# Unity.Collections
A C# collections library providing data structures that can be used in jobs, and
optimized by Burst compiler.
### Package CI Summary
[](https://badges.cds.internal.unity3d.com/packages/com.unity.collections/build-info?branch=master) [](https://badges.cds.internal.unity3d.com/packages/com.unity.collections/dependencies-info?branch=master) [](https://badges.cds.internal.unity3d.com/packages/com.unity.collections/dependants-info)  
## Documentation
https://docs.unity3d.com/Packages/com.unity.collections@0.14/manual/index.html
## Data structures
The Unity.Collections package includes the following data structures:
Data structure | Description | Documentation
----------------------- | ----------- | -------------
`BitField32` | Fixed size 32-bit array of bits. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.BitField32.html)
`BitField64` | Fixed size 64-bit array of bits. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.BitField64.html)
`NativeBitArray` | Arbitrary sized array of bits. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.NativeBitArray.html)
`UnsafeBitArray` | Arbitrary sized array of bits, without any thread safety check features. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.LowLevel.Unsafe.UnsafeBitArray.html)
`NativeHashMap` | Unordered associative array, a collection of keys and values. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.NativeHashMap-2.html)
`UnsafeHashMap` | Unordered associative array, a collection of keys and values, without any thread safety check features. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.LowLevel.Unsafe.UnsafeHashMap-2.html)
`NativeHashSet` | Set of values. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.NativeHashSet-1.html)
`UnsafeHashSet` | Set of values, without any thread safety check features. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.LowLevel.Unsafe.UnsafeHashSet-1.html)
`NativeList` | An unmanaged, resizable list. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.NativeList-1.html)
`UnsafeList` | An unmanaged, resizable list, without any thread safety check features. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.LowLevel.Unsafe.UnsafeList-1.html)
`NativeMultiHashMap` | Unordered associative array, a collection of keys and values. This container can store multiple values for every key. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.NativeMultiHashMap-2.html)
`UnsafeMultiHashMap` | Unordered associative array, a collection of keys and values, without any thread safety check features. This container can store multiple values for every key. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.LowLevel.Unsafe.UnsafeMultiHashMap-2.html)
`NativeStream` | A deterministic data streaming supporting parallel reading and parallel writing. Allows you to write different types or arrays into a single stream. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.NativeStream.html)
`UnsafeStream` | A deterministic data streaming supporting parallel reading and parallel writings, without any thread safety check features. Allows you to write different types or arrays into a single stream. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.LowLevel.Unsafe.UnsafeStream.html)
`NativeReference` | An unmanaged, reference container. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.NativeReference-1.html)
`UnsafeAppendBuffer` | An unmanaged, untyped, buffer, without any thread safety check features. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.LowLevel.Unsafe.UnsafeAppendBuffer.html)
`UnsafeRingQueue` | Fixed-size circular buffer, without any thread safety check features. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.LowLevel.Unsafe.UnsafeRingQueue-1.html)
`UnsafeAtomicCounter32` | 32-bit atomic counter. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.LowLevel.Unsafe.UnsafeAtomicCounter32.html)
`UnsafeAtomicCounter64` | 64-bit atomic counter. | [Documentation](https://docs.unity3d.com/Packages/com.unity.collections@0.14/api/Unity.Collections.LowLevel.Unsafe.UnsafeAtomicCounter64.html)
[...](https://docs.unity3d.com/Packages/com.unity.collections@0.14/manual/index.html)
The items in this package build upon the [NativeArray<T0>](https://docs.unity3d.com/ScriptReference/Unity.Collections.NativeArray_1),
[NativeSlice<T0>](https://docs.unity3d.com/ScriptReference/Unity.Collections.NativeSlice_1),
and other members of the Unity.Collections namespace, which Unity includes in
the [core module](https://docs.unity3d.com/ScriptReference/UnityEngine.CoreModule).
