package com.tian.controller;
import com.github.pagehelper.PageInfo;
import com.tian.pojo.Retailer;
import com.tian.service.RetailerService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import javax.servlet.http.HttpServletRequest;
import java.util.Date;
import java.util.List;
import java.util.UUID;
/**
* ClassName: RetailerController
* Description: 零售商的控制器
*
* @author Tianjiao
* @date 2021/11/8 16:01
*/
@Controller
@RequestMapping(value = "/retailer")
public class RetailerController {
@Autowired
private RetailerService retailerService;
@RequestMapping(value = "/toHome", method = RequestMethod.GET)
public String getAllRetailers(Model model, HttpServletRequest request) {
String page = request.getParameter("pageNo");
int pageNo = Integer.parseInt(page.equals("") ? "1" : page);
int size = Integer.parseInt((request.getParameter("size") == null || request.getParameter("size").equals("")) ? "5" : request.getParameter("size"));
List<Retailer> allRetailers = retailerService.getAllRetailers(pageNo, size);
//获得与分页相关参数
PageInfo<Retailer> pageInfo = new PageInfo<>(allRetailers);
//当前页数
model.addAttribute("currentPage", pageInfo.getPageNum());
//总的页数
model.addAttribute("totalPage", pageInfo.getPages());
// 总的数据
model.addAttribute("total", pageInfo.getTotal());
model.addAttribute("allRetailers", allRetailers);
return "home";
}
@RequestMapping(value = "/fuzzyQuery", method = RequestMethod.POST)
public String fuzzyQuery(Model model, HttpServletRequest request) {
