package com.action;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import javax.annotation.Resource;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import com.entity.Article;
import com.entity.Cart;
import com.entity.Cate;
import com.entity.City;
import com.entity.Details;
import com.entity.Jiancai;
import com.entity.Orders;
import com.entity.Topic;
import com.entity.Users;
import com.service.ArticleService;
import com.service.CartService;
import com.service.CateService;
import com.service.PeihuoService;
import com.service.CityService;
import com.service.DetailsService;
import com.service.JiancaiService;
import com.service.OrdersService;
import com.service.TopicService;
import com.service.UsersService;
import com.util.VeDate;
//定义为控制器
@Controller
// 设置路径
@RequestMapping("/index")
public class IndexAction extends BaseAction {
@Autowired
@Resource
private UsersService usersService;
@Autowired
@Resource
private ArticleService articleService;
@Autowired
@Resource
private CateService cateService;
@Autowired
@Resource
private CityService cityService;
@Autowired
@Resource
private PeihuoService peihuoService;
@Autowired
@Resource
private JiancaiService jiancaiService;
@Autowired
@Resource
private CartService cartService;
@Autowired
@Resource
private OrdersService ordersService;
@Autowired
@Resource
private DetailsService detailsService;
@Autowired
@Resource
private TopicService topicService;
// 公共方法 提供公共查询数据
private void front() {
this.getRequest().setAttribute("title", "优品果蔬网-力作中国最大的果蔬网站");
List<Cate> cateList = this.cateService.getAllCate();
this.getRequest().setAttribute("cateList", cateList);
List<Jiancai> hotList = this.jiancaiService.getJiancaiByHot();
Collections.shuffle(hotList);
this.getRequest().setAttribute("hotList", hotList);
}
// 首页显示
@RequestMapping("index.action")
public String index() {
this.front();
