>
*
* <p>Additional <a href="https://kotlinlang.org/">Kotlin</a> assertions can be
* found as <em>top-level functions</em> in the {@link org.junit.jupiter.api}
* package.
*
* <h3>Preemptive Timeouts</h3>
*
* <p>The various {@code assertTimeoutPreemptively()} methods in this class
* execute the provided {@code executable} or {@code supplier} in a different
* thread than that of the calling code. This behavior can lead to undesirable
* side effects if the code that is executed within the {@code executable} or
* {@code supplier} relies on {@link ThreadLocal} storage.
*
* <p>One common example of this is the transactional testing support in the Spring
* Framework. Specifically, Spring's testing support binds transaction state to
* the current thread (via a {@code ThreadLocal}) before a test method is invoked.
* Consequently, if an {@code executable} or {@code supplier} provided to
* {@code assertTimeoutPreemptively()} invokes Spring-managed components that
* participate in transactions, any actions taken by those components will not be
* rolled back with the test-managed transaction. On the contrary, such actions
* will be committed to the persistent store (e.g., relational database) even
* though the test-managed transaction is rolled back.
*
* <p>Similar side effects may be encountered with other frameworks that rely on
* {@code ThreadLocal} storage.
*
* <h3>Extensibility</h3>
*
* <p>Although it is technically possible to extend this class, extension is
* strongly discouraged. The JUnit Team highly recommends that the methods
* defined in this class be used via <em>static imports</em>.
*
* @since 5.0
* @see org.opentest4j.AssertionFailedError
* @see Assumptions
*/
@API(status = STABLE, since = "5.0")
public class Assertions {
/**
* Protected constructor allowing subclassing but not direct instantiation.
*
* @since 5.3
*/
@API(status = STABLE, since = "5.3")
protected Assertions() {
/* no-op */
}
// --- fail ----------------------------------------------------------------
/**
* <em>Fail</em> the test <em>without</em> a failure message.
*
* <p>Although failing <em>with</em> an explicit failure message is recommended,
* this method may be useful when maintaining legacy code.
*
* <p>See Javadoc for {@link #fail(String)} for an explanation of this method's
* generic return type {@code V}.
*/
public static <V> V fail() {
AssertionUtils.fail();
return null; // appeasing the compiler: this line will never be executed.
}
/**
* <em>Fail</em> the test with the given failure {@code message}.
*
* <p>The generic return type {@code V} allows this method to be used
* directly as a single-statement lambda expression, thereby avoiding the
* need to implement a code block with an explicit return value. Since this
* method throws an {@link org.opentest4j.AssertionFailedError} before its
* return statement, this method never actually returns a value to its caller.
* The following example demonstrates how this may be used in practice.
*
* <pre>{@code
* Stream.of().map(entry -> fail("should not be called"));
* }</pre>
*/
public static <V> V fail(String message) {
AssertionUtils.fail(message);
return null; // appeasing the compiler: this line will never be executed.
}
/**
* <em>Fail</em> the test with the given failure {@code message} as well
* as the underlying {@code cause}.
*
* <p>See Javadoc for {@link #fail(String)} for an explanation of this method's
* generic return type {@code V}.
*/
public static <V> V fail(String message, Throwable cause) {
AssertionUtils.fail(message, cause);
return null; // appeasing the compiler: this line will never be executed.
}
/**
* <em>Fail</em> the test with the given underlying {@code cause}.
*
* <p>See Javadoc for {@link #fail(String)} for an explanation of this method's
* generic return type {@code V}.
*/
public static <V> V fail(Throwable cause) {
AssertionUtils.fail(cause);
return null; // appeasing the compiler: this line will never be executed.
}
/**
* <em>Fail</em> the test with the failure message retrieved from the
* given {@code messageSupplier}.
*
* <p>See Javadoc for {@link #fail(String)} for an explanation of this method's
* generic return type {@code V}.
*/
public static <V> V fail(Supplier<String> messageSupplier) {
AssertionUtils.fail(messageSupplier);
return null; // appeasing the compiler: this line will never be executed.
