> # ♻️ 资源
> **大小:** 12.2MB
> **文档链接:**[**https://www.yuque.com/sxbn/ks/100013183**](https://www.yuque.com/sxbn/ks/100013183)
**➡️ 资源下载:**[**https://download.csdn.net/download/s1t16/88217565**](https://download.csdn.net/download/s1t16/88217565)
**注:如当前文章或代码侵犯了您的权益,请私信作者删除!**
# 1 Project Overview
## 1.1 Project Description
Based on tensorflow and Flask, a web-based image search engine is realized, which can realize simple image search function based on server images database.
## 1.2 Project function requirements
●It contains an input box to upload an image (Formulation);
●Users can preview the query image in the searching window (Formulation);
●It has a search button (Initiation);
●Provide an overview of the results (e.g. the total number of results) (Review);
●Allow changing search parameters (e.g. select certain category/tag) when reviewing results (Refinement);
●Users can take some actions, e.g. add selected images to a favorite list (Use);
# 2 Design and implementation
## 2.1 Design
### 2.1.1 Code design
The program code is divided into two parts: front end and back end. The front and back end realize data transmission through Flask. The back end is python program, and the front end is web application.
The file directory structure is as follows:
├── database
│ ├── dataset
│ └── tags
├── imagenet
├── static
│ ├── favorite
│ ├── images
│ └── result
├── uploads
├── templates
│ └── HTML
└── .py
#### Main function design:
front end:
```javascript
//Show favorite function, bind with favorite button
function favorite_fun()
