
[OpenGL Mathematics](http://glm.g-truc.net/) (*GLM*) is a header only C++ mathematics library for graphics software based on the [OpenGL Shading Language (GLSL) specifications](https://www.opengl.org/registry/doc/GLSLangSpec.4.50.diff.pdf).
*GLM* provides classes and functions designed and implemented with the same naming conventions and functionality than *GLSL* so that anyone who knows *GLSL*, can use *GLM* as well in C++.
This project isn't limited to *GLSL* features. An extension system, based on the *GLSL* extension conventions, provides extended capabilities: matrix transformations, quaternions, data packing, random numbers, noise, etc...
This library works perfectly with *[OpenGL](https://www.opengl.org)* but it also ensures interoperability with other third party libraries and SDK. It is a good candidate for software rendering (raytracing / rasterisation), image processing, physic simulations and any development context that requires a simple and convenient mathematics library.
*GLM* is written in C++98 but can take advantage of C++11 when supported by the compiler. It is a platform independent library with no dependence and it officially supports the following compilers:
- [Apple Clang 6.0](https://developer.apple.com/library/mac/documentation/CompilerTools/Conceptual/LLVMCompilerOverview/index.html) and higher
- [GCC](http://gcc.gnu.org/) 4.7 and higher
- [Intel C++ Composer](https://software.intel.com/en-us/intel-compilers) XE 2013 and higher
- [LLVM](http://llvm.org/) 3.4 and higher
- [Visual C++](http://www.visualstudio.com/) 2013 and higher
- [CUDA](https://developer.nvidia.com/about-cuda) 7.0 and higher (experimental)
- Any C++11 compiler
For more information about *GLM*, please have a look at the [manual](manual.md) and the [API reference documentation](http://glm.g-truc.net/0.9.8/api/index.html).
The source code and the documentation are licensed under both the [Happy Bunny License (Modified MIT) or the MIT License](manual.md#section0).
Thanks for contributing to the project by [submitting issues](https://github.com/g-truc/glm/issues) for bug reports and feature requests. Any feedback is welcome at [glm@g-truc.net](mailto://glm@g-truc.net).
```cpp
#include <glm/vec3.hpp> // glm::vec3
#include <glm/vec4.hpp> // glm::vec4
#include <glm/mat4x4.hpp> // glm::mat4
#include <glm/ext/matrix_transform.hpp> // glm::translate, glm::rotate, glm::scale
#include <glm/ext/matrix_clip_space.hpp> // glm::perspective
#include <glm/ext/constants.hpp> // glm::pi
glm::mat4 camera(float Translate, glm::vec2 const& Rotate)
{
glm::mat4 Projection = glm::perspective(glm::pi<float>() * 0.25f, 4.0f / 3.0f, 0.1f, 100.f);
glm::mat4 View = glm::translate(glm::mat4(1.0f), glm::vec3(0.0f, 0.0f, -Translate));
View = glm::rotate(View, Rotate.y, glm::vec3(-1.0f, 0.0f, 0.0f));
View = glm::rotate(View, Rotate.x, glm::vec3(0.0f, 1.0f, 0.0f));
glm::mat4 Model = glm::scale(glm::mat4(1.0f), glm::vec3(0.5f));
return Projection * View * Model;
}
```
## [Lastest release](https://github.com/g-truc/glm/releases/latest)
## Project Health
| Service | System | Compiler | Status |
| ------- | ------ | -------- | ------ |
| [Travis CI](https://travis-ci.org/g-truc/glm)| MacOSX, Linux 64 bits | Clang 3.6, Clang 5.0, GCC 4.9, GCC 7.3 | [](https://travis-ci.org/g-truc/glm)
| [AppVeyor](https://ci.appveyor.com/project/Groovounet/glm)| Windows 32 and 64 | Visual Studio 2013, Visual Studio 2015, Visual Studio 2017 | [](https://ci.appveyor.com/project/Groovounet/glm)
## Release notes
### [GLM 0.9.9.6](https://github.com/g-truc/glm/tree/master)
#### Improvements:
- Added Visual C++ 2019 detection
- Added Visual C++ 2017 15.8 and 15.9 detection
### [GLM 0.9.9.5](https://github.com/g-truc/glm/releases/tag/0.9.9.5) - 2019-04-01
#### Fixes:
- Fixed build errors when defining GLM_ENABLE_EXPERIMENTAL #884 #883