## Notation
`Native*` container prefix signifies that containers have debug safety mechanisms
which will warn users when a container is used incorrectly in regard with thread-safety,
or memory management. `Unsafe*` containers do not provide those safety warnings, and
the user is fully responsible to guarantee that code will execute correctly. Almost all
`Native*` containers are implemented by using `Unsafe*` container of the same kind
internally. In the release build, since debug safety mechanism is disabled, there
should not be any significant performance difference between `Unsafe*` and `Native*`
containers. `Unsafe*` containers are in `Unity.Collections.LowLevel.Unsafe`
namespace, while `Native*` containers are in `Unity.Collections` namespace.
## Determinism
Populating containers from parallel jobs is never deterministic, except when
using `NativeStream` or `UnsafeStream`. If determinism is required, consider
sorting the container as a separate step or post-process it on a single thread.
## Known Issues
All containers allocated with `Allocator.Temp` on the same thread use a shared
`AtomicSafetyHandle` instance. This is problematic when using `NativeHashMap`,
`NativeMultiHashMap`, `NativeHashSet` and `NativeList` together in situations
where their secondary safety handle is used. This means that operations that
invalidate an enumerator for either of these collections (or the `NativeArray`
returned by `NativeList.AsArray`) will also invalidate all other previously
acquired enumerators. For example, this will throw when safety checks are enabled:
```
var list = new NativeList<int>(Allocator.Temp);
list.Add(1);
// This array uses the secondary safety handle of the list, which is
// shared between all Allocator.Temp allocations.
var array = list.AsArray();
var list2 = new NativeHashSet<int>(Allocator.Temp);
// This invalidates the secondary safety handle, which is also used
// by the list above.
list2.TryAdd(1);
// This th
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
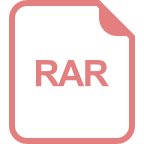
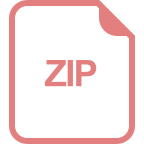
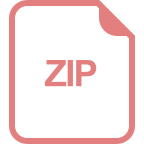
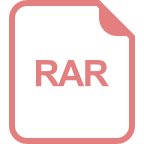
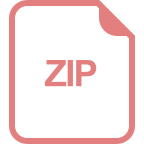
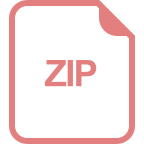
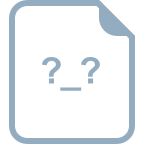
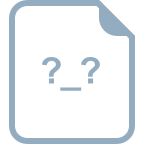
收起资源包目录

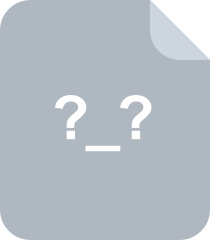
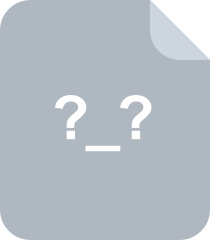
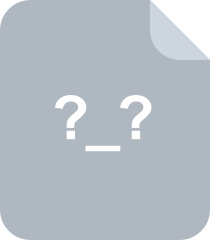
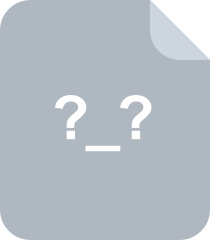
