from skimage import io, transform # skimage模块下的io transform(图像的形变与缩放)模块
import glob # glob 文件通配符模块
import os # os 处理文件和目录的模块
import tensorflow as tf
import numpy as np # 多维数据处理模块
import time
# 数据集地址
path = 'data'
# 模型保存地址
model_path = 'model/fc_model.ckpt'
# 将所有的图片resize成100*100
w = 100
h = 100
c = 1
# 读取图片+数据处理
def read_img(path):
imgs = []
labels = []
for fistname in os.listdir(path):
# print(fistname)
for textname in os.listdir(path + "/" + fistname): # os.listdir() 方法用于返回指定的文件夹包含的文件或文件夹的名字的列表
# print(textname)
for fname in os.listdir(path + "/" + fistname + "/" + textname):
# print(fname)
fpath = os.path.join(path, fistname, textname, fname) # 路径拼接
img = io.imread(fpath) # 按照路径打开文件
# #skimage.transform.resize(image, output_shape)改变图片的尺寸
img = transform.resize(img, (w, h))
# 将读取的图片数据加载到imgs[]列表中
imgs.append(img)
# 将图片的label加载到labels[]中,与上方的imgs索引对应
label = int(fistname[-1])
labels.append(label)
# 将读取的图片和labels信息,转化为numpy结构的ndarr(N维数组对象(矩阵))数据信息
imgs = np.array(imgs)
imgs = np.reshape(imgs, [-1, w, h, 1])
print("shape of datas: {}\tshape of labels: {}".format(np.array(imgs).shape, np.array(labels).shape))
return np.asarray(imgs, np.float32), np.asarray(labels, np.int32)
# 调用读取图片的函数,得到图片和labels的数据集
data, label = read_img(path)
# 打乱顺序
# 读取data矩阵的第一维数(图片的个数)
num_example = data.shape[0]
# 产生一个num_example范围,步长为1的序列
arr = np.arange(num_example)
# 调用函数,打乱顺序
np.random.shuffle(arr)
# 按照打乱的顺序,重新排序
data = data[arr]
label = label[arr]
# 将所有数据分为训练集和验证集
ratio = 0.8
