/**
* Vietnamese Input Method Editor (VietIME)
* @author: Quan Nguyen
* @version: 1.0.1, 22 June 2002
* @see: http://vietime.sourceforge.net
*
* Copyright (C) 2002 Quan Nguyen (nguyenq@users.sourceforge.net)
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
*/
package ime.viet;
import java.awt.*;
import java.awt.event.*;
import java.awt.font.*;
import java.awt.im.*;
import java.awt.im.spi.*;
import java.io.*;
import java.text.*;
import java.util.*;
import java.util.Locale;
import javax.swing.*;
public class VietInputMethod implements InputMethod {
private static Locale VIET_VNI_SM = new Locale("vi", "", "VNI SmartMark");
private static Locale VIET_VIQR_SM = new Locale("vi", "", "VIQR SmartMark");
private static Locale VIET_TELEX_SM = new Locale("vi", "", "Telex SmartMark");
private static Locale VIET_VNI = new Locale("vi", "", "VNI");
private static Locale VIET_VIQR = new Locale("vi", "", "VIQR");
private static Locale VIET_TELEX = new Locale("vi", "", "Telex");
private static Locale[] SUPPORTED_LOCALES = {VIET_VNI_SM, VIET_VIQR_SM, VIET_TELEX_SM,
VIET_VNI, VIET_VIQR, VIET_TELEX};
// final static String NEWLINE = System.getProperty("line.separator");
static final char ESCAPE_CHAR = '\\';
static final String CONSONANTS = "BCFGHJKLMNPQRSTVXZbcfghjklmnpqrstvxz";
static Robot robot;
// lookup table - shared by all instances of this input method
// private static Properties vietSymbols;
// windows - shared by all instances of this input method
private static Window statusWindow;
// per-instance state
private InputMethodContext inputMethodContext;
private Locale locale;
private boolean converted;
private StringBuffer rawText;
private String convertedText;
private String selectedInputMethod = null;
private boolean smartMarkingOn;
public VietInputMethod() throws IOException {
// if (vietSymbols == null) {
// vietSymbols = loadProperties("/resources/names.properties");
// }
try {
robot = new Robot();
}
catch (AWTException ae) {
}
rawText = new StringBuffer();
}
// private Properties loadProperties(String fileName) throws IOException {
// Properties props = new Properties();
// InputStream stream = this.getClass().getResourceAsStream(fileName);
// props.load(stream);
// stream.close();
// return props;
// }
public void setInputMethodContext(InputMethodContext context) {
inputMethodContext = context;
if (statusWindow == null) {
statusWindow = context.createInputMethodWindow("Viet Input Method", false);
Label label = new Label();
label.setBackground(Color.white);
label.setAlignment(Label.CENTER);
statusWindow.add(label);
updateStatusWindow(locale);
label.setSize(200, 50);
statusWindow.pack();
Dimension d = Toolkit.getDefaultToolkit().getScreenSize();
statusWindow.setLocation(d.width - statusWindow.getWidth(),
d.height - statusWindow.getHeight());
}
}
public boolean setLocale(Locale locale) {
for (int i = 0; i < SUPPORTED_LOCALES.length; i++) {
if (locale.equals(SUPPORTED_LOCALES[i])) {
this.locale = locale;
if (statusWindow != null) {
updateStatusWindow(locale);
}
return true;
}
}
return false;
}
public Locale getLocale() {
return locale;
}
public boolean isCompositionEnabled() {
return true;
}
void updateStatusWindow(Locale locale) {
synchronized (statusWindow) {
Label label = (Label) statusWindow.getComponent(0);
// String localeName = locale == null ? "null" : locale.getDisplayName();
if (locale.equals(VIET_VNI_SM))
selectedInputMethod = "VNI SmartMark";
else if (locale.equals(VIET_VIQR_SM))
selectedInputMethod = "VIQR SmartMark";
else if (locale.equals(VIET_TELEX_SM))
selectedInputMethod = "Telex SmartMark";
else if (locale.equals(VIET_VNI))
selectedInputMethod = "VNI";
else if (locale.equals(VIET_VIQR))
selectedInputMethod = "VIQR";
else if (locale.equals(VIET_TELEX))
selectedInputMethod = "Telex";
smartMarkingOn = selectedInputMethod.endsWith("SmartMark");
if (!label.getText().equals(selectedInputMethod)) {
label.setText(selectedInputMethod);
statusWindow.pack();
}
}
}
public void setCharacterSubsets(Character.Subset[] subsets) {
