根据提供的文件信息,本文将详细解释“多项式的加、减法和乘法”这一主题,尤其是在计算机科学领域内的实现方式。 ### 多项式的表示与基本操作 在数学中,多项式是由变量(如 x)的非负整数次幂与系数相乘构成的表达式。例如:\[P(x) = 3x^2 + 2x + 1\]。对于多项式的加法、减法和乘法,我们通常遵循以下步骤: 1. **加法**:两个多项式的加法就是将相同指数的项合并,即对应项的系数相加。 2. **减法**:两个多项式的减法实质上是将第二个多项式的每个系数取相反数后,再进行加法运算。 3. **乘法**:两个多项式的乘法则更加复杂,需要将一个多项式的每一项与另一个多项式的每一项相乘,然后将结果合并。 ### 使用链表表示多项式 在提供的代码片段中,作者使用了链表来存储多项式的各项。这种数据结构非常适合处理动态长度的数据集合,比如多项式的项数未知的情况。链表中的每一个节点都包含了系数(`coeficient`)、指数(`exponent`)以及指向下一个节点的指针(`next`)。 ### 文件读取与数据处理 程序首先打开了一个名为 `poly.in` 的文件,并从中读取两个多项式的定义。这里通过循环和条件判断实现了对字符串的解析,从而提取出每个项的系数和指数。值得注意的是,代码中还包含了一些细节处理,例如处理负号和默认指数为 1 的情况(当 x 后面没有显式给出指数时)。 ### 多项式的基本运算实现 接下来,程序会根据输入的运算符执行相应的操作: - **加法** (`+`):实现两个多项式的加法,即将相同指数的项合并。 - **减法** (`-`):实现两个多项式的减法,实质上是对第二个多项式的每个系数取反后再进行加法。 - **乘法** (`*`):实现两个多项式的乘法,需要遍历两个多项式的每一项并计算乘积。 #### 具体算法实现 1. **加法**:遍历两个链表,如果遇到相同指数的项,则将系数相加;如果某个多项式中的某一项的指数不存在于另一个多项式中,则直接加入结果链表中。 2. **减法**:可以看作是将第二个多项式的每个系数取反后进行加法。 3. **乘法**:对于乘法而言,需要遍历两个多项式的每一项,计算它们的乘积,并将结果按照指数大小排序后存储到新的链表中。这个过程涉及到大量的计算和排序操作,因此在实际编程时需要考虑效率问题。 ### 小结 本篇文章通过提供的代码片段介绍了如何使用链表来表示多项式,并在此基础上实现多项式的加法、减法和乘法。这种实现方法不仅能够有效处理多项式的运算,还能很好地适应不同长度的多项式,具有很高的灵活性和扩展性。在实际应用中,理解这些基础概念和算法是非常重要的,可以帮助我们在各种计算机科学和工程领域中更好地解决问题。
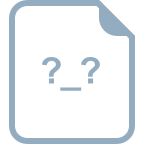
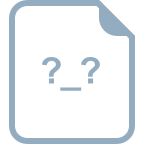
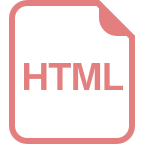
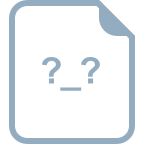
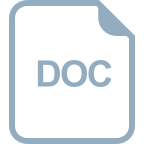
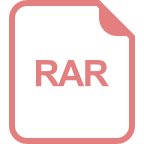
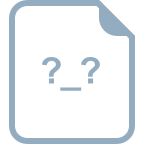
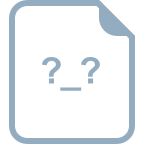
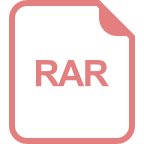
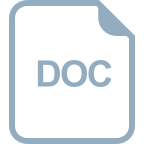
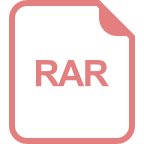
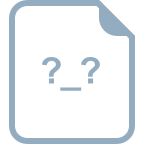
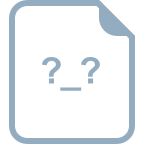
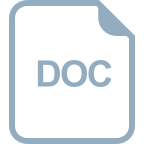
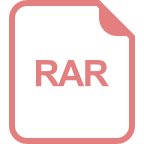
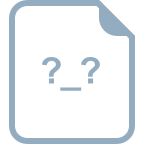
#include <stdlib.h>
#include <ctype.h>
#define Max 40
typedef struct data
{
int coeficient;
int exponent;
struct data *next;
} Data;
Data *head1, *head2, *ptr, *ptrD;
void init (Data *);
void get_data (char *, FILE *);
void Add ();
void Multiply ();
void Subtract ();
void swap(Data *, Data*);
int main()
{
FILE *fin;
char str[10], c, oper;
int i,token = 1;
if ((fin = fopen ("poly.in", "r")) == NULL)
{
fprintf (stderr, "Usage: cannot open the istream file\n");
exit (EXIT_FAILURE);
}
head1 = (Data *)malloc (sizeof (Data));
head2 = (Data *)malloc (sizeof (Data));
init (head2);
ptrD = head1;
while ((c = fgetc (fin)) == ' ' || c == '\t');
ungetc (c, fin);
while ((c = fgetc (fin)) != '\n' || feof (fin))
{
ungetc (c, fin);
get_data (str, fin);
ptr = (Data *)malloc (sizeof (Data));
init (ptr);
for (i = 0; str[i] != 'x' && str[i] != '\0'; i++)
{
if (str[i] == '+')
break;
else if (str[i] == '-')
token = -1;
else if (isdigit (str[i]))
ptr->coeficient = ptr->coeficient * 10 + str[i ]- '0';
}
ptr->coeficient *= token;
if (str[i ]== 'x')
{
if (ptr->coeficient == 0)
ptr->coeficient = token;
if (str[i+1] == '\0')
ptr->exponent = 1;
else
for (++i; str[i] != '\0'; i++)
ptr->exponent = ptr->exponent * 10 + str[i] - '0';
剩余11页未读,继续阅读
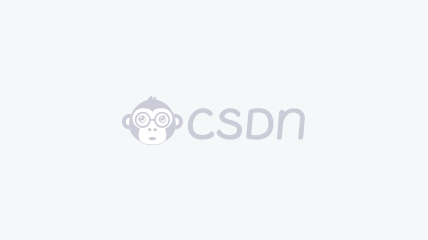

- 粉丝: 0
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

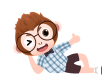
最新资源
- 汽车制造:ECU软件刷写技术及优化方法提升主机厂生产效率
- stm32f1x必要文件.7z
- 三次贝塞尔最小二乘拟-Cubic Bezier Least Square Fitting
- 基因频率的稳定性和遗传特性在自然选择下仿真
- 一本关于 numpy 矢量化技术的开放获取书籍,Nicolas P. Rougier,2017 年.zip
- Office2021 命令式下载和安装工具
- 多目标流向算法(MOFDA)Multi-Objective Flow Direction Algorithm
- 车载以太网协议及其在AUTOSAR架构中的实现
- 计算机网络分类.docx
- 车载诊断系统中功能安全的设计要求与应对方法

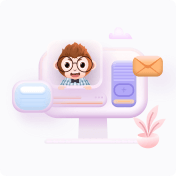