s = np.int(num_example * ratio)
x_train = data[:s]
y_train = label[:s]
x_val = data[s:]
y_val = label[s:]
# -----------------构建网络----------------------
# 本程序cnn网络模型,共有7层,前三层为卷积层,后三层为全连接层,前三层中,每层包含卷积、激活、池化层
# 占位符设置输入参数的大小和格式
x = tf.placeholder(tf.float32, shape=[None, w, h, c], name='x')
y_ = tf.placeholder(tf.int32, shape=[None, ], name='y_')
def inference(input_tensor, train, regularizer):
# -----------------------第一层----------------------------
with tf.variable_scope('layer1-conv1'):
# 初始化权重conv1_weights为可保存变量,大小为5x5,3个通道(RGB),数量为32个
conv1_weights = tf.get_variable("weight", [5, 5, 1, 32],
initializer=tf.truncated_normal_initializer(stddev=0.1))
# 初始化偏置conv1_biases,数量为32个
conv1_biases = tf.get_variable("bias", [32], initializer=tf.constant_initializer(0.0))
# 卷积计算,tf.nn.conv2d为tensorflow自带2维卷积函数,input_tensor为输入数据,
# conv1_weights为权重,strides=[1, 1, 1, 1]表示左右上下滑动步长为1,padding='SAME'表示输入和输出大小一样,即补0
conv1 = tf.nn.conv2d(input_tensor, conv1_weights, strides=[1, 1, 1, 1], padding='SAME')
# 激励计算,调用tensorflow的relu函数
relu1 = tf.nn.relu(tf.nn.bias_add(conv1, conv1_biases))
with tf.name_scope("layer2-pool1"):
# 池化计算,调用tensorflow的max_pool函数,strides=[1,2,2,1],表示池化边界,2个对一个生成,padding="VALID"表示不操作。
pool1 = tf.nn.max_pool(relu1, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding="VALID")
# -----------------------第二层----------------------------
with tf.variable_scope("layer3-conv2"):
# 同上,不过参数的有变化,根据卷积计算和通道数量的变化,设置对应的参数
conv2_weights = tf.get_variable("weight", [5, 5, 32, 64],
