CMAKE_MINIMUM_REQUIRED (VERSION 3.1.0)
INCLUDE (CheckIncludeFiles)
INCLUDE (CheckTypeSize)
INCLUDE (CheckFunctionExists)
INCLUDE (CheckSymbolExists)
INCLUDE (CheckCSourceCompiles)
INCLUDE (CheckStructHasMember)
INCLUDE (CheckLibraryExists)
INCLUDE (GNUInstallDirs)
PROJECT (c-ares C)
# Set this version before release
SET (CARES_VERSION "1.15.0")
# This is for libtool compatibility, and specified in a form that is easily
# translatable from libtool (even if the actual form doesn't make sense).
# For instance, in an autotools project, in Makefile.am there is a line that
# contains something like:
# -version-info 4:0:2
# This breaks down into sections of current:revision:age
# This then generates a version of "(current-age).age.revision" with an
# interface version of "(current-age)"
# For example, a version of 4:0:2 would generate output such as:
# libname.so -> libname.so.2
# libname.so.2 -> libname.so.2.2.0
SET (CARES_LIB_VERSIONINFO "5:0:3")
OPTION (CARES_STATIC "Build as a static library" OFF)
OPTION (CARES_SHARED "Build as a shared library" ON)
OPTION (CARES_INSTALL "Create installation targets (chain builders may want to disable this)" ON)
OPTION (CARES_STATIC_PIC "Build the static library as PIC (position independent)" OFF)
OPTION (CARES_BUILD_TESTS "Build and run tests" OFF)
OPTION (CARES_BUILD_TOOLS "Build tools" ON)
# allow linking against the static runtime library in msvc
IF (MSVC)
OPTION (CARES_MSVC_STATIC_RUNTIME "Link against the static runtime library" OFF)
IF (CARES_MSVC_STATIC_RUNTIME)
# CMAKE_CONFIGURATION_TYPES is empty on non-IDE generators (Ninja, NMake)
# and that's why we also use CMAKE_BUILD_TYPE to cover for those generators.
# For IDE generators, CMAKE_BUILD_TYPE is usually empty
FOREACH (config_type ${CMAKE_CONFIGURATION_TYPES} ${CMAKE_BUILD_TYPE})
STRING (TOUPPER ${config_type} upper_config_type)
SET (flag_var "CMAKE_C_FLAGS_${upper_config_type}")
IF (${flag_var} MATCHES "/MD")
STRING (REGEX REPLACE "/MD" "/MT" ${flag_var} "${${flag_var}}")
ENDIF ()
ENDFOREACH ()
# clean up
SET (upper_config_type)
SET (config_type)
SET (flag_var)
ENDIF ()
ENDIF ()
# Keep build organized.
SET (CMAKE_RUNTIME_OUTPUT_DIRECTORY ${PROJECT_BINARY_DIR}/bin)
SET (CMAKE_LIBRARY_OUTPUT_DIRECTORY ${PROJECT_BINARY_DIR}/lib)
SET (CMAKE_ARCHIVE_OUTPUT_DIRECTORY ${PROJECT_BINARY_DIR}/lib)
SET (PACKAGE_DIRECTORY ${PROJECT_BINARY_DIR}/package)
