# 图像过滤和混合图像
### 实验原理:
这项任务的目标是编写一个图像过滤功能,并使用它来创建混合图像,使用Oliva,Torralba和Schyns 的SIGGRAPH 2006 论文的简化版本。 混合图像是静态图像,其在解释中随观看距离而变化。基本思想是高频率在可用时倾向于支配感知,但是,在远处,只能看到信号的低频(平滑)部分。通过将一个图像的高频部分与另一个图像的低频部分混合,您可以获得混合图像,从而在不同距离处产生不同的解释。
### 实验目的:
对不同图像分别进行高通和低通滤波,融合图片
### 实验内容:
**图像过滤**:图像过滤(或卷积)是一种基本的图像处理工具。您将编写自己的函数以从头开始实现图像过滤。更具体地说,您将实现 在OpenCV库中my_imfilter()模仿该filter2D函数。如上所述student.py,过滤算法必须
- 支持灰度和彩色图像
- 支持任意形状的滤镜,只要两个尺寸都是奇数(例如7x9滤镜但不是4x5滤镜)
- 用零填充输入图像或反射图像内容和
- 返回与输入图像具有相同分辨率的滤波图像。
**混合图像**:混合图像是一个图像的低通滤波版本和第二图像的高通滤波版本的总和。有一个自由参数,其可被调谐为每个图像对,其控制多少高频到从所述第一图像和多少低频到所述第二图像中离开除去。这被称为“截止频率”。在论文中,建议使用两个截止频率(每个图像调整一个),你也可以自由尝试。在起始码中,通过改变用于构造混合图像的Gausian滤波器的标准偏差来控制截止频率。您将create_hybrid_image()根据入门代码实现student.py。你的功能会打电话my_imfilter() 创建低频和高频图像,然后将它们组合成混合图像。
### 实验器材(环境配置):
Windows:
CPU: Intel Core i7-6700HQ CPU @ 2.60GHz
Cache:
- L1:256KB
- L2:1.0MB,
- L3:6.0MB
开发环境:python 3.6
### 实验步骤及操作:
利用滤波函数操作图像
my_imfilter()实现
```python
def my_imfilter1(image, filter):
"""
Your function should meet the requirements laid out on the project webpage.
Apply a filter to an image. Return the filtered image.
Inputs:
- image -> numpy nd-array of dim (m, n, c)
- filter -> numpy nd-array of odd dim (k, l)
Returns
- filtered_image -> numpy nd-array of dim (m, n, c)
Errors if:
- filter has any even dimension -> raise an Exception with a suitable error message.
"""
filter_reshape = filter.reshape(-1,1)
filter_size = filter.size
filter_size_sqrt = round(math.sqrt(filter_size))
filter_size_floor = math.floor(filter_size_sqrt/2)
filtered_image = np.zeros(image.shape)
image_padding = np.zeros([image.shape[0] + 2 * filter_size_floor,
image.shape[1] + 2 * filter_size_floor, image.shape[2]])
image_padding[filter_size_floor:image_padding.shape[0]-filter_size_floor,
filter_size_floor:image_padding.shape[1]-filter_size_floor] = image
for i in range(image.shape[0]):
for j in range(image.shape[1]):
convolute_image = image_padding[i:i+filter_size_sqrt, j:j+filter_size_sqrt]
reshape_image = np.reshape(convolute_image[0:filter_size_sqrt,
0:filter_size_sqrt], (filter_size,3))
filtered_image[i,j] = sum(np.multiply(reshape_image, filter_reshape))
return filtered_image
```
生成高低通分量滤除图片,图像融合
gen_hybrid_image()实现
```c++
def gen_hybrid_image(image1, image2, cutoff_frequency):
"""
Inputs:
- image1 -> The image from which to take the low frequencies.
- image2 -> The image from which to take the high frequencies.
- cutoff_frequency -> The standard deviation, in pixels, of the Gaussian
blur that will remove high frequencies.
Task:
- Use my_imfilter to create 'low_frequencies' and 'high_frequencies'.
- Combine them to create 'hybrid_image'.