initializer=tf.truncated_normal_initializer(stddev=0.1))
conv2_biases = tf.get_variable("bias", [64], initializer=tf.constant_initializer(0.0))
conv2 = tf.nn.conv2d(pool1, conv2_weights, strides=[1, 1, 1, 1], padding='SAME')
relu2 = tf.nn.relu(tf.nn.bias_add(conv2, conv2_biases))
with tf.name_scope("layer4-pool2"):
pool2 = tf.nn.max_pool(relu2, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='VALID')
# -----------------------第三层----------------------------
# 同上,不过参数的有变化,根据卷积计算和通道数量的变化,设置对应的参数
with tf.variable_scope("layer5-conv3"):
conv3_weights = tf.get_variable("weight", [3, 3, 64, 128],
initializer=tf.truncated_normal_initializer(stddev=0.1))
conv3_biases = tf.get_variable("bias", [128], initializer=tf.constant_initializer(0.0))
conv3 = tf.nn.conv2d(pool2, conv3_weights, strides=[1, 1, 1, 1], padding='SAME')
relu3 = tf.nn.relu(tf.nn.bias_add(conv3, conv3_biases))
with tf.name_scope("layer6-pool3"):
pool3 = tf.nn.max_pool(relu3, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='VALID')
# -----------------------第四层----------------------------
# 同上,不过参数的有变化,根据卷积计算和通道数量的变化,设置对应的参数
with tf.variable_scope("layer7-conv4"):
conv4_weights = tf.get_variable("weight", [3, 3, 128, 128],
initializer=tf.truncated_normal_initializer(stddev=0.1))
conv4_biases = tf.get_variable("bias", [128], initializer=tf.constant_initializer(0.0))
conv4 = tf.nn.conv2d(pool3, conv4_weights, strides=[1, 1, 1, 1], padding='SAME')
