/****************************************************************************
**
** Copyright (C) 2014 Digia Plc and/or its subsidiary(-ies).
** Contact: http://www.qt-project.org/legal
**
** This file is part of the QtCore module of the Qt Toolkit.
**
** $QT_BEGIN_LICENSE:LGPL$
** Commercial License Usage
** Licensees holding valid commercial Qt licenses may use this file in
** accordance with the commercial license agreement provided with the
** Software or, alternatively, in accordance with the terms contained in
** a written agreement between you and Digia. For licensing terms and
** conditions see http://qt.digia.com/licensing. For further information
** use the contact form at http://qt.digia.com/contact-us.
**
** GNU Lesser General Public License Usage
** Alternatively, this file may be used under the terms of the GNU Lesser
** General Public License version 2.1 as published by the Free Software
** Foundation and appearing in the file LICENSE.LGPL included in the
** packaging of this file. Please review the following information to
** ensure the GNU Lesser General Public License version 2.1 requirements
** will be met: http://www.gnu.org/licenses/old-licenses/lgpl-2.1.html.
**
** In addition, as a special exception, Digia gives you certain additional
** rights. These rights are described in the Digia Qt LGPL Exception
** version 1.1, included in the file LGPL_EXCEPTION.txt in this package.
**
** GNU General Public License Usage
** Alternatively, this file may be used under the terms of the GNU
** General Public License version 3.0 as published by the Free Software
** Foundation and appearing in the file LICENSE.GPL included in the
** packaging of this file. Please review the following information to
** ensure the GNU General Public License version 3.0 requirements will be
** met: http://www.gnu.org/copyleft/gpl.html.
**
**
** $QT_END_LICENSE$
**
****************************************************************************/
#ifndef QGLOBAL_H
#define QGLOBAL_H
#include <stddef.h>
#define QT_VERSION_STR "4.8.6"
/*
QT_VERSION is (major << 16) + (minor << 8) + patch.
*/
#define QT_VERSION 0x040806
/*
can be used like #if (QT_VERSION >= QT_VERSION_CHECK(4, 4, 0))
*/
#define QT_VERSION_CHECK(major, minor, patch) ((major<<16)|(minor<<8)|(patch))
#define QT_PACKAGEDATE_STR "2014-04-10"
#define QT_PACKAGE_TAG "68a911862e05400ced87971c43fb27fb5d5d8ebd"
#if !defined(QT_BUILD_MOC)
#include <QtCore/qconfig.h>
#endif
#ifdef __cplusplus
#ifndef QT_NO_STL
#include <algorithm>
#endif
#ifndef QT_NAMESPACE /* user namespace */
# define QT_PREPEND_NAMESPACE(name) ::name
# define QT_USE_NAMESPACE
# define QT_BEGIN_NAMESPACE
# define QT_END_NAMESPACE
# define QT_BEGIN_INCLUDE_NAMESPACE
# define QT_END_INCLUDE_NAMESPACE
# define QT_BEGIN_MOC_NAMESPACE
# define QT_END_MOC_NAMESPACE
# define QT_FORWARD_DECLARE_CLASS(name) class name;
# define QT_FORWARD_DECLARE_STRUCT(name) struct name;
# define QT_MANGLE_NAMESPACE(name) name
#else /* user namespace */
# define QT_PREPEND_NAMESPACE(name) ::QT_NAMESPACE::name
# define QT_USE_NAMESPACE using namespace ::QT_NAMESPACE;
# define QT_BEGIN_NAMESPACE namespace QT_NAMESPACE {
# define QT_END_NAMESPACE }
# define QT_BEGIN_INCLUDE_NAMESPACE }
# define QT_END_INCLUDE_NAMESPACE namespace QT_NAMESPACE {
# define QT_BEGIN_MOC_NAMESPACE QT_USE_NAMESPACE
# define QT_END_MOC_NAMESPACE
# define QT_FORWARD_DECLARE_CLASS(name) \
QT_BEGIN_NAMESPACE class name; QT_END_NAMESPACE \
using QT_PREPEND_NAMESPACE(name);
# define QT_FORWARD_DECLARE_STRUCT(name) \
QT_BEGIN_NAMESPACE struct name; QT_END_NAMESPACE \
using QT_PREPEND_NAMESPACE(name);
# define QT_MANGLE_NAMESPACE0(x) x
# define QT_MANGLE_NAMESPACE1(a, b) a##_##b
# define QT_MANGLE_NAMESPACE2(a, b) QT_MANGLE_NAMESPACE1(a,b)
# define QT_MANGLE_NAMESPACE(name) QT_MANGLE_NAMESPACE2( \
QT_MANGLE_NAMESPACE0(name), QT_MANGLE_NAMESPACE0(QT_NAMESPACE))
namespace QT_NAMESPACE {}
# ifndef QT_BOOTSTRAPPED
# ifndef QT_NO_USING_NAMESPACE
/*
This expands to a "using QT_NAMESPACE" also in _header files_.