}
public void setCompositionEnabled(boolean enable) {
throw new UnsupportedOperationException();
}
public void notifyClientWindowChange(Rectangle location) {
}
public void dispatchEvent(AWTEvent event) {
if (event.getID() == KeyEvent.KEY_TYPED) {
KeyEvent e = (KeyEvent) event;
if (e.isControlDown())
return; // eliminate undesirable effects from Ctrl-key combinations
if (handleCharacter(e.getKeyChar())) {
e.consume();
}
}
if (event.getID() == KeyEvent.KEY_PRESSED) {
KeyEvent e = (KeyEvent) event;
int keyCode = e.getKeyCode();
switch (keyCode) {
case KeyEvent.VK_LEFT:
case KeyEvent.VK_RIGHT:
case KeyEvent.VK_UP:
case KeyEvent.VK_DOWN:
case KeyEvent.VK_HOME:
case KeyEvent.VK_END:
case KeyEvent.VK_PAGE_UP:
case KeyEvent.VK_PAGE_DOWN:
endComposition(); // commit if any caret move
break;
}
}
}
public void activate() {
if (!statusWindow.isVisible()) {
statusWindow.setVisible(true);
}
updateStatusWindow(locale);
}
public void deactivate(boolean isTemporary) {
}
public void hideWindows() {
statusWindow.hide();
}
public void removeNotify() {
}
public void endComposition() {
if (rawText.length() != 0) {
commit();
}
}
public void dispose() {
}
public Object getControlObject() {
return null;
}
public void reconvert() {
throw new UnsupportedOperationException();
}
//
// Mapping for diacritical marks:
// 1:', 2:`, 3:?, 4:~, 5:., 6:^, 7:+, 8:(, 9:-
//
// Process key input and return appropriate accent based on selected input method
public char getAccentMark(char key) {
if (rawText.length() == 0) return key;
char base = rawText.charAt(rawText.length() - 1);
char accent = 0;
if (base != ESCAPE_CHAR && !Character.isLetter(base)) // if base character is not \ and not letter
return 0;
// VNI Input Method
if (selectedInputMethod.startsWith("VNI")) {
if (!Character.isDigit(key)) // check for accent keys
return key;
accent = key;
}
// VIQR Input Method
else if (selectedInputMethod.startsWith("VIQR")) {
if (!Character.isLetterOrDigit(key)) {
switch (key) {
case '\'': accent = '1'; break;
case '`': accent = '2'; break;
case '?': accent = '3'; break;
case '~': accent = '4'; break;
case '.': accent = '5'; break;
case '^': accent = '6'; break;
case '*':
case '+': accent = '7'; break;
case '(': accent = '8'; break;
}
}
else if (key == 'D' || key == 'd')
accent = '9';
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
跨平台多系统用输入法程序源码 VietIME uses the input method framework in the Java 2 platform (J2SE 1.4 or higher) to enable the collaboration between text editing components and input methods in entering Vietnamese text with any Java runtime environment. Text editing components that use the input method framework run on any Java application environment and support any text input methods available on that Java application environment without modifying or recompiling the text editing component.
资源推荐
资源详情
资源评论
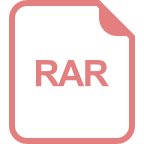
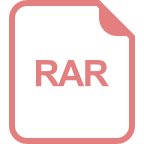
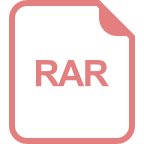
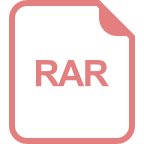
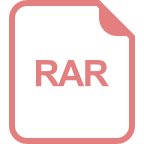
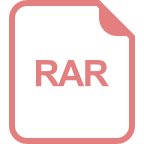
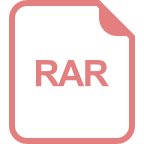
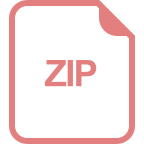
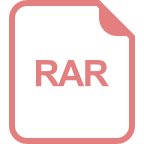
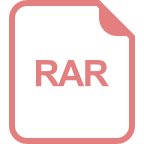
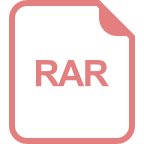
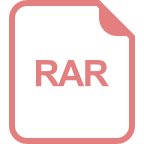
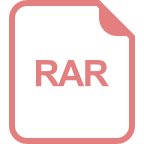
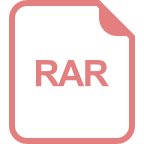
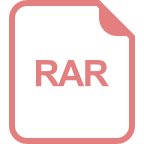
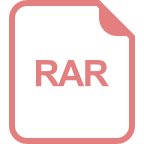
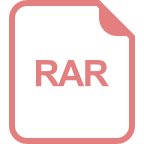
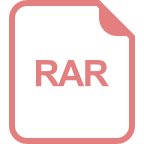
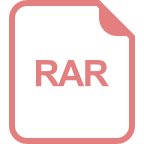
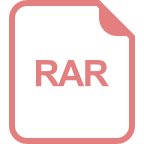
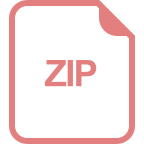
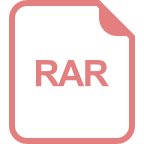
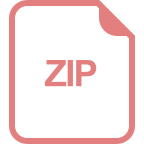
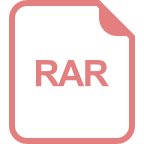
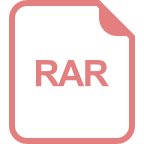
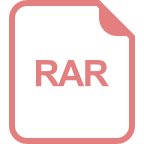
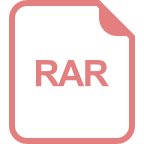
收起资源包目录