List<Cate> cateList = this.cateService.getCateFront();
List<Cate> frontList = new ArrayList<Cate>();
for (Cate cate : cateList) {
List<Jiancai> flimList = this.jiancaiService.getJiancaiByCate(cate.getCateid());
cate.setFlimList(flimList);
frontList.add(cate);
}
this.getRequest().setAttribute("frontList", frontList);
return "users/index";
}
// 首页显示
@RequestMapping("network.action")
public String network() {
this.front();
List<Cate> cateList = this.cateService.getCateFront();
List<Cate> frontList = new ArrayList<Cate>();
for (Cate cate : cateList) {
List<Jiancai> flimList = this.jiancaiService.getJiancaiByCate(cate.getCateid());
cate.setFlimList(flimList);
frontList.add(cate);
}
this.getRequest().setAttribute("frontList", frontList);
return "users/network";
}
// 公告
@RequestMapping("article.action")
public String article(String number) {
this.front();
List<Article> articleList = new ArrayList<Article>();
List<Article> tempList = this.articleService.getAllArticle();
int pageNumber = tempList.size();
int maxPage = pageNumber;
if (maxPage % 12 == 0) {
maxPage = maxPage / 12;
} else {
maxPage = maxPage / 12 + 1;
}
if (number == null) {
number = "0";
}
int start = Integer.parseInt(number) * 12;
int over = (Integer.parseInt(number) + 1) * 12;
int count = pageNumber - over;
if (count <= 0) {
over = pageNumber;
}
for (int i = start; i < over; i++) {
Article x = tempList.get(i);
articleList.add(x);
}
String html = "";
StringBuffer buffer = new StringBuffer();
buffer.append(" 共为");
buffer.append(maxPage);
buffer.append("页 共有");
buffer.append(pageNumber);
buffer.append("条 当前为第");
buffer.append((Integer.parseInt(number) + 1));
buffer.append("页 ");
if ((Integer.parseInt(number) + 1) == 1) {
buffer.append("首页");
} else {
buffer.append("<a href=\"index/article.action?number=0\">首页</a>");
}
buffer.append(" ");
if ((Integer.parseInt(number) + 1) == 1) {
buffer.append("上一页");