It is the only way the feature can be used without too much
pain, but if people _really_ do not want it they can add
DEFINES += QT_NO_USING_NAMESPACE to their .pro files.
*/
QT_USE_NAMESPACE
# endif
# endif
#endif /* user namespace */
#else /* __cplusplus */
# define QT_BEGIN_NAMESPACE
# define QT_END_NAMESPACE
# define QT_USE_NAMESPACE
# define QT_BEGIN_INCLUDE_NAMESPACE
# define QT_END_INCLUDE_NAMESPACE
#endif /* __cplusplus */
#if defined(Q_OS_MAC) && !defined(Q_CC_INTEL)
#define QT_BEGIN_HEADER extern "C++" {
#define QT_END_HEADER }
#define QT_BEGIN_INCLUDE_HEADER }
#define QT_END_INCLUDE_HEADER extern "C++" {
#else
#define QT_BEGIN_HEADER
#define QT_END_HEADER
#define QT_BEGIN_INCLUDE_HEADER
#define QT_END_INCLUDE_HEADER extern "C++"
#endif
/*
The operating system, must be one of: (Q_OS_x)
DARWIN - Darwin OS (synonym for Q_OS_MAC)
SYMBIAN - Symbian
MSDOS - MS-DOS and Windows
OS2 - OS/2
OS2EMX - XFree86 on OS/2 (not PM)
WIN32 - Win32 (Windows 2000/XP/Vista/7 and Windows Server 2003/2008)
WINCE - WinCE (Windows CE 5.0)
CYGWIN - Cygwin
SOLARIS - Sun Solaris
HPUX - HP-UX
ULTRIX - DEC Ultrix
LINUX - Linux
FREEBSD - FreeBSD
NETBSD - NetBSD
OPENBSD - OpenBSD
BSDI - BSD/OS
IRIX - SGI Irix
OSF - HP Tru64 UNIX
SCO - SCO OpenServer 5
UNIXWARE - UnixWare 7, Open UNIX 8
AIX - AIX
HURD - GNU Hurd
DGUX - DG/UX
RELIANT - Reliant UNIX
DYNIX - DYNIX/ptx
QNX - QNX
LYNX - LynxOS
BSD4 - Any BSD 4.4 system