- Fixed 'if constexpr' warning #887
- Fixed missing declarations for frexp and ldexp #886
### [GLM 0.9.9.4](https://github.com/g-truc/glm/releases/tag/0.9.9.4) - 2019-03-19
#### Features:
- Added mix implementation for matrices in EXT_matrix_common #842
- Added BUILD_SHARED_LIBS and BUILD_STATIC_LIBS build options #871
#### Improvements:
- Added GLM_FORCE_INTRINSICS to enable SIMD instruction code path. By default, it's disabled allowing constexpr support by default. #865
- Optimized inverseTransform #867
#### Fixes:
- Fixed in mat4x3 conversion #829
- Fixed constexpr issue on GCC #832 #865
- Fixed mix implementation to improve GLSL conformance #866
- Fixed int8 being defined as unsigned char with some compiler #839
- Fixed vec1 include #856
- Ignore .vscode #848
### [GLM 0.9.9.3](https://github.com/g-truc/glm/releases/tag/0.9.9.3) - 2018-10-31
#### Features:
- Added equal and notEqual overload with max ULPs parameters for scalar numbers #121
- Added GLM_FORCE_SILENT_WARNINGS to silent GLM warnings when using language extensions but using W4 or Wpedantic warnings #814 #775
- Added adjugate functions to GTX_matrix_operation #151
- Added GLM_FORCE_ALIGNED_GENTYPES to enable aligned types and SIMD instruction are not enabled. This disable constexpr #816
#### Improvements:
- Added constant time ULP distance between float #121
- Added GLM_FORCE_SILENT_WARNINGS to suppress GLM warnings #822
#### Fixes:
- Fixed simplex noise build with double #734
- Fixed bitfieldInsert according to GLSL spec #818
- Fixed refract for negative 'k' #808
### [GLM 0.9.9.2](https://github.com/g-truc/glm/releases/tag/0.9.9.2) - 2018-09-14
#### Fixes:
- Fixed GLM_FORCE_CXX** section in the manual
- Fixed default initialization with vector and quaternion types using GLM_FORCE_CTOR_INIT #812
### [GLM 0.9.9.1](https://github.com/g-truc/glm/releases/tag/0.9.9.1) - 2018-09-03
#### Features:
- Added bitfieldDeinterleave to GTC_bitfield
- Added missing equal and notEqual with epsilon for quaternion types to GTC_quaternion
- Added EXT_matrix_relational: equal and notEqual with epsilon for matrix types
- Added missing aligned matrix types to GTC_type_aligned
- Added C++17 detection
- Added Visual C++ language standard version detection
- Added PDF manual build from markdown
#### Improvements:
- Added a section to the manual for contributing to GLM
- Refactor manual, lists all configuration defines
- Added missing vec1 based constructors
- Redesigned constexpr support which excludes both SIMD and constexpr #783
- Added detection of Visual C++ 2017 toolsets
- Added identity functions #765
- Splitted headers into EXT extensions to improve compilation time #670
- Added separated performance tests
- Clarified refract valid range of the indices of refraction, between -1 and 1 inclusively #806
#### Fixes:
- Fixed SIMD detection on Clang and GCC
- Fixed build problems due to printf and std::clock_t #778
- Fixed int mod
- Anonymous unions require C++ language extensions
- Fixed ortho #790
- Fixed Visual C++ 2013 warnings in vector relational code #782
- Fixed ICC build errors with constexpr #704
- Fixed defaulted operator= and constructors #791
- Fixed invalid conversion from int scalar with vec4 constructor when using SSE instruction
- Fixed infinite loop in random functions when using negative radius values using an assert #739
### [GLM 0.9.9.0](https://github.com/g-truc/glm/releases/tag/0.9.9.0) - 2018-05-22
#### Features:
- Added RGBM encoding in GTC_packing #420
- Added GTX_color_encoding extension
- Added GTX_vec_swizzle, faster compile time swizzling then swizzle operator #558
- Added GTX_exterior_product with a vec2 cross implementation #621
- Added GTX_matrix_factorisation to factor matrices in various forms #654
- Added [GLM_ENABLE_EXPERIMENTAL](manual.md#section7_4) to enable experimental features.