//Add a favorite function, bound to the Add to favorites button
function addFavorite_fun()
//Confirm the collection function, bind to the Confirm collection button
function confirm_fun()
//Delete favorite image function, bind with Delete button
function delete_fun()
//Confirm delete function, bind with Confirm delete button
function confirmdelete_fun()
//Tag selection function, binding to each tag button except favorite, passing arguments by str
function tag_fun(str)
//Search function, bound to the Search button
function fun()
```
back end:
```python
# This function is used to do the image search/image retrieval
@app.route('/imgUpload', methods=['GET', 'POST']) # /imgUpload matches the url in ajax
def upload_img():
# This function is tag
@app.route('/tag', methods=['GET', 'POST'])
def tag():
# This function is addFavorite
@app.route('/addFavorite', methods=['GET', 'POST'])
def addFavorite():
# This function is displaying favorites
@app.route('/favorite', methods=['GET', 'POST'])
def favorite():
# This function is deleting favorites
@app.route('/deleteFavorite', methods=['GET', 'POST'])
def deleteFavorite():
```
### 2.1.2 interface design
#### Start interface:
Include theme、upload file button、search button、preview image、tag bar and my favorites.
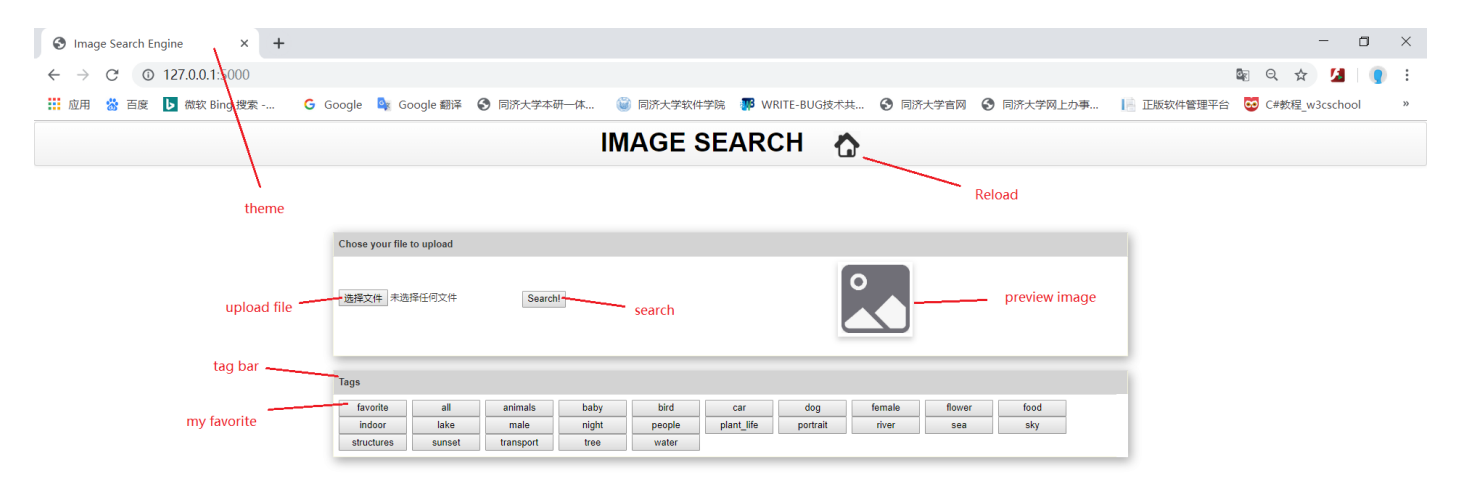
#### Search results interface:
Show search results and description of search results:
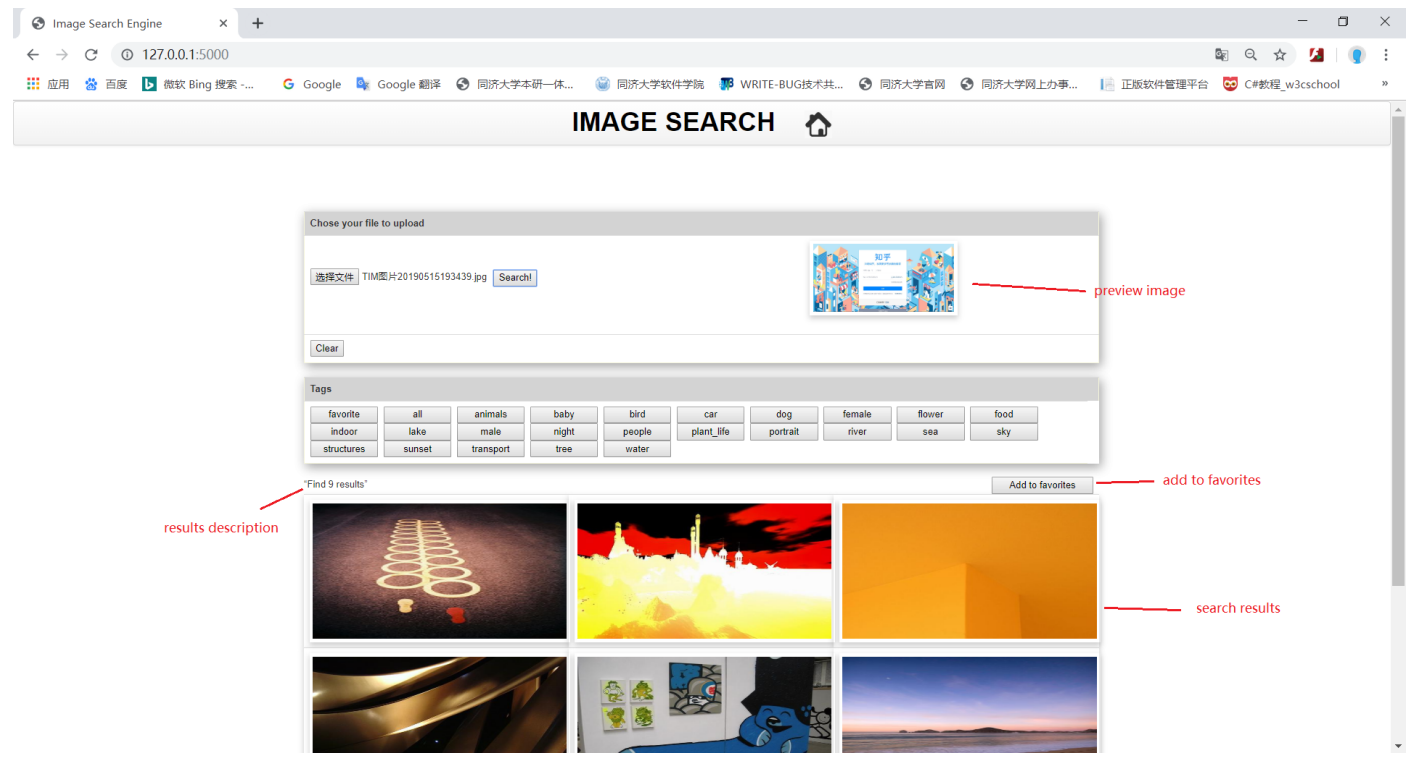
#### My favorites interface:
Show a collection of pictures, a description of the number of pictures.
