PdfLayoutManager
================
This project is the precursor to [PdfLayoutMgr2](https://github.com/PlanBase/PdfLayoutMgr2)
which adds inline styles, justified text, font loading/caching, and other improvements.
LayoutManager1 may still be updated to ease users transition to LayoutMgr2 by copying the API of similar classes.
Significant new features will only be supported by LayoutMgr2.
---
LayoutManager1 is a wrapper for PDFBox to add line-breaking, page-breaking, and tables.
It uses a box-model (like HTML) for styles.
Requires PDFBox which in turn requires Log4J or apache commons Logging.

Usage
=====
Example: [TestManualllyPdfLayoutMgr.java](src/test/java/TestManualllyPdfLayoutMgr.java)
API Docs are available from maven central.
I think the character encoding issues in an old (pre 2.0) version of PDFBox have been fixed. So I removed my Transliteration code for Russian which also converted any "High ANSI characters" to lower ANSI equivalents, or to bullets if no equivalent was available. If you get exceptions about character support, you now need to load a font that includes those characters, or trap them yourself. Here's how to load a font: https://pdfbox.apache.org/1.8/cookbook/workingwithfonts.html
Here's how I used to trap them (incomplete and no longer working with the new version of PDFBox):
https://github.com/GlenKPeterson/PdfLayoutManager/blob/master/src/main/java/com/planbase/pdf/layoutmanager/PdfLayoutMgr.java#L892
[](https://maven-badges.herokuapp.com/maven-central/com.planbase.pdf/PdfLayoutManager)
[](https://javadoc.io/doc/com.planbase.pdf/PdfLayoutManager)
Building from Source
====================
Requires Maven 3 and Java JDK 1.8+. Jar file ends up in the `target/` sub-folder.
API documentation can be built with `mvn javadoc:javadoc` and is then found at `target/site/apidocs/index.html`
A sample PDF named `test.pdf` shows up in the root folder of this project when you run `mvn test`.
A jar file can be built with `mvn clean package` and ends up in the `target/` sub-folder. Or type `mvn clean install` to build and install into your local maven repository.
FAQ
===
***Q: What languages/character sets does PdfLayoutManager support?***
**A:** The PDF spec guarantees support for [WinAnsiEncoding AKA Windows Code Page 1252](http://en.wikipedia.org/wiki/Windows-1252) and maybe four PDType1Fonts fonts without any font embedding. WinAnsi covers the following languages:
Afrikaans (af), Albanian (sq), Basque (eu), Catalan (ca), Danish (da), Dutch (nl), English (en), Faroese (fo), Finnish (fi), French (fr), Galician (gl), German (de), Icelandic (is), Irish (ga), Italian (it), Norwegian (no), Portuguese (pt), Scottish (gd), Spanish (es), and Swedish (sv)
In addition, PdfLayoutManager uses Romanized substitutions for the Cyrillic characters of the modern Russian (ru) alphabet according to ISO 9:1995 with the following phonetic substitutions: 'Ch' for Ч and 'Shch' for Щ.
This character set is good enough for many purposes. If a character is not supported, it is converted to a bullet, so that the omission is politely, professionally visible. See: [Transliteration Details](src/main/java/com/planbase/pdf/layoutmanager/PdfLayoutMgr.java#L841)
***Q: I want different fonts and more characters!***
**A:** Fonts that support a wide range of characters tend to be large (over one megabyte). Embedding such a font in every PDF file is unacceptable for most people who have to build PDF files on the fly for users to download, or to send in email. To avoid this, we would have to keep track of what characters are used, then embed an appropriate subset of a font in the resulting PDF. Different fonts are already divided into subsets, but not necessarily the same subsets. Almost no font has every character, and it would be your responsibility to provide fonts, and maybe fallback fonts that cover all the characters you might need.
If we supported this, I don't know how much it would slow down PDF creation. Maybe we'd have a separate project that just maps characters to font fragments. You'd run that first, then pass the ideally formatted/partitioned output to PdfLayoutManger to use quickly on-the-fly. Such a solution will require you to do some work and to understand some underlying character/font issues that our current character-set limitations allow us (and you) to ignore.
Last I checked, PDFBox had hard-coded a character encoding that made it difficult for me to work with alternative character encodings. What they did might be correct, but I looked at it, got confused and frustrated, then gave up. Another volunteer started playing with this and gave up too.
That said, this is definitely a solvable problem. There is a broad spectrum of for-profit PDF-producing software. One of the main reasons they can charge money for their products is because this problem is so hard.
**UPDATE 2016-01-20:** PDFBox (which this project is built on top of) 2.0 has Unicode support, which previous versions did not have. It's currently in Release-Candidate 3. I haven't had a chance to try it yet. Here's the fixed issue that I think should make what you want possible: https://issues.apache.org/jira/browse/PDFBOX-922
***Q: I don't want text wrapping. I just want to set the size of a cell and let it chop off whatever I put in there.***
**A:** PdfLayoutManager was intended to provide html-table-like flowing of text and resizing of cells to fit whatever you put in them, even across multiple pages. If you don't need that, use PDFBox directly. If you need other features of PdfLayoutManager, there is a minHeight() setting on table rows. Combined with padding and alignment, that may get you what you need to layout things that will always fit in the box.
***Q: Will PdfLayoutManager ever support cropping the contents of a fixed-size box?***
**A:** If individual letters or images have a dimension which is bigger than the same dimension of their bounding box, we either have to suppress their display, or crop them. The PDF spec mentions something about a "clipping path" that might be usable for cropping overflow if you turn it on, render your object, then turn it off again. I'm not currently aware of PDFBox support for this (if it's even possible).
If the contents are all little things, we could just show as many little letters or images as completely fit, then no more (truncate the list of contents). Showing none could make truncation work for big objects too, but I'm not in a rush to implement that since it's conceptually so different from the very reason for the existence of PdfLayoutManager.
Maybe some day I'll provide some sample code so you can do truncation yourself. [TextStyle](src/main/java/com/planbase/pdf/layoutmanager/TextStyle.java) has lineHeight() and stringWidthInDocUnits() that you may find useful for writing your own compatible cropping algorithm. If you do that (and it works well), I hope you'll consider contributing it back to PdfLayoutManager (at least to this doc) so that others can benefit!
***Q: Why doesn't PdfLayoutManager line-wrap my insanely long single-word test string properly?***
**A:** For text wrapping to work, the text needs occasional whitespace. In HTML, strings without whitespace do not wrap at all! In PdfLayoutManager, a long enough string will wrap at some point wider than the cell.
The text wrapping algorithm picks a slightly long starting guess for where to wrap the text, then steps backward looking for whitespace. If it doesn't find any whitspace, it splits the first line at it's original guess length and continues trying to wrap the rest of the text on the next line.
Recent Changes
==============
### 2020-10-06 Version 1.0.1
- Bumped dependencies
### 2020-04-20 Version 1