} else {
buffer.append("<a href=\"index/article.action?number=" + (Integer.parseInt(number) - 1) + "\">上一页</a>");
}
buffer.append(" ");
if (maxPage <= (Integer.parseInt(number) + 1)) {
buffer.append("下一页");
} else {
buffer.append("<a href=\"index/article.action?number=" + (Integer.parseInt(number) + 1) + "\">下一页</a>");
}
buffer.append(" ");
if (maxPage <= (Integer.parseInt(number) + 1)) {
buffer.append("尾页");
} else {
buffer.append("<a href=\"index/article.action?number=" + (maxPage - 1) + "\">尾页</a>");
}
html = buffer.toString();
this.getRequest().setAttribute("html", html);
this.getRequest().setAttribute("articleList", articleList);
return "users/article";
}
// 阅读公告
@RequestMapping("read.action")
public String read(String id) {
this.front();
Article article = this.articleService.getArticleById(id);
article.setHits("" + (Integer.parseInt(article.getHits()) + 1));
this.articleService.updateArticle(article);
this.getRequest().setAttribute("article", article);
return "users/read";
}
// 准备登录
@RequestMapping("preLogin.action")
public String prelogin() {
this.front();
return "users/login";
}
// 用户登录
@RequestMapping("login.action")
public String login() {
this.front();
String username = this.getRequest().getParameter("username");
String password = this.getRequest().getParameter("password");
Users u = new Users();
u.setUsername(username);
List<Users> usersList = this.usersService.getUsersByCond(u);
if (usersList.size() == 0) {
this.getSession().setAttribute("message", "用户名不存在");
return "redirect:/index/preLogin.action";
} else {
Users users = usersList.get(0);
if (password.equals(users.getPassword())) {
this.getSession().setAttribute("userid", users.getUsersid());
this.getSession().setAttribute("username", users.getUsername());
this.getSession().setAttribute("users", users);
return "redirect:/index/index.action";
} else {
this.getSession().setAttribute("message", "密码错误");
