#include "mainwindow.h"
#include "ui_mainwindow.h"
extern QString myNickname;
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
tcpSocket =NULL;
//分配空间指定父对象
tcpSocket = new QTcpSocket(this);
isStart = true; //是没有传文件状态
sendForName = ""; //聊天对象
haveOtherName = false; //有聊天对象
ui->label_3->setText(QString("我是%1").arg(myNickname));
connect(tcpSocket,&QTcpSocket::connected,[=]()
{
//qDebug()<<"yyyy";
ui->msgRead->append("成功和服务器建立好连接");
link(); //发送建立连接函数
});
//读数据连接
connect(tcpSocket,SIGNAL(readyRead()),this,SLOT(readData()));
//定时器连接槽函数发送文件
pathTimer = new QTimer(this);
connect(pathTimer,SIGNAL(timeout()),this,SLOT(TimeSendData()));
}
MainWindow::~MainWindow()
{
delete ui;
}
//连接
void MainWindow::on_pushButton_connect_clicked()
{
QString ip = ui->lineEdit_ip->text();
qint16 port = ui->lineEdit_port->text().toInt();
tcpSocket->connectToHost(QHostAddress(ip),port);
}
//发送文本点击
void MainWindow::on_pushButton_send_clicked()
{
if(haveOtherName == true)
{
QString str = ui->msgWrite->toPlainText();
//组包发送
QString sendStr = "AAAA"+sendForName+"##"+str;
tcpSocket->write(sendStr.toUtf8().data());
ui->msgWrite->clear();
ui->msgWrite->setFocus();
}else {
QMessageBox::information(NULL,"提示信息","先选择需要发送的用户");
}
}
//读数据槽
void MainWindow::readData()
{
QByteArray buf = tcpSocket->readAll();
if(true == isStart)
{
QString strbuf = QString(buf);
qDebug()<<strbuf;
if(strbuf.mid(0,4) == "AAAA") //普通聊天
{
QString mycmd = strbuf.mid(4);
QString otherName = mycmd.section("##",0,0);
QString text = mycmd.section("##",1,1);
QDateTime time = QDateTime::currentDateTime();
QString strtime = time.toString("yyyy-MM-dd hh:mm:ss");
ui->msgRead->setTextColor(QColor(Qt::green)); //变成绿色字体
ui->msgRead->append(QString("[%1%2发布的私聊]::%3").arg(strtime).arg(otherName).arg(text));
}
else if(strbuf.mid(0,4) == "BBBB") //上线
{
QString otherName = strbuf.mid(4);
QString str = otherName+"已上线";
QDateTime time = QDateTime::currentDateTime();
QString strtime = time.toString("yyyy-MM-dd hh:mm:ss");