UNIX - Any UNIX BSD/SYSV system
*/
#if defined(__APPLE__) && (defined(__GNUC__) || defined(__xlC__) || defined(__xlc__))
# define Q_OS_DARWIN
# define Q_OS_BSD4
# ifdef __LP64__
# define Q_OS_DARWIN64
# else
# define Q_OS_DARWIN32
# endif
#elif defined(__SYMBIAN32__) || defined(SYMBIAN)
# define Q_OS_SYMBIAN
# define Q_NO_POSIX_SIGNALS
# define QT_NO_GETIFADDRS
#elif defined(__CYGWIN__)
# define Q_OS_CYGWIN
#elif defined(MSDOS) || defined(_MSDOS)
# define Q_OS_MSDOS
#elif defined(__OS2__)
# if defined(__EMX__)
# define Q_OS_OS2EMX
# else
# define Q_OS_OS2
# endif
#elif !defined(SAG_COM) && (defined(WIN64) || defined(_WIN64) || defined(__WIN64__))
# define Q_OS_WIN32
# define Q_OS_WIN64
#elif !defined(SAG_COM) && (defined(WIN32) || defined(_WIN32) || defined(__WIN32__) || defined(__NT__))
# if defined(WINCE) || defined(_WIN32_WCE)
# define Q_OS_WINCE
# else
# define Q_OS_WIN32
# endif
#elif defined(__MWERKS__) && defined(__INTEL__)
# define Q_OS_WIN32
#elif defined(__sun) || defined(sun)
# define Q_OS_SOLARIS
#elif defined(hpux) || defined(__hpux)
# define Q_OS_HPUX
#elif defined(__ultrix) || defined(ultrix)
# define Q_OS_ULTRIX
#elif defined(sinix)
# define Q_OS_RELIANT
#elif defined(__native_client__)
# define Q_OS_NACL
#elif defined(__linux__) || defined(__linux)
# define Q_OS_LINUX
#elif defined(__FreeBSD__) || defined(__DragonFly__)
# define Q_OS_FREEBSD
# define Q_OS_BSD4
#elif defined(__NetBSD__)
