<p align="center">
<img src="https://raw.githubusercontent.com/CocoaLumberjack/CocoaLumberjack/master/LumberjackLogo.png" title="Lumberjack logo" float=left>
</p>
CocoaLumberjack
===============
[](https://github.com/CocoaLumberjack/CocoaLumberjack/releases/latest)
[](https://opensource.org/licenses/BSD-3-Clause)
[](https://cocoadocs.org/docsets/CocoaLumberjack/)
[](https://github.com/Carthage/Carthage)

[](https://codecov.io/gh/CocoaLumberjack/CocoaLumberjack)
[](https://codebeat.co/projects/github-com-cocoalumberjack-cocoalumberjack-master)
**CocoaLumberjack** is a fast & simple, yet powerful & flexible logging framework for macOS, iOS, tvOS and watchOS.
## How to get started
First, install CocoaLumberjack via [CocoaPods](https://cocoapods.org), [Carthage](https://github.com/Carthage/Carthage), [Swift Package Manager](https://swift.org/package-manager/) or manually.
Then use `DDOSLogger` for iOS 10 and later, or `DDTTYLogger` and `DDASLLogger` for earlier versions to begin logging messages.
### CocoaPods
```ruby
platform :ios, '11.0'
target 'SampleTarget' do
use_frameworks!
pod 'CocoaLumberjack/Swift'
end
```
Note: `Swift` is a subspec which will include all the Obj-C code plus the Swift one, so this is sufficient.
For more details about how to use Swift with Lumberjack, see [this conversation](https://github.com/CocoaLumberjack/CocoaLumberjack/issues/405).
For Objective-C use the following:
```ruby
platform :ios, '11.0'
target 'SampleTarget' do
pod 'CocoaLumberjack'
end
```
### Carthage
Carthage is a lightweight dependency manager for Swift and Objective-C. It leverages CocoaTouch modules and is less invasive than CocoaPods.
To install with Carthage, follow the instruction on [Carthage](https://github.com/Carthage/Carthage)
Cartfile
```
github "CocoaLumberjack/CocoaLumberjack"
```
### Swift Package Manager
As of CocoaLumberjack 3.6.0, you can use the Swift Package Manager as integration method.
If you want to use the Swift Package Manager as integration method, either use Xcode to add the package dependency or add the following dependency to your Package.swift:
```swift
.package(url: "https://github.com/CocoaLumberjack/CocoaLumberjack.git", from: "3.8.0"),
```
Note that you may need to add both products, `CocoaLumberjack` and `CocoaLumberjackSwift` to your target since SPM sometimes fails to detect that `CocoaLumerjackSwift` depends on `CocoaLumberjack`.
### Install manually
If you want to install CocoaLumberjack manually, read the [manual installation](Documentation/GettingStarted.md#manual-installation) guide for more information.
### Swift Usage
Usually, you can simply `import CocoaLumberjackSwift`. If you installed CocoaLumberjack using CocoaPods, you need to use `import CocoaLumberjack` instead.
```swift
DDLog.add(DDOSLogger.sharedInstance) // Uses os_log
let fileLogger: DDFileLogger = DDFileLogger() // File Logger
fileLogger.rollingFrequency = 60 * 60 * 24 // 24 hours
fileLogger.logFileManager.maximumNumberOfLogFiles = 7
DDLog.add(fileLogger)
...
DDLogVerbose("Verbose")
DDLogDebug("Debug")
DDLogInfo("Info")
DDLogWarn("Warn")
DDLogError("Error")
```
### Obj-C usage
If you're using Lumberjack as a framework, you can `@import CocoaLumberjack;`.
Otherwise, `#import <CocoaLumberjack/CocoaLumberjack.h>`
```objc
[DDLog addLogger:[DDOSLogger sharedInstance]]; // Uses os_log
DDFileLogger *fileLogger = [[DDFileLogger alloc] init]; // File Logger
fileLogger.rollingFrequency = 60 * 60 * 24; // 24 hour rolling
fileLogger.logFileManager.maximumNumberOfLogFiles = 7;
[DDLog addLogger:fileLogger];
...
DDLogVerbose(@"Verbose");
DDLogDebug(@"Debug");
DDLogInfo(@"Info");
DDLogWarn(@"Warn");
DDLogError(@"Error");
```
### Objective-C ARC Semantic Issue
When integrating Lumberjack into an existing Objective-C it is possible to run into `Multiple methods named 'tag' found with mismatched result, parameter type or attributes` build error.
Add `#define DD_LEGACY_MESSAGE_TAG 0` before importing CocoaLumberjack or add `#define DD_LEGACY_MESSAGE_TAG 0` or add `-DDD_LEGACY_MESSAGE_TAG=0` to *Other C Flags*/*OTHER_CFLAGS* in your Xcode project.
## [swift-log](https://github.com/apple/swift-log) backend
CocoaLumberjack also ships with a backend implementation for [swift-log](https://github.com/apple/swift-log).
Simply add CocoaLumberjack as dependency to your SPM target (see above) and also add the `CocoaLumberjackSwiftLogBackend` product as dependency to your target.
You can then use `DDLogHandler` as backend for swift-log, which will forward all messages to CocoaLumberjack's `DDLog`. You will still configure the loggers and log formatters you want via `DDLog`, but writing log messages will be done using `Logger` from swift-log.
In your own log formatters, you can make use of the `swiftLogInfo` property on `DDLogMessage` to retrieve the details of a message that is logged via swift-log.
To use swift-log with CocoaLumberjack, take a look the following code snippet to see how to get started.
```swift
import CocoaLumberjack
import CocoaLumberjackSwiftLogBackend
import Logging
// In your application's entry point (e.g. AppDelegate):
DDLog.add(DDOSLogger.sharedInstance) // Configure loggers
LoggingSystem.bootstrapWithCocoaLumberjack() // Use CocoaLumberjack as swift-log backend
```
## More information
- read the [Getting started](Documentation/GettingStarted.md) guide, check out the [FAQ](Documentation/FAQ.md) section or the other [docs](Documentation/)
- if you find issues or want to suggest improvements, create an issue or a pull request
- for all kinds of questions involving CocoaLumberjack, use the [Google group](https://groups.google.com/group/cocoalumberjack) or StackOverflow (use [#lumberjack](https://stackoverflow.com/questions/tagged/lumberjack)).
## CocoaLumberjack 3
### Migrating to 3.x
* To be determined
## Features
### Lumberjack is Fast & Simple, yet Powerful & Flexible.
It is similar in concept to other popular logging frameworks such as log4j, yet is designed specifically for Objective-C, and takes advantage of features such as multi-threading, grand central dispatch (if available), lockless atomic operations, and the dynamic nature of the Objective-C runtime.
### Lumberjack is Fast
In most cases it is an order of magnitude faster than NSLog.
### Lumberjack is Simple
It takes as little as a single line of code to configure lumberjack when your application launches. Then simply replace your NSLog statements with DDLog statements and that's about it. (And the DDLog macros have the exact same format and syntax as NSLog, so it's super easy.)
### Lumberjack is Powerful:
One log statement can be sent to multiple loggers, meaning you can log to a file and the console simultaneously. Want more? Create your own loggers (it's easy) and send your log statements over the network. Or to a database or distributed file system. The sky is the limit.
### Lumberjack is Flexible:
Configure your logging however you want. Change log levels per file (perfect for debugging). Change log levels per logger (verbose console, but concise log file). Change log levels per xcode configuration (verbose debug, but concise release). Have your log statements compiled out of the release build. Customize the number of log levels for your application. Add your own fine-grained logg