# Destinations for installing different kinds of targets (pass to install command).
SET (TARGETS_INST_DEST
RUNTIME DESTINATION ${CMAKE_INSTALL_BINDIR}
LIBRARY DESTINATION ${CMAKE_INSTALL_LIBDIR}
ARCHIVE DESTINATION ${CMAKE_INSTALL_LIBDIR}
INCLUDES DESTINATION ${CMAKE_INSTALL_INCLUDEDIR}
)
# Look for dependent/required libraries
CHECK_LIBRARY_EXISTS (resolv res_servicename "" HAVE_RES_SERVICENAME_IN_LIBRESOLV)
IF (HAVE_RES_SERVICENAME_IN_LIBRESOLV)
SET (HAVE_LIBRESOLV 1)
ENDIF ()
IF (APPLE)
CHECK_C_SOURCE_COMPILES ("
#include <stdio.h>
#include <TargetConditionals.h>
int main() {
#if TARGET_OS_IPHONE == 0
#error Not an iPhone target
#endif
return 0;
}
"
IOS)
CHECK_C_SOURCE_COMPILES ("
#include <stdio.h>
#include <TargetConditionals.h>
int main() {
#if TARGET_OS_IPHONE == 0 || __IPHONE_OS_VERSION_MIN_REQUIRED < 100000
# error Not iOS v10
#endif
return 0;
}
"
IOS_V10)
CHECK_C_SOURCE_COMPILES ("
#include <stdio.h>
#include <AvailabilityMacros.h>
#ifndef MAC_OS_X_VERSION_10_12
# define MAC_OS_X_VERSION_10_12 101200
#endif
int main() {
#if MAC_OS_X_VERSION_MIN_REQUIRED < MAC_OS_X_VERSION_10_12
# error Not MacOSX 10.12 or higher
#endif
return 0;
}
"
MACOS_V1012)
ENDIF ()
IF (IOS AND HAVE_LIBRESOLV)
SET (CARES_USE_LIBRESOLV 1)
ENDIF()
CHECK_LIBRARY_EXISTS (nsl gethostbyname "" HAVE_LIBNSL)
CHECK_LIBRARY_EXISTS (socket gethostbyname "" HAVE_GHBN_LIBSOCKET)
CHECK_LIBRARY_EXISTS (socket socket "" HAVE_SOCKET_LIBSOCKET)
IF (HAVE_GHBN_LIBSOCKET OR HAVE_SOCKET_LIBSOCKET)
SET(HAVE_LIBSOCKET TRUE)
ENDIF ()
CHECK_LIBRARY_EXISTS (rt clock_gettime "" HAVE_LIBRT)
# Look for necessary includes
CHECK_INCLUDE_FILES (sys/types.h HAVE_SYS_TYPES_H)
CHECK_INCLUDE_FILES (sys/socket.h HAVE_SYS_SOCKET_H)
CHECK_INCLUDE_FILES (arpa/inet.h HAVE_ARPA_INET_H)
CHECK_INCLUDE_FILES (arpa/nameser_compat.h HAVE_ARPA_NAMESER_COMPAT_H)
CHECK_INCLUDE_FILES (arpa/nameser.h HAVE_ARPA_NAMESER_H)
CHECK_INCLUDE_FILES (assert.h HAVE_ASSERT_H)
CHECK_INCLUDE_FILES (errno.h HAVE_ERRNO_H)
CHECK_INCLUDE_FILES (fcntl.h HAVE_FCNTL_H)
CHECK_INCLUDE_FILES (inttypes.h HAVE_INTTYPES_H)
CHECK_INCLUDE_FILES (limits.h HAVE_LIMITS_H)
CHECK_INCLUDE_FILES (malloc.h HAVE_MALLOC_H)
CHECK_INCLUDE_FILES (memory.h HAVE_MEMORY_H)
CHECK_INCLUDE_FILES (netdb.h HAVE_NETDB_H)
CHECK_INCLUDE_FILES (netinet/in.h HAVE_NETINET_IN_H)
CHECK_INCLUDE_FILES (netinet/tcp.h HAVE_NETINET_TCP_H)
CHECK_INCLUDE_FILES (net/if.h HAVE_NET_IF_H)
CHECK_INCLUDE_FILES (signal.h HAVE_SIGNAL_H)
CHECK_INCLUDE_FILES (socket.h HAVE_SOCKET_H)
CHECK_INCLUDE_FILES (stdbool.h HAVE_STDBOOL_H)
CHECK_INCLUDE_FILES (stdint.h HAVE_STDINT_H)
CHECK_INCLUDE_FILES (stdlib.h HAVE_STDLIB_H)
CHECK_INCLUDE_FILES (strings.h HAVE_STRINGS_H)
CHECK_INCLUDE_FILES (string.h HAVE_STRING_H)
CHECK_INCLUDE_FILES (stropts.h HAVE_STROPTS_H)
CHECK_INCLUDE_FILES (sys/ioctl.h HAVE_SYS_IOCTL_H)
CHECK_INCLUDE_FILES (sys/param.h HAVE_SYS_PARAM_H)
CHECK_INCLUDE_FILES (sys/select.h HAVE_SYS_SELECT_H)
CHECK_INCLUDE_FILES (sys/socket.h HAVE_SYS_SOCKET_H)
CHECK_INCLUDE_FILES (sys/stat.h HAVE_SYS_STAT_H)
CHECK_INCLUDE_FILES (sys/time.h HAVE_SYS_TIME_H)
CHECK_INCLUDE_FILES (sys/types.h HAVE_SYS_TYPES_H)
CHECK_INCLUDE_FILES (sys/uio.h HAVE_SYS_UIO_H)
CHECK_INCLUDE_FILES (time.h HAVE_TIME_H)
CHECK_INCLUDE_FILES (dlfcn.h HAVE_DLFCN_H)
CHECK_INCLUDE_FILES (unistd.h HAVE_UNISTD_H)
