# Gin Web Framework
<img align="right" width="159px" src="https://raw.githubusercontent.com/gin-gonic/logo/master/color.png">
[](https://travis-ci.org/gin-gonic/gin)
[](https://codecov.io/gh/gin-gonic/gin)
[](https://goreportcard.com/report/github.com/gin-gonic/gin)
[](https://godoc.org/github.com/gin-gonic/gin)
[](https://gitter.im/gin-gonic/gin?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
[](https://sourcegraph.com/github.com/gin-gonic/gin?badge)
[](https://www.codetriage.com/gin-gonic/gin)
[](https://github.com/gin-gonic/gin/releases)
Gin is a web framework written in Go (Golang). It features a martini-like API with much better performance, up to 40 times faster thanks to [httprouter](https://github.com/julienschmidt/httprouter). If you need performance and good productivity, you will love Gin.

## Contents
- [Installation](#installation)
- [Prerequisite](#prerequisite)
- [Quick start](#quick-start)
- [Benchmarks](#benchmarks)
- [Gin v1.stable](#gin-v1-stable)
- [Build with jsoniter](#build-with-jsoniter)
- [API Examples](#api-examples)
- [Using GET,POST,PUT,PATCH,DELETE and OPTIONS](#using-get-post-put-patch-delete-and-options)
- [Parameters in path](#parameters-in-path)
- [Querystring parameters](#querystring-parameters)
- [Multipart/Urlencoded Form](#multiparturlencoded-form)
- [Another example: query + post form](#another-example-query--post-form)
- [Map as querystring or postform parameters](#map-as-querystring-or-postform-parameters)
- [Upload files](#upload-files)
- [Grouping routes](#grouping-routes)
- [Blank Gin without middleware by default](#blank-gin-without-middleware-by-default)
- [Using middleware](#using-middleware)
- [How to write log file](#how-to-write-log-file)
- [Custom Log Format](#custom-log-format)
- [Model binding and validation](#model-binding-and-validation)
- [Custom Validators](#custom-validators)
- [Only Bind Query String](#only-bind-query-string)
- [Bind Query String or Post Data](#bind-query-string-or-post-data)
- [Bind Uri](#bind-uri)
- [Bind HTML checkboxes](#bind-html-checkboxes)
- [Multipart/Urlencoded binding](#multiparturlencoded-binding)
- [XML, JSON, YAML and ProtoBuf rendering](#xml-json-yaml-and-protobuf-rendering)
- [JSONP rendering](#jsonp)
- [Serving static files](#serving-static-files)
- [Serving data from reader](#serving-data-from-reader)
- [HTML rendering](#html-rendering)
- [Multitemplate](#multitemplate)
- [Redirects](#redirects)
- [Custom Middleware](#custom-middleware)
- [Using BasicAuth() middleware](#using-basicauth-middleware)
- [Goroutines inside a middleware](#goroutines-inside-a-middleware)
- [Custom HTTP configuration](#custom-http-configuration)
- [Support Let's Encrypt](#support-lets-encrypt)
- [Run multiple service using Gin](#run-multiple-service-using-gin)
- [Graceful restart or stop](#graceful-restart-or-stop)
- [Build a single binary with templates](#build-a-single-binary-with-templates)
- [Bind form-data request with custom struct](#bind-form-data-request-with-custom-struct)
- [Try to bind body into different structs](#try-to-bind-body-into-different-structs)
- [http2 server push](#http2-server-push)
- [Define format for the log of routes](#define-format-for-the-log-of-routes)
- [Set and get a cookie](#set-and-get-a-cookie)
- [Testing](#testing)
- [Users](#users)
## Installation
To install Gin package, you need to install Go and set your Go workspace first.
1. Download and install it:
```sh
$ go get -u github.com/gin-gonic/gin
```
2. Import it in your code:
```go
import "github.com/gin-gonic/gin"
```
3. (Optional) Import `net/http`. This is required for example if using constants such as `http.StatusOK`.