"""
assert image1.shape[0] == image2.shape[0]
assert image1.shape[1] == image2.shape[1]
assert image1.shape[2] == image2.shape[2]
# Steps: (1) Remove the high frequencies from image1 by blurring it.
# The amount of blur that works best will vary
# with different image pairs generate a 1x(2k+1) gaussian kernel with mean=0 and sigma = s,
# see https://stackoverflow.com/questions/17190649/how-to-obtain-a-gaussian-filter-in-python
s, k = cutoff_frequency, cutoff_frequency * 2
probs = np.asarray([exp(-z * z / (2 * s * s)) / sqrt(2 * pi * s * s) for z in range(-k, k + 1)], dtype=np.float32)
kernel = np.outer(probs, probs)
large_1d_blur_filter = kernel.reshape(-1,1)
# Your code here:
large_blur_image1 = my_imfilter1(image1, large_1d_blur_filter)
low_frequencies = large_blur_image1
# (2) Remove the low frequencies from image2. The easiest way to do this is to
# subtract a blurred version of image2 from the original version of image2.
# This will give you an image centered at zero with negative values.
# Your code here #
large_blur_image2 = my_imfilter1(image2, large_1d_blur_filter)
# large_blur_image2 = my_imfilter(large_blur_image2, large_1d_blur_filter.T)
high_frequencies = image2 - large_blur_image2 # Replace with your implementation
# (3) Combine the high frequencies and low frequencies
# Your code here #
hybrid_image = low_frequencies + high_frequencies # Replace with your implementation
# (4) At this point, you need to be aware that values larger than 1.0
# or less than 0.0 may cause issues in the functions in Python for saving
# images to disk. These are called in proj1_part2 after the call to
# gen_hybrid_image().
# One option is to clip (also called clamp) all values below 0.0 to 0.0,
# and all values larger than 1.0 to 1.0.
for i in range(hybrid_image.shape[0]):
for j in range(hybrid_image.shape[1]):
for k in range(hybrid_image.shape[2]):
if hybrid_image[i,j,k] > 1.0:
hybrid_image[i,j,k] = 1.0
if hybrid_image[i,j,k] < 0.0:
hybrid_image[i,j,k] = 0.0
return low_frequencies, high_frequencies, hybrid_image
```
主函数
防止存储失败做了一些判断
```c++
# Project Image Filtering and Hybrid Images - Generate Hybrid Image
# Based on previous and current work
# by James Hays for CSCI 1430 @ Brown and
# CS 4495/6476 @ Georgia Tech
import numpy as np
import matplotlib