weixin_38642897
- 粉丝: 3
- 资源: 894
最新资源
- 西门子200SMART系列PLC自由口通讯CRC校验程序 该程序已经实测
- 基于事件触发机制的多智能体系统事件触发控制,Matlab数值仿真实验
- 基于Dart语言的Flutter应用开发设计源码
- 基于SpringBoot与阿里云AI的智慧云相册双端设计源码
- 基于maven-assembly-plugin的Spring Boot项目环境打包设计源码
- 串联混合动力汽车模型预测能量管理程序设计,在MATLAB环境下,利用脚本编写串联模型,并基于CasADi模型预测控制算法工具,结合构型图与参数进行MPC能量算法程序编制,测试工况为CLTC-P工况(可
- microsoft-edge-dev-133.0.3014.0-1.x86_64.rpm
- abaqus 随机喷丸仿真,附带随机喷丸模型生成源程序,模型尺寸,丸粒尺寸,个数,角度,速度等均可自由改动 源程序讲解视频,模型操作,后处理操作,模型文件均有 喷丸微观仿真
- comsol实现激光熔覆的凝固相场树枝晶生长 考虑溶质、 相场 温度场耦合 提供资料 全套的模型文件和参考文献以及讲解视频 利用凝固组织的建模和验证可以减少获得所需组织的迭代成本 结合Marango
- 双馈电机三矢量模型预测控制
- 基于深度混合核极限学习机DHKELM的回归预测,优化算法采用的是北方苍鹰NGO,可替成其他方法
- 多项式曲线拟合,c代码,可实现1阶线性,2-4阶多项式曲线拟合,代码注释详细,方便移植,书写规范 图片有现场拟合参数的1-4阶的keil仿真结果和Excel对照图 备注一下,这是个多项式求解代码,求
- 基于SpringCloud的ZebraCloud设计源码项目,JavaScript与Java双语言支持,正在孵化中...
- 基于Yapi接口平台的接口方法文件自动生成设计源码
- 事件触发,微电网分层下垂控制 有应用图中文献算法的matlab仿真模型
- 基于Python的DNF装备搭配及伤害计算设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


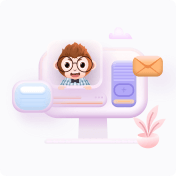