Retailer retailer = new Retailer();
retailer.setName(request.getParameter("name"));
retailer.setTelPhone(request.getParameter("telPhone"));
retailer.setAddress(request.getParameter("address"));
retailer.setStatus(request.getParameter("status"));
int pageNo = Integer.parseInt(request.getParameter("currentPage"));
System.out.println("currentPage: " + pageNo);
List<Retailer> allRetailers = retailerService.fuzzyQuery(retailer, pageNo, 5);
System.out.println("allRetailers: " + allRetailers);
//获得与分页相关参数
PageInfo<Retailer> pageInfo = new PageInfo<>(allRetailers);
//当前页数
model.addAttribute("currentPage", pageInfo.getPageNum());
//总的页数
model.addAttribute("totalPage", pageInfo.getPages());
// 总的数据
model.addAttribute("total", pageInfo.getTotal());
model.addAttribute("allRetailers", allRetailers);
return "home";
}
@RequestMapping(value = "/toEditPage", method = RequestMethod.GET)
public String toEdit(Model model, HttpServletRequest request) {
model.addAttribute("telPhone", request.getParameter("telPhone"));
model.addAttribute("address", request.getParameter("address"));
model.addAttribute("name", request.getParameter("name"));
model.addAttribute("status", request.getParameter("status"));
model.addAttribute("pageNo", request.getParameter("pageNo"));
model.addAttribute("size", request.getParameter("size"));
model.addAttribute("retailerId", request.getParameter("retailerId"));
return "edit";
}
@RequestMapping(value = "/deleteById", method = RequestMethod.GET)