# Include order matters for these windows files.
CHECK_INCLUDE_FILES ("winsock2.h;windows.h" HAVE_WINSOCK2_H)
CHECK_INCLUDE_FILES ("winsock2.h;ws2tcpip.h;windows.h" HAVE_WS2TCPIP_H)
CHECK_INCLUDE_FILES ("winsock.h;windows.h" HAVE_WINSOCK_H)
CHECK_INCLUDE_FILES (windows.h HAVE_WINDOWS_H)
# Set system-specific compiler flags
IF (CMAKE_SYSTEM_NAME STREQUAL "Darwin")
LIST (APPEND SYSFLAGS -D_DARWIN_C_SOURCE)
ELSEIF (CMAKE_SYSTEM_NAME STREQUAL "Linux")
LIST (APPEND SYSFLAGS -D_GNU_SOURCE -D_POSIX_C_SOURCE=199309L -D_XOPEN_SOURCE=600)
ELSEIF (CMAKE_SYSTEM_NAME STREQUAL "SunOS")
LIST (APPEND SYSFLAGS -D__EXTENSIONS__ -D_REENTRANT -D_XOPEN_SOURCE=600)
ELSEIF (CMAKE_SYSTEM_NAME STREQUAL "AIX")
LIST (APPEND SYSFLAGS -D_ALL_SOURCE -D_XOPEN_SOURCE=600 -D_USE_IRS)
ELSEIF (CMAKE_SYSTEM_NAME STREQUAL "FreeBSD")
# Don't define _XOPEN_SOURCE on FreeBSD, it actually reduces visibility instead of increasing it
ELSEIF (WIN32)
LIST (APPEND SYSFLAGS -DWIN32_LEAN_AND_MEAN -D_CRT_SECURE_NO_DEPRECATE -D_CRT_NONSTDC_NO_DEPRECATE -D_WIN32_WINNT=0x0600)
ENDIF ()
ADD_DEFINITIONS(${SYSFLAGS})
# Tell C-Ares about libraries to depend on
IF (HAVE_LIBRESOLV)
LIST (APPEND CARES_DEPENDENT_LIBS resolv)
ENDIF ()
IF (HAVE_LIBNSL)
LIST (APPEND CARES_DEPENDENT_LIBS nsl)
ENDIF ()
IF (HAVE_LIBSOCKET)