- Added packing functions for integer vectors #639
- Added conan packaging configuration #643 #641
- Added quatLookAt to GT
没有合适的资源?快使用搜索试试~ 我知道了~
glm-master.zip
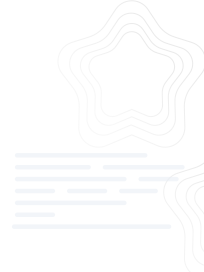
共1512个文件
html:772个
hpp:232个
cpp:163个

需积分: 10 6 下载量 77 浏览量
2019-06-02
18:18:24
上传
评论
收藏 5.82MB ZIP 举报
温馨提示
OpenGL Mathematics (GLM) is a C++ mathematics library based on the OpenGL Shading Language (GLSL) specification. GLM emulates GLSL's approach to vector/matrix operations whenever possible. To use GLM, include glm/glm.hpp. Example from GLM manual: #include <glm/glm.hpp> int foo() { glm::vec4 Position = glm::vec4( glm::vec3( 0.0 ), 1.0 ); glm::mat4 Model = glm::mat4( 1.0 ); Model[3] = glm::vec4( 1.0, 1.0, 0.0, 1.0 ); glm::vec4 Transformed = Model * Position; return 0; }
资源推荐
资源详情
资源评论
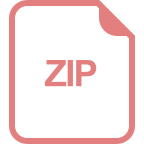
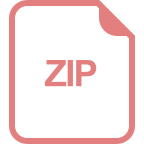
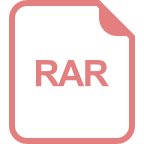
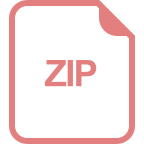
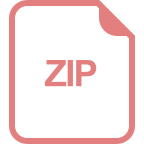