```go
import "net/http"
```
### Use a vendor tool like [Govendor](https://github.com/kardianos/govendor)
1. `go get` govendor
```sh
$ go get github.com/kardianos/govendor
```
2. Create your project folder and `cd` inside
```sh
$ mkdir -p $GOPATH/src/github.com/myusername/project && cd "$_"
```
3. Vendor init your project and add gin
```sh
$ govendor init
$ govendor fetch github.com/gin-gonic/gin@v1.3
```
4. Copy a starting template inside your project
```sh
$ curl https://raw.githubusercontent.com/gin-gonic/gin/master/examples/basic/main.go > main.go
```
5. Run your project
```sh
$ go run main.go
```
## Prerequisite
Now Gin requires Go 1.6 or later and Go 1.7 will be required soon.
## Quick start
```sh
# assume the following codes in example.go file
$ cat example.go
```
```go
package main
import "github.com/gin-gonic/gin"
func main() {
r := gin.Default()
r.GET("/ping", func(c *gin.Context) {
c.JSON(200, gin.H{
"message": "pong",
})
})
r.Run() // listen and serve on 0.0.0.0:8080
}
```
```
# run example.go and visit 0.0.0.0:8080/ping on browser
$ go run example.go
```
## Benchmarks
Gin uses a custom version of [HttpRouter](https://github.com/julienschmidt/httprouter)
[See all benchmarks](/BENCHMARKS.md)
Benchmark name | (1) | (2) | (3) | (4)
--------------------------------------------|-----------:|------------:|-----------:|---------:
