根据给定的文件信息,以下是对“Java面试题”中提到的一些编程问题的详细解析与解答,这些问题旨在考察应聘者的基础编程能力和逻辑思维能力。 ### 1. 组合不重复的三位数 **题目描述**: 有1、2、3、4四个数字,能够组合成多少个互不相同且不重复的三位数,并将这些数字打印出来。 **解题思路**: - 使用三层嵌套的for循环来组合数字。 - 判断是否重复:如果当前组合的数字已经在集合中存在,则跳过本次循环。 - 将不重复的数字添加到集合中。 - 最后通过集合的size()方法获取不重复数字的数量。 **代码示例**: ```java import java.util.ArrayList; public class UniqueThreeDigits { public static void main(String[] args) { ArrayList<Integer> list = new ArrayList<>(); for (int i = 1; i <= 4; i++) { for (int j = 1; j <= 4; j++) { for (int k = 1; k <= 4; k++) { if (i != j && i != k && j != k) { int num = i * 100 + j * 10 + k; if (!list.contains(num)) { list.add(num); System.out.println(num); } } } } } System.out.println("Total unique numbers: " + list.size()); } } ``` ### 2. 数据加密与解密 **题目描述**: 某个公司采用公用电话传递数据,数据是四位的整数,在传递过程中加密,规则如下:每位数字加5,除以10取余代替该数字,再将第一位和第四位互换,第二位和第三位互换。给出具体的实现代码。 **解题思路**: - 将输入的四位数字拆分成各个位上的数字。 - 对每个位上的数字进行加密处理。 - 按照加密后的规则重新组合数字。 **代码示例**: ```java public class DataEncryption { public static void main(String[] args) { int number = 1234; String encrypted = encrypt(number); System.out.println("Encrypted number: " + encrypted); } public static String encrypt(int number) { int first = (number / 1000) % 10; int second = (number / 100) % 10; int third = (number / 10) % 10; int fourth = number % 10; int encryptedFirst = (first + 5) % 10; int encryptedSecond = (second + 5) % 10; int encryptedThird = (third + 5) % 10; int encryptedFourth = (fourth + 5) % 10; return encryptedFourth + "" + encryptedThird + "" + encryptedSecond + "" + encryptedFirst; } } ``` ### 3. 判断某年某月某日是否为当年的第一天 **题目描述**: 输入某年某月某日,判断这一天是否为这一年的第一天。 **解题思路**: - 首先判断输入的日期是否为1月1日。 - 考虑闰年和平年的不同月份天数。 **代码示例**: ```java public class FirstDayOfYear { public static boolean isFirstDayOfYear(int year, int month, int day) { // 判断是否为1月1日 if (month == 1 && day == 1) { return true; } return false; } public static void main(String[] args) { int year = 2023, month = 1, day = 1; if (isFirstDayOfYear(year, month, day)) { System.out.println("It's the first day of the year."); } else { System.out.println("It's not the first day of the year."); } } } ``` ### 4. 输入三个整数并按从小到大的顺序输出 **题目描述**: 输入三个整数x、y、z,按照从小到大的顺序输出这三个数。 **解题思路**: - 使用条件语句比较大小,并调整顺序。 **代码示例**: ```java public class SortNumbers { public static void main(String[] args) { int x = 10, y = 5, z = 20; sort(x, y, z); } public static void sort(int x, int y, int z) { if (x > y) { int temp = x; x = y; y = temp; } if (y > z) { int temp = y; y = z; z = temp; } if (x > y) { int temp = x; x = y; y = temp; } System.out.println("Sorted numbers: " + x + ", " + y + ", " + z); } } ``` 以上是部分题目的详细解析及示例代码,这些题目涵盖了基础的数据结构操作、逻辑判断以及简单的算法设计等内容,非常适合初学者练习。
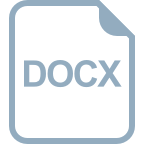
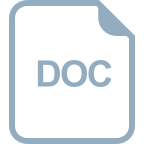
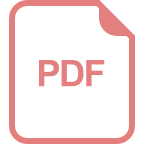
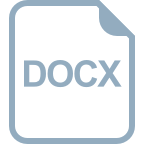
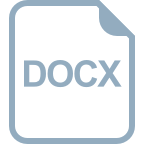
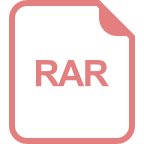
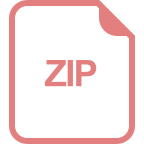
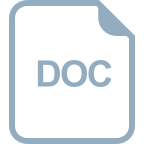
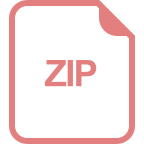
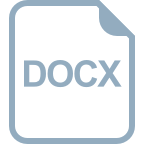
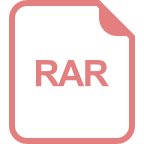
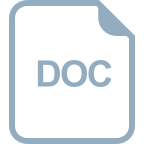
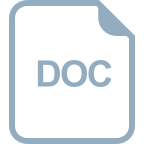
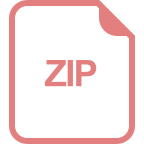
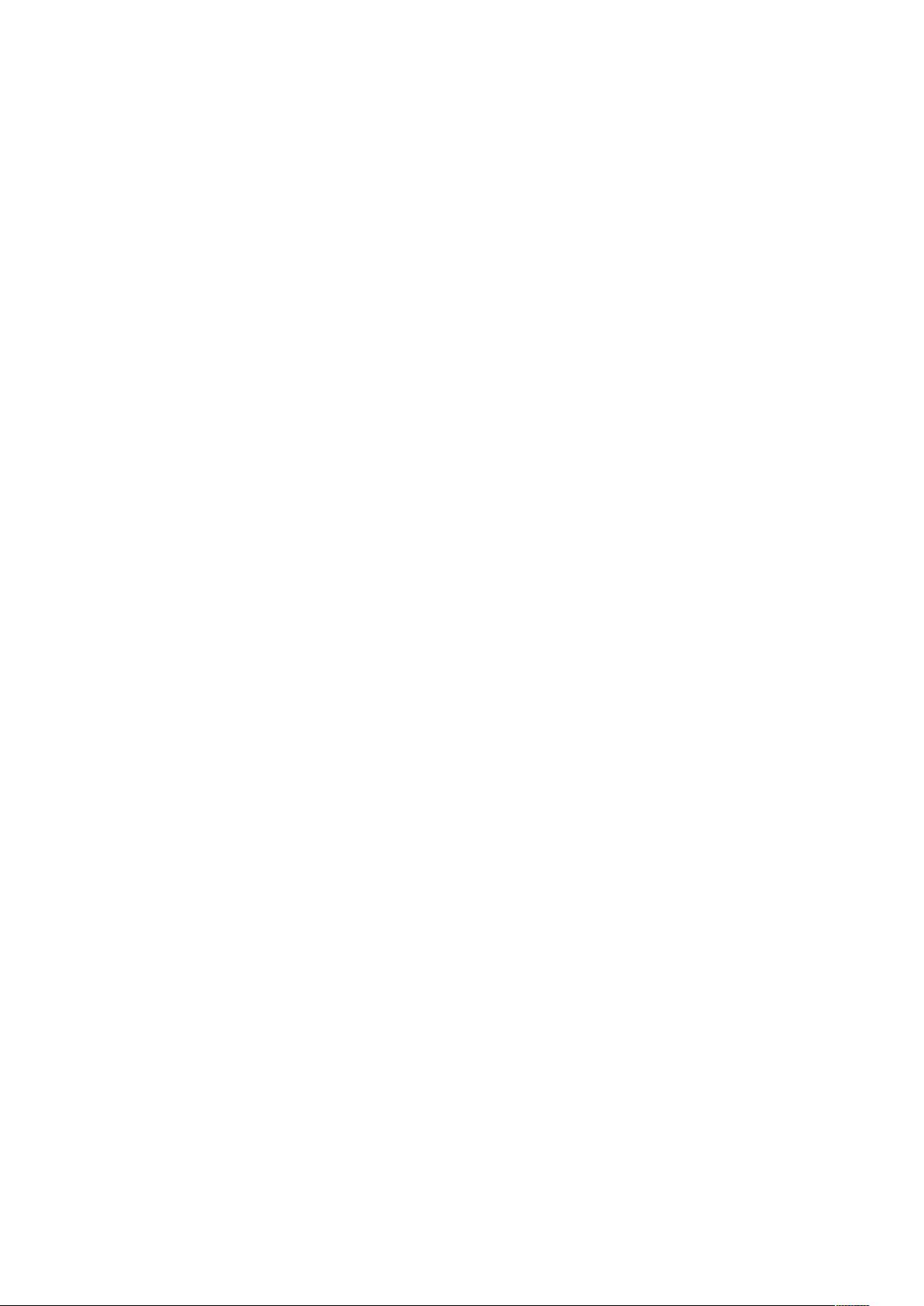
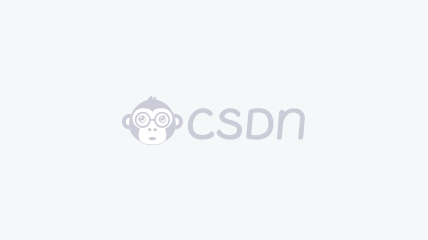

- 粉丝: 2
- 资源: 2
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

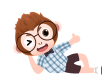
最新资源

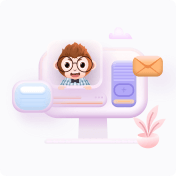