ui->msgRead->setTextColor(QColor(Qt::gray)); //变成灰色字体
ui->msgRead->append(QString("[%1]%2").arg(strtime).arg(str));
// 添加
itemForm *dataItem = new itemForm(this);
dataItem->showData(otherName);
connect(dataItem,SIGNAL(nameBtnCleck(QString)),this,SLOT(sendForNameSlot(QString)));
QString itemName = dataItem->giveName();
QListWidgetItem *item = new QListWidgetItem(itemName);
QIcon icon(":/res/check1.ico");
item->setIcon(icon);
item->setSizeHint(QSize(139,37));
ui->listWidget->addItem(item);
ui->listWidget->setItemWidget(item,dataItem);
}else if(strbuf.mid(0,4) == "CCCC") //下线
{
QString otherName = strbuf.mid(4);
QString str = otherName+"已下线";
QDateTime time = QDateTime::currentDateTime();
QString strtime = time.toString("yyyy-MM-dd hh:mm:ss");
ui->msgRead->setTextColor(QColor(Qt::gray)); //变成灰色字体
ui->msgRead->append(QString("[%1]%2").arg(strtime).arg(str));
int count = ui->listWidget->count();
for(int i = 0;i<count;i++)
{
QListWidgetItem *item = ui->listWidget->item(i);
if(item->text() == otherName) //是这个
{
ui->listWidget->removeItemWidget(item);
delete item;
if(otherName == sendForName)
{
haveOtherName = false;
}
return; //退出
}
}
}else if(strbuf.mid(0,4) == "DDDD") //传输文件名
{
QString mycmd = strbuf.mid(4);
isStart = false;
//取到名字
recvfileName = mycmd.section("##",0,0);
recvfileSize = mycmd.section("##",1,1).toInt();
//谁发来的
recvOtherPathName = mycmd.section("##",2,2);
recvSize = 0;
recvfile.setFileName(recvfileName);
bool isok = recvfile.open(QIODevice::WriteOnly);
if(false == isok)
{
qDebug()<<"接收出错";
}
}else if (strbuf.mid(0,4) == "EEEE")
{ //刚上线时,收到在线的名字
QString textname = strbuf.mid(4);
//王五##李四##
textname.chop(2); //删除后面两个字符(##)
QStringList strList = textname.split("##"); //以##分开
foreach (QString s, strList) {
//添加
itemForm *dataItem = new itemForm(this);
dataItem->showData(s);
connect(dataItem,SIGNAL(nameBtnCleck(QString)),this,SLOT(sendForNameSlot(QString)));
QString itemName = dataItem->giveName();
QListWidgetItem *item = new QListWidgetItem(itemName);