LIST (APPEND CARES_DEPENDENT_LIBS socket)
ENDIF ()
IF (HAVE_LIBRT)
LIST (APPEND CARES_DEPENDENT_LIBS rt)
ENDIF ()
IF (WIN32)
LIST (APPEND CARES_DEPENDENT_LIBS ws2_32)
ENDIF ()
# When checking for symbols, we need to make sure we set the proper
# headers, libraries, and d
没有合适的资源?快使用搜索试试~ 我知道了~
使用eXosip+ffmpeg、ffplay命令行实现sip客户端
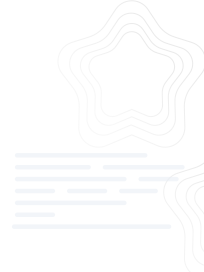
共789个文件
c:158个
h:150个
3:55个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
使用sip做视频通话时,会遇到需要使用ip摄像头作为视频源的情况,查了资料使用pjsip通常也需要改源码。pjsip包含的功能很完整,但有点过于庞大,很多功能并不需要。而且笔者有一个想法,只要有个能处理sip交互的库比如eXosip,音视频这块另外实现,比如先使用ffmpeg和ffplay命令行作为音视频测试,成功后再写代码实现。本文就是测试成功的方案,真正灵活的方式还是要写代码调ffmpeg,本文更多的是提供一种实现思路。本资源为文章附件资源,原文链接:https://blog.csdn.net/u013113678/article/details/132126069
资源推荐
资源详情
资源评论
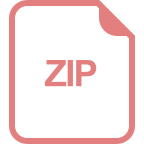
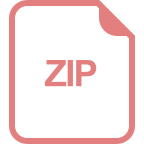
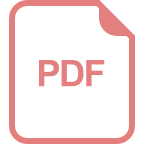
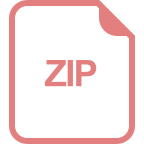
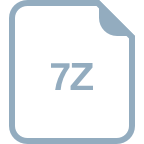
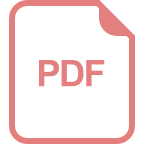
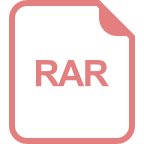
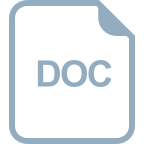
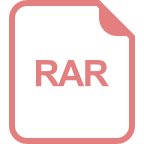
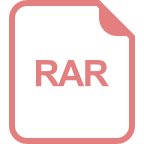
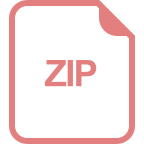
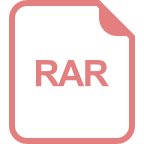
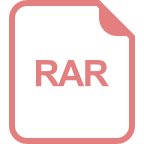
