function [startOffset,M] = dmgPacketDetect(x,varargin)
%dmgPacketDetect DMG packet detection for SC PHY using STF field
%
% STARTOFFSET = dmgPacketDetect(X) returns the offset from the
% start of the input waveform to the start of the detected preamble using
% auto-correlation. The dmgPacketDetect() only detect DMG SC packet
% type.
%
% STARTOFFSET is an integer scalar indicating the location of the start
% of a detected packet as the offset from the start of the matrix X. If
% no packet is detected an empty value is returned.
%
% X is the received time-domain signal. It is an Ns-by-1 matrix of real
% or complex samples, where Ns represents the number of time domain
% samples.
%
% STARTOFFSET = dmgPacketDetect(..., OFFSET) specifies the offset to
% begin the auto-correlation process from the start of the matrix X. The
% STARTOFFSET is relative to the input OFFSET when specified. It is an
% integer scalar greater than or equal to zero. When unspecified a value
% of 0 is used.
%
% STARTOFFSET = dmgPacketDetect(...,OFFSET,THRESHOLD) specifies the
% threshold which the decision statistic must meet or exceed to detect a
% packet. THRESHOLD is a real scalar greater than 0 and less than or
% equal to 1. When unspecified a value of 0.03 is used.
%
% [STARTOFFSET,M] = dmgPacketDetect(...) returns the decision
% statistics of the packet detection algorithm of matrix X. When
% THRESHOLD is set to 1, the decision statistics of the complete waveform
% will be returned and STARTOFFSET will be empty.
%
% M is a real vector of size N-by-1, representing the decision statistics
% based on auto-correlation of the input waveform. The length of N
% depends on the starting location of the auto-correlation process till
% the successful detection of a packet.
%
% Example 1:
% % Detect a received 802.11ad SC packet without channel impairments
%
% cfgDMG = wlanDMGConfig('MCS',1); % Create packet configuration
%
% % Generate transmit waveform
% txWaveform = wlanWaveformGenerator([1;0;0;1],cfgDMG);
%
% % Delay the signal by appending zeros at the start
% rxWaveform = [zeros(20,1);txWaveform];
%
% startOffset = dmgPacketDetect(rxWaveform,0,0.99);
% disp(startOffset)
%
% Example 2:
% % Return the decision statistics of the input waveform. The waveform
% % consists of five WLAN packets. The decision statistics shows five
% % peaks. Each peak corresponds to the detection of a single packet.
%
% cfgDMG = wlanDMGConfig('MCS',1); % Create packet configuration
% txWaveform = wlanWaveformGenerator([1;0;0;1],cfgDMG, ...
% 'NumPackets',5,'IdleTime',20e-6);
% [~,M] = dmgPacketDetect(txWaveform,0,1);
% figure;
% plot(M);
% axis([0 length(M) 0 1.2]);
% Copyright 2017-2018 The MathWorks, Inc.
%#codegen
narginchk(1,3);
nargoutchk(0,2);
% Check if M is requested
if nargout==2
M = [];
end
validateattributes(x, {'double'}, {'2d','finite'}, mfilename, 'signal input');
startOffset = [];
if isempty(x)
return;
end
if nargin == 1
threshold = 0.03; % Default value
offset = 0; % Default value
elseif nargin == 2
threshold = 0.03;
validateattributes(varargin{1}, {'double'}, {'integer','scalar','>=',0}, mfilename, 'offset');
offset = varargin{1};
else
validateattributes(varargin{1}, {'double'}, {'integer','scalar','>=',0}, mfilename, 'offset');
validateattributes(varargin{2}, {'double'}, {'real','scalar','>',0,'<=',1}, mfilename, 'threshold');
offset = varargin{1};
threshold = varargin{2};
end
% Validate Offset value
coder.internal.errorIf(offset>size(x,1)-1, 'wlan:shared:InvalidOffsetValue');
symbolLength = 128; % Length of single repetition of Golay sequence for SC PHY
numRepetitions = 17; % Number of repetition of Golay sequence in the preamble of SC PHY
lenLSTF = symbolLength*numRepetitions; % Length of STF field
lenHalfLSTF = lenLSTF/2; % Length of 1/2 STF field
inpLength = (size(x,1)-offset);
% Append zeros to make the input equal to multiple of STF/2
if inpLength<=lenHalfLSTF
numPadSamples = lenLSTF-inpLength;
else
numPadSamples = lenHalfLSTF*ceil(inpLength/lenHalfLSTF)-inpLength;
end
padSamples = zeros(numPadSamples,size(x,2));
% Process the input waveform in blocks of STF length. The processing
% blocks are offset by half the STF length.