return "redirect:/index/preLogin.action";
}
}
}
// 准备注册
@RequestMapping("preReg.action")
public String preReg() {
this.front();
return "users/register";
}
// 用户注册
@RequestMapping("register.action")
public String register(Users users) {
this.front();
Users u = new Users();
u.setUsername(users.getUsername());
List<Users> usersList = this.usersService.getUsersByCond(u);
if (usersList.size() == 0) {
users.setRegdate(VeDate.getStringDateShort());
this.usersService.insertUsers(users);
} else {
this.getSession().setAttribute("message", "用户名已存在");
return "redirect:/index/preReg.action";
}
return "redirect:/index/preLogin.action";
}
// 退出登录
@RequestMapping("exit.action")
public String exit() {
this.front();
this.getSession().removeAttribute("userid");
this.getSession().removeAttribute("username");
this.getSession().removeAttribute("users");
return "index";
}
// 准备修改密码
@RequestMapping("prePwd.action")
public String prePwd() {
this.front();
if (this.getSession().getAttribute("userid") == null) {
return "redirect:/index/preLogin.action";
}
return "users/editpwd";
}
// 修改密码
@RequestMapping("editpwd.action")
public String editpwd() {
this.front()
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
项目介绍 本项目分为前后台,前台为普通用户登录,后台为管理员登录; 管理员角色包含以下功能: 管理员登录,管理员管理,网站用户管理,新闻公告管理,果蔬类型管理,城市信息管理,配货点管理,果蔬商品管理,果蔬订单管理,果蔬评价管理等功能。 用户角色包含以下功能: 用户首页,用户登录注册,查看某一个商品,加入购物车,填写配货信息,查看我的订单,查看系统公告,查看销售网络等功能。 环境需要 1.运行环境:最好是java jdk 1.8,我们在这个平台上运行的。其他版本理论上也可以。 2.IDE环境:IDEA,Eclipse,Myeclipse都可以。推荐IDEA; 3.tomcat环境:Tomcat 7.x,8.x,9.x版本均可 4.硬件环境:windows 7/8/10 1G内存以上;或者 Mac OS; 5.数据库:MySql 5.7版本; 6.是否Maven项目:否; 技术栈 1. 后端:Spring+SpringMVC+Mybatis 2. 前端:JSP+CSS+JavaScript+jQuery 使用说明 1. 使用Navicat或者其它工具,在mysql中创建对
资源推荐
资源详情
资源评论
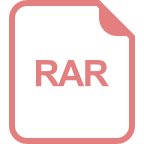
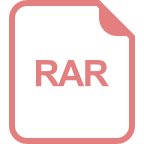
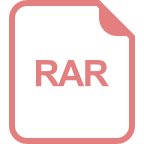