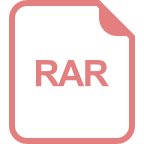
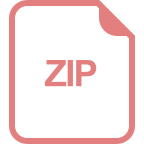
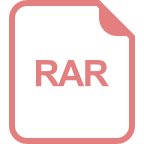
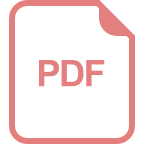
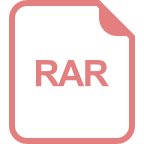
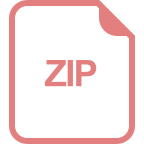
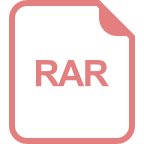
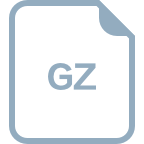
收起资源包目录

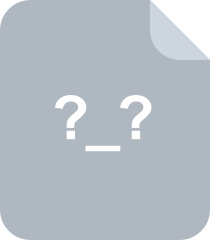
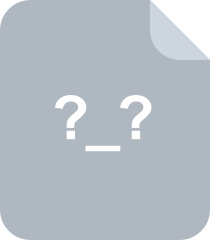
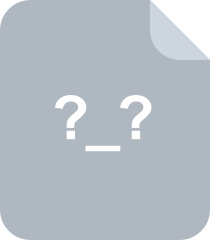
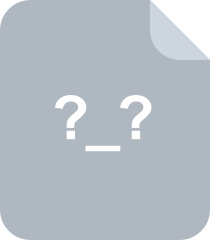
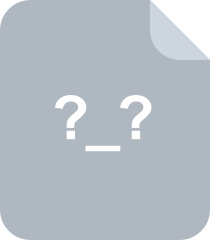
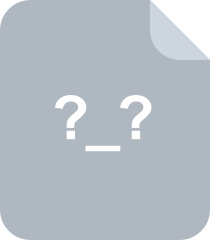
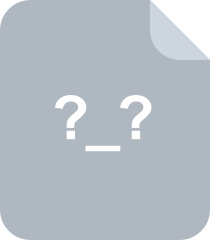
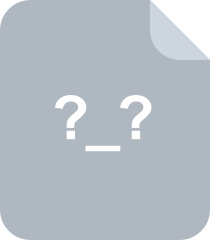
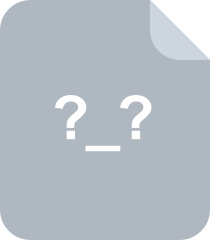
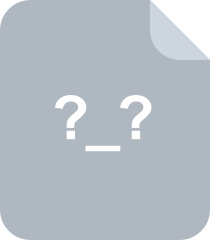
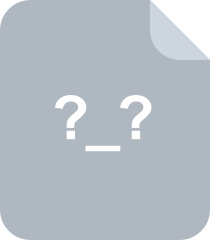
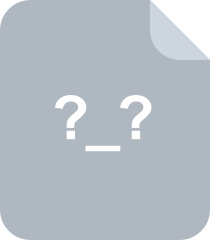
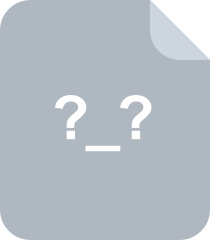
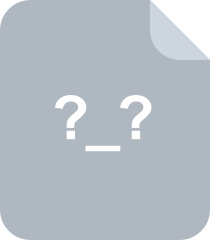
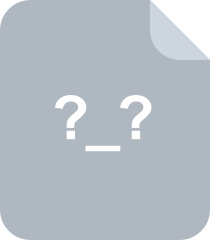
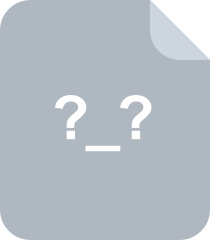
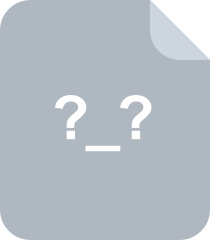
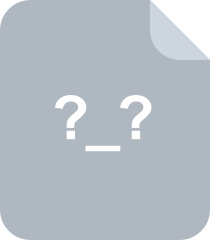
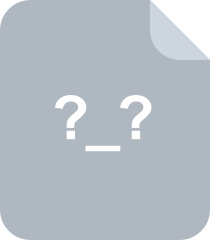
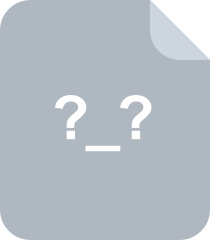
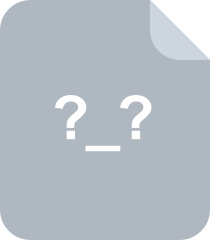
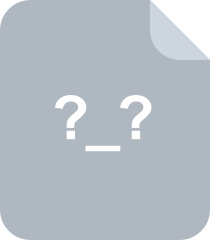
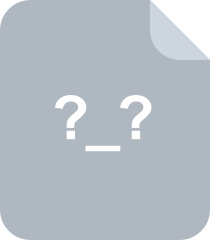