QIcon icon(":/res/check1.ico");
item->setIcon(icon);
item->setSizeHint(QSize(139,37));
ui->listWidget->addItem(item);
ui->listWidget->setItemWidget(item,dataItem);
}
}else if (strbuf.mid(0,4) == "FFFF")
{
QString mycmd = strbuf.mid(4);
QString otherName = mycmd.section("##",0,0);
QString text = mycmd.section("##",1,1);
QDateTime time = QDateTime::currentDateTime();
QString strtime = time.toString("yyyy-MM-dd hh:mm:ss");
ui->msgRead->setTextColor(QColor(Qt::blue)); //变成蓝色字体
ui->msgRead->append(QString("[%1%2发布的群消息]::%3").arg(strtime).arg(otherName).arg(text));
}
}else { //接收文件
qint64 len = recvfile.write(buf);
recvSize += len;
if(recvSize == recvfileSize)
{
qDebug()<<"接收完成";
recvfile.close();
isStart = true;
//显示一下
QString str = QString("接收%1传来的文件%2成功").arg(recvOtherPathName).arg(recvfileName);
QDateTime time = QDateTime::currentDateTime();
QString strtime = time.toString("yyyy-MM-dd hh:mm:ss");
ui->msgRead->setTextColor(QColor(Qt::red)); //变成灰色字体
ui->msgRead->append(QString("[%1]%2").arg(strtime).arg(str));
}
}
}
//上线函数
void MainWindow::link()
{
QString str = "BBBB"+myNickname;
tcpSocket->write(str.toUtf8().data());
}
//下线函数
void MainWindow::unlink()
{
QString str = "CCCC"+myNickname;
tcpSocket->write(str.toUtf8().data());
}
//发送文件点击
void MainWindow::on_pushButton_sendpath_clicked()
{
if(haveOtherName == true)
{
QString filePath = QFileDialog::getOpenFileName(this,"open","../");
if(false == filePath.isEmpty())
{
sendfileName.clear();
sendfile
没有合适的资源?快使用搜索试试~ 我知道了~
window环境下Qt5 基于C++ 语言编写的局域网聊天系统
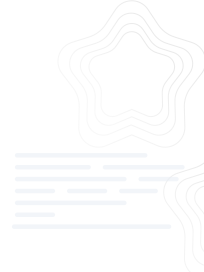
共32个文件
cpp:10个
h:8个
ui:5个

需积分: 17 9 下载量 99 浏览量
2022-07-16
18:17:26
上传
评论 7
收藏 29KB RAR 举报
温馨提示
服务器端功能,主要处理来自局域网下的客户端连接,转发。 客户端功能: 数据库实现注册和登录(与数据库内容进行比对) 私聊、群聊,发送文件(可设置文件大小)清除聊天记录、保存聊天记录、好友上下线提醒; 开发语言c++、连接使用tcp通信; 缺点:界面美化不足。适合初学者;
资源详情
资源评论
资源推荐
收起资源包目录



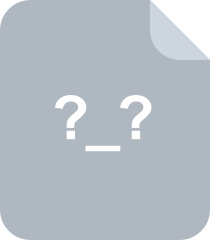
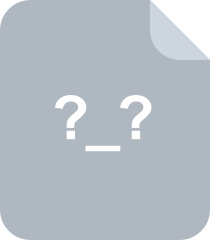
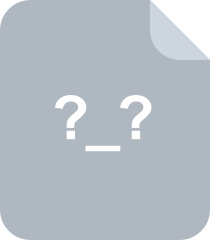