relu4 = tf.nn.relu(tf.nn.bias_add(conv4, conv4_biases))
with tf.name_scope("layer8-pool4"):
pool4 = tf.nn.max_pool(relu4, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='VALID')
nodes = 6 * 6 * 128
reshaped = tf.reshape(pool4, [-1, nodes])
# 使用变形函数转化结构
# -----------------------第五层---------------------------
with tf.variable_scope('layer9-fc1'):
# 初始化全连接层的参数,隐含节点为1024个
fc1_weights = tf.get_variable("weight", [nodes, 1024],
initializer=tf.truncated_normal_initializer(stddev=0.1))
if regularizer != None: tf.add_to_collection('losses', regularizer(fc1_weights)) # 正则化矩阵
fc1_biases = tf.get_variable("bias", [1024], initializer=tf.constant_initializer(0.1))
# 使用relu函数作为激活函数
fc1 = tf.nn.relu(tf.matmul(reshaped, fc1_weights) + fc1_biases)
# 采用dropout层,减少过拟合和欠拟合的程度,保存模型最好的预测效率
if train: fc1 = tf.nn.dropout(fc1, 0.5)
# -----------------------第六层----------------------------
with tf.variable_scope('layer10-fc2'):
# 同上,不过参数的有变化,根据卷积计算和通道数量的变化,设置对应的参数
fc2_weights = tf.get_variable("weight", [1024, 512],
initializer=tf.truncated_normal_initializer(stddev=0.1))
if regularizer != None: tf.add_to_collection('losses', regularizer(fc2_weights))
fc2_biases = tf.get_variable("bias", [512], initializer=tf.const
没有合适的资源?快使用搜索试试~ 我知道了~
Omniglot数据集分类器的设计与实现——TensorFlow期末考核
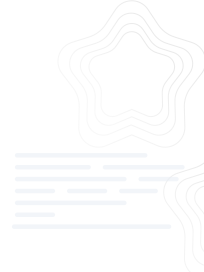
共2000个文件
png:28719个
xml:12个
py:7个

需积分: 45 30 下载量 187 浏览量
2020-04-13
16:25:22
上传
评论 4
收藏 182.77MB ZIP 举报
温馨提示