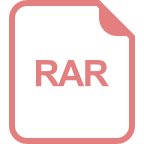
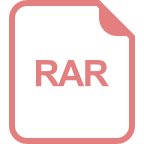
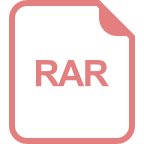
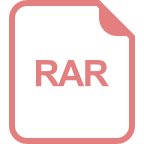
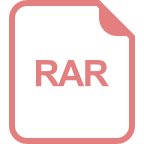
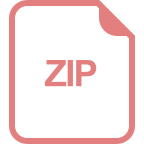
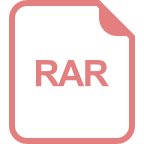
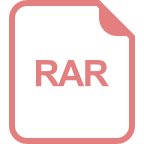
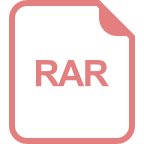
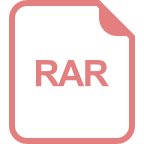
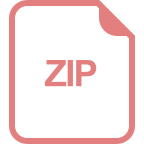
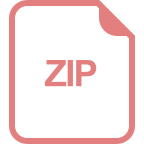
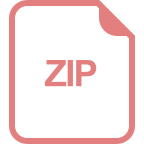
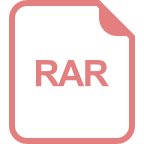
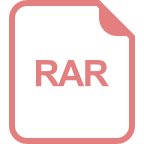
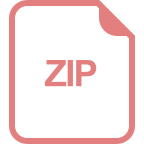
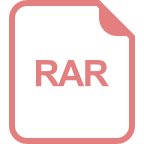
收起资源包目录

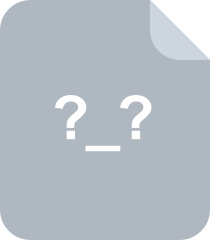
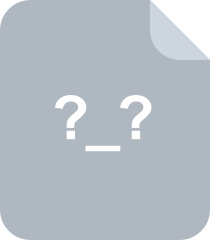
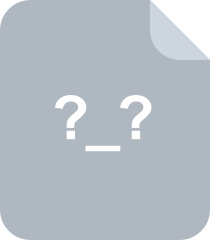
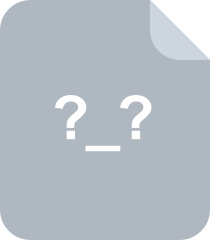
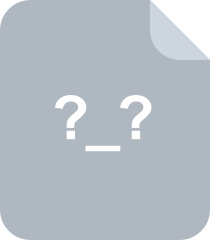
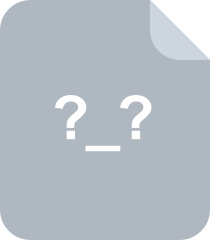
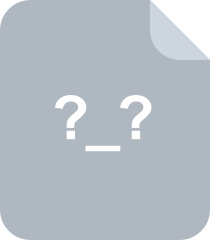
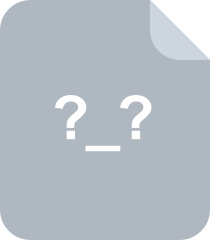
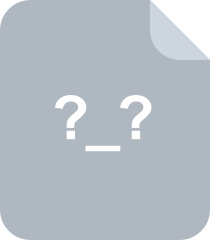
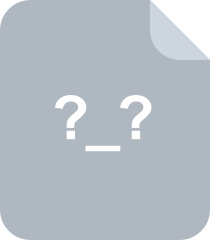
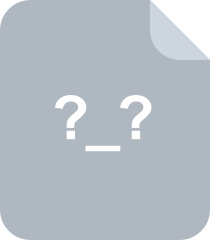
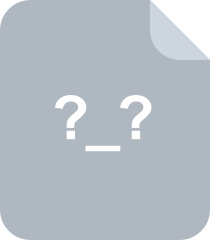
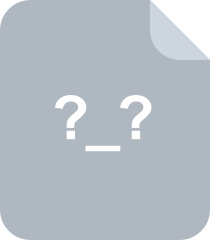
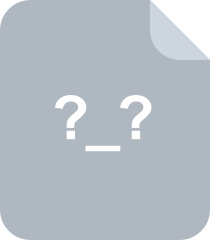
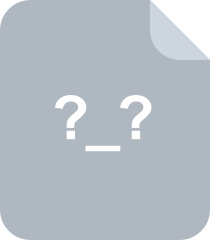
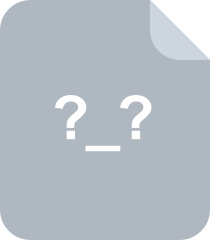
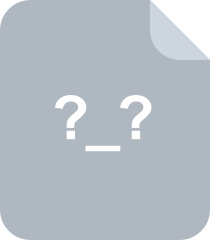
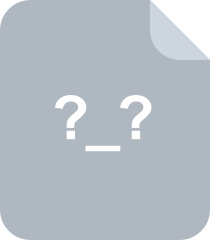
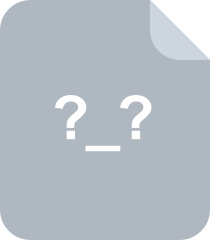
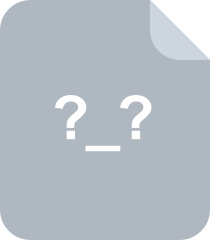
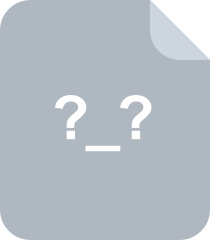
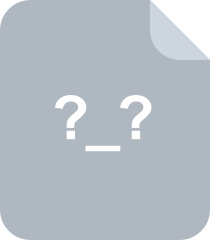
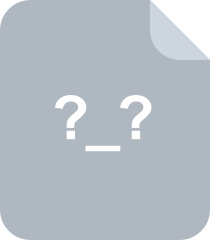
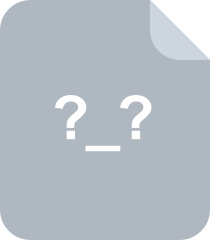
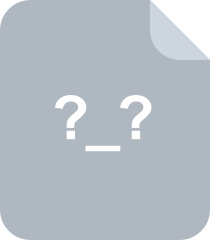
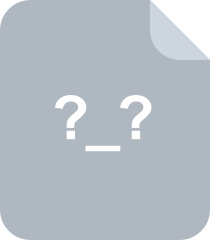
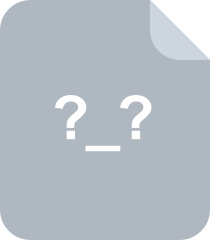
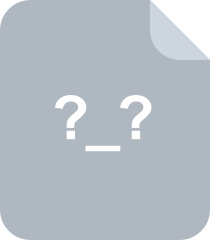
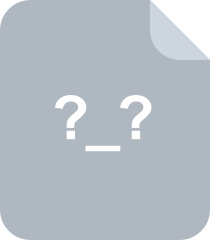