冯汉栩
- 粉丝: 328
- 资源: 522
最新资源
- 基于redis全站抓取资料齐全+文档+源码.zip
- 基于pybullet和stable baseline3 的法奥机械臂的强化学习抓取训练代码资料齐全+文档+源码.zip
- 基于Redis实现的一套分布式定向抓取工程。资料齐全+文档+源码.zip
- 基于RSS订阅自动抓取文章生成站点,这是个实验性功能。资料齐全+文档+源码.zip
- 基于scrapy+selenium+phantomjs的爬虫程序,用于抓取多个学校的学术报告信息资料齐全+文档+源码.zip
- 基于scrapy的danbooru图片抓取工具资料齐全+文档+源码.zip
- 基于scrapy的上市公司信息抓取工具资料齐全+文档+源码.zip
- 基于Scrapy框架,用于抓取新浪微博数据,主要包括微博内容,评论以及用户信息资料齐全+文档+源码.zip
- 基于scrapy的时尚网站商品数据抓取资料齐全+文档+源码.zip
- 基于scrapy框架使用redis实现对shopee商城的增量抓取资料齐全+文档+源码.zip
- 基于Scrapy爬虫对某守望先锋网站数据的动态抓取资料齐全+文档+源码.zip
- 基于scrapy实现几大主流司法拍卖网站抓取资料齐全+文档+源码.zip
- 基于Selelium图片抓取资料齐全+文档+源码.zip
- 基于swoole扩展的爬虫,php多进程多线程抓取资料齐全+文档+源码.zip
- 基于Thinkphp5实现数据信息抓取、基于整理的API接口 + 招聘信息抓取(前程无忧智联招聘boss直聘拉勾网)数据接口 + 新闻分类(头条军事娱乐体
- FSCapture Ver. 8.9:屏幕截图与录制工具,图像编辑与快捷键支持,支持全屏、窗口、区域截图,滚动截图与视频录制,自动上传与FTP上传,适用于教学、设计、技术支持与文档制作
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


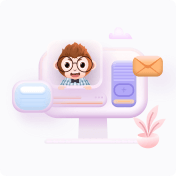