# Universal Image Loader for Android
This project aims to provide a reusable instrument for asynchronous image loading, caching and displaying. It is originally based on [Fedor Vlasov's project](https://github.com/thest1/LazyList) and has been vastly refactored and improved since then.
**Download:** [JAR library](https://github.com/nostra13/Android-Universal-Image-Loader/downloads); [sources](https://github.com/nostra13/Android-Universal-Image-Loader/downloads) (you can attach it to project as _source attachment_ so you can see Java docs)

## Features
* Multithread image loading
* Possibility of wide tuning ImageLoader's configuration (thread pool size, HTTP options, memory and disc cache, display image options, and others)
* Possibility of image caching in memory and/or on device's file sysytem (or SD card)
* Possibility to "listen" loading process
* Possibility to customize every display image call with separated options
* Widget support
## Documentation
* Universal Image Loader. Part 1 - Introduction [[RU](http://nostra13android.blogspot.com/2012/03/4-universal-image-loader-part-1.html) | [EN](http://www.intexsoft.com/blog/item/68-universal-image-loader-part-1.html)]
* Universal Image Loader. Part 2 - Configuration [[RU](http://nostra13android.blogspot.com/2012/03/5-universal-image-loader-part-2.html) | [EN](http://www.intexsoft.com/blog/item/72-universal-image-loader-part-2.html)]
* Universal Image Loader. Part 3 - Usage [[RU](http://nostra13android.blogspot.com/2012/03/6-universal-image-loader-part-3-usage.html) | [EN](http://www.intexsoft.com/blog/item/74-universal-image-loader-part-3.html)]
### [Support](http://stackoverflow.com/questions/tagged/universal-image-loader)
First look at [Useful info](https://github.com/nostra13/Android-Universal-Image-Loader#useful-info).
If you have some question about Universal Image Loader you can ask it on [StackOverFlow](http://stackoverflow.com) with **[universal-image-loader]** tag. Also add **[java]** and **[android]** tags.
Bugs and feature requests place **[here](https://github.com/nostra13/Android-Universal-Image-Loader/issues/new)**.
### [Changelog](https://github.com/nostra13/Android-Universal-Image-Loader/commits/master)
## Usage
### Simple
``` java
ImageView imageView = ...
String imageUrl = "http://site.com/image.png"; // or "file:///mnt/sdcard/images/image.jpg"
// Get singletone instance of ImageLoader
ImageLoader imageLoader = ImageLoader.getInstance();
// Initialize ImageLoader with configuration. Do it once.
imageLoader.init(ImageLoaderConfiguration.createDefault(context));
// Load and display image asynchronously
imageLoader.displayImage(imageUrl, imageView);
```
### Most detailed
``` java
ImageView imageView = ...
String imageUrl = "http://site.com/image.png"; // or "file:///mnt/sdcard/images/image.jpg"
ProgressBar spinner = ...
File cacheDir = StorageUtils.getOwnCacheDirectory(getApplicationContext(), "UniversalImageLoader/Cache");
// Get singletone instance of ImageLoader
ImageLoader imageLoader = ImageLoader.getInstance();
// Create configuration for ImageLoader (all options are optional, use only those you really want to customize)
ImageLoaderConfiguration config = new ImageLoaderConfiguration.Builder(getApplicationContext())
.memoryCacheExtraOptions(480, 800) // max width, max height
.discCacheExtraOptions(480, 800, CompressFormat.JPEG, 75) // Can slow ImageLoader, use it carefully (Better don't use it)
.threadPoolSize(3)
.threadPriority(Thread.NORM_PRIORITY - 1)
.denyCacheImageMultipleSizesInMemory()
.offOutOfMemoryHandling()
.memoryCache(new UsingFreqLimitedMemoryCache(2 * 1024 * 1024)) // You can pass your own memory cache implementation
.discCache(new UnlimitedDiscCache(cacheDir)) // You can pass your own disc cache implementation
.discCacheFileNameGenerator(new HashCodeFileNameGenerator())
.imageDownloader(new URLConnectionImageDownloader(5 * 1000, 20 * 1000)) // connectTimeout (5 s), readTimeout (20 s)
.defaultDisplayImageOptions(DisplayImageOptions.createSimple())
.enableLogging()
.build();
// Initialize ImageLoader with created configuration. Do it once on Application start.
imageLoader.init(config);
// Creates display image options for custom display task (all options are optional)
DisplayImageOptions options = new DisplayImageOptions.Builder()
.showStubImage(R.drawable.stub_image)
.showImageForEmptyUri(R.drawable.image_for_empty_url)
.cacheInMemory()
.cacheOnDisc()
.imageScaleType(ImageScaleType.POWER_OF_2)
.displayer(new RoundedBitmapDisplayer(0xff424242, 20))
.build();
// Load and display image
imageLoader.displayImage(imageUrl, imageView, options, new ImageLoadingListener() {
@Override
public void onLoadingStarted() {
spinner.show();
}
@Override
public void onLoadingFailed(FailReason failReason) {
spinner.hide();
}
@Override
public void onLoadingComplete(Bitmap loadedImage) {
spinner.hide();
}
@Override
public void onLoadingCancelled() {
// Do nothing
}
});
```
## Useful info
1. **Caching is NOT enabled by default.** If you want loaded images will be cached in memory and/or on disc then you should enable caching in DisplayImageOptions this way:
``` java
// Create default options which will be used for every
// displayImage(...) call if no options will be passed to this method
DisplayImageOptions defaultOptions = new DisplayImageOptions.Builder()
...
.cacheInMemory()
.cacheOnDisc()
...
.build();
ImageLoaderConfiguration config = new ImageLoaderConfiguration.Builder(getApplicationContext())
...
.defaultDisplayImageOptions(defaultOptions)
...
.build();
ImageLoader.getInstance().init(config); // Do it on Application start
```
``` java
// Then later, when you want to display image
ImageLoader.getInstance().displayImage(imageUrl, imageView); // Default options will be used
```
or this way:
``` java
DisplayImageOptions options = new DisplayImageOptions.Builder()
...
.cacheInMemory()
.cacheOnDisc()
...
.build();
ImageLoader.getInstance().displayImage(imageUrl, imageView, options); // Incoming options will be used
```
2. How UIL define Bitmap size needed for exact ImageView? Search defined parameters top-down:
* Get ```android:layout_width``` or ```android:layout_height``` parameters
* Get ```android:maxWidth``` and ```android:maxHeight``` parameters
* Get maximum size parameters from configuration (```memoryCacheExtraOptions(int, int)``` option)
Set ```android:layout_width```|```android:layout_height``` or ```android:maxWidth```|```android:maxHeight``` parameters for ImageView if you know approximate maximum size of it. It will help correctly compute Bitmap size needed for this view and **save memory**.
3. If you often got **OutOfMemoryError** in your app using Universal Image Loader then try set WeakMemoryCache into configuration:
``` java
ImageLoaderConfiguration config = new ImageLoaderConfiguration.Builder(getApplicationContext())
...
.memoryCache(new WeakMemoryCache())
...
.build();
```
4. For memory cache configuration (ImageLoaderConfiguration.Builder.memoryCache(...)) you can use already prepared implementations:
* UsingFreqLimitedMemoryCache (The least frequently used bitmap is deleted when cache size limit is exceeded) - Used by default
* LRULimitedMemoryCache (Least recently used bitmap is deleted when cache size limit is exceeded)
* FIFOLimitedMemoryCache (FIFO rule is used for deletion when cache size limit is exceeded)
* LargestLimitedMemoryCache (The largest bitmap is deleted when cache size limit is exceeded)
* LimitedAgeMemoryCache (Decorator. Cached object is deleted when its age exceeds defined val

