%OPTPARSE Standard option parser for Toolbox functions
%
% OPTOUT = TB_OPTPARSE(OPT, ARGLIST) is a generalized option parser for
% Toolbox functions. OPT is a structure that contains the names and
% default values for the options, and ARGLIST is a cell array containing
% option parameters, typically it comes from VARARGIN. It supports options
% that have an assigned value, boolean or enumeration types (string or
% int).
%
% The software pattern is:
%
% function(a, b, c, varargin)
% opt.foo = false;
% opt.bar = true;
% opt.blah = [];
% opt.stuff = {};
% opt.choose = {'this', 'that', 'other'};
% opt.select = {'#no', '#yes'};
% opt = tb_optparse(opt, varargin);
%
% Optional arguments to the function behave as follows:
% 'foo' sets opt.foo := true
% 'nobar' sets opt.foo := false
% 'blah', 3 sets opt.blah := 3
% 'blah',{x,y} sets opt.blah := {x,y}
% 'that' sets opt.choose := 'that'
% 'yes' sets opt.select := (the second element)
% 'stuff', 5 sets opt.stuff to {5}
% 'stuff', {'k',3} sets opt.stuff to {'k',3}
%
% and can be given in any combination.
%
% If neither of 'this', 'that' or 'other' are specified then opt.choose := 'this'.
% Alternatively if:
% opt.choose = {[], 'this', 'that', 'other'};
% then if neither of 'this', 'that' or 'other' are specified then opt.choose := []
%
% If neither of 'no' or 'yes' are specified then opt.select := 1.
%
% Note:
% - That the enumerator names must be distinct from the field names.
% - That only one value can be assigned to a field, if multiple values
% are required they must placed in a cell array.
% - To match an option that starts with a digit, prefix it with 'd_', so
% the field 'd_3d' matches the option '3d'.
% - OPT can be an object, rather than a structure, in which case the passed
% options are assigned to properties.
%
% The return structure is automatically populated with fields: verbose and
% debug. The following options are automatically parsed:
% 'verbose' sets opt.verbose := true
% 'verbose=2' sets opt.verbose := 2 (very verbose)
% 'verbose=3' sets opt.verbose := 3 (extremeley verbose)
% 'verbose=4' sets opt.verbose := 4 (ridiculously verbose)
% 'debug', N sets opt.debug := N
% 'showopt' displays opt and arglist
% 'setopt',S sets opt := S, if S.foo=4, and opt.foo is present, then
% opt.foo is set to 4.
%
% The allowable options are specified by the names of the fields in the
% structure opt. By default if an option is given that is not a field of
% opt an error is declared.
%
% [OPTOUT,ARGS] = TB_OPTPARSE(OPT, ARGLIST) as above but returns all the
% unassigned options, those that don't match anything in OPT, as a cell
% array of all unassigned arguments in the order given in ARGLIST.
%
% [OPTOUT,ARGS,LS] = TB_OPTPARSE(OPT, ARGLIST) as above but if any
% unmatched option looks like a MATLAB LineSpec (eg. 'r:') it is placed in LS rather
% than in ARGS.
%
% [OBJOUT,ARGS,LS] = TB_OPTPARSE(OPT, ARGLIST, OBJ) as above but properties
% of OBJ with matching names in OPT are set.
% Ryan Steindl based on Robotics Toolbox for MATLAB (v6 and v9)
%
% Copyright (C) 1993-2017, by Peter I. Corke
%
% This file is part of The Robotics Toolbox for MATLAB (RTB).
%
% RTB is free software: you can redistribute it and/or modify
% it under the terms of the GNU Lesser General Public License as published by
% the Free Software Foundation, either version 3 of the License, or
% (at your option) any later version.
%
% RTB is distributed in the hope that it will be useful,
% but WITHOUT ANY WARRANTY; without even the implied warranty of
% MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
% GNU Lesser General Public License for more details.
%
% You should have received a copy of the GNU Leser General Public License
% along with RTB. If not, see <http://www.gnu.org/licenses/>.