public String deleteById(HttpServletRequest request) {
String retailerId = request.getParameter("retailerId");
retailerService.deleteRetailerById(retailerId);
return "forward:/retailer/toHome";
}
@RequestMapping(value = "/updateById", method = RequestMethod.POST)
public String updateById(HttpServletRequest request) {
String pageNo = request.getParameter("pageNo");
String size = request.getParameter("size");
Retailer retailer = new Retailer();
retailer.setName(request.getParameter("name"));
retailer.setTelPhone(request.getParameter("telPhone"));
retailer.setAddress(request.getParameter("address"));
retailer.setStatus(request.getParameter("status"));
retailer.setRetailerId(request.getParameter("retailerId"));
retailerService.updateRetailerById(retailer);
return "redirect:/retailer/toHome?pageNo=" + pageNo + "&size=" + size + "";
}
@RequestMapping(value = "/toAddRetailerPage", method = RequestMethod.GET)
public String toAddRetailerPage() {
return "addRegister";
}
@RequestMapping(value = "/addNewRetailer", method = RequestMethod.POST)
public String addNewRetailer(HttpServletRequest request) {
Retailer retailer = new Retailer();
retailer.setRetailerId(UUID.randomUUID().toString());
retailer.setCreateTime(new Date());
retailer.setStatus("1");
retailer.setName(request.getParameter("name"));
retailer.setAddress(request.getParameter("address"));
retailer.setTelPhone(request.getParameter("telPhone"));
retailerService.addNewRetailer(retailer);