荣华富贵8
- 粉丝: 225
- 资源: 7653
最新资源
- 基于Java语言的HBase分布式数据库设计源码分析
- BLCN_v_0_0_2.zip
- 基于HTML、CSS、JavaScript的购物商城设计源码
- 基于Vue、JavaScript、CSS、HTML的交通事故管理系统设计源码
- 基于Comsol声波阵面调控技术的压力声学与固体力学模块研究:3258-3824hz扫频在Comsol6.1版本中的应用,基于Comsol声波阵面调控技术的压力声学与固体力学模块研究:3258-382
- 基于Nodejs扩展宿主的coc.nvim设计源码,支持多种编程语言和语言服务器
- ESP-IDFESP32C6使用ESP-IDF5.4驱动ST7789V
- 基于VDLL算法的矢量型GPS信号跟踪算法MATLAB仿真研究:程序与Word设计文档详解,基于VDLL算法的矢量型GPS信号跟踪算法MATLAB仿真研究:程序与Word设计文档详解,基于VDLL的矢
- 循环温度的边界条件设置:双法实现与复杂温度变化的深度探讨,基于循环温度调控的双方法边界条件设置技术及复杂温度变化处理方案,两种方法实现循环温度的边界条件设置 复杂的温度变化 ,循环温度的边界条件设
- 基于Vue框架的智联铁塔前端开发设计源码
- 基于C#游戏逻辑的方块闯关游戏设计源码
- 基于STM3F4源码的VESC非线性磁链观测器:零速启动与详细注释,助您学习磁链观测技术,包含simulink仿真与文献参考,基于STM3F4源码的VESC非线性磁链观测器:零速启动与详细注释,sim
- 基于Java的公寓租赁平台移动端与后台管理系统设计源码
- 西门子Smart SB CM01与台达DT330温控器485通讯程序设计与实现(基于S7-200 Smart PLC控制),西门子Smart SB CM01与台达DT330温控器485通讯程序:PLC
- 基于JavaScript、CSS、HTML的贷款H5页面设计源码
- easy-test-app.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


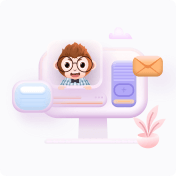