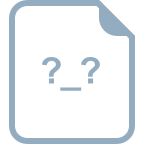
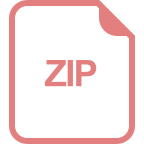
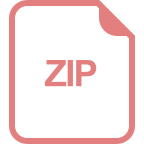
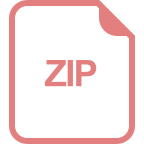
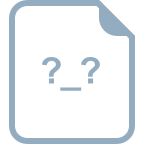
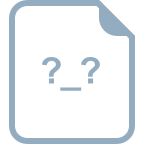
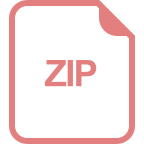
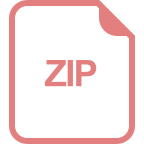
收起资源包目录

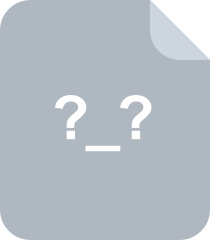
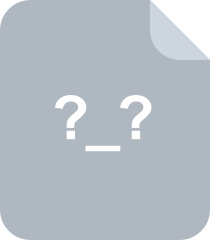
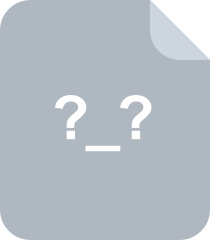
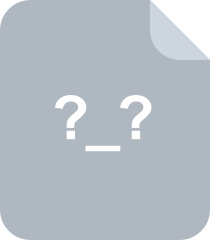
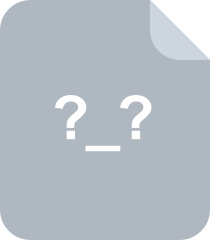
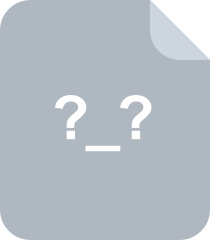
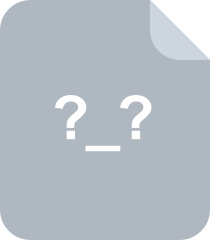
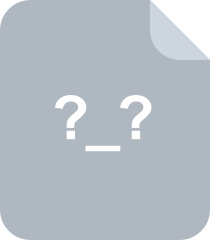
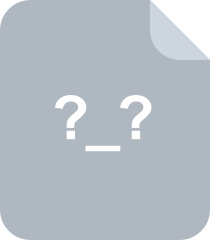
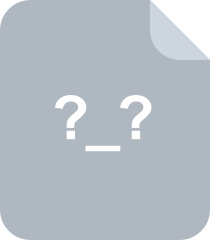
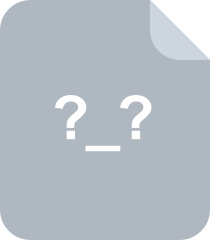
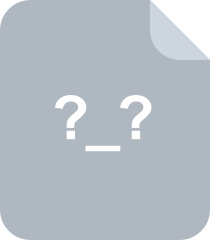
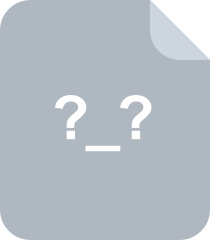
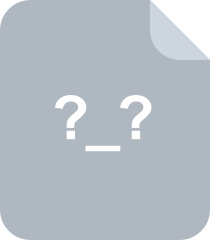
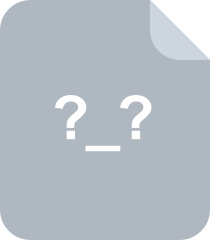
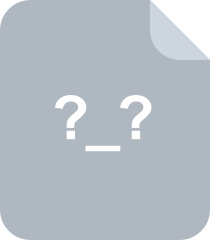
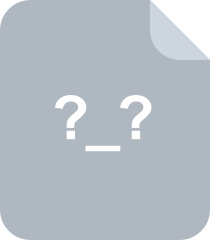