return "redirect:/retailer/toHome?pageNo=1";
}
}
《基于SSM+MySQL+JSP的水果商城管理系统详解》 在信息技术日新月异的今天,企业对高效、便捷的管理系统的需求日益增强。本文将详细介绍一个基于SSM(Spring、SpringMVC、MyBatis)框架,结合MySQL数据库以及JSP技术实现的水果商城管理系统。该系统旨在提供全面的业务流程支持,包括用户注册与登录、零售商管理、用户设置、货物管理和购销合同等关键功能。 一、系统架构 SSM框架是Java领域中广泛应用的开发框架组合,它整合了Spring的依赖注入(DI)、SpringMVC的模型视图控制器(MVC)以及MyBatis的持久层操作。Spring作为核心,负责管理对象及事务处理;SpringMVC处理HTTP请求,实现前端逻辑与后端业务的解耦;MyBatis则作为数据访问层,简化了SQL操作,提高了数据库交互效率。 二、功能模块 1. 注册与登录功能:系统提供用户注册和登录服务,通过验证用户名和密码确保用户身份安全。注册时,系统会检查用户名的唯一性,登录时,利用MD5或更安全的加密算法对密码进行存储和验证,保护用户信息不被泄露。 2. 零售商管理:管理员可以添加、删除和修改零售商信息,包括零售商名称、地址、联系方式等,以便于对供应商进行统一管理,确保供应链的稳定。 3. 用户设置:用户在登录后可进行个人资料的编辑,如修改密码、完善个人信息等,提升用户体验。 4. 货物管理:此模块涵盖了商品的上架、下架、库存管理、价格调整等功能。管理员可以通过后台管理系统对商品信息进行维护,确保商品信息的准确性和实时性。 5. 购销合同:系统支持购销合同的创建、审批和管理,合同内容包括采购数量、单价、交货日期等,有助于规范交易行为,保障双方权益。 三、数据库设计 使用MySQL数据库来存储系统数据,包括用户表、零售商表、商品表、购销合同表等。合理的设计数据库结构和建立关联关系,可以有效提高查询效率,保证数据的一致性和完整性。 四、JSP技术应用 JSP(JavaServer Pages)是Java Web开发中的视图技术,用于生成动态网页。在本系统中,JSP结合Servlet处理用户请求,展示页面内容,实现前后端的交互。 总结,基于SSM+MySQL+JSP的水果商城管理系统,利用先进的技术栈实现了全方位的商城管理功能,提高了业务处理效率,降低了运营成本。其灵活的架构设计和强大的功能支持,使得系统具有良好的可扩展性和可维护性,为企业提供了可靠的数字化解决方案。

























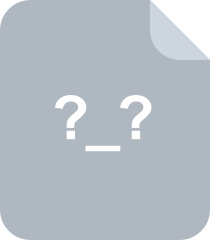
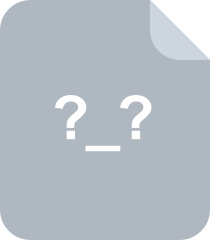
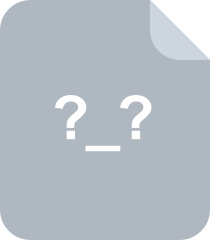
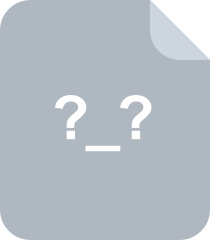
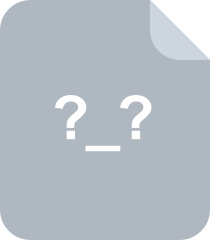
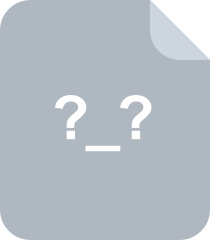
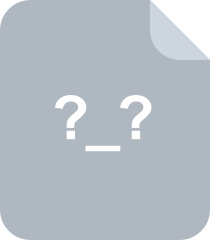
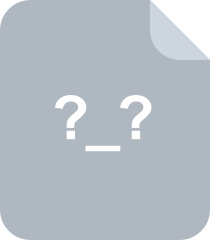
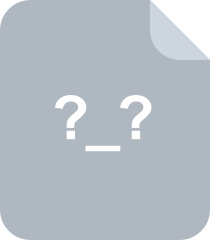