**BenchmarkGin_GithubAll** | **30000** | **48375** | **0** | **0**
BenchmarkAce_GithubAll | 10000 | 134059 | 13792 | 167
BenchmarkBear_GithubAll | 5000 | 534445 | 86448 | 943
BenchmarkBeego_GithubAll | 3000 | 592444 | 74705 | 812
BenchmarkBone_GithubAll | 200 | 6957308 | 698784 | 8453
BenchmarkDenco_GithubAll | 10000 | 158819 | 20224 | 167
BenchmarkEcho_GithubAll | 10000 | 154700 | 6496 | 203
BenchmarkGocraftWeb_GithubAll | 3000 | 570806 | 131656 | 1686
BenchmarkGoji_GithubAll | 2000 | 818034 | 56112 | 334
BenchmarkGojiv2_GithubAll | 2000 | 1213973 | 274768 | 3712
BenchmarkGoJsonRest_GithubAll | 2000 | 785796 | 134371 | 2737
BenchmarkGoRestful_GithubAll | 300 | 5238188 | 689672 | 4519
BenchmarkGorillaMux_GithubAll | 100 | 10257726 | 211840 | 2272
BenchmarkHttpRouter_GithubAll | 20000 | 105414 | 13792 | 167
BenchmarkHttpTreeMux_GithubAll | 10000 | 319934 | 65856 | 671
BenchmarkKocha_GithubAll | 10000 | 209442 | 23304 | 843
BenchmarkLARS_GithubAll | 20000 | 62565 | 0 | 0
BenchmarkMacaron_GithubAll | 2000 | 1161270 | 204194 | 2000
BenchmarkMartini_GithubAll | 200 | 9991713 | 226549 | 2325
BenchmarkPat_GithubAll | 200 | 5590793 | 1499568 | 27435
BenchmarkPossum_GithubAll | 10000 | 319768 | 84448 | 609
BenchmarkR2router_GithubAll | 10000 | 305134 | 77328 | 979
BenchmarkRivet_Gi