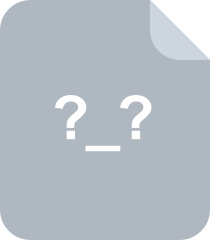
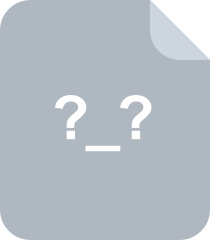
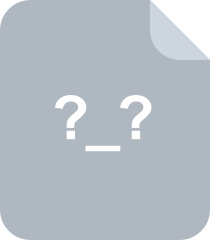
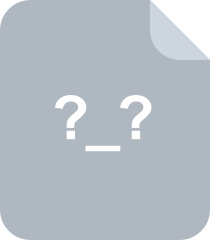
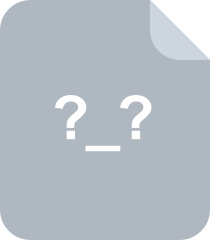
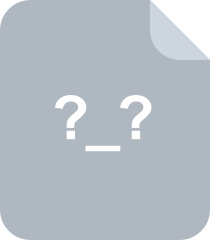
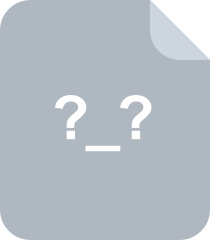
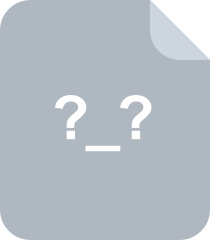
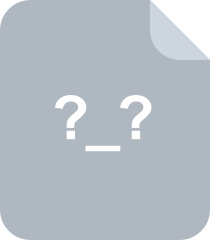
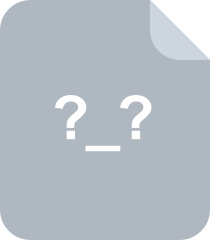
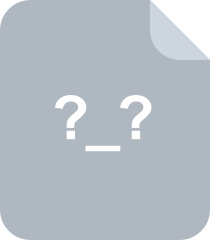
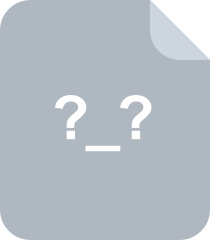
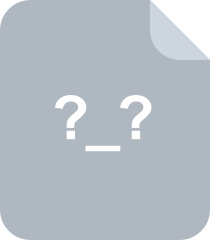
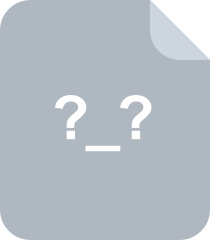
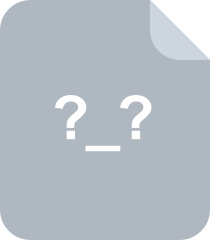
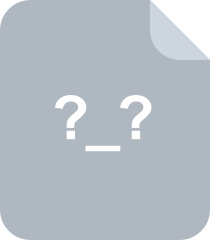
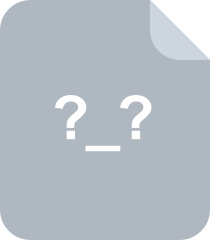
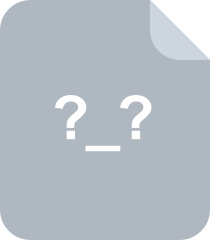
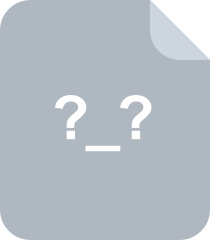
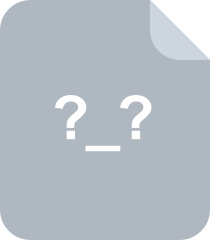
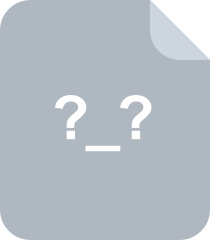
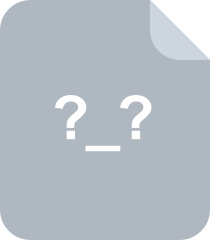
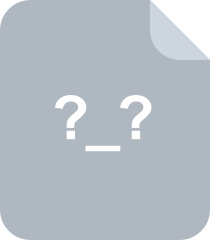
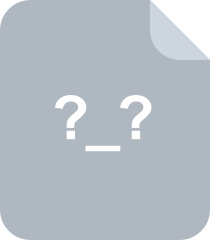
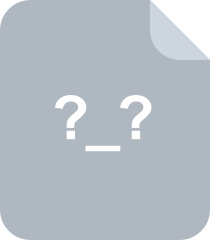
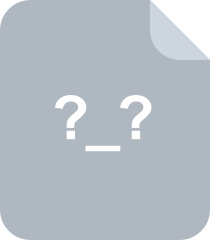
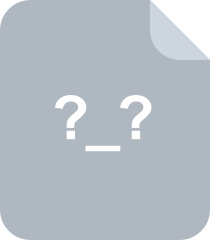
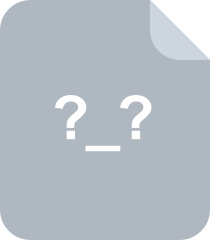
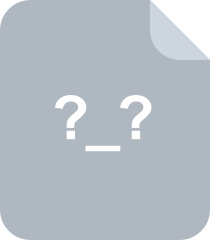
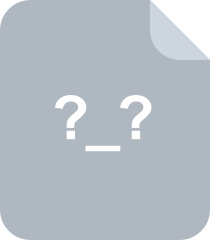
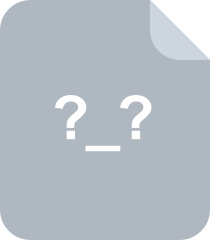
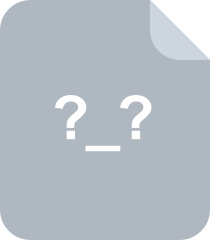
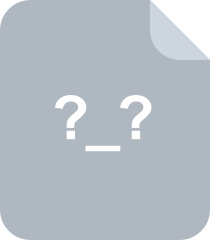
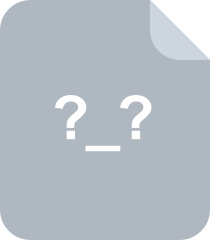
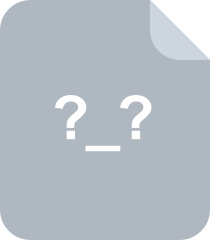
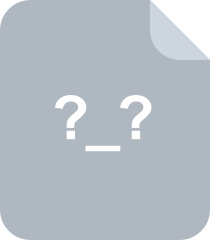
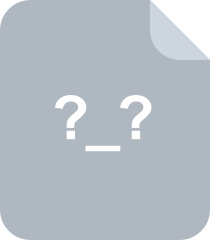
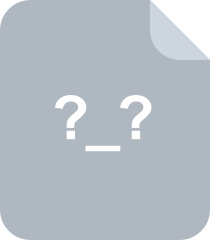
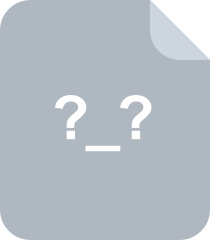
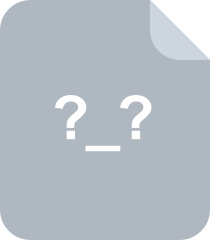
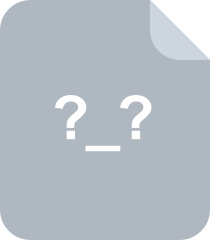
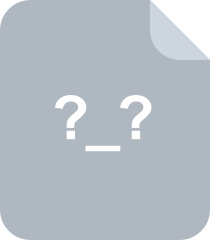
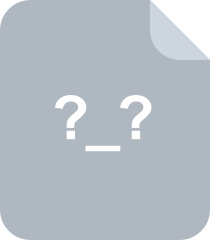
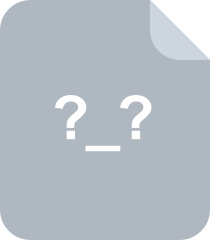
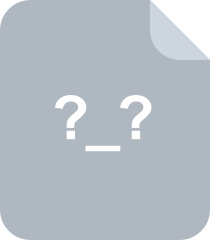