numBlocks = (inpLength+numPadSamples)/lenHalfLSTF;
if nargout==2
% Define decision statistics vector
DS = coder.nullcopy(zeros(size(x,1)+length(padSamples)-offset-2*symbolLength+1,1));
if numBlocks > 2
for n=1:numBlocks-2
% Update buffer
buffer = x((n-1)*lenHalfLSTF+(1:lenLSTF)+offset,:);
[startOffset,out] = correlateSamples(buffer,symbolLength,threshold);
DS((n-1)*lenHalfLSTF+1:lenHalfLSTF*n,1) = out(1:lenHalfLSTF);
if ~(isempty(startOffset))
% Packet detected
startOffset = startOffset+(n-1)*lenHalfLSTF;
DS((n-1)*lenHalfLSTF+(1:length(out)),1) = out;
% Resize decision statistics
M = DS(1:(n-1)*lenHalfLSTF+length(out));
return;
end
end
% Process last block of data
blkOffset = lenHalfLSTF*(numBlocks-2);
buffer = [x(blkOffset+1+offset:end,:);padSamples];
[startOffset,out] = correlateSamples(buffer,symbolLength,threshold);
if ~(isempty(startOffset))
startOffset = startOffset+blkOffset; % Packet detected
end
DS(blkOffset+1:end,1)= out;
M = DS(1:end-length(padSamples));
else
buffer = [x(offset+1:end,:);padSamples];
[startOffset,out] = correlateSamples(buffer,symbolLength,threshold);
M = out;
end
else
if numBlocks > 2
for n=1:numBlocks-2
buffer = x((n-1)*lenHalfLSTF+(1:lenLSTF)+offset,:); % Update buffer
startOffset = correlateSamples(buffer,symbolLength,threshold);
if ~(isempty(startOffset))
startOffset = startOffset+(n-1)*lenHalfLSTF; % Packet detected
return;
end
end
% Process last block of data
blkOffset = lenHalfLSTF*(numBlocks-2);
buffer = [x(blkOffset+1+offset:end,:);padSamples];
startOffset = correlateSamples(buffer,symbolLength,threshold);
if ~(isempty(startOffset))
startOffset = startOffset+blkOffset; % Packet detected
end
else
buffer = [x(offset+1:end,:);padSamples];
startOffset = correlateSamples(buffer,symbolLength,threshold);
end
end
end
function [packetStart,Mn] = correlateSamples(rxSig,symbolLength,threshold)
% Estimate the start offset of the preamble of the receive WLAN packet,
% using auto-correlation method [1].
% [1] OFDM Wireless LANs: A Theoretical and Practical Guide 1st Edition
% by Juha Heiskala (Author),John Terry Ph.D. ISBN-13:978-0672321573
pNoise = eps; % Adding noise to avoid the divide by zero
weights = ones(symbolLength,1);
packetStart = []; % Initialize output
% Shift data for correlation
rxDelayed = rxSig(symbolLength+1:end,:); % Delayed samples
rx = rxSig(1:end-symbolLength,:); % Actual samples
% Sum output on multiple receive antennas
C = sum(filter(weights,1,(conj(rxDelayed).*rx)),2);
CS = C(symbolLength:end)./symbolLength;
% Sum output on multiple receive antennas
P = sum(filter(weights,1,abs(rxDelayed).^2./symbolLength),2);
PS = P(symbolLength:end)+pNoise;
Mn = abs(CS).^2./PS.^2;
N = Mn > threshold;
% The scalingFactor is used to scale the length of continuous region of
% correlation samples above the threshold.