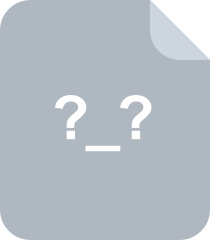
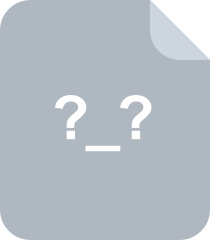
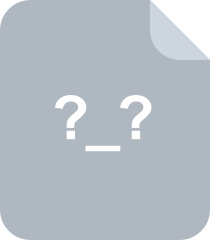
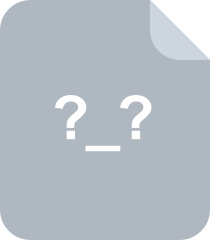
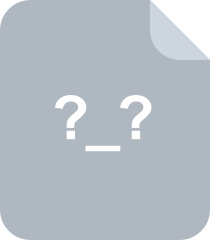
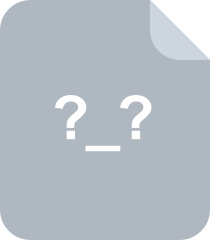
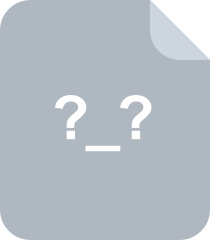
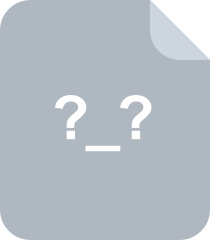
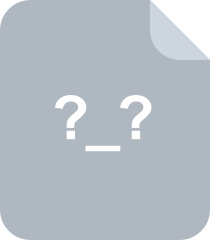
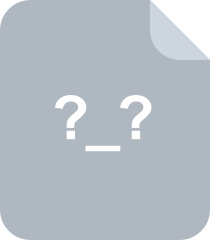
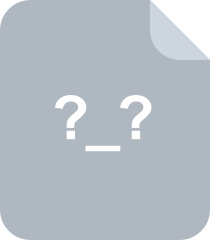
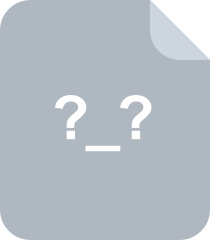
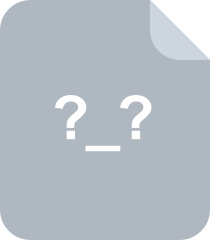
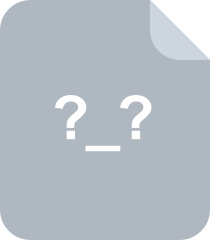
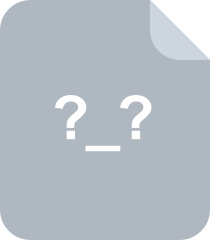
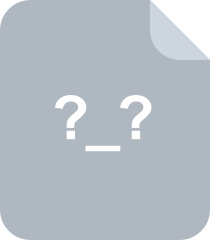
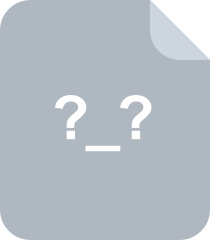
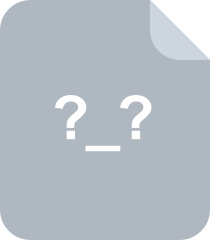
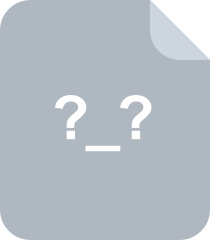
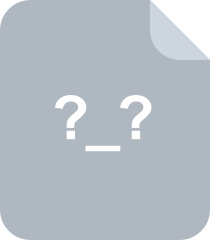
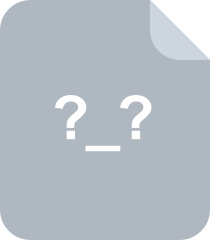
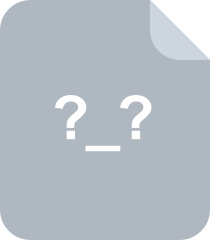
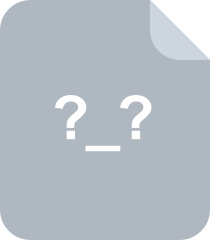
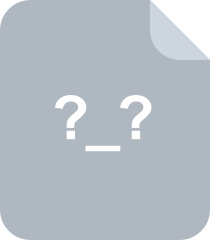
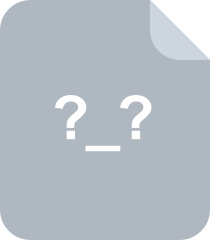
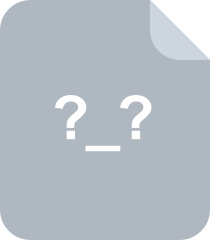
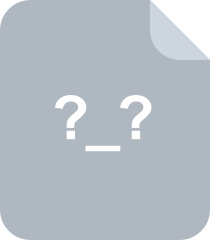
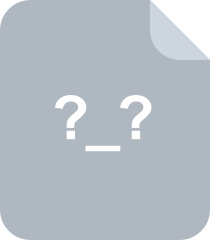
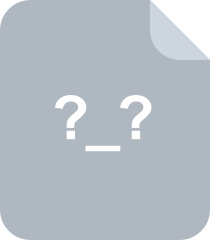
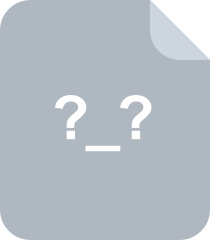
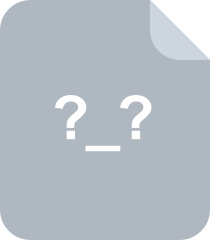