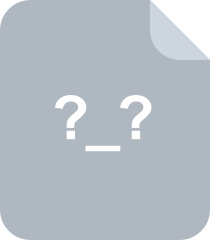
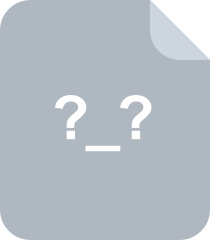
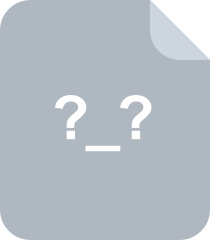
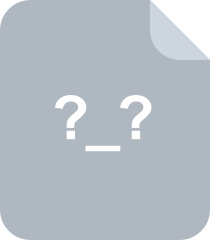
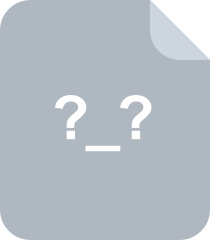
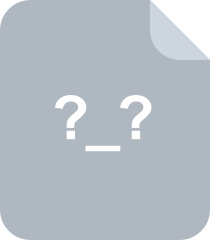
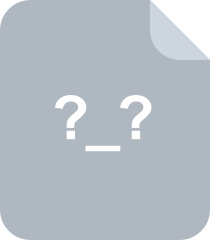
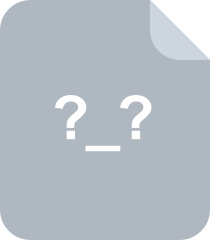
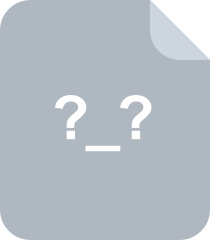
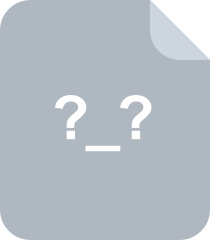
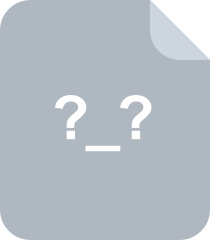
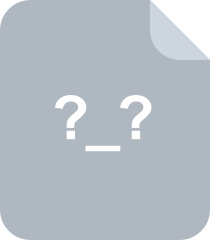
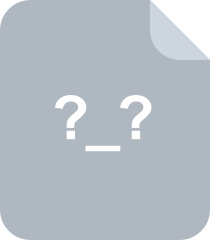
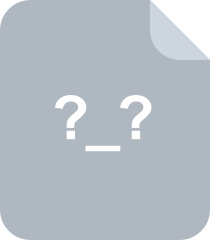
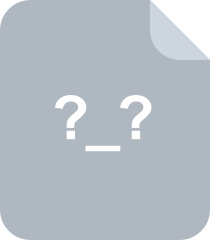
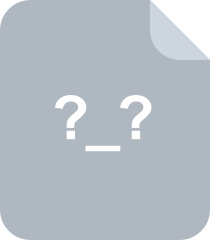
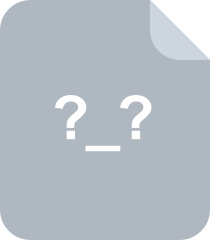
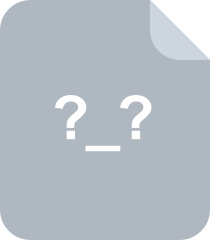
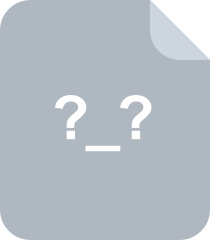
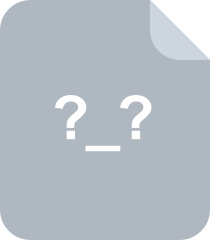
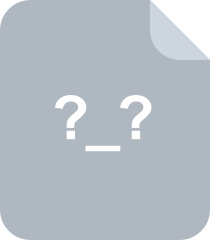
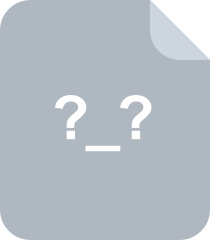
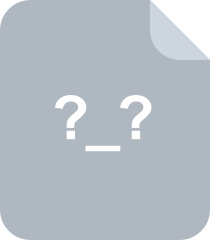
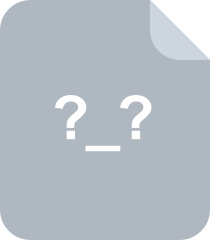
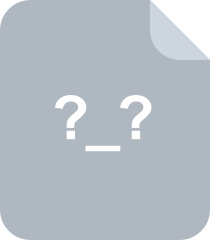
