在iOS应用开发中,有时我们需要为用户界面提供额外的功能,比如添加隐藏键盘的选项。这篇教程将详细介绍如何在iOS应用中为键盘添加隐藏键盘的功能,主要涉及的技术点包括自定义UIBarButtonItem、UIView以及利用inputAccessoryView。 我们需要创建一个自定义的UIBarButtonItem,这个按钮将用于触发隐藏键盘的操作。为此,我们定义一个名为XMCustomKeyBoardBtn的类别,其中包含一个editableView属性,用来存储需要添加隐藏键盘功能的控件(如UITextField、UITextView或UISearchBar)。 ```objc @interface XMCustomKeyBoardBtn : UIBarButtonItem @property (strong, nonatomic) id editableView; @end @implementation XMCustomKeyBoardBtn @end ``` 接下来,创建一个自定义的UIView子类XMCustomKeyBoard。这个类的作用是在keyWindow上添加一个视图,并动态绑定隐藏键盘的方法。这是因为只有UIView的子类才能添加到keyWindow上,同时为了保持方法的活性,我们需要让这个类始终存活。 ```objc @interface XMCustomKeyBoard : UIView + (void) CancelableKeyboard:(id) editableView; + (void) CancelableKeyboard:(id) editableView CustomButtonItem:(UIBarButtonItem *)btn; @end @implementation XMCustomKeyBoard + (void) CancelableKeyboard:(id) editableView {...} + (void) CancelableKeyboard:(id) editableView CustomButtonItem:(UIBarButtonItem *)btn {...} @end ``` 在XMCustomKeyBoard的两个类方法中,我们为传入的编辑视图(editableView)设置了一个inputAccessoryView,这是一个显示在键盘上方的工具栏。在这个工具栏上,我们添加了一个隐藏键盘的按钮。`CancelableKeyboardToolBar:` 方法负责创建这个工具栏,并添加所需的按钮。 ```objc + (UIToolbar *)CancelableKeyboardToolBar:(id) editableView CustomButtonItem:(UIBarButtonItem *)btn addTarget:(id) target{ UIToolbar *toolbar = [[UIToolbar alloc] initWithFrame:CGRectMake(0, 0, CGRectGetWidth([UIApplication sharedApplication].keyWindow.frame), 40)]; toolbar.backgroundColor = [UIColor lightGrayColor]; UIBarButtonItem *button = btn ? btn : [UIBarButtonItem itemWithBarButtonSystemItem:UIBarButtonSystemItemCancel target:target action:@selector(hideKeyboard:)]; UIBarButtonItem *flexibleSpace = [[UIBarButtonItem alloc] initWithBarButtonSystemItem:UIBarButtonSystemItemFlexibleSpace target:nil action:nil]; toolbar.items = @[flexibleSpace, button]; return toolbar; } ``` 在`hideKeyboard:`方法中,我们将实现隐藏键盘的实际逻辑,这可能包括调用`resignFirstResponder`方法来让当前的文本输入视图失去焦点,从而收起键盘。 ```objc - (void)hideKeyboard:(UIBarButtonItem *)sender { [(UITextField *)sender.editableView resignFirstResponder]; } ``` 总结起来,实现iOS键盘隐藏功能的关键步骤包括: 1. 创建自定义的UIBarButtonItem子类,用于存储关联的编辑视图。 2. 创建自定义的UIView子类,用于添加到keyWindow并保持方法活性。 3. 在需要隐藏键盘的编辑视图上调用XMCustomKeyBoard的类方法,设置inputAccessoryView为包含隐藏键盘按钮的工具栏。 4. 实现隐藏键盘的方法,调用`resignFirstResponder`来隐藏键盘。 通过这种方式,用户在使用键盘时可以方便地点击隐藏按钮,快速收起键盘,提高了用户体验。在实际应用中,可以根据需求调整工具栏的样式和按钮的行为,以满足不同场景的需求。
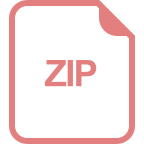
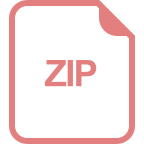
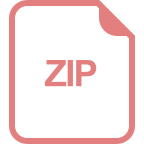
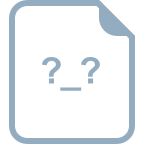
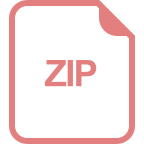
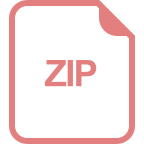
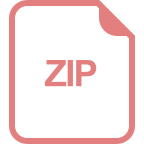
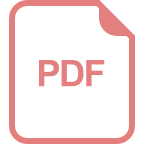
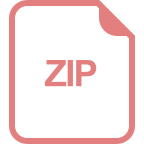
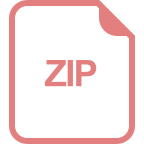
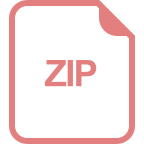
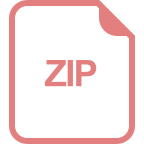
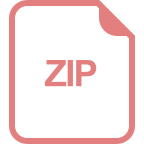
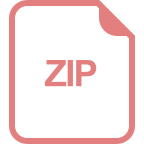
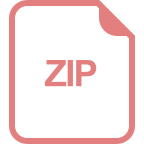
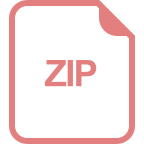
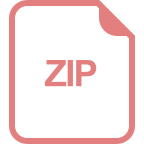
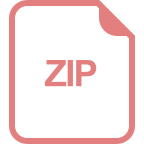
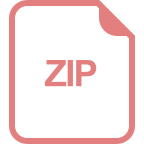
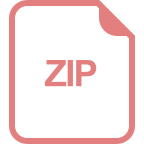
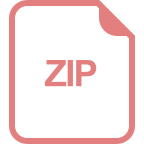
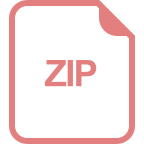
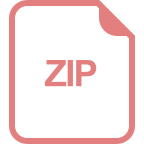
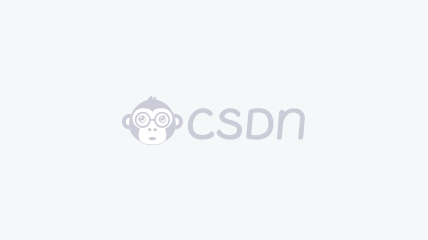

- 粉丝: 4
- 资源: 936
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

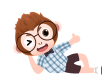
最新资源
- Python大作业-爬虫(高分大作业)
- Python 图片压缩工具
- qt4.8.6资源,用户qt安装,编译与学习
- (176465412)电气设计视频教程-Eplan.P8
- Python大作业爬虫项目并且用web展示爬虫的内容(高分项目)源码+说明
- Python项目-实例-27 生成词云图.zip
- (176566822)数据库课程设计ssm027学校运动会信息管理系统+jsp.sql
- C# WPF-激光焊接机配套软件源码及文档(带视觉需halcon)
- (177333248)c++实现的仿QQ贪吃蛇大作战多人联机游戏.zip
- Python大作业-爬虫(高分大作业).zip
- (177487602)c++ 家谱管理系统.zip
- IMG-8274.GIF
- (177938850)115-基于51单片机和PROTEUS的基于C51单片机的智能交通灯设计.zip
- 基于微信小程序的宏华水利小程序.zip
- (OC)数据加载SVG图片
- linux3.8.6内核资源

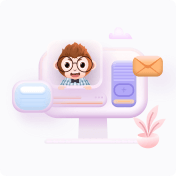