}
// --- assertTrue ----------------------------------------------------------
/**
* <em>Assert</em> that the supplied {@code condition} is {@code true}.
*/
public static void assertTrue(boolean condition) {
AssertTrue.assertTrue(condition);
}
/**
* <em>Assert</em> that the supplied {@code condition} is {@code true}.
* <p>If necessary, the failure message will be retrieved lazily from the supplied {@code messageSupplier}.
*/
public static void assertTrue(boolean condition, Supplier<String> messageSupplier) {
AssertTrue.assertTrue(condition, messageSupplier);
}
/**
* <em>Assert</em> that the boolean condition supplied by {@code booleanSupplier} is {@code true}.
*/
public static void assertTrue(BooleanSupplier booleanSupplier) {
AssertTrue.assertTrue(booleanSupplier);
}
/**
* <em>Assert</em> that the boolean condition supplied by {@code booleanSupplier} is {@code true}.
* <p>Fails with the supplied failure {@code message}.
*/
public static void assertTrue(BooleanSupplier booleanSupplier, String message) {
AssertTrue.assertTrue(booleanSupplier, message);
}
/**
* <em>Assert</em> that the supplied {@code condition} is {@code true}.
* <p>Fails with the supplied failure {@code message}.
*/
public static void assertTrue(b
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
内容概要: ALevin配置环境所需要的基础包——JUnit5.8.2版 适合人群: 对虚拟网络嵌入算法感兴趣的人 能学到什么: 可以帮助大家更快的配置好ALevin运行环境 阅读建议: ALevin作为一个很好的虚拟网络仿真平台,它的开源给我们的学习带来很大方便,但在这之前我们先要将环境配置好,才能更好的使用着个开源工具,由于ALevin要求JUnit 4.5 或更高版本, 因此为了方便大家交流学习, 这里分享一个目前最新版本的junit,如果需要其他版本请到http://www.junit.org下载,下载完成后,请修改“.classpath”中的相应条目以反映相应库的系统范围位置,可以进行调试已检测是否正确安装,如果安装过程中,欢迎与博主一起交流,让我们一起进步
资源详情
资源评论
资源推荐
收起资源包目录

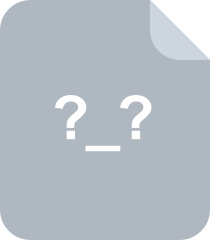
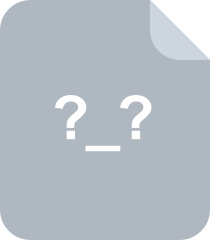
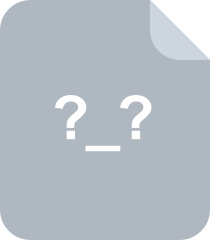
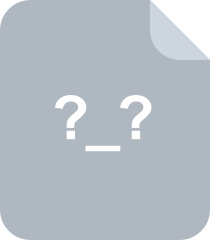
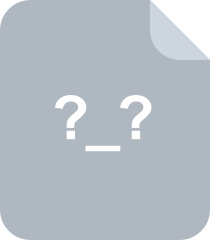
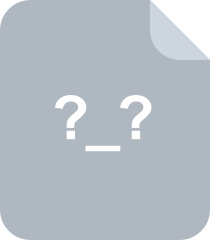
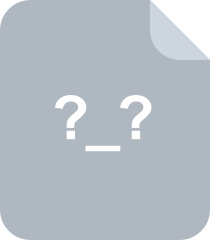
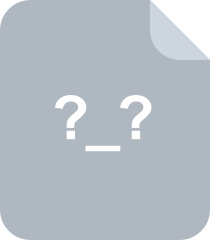
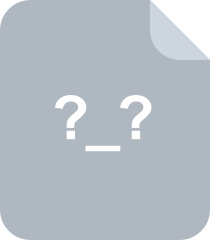
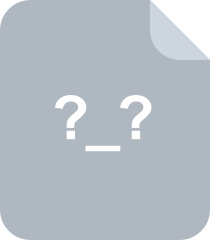
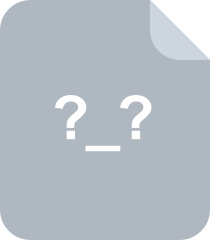
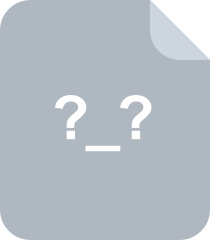
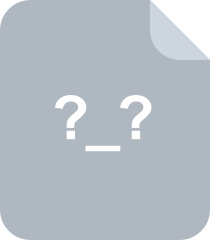
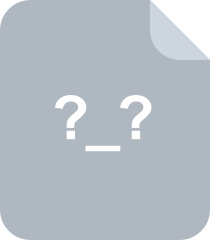
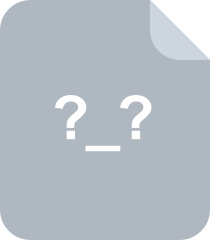
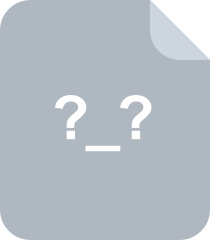
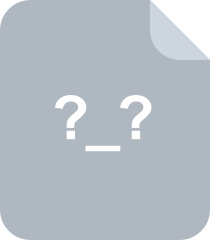
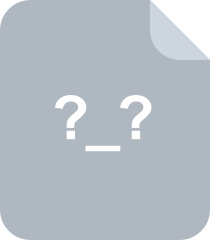
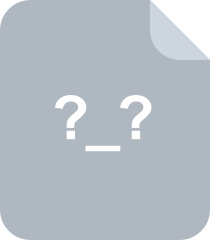