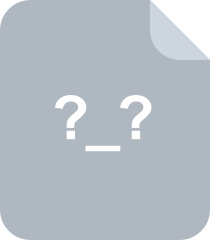
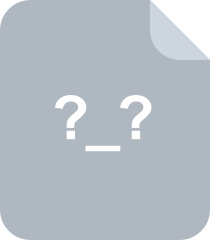
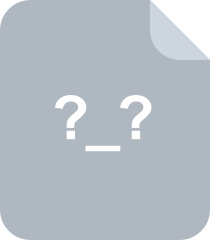
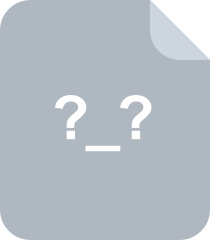
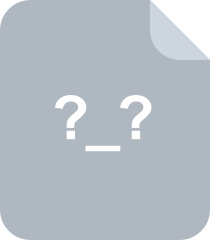
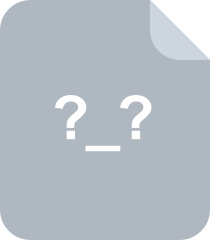
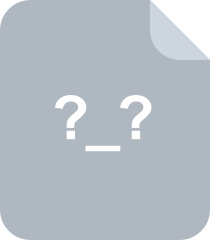
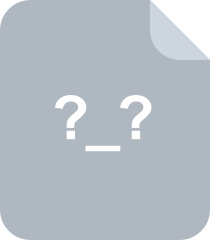
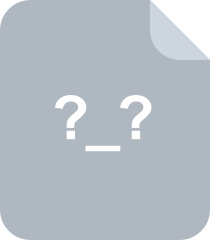
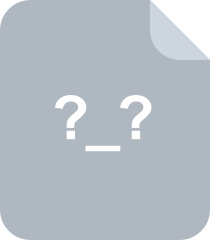
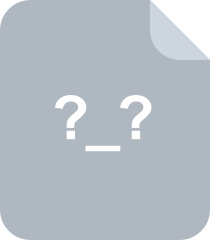
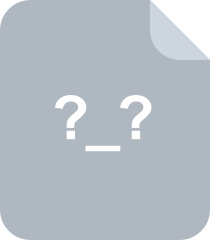
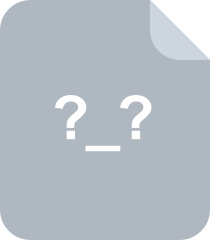
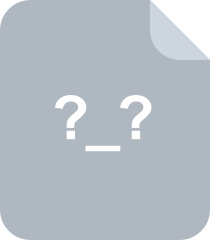
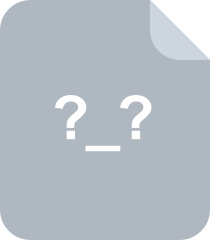
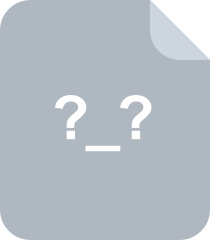
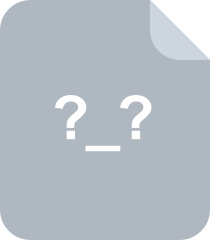
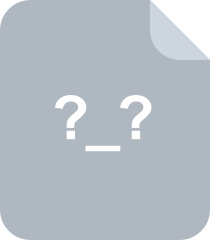
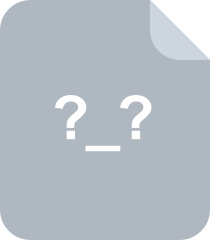
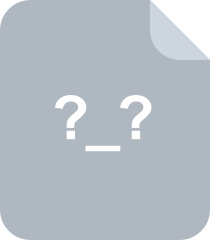
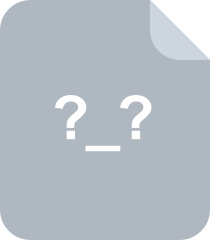
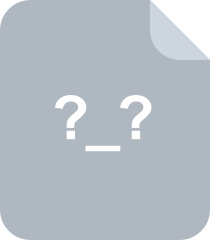
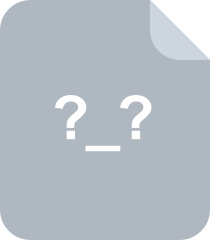
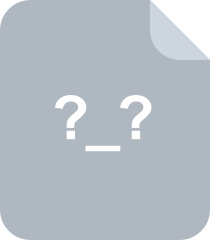
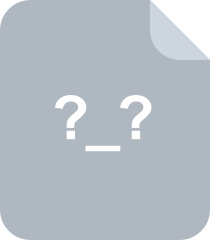