此次资源为期末考核作品,最终评定4.0绩点。(包括数据集、代码<保证可以运行>、设计文档、训练集)使用数据集为Omniglot,也可以使用自己的数据集进行实现,采用框架,采用多次卷积,最终实现5分类(准确率100%),10分类(99%),30分类(50%多,样本集特征不够明显,不能怪我)。大家可以自己参考
资源推荐
资源详情
资源评论
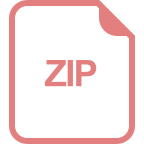
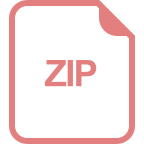
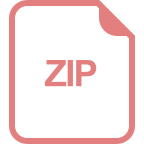
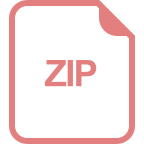
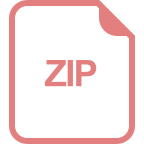
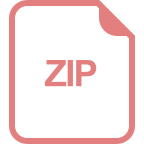
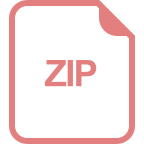
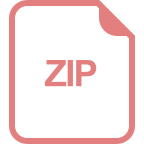
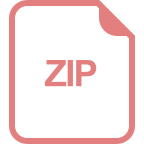
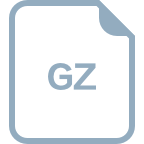
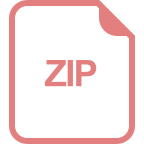
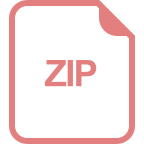
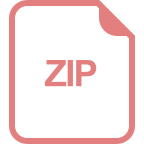
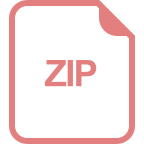
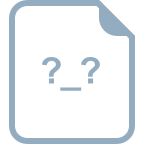
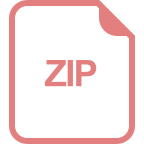
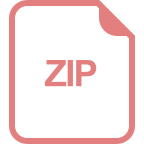
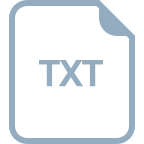
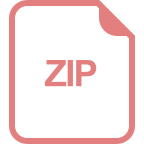
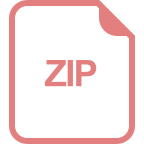
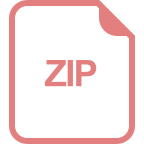
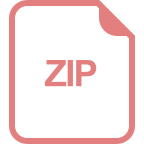
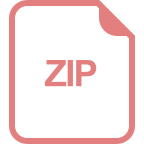
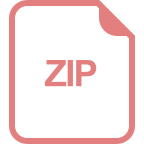
收起资源包目录

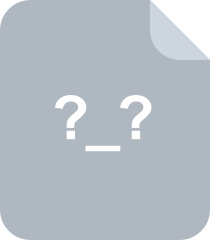
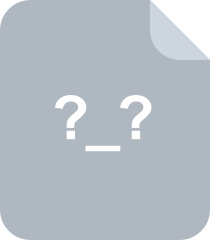
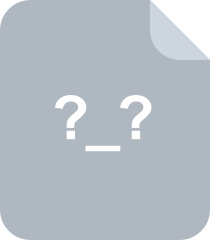
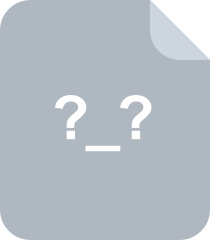
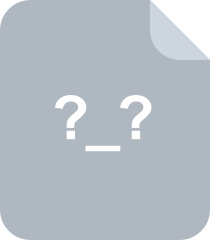
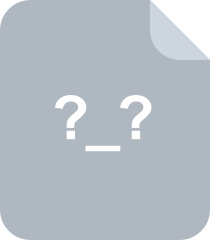
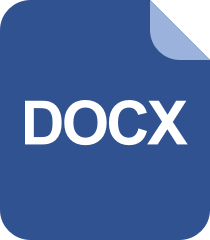
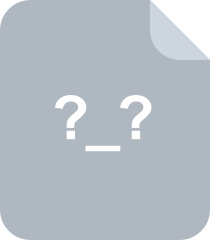
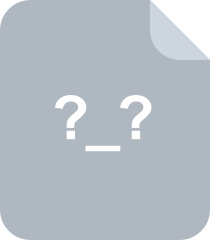