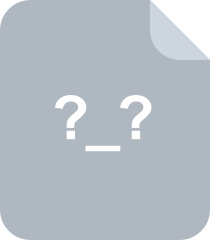
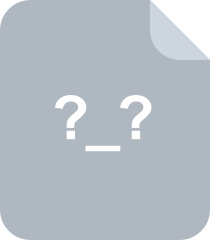
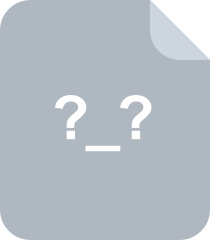
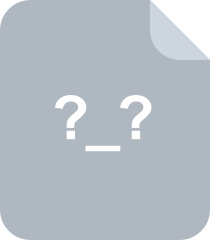
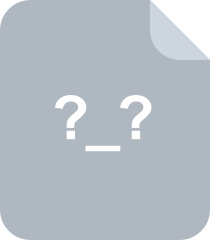
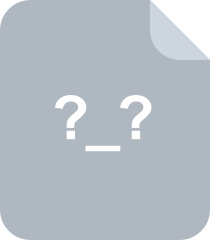
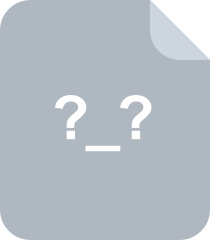
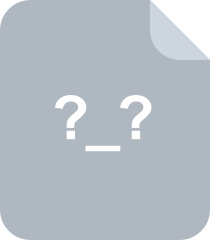
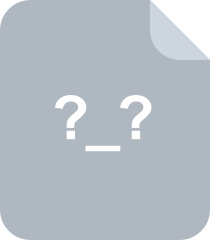
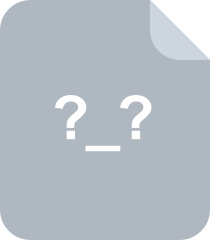
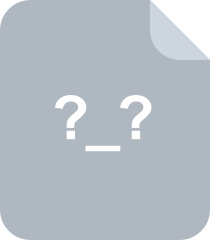
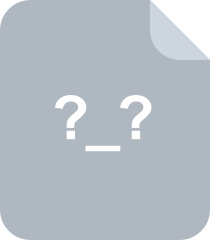
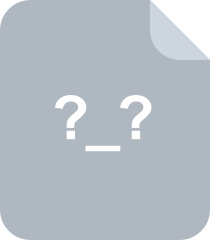
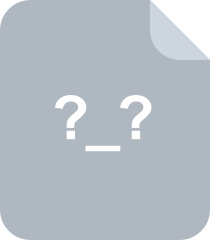
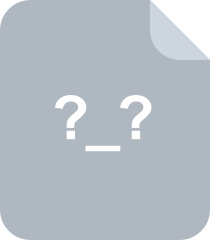
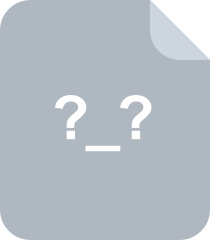
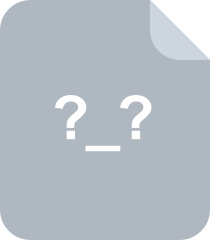
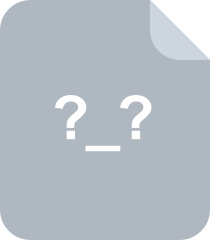
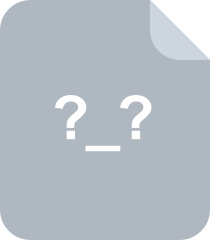
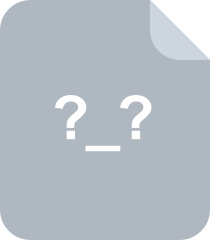
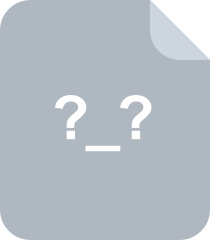
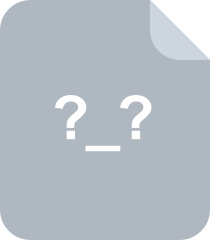
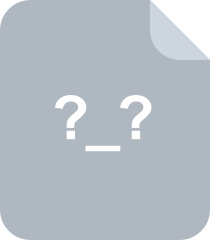
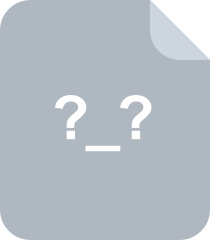
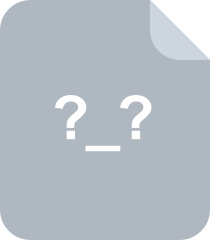
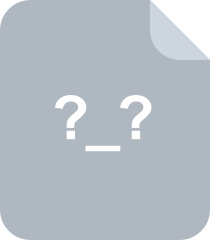
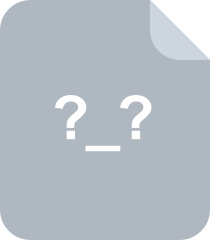
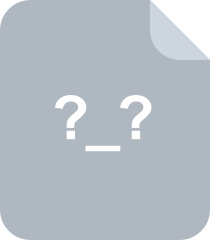
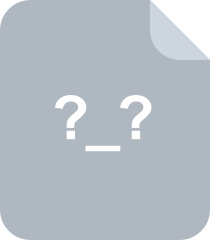
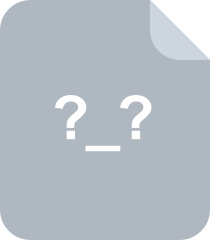
