/**************************************************************************************
//------------------ MMC/SD-Card Reading and Writing implementation -------------------
//FileName : mmc.c
//Function : Connect AVR to MMC/SD
//Created by : ZhengYanbo
//Created date : 15/08/2005
//Version : V1.2
//Last Modified: 19/08/2005
//Filesystem : Read or Write MMC without any filesystem
//CopyRight (c) 2005 ZhengYanbo
//Email: Datazyb_007@163.com
****************************************************************************************/
#include "mmc.h"
#if USE_MMC
//****************************************************************************
// Port Init
void MMC_Port_Init(void)
//****************************************************************************
{
//Config ports
MMC_Direction_REG.SPI_DI=0; //Set Pin MMC_DI as Input
MMC_Direction_REG.SPI_Clock=1; //Set Pin MMC_Clock as Output
MMC_Direction_REG.SPI_DO=1; //Set Pin MMC_DO as Output
MMC_Direction_REG.MMC_Chip_Select=1; //Set Pin MMC_Chip_Select as Output
//busy led port init
MMC_Direction_REG.SPI_BUSY=1; //Set spi busy led port output
MMC_BUSY_LED=1; //busy led off
MMC_CS_PIN=1; //Set MMC_Chip_Select to High,MMC/SD Invalid.
}
//****************************************************************************
//Routine for Init MMC/SD card(SPI-MODE)
unsigned char MMC_Init(void)
//****************************************************************************
{
unsigned char retry,temp;
unsigned char i;
unsigned char CMD[] = {0x40,0x00,0x00,0x00,0x00,0x95};
//MMC_Port_Init(); //Init SPI port
for(i=0;i<200;i++) //Wait MMC/SD ready...
{
#asm("nop");
}
Init_Flag=1; //Set the init flag
for (i=0;i<0x0f;i++)
{
Write_Byte_MMC(0xff); //send 74 clock at least!!!
}
//Send Command CMD0 to MMC/SD Card
retry=0;
do
{ //retry 200 times to send CMD0 command
temp=Write_Command_MMC(CMD);
retry++;
if(retry==200)
{ //time out
return(INIT_CMD0_ERROR);//CMD0 Error!
}
}
while(temp!=1);
//Send Command CMD1 to MMC/SD-Card
CMD[0] = 0x41; //Command 1
CMD[5] = 0xFF;
retry=0;
do
{ //retry 100 times to send CMD1 command
temp=Write_Command_MMC(CMD);
retry++;
if(retry==100)
{ //time out
return(INIT_CMD1_ERROR);//CMD1 Error!
}
}
while(temp!=0);
Init_Flag=0; //Init is completed,clear the flag
MMC_Disable(); //set MMC_Chip_Select to high
return(0); //All commands have been taken.
}
//****************************************************************************
//returns the :
// size of the card in MB ( ret * 1024^2) == bytes
// sector count and multiplier MB are in u08 == C_SIZE / (2^(9-C_SIZE_MULT))
// name of the media
void MMC_get_volume_info(void)
//****************************************************************************
{ unsigned char i;
VOLUME_INFO_TYPE MMC_volume_Info,*vinf;
vinf=&MMC_volume_Info; //Init the pointoer;
// read the CSD register
Read_CSD_MMC(sectorBuffer.data);
// get the C_SIZE value. bits [73:62] of data
// [73:72] == sectorBuffer.data[6] && 0x03
// [71:64] == sectorBuffer.data[7]
// [63:62] == sectorBuffer.data[8] && 0xc0
vinf->sector_count = sectorBuffer.data[6] & 0x03;
vinf->sector_count <<= 8;
vinf->sector_count += sectorBuffer.data[7];
vinf->sector_count <<= 2;
vinf->sector_count += (sectorBuffer.data[8] & 0xc0) >> 6;
// get the val for C_SIZE_MULT. bits [49:47] of sectorBuffer.data
// [49:48] == sectorBuffer.data[5] && 0x03
// [47] == sectorBuffer.data[4] && 0x80
vinf->sector_multiply = sectorBuffer.data[9] & 0x03;
vinf->sector_multiply <<= 1;
vinf->sector_multiply += (sectorBuffer.data[10] & 0x80) >> 7;
// work out the MBs
// mega bytes in u08 == C_SIZE / (2^(9-C_SIZE_MULT))
vinf->size_MB = vinf->sector_count >> (9-vinf->sector_multiply);
// get the name of the card
Read_CID_MMC(sectorBuffer.data);
vinf->name[0] = sectorBuffer.data[3];
vinf->name[1] = sectorBuffer.data[4];
vinf->name[2] = sectorBuffer.data[5];
vinf->name[3] = sectorBuffer.data[6];
vinf->name[4] = sectorBuffer.data[7];
vinf->name[5] = 0x00; //end flag
//----------------------------------------------------------
LCDclrscr();
//Print Product name on lcd
i=0;
writestring("Product:");
while((vinf->name[i]!=0x00)&&(i<16)) writechar(vinf->name[i++]);
//Print Card Size(eg:128MB)
gotoxy(1,0);
writestring("Tot:");
writeNumber(vinf->size_MB); writestring("MB ");
//gotoxy(2,0);
//writestring("sector_mult:"); writeNumber(vinf->sector_multiply);
//gotoxy(3,0);
//writestring("sect_cnt:"); writeNumber(vinf->sector_count);
}
//****************************************************************************
//Send a Command to MMC/SD-Card
//Return: the second byte of response register of MMC/SD-Card
unsigned char Write_Command_MMC(unsigned char *CMD)
//****************************************************************************
{
unsigned char tmp;
unsigned char retry=0;
unsigned char i;
//set MMC_Chip_Select to high (MMC/SD-Card disable)
MMC_Disable();
//send 8 Clock Impulse
Write_Byte_MMC(0xFF);
//set MMC_Chip_Select to low (MMC/SD-Card active)
MMC_Enable();
//send 6 Byte Command to MMC/SD-Card
for (i=0;i<0x06;i++)
{
Write_Byte_MMC(*CMD++);
}
//get 16 bit response
Read_Byte_MMC(); //read the first byte,ignore it.