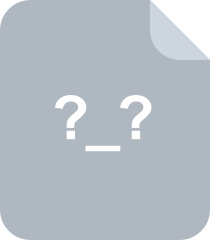
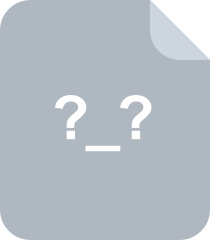
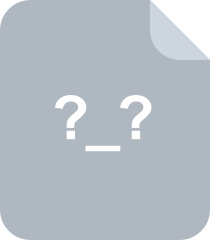
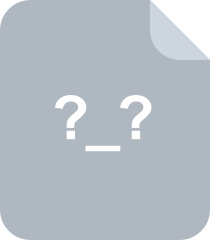
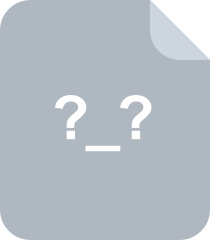
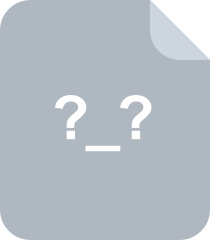
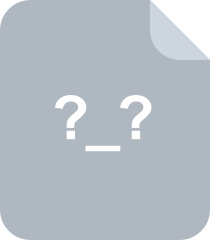
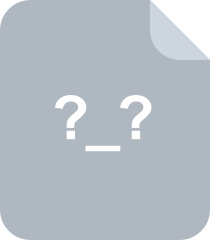
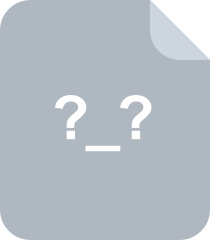
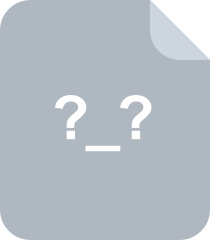
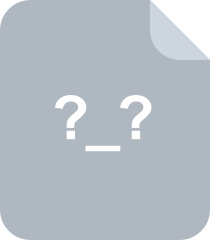
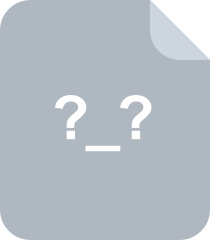
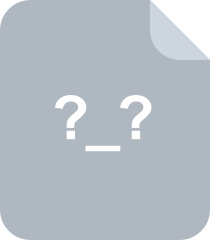
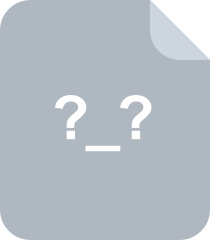
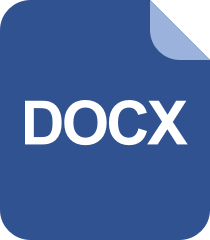
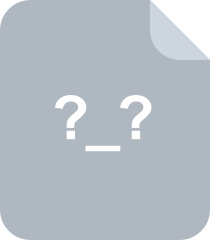
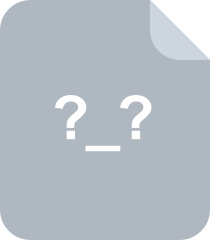
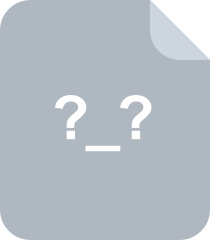
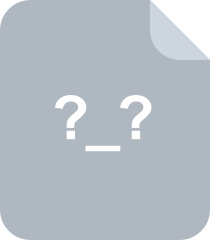
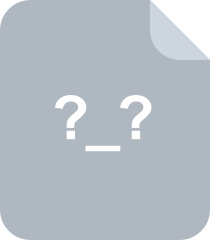
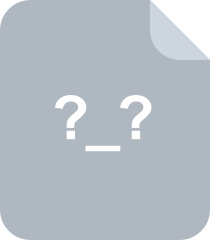
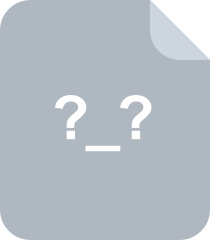
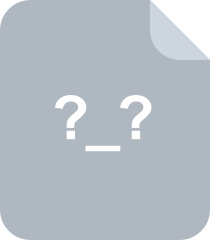
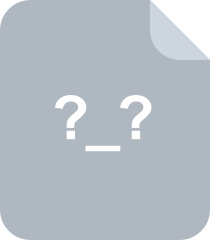
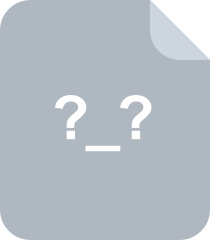
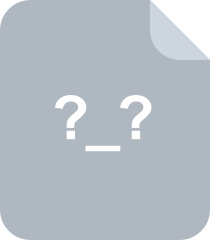
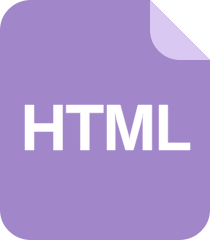
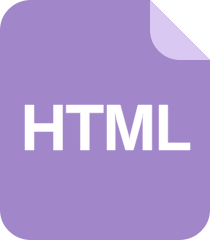
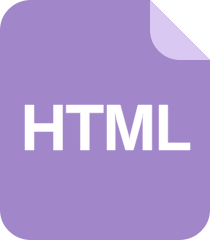
