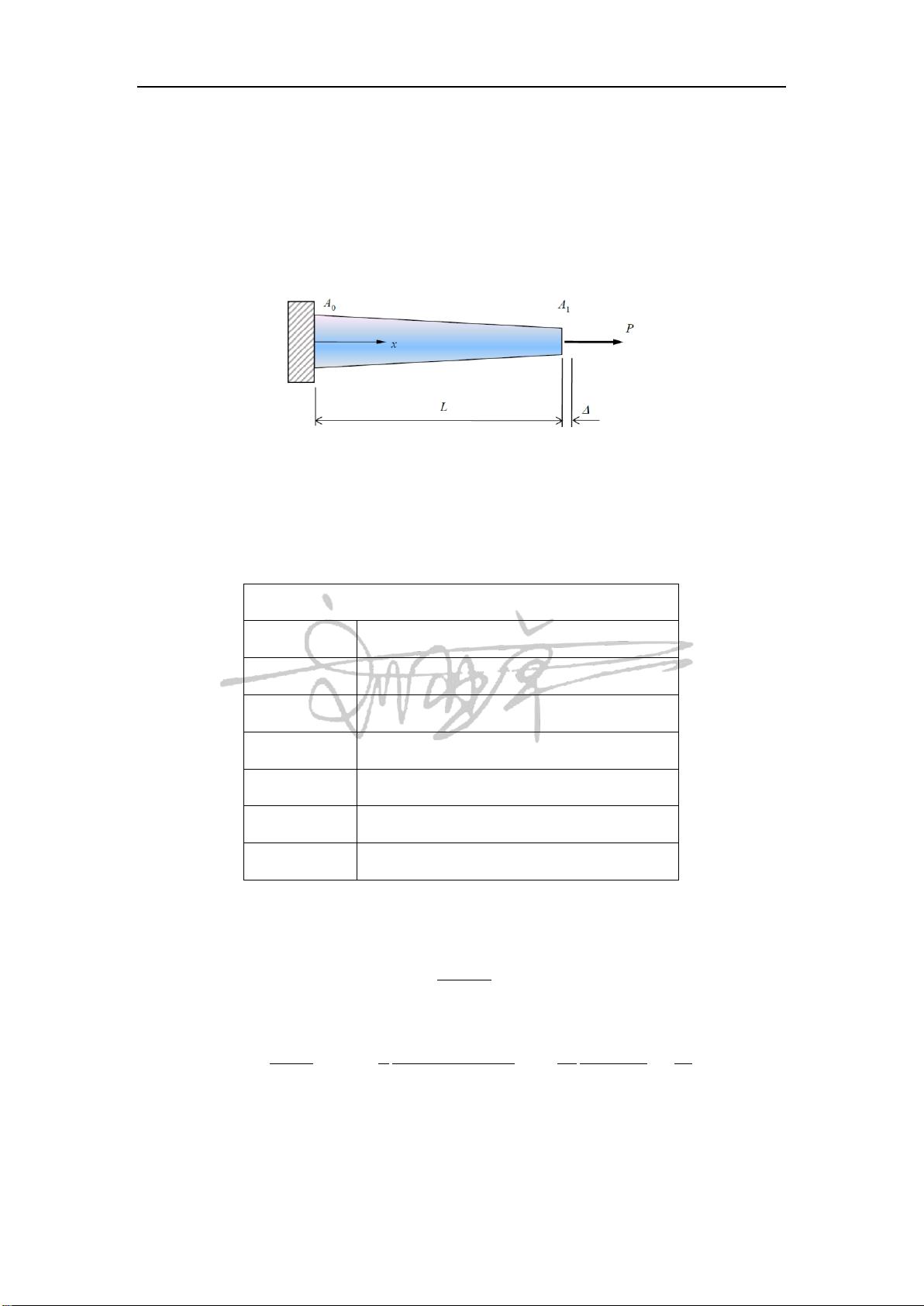

鹰忍
- 粉丝: 84
- 资源: 4700
最新资源
- 基于蒙特卡洛法的概率潮流分析:研究IEEE 33节点电网光伏与风电概率出力模型及其电压概率出力曲线,基于蒙特卡洛法的光伏和风电概率出力模型在IEEE33节点电网的概率潮流计算与电压概率出力曲线研究,基
- 多智能体协同与分布式博弈的优化一致性方法,基于多智能体的分布式博弈策略与一致性协调机制,多智能体一致性的分布式博弈方法 ,多智能体一致性; 分布式博弈; 方法,分布式博弈:多智能体一致性协同算法研究
- 基于瞬时无功功率理论的并联型两电平有源电力滤波器仿真计算与实现:谐波抑制、无功补偿与电网电压条件下的高效稳定运行,基于瞬时无功功率理论的并联型两电平有源电力滤波器仿真计算与实现:谐波抑制、无功补偿及电
- 可编程滤波器ADA2200的配置和应用解析
- DeepSeek-R1论文中英文对照版.pdf
- 使用CarSim和Simulink联合仿真实现无人驾驶跟踪双移线轨迹技术挑战的探索,使用CarSim和Simulink联合仿真实现无人驾驶跟踪双移线轨迹的挑战与优势,无人驾驶 carsim+simul
- DeepSeek如何赋能职场应用?从提示语技巧到多场景应用-清华大学.pdf
- Labview与西门子PLC联机通讯实战:拓展性强且附赠完整源码解析,Labview与西门子PLC联机通讯测试实践:拓展性强并附赠通讯源码详解,1.Labview与西门子PLC联机通讯实现测试 2.拓
- DeepSeek 与 DeepSeek-R1 专业研究报告.pdf
- 高电流热插拔应用中TPS2393A控制器的优化设计与应用
- Ansys Comsol力磁耦合仿真:电磁无损检测技术与流固耦合分析的深度探索,Ansys Comsol力磁耦合仿真:电磁无损检测技术与流固耦合分析的深度探究,Ansys Comsol 力磁耦合仿真
- 基于Matlab的GC5322 DPD系统数据分析方法和技术要点
- 户外储能电源设计方案:2KW双向逆变器主板技术资料汇编 该资料包含原理文件、PCB文件、源代码、BOM表及非标件电感与变压器规格参数等,适用于2KW(可调至3KW)户外储能电源项目 该方案采用双向D
- 腾讯详解-详解DeepSeek: 模型训练、优化及数据处理的技术精髓
- hello-algo-1.2.0-zh-hant-java版本hello算法
- DC-DC变换Boost与Buck电路的双闭环控制策略:占空比调控下的电压调整技术,DC-DC变换Boost与Buck电路双闭环控制技术研究:占空比调控输入与输出电压的精准策略,DC-DC变的Boos
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


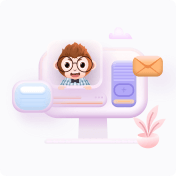