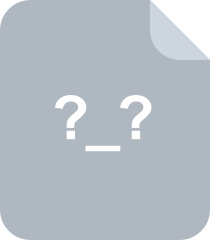
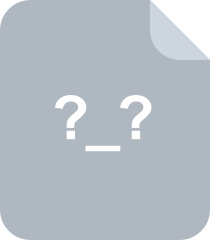
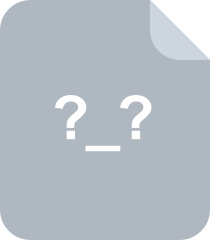
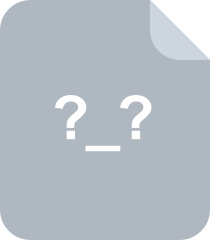
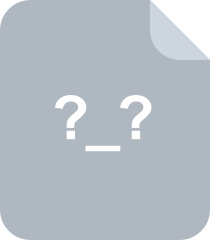
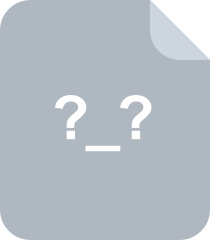
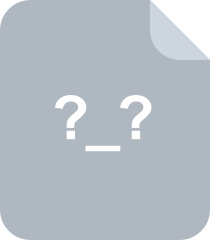
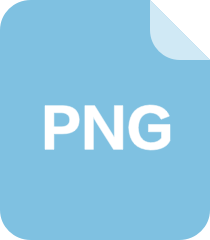
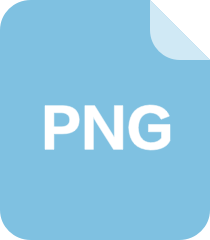
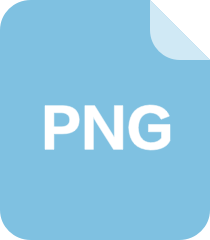
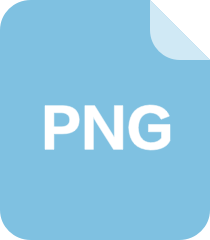
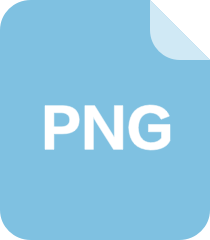
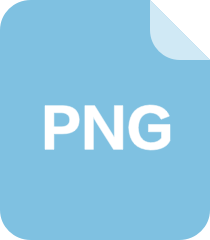
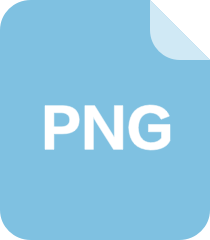
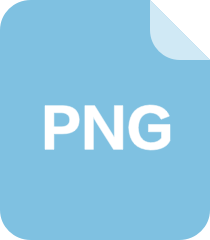
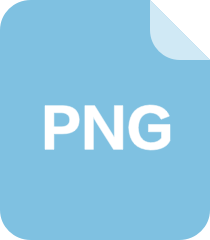
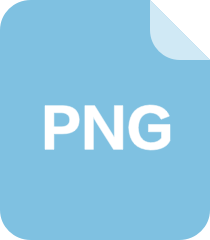
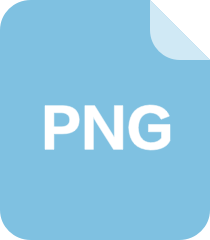
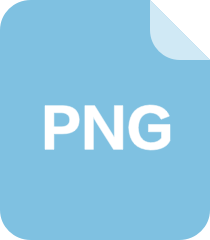
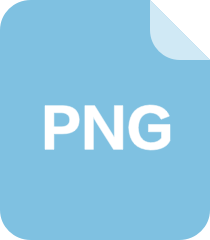
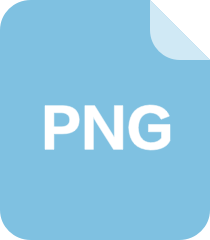
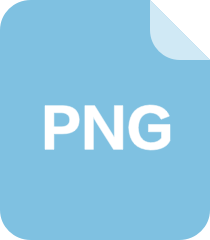
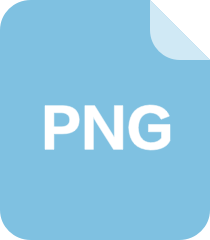
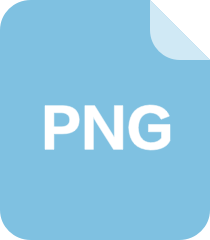
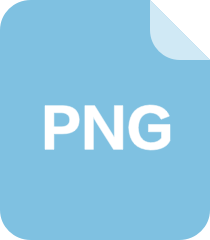
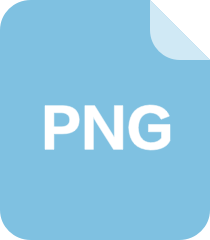
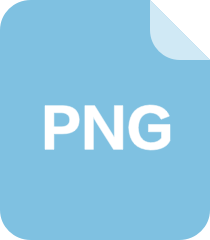
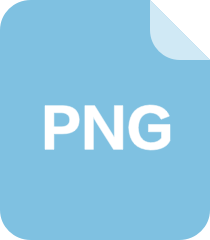
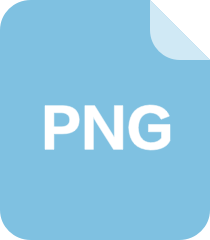
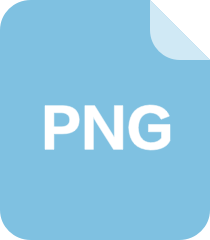
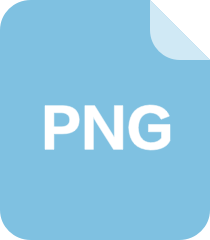
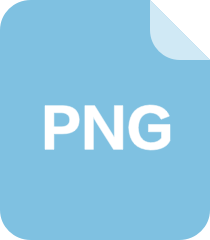
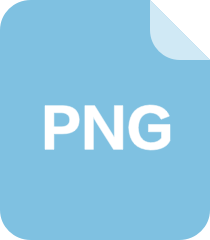
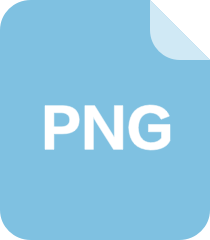
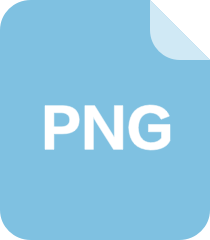
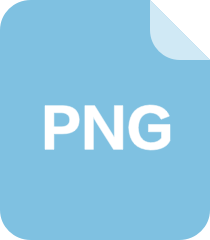