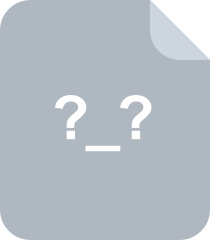
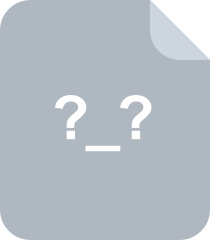
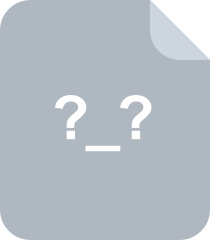
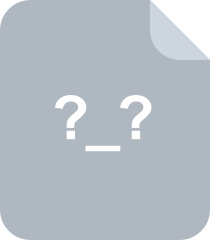
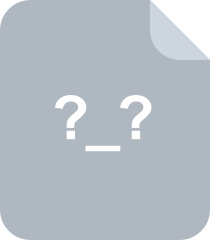
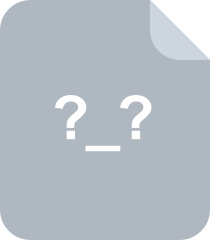
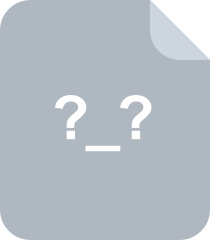
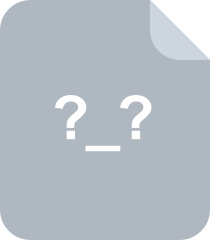
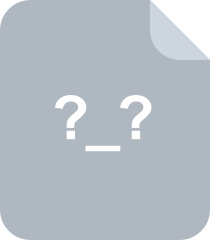
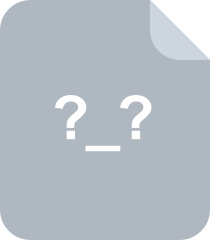
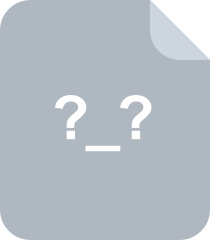
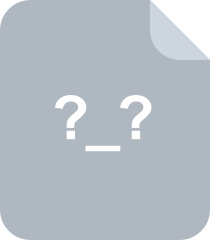
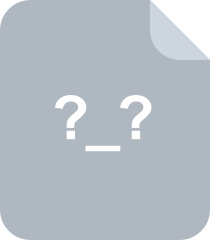
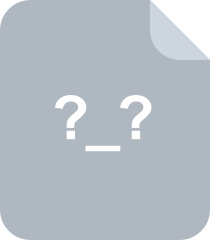
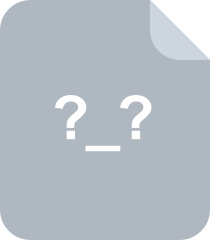
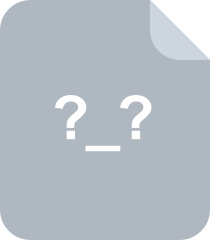
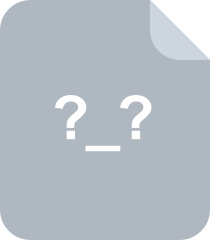
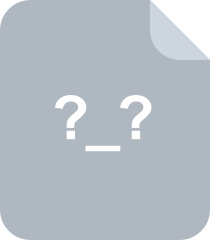
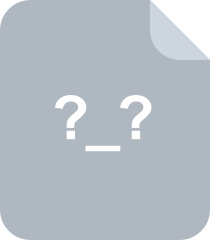
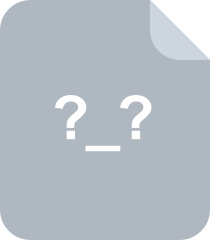
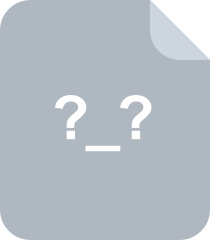
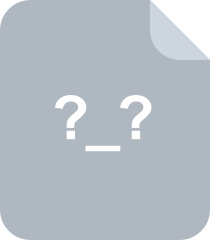
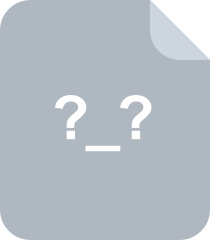
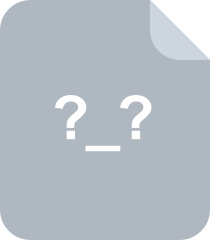
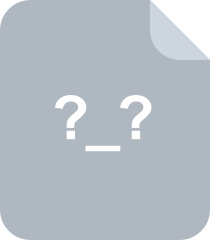
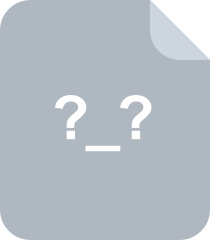
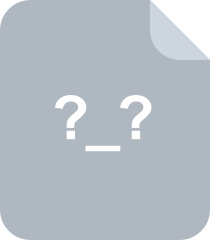
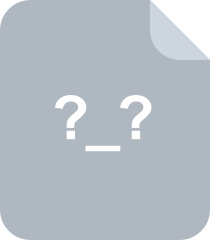
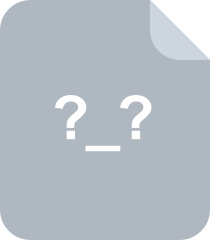
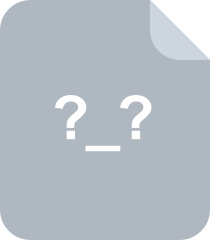
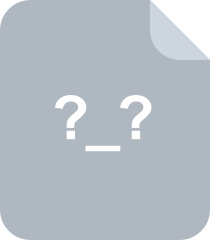