# define Q_OS_NETBSD
# define Q_OS_BSD4
#elif defined(__OpenBSD__)
# define Q_OS_OPENBSD
# define Q_OS_BSD4
#elif defined(__bsdi__)
# define Q_OS_BSDI
# define Q_OS_BSD4
#elif defined(__sgi)
# define Q_OS_IRIX
#elif defined(__osf__)
# define Q_OS_OSF
#elif defined(_AIX)
# define Q_OS_AIX
#elif defined(__Lynx__)
# define Q_OS_LYNX
#elif defined(__GNU__)
# define Q_OS_HURD
#elif defined(__DGUX__)
# define Q_OS_DGUX
#elif defined(__QNXNTO__)
# define Q_OS_QNX
#elif defined(_SEQUENT_)
# define Q_OS_DYNIX
#elif defined(_SCO_DS) /* SCO OpenServer 5 + GCC */
# define Q_OS_SCO
#elif defined(__USLC__) /* all SCO platforms + UDK or OUDK */
# define Q_OS_UNIXWARE
#elif defined(__svr4__) && defined(i386) /* Open UNIX 8 + GCC */
# define Q_OS_UNIXWARE
#elif
没有合适的资源?快使用搜索试试~ 我知道了~
qt4.8.6资源,用户qt安装,编译与学习
需积分: 0 0 下载量 94 浏览量
2024-12-26
10:51:53
上传
评论
收藏 45.05MB GZ 举报
温馨提示
qt4.8.6资源,用户qt安装,编译与学习
资源推荐
资源详情
资源评论
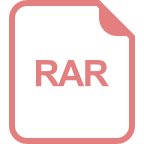
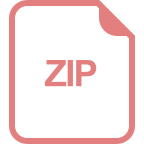
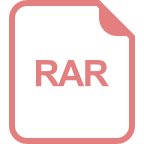
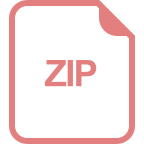
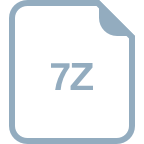
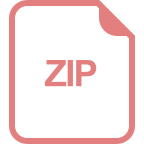
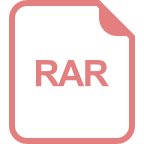
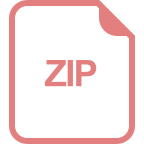
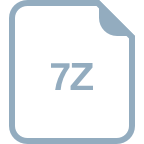
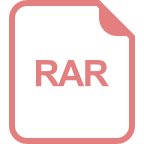
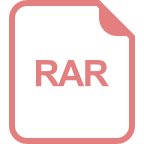
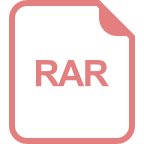