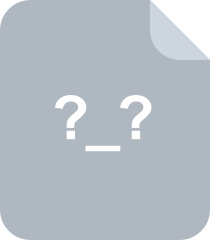
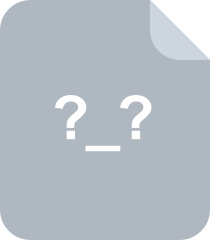
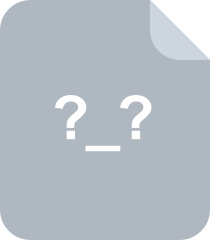
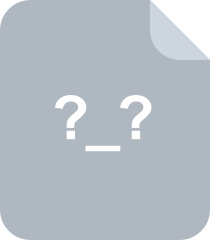
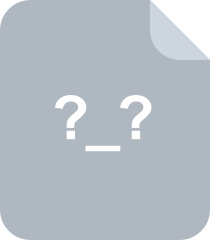
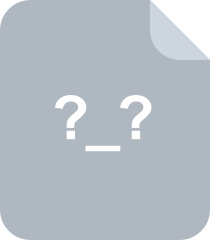
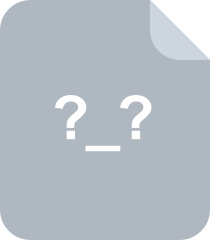
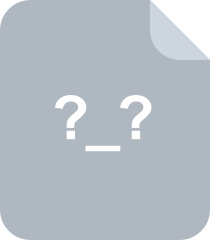
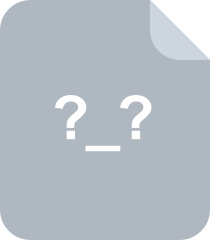
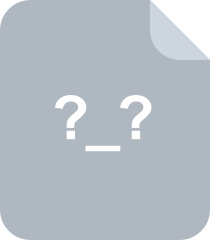
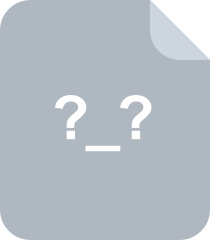
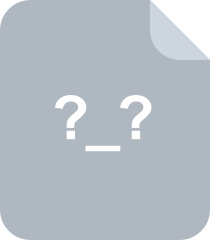
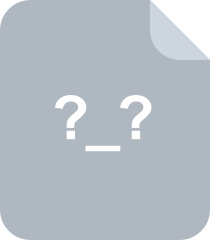
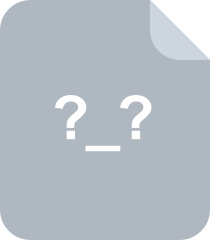
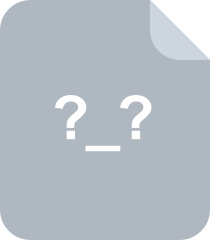
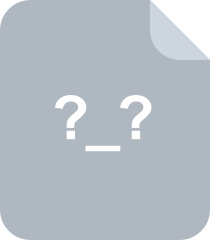
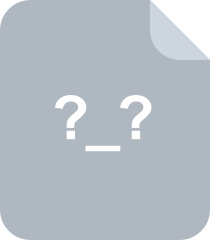
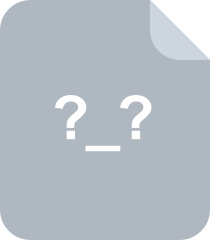
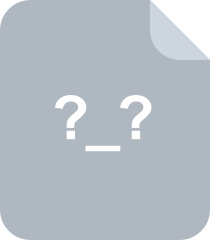
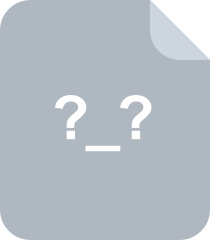
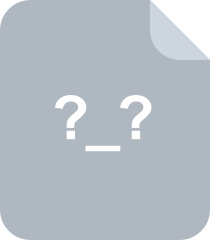
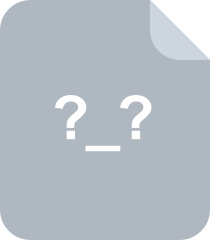
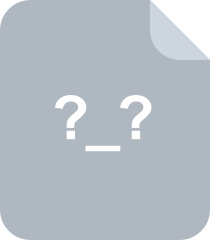
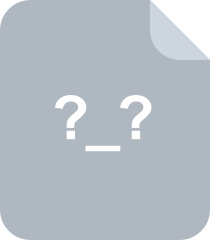
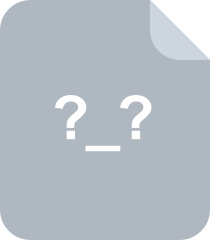
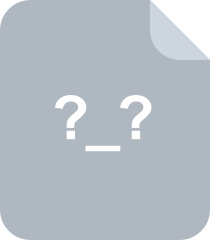
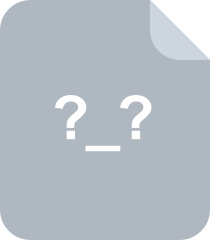
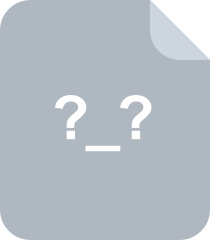
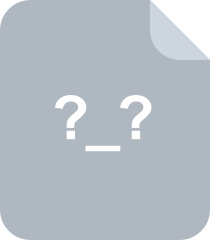
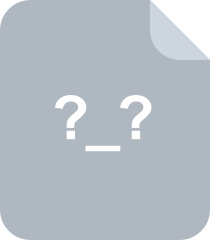
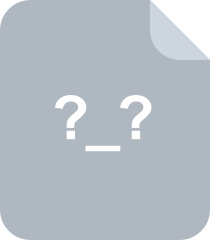