import matplotlib.pyplot as plt
from helpers import vis_hybrid_image, load_image, save_image, my_imfilter, gen_hybrid_image, gen_hybrid_image_cv
# Before trying to construct hybrid images, it is suggested that you
# implement my_imfilter in helpers.py and then debug it using proj1_part1.py
# Debugging tip: You can split your python code and print in between
# to check if the current states of variables are expected.
## Setup
# Read images and convert to floating point format
image1 = load_image('../data/bird.bmp')
image2 = load_image('../data/plane.bmp')
# display the dog and cat images
# plt.figure(figsize=(3,3)); plt.imshow((image1*255).astype(np.uint8));#
# plt.figure(figsize=(3,3)); plt.imshow((image2*255).astype(np.uint8));
# For your write up, there are several additional test cases in 'data'.
# Feel free to make your own, too (you'll need to align the images in a
# photo editor such as Photoshop).
# The hybrid images will differ depending on which image you
# assign as image1 (which will provide the low frequencies) and which image
# you asign as image2 (which will provide the high f
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
这项任务的目标是编写一个图像过滤功能,并使用它来创建混合图像,使用Oliva,Torralba和Schyns 的SIGGRAPH 2006 论文的简化版本。 混合图像是静态图像,其在解释中随观看距离而变化。基本思想是高频率在可用时倾向于支配感知,但是,在远处,只能看到信号的低频(平滑)部分。通过将一个图像的高频部分与另一个图像的低频部分混合,您可以获得混合图像,从而在不同距离处产生不同的解释。 详细介绍参考:https://blog.csdn.net/newlw/article/details/132531511
资源推荐
资源详情
资源评论
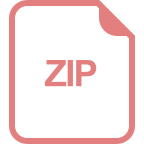
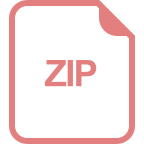
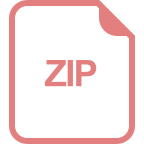
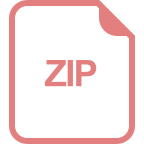
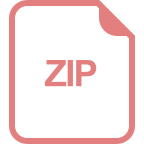
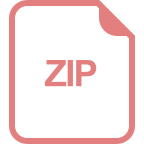
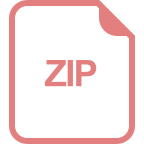
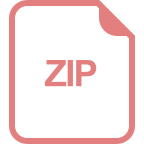
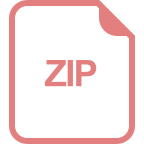
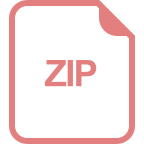
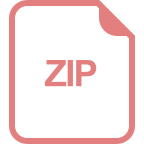
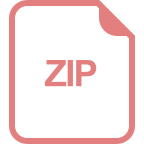
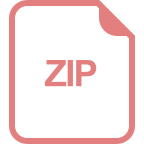
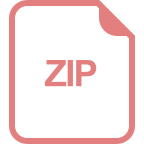
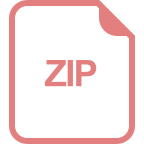
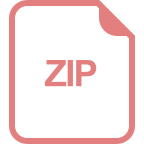
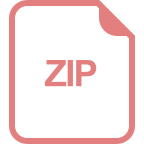
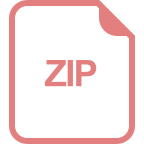
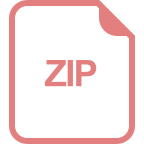
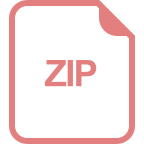
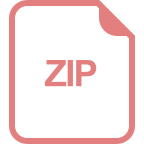