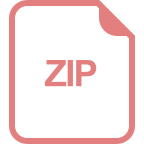
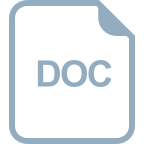
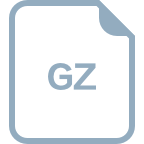
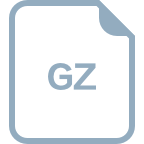
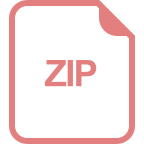
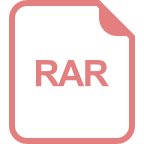
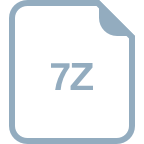
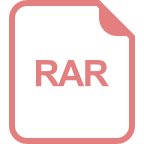
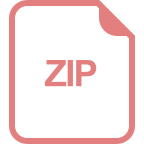
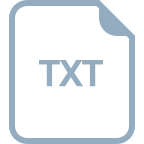
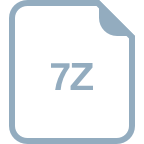
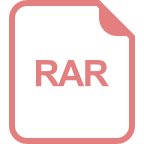
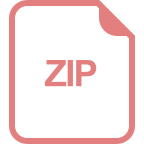
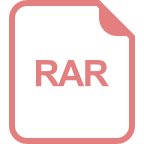
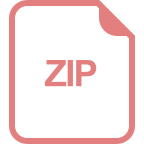
收起资源包目录

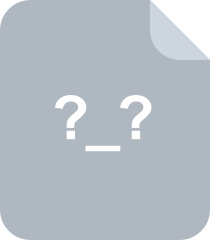
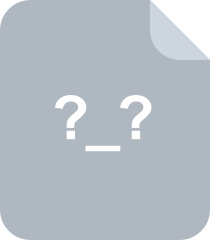
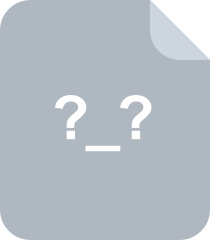
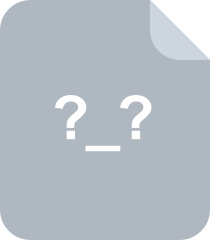
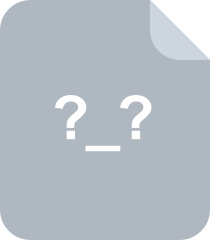
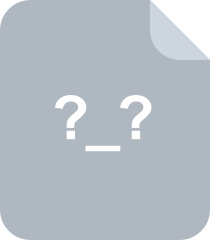
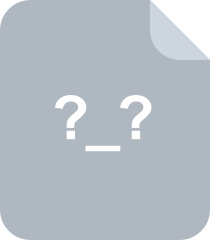
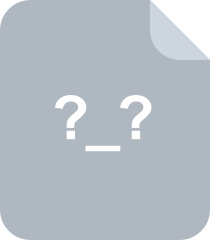
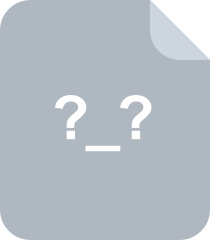
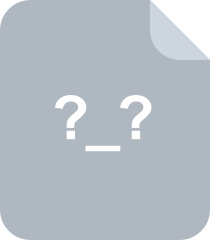
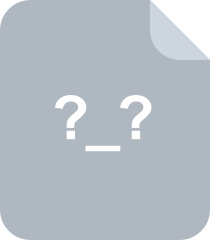
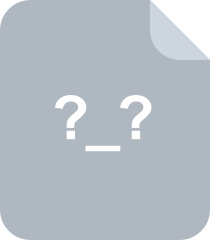
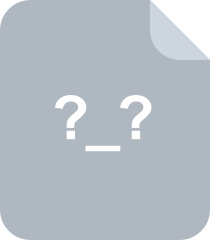
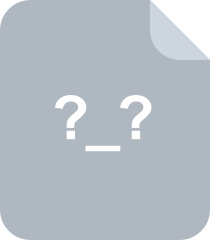
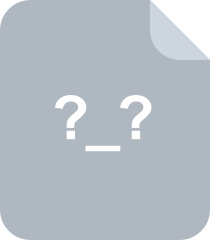
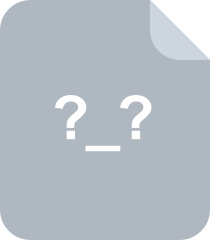
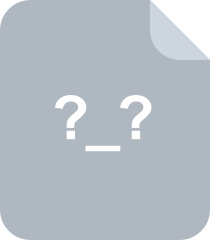
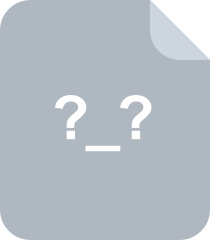
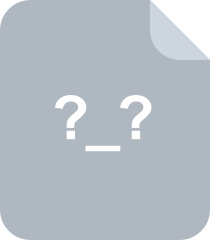
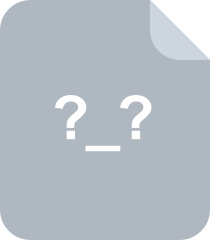