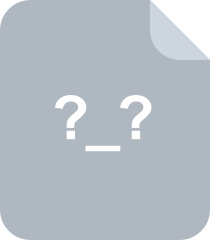
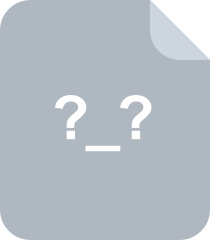
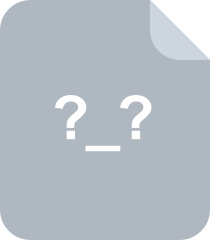
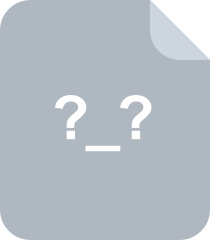
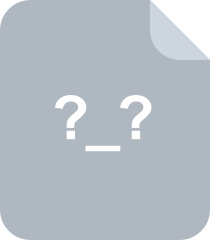
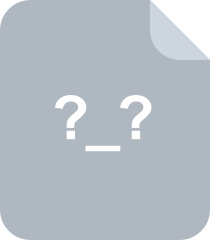
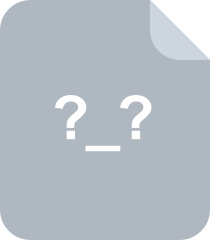
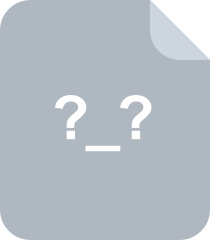
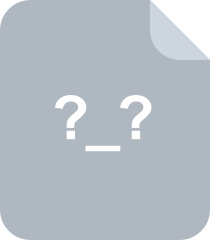
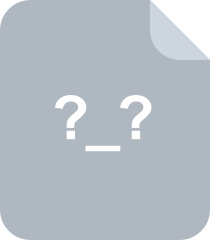
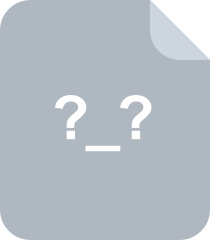
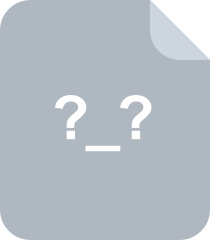
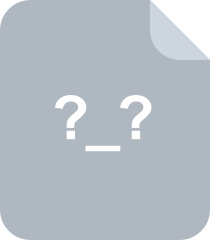
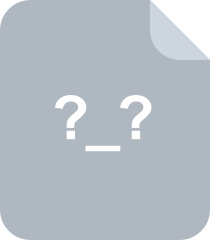
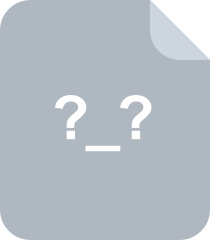
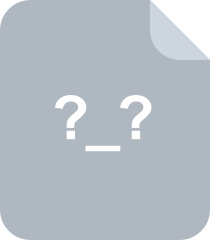
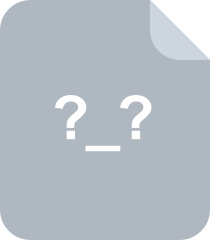
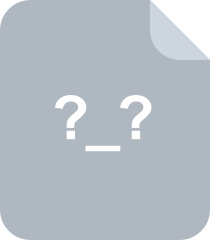
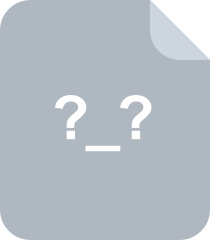
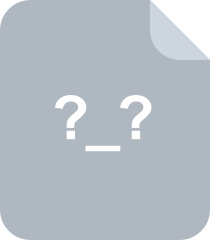

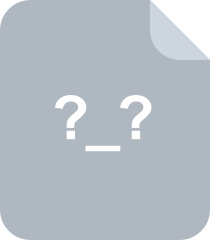
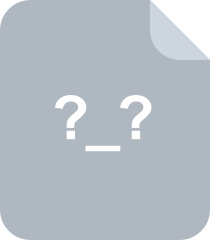
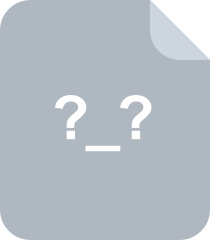
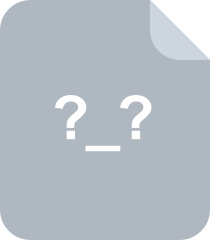
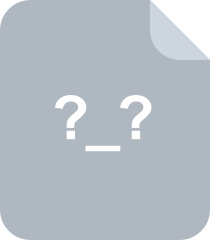
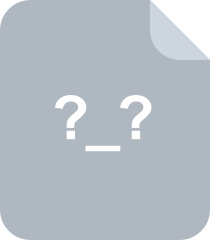
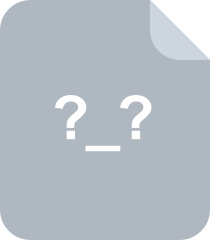
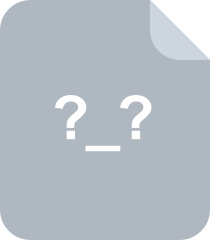
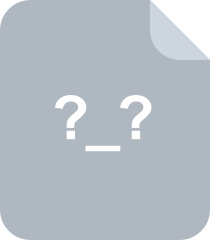
共 32 条
- 1
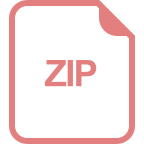
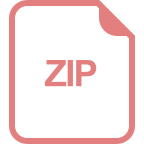
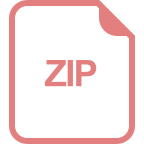
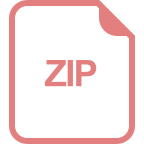
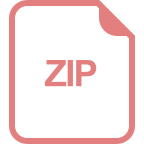
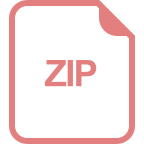
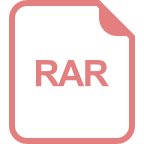
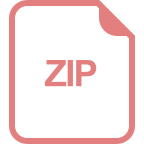
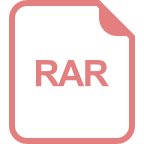
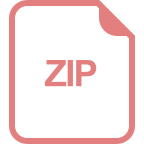
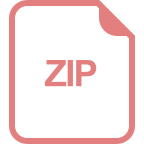
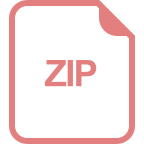
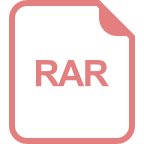
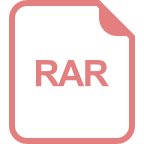
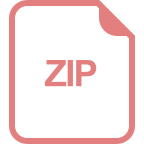

佬酒
- 粉丝: 5272
- 资源: 8
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

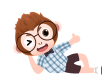
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0