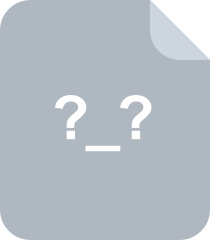
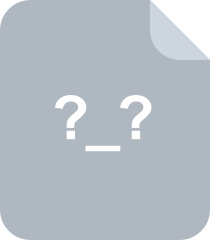
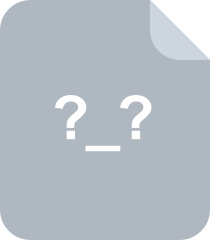
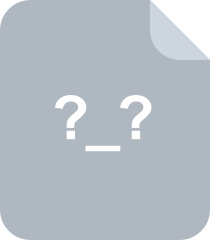
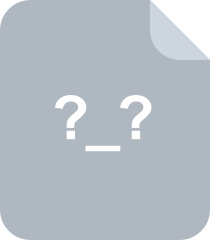
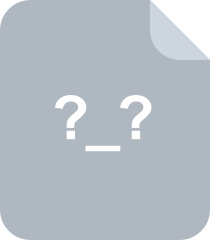
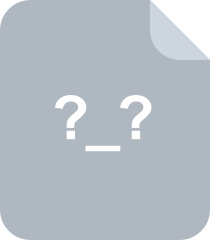
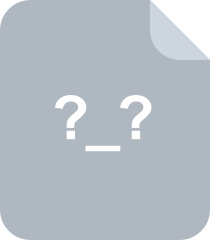
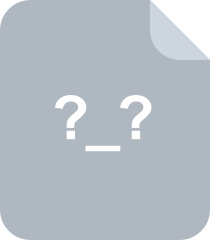
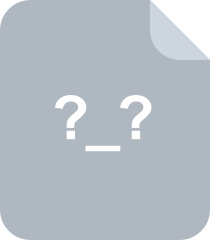
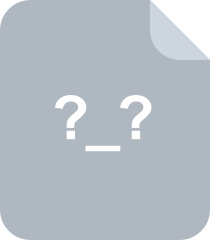
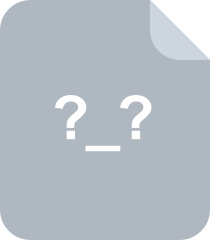
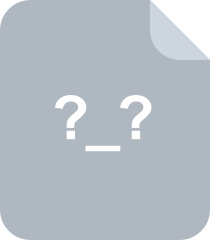
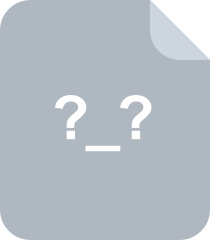
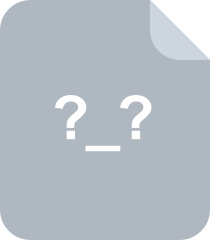
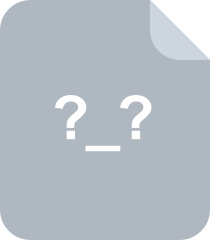
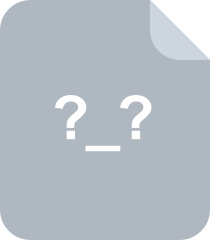
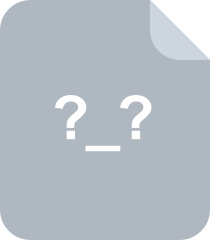
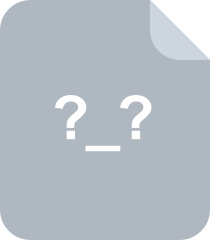
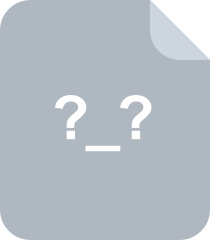
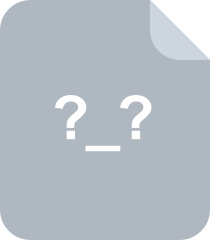
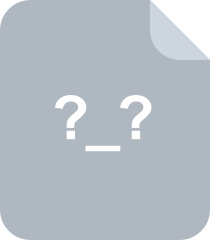
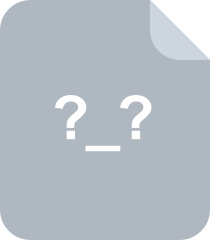
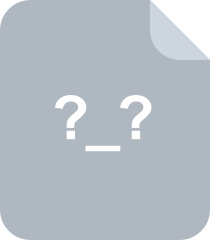
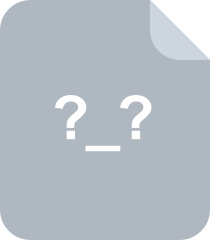
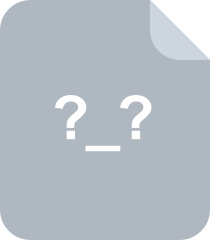
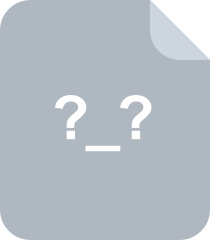
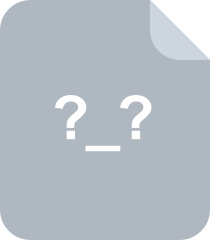
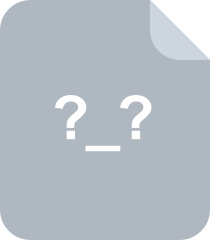
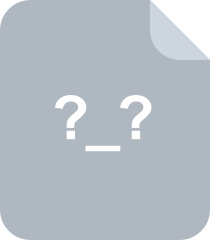
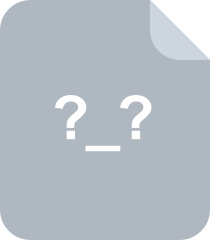
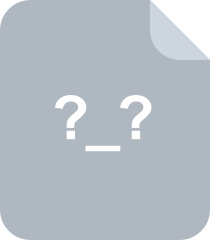
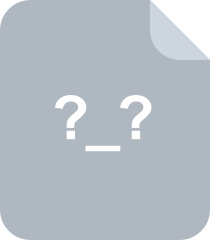
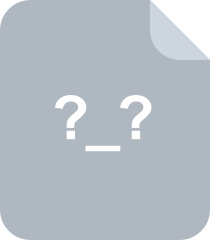
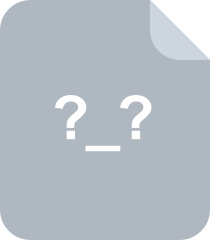
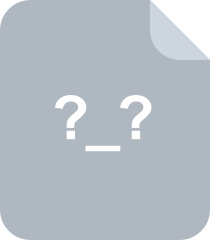