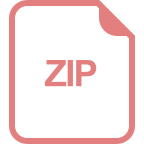
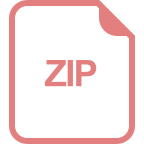
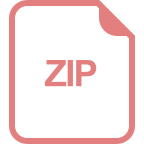
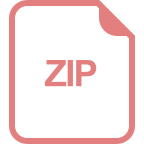
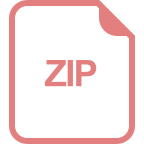
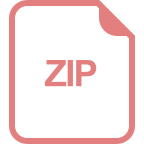
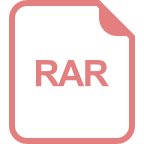
收起资源包目录



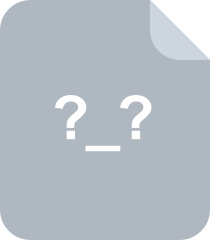
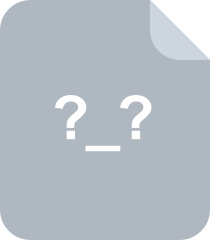
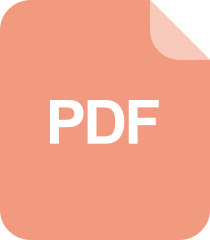

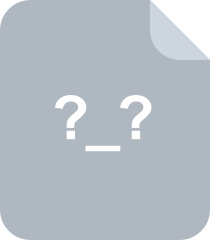
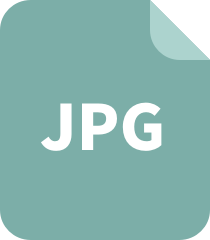
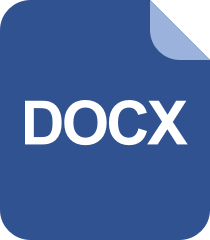

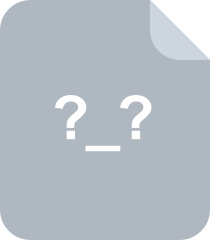
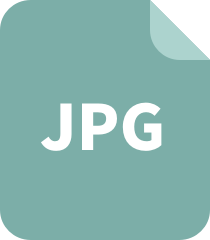

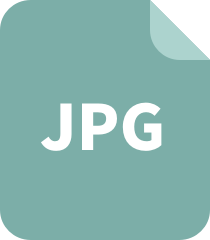

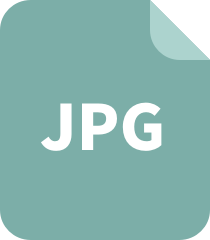
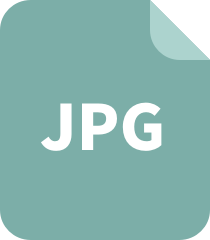
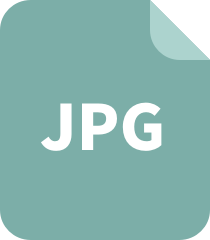
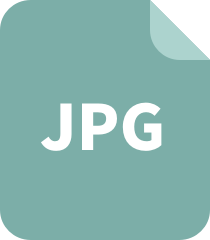

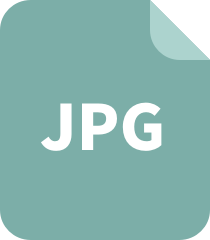
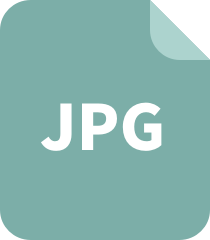
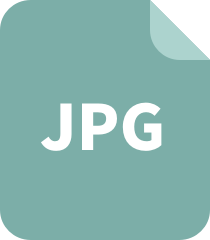
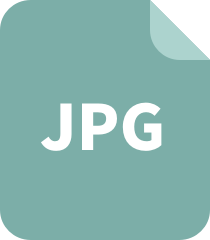
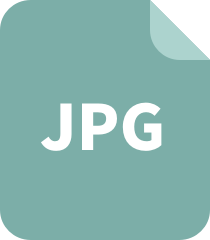
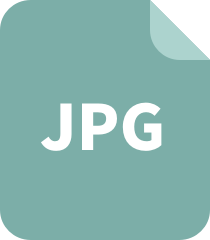

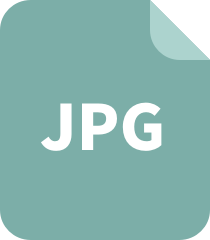
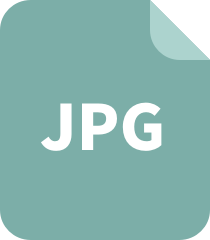
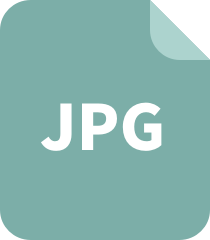
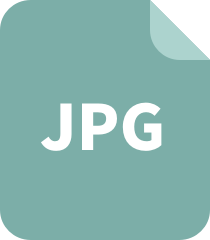
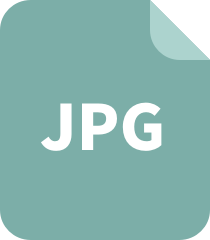
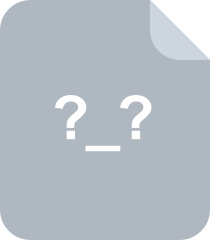

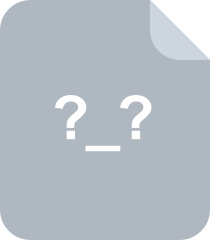
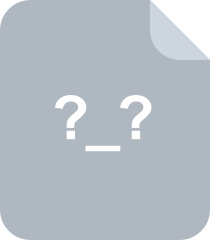

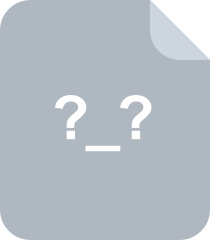
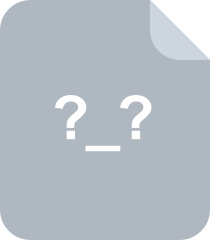
共 29 条
- 1
资源评论
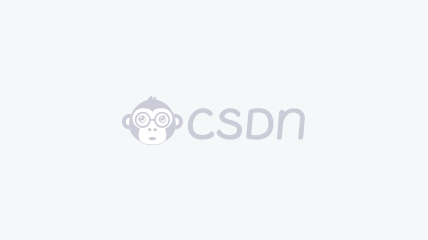

shejizuopin
- 粉丝: 1w+
- 资源: 1300
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

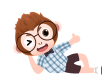
最新资源
- 基于Python的迷宫小游戏-2024-12.zip、生成迷宫、随机生成任务、任务完成后移除任务点、显示胜利确认窗口,玩家选择是否进入下一个迷宫等等含音效
- 章节2:编程基本概念之26:字符串切片slice操作-逆序.rar
- 章节2:编程基本概念之25:字符串-str-字符提取-replace替换-内存分析.rar
- EasyBoot-v6.6.0.800单文件注册便携版
- 章节2:编程基本概念之24:字符串-转义字符-字符串拼接-字符串复制-input获取键盘输入.rar
- 包装食品检测30-YOLO(v5至v9)数据集合集.rar
- 设计模式 jQuery源码分析.rar
- 3rdParty-VC10-x86-x64, OSG第三方编译资源包3rdpartyVs2010-x86
- 包装食品检测29-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- 嵌入式系统应用-LVGL的应用-平衡球游戏 part2
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


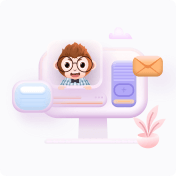
安全验证
文档复制为VIP权益,开通VIP直接复制