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
嵌入式优质项目,资源经过严格测试可直接运行成功且功能正常的情况才上传,可轻松copy复刻,拿到资料包后可轻松复现出一样的项目。 本人单片机开发经验充足,深耕嵌入式领域,有任何使用问题欢迎随时与我联系,我会及时为你解惑,提供帮助。 【资源内容】:包含完整源码+工程文件+说明,项目具体内容可查看下方的资源详情。 【附带帮助】: 若还需要嵌入式物联网单片机相关领域开发工具、学习资料等,我会提供帮助,提供资料,鼓励学习进步。 【本人专注嵌入式领域】: 有任何使用问题欢迎随时与我联系,我会及时解答,第一时间为你提供帮助,CSDN博客端可私信,为你解惑,欢迎交流。 【建议小白】: 在所有嵌入式开发中硬件部分若不会画PCB/电路,可选择根据引脚定义将其代替为面包板+杜邦线+外设模块的方式,只需轻松简单连线,下载源码烧录进去便可轻松复刻出一样的项目 【适合场景】: 相关项目设计中,皆可应用在项目开发、毕业设计、课程设计、期末/期中/大作业、工程实训、大创等学科竞赛比赛、初期项目立项、学习/练手等方面中 可借鉴此优质项目实现复刻,也可以基于此项目进行扩展来开发出更多功能
资源推荐
资源详情
资源评论
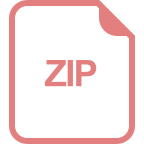
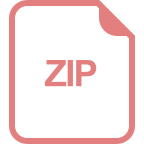
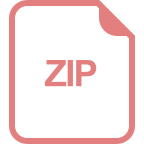
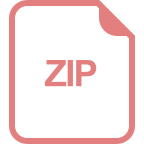
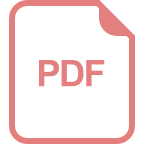
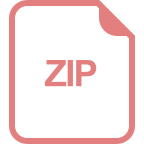
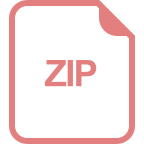
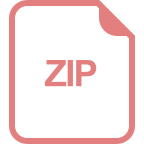
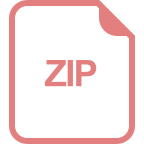
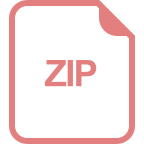
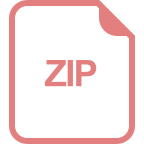
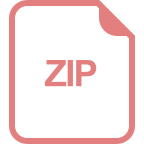
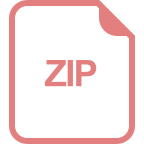
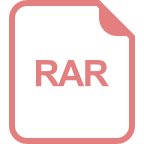
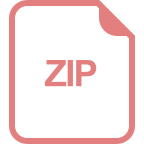
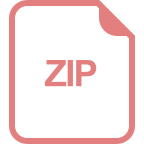
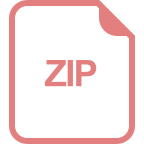
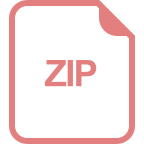
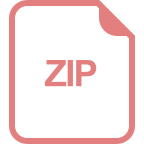
