AOV网络的topu排序.rar_aov_拓扑排序
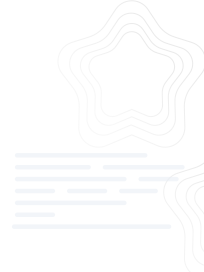

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
在计算机科学领域,AOV(Activity On Vertex,节点上的活动)网络是一种用于表示有向无环图(DAG,Directed Acyclic Graph)的数据结构。拓扑排序是针对这种数据结构的一种重要操作,它能对图中的节点进行线性排序,使得对于任何一条有向边 (u, v),节点 u 总是出现在节点 v 之前。拓扑排序在项目管理、任务调度、依赖关系分析等场景中有着广泛的应用。 AOV网络的拓扑排序算法通常有两种主要方法:深度优先搜索(DFS)和广度优先搜索(BFS)。下面将详细解释这两种方法: 1. 深度优先搜索(DFS)拓扑排序: - 初始化一个空栈,用于存放排序结果。 - 找到所有入度为0的节点,即没有前驱的节点,将它们加入栈中。 - 对每个栈中的节点执行DFS,访问其相邻节点,将其入度减1。如果发现某个相邻节点的入度变为0,将其加入栈中。 - 这个过程一直持续,直到所有节点都被访问过。最后栈中保存的就是一个拓扑排序的结果。如果在过程中发现存在环,则说明图不是有向无环图,无法进行拓扑排序。 2. 广度优先搜索(BFS)拓扑排序: - 使用队列来代替DFS中的栈,同样从所有入度为0的节点开始。 - 将这些节点放入队列中,然后进行BFS遍历。每次从队列头部取出一个节点,访问其所有邻居,将邻居的入度减1。如果某个邻居的入度变为0,将其加入队列。 - BFS过程持续到队列为空,此时队列中的节点顺序就是拓扑排序的结果。 AOV网络的topu排序.cpp 文件很可能是实现拓扑排序算法的C++代码,其中可能包含了DFS或BFS的具体实现。www.pudn.com.txt 文件可能是下载源或者相关资料的链接,但具体内容需要打开文件查看才能确定。 在实际应用中,拓扑排序对于解决如课程安排、生产流程规划等问题非常有用。例如,当安排课程时,需要确保先修课程在后修课程之前完成,这就对应了图中的有向边关系。通过拓扑排序,可以得到一个满足所有依赖关系的合理课程顺序。 AOV网络的拓扑排序是一种有效的处理有向无环图的方法,它能够揭示图中节点间的自然顺序,帮助我们优化任务的执行顺序。了解并掌握这个算法对于理解和解决依赖关系问题至关重要。
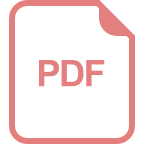
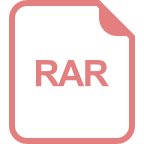
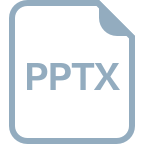
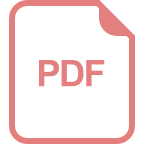
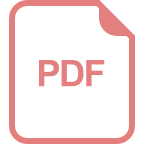
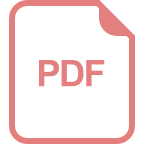
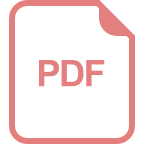
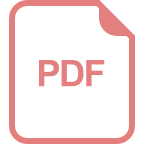
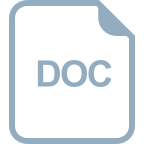
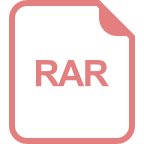
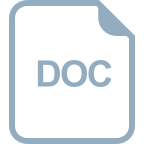
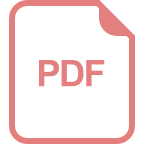
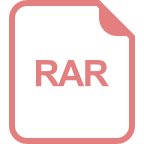
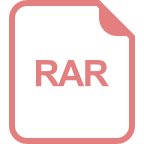
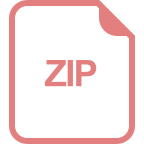
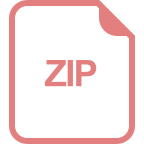
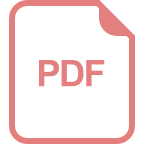
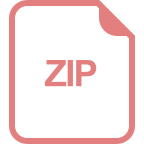
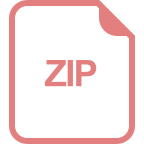

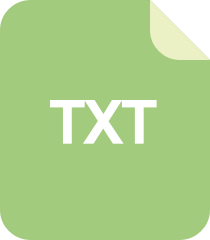
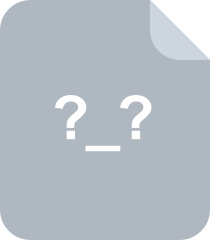
- 1
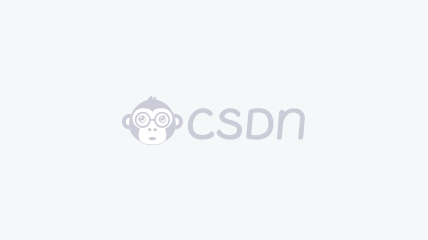
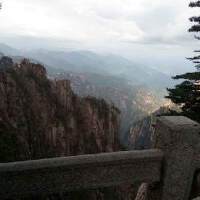
- 粉丝: 104
- 资源: 1万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

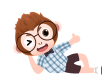
最新资源
- 基于机器学习的泊位调度优化与船舶到达时间预测提升港口服务质量和效率的研究
- 基于数据驱动进化算法的风电场布局优化研究与应用
- 电气工程中无铁芯永磁线性电机的设计与磁悬浮应用研究
- 雷达信号处理中的基于流形分离的最大似然联合DOA与极化估计方法
- 无人驾驶 carsim+simulink联合仿真 跟踪双移线轨迹
- 精选毕设项目-爱跑腿外卖.zip
- 精选毕设项目-爱拼宝宝商城.zip
- 精选毕设项目-百度小说.zip
- 精选毕设项目-百度小说搜索.zip
- 精选毕设项目-备忘录.zip
- 精选毕设项目-辩论倒计时.zip
- 精选毕设项目-步步高字典.zip
- 精选毕设项目-侧滑布局.zip
- 精选毕设项目-查拼音.zip
- 精选毕设项目-茶叶商城.zip
- 精选毕设项目-查看电影文章.zip

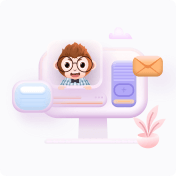