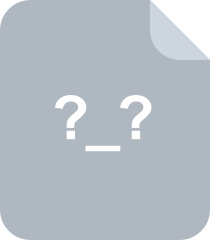
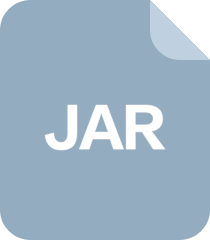
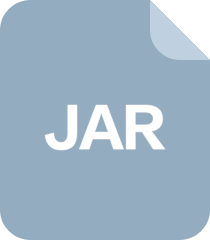
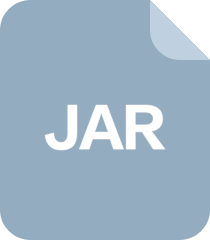
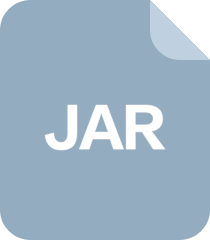
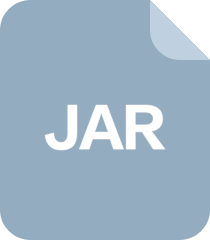
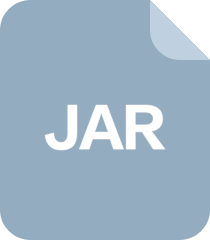
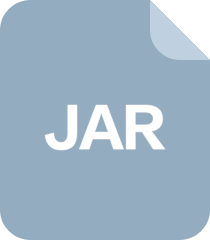
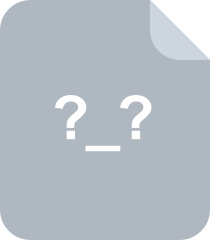
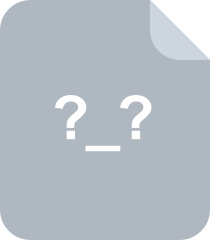
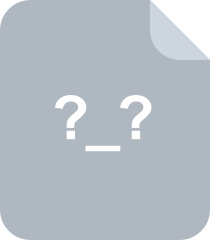
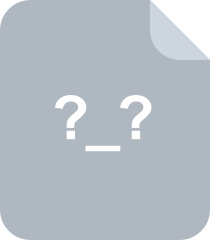
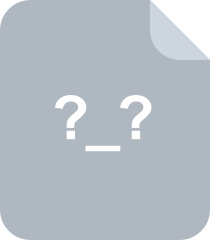
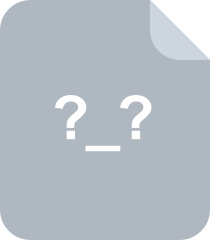
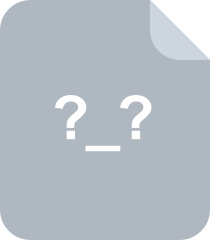
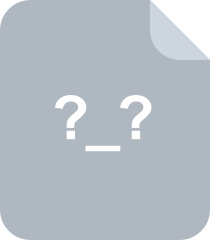
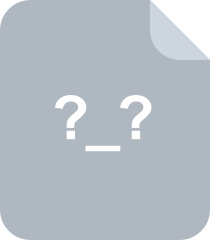
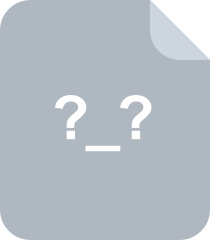
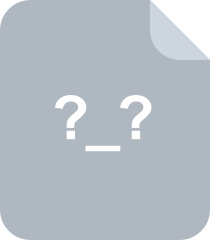
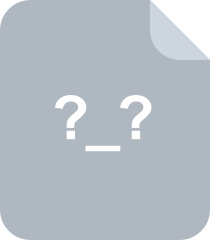
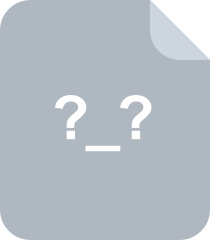
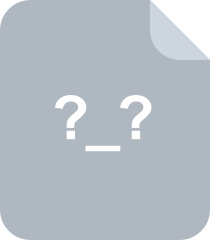
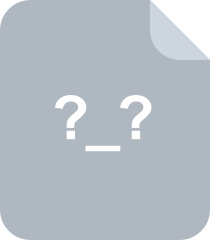
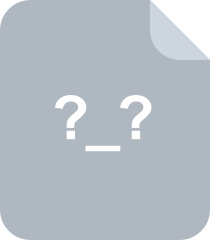
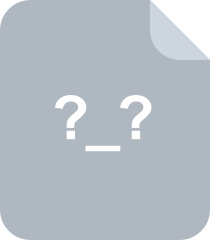
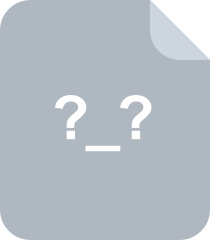
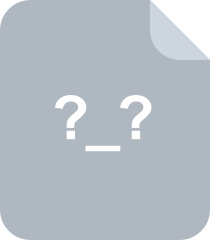
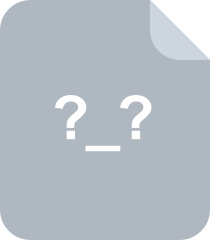
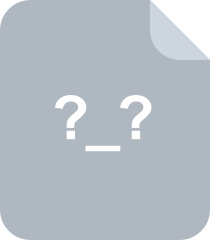
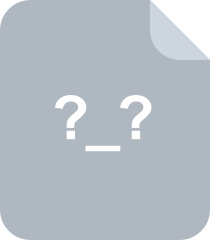