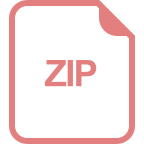
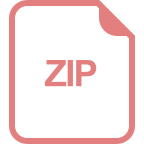
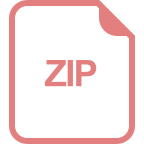
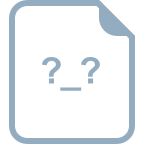
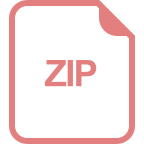
收起资源包目录

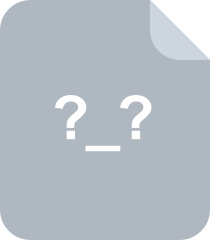
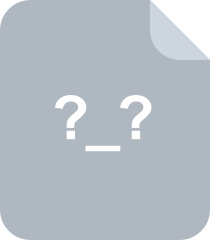
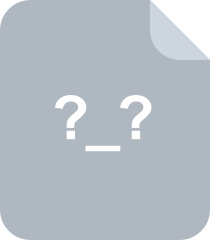
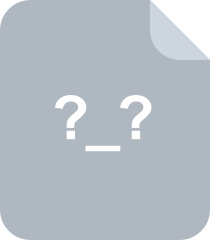
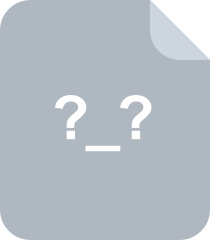
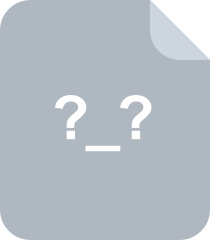
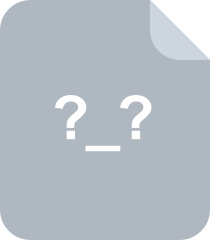
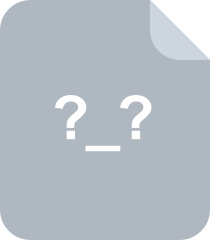
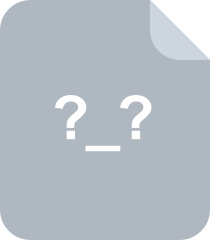
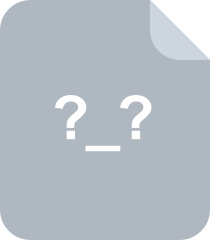
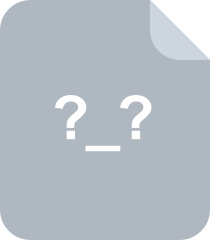
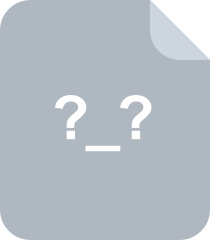
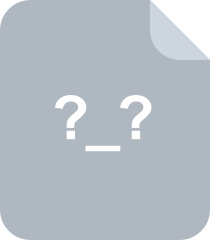
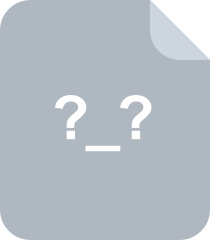
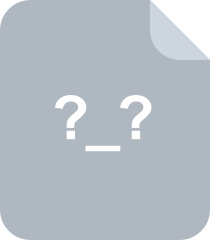
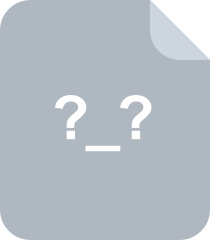
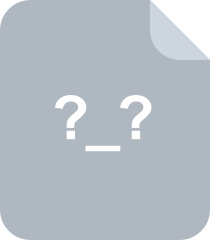
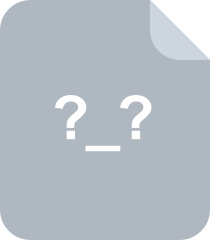
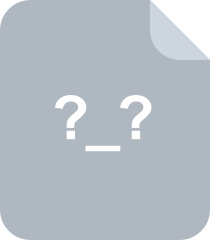
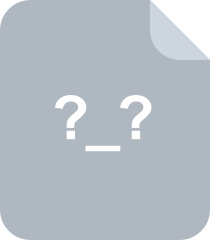
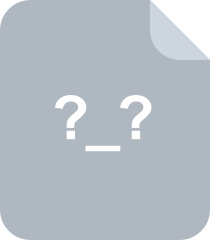
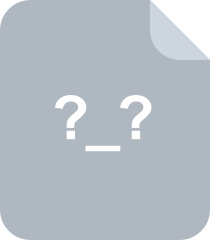
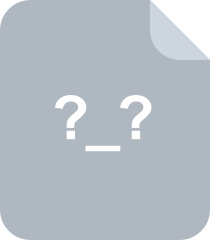
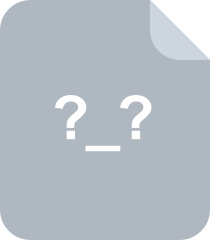
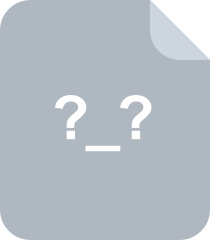
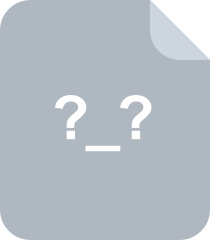