scalingFactor = 5;
if (sum(N) >= symbolLength*scalingFactor)
% Convert mxArray to a known data type. Needed for codeGen
startOnes = patternMatch([N(1)~=1 N.'],[0 1]);
endOnes = patternMatch([N.' N(end)~=1],[1 0]);
lengthOnes = endOnes - startOnes + 1;
i
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
一、前言 此示例展示了如何在 Simulink中对 IEEE 802.11ad单载波链路进行建模,其中包括具有射频波束成形功能的相控阵天线。 二、介绍 此模型模拟具有射频波束成形的 802.11ad 单载波 (SC)链路。多个数据包通过自由空间传输,然后射频波束成形、解调和 PLCP 服务数据单元 (PSDU) 被恢复。将PSDU与传输的PSDU进行比较以确定数据包错误率。接收器执行数据包检测、定时同步、载波频率偏移校正和独特的基于字的相位跟踪。 三、系统架构 该系统包括: • 生成随机 PSDU 和 802.11ad SC 数据包的基带发射器。 • 一个自由空间频道。 • 支持多达 16 个元件的接收天线阵列。该模块允许控制阵列几何形状、阵列中的元件数量、工作频率和接收器方向。 • 用于处理射频信号的 16 通道射频接收器模块。该接收器模块包括低噪声放大器、移相器、Wilkinson 16:1 合路器和一个下变频器。该模块允许控制用于计算相应相移的波束成形方向。 • 一种基带接收器,通过执行数据包检测、时间和频率同步、信道估计、PSDU解调和解码来恢复传输的PSDU。 系统诊断包括均
资源推荐
资源详情
资源评论
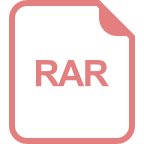
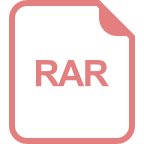
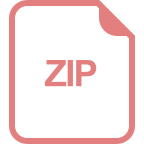
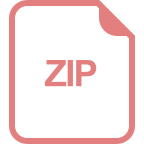
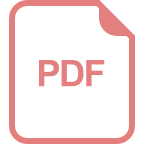
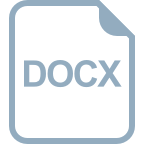
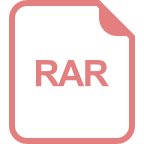
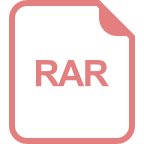
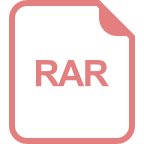
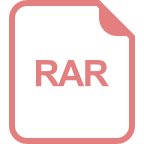
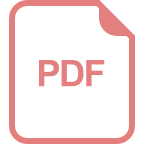
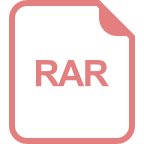
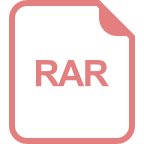
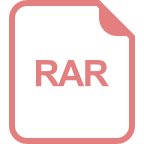