do
{ //Only last 8 bit is used here.Read it out.
tmp = Read_Byte_MMC();
retry++;
}
while((tmp==0xff)&&(retry<100));
return(tmp);
}
//****************************************************************************
//Routine for reading a byte from MMC/SD-Card
unsigned char Read_Byte_MMC(void)
//****************************************************************************
{
unsigned char temp=0;
unsigned char i;
MMC_BUSY_LED=0;
//Software SPI
for (i=0; i<8; i++) //MSB First
{
MMC_CLK_PIN=0; //Clock Impuls (Low)
if(Init_Flag) delay_us(8);
temp = (temp << 1) + MMC_DO_PIN; //read mmc data out pin
MMC_CLK_PIN=1; //set Clock Impuls High
if(Init_Flag) delay_us(8);
}
MMC_BUSY_LED=1;
return (temp);
}
//****************************************************************************
//Routine for sending a byte to MMC/SD-Card
void Write_Byte_MMC(unsigned char value)
//****************************************************************************
{
unsigned char i;
MMC_BUSY_LED=0;
//Software SPI
for (i=0; i<8; i++)
{ //write a byte
if (((value >> (7-i)) & 0x01)==0x01) MMC_DI_PIN=1; //Send bit by bit(MSB First)
else MMC_DI_PIN=0;
MMC_CLK_PIN=0; //set Clock Impuls low
if(Init_Flag) delay_us(8);
MMC_CLK_PIN=1; //set Clock Impuls High
if(Init_Flag) delay_us(8);
}//write a byte
MMC_DI_PIN=1; //set Output High
MMC_BUSY_LED=1;
}
//****************************************************************************
//Routine for writing a Block(512Byte) to MMC/SD-Card
//Return 0 if sector writing is completed.
unsigned char MMC_write_sector(unsigned long addr,unsigned char *Buffer)
//****************************************************************************
{
unsigned char tmp,retry;
unsigned int i;
//Command 24 is a writing blocks command for MMC/SD-Card.
unsigned char CMD[] = {0x58,0x00,0x00,0x00,0x00,0xFF};
#asm("cli"); //clear all interrupt.