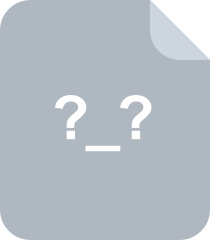
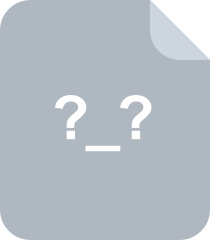
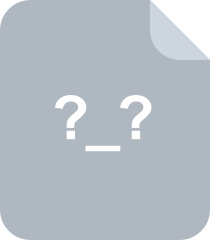
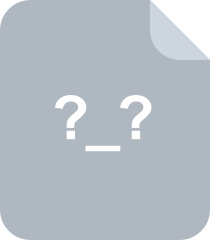
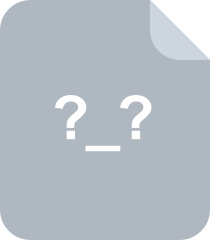
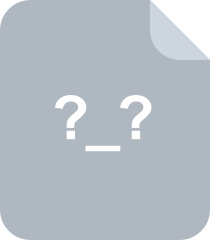
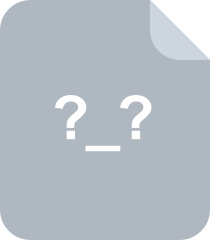
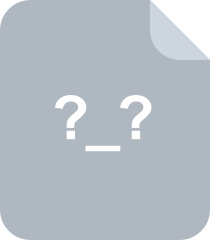
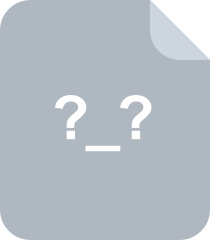
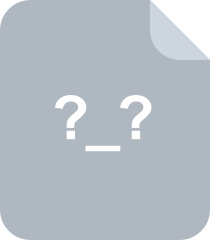
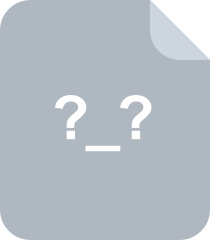
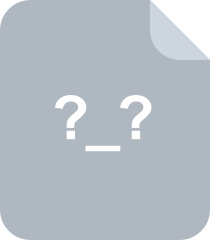
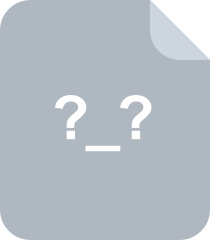
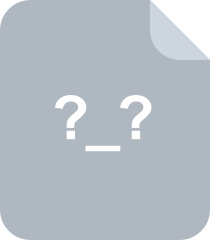
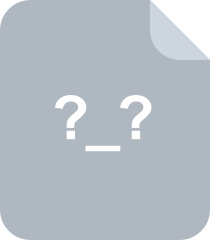
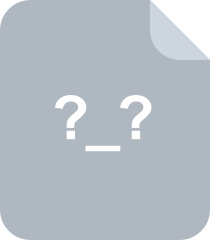
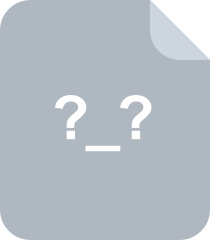
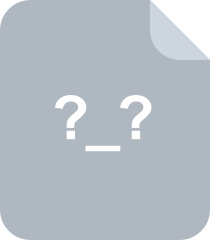
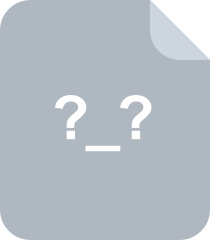
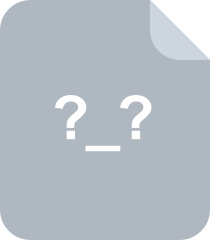
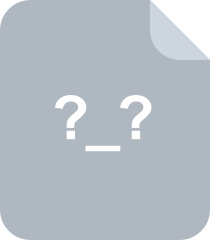
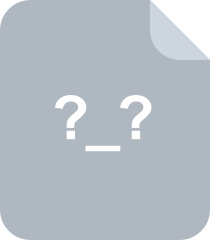
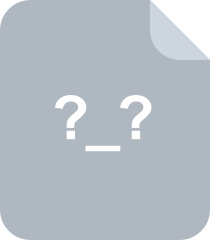
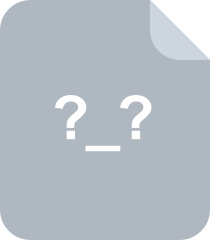
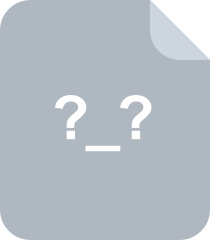
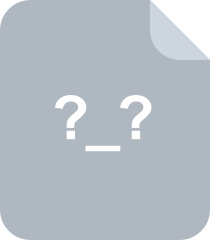
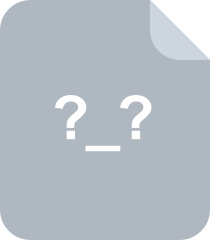
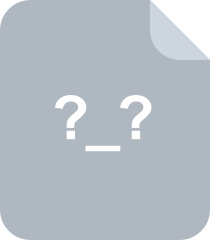
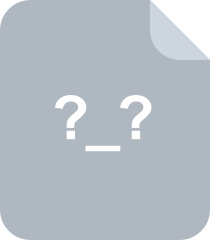
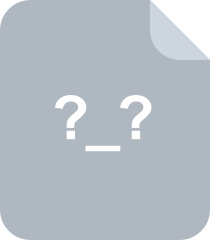
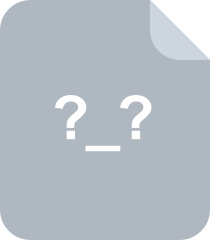
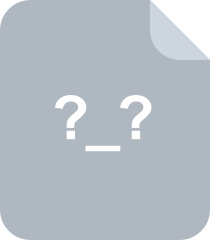
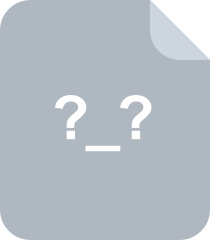
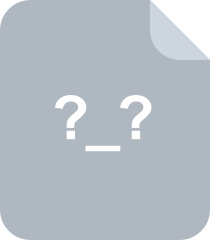