%
% http://www.petercorke.com
% Modifications by Joern Malzahn to support classes in addition to structs
function [opt,others,ls] = tb_optparse(in, argv, cls)
if nargin == 1
argv = {};
end
if nargin < 3
cls = [];
end
if ~iscell(argv)
error('RTB:tboptparse:badargs', 'input must be a cell array');
end
arglist = {};
argc = 1;
opt = in;
if ~isfield(opt, 'verbose')
opt.verbose = false;
end
if ~isfield(opt, 'debug')
opt.debug = 0;
end
showopt = false;
choices = [];
while argc <= length(argv)
% index over every passed option
option = argv{argc};
assigned = false;
if isstr(option)
switch option
% look for hardwired options
case 'verbose'
opt.verbose = true;
assigned = true;
case 'verbose=2'
opt.verbose = 2;
assigned = true;
case 'verbose=3'
opt.verbose = 3;
assigned = true;
case 'verbose=4'
opt.verbose = 4;
assigned = true;
case 'debug'
opt.debug = argv{argc+1};
argc = argc+1;
assigned = true;
case 'setopt'
new = argv{argc+1};
argc = argc+1;
assigned = true;
% copy matching field names from new opt struct to current one
for f=fieldnames(new)'
if isfield(opt, f{1})
opt.(f{1}) = new.(f{1});
end
end
case 'showopt'
showopt = true;
assigned = true;
otherwise
% does the option match a field in the opt structure?
% if isfield(opt, option) || isfield(opt, ['d_' option])
% if any(strcmp(fieldnames(opt),option)) || any(strcmp(fieldnames(opt),))
if isfield(opt, option) || isfield(opt, ['d_' option]) || isprop(opt, option)
% handle special case if we we have opt.d_3d, this
% means we are looking for an option '3d'
if isfield(opt, ['d_' option]) || isprop(opt, ['d_' option])
option = ['d_' option];
end
%** BOOLEAN OPTION
val = opt.(option);
if islogical(val)
% a logical variable can only be set by an option
opt.(option) = true;
else
%** OPTION IS ASSIGNED VALUE FROM NEXT ARG
% otherwise grab its value from the next arg
try
opt.(option) = argv{argc+1};
if iscell(in.(option)) && isempty(in.(option))
% input was an empty cell array
if ~iscell(opt.(option))
% make it a cell
opt.(option) = cell( opt.(option) );
end
end
catch me
if strcmp(me.identifier, 'MATLAB:badsubscript')
error('RTB:tboptparse:badargs', 'too few arguments provided for option: [%s]', option);
else
rethrow(me);
end
end
argc = argc+1;
end
assigned = true;
elseif length(option)>2 && strcmp(option(1:2), 'no') && isfield(opt, option(3:end))
%* BOOLEAN OPTION PREFIXED BY 'no'

四散
- 粉丝: 68
- 资源: 1万+
最新资源
- 白色简洁风格的Zero企业网站模板.zip
- 白色简洁风格的奥迪mini跑车企业网站模板.zip
- 白色简洁风格的办公office企业网站模板下载.zip
- 白色简洁风格的办公管理后台系统源码下载.zip
- 白色简洁风格的办公室装修公司企业网站模板.zip
- 白色简洁风格的办公平台登录表源码下载.zip
- 白色简洁风格的办公室室内设计门户网站模板下载.zip
- 白色简洁风格的别墅设计装修整站网站模板.zip
- 白色简洁风格的别墅整站网站模板.zip
- 白色简洁风格的博客论坛后台系统源码下载.zip
- 白色简洁风格的餐厅菜品系列源码下载.zip
- 白色简洁风格的博客论坛后台统计源码下载.zip
- 白色简洁风格的餐厅会员登录框源码下载.zip
- 白色简洁风格的餐厅服务团队整站网站源码下载.zip
- 白色简洁风格的餐厅美味食谱整站网站源码下载.zip
- 白色简洁风格的餐饮食材食谱整站网站源码下载.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


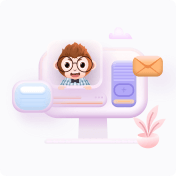