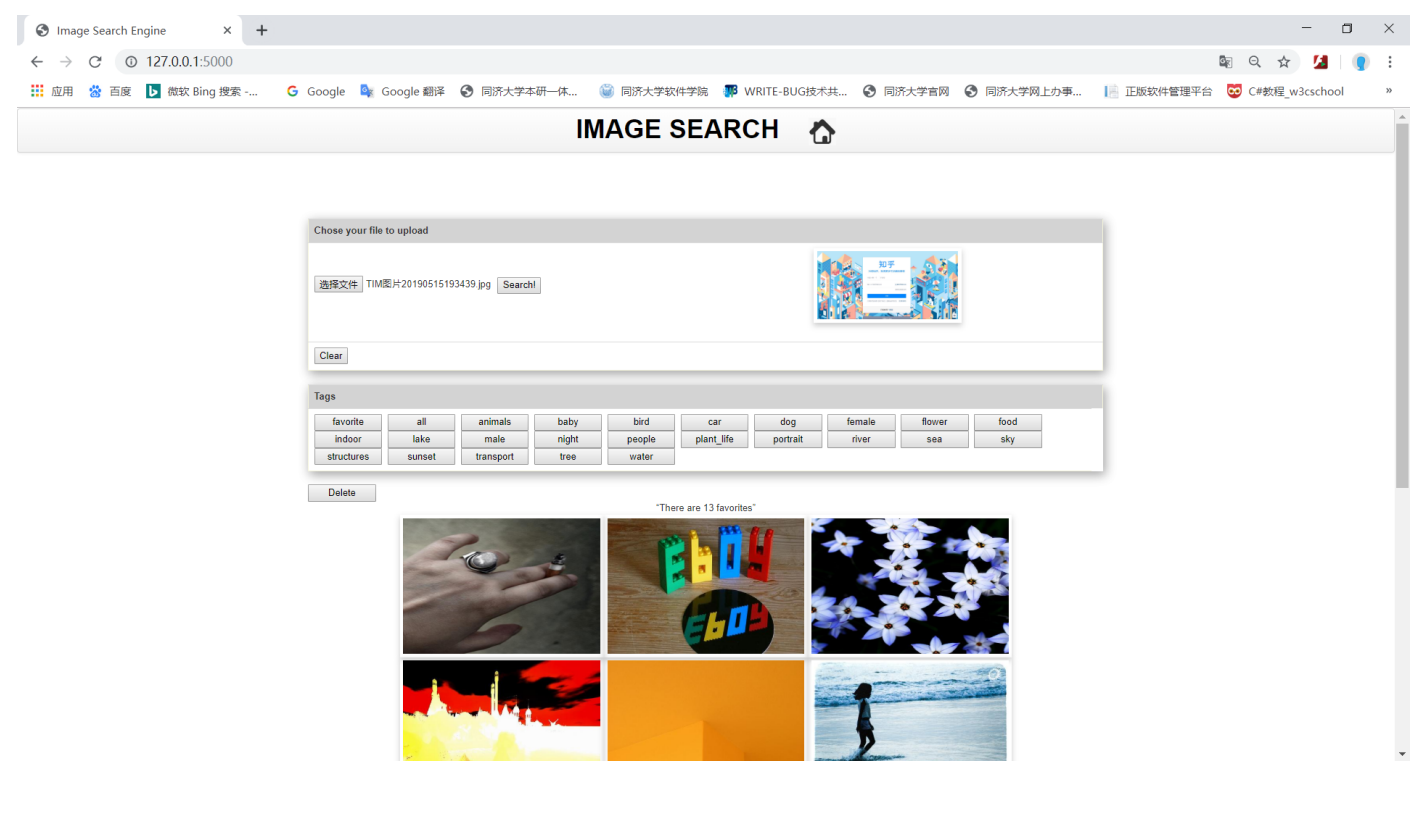
## 2.2 Implementation
### 2.2.1 Main function implementation
#### Show search results
#### front end:
```javascript
function fun(){
$('#div2').hide();//Hide favorites content
$('#load').show();//Display loading animation
$("#confirm").hide();//Hide Confirm Favorites button
$("#addFavorite").show();//Show Add to favorites button
$("form").submit(function(evt){
evt.preventDefault();
var formData = new FormData($(this)[0]);
$.ajax({
url: 'imgUpload',
type: 'POST',
data: formData,
//async: false,
cache: false,
contentType: false,
enctype: 'multipart/form-data',
processData: false,
success: function (response) {//Response returns the searched image path
$('#load').hide();//Hidden loading animation
$('#clearrow').show();//Show Clear button
//Show searched images
$("#img0").show();
$("#img1").show();
$("#img2").show();
$("#img3").show();
$("#img4").show();
$("#img5").show();
$("#img6").show();
$("#img7").show();
$("#img8").show();
//Assign values to each image path
document.getElementById("img0").src = response.image0;
console.log(response.image0);
console.log(document.getElementById("img0").src);
document.getElementById("img1").src = response.image1;
document.getElementById("img2").src = response.image2;
document.getElementById("img3").src = response.image3;
document.getElementById("img4").src = response.image4;
document.getElementById("img5").src = response.image5;
document.getElementById("img6").src = response.image6;
document.getElementById("img7").src = response.image7;
document.getElementById("img8").src = response.image8;
$('#table').show();//Display picture table
$('#resultStats').show();//Display result statement
$('#clear').show();//Show Clear button