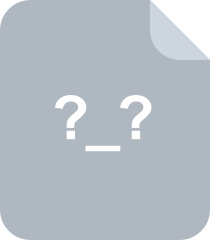
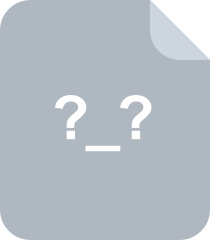
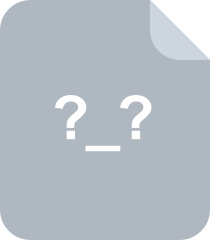
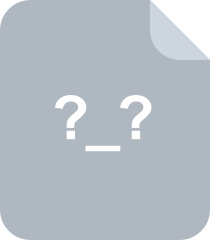
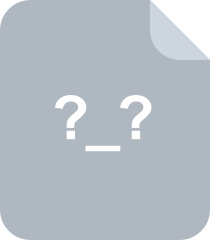
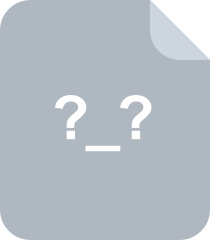
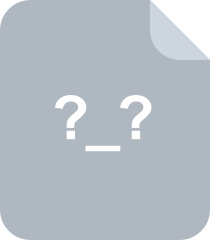
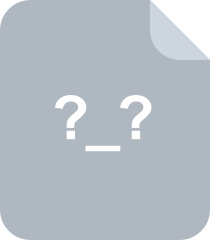
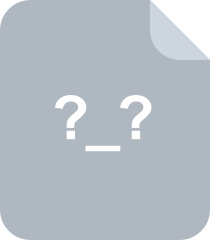
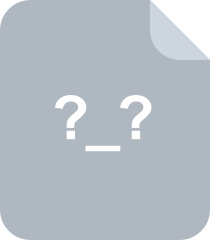
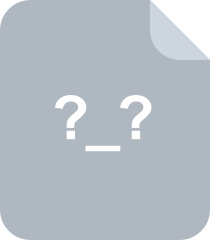
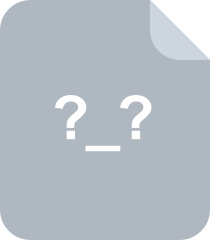
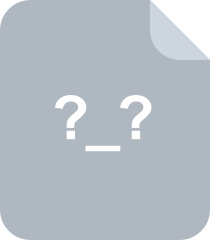
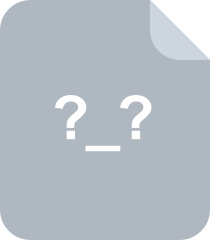
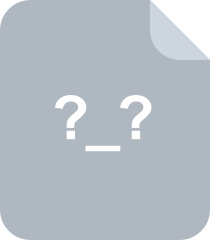
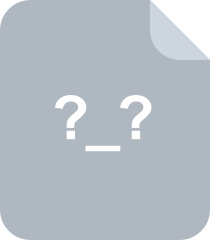
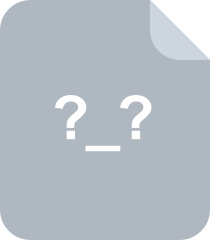
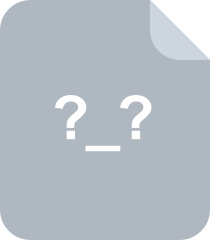
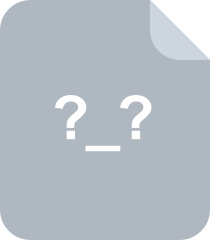
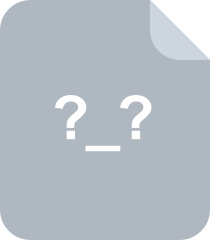
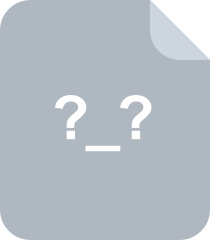
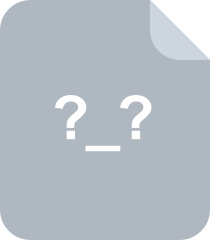
共 1594 条
- 1
- 2
- 3
- 4
- 5
- 6
- 16
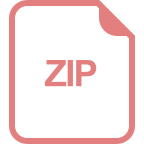
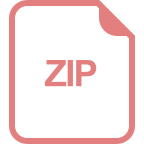
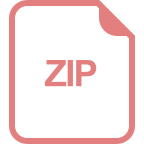
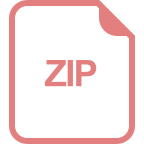
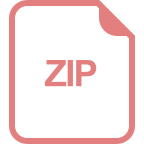
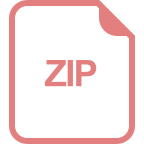