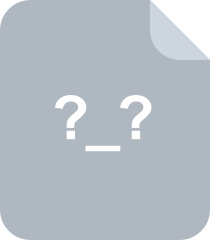
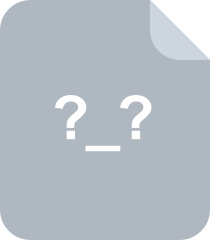
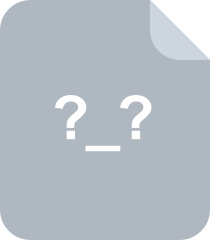
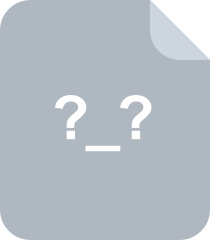
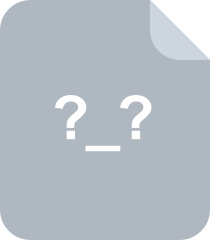
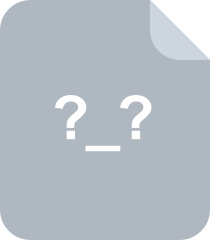
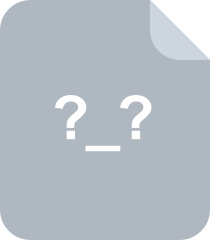
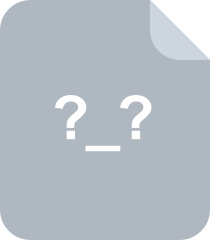
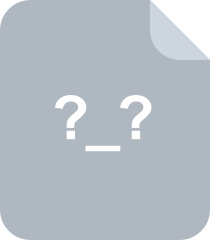
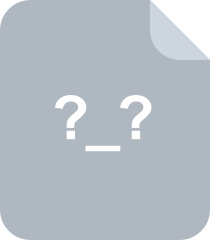
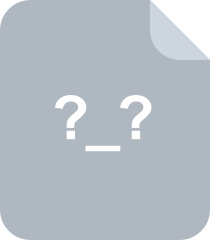
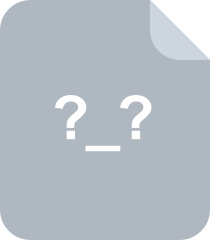
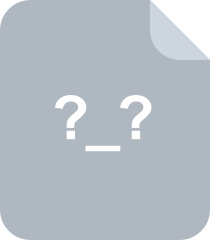
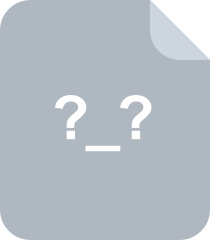
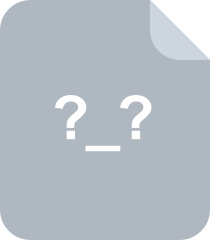
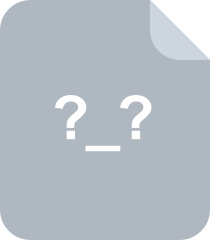
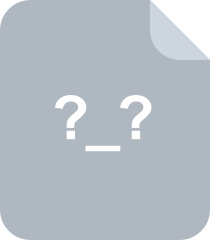
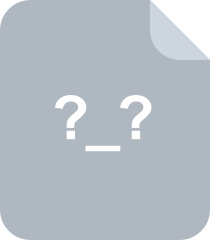
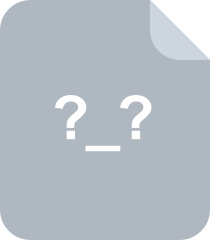
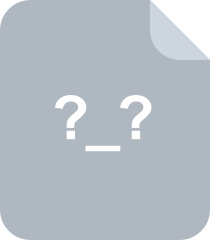
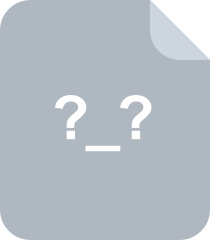
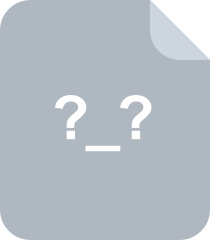
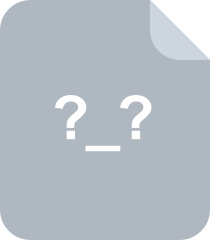
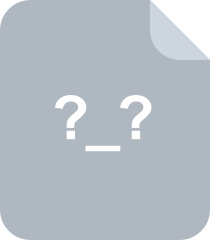
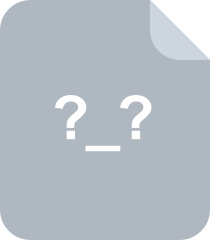
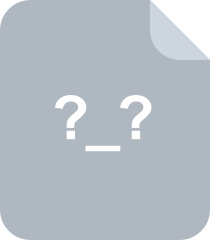
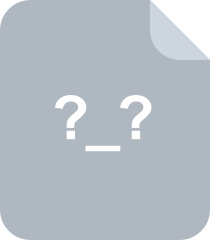
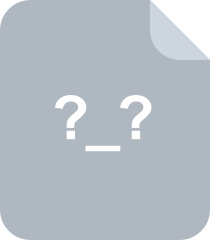
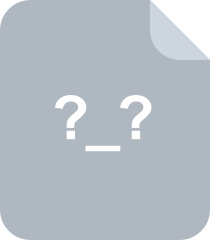
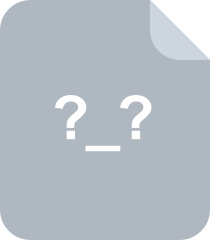
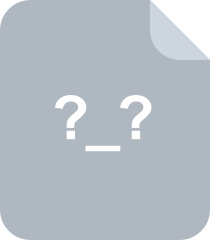