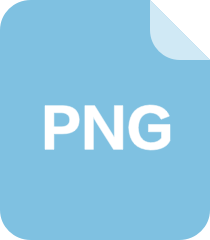
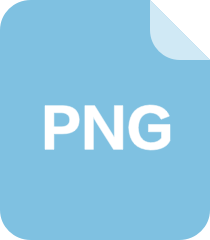
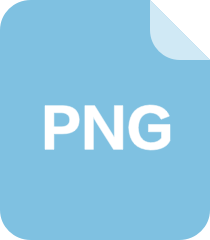
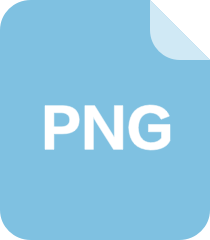
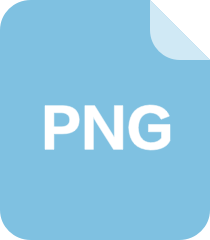
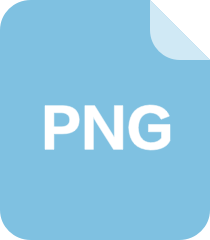
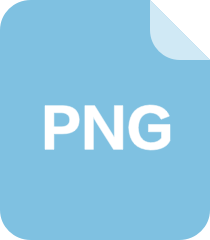
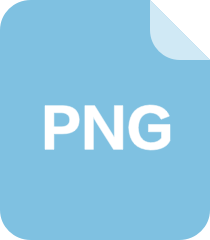
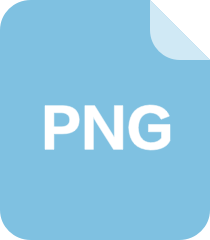
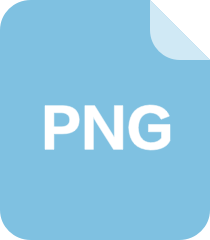
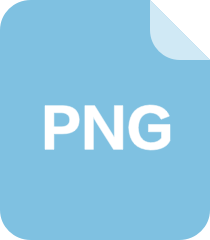
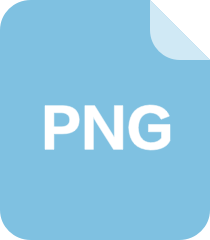
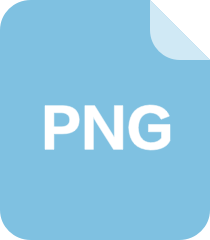
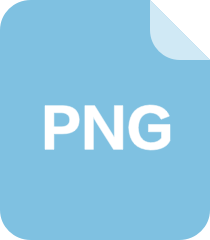
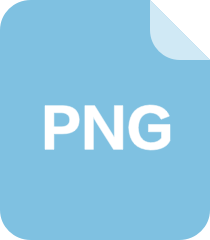
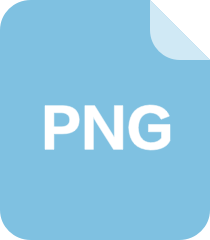
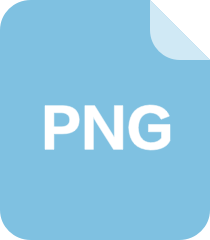
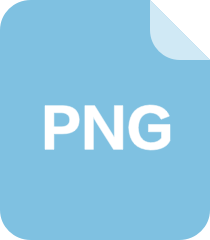
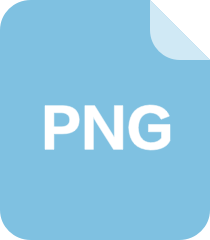
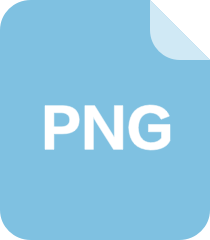
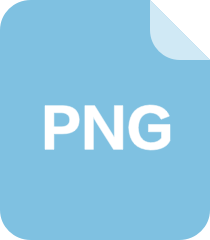
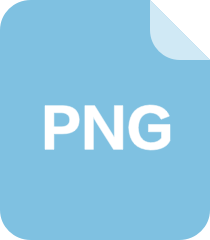
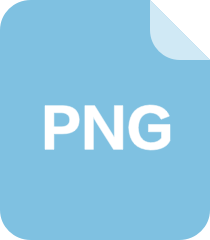
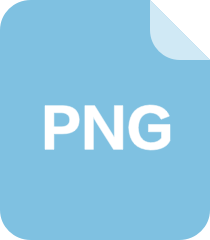
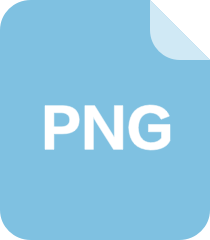
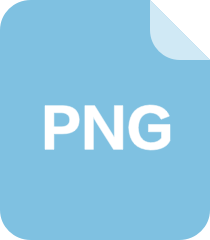
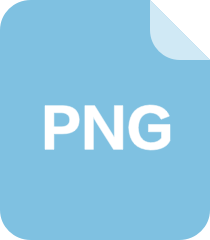
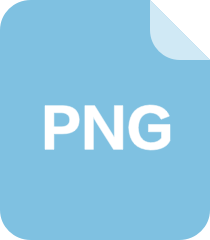
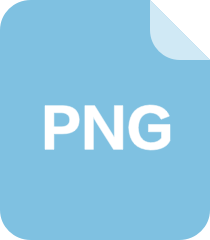
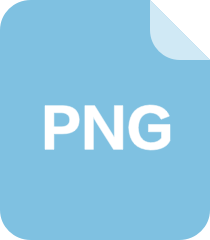
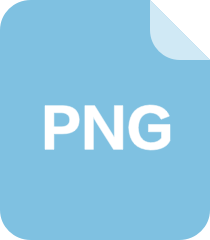
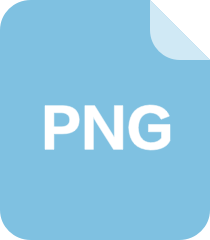
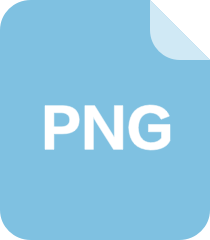
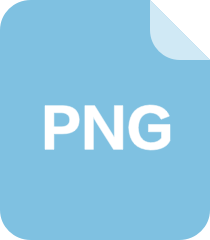
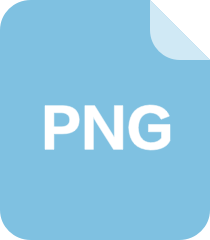
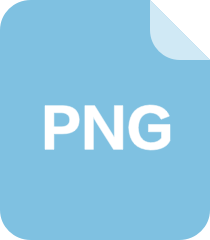