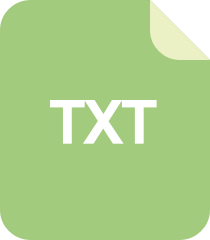


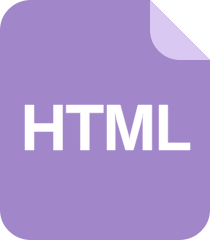


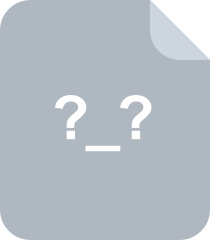

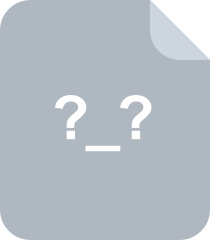

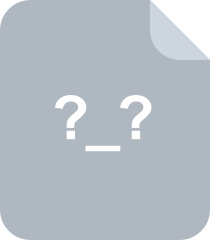
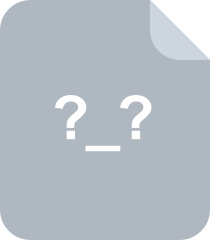
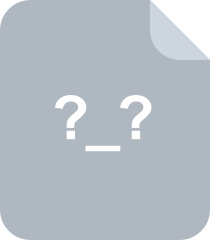
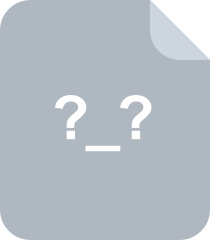
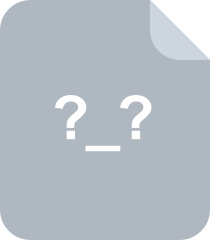
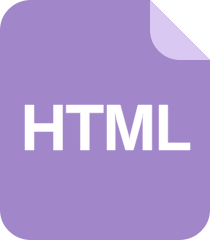
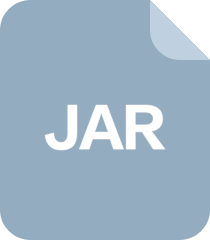
共 11 条
- 1
资源评论
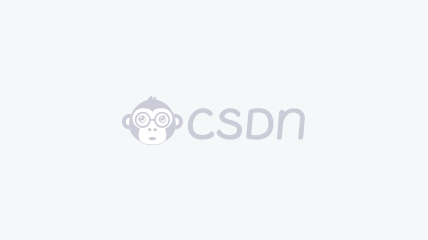
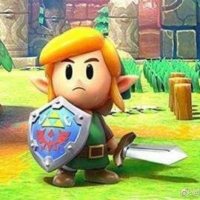
御道御小黑
- 粉丝: 79
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

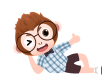
最新资源
- 漂亮动态效果PPT柱形图-3.pptx
- 山形柱状图数据分析PPT模板-1.pptx
- 长阴影扁平化PPT柱形图模板-1.pptx
- 山形锥形柱状图PPT模板素材-1.pptx
- 条形图-数据图表-简约扁平-3.pptx
- 条形图-数据图表-时尚红蓝-PPT模板-3.pptx
- 小人人数比例分析说明PPT模板-1.pptx
- 柱状图-数据图表-高端商务-3.pptx
- 柱状图-数据图表-扁平简洁-3.pptx
- 柱状图-数据图表-简约扁平 -3.pptx
- 柱状图-数据图表-清新活泼-3.pptx
- 柱状图-数据图表-折纸简洁-3.pptx
- 柱状图-数据图表-简约扁平--1.pptx
- windows tcp连通性测试工具tcping64
- CDN(内容分发网络)核心技术解析及其在网络优化中的应用
- 饼图-数据图表-简约清新 -3.pptx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


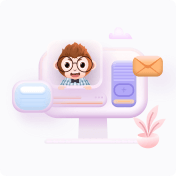
安全验证
文档复制为VIP权益,开通VIP直接复制