addr = addr << 9; //addr = addr * 512
CMD[1] = ((addr & 0xFF000000) >>24 );
CMD[2] = ((addr & 0x00FF0000) >>16 )
标题中的“mmc.sd.rar_SD_SPI SD_sd spi_sd spi_sd读写”暗示了这是一个关于SPI接口操作MMC(MultiMediaCard)或SD(Secure Digital)存储卡的代码资源。SPI(Serial Peripheral Interface)是一种常见的串行通信协议,常用于微控制器与外部设备之间的通信,如存储卡、传感器等。在本例中,它被用来实现对MMC和SD卡的读写功能。 描述中提到的"software spi操作mmc/sd代码,实现mmc/sd读写操作"进一步明确了这个压缩包的内容。Software SPI,或称为Bit-Banging SPI,是通过软件模拟SPI协议来实现的,通常在硬件SPI接口不可用或需要高度灵活性时使用。这种方式需要微控制器的CPU直接控制数据线来完成SPI通信。 标签中的“sd spi_sd sd__spi sd_spi sd读写”是关键词,强调了与SD卡和SPI接口相关的操作以及读写功能。`spi_sd`可能指的是一个特定的库或函数集,用于通过SPI与SD卡交互。 在压缩包的文件名称列表中,我们可以看到: 1. `mmc.c`:这是一个C语言源代码文件,很可能包含了实现SPI接口与MMC/SD卡交互的函数和逻辑。 2. `mmc.h`:这是对应的头文件,通常包含函数原型声明、枚举、结构体等,供`mmc.c`或其他模块使用。 3. `www.pudn.com.txt`:这可能是一个文本文件,可能是下载资源的来源信息或者版权说明。 这个压缩包提供的资源是关于如何使用Software SPI在嵌入式系统中实现对MMC/SD卡的读写操作。开发者可以通过`mmc.c`和`mmc.h`中的函数来控制SD卡,进行数据的存取。这些代码可能包括初始化SPI接口、发送命令、读取响应、传输数据等步骤。对于学习和理解SPI通信协议,以及在没有硬件SPI支持的平台上与SD卡交互,这份代码会是一个很好的参考资料。开发者可以在此基础上进行修改和优化,以适应不同的应用场景。
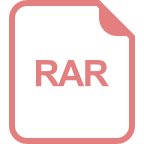
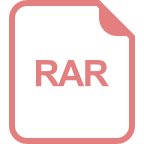
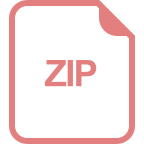
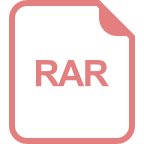
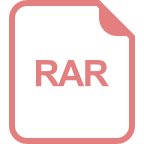
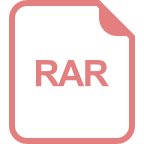
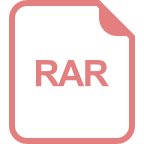
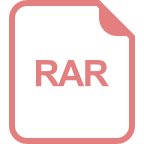
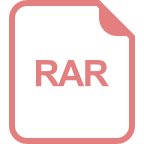
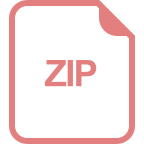
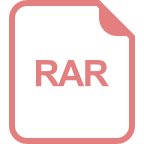
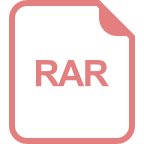
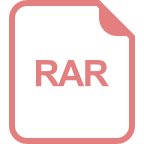
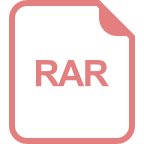
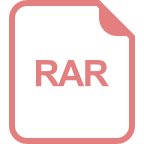
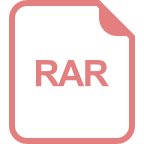
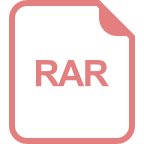
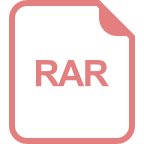

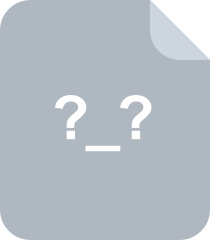
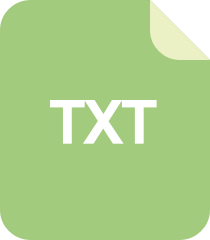
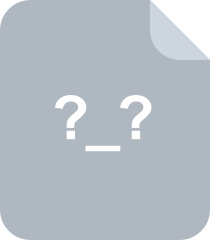
- 1
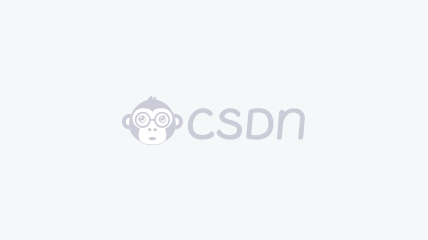
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整

- 粉丝: 80
- 资源: 1万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

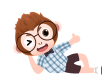
最新资源
- 【制度】新员工导师制.doc
- 自组织映射算法结合BP模型做SOMBP模型的多分类建模,数据是多变量输入,单变量输出做分类预测,可以出分类预测图和混淆矩阵图,要求matlab版本在2018b及以上
- 师带徒考评记录表模板.xls
- 导师带徒考核打分表.xls
- 师带徒绩效考核表2.xls
- 师带徒培训计划(机修).xls
- 导师导师制评分细则.xlsx
- 师带徒培养计划机械.xls
- 【方法】如何在企业推行导师制.docx
- 汇川MD500E变频器开发方案 源码+解析,全套齐全资料, 是资料,不是实物 MD500E代码方案和解析文档+原理图+送仿真资料 资料全 包含pmsm的foc控制算法,电阻、电感、磁链
- 【表格】导师导师制评分细则.xlsx
- 【表格】导师带徒考核打分表.xls
- 【制度】导师制管理制度.docx
- 大炮打蚊子c-test-day-4.8.rar
- 导师制培养方案.pptx
- 在岗辅导与导师制.ppt

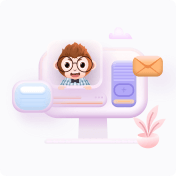