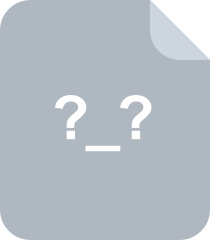
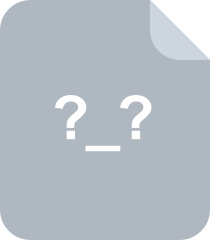
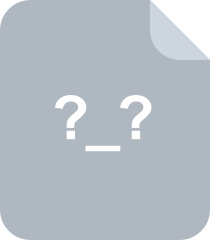
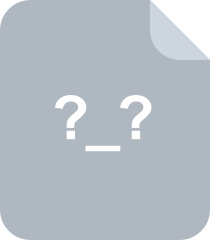
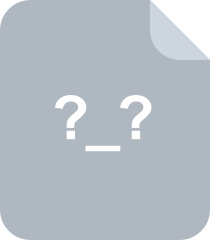
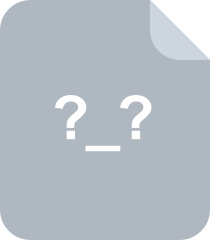
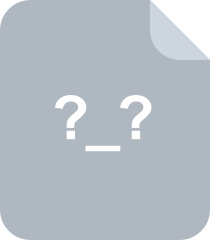
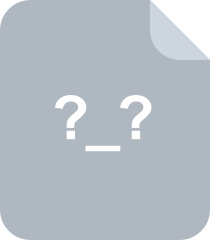
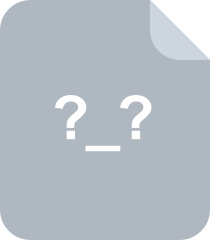
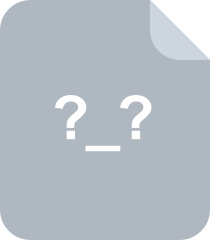
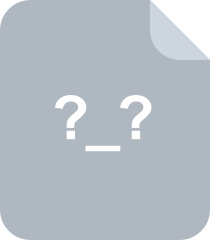
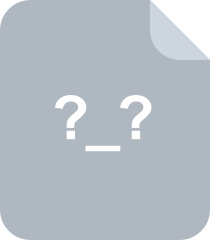
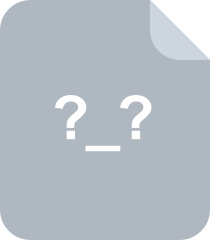
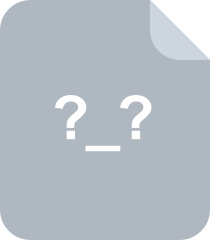
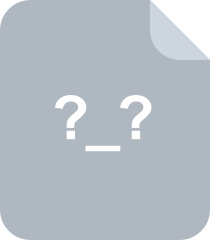
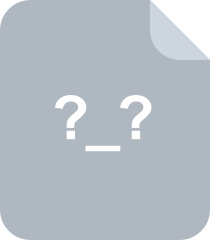
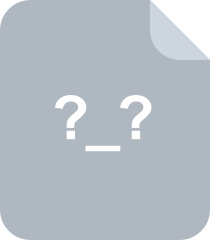
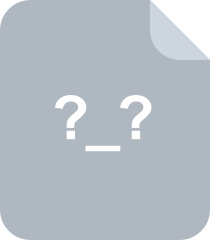
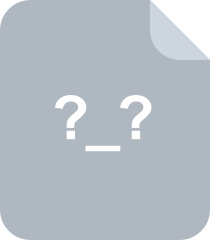
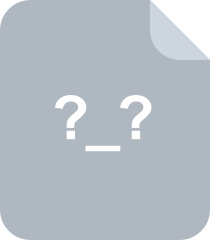
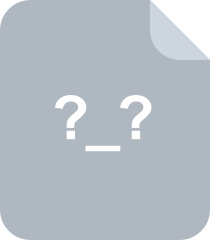
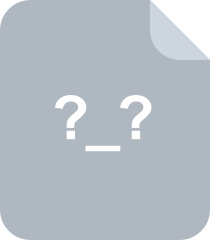
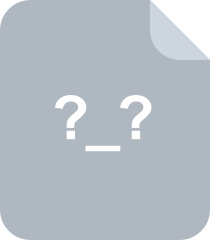
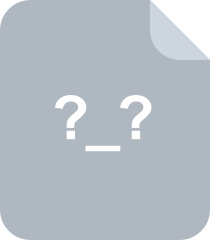
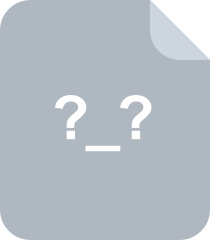
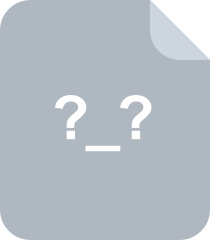
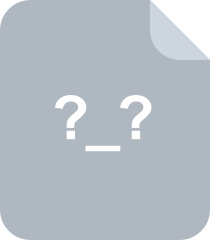
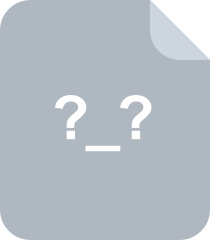
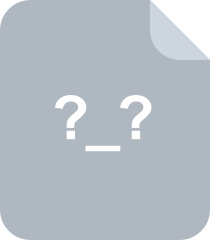
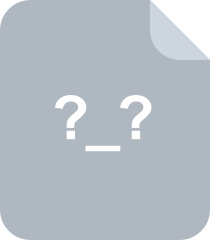
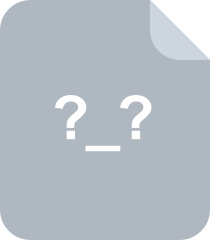
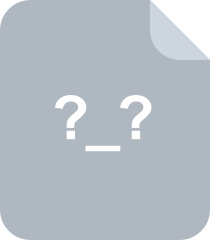
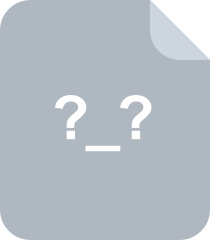