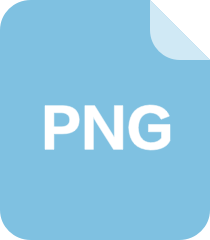
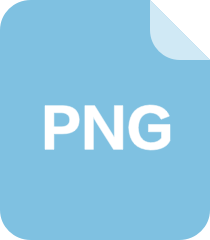
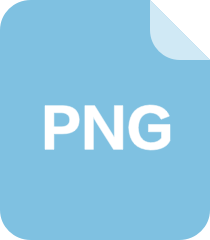
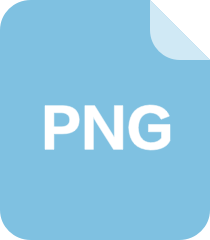
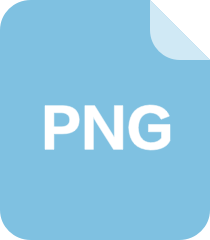
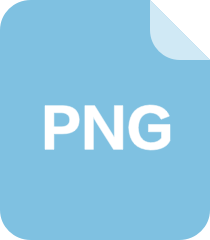
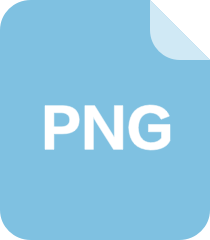
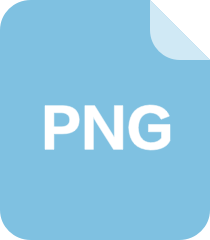
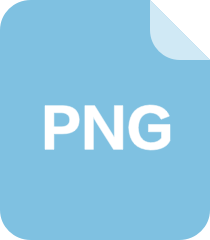
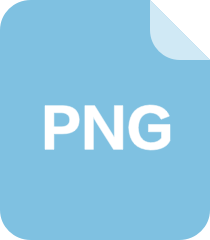
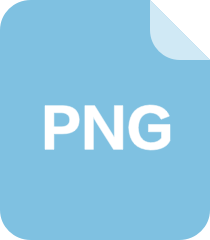
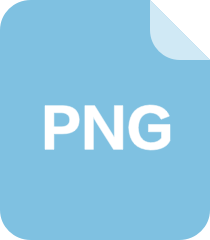
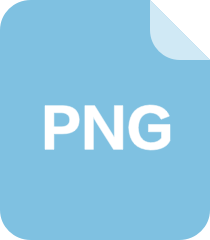
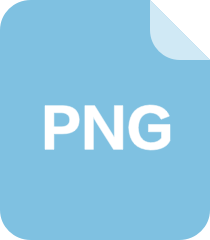
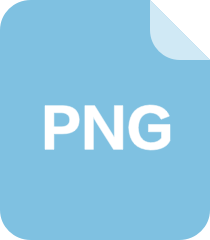
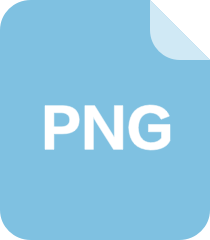
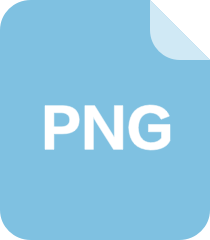
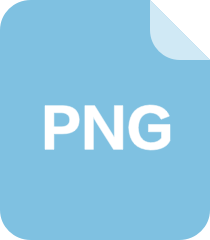
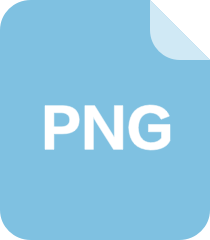
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
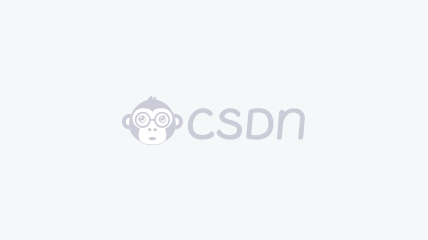

sirwsl
- 粉丝: 8006
- 资源: 13
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

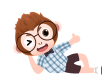
最新资源
- vlmcsd-1113-2020-03-28-Hotbird64(最新版本KMS)
- 433.基于SpringBoot的冷链物流系统(含报告).zip
- com.harmonyos4.exception.PowerFailureException(怎么解决).md
- 使用 Python 字典统计字符串中每个字符的出现次数.docx
- com.harmonyos4.exception.SystemBootFailureException(怎么解决).md
- 球队获胜数据集.zip
- ERR-NULL-POINTER(解决方案).md
- <项目代码>YOLOv8 航拍行人识别<目标检测>
- 计算机网络-socket-inet-master.zip
- Java编程学习路线:从基础到实战全攻略
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


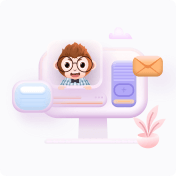
安全验证
文档复制为VIP权益,开通VIP直接复制