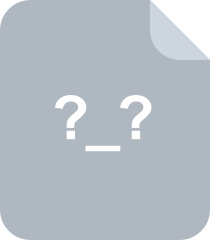
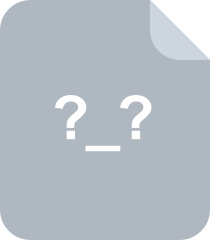
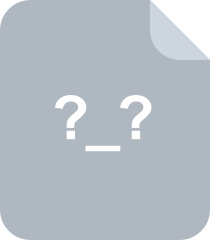
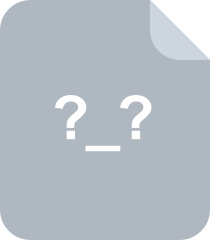
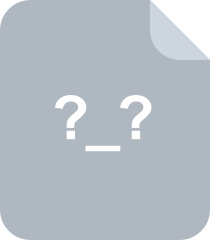
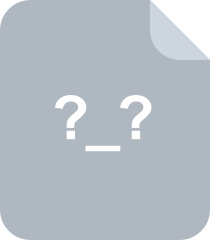
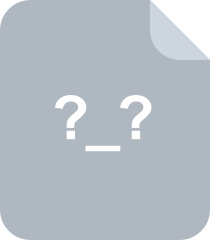
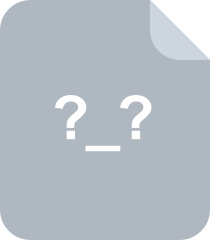
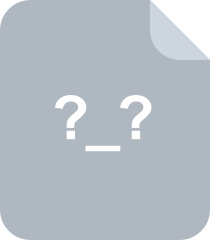
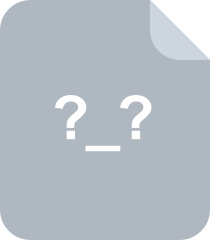
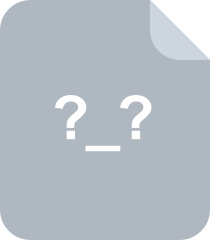
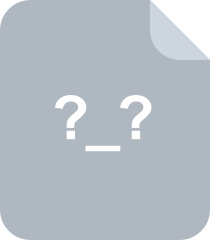
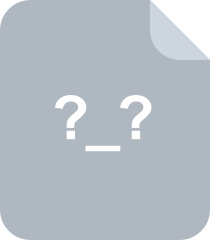
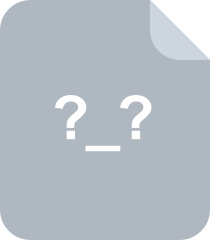
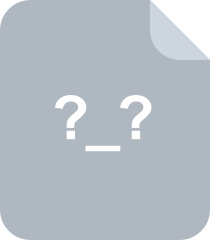
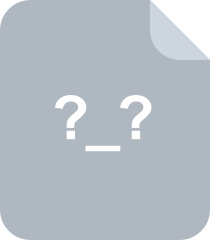
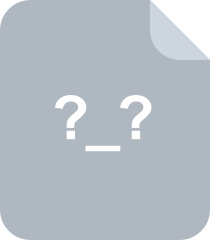
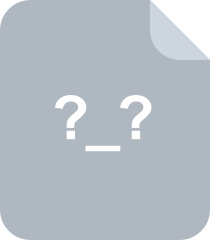
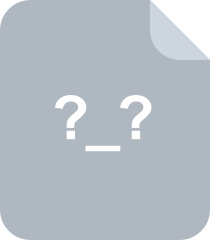
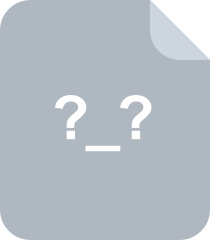
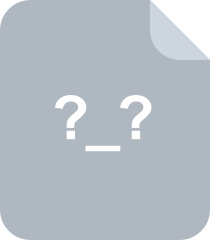
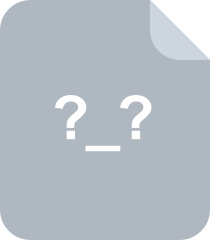
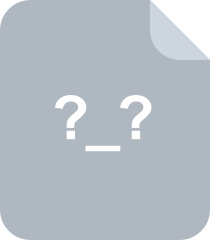
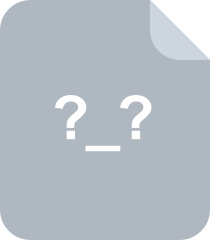
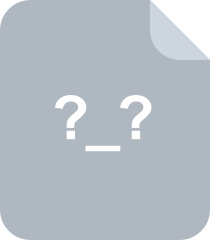
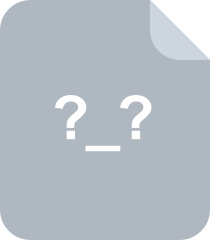
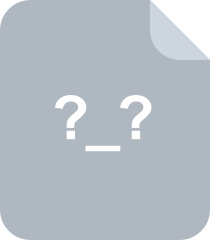
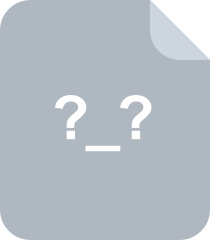
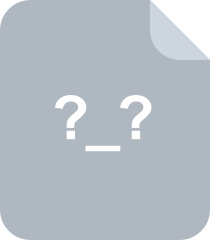
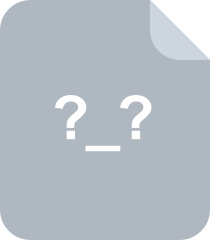
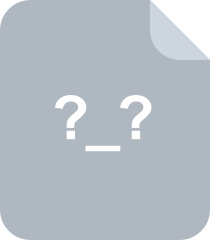
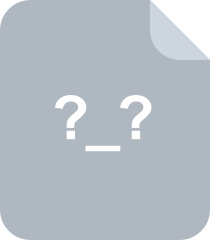
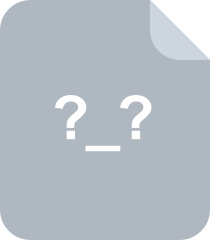
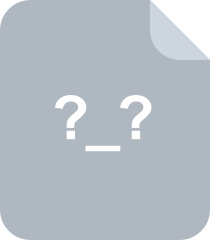
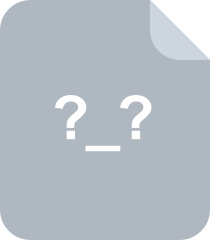