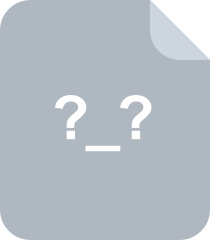
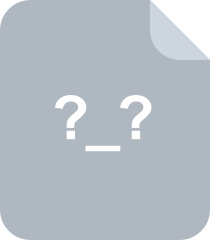
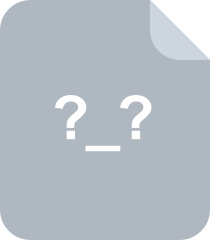
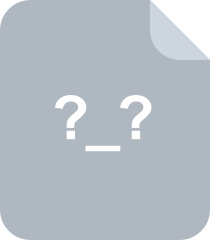
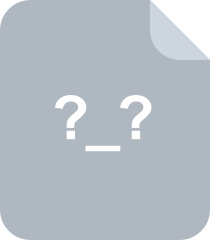
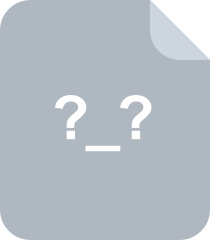
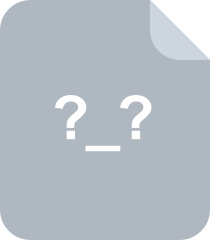
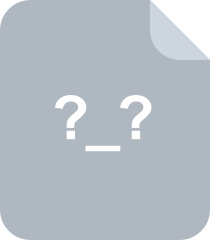
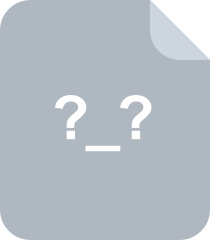
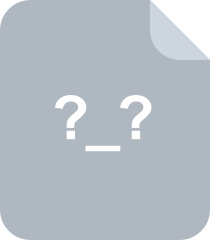
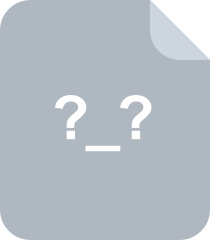
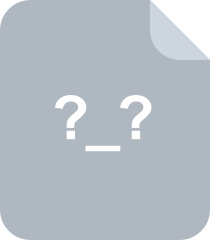
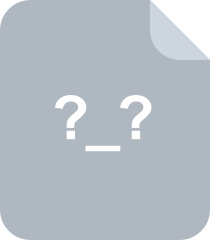
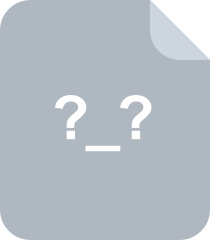
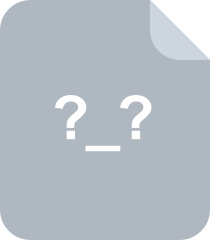
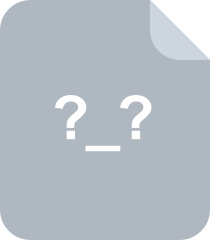
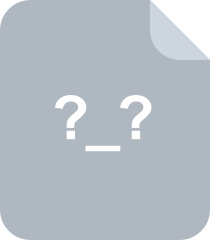
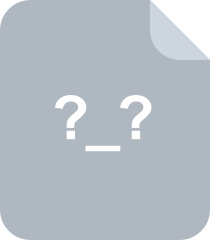
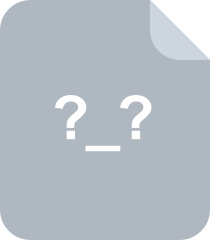
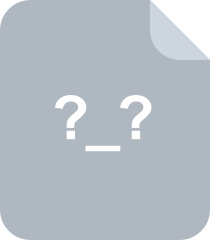
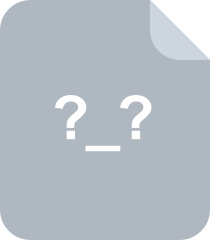
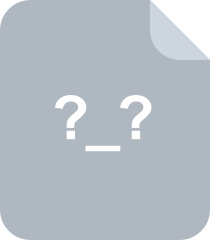
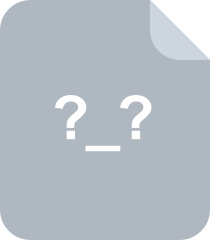
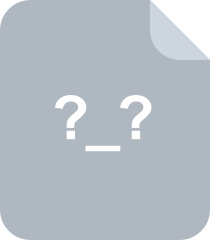
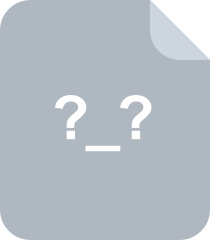