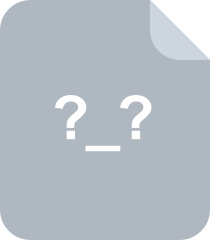
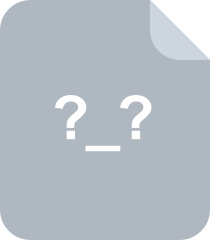
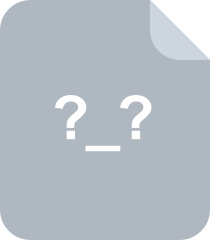
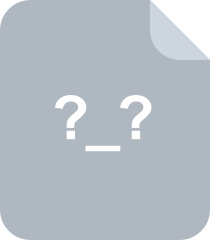
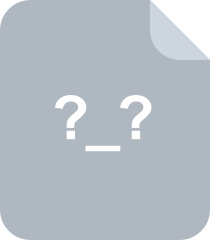
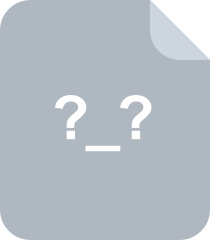
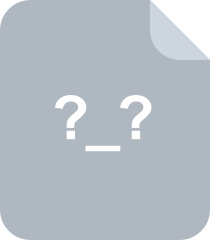
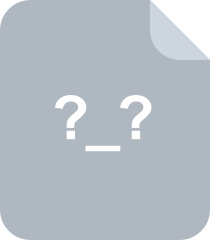
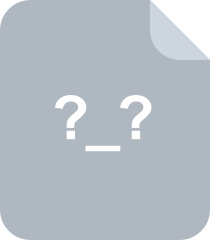
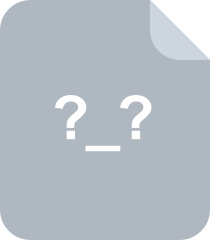
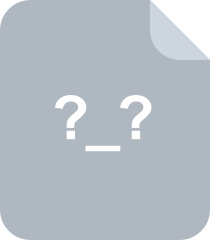
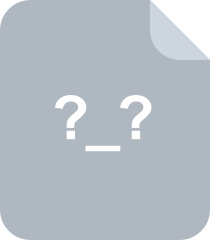
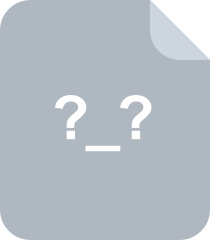
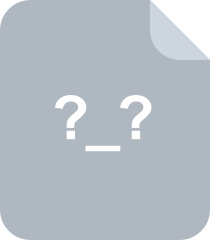
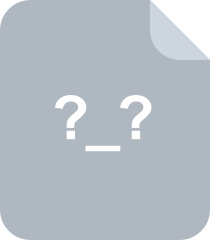
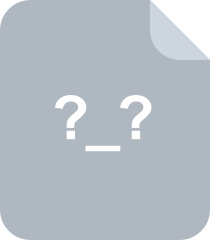
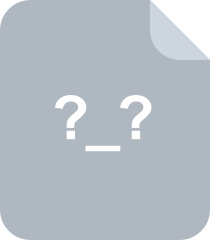
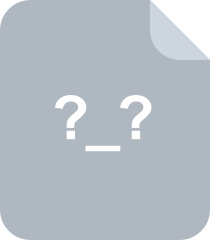
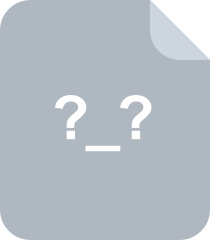
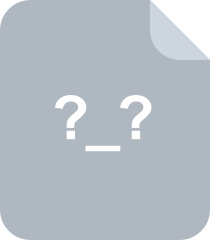
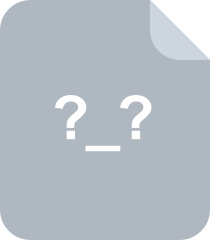
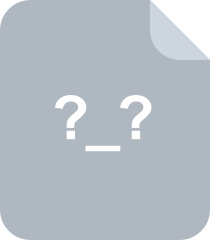
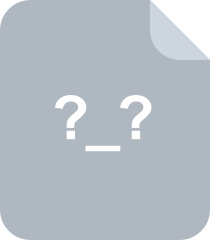
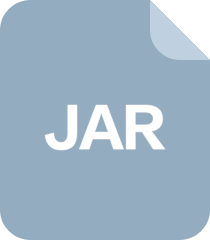
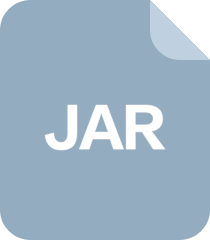
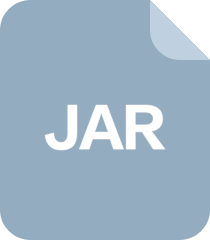
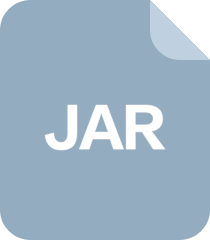
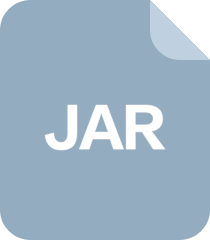
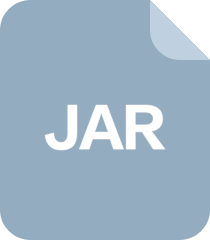
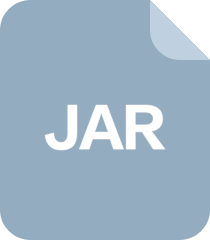
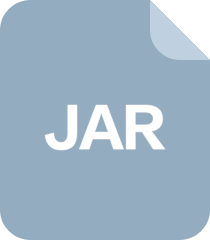
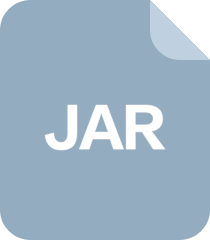
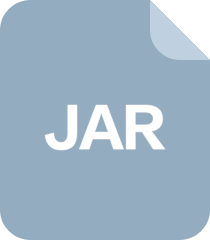
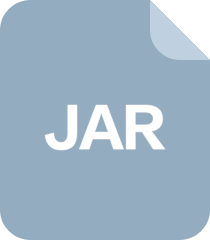
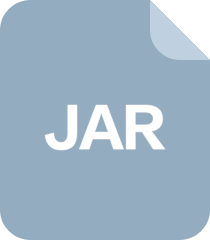
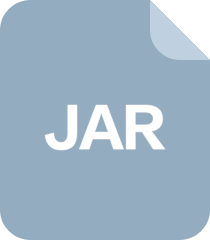