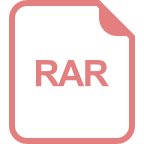
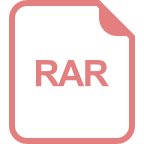
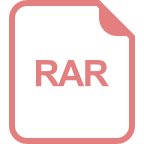
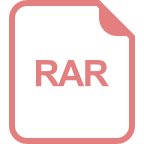
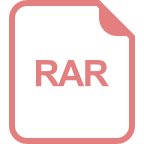
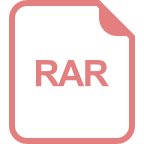
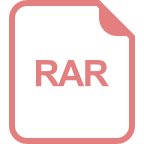
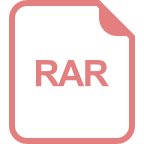
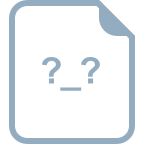
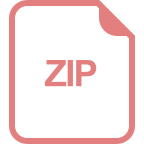
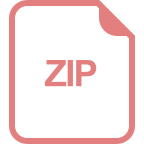
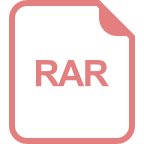
收起资源包目录


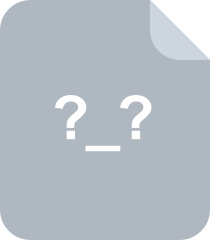
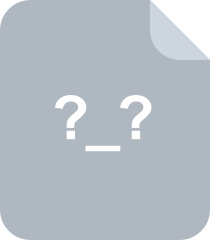
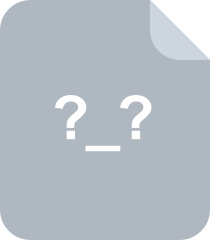
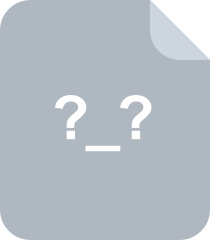
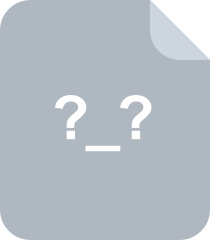
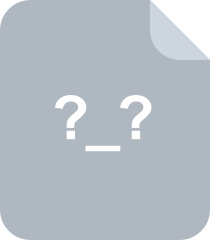
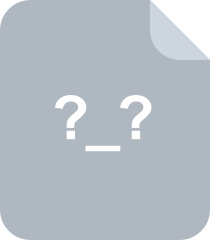
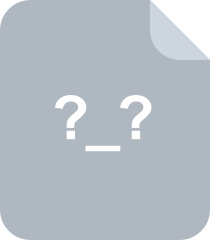
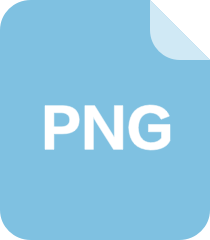
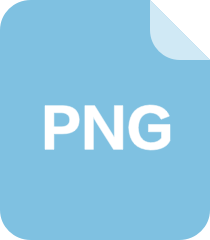
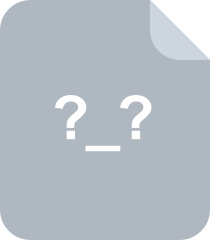
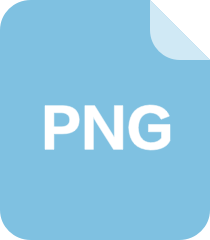
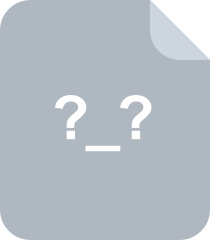
共 13 条
- 1
资源评论
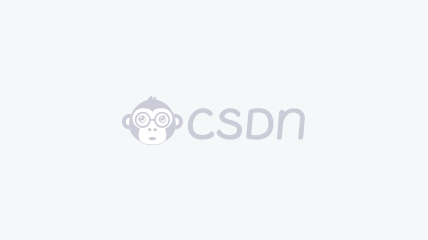
- coee_7723402024-03-21这个资源值得下载,资源内容详细全面,与描述一致,受益匪浅。
- strong1052024-04-07资源是宝藏资源,实用也是真的实用,感谢大佬分享~

珞瑜·
- 粉丝: 12w+
- 资源: 500

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

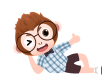
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


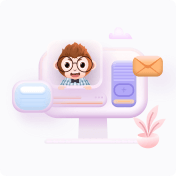
安全验证
文档复制为VIP权益,开通VIP直接复制