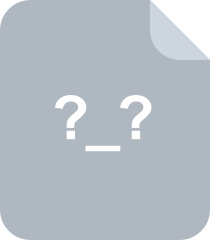
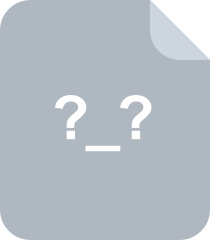
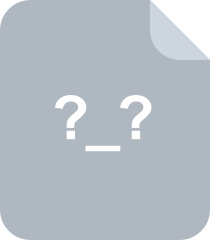
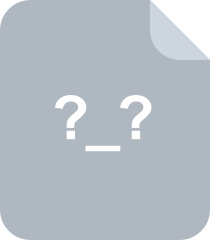
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
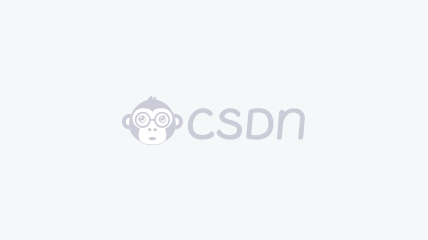
- 明儿去打球2023-07-27感谢这份引导文件,它为我提供了一个很好的起点,让我对Unity的开发有了更深入的了解。
- 我只匆匆而过2023-07-27引导文件中的案例分析非常实用,能够帮助我更好地理解并运用Unity的各种功能。
- 我有多作怪2023-07-27在这份引导文件中,我学到了很多实用的技巧和技术,感觉自己的游戏制作能力大幅提升。
- 王元祺2023-07-27这份Unity新手引导文件详细介绍了Unity引擎的基础知识,非常适合初学者入门。
- 永远的122023-07-27文件内容清晰明了,让我快速掌握了Unity的基本操作和开发流程。

国家一级摸鱼选手
- 粉丝: 107
- 资源: 14
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

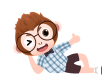
最新资源
- Python大作业-爬虫(高分大作业)
- Python 图片压缩工具
- qt4.8.6资源,用户qt安装,编译与学习
- (176465412)电气设计视频教程-Eplan.P8
- Python大作业爬虫项目并且用web展示爬虫的内容(高分项目)源码+说明
- Python项目-实例-27 生成词云图.zip
- (176566822)数据库课程设计ssm027学校运动会信息管理系统+jsp.sql
- C# WPF-激光焊接机配套软件源码及文档(带视觉需halcon)
- (177333248)c++实现的仿QQ贪吃蛇大作战多人联机游戏.zip
- Python大作业-爬虫(高分大作业).zip
- (177487602)c++ 家谱管理系统.zip
- IMG-8274.GIF
- (177938850)115-基于51单片机和PROTEUS的基于C51单片机的智能交通灯设计.zip
- 基于微信小程序的宏华水利小程序.zip
- (OC)数据加载SVG图片
- linux3.8.6内核资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


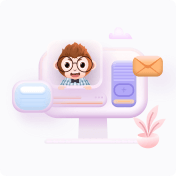
安全验证
文档复制为VIP权益,开通VIP直接复制