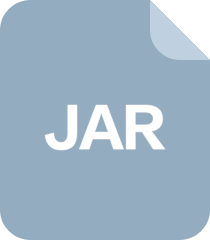
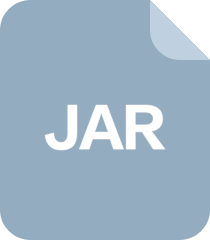
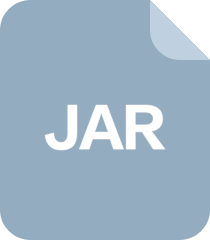
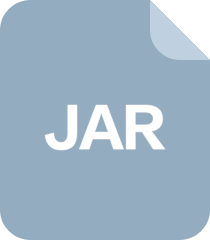
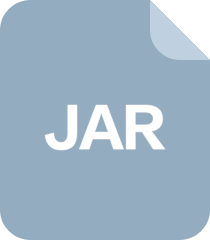
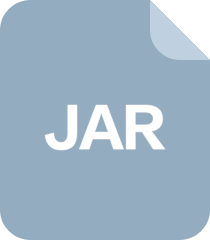
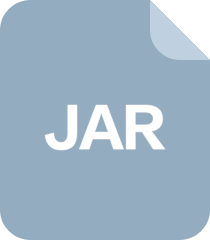
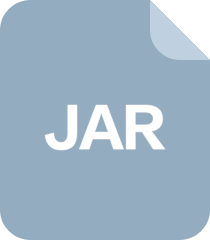
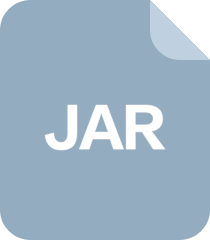
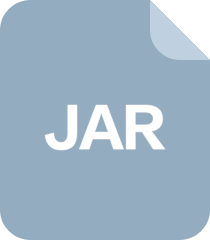
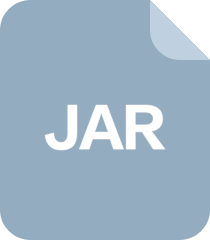
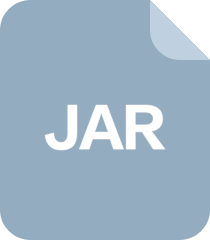
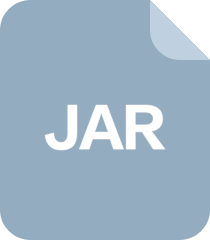
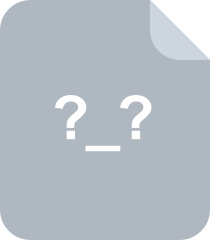
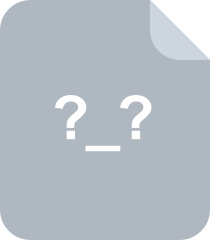
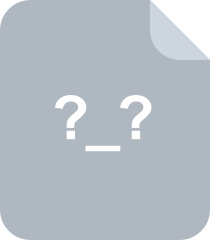
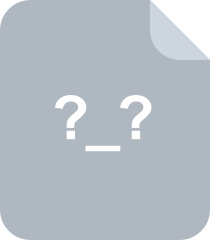
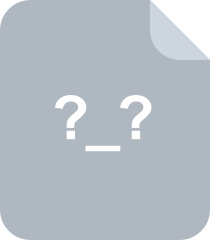
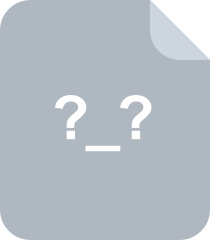
- 1
- 2
- 3
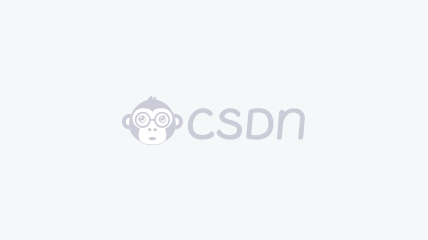
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整


- 粉丝: 5207
- 资源: 5164
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

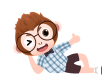
最新资源
- 管家婆辉煌pos1322安卓版.zip
- 专业绘图软件-EdrawMax免安装版.zip
- 管家婆辉煌pos15.11(windows版).zip
- 管家婆辉煌pos15.2(windows版).zip
- zabbix 7.0.9 rpm 安装包
- 管家婆辉煌pos安卓版15.0.zip
- 管家婆辉煌pos安卓版13.32.zip
- 管家婆辉煌pos1332.zip
- 管家婆辉煌移动pos安卓版 1.0.zip
- 管家婆辉煌移动pos安卓版 5.1.zip
- 管家婆辉煌pos手机版 2.1.zip
- BS_管家婆安卓POS收银机安装包CCv15.11.1.zip
- BS_管家婆安卓POS收银机安装包v15.2.zip
- 数字化电子(医疗服务(住院))发票版式文件说明
- BS_管家婆辉煌安卓手机V15.2.zip
- BS_管家婆辉煌安卓手机V15.11.1.zip

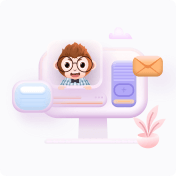