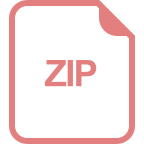
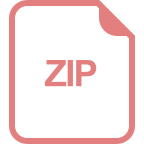
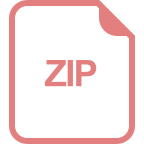
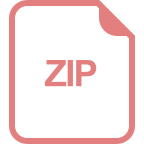
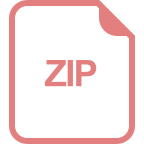
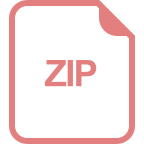
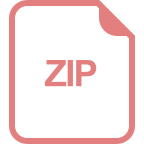
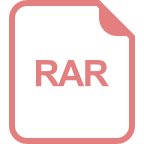
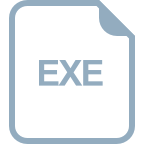
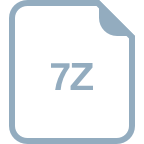
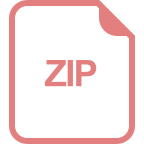
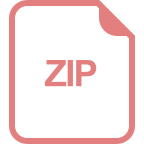
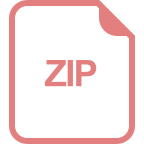
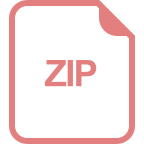
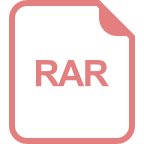

已黑化的小白
- 粉丝: 766
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

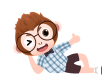
最新资源
- 一款由Java写的射击游戏.zip算法资源
- 一些java的小游戏项目,贪吃蛇啥的.zip用户手册
- 在线实时的斗兽棋游戏,时间赶,粗暴的使用jQuery + websoket 实现实时H5对战游戏 + java.zip课程设计
- HTML5酒店网站模板.zip
- 基于SpringBoot开发的支付系统(包括支付宝支付,微信支付,订单系统).zip
- C基于Qt的学生成绩管理系统.zip毕业设计
- 基于深度卷积神经网络(CNN)模型的图像着色研究与应用系统实现
- Java Web实验报告五:基于JSP的留言本
- Java Web实验报告四:基于AJAX的级联下拉菜单
- springboot洗衣店订单管理系统(代码+数据库+LW)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


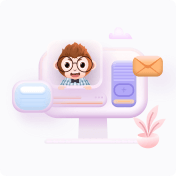
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0