}
});
return false;
})
}
```
#### back end:
```python
@app.route('/imgUpload', methods=['GET', 'POST']) # /imgUpload matches the url in ajax
def upload_img():
print("image upload")
result = 'static/result'
if not gfile.Exists(result): # If there is no result folder, create it
os.mkdir(result)
shutil.rmtree(result) # Empty the result folder
if request.method == 'POST' or request.method == 'GET':
print(request.method)
# check if the post request has the file part
if 'file' not in request.files:
print('No file part')
return redirect(request.url)
file = request.files['file']
print(file.filename)
# if user does not select file, browser also
# submit a empty part without filename
if file.filename == '':
print('No selected file')
return redirect(request.url)
if file: # and allowed_file(file.filename):
filename = secure_filename(file.filename)
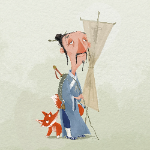
神仙别闹
- 粉丝: 4137
- 资源: 7484
最新资源
- 基于大数据的压缩包密码破解软件详细文档+全部资料+源码.zip
- 基于大数据、人工智能的招聘大数据分析展示系统——前端可视化详细文档+全部资料+源码.zip
- 基于大数据的图书推荐系统详细文档+全部资料+源码.zip
- 基于电子商城项目,包含:数据库操作(mysql)、Swing界面等详细文档+全部资料+源码.zip
- 基于大数据挖掘的饮食推荐小程序详细文档+全部资料+源码.zip
- 基于豆瓣影视数据(不完整)的影视大数据分析平台。爬虫(影视数据和用户数据),推荐系统(基于用户的协同过滤算法),后台(flask实现的简易后台)。详细文档+全部资料+源码.zip
- 基于多种编程语言开源免费不限制提供生活常用,出行服务,开发工具,金融服务,通讯服务和公益大数据的平台详细文档+全部资料+源码.zip
- 基于开源Litemall电商项目的大数据项目数据仓库(五层)、实时计算和用户画像。大数据平台采用CDH6.3.2(已使用vagrant+ansible脚本化),
- 基于注解和反射Excel的简单、大数据量、基于模板、多sheet页的导入导出详细文档+全部资料+源码.zip
- 基于垃圾短信基于文本内容的识别详细文档+全部资料+源码.zip
- 计算机网络资源文件1111
- 基于云平台的大数据路网流量管控详细文档+全部资料+源码.zip
- Delphi 12 控件之DevExpressVCLProducts-24.2.3.exe.zip
- 政府绿色采购明细数据(2015-2024.3).zip
- 天工CAD软件的测试反馈表
- Springboot3声明式客户端
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


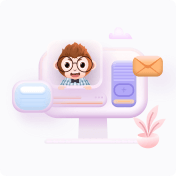