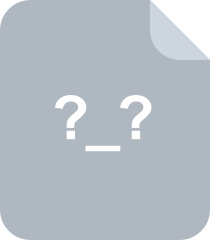
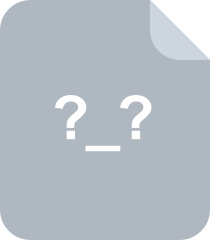
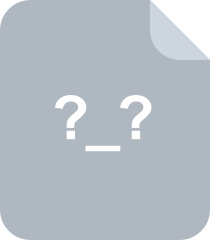
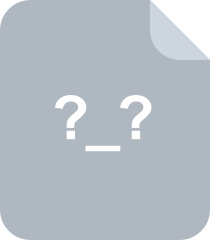
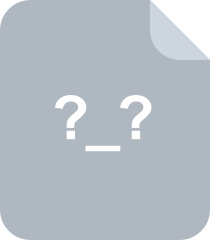
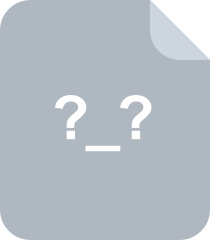
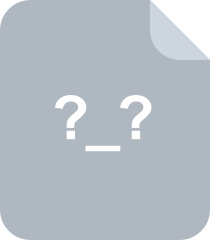
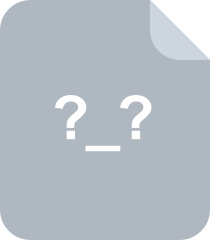
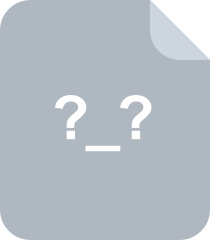
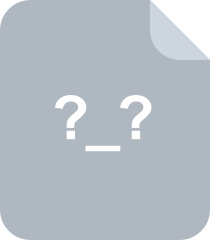
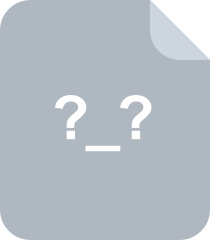
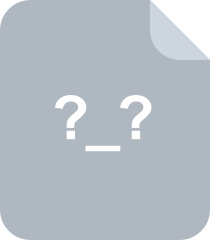
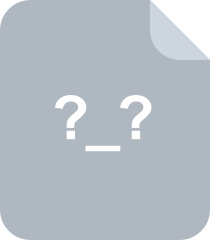
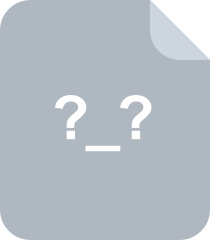
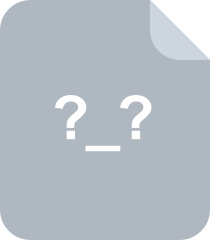
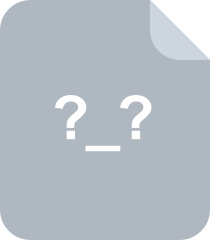
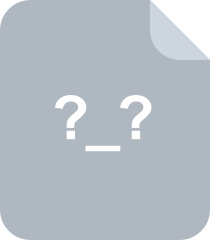
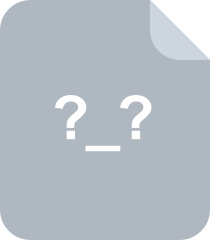
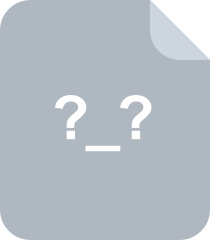
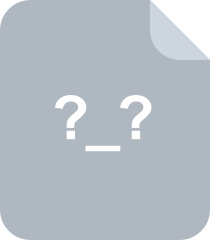
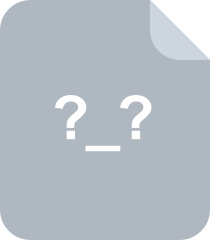
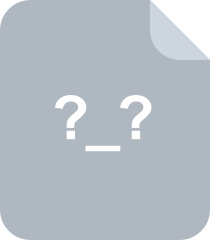
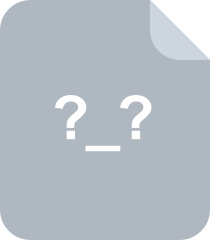
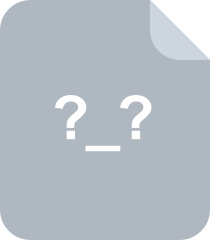
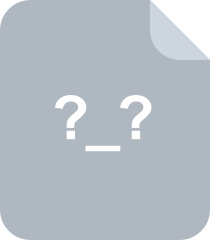
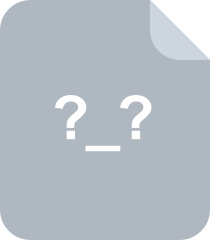
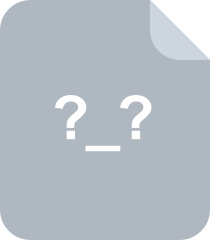
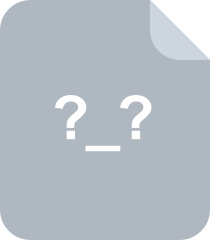
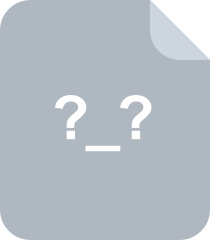
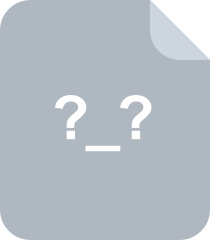
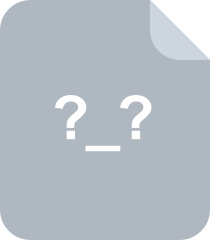
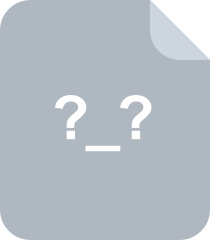