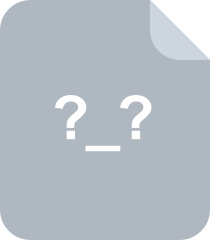
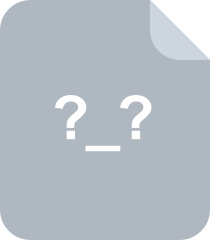
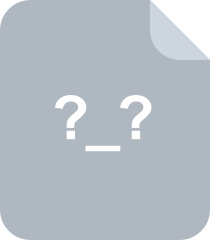
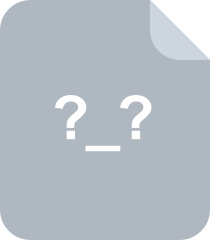
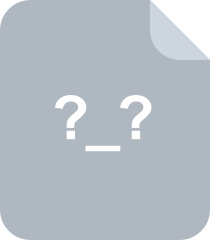
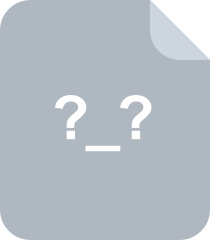
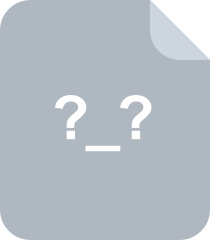
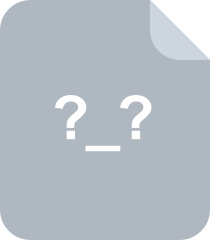
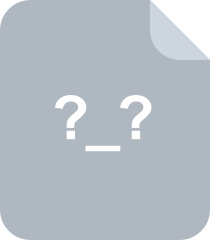
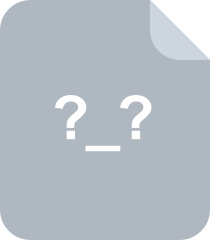
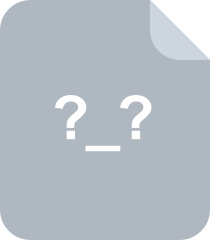
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
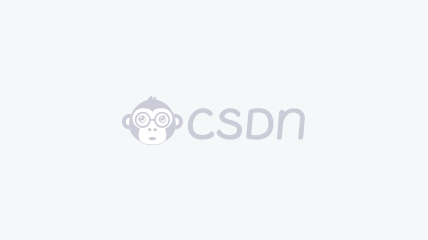


software4y
- 粉丝: 2
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

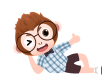
最新资源
- CTF - Misc - 图片隐写 - 002-dog
- 包含思科 Nexus 交换机全系列的命名规则,以及相关板卡、交换矩阵的命名细节
- QtScrcpy-win-x64-v3.0.1.zip
- MobaXterm-Portable-v24 是一款多功能远程管理工具,支持SSH、X11、SFTP等协议,适用于Windows、Linux和Unix系统
- 定子组装机自动摆料版3D图纸和工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 屏幕磁铁组装检测设备工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 毕设-struts+hibernate实现的网络购物系统
- 富士施乐s1810维修手册:故障诊断与维护标准流程(只有第一到第三章)
- visio:华为、戴尔、惠普服务器模具下载
- c&c++课程设计KTV歌曲系统,学生档案管理系统,个人收支系统,职工管理系统等.zip
- c&c++课程设计-学生成绩管理系统.zip
- 10个数据结构课程设计实例二叉树建立遍历冒泡排序快速排序等.zip
- 毕设-期刊信息管理系统(SQL).zip
- 毕设-基于PHP的图片共享系统的设计与实现.zip
- visio:华为、戴尔、惠普服务器模具下载
- visio:华为、戴尔、惠普服务器模具下载
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


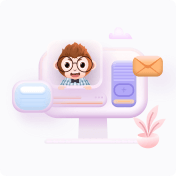
安全验证
文档复制为VIP权益,开通VIP直接复制