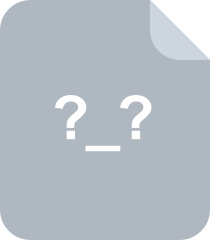
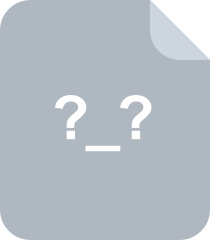
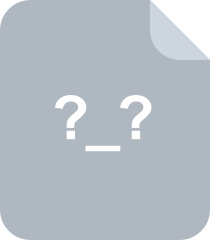
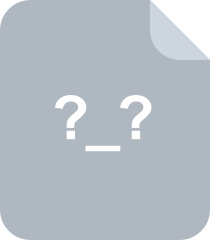
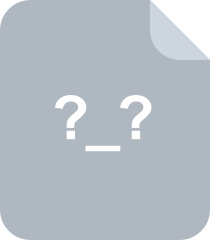
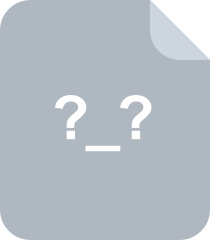
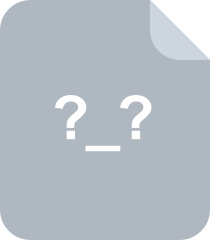
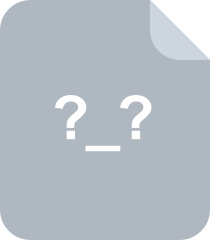
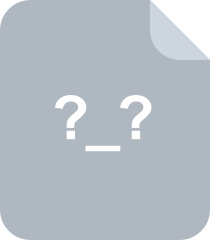
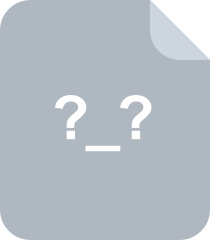
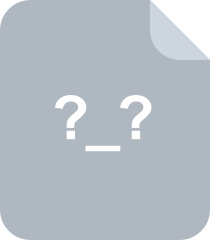
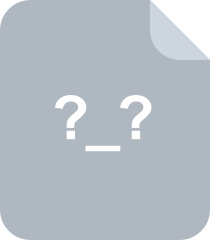
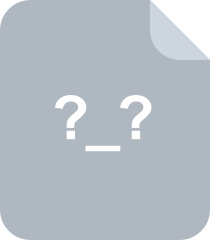
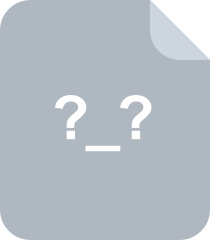
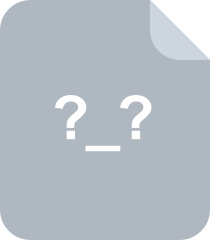
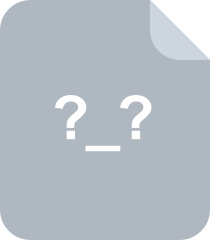
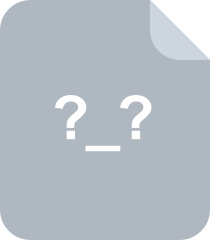
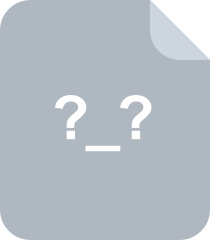
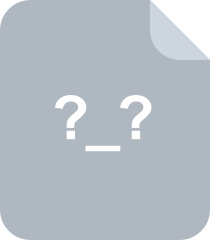
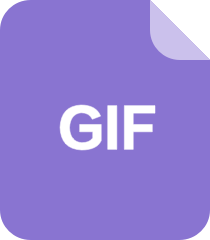
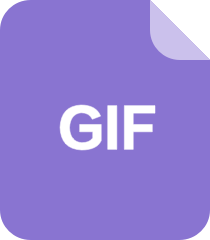
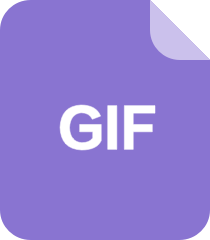
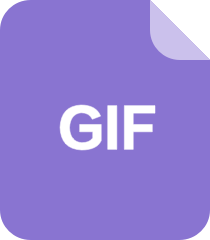
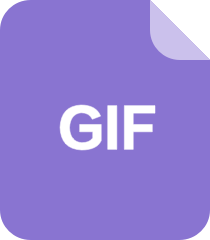
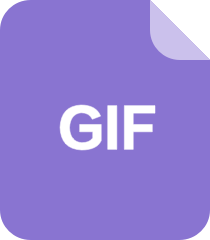
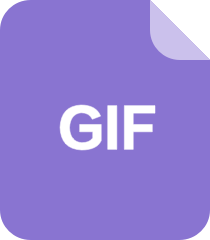
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
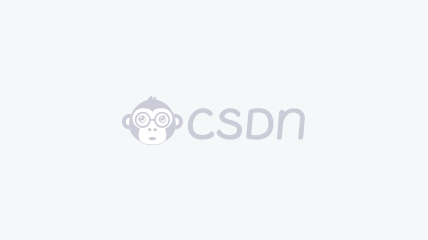
- m0_543726912023-01-09资源简直太好了,完美解决了当下遇到的难题,这样的资源很难不支持~

beyondwild
- 粉丝: 9862
- 资源: 4911
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

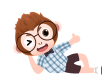
最新资源
- 数据库设计管理课程设计系统设计报告(powerdesign+sql+DreamweaverCS)医院管理系统设计与开发
- VMware 学习教程(入门到实践)
- 数据库设计管理课程设计系统设计报告(powerdesign+sql+DreamweaverCS)学生选课管理系统2
- LLMS&隐写术12345
- 关于内置谷歌中文输入法apk
- 数据库设计管理课程设计系统设计报告(powerdesign+sql+DreamweaverCS)学生选课管理系统
- 基于realsense d435i相机和yolov5的目标检测机器人项目含运行说明(自动返回位置信息).zip
- 2025年Gartner重要战略技术趋势及对企业数字化转型的影响
- 数据库设计管理课程设计系统设计报告(powerdesign+sql+DreamweaverCS)学生管理系统设计与开发2
- Java 学习教程(基础到实践)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


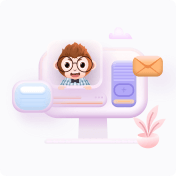
安全验证
文档复制为VIP权益,开通VIP直接复制