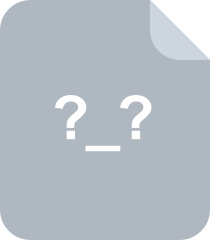
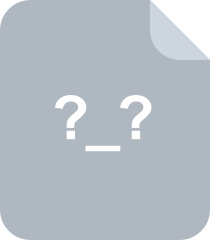
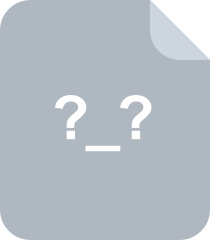
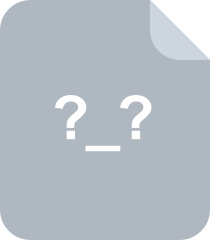
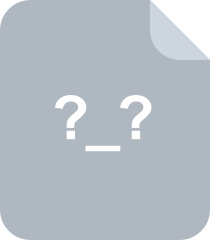
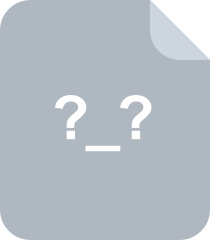
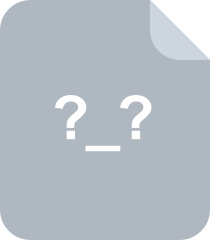
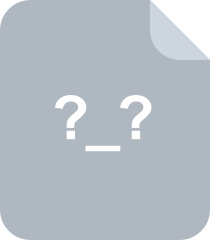
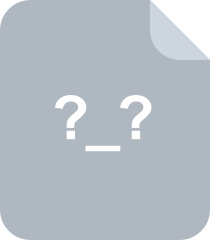
共 524 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
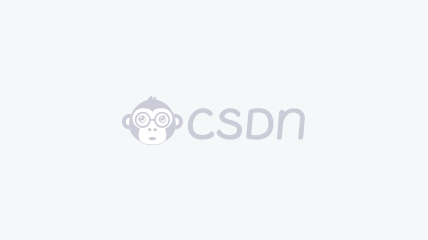

阿齐Archie
- 粉丝: 3w+
- 资源: 2474

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

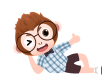
最新资源
- 硅酸钠块行业分析:2023年全球市场规模大约为349百万美元.docx
- 光学扩散膜行业分析:2023年全球市场规模大约为352百万美元.docx
- 合成生物学技术行业分析:全球收入达到1279.6百万美元.docx
- 海上能源无人潜水器市场分析:2023年全球市场规模为854百万美元.docx
- 呼气分子诊断行业分析:2023年全球市场规模大约为234百万美元.docx
- 环氧豆油丙烯酸酯行业分析:2023年全球市场规模大约为871百万美元.docx
- 滑雪头盔式耳机行业分析:2023年全球市场规模大约为51.5百万美元.docx
- 幻想(虚拟)体育行业分析:北美和欧洲占全球约70%的市场份额.docx
- 会话营销软件行业分析:全球收入约为564.9百万美元.docx
- 火灾警报设备行业分析:全球市场收入约为19130百万美元.docx
- 基础unity,控制物体的简单移动,值得学习一下
- 活动行业分析:全球收入约为342100百万美元.docx
- 机械键盘行业分析:2023年全球市场规模大约为1245百万美元.docx
- 即时物流行业分析:2023年全球市场规模大约为23770百万美元.docx
- 奖励管理软件行业研究:全球收入约为692.5百万美元.docx
- 洁净室环境在线监测软件行业分析:北美占有约25%的全球市场份额.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


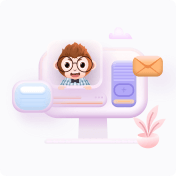
安全验证
文档复制为VIP权益,开通VIP直接复制