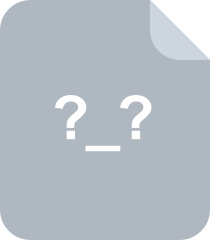
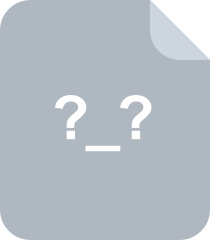
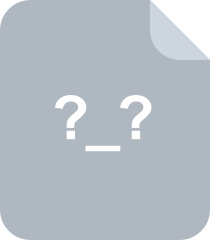
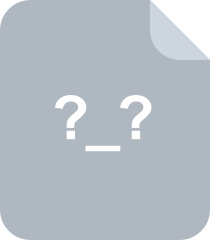
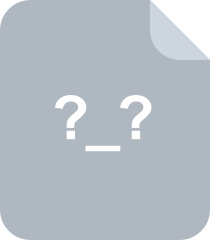
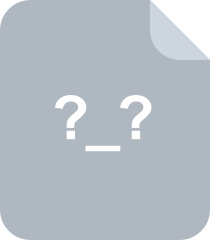
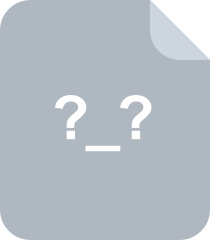
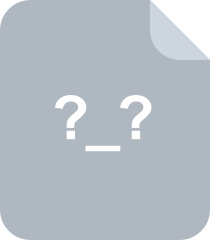
共 1512 条
- 1
- 2
- 3
- 4
- 5
- 6
- 16
资源评论
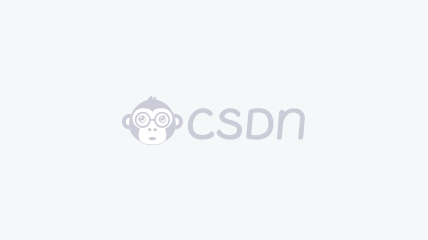

及拉
- 粉丝: 0
- 资源: 7
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

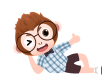
最新资源
- 基于Java Swing实现的飞机大战游戏.zip
- frida-server魔改 深度魔改
- 基于Java的奖励养成类蓝牙联机游戏.zip
- 基于Java+Swing的石头剪刀布游戏.zip
- Java作战小游戏.zip学习资料程序大作业
- Easyx的小游戏,飞翔的小鸟
- Tetris GUI game based on Java language development(基于Java语言开发的俄罗斯方块GUI小游戏 ).zip
- html常规学习.zip资源资料用户手册
- Semester Examination Works. 烟台科技学院,智能工程学院,Java编程基础课设 Java打字游戏.zip
- PingFang SC、HK、TC(Win 完美协作-修改版).apk
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


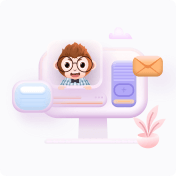
安全验证
文档复制为VIP权益,开通VIP直接复制