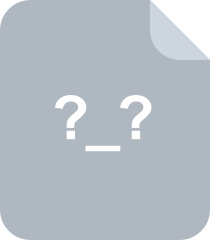
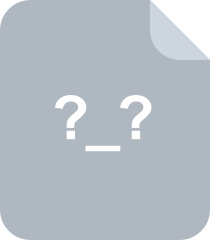
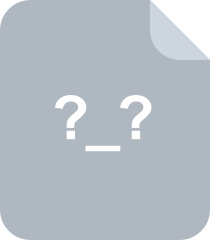
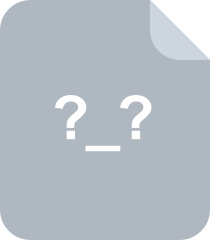
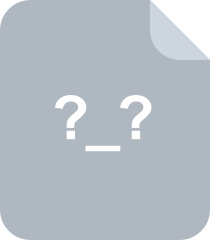
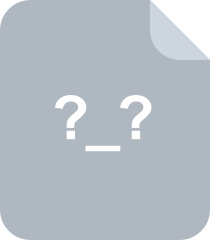
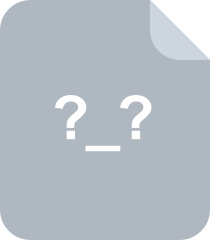
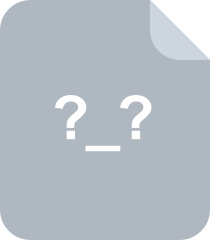
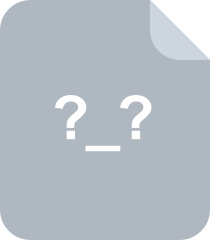
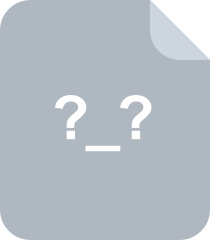
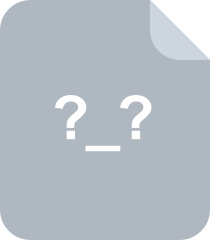
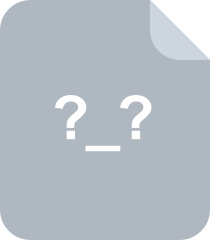
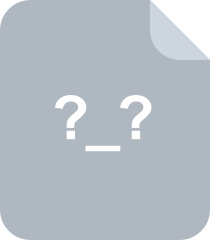
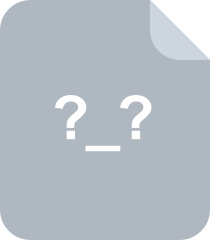
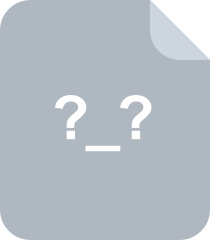
共 789 条
- 1
- 2
- 3
- 4
- 5
- 6
- 8


CodeOfCC
- 粉丝: 666
- 资源: 71
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

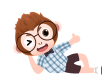
最新资源
- 美宝莲郑州国贸360店图纸增加灯片完稿.rar
- 基于C++实现的Hough Forests算法用于人体动作识别检测(提供了可视化功能).zip
- this is a GPU word
- 成都金楠天街活动包店.rar
- 【cocos creator】下拉框
- 基于pytorch实现3D ResNet网络的视频动作分类项目源码+运行说明+模型(支持得分模式和特征模式).zip
- 360国贸纽约城市&女神像.rar
- 更新城市蔓延指数数据集(1990-2023年).xlsx
- 动作识别基于PyTorch的3D ResNets模型实现的动作识别任务+运行说明(含训练、微调和测试、在UCF-101和HMDB-51等多数据集训练).zip
- datafor3dgs
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


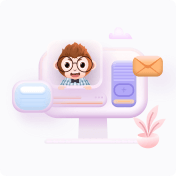
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页